# QtAwesome
[](./LICENSE)
[](https://pypi.org/project/qtawesome/)
[](https://www.anaconda.com/download/)
[](https://www.anaconda.com/download/)
[](#backers)
[](https://gitter.im/spyder-ide/public)<br>
[](https://github.com/spyder-ide/qtawesome)
[](https://github.com/spyder-ide/qtawesome/actions)
[](https://github.com/spyder-ide/qtawesome/actions)
[](https://github.com/spyder-ide/qtawesome/actions)
[](https://qtawesome.readthedocs.io/en/latest/?badge=latest)
[](https://codecov.io/gh/spyder-ide/qtawesome)
*Copyright © 2015- Spyder Project Contributors*
## Description
QtAwesome enables iconic fonts such as Font Awesome and Elusive Icons
in PyQt and PySide applications.
It started as a Python port of the [QtAwesome](
https://github.com/Gamecreature/qtawesome)
C++ library by Rick Blommers.
## Installation
Using `conda`:
```
conda install qtawesome
```
or using `pip` (only if you don't have conda installed):
```
pip install qtawesome
```
## Usage
### Supported Fonts
QtAwesome identifies icons by their **prefix** and their **icon name**, separated by a *period* (`.`) character.
The following prefixes are currently available to use:
- [**FontAwesome**](https://fontawesome.com):
- FA 5.15.4 features 1,608 free icons in different styles:
- `fa5` prefix has [151 icons in the "**regular**" style.](https://fontawesome.com/v5/search?o=r&m=free&s=regular)
- `fa5s` prefix has [1001 icons in the "**solid**" style.](https://fontawesome.com/v5/search?o=r&m=free&s=solid)
- `fa5b` prefix has [456 icons of various **brands**.](https://fontawesome.com/v5/search?o=r&m=free&f=brands)
- `fa` is the legacy [FA 4.7 version with its 675 icons](https://fontawesome.com/v4.7.0/icons/) but **all** of them (*and more!*) are part of FA 5.x so you should probably use the newer version above.
- `ei` prefix holds [**Elusive Icons** 2.0 with its 304 icons](http://elusiveicons.com/icons/).
- [**Material Design Icons**](https://pictogrammers.com/library/mdi/)
- `mdi6` prefix holds [**Material Design Icons** 6.9.96 with its 6997 icons.](https://cdn.materialdesignicons.com/6.9.96/)
- `mdi` prefix holds [**Material Design Icons** 5.9.55 with its 5955 icons.](https://cdn.materialdesignicons.com/5.9.55/)
- `ph` prefix holds [**Phosphor** 1.3.0 with its 4470 icons (894 icons * 5 weights: Thin, Light, Regular, Bold and Fill).](https://github.com/phosphor-icons/phosphor-icons)
- `ri` prefix holds [**Remix Icon** 2.5.0 with its 2271 icons.](https://github.com/Remix-Design/RemixIcon)
- `msc` prefix holds Microsoft's [**Codicons** 0.0.35 with its 540 icons.](https://github.com/microsoft/vscode-codicons)
### Examples
```python
import qtawesome as qta
```
- Use Font Awesome, Elusive Icons, Material Design Icons, Phosphor, Remix Icon or Microsoft's Codicons.
```python
# Get FontAwesome 5.x icons by name in various styles:
fa5_icon = qta.icon('fa5.flag')
fa5_button = QtWidgets.QPushButton(fa5_icon, 'Font Awesome! (regular)')
fa5s_icon = qta.icon('fa5s.flag')
fa5s_button = QtWidgets.QPushButton(fa5s_icon, 'Font Awesome! (solid)')
fa5b_icon = qta.icon('fa5b.github')
fa5b_button = QtWidgets.QPushButton(fa5b_icon, 'Font Awesome! (brands)')
# or Elusive Icons:
asl_icon = qta.icon('ei.asl')
elusive_button = QtWidgets.QPushButton(asl_icon, 'Elusive Icons!')
