# RyuzakiLib

[](https://pypi.org/project/RyuzakiLib)
[](https://pypi.org/project/RyuzakiLib)
[](https://github.com/TeamKillerX/RyuzakiLib/graphs/commit-activity)
[](https://github.com/TeamKillerX/RyuzakiLib)
[](https://makeapullrequest.com)

[](https://results.pre-commit.ci/latest/github/TeamKillerX/RyuzakiLib/dev)
# Disclaimer
> [!WARNING]
> RyuzakiLib is used to help your account activities on Telegram<br>We are not responsible for what you misuse in this repository!<br>Be careful when using this repository!<br>If one of the members misuses this repository, we are forced to ban you<br>Never ever abuse this repository
# Installing
* `pip3 install -U RyuzakiLib`
* windows or linux
```
pip3 install git+https://github.com/TeamKillerX/RyuzakiLib
```
### Import Here
```python
from RyuzakiLib.hackertools.chatgpt import RendyDevChat
from RyuzakiLib.hackertools.github import GithubUsername
from RyuzakiLib.hackertools.rmbg import RemoveBg
from RyuzakiLib.hackertools.reverse import GoogleReverseImage
from RyuzakiLib.hackertools.ipinfo import WhoisIpHacker
from RyuzakiLib.hackertools.ocrapi import OcrApiUrl
from RyuzakiLib.hackertools.prefixes import CustomPrefixes
```
### Learn Python
```python
import asyncio
class example_python:
def __init__(self):
pass
def hello_world(self):
asyncio.sleep(5)
# examples usage
ok = example_python()
test = ok.hello_world()
```
### Tutorial FastAPI
```python
from fastapi import FastAPI
from RyuzakiLib.hackertools.chatgpt import RendyDevChat
from RyuzakiLib.hackertools.openai import OpenAiToken
app = FastAPI()
@app.get("/read")
def hello():
return {"message": "Hello World"}
if __name__ == "__main__":
uvicorn.run(app, host="0.0.0.0")
```
- Like example this [`RyuzakiLib API`](https://private.randydev.my.id)
* [x] Requirements: `fastapi` and `RyuzakiLib`
### Spamwatch
• Example usage
```python
from RyuzakiLib.spamwatch.clients import SibylBan
clients = SibylBan(api_key="your_api_key_here")
message = clients.add_ban(user_id=client.me.id, reason="scammer", is_banned=True)
await message.reply_text(message)
# Part 2
showing = clients.get_ban(user_id=client.me.id, banlist=True)
print(showing)
# Part 3
results = clients.get_all_banlist()
print(results)
```
### Profile Clone
• Example usage
```python
from RyuzakiLib.profile.user import ProfileClone
clients = ProfileClone(api_key="your_api_key_here")
message = clients.add_profile_clone() # need parameter
showing = clients.get_profile_clone() # need parameter
print(showing)
```
### Learn Telegram Bot API
```python
from RyuzakiLib.bot import API
api = API(bot_token="your_token")
# supported here
api.send_message()
api.send_photo()
api.send_sticker()
api.send_audio()
api.send_document()
api.forward_message()
# you can pass
sent_message = api.send_message(chat_id=chat_id, text=text)
sent_sticker = api.send_sticker(chat_id=chat_id, sticker=sticker)
# your own developer
urls = api.telegram("SendMessage")
payload = {}
headers = {}
response = requests.post(urls, json=payload, headers=headers)
print(response.text)
```
### Chatgpt New?
* Chatgpt's new features are available here
- parameter
```python
from RyuzakiLib.hackertools.chatgpt import RendyDevChat
query = "Hello World"
code = RendyDevChat(query)
message_output = code.get_response(message, latest_version=True)
message_output_2 = code.get_response_beta(joke=True)
message_output_3 = code.get_response_bing(bing=True)
message_output_4 = code.get_response_model() # parameter model_id: integers and is_models: boolean
message_output_5 = code.get_response_llama(llama=True)
message_output_6 = code.get_response_gemini_pro(api_key=api_key, re_json=True, is_gemini_pro=True)
message_output_7 = code.get_response_google_ai(api_key=api_key, re_json=True, is_chat_bison=True, is_google=True)
message_output_8 = code.multi_chat_response(api_key=api_key, is_multi_chat=True)
print(message_output)
print(message_output_2)
print(message_output_3)
print(message_output_4)
print(message_output_5)
print(message_output_6)
print(message_output_7)
print(message_output_8)
