# Aelog
An simple, async, full package name path, log rotating, different colored log library.
aelog aims to make using python log as simple as possible. as a result, it drastically
simplifies using python logging.
aelog's design objectives:
- Make using python log as simple as possible.
- Output log contains the full package name path.
- Provide asynchronous log output function, at the same time, contains common log output.
- Output according to the log level to mark the different colors separately.
- Provide a log file rotating, automatic backup.
- Output to the terminal and file, default output to the terminal, if you don't provide the log file path.
# Installing aelog
- ```pip install aelog```
# init aelog
```
import aelog
app = Flask(__name__)
aelog.init_app(app)
# or
aelog.init_app(aelog_access_file='aelog_access_file.log', aelog_error_file='aelog_error_file.log',
aelog_console=False)
```
# aelog config
List of configuration keys that the aelog extension recognizes:
| configuration key | the meaning of the configuration key |
| ------ | ------ |
| AELOG_ACCESS_FILE | Access file path, default None. |
| AELOG_ERROR_FILE | Error file path, default None. |
| AELOG_CONSOLE | Whether it is output at the terminal, default false. |
| AELOG_LEVEL | log level, default 'DEBUG'. |
| AELOG_MAX_BYTES | Log file size, default 50M. |
| AELOG_BACKUP_COUNT | Rotating file count, default 5.|
# Usage
### simple using, output log to terminal.
```
import aelog
aelog.init_app(aelog_console=True)
def test_aelog_output_console():
"""
Args:
Returns:
"""
aelog.debug("simple debug message", "other message")
aelog.info("simple info message", "other message")
aelog.warning("simple warning message", "other message")
aelog.error("simple error message", "other message")
aelog.critical("simple critical message", "other message")
try:
5 / 0
except Exception as e:
aelog.exception(e)
```
This will output to the terminal.

- Different levels of logging, different color, the color is cyan, green, yellow, red and 'bold_red,bg_white' in turn.
### output log to file and terminal.
```
import aelog
from flask import Flask
app = Flask(__name__)
aelog.init_app(app) # Output to the test.log file and terminal
def test_aelog_output_file():
"""
Args:
Returns:
"""
aelog.debug("simple debug message", "other message")
aelog.info("simple info message", "other message")
aelog.warning("simple warning message", "other message")
aelog.error("simple error message", "other message")
aelog.critical("simple critical message", "other message")
try:
5 / 0
except Exception as e:
aelog.exception(e)
```
This will output to the test.log file and terminal.

- Automatic output is greater than the error information to the 'test_error.log' file.
- Different levels of logging, different color, the color is cyan, green, yellow, red and 'bold_red,bg_white' in turn.
### asynchronous output log to file and terminal.
```
import asyncio
import aelog
from sanic import Sanic
app = Sanic(__name__)
aelog.init_aelog(app) # Output to the test.log file and terminal
async def test_async_output():
await aelog.async_debug("simple debug message", "other message")
await aelog.async_info("simple info message", "other message")
await aelog.async_warning("simple warning message", "other message")
await aelog.async_error("simple error message", "other message")
await aelog.async_critical("simple critical message", "other message")
try:
5 / 0
except Exception as e:
await aelog.async_exception(e)
if "__name__"=="__main__":
loop = asyncio.get_event_loop()
loop.run_until_complete(test_async_output())
```
This will output to the test.log file and terminal.
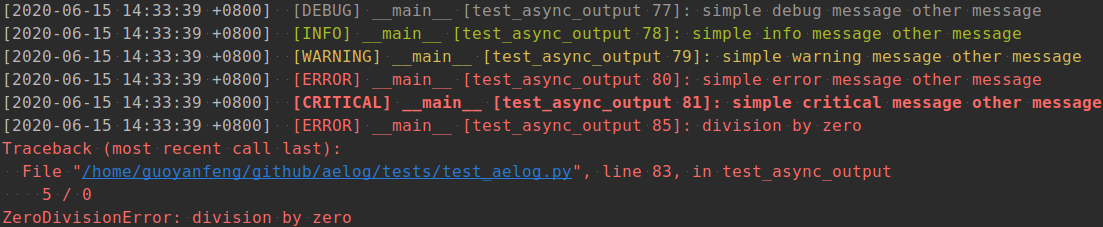
- Automatic output is greater than the error information to the 'test_error.log' file.
- Different levels of logging, different color, the color is cyan, green, yellow, red and 'bold_red,bg_white' in turn.