# or Material Design Icons:
apn_icon = qta.icon('mdi6.access-point-network')
mdi6_button = QtWidgets.QPushButton(apn_icon, 'Material Design Icons!')
# or Phosphor:
mic_icon = qta.icon('ph.microphone-fill')
ph_button = QtWidgets.QPushButton(mic_icon, 'Phosphor!')
# or Remix Icon:
truck_icon = qta.icon('ri.truck-fill')
ri_button = QtWidgets.QPushButton(truck_icon, 'Remix Icon!')
# or Microsoft's Codicons:
squirrel_icon = qta.icon('msc.squirrel')
msc_button = QtWidgets.QPushButton(squirrel_icon, 'Codicons!')
```
- Apply some styling
```python
# Styling icons
styling_icon = qta.icon('fa5s.music',
active='fa5s.balance-scale',
color='blue',
color_active='orange')
music_button = QtWidgets.QPushButton(styling_icon, 'Styling')
```
- Set alpha in colors
```python
# Setting an alpha of 120 to the color of this icon. Alpha must be a number
# between 0 and 255.
icon_with_alpha = qta.icon('mdi.heart',
color=('red', 120))
heart_button = QtWidgets.QPushButton(icon_with_alpha, 'Setting alpha')
```
- Stack multiple icons
```python
# Stacking icons
camera_ban = qta.icon('fa5s.camera', 'fa5s.ban',
options=[{'scale_factor': 0.5,
'active': 'fa5s.balance-scale'},
{'color': 'red'}])
stack_button = QtWidgets.QPushButton(camera_ban, 'Stack')
stack_button.setIconSize(QtCore.QSize(32, 32))
```
- Define the way to draw icons (`text`- default for icons without animation, `path` - default for icons with animations, `glyphrun` and `image`)
```python
# Icon drawn with the `image` option
drawn_image_icon = qta.icon('ri.truck-fill',
options=[{'draw': 'image'}])
drawn_image_button = QtWidgets.QPushButton(drawn_image_icon,
'Icon drawn as an image')
```
- Animations
```python
# Spining icons
spin_button = QtWidgets.QPushButton(' Spinning icon')
animation = qta.Spin(spin_button)
spin_icon = qta.icon('fa5s.spinner', color='red', animation=animation)
spin_button.setIcon(spin_icon)
# Stop the animation when needed
animation.stop()
```
- Display Icon as a widget
```python
# Spining icon widget
spin_widget = qta.IconWidget()
animation = qta.Spin(spin_widget, autostart=False)
spin_icon = qta.icon('mdi.loading', color='red', animation=animation)
spin_widget.setIcon(spin_icon)
# Simple icon widget
simple_widget = qta.IconWidget('mdi.web', color='blue',
size=QtCore.QSize(16, 16))
# Start and stop the animation when needed
animation.start()
animation.stop()
```
- Screenshot
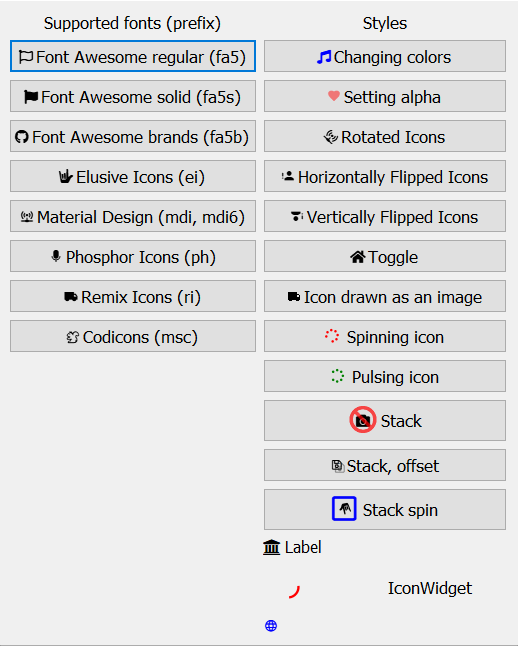
To check these options you can launch the `example.py` script and pass to it the options as arguments. For example, to test how the icons could look using the `glyphrun` draw option, you can run something like:
```
python example.py draw=glyphrun
```
## Other features
- QtAwesome comes bundled with _Font Awesome_, _Elusive Icons_, _Material Design_
_Icons_, _Phosphor_, _Remix Icon_ and Microsoft's _Codicons_
but it can also be used with other iconic fonts. The `load_font`
function allows to load other fonts dynamically.