```
### AI image Generator New?
* AI image Generator new features are available here
- parameter
```python
import base64
from io import BytesIO
from PIL import Image
from RyuzakiLib.hackertools.chatgpt import RendyDevChat
query = "Hello World"
code = RendyDevChat(query)
response = code.get_risma_image_generator(api_key=api_key, is_opendalle=True)
image_data = base64.b64decode(response["randydev"].get("data"))
image = Image.open(BytesIO(image_data))
```
### Continue Conversation
```python
from RyuzakiLib import OpenAiToken
api_base = "https://api.example.com/v1"
api_key = ""
query = "hello world"
response = OpenAiToken(api_key=api_key, api_base=api_base).chat_message_turbo(
query=query,
model="gpt-4",
is_stream=False
)
print(response[0])
```
### Example Chatgpt
```python
from pyrogram import Client, filters
from pyrogram.types import Message
from RyuzakiLib.hackertools.chatgpt import RendyDevChat
query = "Hello World"
response = RendyDevChat(query).get_response(message, latest_version=True)
await message.reply(response)
```
### Example Google Reverse Image
```python
from pyrogram import Client, filters
from pyrogram.types import Message
from RyuzakiLib.hackertools.reverse import GoogleReverseImage
url = "https://example/jpg"
apikey = "api key token"
response = GoogleReverseImage(url, apikey)
results = response.get_reverse()
print(results)
```
### New Features
```python
# Create by @xtdevs
# Prefixes Custom
from pyrogram import Client, filters
from pyrogram.types import Message
from RyuzakiLib.hackertools.prefixes import CustomPrefixes
from pymongo import MongoClient
client = MongoClient("mongodb://localhost:27017/")
db = client["your_database_name"]
collection = db["your_collection_name"]
db_name = "custom_prefixes"
user_id = message.from_user.id
prefix = [".", "+", "-"]
set_handler = CustomPrefixes(db_name, user_id, prefix, collection, True) # parameter upsert using set True or False
set_handler.add_prefixes()
now_show_prefix = set_handler.get_prefix()
print(now_show_prefix)
```
### Gemini AI Pro (Free)
- No authorization needed
- Gemini Pro (Without RyuzakiLib API keys)
- Multi-Turn Conversation
```python
from RyuzakiLib import GeminiLatest
mongo_url = "....."
user_id = 0
gt = GeminiLatest(api_key=api_key, mongo_url=mongo_url, user_id=user_id)
# You don't use this Close
gt.__del__()
# Get response
message _, = gt._get_response_gemini(query)
# OR RyuzakiLib API
import requests
url = "https://randydev-ryuzaki-api.hf.space/ryuzaki/gemini-ai-pro"
payload = {
"query": "string",
"user_id": 12345,
"mongo_url": "string",
"gemini_api_key": "string", # you can leave it empty
"is_login": False,
"is_multi_chat": True
}
response = requests.post(url, json=payload).json()
```
- Gemini AI pro: Get [API key Here](https://makersuite.google.com/app/apikey) from Google Dev
- You can ask support [@KillerXSupport](https://t.me/KillerXSupport)
### Test your bots
```bash
- git clone https://github.com/TeamKillerX/RyuzakiLib
- cd RyuzakiLib
- pip3 install -r ryuzaki.txt
- nano buildbot/secrets/env.py
- ctrl s + x to save
- bash start.sh
```
### RyuzakiLib API

## Authentication
> **Warning** Do not expose the `__Secure-1PSID`
```python
import requests
url ="https://randydev-ryuzaki-api.hf.space/ryuzaki/gemini-ai-pro"
payload = {
"query": "hello world",
"bard_api_key": "cookie token here",
"is_login": True
}
headers = {
"accept": "application/json",
"api-key": "get api key from @randydev_bot"
}
response = requests.post(url, headers=headers, json=payload).json()
print(response)
```
* `bard_api_key` : (optional)
* `is_login` : default `False` (optional)
1. Visit https://bard.google.com/
2. F12 for console
3. Session: Application → Cookies → Copy the value of `__Secure-1PSID` cookie.
Note that while I referred to `__Secure-1PSID` value as an API key for convenience, it is not an officially provided API key. Cookie value subject to frequent changes. Verify the value again if an error occurs. Most errors occur when an invalid cookie value is entered.
