# CTkListbox
This is a **listbox widget** for customtkinter, works just like the tkinter listbox.
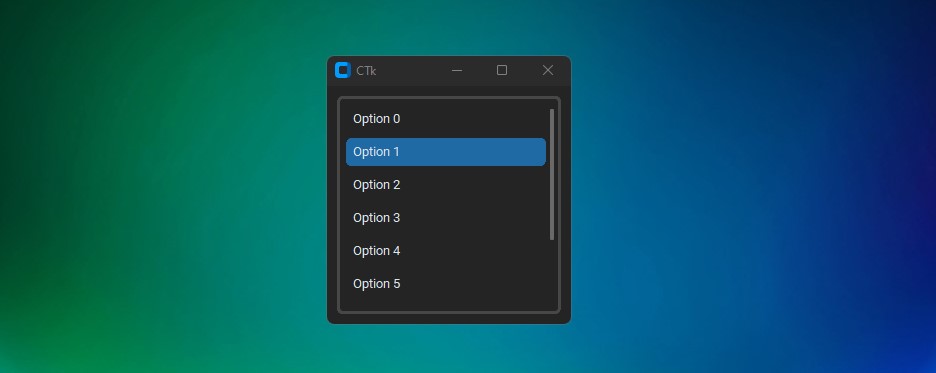
## Installation
```
pip install CTkListbox
```
### [<img alt="GitHub repo size" src="https://img.shields.io/github/repo-size/Akascape/CTkListbox?&color=white&label=Download%20Source%20Code&logo=Python&logoColor=yellow&style=for-the-badge" width="400">](https://github.com/Akascape/CTkListbox/archive/refs/heads/main.zip)
## Usage
```python
import customtkinter
from CTkListbox import *
def show_value(selected_option):
print(selected_option)
root = customtkinter.CTk()
listbox = CTkListbox(root, command=show_value)
listbox.pack(fill="both", expand=True, padx=10, pady=10)
listbox.insert(0, "Option 0")
listbox.insert(1, "Option 1")
listbox.insert(2, "Option 2")
listbox.insert(3, "Option 3")
listbox.insert(4, "Option 4")
listbox.insert(5, "Option 5")
listbox.insert(6, "Option 6")
listbox.insert(7, "Option 7")
listbox.insert("END", "Option 8")
root.mainloop()
```
## Arguments
| Parameter | Description |
|-----------| ------------|
| **master** | parent widget |
| width | **optional**, set width of the listbox |
| height | **optional**, set height of the listbox |
| fg_color | foreground color of the listbox |
| border_color | border color of the listbox frame |
| border_width | width of the border frame |
| text_color | set the color of the option text |
| hover_color | set hover color of the options |
| button_color | set color of unselected buttons |
| highlight_color | set the selected color of the option |
| font | set font of the option text |
| command | calls a command when a option is selected |
| multiple_selection | select multiple options in the listbox, `default=False`|
| listvariable | use a tkinter variable to change the listbox content |
| *other_parameters | _all other parameters of ctk_scrollable frame can be passed_ |
## Methods
- **.insert(index, option)**
add new option to the listbox
- **.get()**
get the selected option(s)
- **.delete(index)**
delete any option from the listbox. `.delete("all")` deletes all options
- **.size()**
get the size of the listbox
- **.activate(index)**
activate any option
- **.deactivate(index)**
deactivate any option
- **.curselection()**
returns indexes of selected options
- **.configure()**
change some parameters for the listbox.
- **.move_up(index)/.move_down(index)**
Reorder options in the listbox
### Thanks for visiting! Hope it will help :)
Raw data
{
"_id": null,
"home_page": "https://github.com/Akascape/CTkListbox",
"name": "CTkListbox",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "customtkinter,tkinter,listbox-widget,listbox,modern-listbox,option menu,list-box,ctklistbox",
"author": "Akash Bora",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/9e/1e/38f76ca0a1a68cae702b4cbc7534cbbde22853e5d4e950e138840f030902/CTkListbox-1.3.tar.gz",
"platform": null,
"description": "# CTkListbox\r\nThis is a **listbox widget** for customtkinter, works just like the tkinter listbox.\r\n\r\n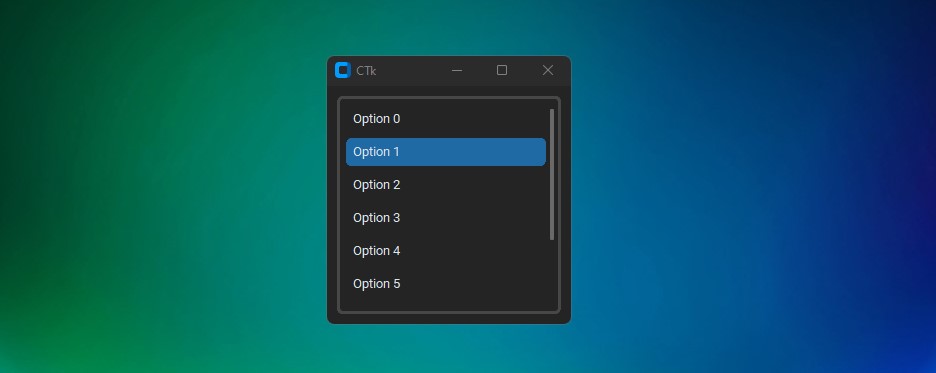\r\n\r\n## Installation\r\n```\r\npip install CTkListbox\r\n```\r\n### [<img alt=\"GitHub repo size\" src=\"https://img.shields.io/github/repo-size/Akascape/CTkListbox?