- QtAwesome relies on the [QtPy](https://github.com/spyder-ide/qtpy.git)
project as a compatibility layer on the top ot PyQt or PySide.
### Icon Browser
QtAwesome ships with a browser that displays all the available icons. You can
use this to search for an icon that suits your requirements and then copy the
name that should be used to create that icon!
Once installed, run `qta-browser` from a shell to start the browser.
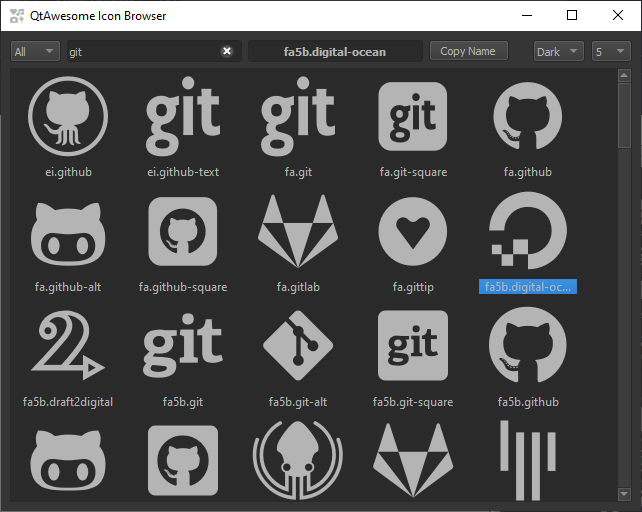
## License
MIT License. Copyright 2015 - The Spyder development team.
See the [LICENSE](LICENSE) file for details.
The [Font Awesome](https://github.com/FortAwesome/Font-Awesome/blob/master/LICENSE.txt) and [Elusive Icons](http://elusiveicons.com/license/) fonts are licensed under the [SIL Open Font License](http://scripts.sil.org/OFL).
The Phosphor font is licensed under the [MIT License](https://github.com/phosphor-icons/phosphor-icons/blob/master/LICENSE).
The [Material Design Icons](https://github.com/Templarian/MaterialDesign/blob/master/LICENSE) font is licensed under the [Apache License Version 2.0](http://www.apache.org/licenses/LICENSE-2.0).
The Remix Icon font is licensed under the [Apache License Version 2.0](https://github.com/Remix-Design/remixicon/blob/master/License).
Microsoft's Codicons are licensed under a [Creative Commons Attribution 4.0 International Public License](https://github.com/microsoft/vscode-codicons/blob/master/LICENSE).