`bard_api_key={__Secure-1PSID}`
### Tutorial Requests in Python
>Request body schema
* `json=payload`
```python
response = requests.post(url, headers=headers, json=payload).json()
```
> Query Parameters
* `params=params`
```python
response = requests.post(url, headers=headers, params=params).json()
```
You can find the [`Ryuzaki API`](https://private.randydev.my.id)
### Ryuzaki API Request (API Keys)
- Full Name : (...)
- Email address : (...)
- Telegram Username : (....)
- Project Domain [Optional] : (....)
- Why you want to use this API and or why we should give you access?
(......)
* Choose your one
1. [ ] ChatGPT-4 Free Access
2. [ ] I wont use this API for anything illegal
3. [ ] 5 USD/Month - Etc
~ Starting january 1, 2024, remove the `/token_api_key` command in [ryuzaki bots](https://t.me/randydev_bot)
### Ryuzaki API Pricing (API keys)
> [!NOTE]
> - Picsart Pro (7 days)
> - Gemini AI Ultra (7 days)
> - Beta3 Google AI (7 days)
> - Beta2 Google AI (7 days)
> - Anime Styled (7 days)
* Only Developed by
- [@xtdevs](https://t.me/xtdevs)
- [@TrueSaiyan](https://t.me/TrueSaiyan)
- [@moiusrname](https://t.me/moiusrname)
- [@Hackintush](https://t.me/Hackintush)
* Contact Support: [@xtdevs](https://t.me/xtdevs)
### Troubleshoot
Sometimes errors occur, but we are here to help! This guide covers some of the most common issues we’ve seen and how you can resolve them. However, this guide isn’t meant to be a comprehensive collection of every 🤗 FastAPI issue. For more help with troubleshooting your issue, try:
* [Contact Support](https://t.me/xtdevs)
# License
[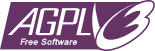](LICENSE)
TeamKillerX is licensed under [GNU Affero General Public License](https://www.gnu.org/licenses/agpl-3.0.en.html) v3 or later.
<h4 align="center">Copyright (C) 2019 - 2024 The RyuzakiLib <a href="https://github.com/TeamKillerX">TeamKillerX</a>
<a href="https://t.me/xtdevs">@xtdevs</a>
</h4>
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
Project [RyuzakiLib](https://github.com/TeamKillerX/) is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <https://www.gnu.org/licenses/>.
### Contributing
* [Fork the project](https://github.com/TeamKillerX/RyuzakiLib) and send pull requests
<a href="https://github.com/TeamKillerX/RyuzakiLib/graphs/contributors">
<img src="https://contrib.rocks/image?repo=TeamKillerX/RyuzakiLib" />
</a>
### Credits
* [](https://t.me/xtdevs)
* Pyrogram by : [Dan](https://github.com/pyrogram/pyrogram)
* PY-Tgcalls by : [Pytgcalls](https://github.com/pytgcalls/pytgcalls)
Raw data
{
"_id": null,
"home_page": "https://github.com/TeamKillerX/RyuzakiLib/",
"name": "RyuzakiLib",
"maintainer": "",
"docs_url": null,
"requires_python": "~=3.7",
"maintainer_email": "",
"keywords": "API,RyuzakiLib,Ryuzaki_Library,Ryuzaki-API",
"author": "TeamKillerX",
"author_email": "killerx@randydev.my.id",
"download_url": "https://files.pythonhosted.org/packages/a3/89/d4a8393e32fe6f51cffc23a9eb910ad36200f1e9869c0a18fa9ebf943cac/RyuzakiLib-1.1.4.tar.gz",
"platform": null,