&color=white&label=Download%20Source%20Code&logo=Python&logoColor=yellow&style=for-the-badge\" width=\"400\">](https://github.com/Akascape/CTkListbox/archive/refs/heads/main.zip)\r\n\r\n## Usage\r\n```python\r\nimport customtkinter\r\nfrom CTkListbox import *\r\n\r\ndef show_value(selected_option):\r\n print(selected_option)\r\n \r\nroot = customtkinter.CTk()\r\n\r\nlistbox = CTkListbox(root, command=show_value)\r\nlistbox.pack(fill=\"both\", expand=True, padx=10, pady=10)\r\n\r\nlistbox.insert(0, \"Option 0\")\r\nlistbox.insert(1, \"Option 1\")\r\nlistbox.insert(2, \"Option 2\")\r\nlistbox.insert(3, \"Option 3\")\r\nlistbox.insert(4, \"Option 4\")\r\nlistbox.insert(5, \"Option 5\")\r\nlistbox.insert(6, \"Option 6\")\r\nlistbox.insert(7, \"Option 7\")\r\nlistbox.insert(\"END\", \"Option 8\")\r\n\r\nroot.mainloop()\r\n```\r\n## Arguments\r\n| Parameter | Description |\r\n|-----------| ------------|\r\n| **master** | parent widget |\r\n| width | **optional**, set width of the listbox |\r\n| height | **optional**, set height of the listbox |\r\n| fg_color | foreground color of the listbox |\r\n| border_color | border color of the listbox frame |\r\n| border_width | width of the border frame |\r\n| text_color | set the color of the option text |\r\n| hover_color | set hover color of the options |\r\n| button_color | set color of unselected buttons |\r\n| highlight_color | set the selected color of the option |\r\n| font | set font of the option text |\r\n| command | calls a command when a option is selected |\r\n| multiple_selection | select multiple options in the listbox, `default=False`|\r\n| listvariable | use a tkinter variable to change the listbox content |\r\n| *other_parameters | _all other parameters of ctk_scrollable frame can be passed_ |\r\n\r\n## Methods\r\n- **.insert(index, option)**\r\n add new option to the listbox\r\n- **.get()**\r\n get the selected option(s)\r\n- **.delete(index)**\r\n delete any option from the listbox. `.delete(\"all\")` deletes all options\r\n- **.size()**\r\n get the size of the listbox\r\n- **.activate(index)**\r\n activate any option\r\n- **.deactivate(index)**\r\n deactivate any option\r\n- **.curselection()**\r\n returns indexes of selected options\r\n- **.configure()**\r\n change some parameters for the listbox.\r\n- **.move_up(index)/.move_down(index)**\r\n Reorder options in the listbox\r\n \r\n### Thanks for visiting! Hope it will help :)\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Customtkinter Listbox widget",
"version": "1.3",
"project_urls": {
"Homepage": "https://github.com/Akascape/CTkListbox"
},
"split_keywords": [
"customtkinter",
"tkinter",
"listbox-widget",
"listbox",
"modern-listbox",
"option menu",
"list-box",
"ctklistbox"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "41f307d19c6dc99aacacefbef89a957f7df7009268ed2676290fe4ded5d14c77",
"md5": "7d1005bee470e51559e0af1e1ab2813d",
"sha256": "3a655437dd7ce4c2d0d87e8ee45d3f4bae34a3a164e53795c694bf4205284975"
},
"downloads": -1,
"filename": "CTkListbox-1.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "7d1005bee470e51559e0af1e1ab2813d",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 6544,
"upload_time": "2024-02-12T11:37:38",
"upload_time_iso_8601": "2024-02-12T11:37:38.887719Z",
"url": "https://files.pythonhosted.org/packages/41/f3/07d19c6dc99aacacefbef89a957f7df7009268ed2676290fe4ded5d14c77/CTkListbox-1.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9e1e38f76ca0a1a68cae702b4cbc7534cbbde22853e5d4e950e138840f030902",
"md5": "97948de56fda22a408c3bdc8c625905f",
"sha256": "3fa850d2b573e3ebb8cb813355e5f8c93a133eb2189ff51a8afcd1e3b3dbf7d9"
},
"downloads": -1,
"filename": "CTkListbox-1.3.tar.gz",
"has_sig": false,
"md5_digest": "97948de56fda22a408c3bdc8c625905f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 6234,
"upload_time": "2024-02-12T11:37:41",
"upload_time_iso_8601": "2024-02-12T11:37:41.694611Z",
"url": "https://files.pythonhosted.org/packages/9e/1e/38f76ca0a1a68cae702b4cbc7534cbbde22853e5d4e950e138840f030902/CTkListbox-1.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-02-12 11:37:41",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Akascape",
"github_project": "CTkListbox",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "ctklistbox"
}