## Sponsors
Spyder and its subprojects are funded thanks to the generous support of
[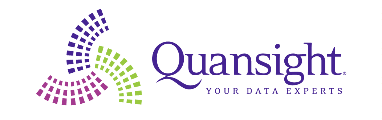](https://www.quansight.com/)[](https://numfocus.org/)
and the donations we have received from our users around the world through [Open Collective](https://opencollective.com/spyder/):
[](https://opencollective.com/spyder#support)
Raw data
{
"_id": null,
"home_page": "https://github.com/spyder-ide/qtawesome",
"name": "QtAwesome",
"maintainer": "Spyder Development Team and QtAwesome Contributors",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "spyder.python@gmail.com",
"keywords": "PyQt, PySide, Icons, Font Awesome, Fonts",
"author": "Sylvain Corlay and the Spyder Development Team",
"author_email": "spyder.python@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/a4/be/3fa8df267d0651597acdefe124cd1595d3839aed3ce97da902efc44a2160/QtAwesome-1.3.1.tar.gz",
"platform": "OS-independent",
"description": "# QtAwesome\r\n\r\n[](./LICENSE)\r\n[](https://pypi.org/project/qtawesome/)\r\n[](https://www.anaconda.com/download/)\r\n[](https://www.anaconda.com/download/)\r\n[](#backers)\r\n[](https://gitter.im/spyder-ide/public)<br>\r\n[](https://github.com/spyder-ide/qtawesome)\r\n[](https://github.com/spyder-ide/qtawesome/actions)\r\n[](https://github.com/spyder-ide/qtawesome/actions)\r\n[](https://github.com/spyder-ide/qtawesome/actions)\r\n[](https://qtawesome.readthedocs.io/en/latest/?badge=latest)\r\n[](https://codecov.io/gh/spyder-ide/qtawesome)\r\n\r\n*Copyright \u00a9 2015- Spyder Project Contributors*\r\n\r\n\r\n## Description\r\n\r\nQtAwesome enables iconic fonts such as Font Awesome and Elusive Icons\r\nin PyQt and PySide applications.\r\n\r\nIt started as a Python port of the [QtAwesome](\r\nhttps://github.com/Gamecreature/qtawesome)\r\nC++ library by Rick Blommers.\r\n\r\n\r\n## Installation\r\n\r\nUsing `conda`:\r\n\r\n```\r\nconda install qtawesome\r\n```\r\n\r\nor using `pip` (only if you don't have conda installed):\r\n\r\n```\r\npip install qtawesome\r\n```\r\n\r\n\r\n## Usage\r\n\r\n### Supported Fonts\r\n\r\nQtAwesome identifies icons by their **prefix** and their **icon name**, separated by a *period* (`.`) character.\r\n\r\nThe following prefixes are currently available to use:\r\n\r\n- [**FontAwesome**](https://fontawesome.com):\r\n\r\n - FA 5.15.4 features 1,608 free icons in different styles:\r\n\r\n - `fa5` prefix has [151 icons in the \"**regular**\" style.](https://fontawesome.com/v5/search?o=r&m=free&s=regular)\r\n - `fa5s` prefix has [1001 icons in the \"**solid**\" style.](https://fontawesome.com/v5/search?o=r&m=free&s=solid)\r\n - `fa5b` prefix has [456 icons of various **brands**.](https://fontawesome.com/v5/search?o=r&m=free&f=brands)\r\n\r\n - `fa` is the legacy [FA 4.7 version with its 675 icons](https://fontawesome.com/v4.7.0/icons/) but **all** of them (*and more!