"description": "# RyuzakiLib\n\n\n\n[](https://pypi.org/project/RyuzakiLib)\n[](https://pypi.org/project/RyuzakiLib)\n[](https://github.com/TeamKillerX/RyuzakiLib/graphs/commit-activity)\n[](https://github.com/TeamKillerX/RyuzakiLib)\n[](https://makeapullrequest.com)\n\n[](https://results.pre-commit.ci/latest/github/TeamKillerX/RyuzakiLib/dev)\n\n# Disclaimer\n> [!WARNING]\n> RyuzakiLib is used to help your account activities on Telegram<br>We are not responsible for what you misuse in this repository!<br>Be careful when using this repository!<br>If one of the members misuses this repository, we are forced to ban you<br>Never ever abuse this repository\n\n# Installing\n* `pip3 install -U RyuzakiLib`\n* windows or linux\n```\npip3 install git+https://github.com/TeamKillerX/RyuzakiLib\n```\n\n### Import Here\n```python\nfrom RyuzakiLib.hackertools.chatgpt import RendyDevChat\nfrom RyuzakiLib.hackertools.github import GithubUsername\nfrom RyuzakiLib.hackertools.rmbg import RemoveBg\nfrom RyuzakiLib.hackertools.reverse import GoogleReverseImage\nfrom RyuzakiLib.hackertools.ipinfo import WhoisIpHacker\nfrom RyuzakiLib.hackertools.ocrapi import OcrApiUrl\nfrom RyuzakiLib.hackertools.prefixes import CustomPrefixes\n```\n\n### Learn Python\n```python\nimport asyncio\n\nclass example_python:\n def __init__(self):\n pass\n\n def hello_world(self):\n asyncio.sleep(5)\n\n# examples usage\nok = example_python()\ntest = ok.hello_world()\n```\n\n### Tutorial FastAPI\n```python\nfrom fastapi import FastAPI\nfrom RyuzakiLib.hackertools.chatgpt import RendyDevChat\nfrom RyuzakiLib.hackertools.openai import OpenAiToken\n\napp = FastAPI()\n\n@app.get(\"/read\")\ndef hello():\n return {\"message\": \"Hello World\"}\n\nif __name__ == \"__main__\":\n uvicorn.run(app, host=\"0.0.0.0\")\n```\n- Like example this [`RyuzakiLib API`](https://private.randydev.my.id)\n* [x] Requirements: `fastapi` and `RyuzakiLib`\n\n### Spamwatch\n\u2022 Example usage\n```python\nfrom RyuzakiLib.spamwatch.clients import SibylBan\n\nclients = SibylBan(api_key=\"your_api_key_here\")\n\nmessage = clients.add_ban(user_id=client.me.id, reason=\"scammer\", is_banned=True)\nawait message.reply_text(message)\n\n# Part 2\nshowing = clients.get_ban(user_id=client.me.id, banlist=True)\nprint(showing)\n\n# Part 3\nresults = clients.get_all_banlist()\nprint(results)\n```\n\n### Profile Clone\n\u2022 Example usage\n```python\nfrom RyuzakiLib.profile.user import ProfileClone\n\nclients = ProfileClone(api_key=\"your_api_key_here\")\n\nmessage = clients.add_profile_clone() # need parameter\n\nshowing = clients.get_profile_clone() # need parameter\nprint(showing)\n```\n\n### Learn Telegram Bot API\n```python\n\nfrom RyuzakiLib.bot import API\n\napi = API(bot_token=\"your_token\")\n\n# supported here\napi.send_message()\napi.send_photo()\napi.send_sticker()\napi.send_audio()\napi.send_document()\napi.forward_message()\n\n# you can pass\nsent_message = api.send_message(chat_id=chat_id, text=text)\n\nsent_sticker = api.send_sticker(chat_id=chat_id, sticker=sticker)\n\n# your own developer\nurls = api.telegram(\"SendMessage\")\npayload = {}\nheaders = {}\nresponse = requests.post(urls, json=payload, headers=headers)\nprint(response.text)\n```\n\n### Chatgpt New?\n* Chatgpt's new features are available here\n- parameter\n\n```python\nfrom RyuzakiLib.hackertools.chatgpt import RendyDevChat\n\nquery = \"Hello World\"\n\ncode = RendyDevChat(query)\nmessage_output = code.get_response(message, latest_version=True)\nmessage_output_2 = code.get_response_beta(joke=True)\nmessage_output_3 = code.get_response_bing(bing=True)\nmessage_output_4 = code.get_response_model() # parameter model_id: integers and is_models: boolean\nmessage_output_5 = code.get_response_llama(llama=True)\nmessage_output_6 = code.get_response_gemini_pro(api_key=api_key, re_json=True, is_gemini_pro=True)\nmessage_output_7 = code.get_response_google_ai(api_key=api_key, re_json=True, is_chat_bison=True, is_google=True)\nmessage_output_8 = code.multi_chat_response(api_key=api_key, is_multi_chat=True)\n\nprint(message_output)\nprint(message_output_2)\nprint(message_output_3)\nprint(message_output_4)\nprint(message_output_5)\nprint(message_output_6)\nprint(message_output_7)\nprint(message_output_8)\n```\n\n### AI image Generator New?