Raw data
{
"_id": null,
"home_page": "https://github.com/tinybees/aelog",
"name": "aelog",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "log,logging,colored,async,asynchronous,simple,rotating",
"author": "TinyBees",
"author_email": "a598824322@qq.com",
"download_url": "",
"platform": "",
"description": "# Aelog\nAn simple, async, full package name path, log rotating, different colored log library.\n\naelog aims to make using python log as simple as possible. as a result, it drastically \nsimplifies using python logging.\n\naelog's design objectives:\n\n- Make using python log as simple as possible.\n- Output log contains the full package name path.\n- Provide asynchronous log output function, at the same time, contains common log output.\n- Output according to the log level to mark the different colors separately.\n- Provide a log file rotating, automatic backup.\n- Output to the terminal and file, default output to the terminal, if you don't provide the log file path.\n\n# Installing aelog\n- ```pip install aelog```\n\n# init aelog \n```\nimport aelog \n\napp = Flask(__name__)\n\naelog.init_app(app)\n# or \naelog.init_app(aelog_access_file='aelog_access_file.log', aelog_error_file='aelog_error_file.log', \n aelog_console=False)\n```\n# aelog config\nList of configuration keys that the aelog extension recognizes:\n\n\n| configuration key | the meaning of the configuration key |\n| ------ | ------ |\n| AELOG_ACCESS_FILE | Access file path, default None. |\n| AELOG_ERROR_FILE | Error file path, default None. |\n| AELOG_CONSOLE | Whether it is output at the terminal, default false. |\n| AELOG_LEVEL | log level, default 'DEBUG'. |\n| AELOG_MAX_BYTES | Log file size, default 50M. |\n| AELOG_BACKUP_COUNT | Rotating file count, default 5.|\n\n# Usage\n### simple using, output log to terminal.\n```\nimport aelog\n\naelog.init_app(aelog_console=True)\n\ndef test_aelog_output_console():\n \"\"\"\n\n Args:\n\n Returns:\n\n \"\"\"\n aelog.debug(\"simple debug message\", \"other message\")\n aelog.info(\"simple info message\", \"other message\")\n aelog.warning(\"simple warning message\", \"other message\")\n aelog.error(\"simple error message\", \"other message\")\n aelog.critical(\"simple critical message\", \"other message\")\n try:\n 5 / 0\n except Exception as e:\n aelog.exception(e)\n```\nThis will output to the terminal. \n\n- Different levels of logging, different color, the color is cyan, green, yellow, red and 'bold_red,bg_white' in turn.\n\n### output log to file and terminal.\n```\nimport aelog\nfrom flask import Flask\n\napp = Flask(__name__)\n\naelog.init_app(app) # Output to the test.log file and terminal \n\ndef test_aelog_output_file():\n \"\"\"\n\n Args:\n\n Returns:\n\n \"\"\"\n aelog.debug(\"simple debug message\", \"other message\")\n aelog.info(\"simple info message\", \"other message\")\n aelog.warning(\"simple warning message\", \"other message\")\n aelog.error(\"simple error message\", \"other message\")\n aelog.critical(\"simple critical message\", \"other message\")\n try:\n 5 / 0\n except Exception as e:\n aelog.exception(e)\n```\nThis will output to the test.log file and terminal.\n\n- Automatic output is greater than the error information to the 'test_error.log' file.\n- Different levels of logging, different color, the color is cyan, green, yellow, red and 'bold_red,bg_white' in turn.\n\n### asynchronous output log to file and terminal.\n```\nimport asyncio\nimport aelog\nfrom sanic import Sanic\n\napp = Sanic(__name__)\n\naelog.init_aelog(app) # Output to the test.log file and terminal \n\nasync def test_async_output():\n await aelog.async_debug(\"simple debug message\", \"other message\")\n await aelog.async_info(\"simple info message\", \"other message\")\n await aelog.async_warning(\"simple warning message\", \"other message\")\n await aelog.async_error(\"simple error message\", \"other message\")\n await aelog.async_critical(\"simple critical message\", \"other message\")\n try:\n 5 / 0\n except Exception as e:\n await aelog.async_exception(e)\n\nif \"__name__\"==\"__main__\":\n loop = asyncio.get_event_loop()\n loop.run_until_complete(test_async_output())\n```\nThis will output to the test.log file and terminal.\n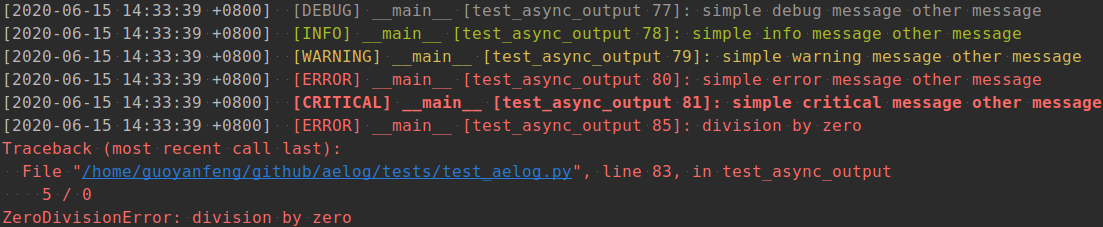\n- Automatic output is greater than the error information to the 'test_error.log' file. \n- Different levels of logging, different color, the color is cyan, green, yellow, red and 'bold_red,bg_white' in turn.\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "An simple, async, full package name path, log rotating, different colored log library.",
"version": "1.0.9",
"split_keywords": [
"log",
"logging",
"colored",
"async",
"asynchronous",
"simple",
"rotating"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "863674695587078f5101f2b7cefed205ace2ba29efcde85ddf6e7cd8c235a7d4",
"md5": "bd91c50850c13435903556e621817661",
"sha256": "8ac17de13b60256bebcd82cd11910c52a4ac7cfc90da300199df36c0a9f79d5f"
},
"downloads": -1,
"filename": "aelog-1.0.9-py3-none-any.whl",
"has_sig": false,
"md5_digest": "bd91c50850c13435903556e621817661",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 7510,
"upload_time": "2020-11-12T01:56:14",
"upload_time_iso_8601": "2020-11-12T01:56:14.959249Z",
"url": "https://files.pythonhosted.org/packages/86/36/74695587078f5101f2b7cefed205ace2ba29efcde85ddf6e7cd8c235a7d4/aelog-1.0.9-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2020-11-12 01:56:14",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "tinybees",
"github_project": "aelog",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [
{
"name": "colorlog",
"specs": [
[
">=",
"3.1.0"
]
]
}
],
"lcname": "aelog"
}