*) are part of FA 5.x so you should probably use the newer version above.\r\n\r\n- `ei` prefix holds [**Elusive Icons** 2.0 with its 304 icons](http://elusiveicons.com/icons/).\r\n\r\n- [**Material Design Icons**](https://pictogrammers.com/library/mdi/)\r\n\r\n - `mdi6` prefix holds [**Material Design Icons** 6.9.96 with its 6997 icons.](https://cdn.materialdesignicons.com/6.9.96/)\r\n\r\n - `mdi` prefix holds [**Material Design Icons** 5.9.55 with its 5955 icons.](https://cdn.materialdesignicons.com/5.9.55/)\r\n\r\n- `ph` prefix holds [**Phosphor** 1.3.0 with its 4470 icons (894 icons * 5 weights: Thin, Light, Regular, Bold and Fill).](https://github.com/phosphor-icons/phosphor-icons)\r\n\r\n- `ri` prefix holds [**Remix Icon** 2.5.0 with its 2271 icons.](https://github.com/Remix-Design/RemixIcon)\r\n\r\n- `msc` prefix holds Microsoft's [**Codicons** 0.0.35 with its 540 icons.](https://github.com/microsoft/vscode-codicons)\r\n\r\n### Examples\r\n\r\n```python\r\nimport qtawesome as qta\r\n```\r\n\r\n- Use Font Awesome, Elusive Icons, Material Design Icons, Phosphor, Remix Icon or Microsoft's Codicons.\r\n\r\n```python\r\n# Get FontAwesome 5.x icons by name in various styles:\r\nfa5_icon = qta.icon('fa5.flag')\r\nfa5_button = QtWidgets.QPushButton(fa5_icon, 'Font Awesome! (regular)')\r\nfa5s_icon = qta.icon('fa5s.flag')\r\nfa5s_button = QtWidgets.QPushButton(fa5s_icon, 'Font Awesome! (solid)')\r\nfa5b_icon = qta.icon('fa5b.github')\r\nfa5b_button = QtWidgets.QPushButton(fa5b_icon, 'Font Awesome! (brands)')\r\n\r\n# or Elusive Icons:\r\nasl_icon = qta.icon('ei.asl')\r\nelusive_button = QtWidgets.QPushButton(asl_icon, 'Elusive Icons!')\r\n\r\n# or Material Design Icons:\r\napn_icon = qta.icon('mdi6.access-point-network')\r\nmdi6_button = QtWidgets.QPushButton(apn_icon, 'Material Design Icons!')\r\n\r\n# or Phosphor:\r\nmic_icon = qta.icon('ph.microphone-fill')\r\nph_button = QtWidgets.QPushButton(mic_icon, 'Phosphor!')\r\n\r\n# or Remix Icon:\r\ntruck_icon = qta.icon('ri.truck-fill')\r\nri_button = QtWidgets.QPushButton(truck_icon, 'Remix Icon!')\r\n\r\n# or Microsoft's Codicons:\r\nsquirrel_icon = qta.icon('msc.squirrel')\r\nmsc_button = QtWidgets.QPushButton(squirrel_icon, 'Codicons!')\r\n\r\n```\r\n\r\n- Apply some styling\r\n\r\n```python\r\n# Styling icons\r\nstyling_icon = qta.icon('fa5s.music',\r\n active='fa5s.balance-scale',\r\n color='blue',\r\n color_active='orange')\r\nmusic_button = QtWidgets.QPushButton(styling_icon, 'Styling')\r\n```\r\n\r\n- Set alpha in colors\r\n\r\n```python\r\n# Setting an alpha of 120 to the color of this icon. Alpha must be a number\r\n# between 0 and 255.