\n* AI image Generator new features are available here\n- parameter\n```python\nimport base64\nfrom io import BytesIO\nfrom PIL import Image\nfrom RyuzakiLib.hackertools.chatgpt import RendyDevChat\n\nquery = \"Hello World\"\ncode = RendyDevChat(query)\nresponse = code.get_risma_image_generator(api_key=api_key, is_opendalle=True)\n\nimage_data = base64.b64decode(response[\"randydev\"].get(\"data\"))\nimage = Image.open(BytesIO(image_data))\n```\n\n### Continue Conversation\n```python\nfrom RyuzakiLib import OpenAiToken\n\napi_base = \"https://api.example.com/v1\"\napi_key = \"\"\nquery = \"hello world\"\nresponse = OpenAiToken(api_key=api_key, api_base=api_base).chat_message_turbo(\n query=query,\n model=\"gpt-4\",\n is_stream=False\n)\n\nprint(response[0])\n```\n\n### Example Chatgpt\n```python\nfrom pyrogram import Client, filters\nfrom pyrogram.types import Message\n\nfrom RyuzakiLib.hackertools.chatgpt import RendyDevChat\n\nquery = \"Hello World\"\nresponse = RendyDevChat(query).get_response(message, latest_version=True)\nawait message.reply(response)\n```\n\n### Example Google Reverse Image\n```python\nfrom pyrogram import Client, filters\nfrom pyrogram.types import Message\n\nfrom RyuzakiLib.hackertools.reverse import GoogleReverseImage\n\nurl = \"https://example/jpg\"\napikey = \"api key token\"\nresponse = GoogleReverseImage(url, apikey)\nresults = response.get_reverse()\nprint(results)\n```\n### New Features\n```python\n# Create by @xtdevs\n# Prefixes Custom\n\nfrom pyrogram import Client, filters\nfrom pyrogram.types import Message\n\nfrom RyuzakiLib.hackertools.prefixes import CustomPrefixes\nfrom pymongo import MongoClient\n\nclient = MongoClient(\"mongodb://localhost:27017/\")\ndb = client[\"your_database_name\"]\ncollection = db[\"your_collection_name\"]\n\ndb_name = \"custom_prefixes\"\nuser_id = message.from_user.id\nprefix = [\".\", \"+\", \"-\"]\nset_handler = CustomPrefixes(db_name, user_id, prefix, collection, True) # parameter upsert using set True or False\nset_handler.add_prefixes()\nnow_show_prefix = set_handler.get_prefix()\nprint(now_show_prefix)\n```\n\n### Gemini AI Pro (Free)\n- No authorization needed\n- Gemini Pro (Without RyuzakiLib API keys)\n- Multi-Turn Conversation\n\n```python\nfrom RyuzakiLib import GeminiLatest\n\nmongo_url = \".....\"\nuser_id = 0\ngt = GeminiLatest(api_key=api_key, mongo_url=mongo_url, user_id=user_id)\n\n# You don't use this Close\ngt.__del__()\n\n# Get response\nmessage _, = gt._get_response_gemini(query)\n\n# OR RyuzakiLib API\nimport requests\n\nurl = \"https://randydev-ryuzaki-api.hf.space/ryuzaki/gemini-ai-pro\"\n\npayload = {\n\u00a0\u00a0\u00a0 \"query\": \"string\",\n \"user_id\": 12345,\n \"mongo_url\": \"string\",\n \"gemini_api_key\": \"string\", # you can leave it empty\n\u00a0\u00a0\u00a0 \"is_login\": False,\n\u00a0\u00a0\u00a0 \"is_multi_chat\": True\n}\nresponse = requests.post(url, json=payload).json()\n```\n\n- Gemini AI pro: Get [API key Here](https://makersuite.google.com/app/apikey) from Google Dev\n\n- You can ask support [@KillerXSupport](https://t.me/KillerXSupport)\n\n### Test your bots\n```bash\n- git clone https://github.com/TeamKillerX/RyuzakiLib\n- cd RyuzakiLib\n- pip3 