\r\nicon_with_alpha = qta.icon('mdi.heart',\r\n color=('red', 120))\r\nheart_button = QtWidgets.QPushButton(icon_with_alpha, 'Setting alpha')\r\n```\r\n\r\n- Stack multiple icons\r\n\r\n```python\r\n# Stacking icons\r\ncamera_ban = qta.icon('fa5s.camera', 'fa5s.ban',\r\n options=[{'scale_factor': 0.5,\r\n 'active': 'fa5s.balance-scale'},\r\n {'color': 'red'}])\r\nstack_button = QtWidgets.QPushButton(camera_ban, 'Stack')\r\nstack_button.setIconSize(QtCore.QSize(32, 32))\r\n```\r\n\r\n- Define the way to draw icons (`text`- default for icons without animation, `path` - default for icons with animations, `glyphrun` and `image`)\r\n\r\n```python\r\n# Icon drawn with the `image` option\r\ndrawn_image_icon = qta.icon('ri.truck-fill',\r\n options=[{'draw': 'image'}])\r\ndrawn_image_button = QtWidgets.QPushButton(drawn_image_icon,\r\n 'Icon drawn as an image')\r\n```\r\n\r\n- Animations\r\n\r\n```python\r\n# Spining icons\r\nspin_button = QtWidgets.QPushButton(' Spinning icon')\r\nanimation = qta.Spin(spin_button)\r\nspin_icon = qta.icon('fa5s.spinner', color='red', animation=animation)\r\nspin_button.setIcon(spin_icon)\r\n\r\n# Stop the animation when needed\r\nanimation.stop()\r\n```\r\n\r\n- Display Icon as a widget\r\n\r\n```python\r\n# Spining icon widget\r\nspin_widget = qta.IconWidget()\r\nanimation = qta.Spin(spin_widget, autostart=False)\r\nspin_icon = qta.icon('mdi.loading', color='red', animation=animation)\r\nspin_widget.setIcon(spin_icon)\r\n\r\n# Simple icon widget\r\nsimple_widget = qta.IconWidget('mdi.web', color='blue', \r\n size=QtCore.QSize(16, 16))\r\n\r\n# Start and stop the animation when needed\r\nanimation.start()\r\nanimation.stop()\r\n```\r\n\r\n- Screenshot\r\n\r\n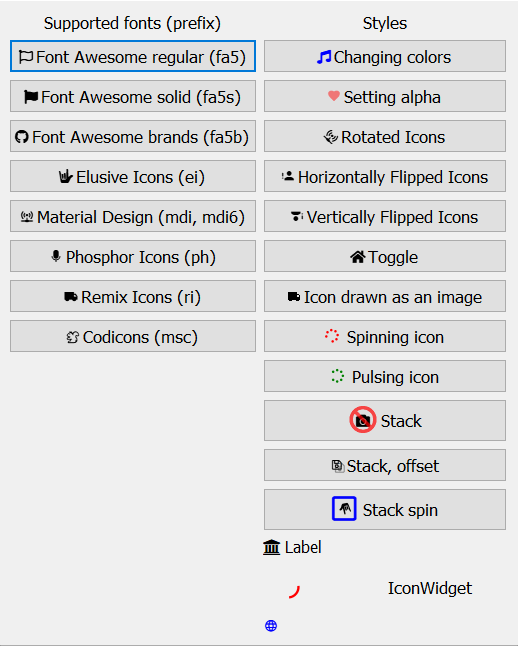\r\n\r\n\r\nTo check these options you can launch the `example.py` script and pass to it the options as arguments. For example, to test how the icons could look using the `glyphrun` draw option, you can run something like:\r\n\r\n```\r\npython example.py draw=glyphrun\r\n```\r\n\r\n## Other features\r\n\r\n- QtAwesome comes bundled with _Font Awesome_, _Elusive Icons_, _Material Design_\r\n _Icons_, _Phosphor_, _Remix Icon_ and Microsoft's _Codicons_\r\n but it can also be used with other iconic fonts. The `load_font`\r\n function allows to load other fonts dynamically.\r\n- QtAwesome relies on the [QtPy](https://github.com/spyder-ide/qtpy.git)\r\n project as a compatibility layer on the top ot PyQt or PySide.\r\n\r\n### Icon Browser\r\n\r\nQtAwesome ships with a browser that displays all the available icons. You can\r\nuse this to search for an icon that suits your requirements and then copy the\r\nname that should be used to create that icon!\r\n\r\nOnce installed, run `qta-browser` from a shell to start the browser.\r\n\r\n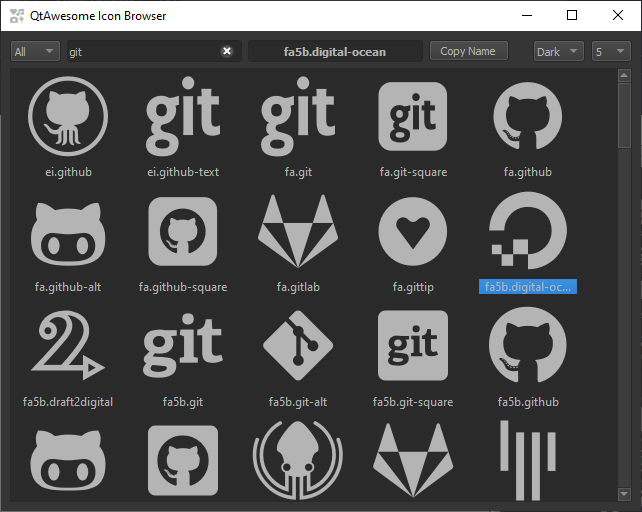\r\n\r\n\r\n## License\r\n\r\nMIT License. Copyright 2015 - The Spyder development team.\r\nSee the [LICENSE](LICENSE) file for details.\r\n\r\nThe [Font Awesome](https://github.com/FortAwesome/Font-Awesome/blob/master/LICENSE.txt) and [Elusive Icons](http://elusiveicons.com/license/) fonts are licensed under the [SIL Open Font License](http://scripts.sil.org/OFL).