install -r ryuzaki.txt\n- nano buildbot/secrets/env.py\n- ctrl s + x to save\n- bash start.sh\n```\n\n### RyuzakiLib API\n\n\n\n## Authentication\n> **Warning** Do not expose the `__Secure-1PSID`\n```python\nimport requests\n\nurl =\"https://randydev-ryuzaki-api.hf.space/ryuzaki/gemini-ai-pro\"\n\npayload = {\n \"query\": \"hello world\",\n \"bard_api_key\": \"cookie token here\",\n \"is_login\": True\n}\n\nheaders = {\n \"accept\": \"application/json\",\n \"api-key\": \"get api key from @randydev_bot\"\n}\nresponse = requests.post(url, headers=headers, json=payload).json()\nprint(response)\n```\n\n* `bard_api_key` : (optional)\n* `is_login` : default `False` (optional)\n\n1. Visit https://bard.google.com/\n2. F12 for console\n3. Session: Application \u2192 Cookies \u2192 Copy the value of `__Secure-1PSID` cookie.\n\nNote that while I referred to `__Secure-1PSID` value as an API key for convenience, it is not an officially provided API key. Cookie value subject to frequent changes. Verify the value again if an error occurs. Most errors occur when an invalid cookie value is entered.\n\n`bard_api_key={__Secure-1PSID}`\n\n\n### Tutorial Requests in Python\n>Request body schema\n* `json=payload`\n```python\nresponse = requests.post(url, headers=headers, json=payload).json()\n```\n\n> Query Parameters\n* `params=params`\n```python\nresponse = requests.post(url, headers=headers, params=params).json()\n```\n\nYou can find the [`Ryuzaki API`](https://private.randydev.my.id)\n\n### Ryuzaki API Request (API Keys)\n\n- Full Name : (...)\n- Email address : (...)\n- Telegram Username : (....)\n- Project Domain [Optional] : (....)\n\n- Why you want to use this API and or why we should give you access?\n\n(......)\n\n* Choose your one\n\n1. [ ] ChatGPT-4 Free Access\n2. [ ] I wont use this API for anything illegal\n3. [ ] 5 USD/Month - Etc\n\n~ Starting january 1, 2024, remove the `/token_api_key` command in [ryuzaki bots](https://t.me/randydev_bot)\n\n### Ryuzaki API Pricing (API keys)\n> [!NOTE]\n> - Picsart Pro (7 days)\n> - Gemini AI Ultra (7 days)\n> - Beta3 Google AI (7 days)\n> - Beta2 Google AI (7 days)\n> - Anime Styled (7 days)\n\n* Only Developed by\n- [@xtdevs](https://t.me/xtdevs)\n- [@TrueSaiyan](https://t.me/TrueSaiyan)\n- [@moiusrname](https://t.me/moiusrname)\n- [@Hackintush](https://t.me/Hackintush)\n\n* Contact Support: [@xtdevs](https://t.me/xtdevs)\n\n### Troubleshoot\nSometimes errors occur, but we are here to help! This guide covers some of the most common issues we\u2019ve seen and how you can resolve them. However, this guide isn\u2019t meant to be a comprehensive collection of every \ud83e\udd17 FastAPI issue. For more help with troubleshooting your issue, try:\n* [Contact Support](https://t.me/xtdevs)\n\n# License\n[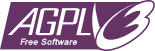](LICENSE)\nTeamKillerX is licensed under [GNU Affero General Public License](https://www.gnu.org/licenses/agpl-3.0.en.html) v3 or later.\n\n<h4 align=\"center\">Copyright (C) 2019 - 2024 The RyuzakiLib <a href=\"https://github.com/TeamKillerX\">TeamKillerX</a>\n<a href=\"https://t.me/xtdevs\">@xtdevs</a>\n</h4>\n\nPermission is hereby granted, free of charge, to any person obtaining a copy\nof this software and associated documentation files (the \"Software\"), to deal\nin the Software without restriction, including without limitation the rights\nto use, copy, modify, merge, publish, distribute, sublicense, and/or sell\ncopies of the Software, and to permit persons to whom the Software is\nfurnished to do so, subject to the following conditions:\n\nThe above copyright notice and this permission notice shall be included in all\ncopies or substantial portions of the Software.