\r\n\r\nThe Phosphor font is licensed under the [MIT License](https://github.com/phosphor-icons/phosphor-icons/blob/master/LICENSE).\r\n\r\nThe [Material Design Icons](https://github.com/Templarian/MaterialDesign/blob/master/LICENSE) font is licensed under the [Apache License Version 2.0](http://www.apache.org/licenses/LICENSE-2.0).\r\n\r\nThe Remix Icon font is licensed under the [Apache License Version 2.0](https://github.com/Remix-Design/remixicon/blob/master/License).\r\n\r\nMicrosoft's Codicons are licensed under a [Creative Commons Attribution 4.0 International Public License](https://github.com/microsoft/vscode-codicons/blob/master/LICENSE).\r\n\r\n## Sponsors\r\n\r\nSpyder and its subprojects are funded thanks to the generous support of\r\n\r\n[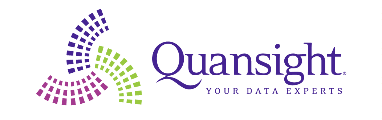](https://www.quansight.com/)[](https://numfocus.org/)\r\n\r\nand the donations we have received from our users around the world through [Open Collective](https://opencollective.com/spyder/):\r\n\r\n[](https://opencollective.com/spyder#support)\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "FontAwesome icons in PyQt and PySide applications",
"version": "1.3.1",
"project_urls": {
"Homepage": "https://github.com/spyder-ide/qtawesome"
},
"split_keywords": [
"pyqt",
" pyside",
" icons",
" font awesome",
" fonts"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "35b0f5d87ddd388917965377c64ae8ee70f3fa6cb565823be78f5c4bd0383ec5",
"md5": "480c016bf9438c2e239658cb7276ed05",
"sha256": "6078449035cd5311bc461e99940a426c27cb919fb4211c8cfc64506cb71e2dd7"
},
"downloads": -1,
"filename": "QtAwesome-1.3.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "480c016bf9438c2e239658cb7276ed05",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 2355279,
"upload_time": "2024-03-27T22:32:45",
"upload_time_iso_8601": "2024-03-27T22:32:45.440047Z",
"url": "https://files.pythonhosted.org/packages/35/b0/f5d87ddd388917965377c64ae8ee70f3fa6cb565823be78f5c4bd0383ec5/QtAwesome-1.3.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a4be3fa8df267d0651597acdefe124cd1595d3839aed3ce97da902efc44a2160",
"md5": "078a930c86cca0a2cd48df3135fe6854",
"sha256": "075b2c9ee01cbaf5e3a4bebed0e5529ee8605981355f21dea051b15c1b869e1b"
},
"downloads": -1,
"filename": "QtAwesome-1.3.1.tar.gz",
"has_sig": false,
"md5_digest": "078a930c86cca0a2cd48df3135fe6854",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 2372190,
"upload_time": "2024-03-27T22:32:49",
"upload_time_iso_8601": "2024-03-27T22:32:49.029138Z",
"url": "https://files.pythonhosted.org/packages/a4/be/3fa8df267d0651597acdefe124cd1595d3839aed3ce97da902efc44a2160/QtAwesome-1.3.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-27 22:32:49",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "spyder-ide",
"github_project": "qtawesome",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "qtawesome"
}