\n\nTHE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR\nIMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,\nFITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE\nAUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER\nLIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,\nOUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE\nSOFTWARE.\n\nProject [RyuzakiLib](https://github.com/TeamKillerX/) is free software: you can redistribute it and/or modify\nit under the terms of the GNU General Public License as published by\nthe Free Software Foundation, either version 3 of the License, or\n(at your option) any later version.\nThis program is distributed in the hope that it will be useful,\nbut WITHOUT ANY WARRANTY; without even the implied warranty of\nMERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the\nGNU General Public License for more details.\nYou should have received a copy of the GNU General Public License\nalong with this program. If not, see <https://www.gnu.org/licenses/>.\n\n### Contributing\n* [Fork the project](https://github.com/TeamKillerX/RyuzakiLib) and send pull requests\n\n<a href=\"https://github.com/TeamKillerX/RyuzakiLib/graphs/contributors\">\n <img src=\"https://contrib.rocks/image?repo=TeamKillerX/RyuzakiLib\" />\n</a>\n\n### Credits\n* [](https://t.me/xtdevs)\n* Pyrogram by : [Dan](https://github.com/pyrogram/pyrogram)\n* PY-Tgcalls by : [Pytgcalls](https://github.com/pytgcalls/pytgcalls)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "RyuzakiLib Python Wrapper For API etc",
"version": "1.1.4",
"project_urls": {
"Homepage": "https://github.com/TeamKillerX/RyuzakiLib/"
},
"split_keywords": [
"api",
"ryuzakilib",
"ryuzaki_library",
"ryuzaki-api"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "ba84de81b7e3e43ae9b600e71788827a82f8fb0b3a3b4214627c7f49ed17fdc7",
"md5": "e10d460ba602710b94956819b7a7c6c7",
"sha256": "e55e98527e6bfc5d68276f2f0dce61ccf392a33a2f2a46dcca83658205c66dde"
},
"downloads": -1,
"filename": "RyuzakiLib-1.1.4-py3-none-any.whl",
"has_sig": false,
"md5_digest": "e10d460ba602710b94956819b7a7c6c7",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "~=3.7",
"size": 162207,
"upload_time": "2024-01-12T16:22:57",
"upload_time_iso_8601": "2024-01-12T16:22:57.026205Z",
"url": "https://files.pythonhosted.org/packages/ba/84/de81b7e3e43ae9b600e71788827a82f8fb0b3a3b4214627c7f49ed17fdc7/RyuzakiLib-1.1.4-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a389d4a8393e32fe6f51cffc23a9eb910ad36200f1e9869c0a18fa9ebf943cac",
"md5": "aaf1e90ef03d4ddf423445f8baac03d4",
"sha256": "7663c48fa4619ec57a14fd7e2ad298c4bcad39ff6148bd4ec31c819c2352a9cc"
},
"downloads": -1,
"filename": "RyuzakiLib-1.1.4.tar.gz",
"has_sig": false,
"md5_digest": "aaf1e90ef03d4ddf423445f8baac03d4",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "~=3.7",
"size": 96193,
"upload_time": "2024-01-12T16:22:58",
"upload_time_iso_8601": "2024-01-12T16:22:58.654804Z",
"url": "https://files.pythonhosted.org/packages/a3/89/d4a8393e32fe6f51cffc23a9eb910ad36200f1e9869c0a18fa9ebf943cac/RyuzakiLib-1.1.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-01-12 16:22:58",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "TeamKillerX",
"github_project": "RyuzakiLib",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "ryuzakilib"
}