# Botasaurus Driver
Botasaurus Driver is a powerful Driver Automation Python library that offers the following benefits:
- It is really humane; it looks and works exactly like a real browser, allowing it to access any website.
- Compared to Selenium and Playwright, it is super fast to launch and use.
- The API is designed by and for web scrapers, and you will love it.
## Installation
```bash
pip install botasaurus-driver
```
## Bypassing Bot Detection: Code Example
```python
from botasaurus_driver import Driver
driver = Driver()
driver.google_get("https://www.g2.com/products/github/reviews.html?page=5&product_id=github", bypass_cloudflare=True)
driver.prompt()
heading = driver.get_text('.product-head__title [itemprop="name"]')
print(heading)
```
**Result**
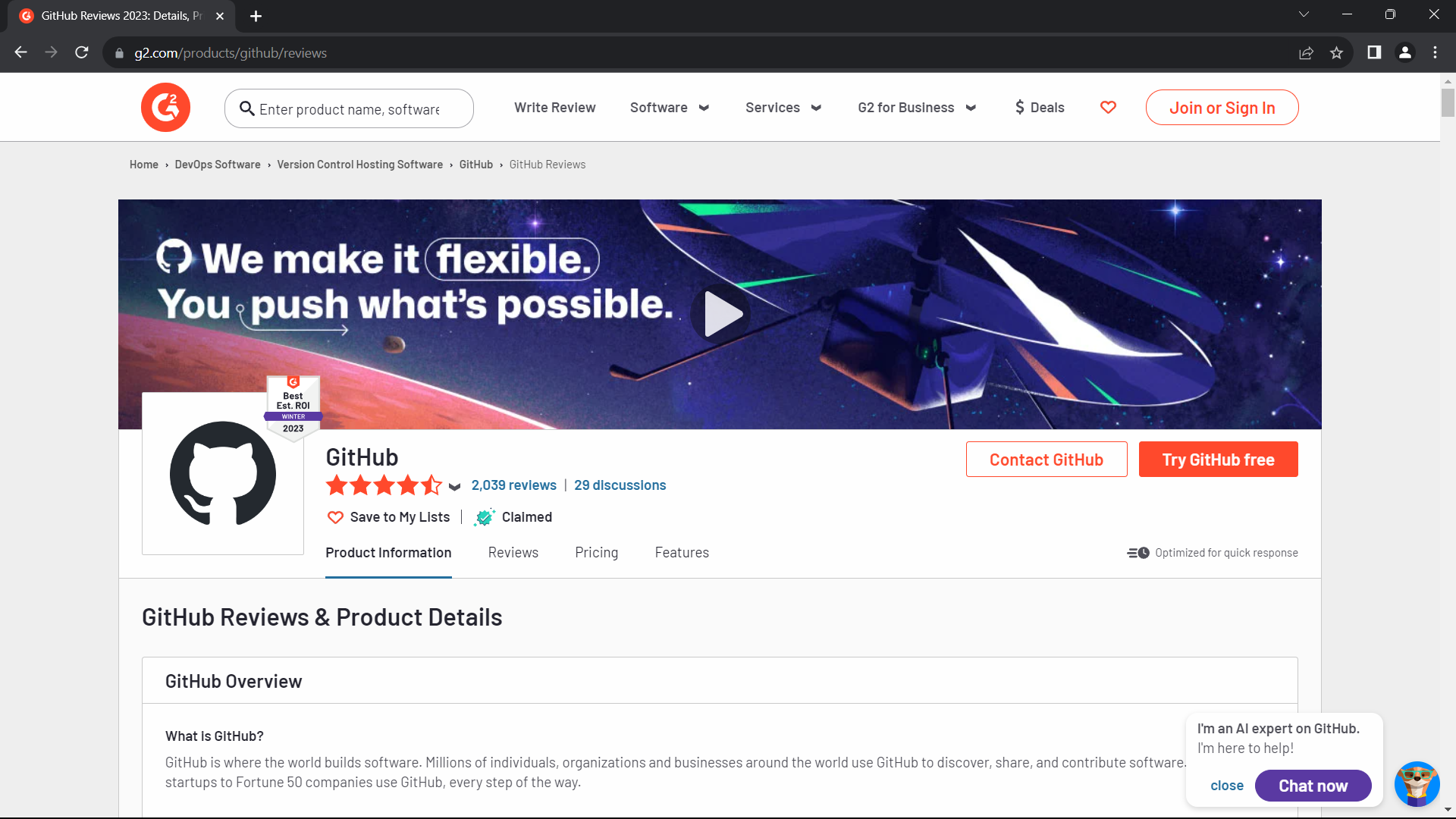
## API
Botasaurus Driver provides several handy methods for web automation tasks such as:
- Navigate to URLs:
```python
driver.get("https://www.example.com")
driver.google_get("https://www.example.com") # Use Google as the referer [Recommended]
driver.get_via("https://www.example.com", referer="https://duckduckgo.com/") # Use custom referer
driver.get_via_this_page("https://www.example.com") # Use current page as referer
```
- For finding elements:
```python
from botasaurus.browser import Wait
search_results = driver.select(".search-results", wait=Wait.SHORT) # Wait for up to 4 seconds for the element to be present, return None if not found
search_results = driver.wait_for_element(".search-results", wait=Wait.LONG) # Wait for up to 8 seconds for the element to be present, raise exception if not found
hello_mom = driver.get_element_with_exact_text("Hello Mom", wait=Wait.VERY_LONG) # Wait for up to 16 seconds for an element having the exact text "Hello Mom"
```
- Interact with elements:
```python
driver.type("input[name='username']", "john_doe") # Type into an input field
driver.click("button.submit") # Clicks an element
element = driver.select("button.submit")
element.click() # Click on an element
```
- Retrieve element properties:
```python
header_text = driver.get_text("h1") # Get text content
error_message = driver.get_element_containing_text("Error: Invalid input")
image_url = driver.select("img.logo").get_attribute("src") # Get attribute value
```
- Work with parent-child elements:
```python
parent_element = driver.select(".parent")
child_element = parent_element.select(".child")
child_element.click() # Click child element
```
- Execute JavaScript:
```python
result = driver.run_js("return document.title")
text_content = element.run_js("(el) => el.textContent")
```
- Working with iframes:
```python
driver.get("https://www.freecodecamp.org/news/using-entity-framework-core-with-mongodb/")
iframe = driver.get_iframe_by_link("www.youtube.com/embed")
# OR following works as well
# iframe = driver.select(".embed-wrapper iframe")
freecodecamp_youtube_subscribers_count = iframe.select(".ytp-title-expanded-subtitle").text
```
- Miscellaneous:
```python
form.type("input[name='password']", "secret_password") # Type into a form field
container.is_element_present(".button") # Check element presence
page_html = driver.page_html # Current page HTML
driver.select(".footer").scroll_into_view() # Scroll element into view
driver.close() # Close the browser
```
## Love It? [Star It ⭐!](https://github.com/omkarcloud/botasaurus-driver)
Become one of our amazing stargazers by giving us a star ⭐ on GitHub!
It's just one click, but it means the world to me.
[](https://github.com/omkarcloud/botasaurus-driver/stargazers)
## Made with ❤️ using [Botasaurus Web Scraping Framework](https://github.com/omkarcloud/botasaurus)
Raw data
{
"_id": null,
"home_page": "https://github.com/omkarcloud/botasaurus-driver",
"name": "botasaurus-driver",
"maintainer": "Chetan",
"docs_url": null,
"requires_python": ">=3.5",
"maintainer_email": "chetan@omkar.cloud",
"keywords": "webdriver, browser, captcha, web-scraping, selenium, navigator, python3, cloudflare, anti-delect, anti-bot, bot-detection, cloudflare-bypass, distil, anti-detection",
"author": "Chetan",
"author_email": "chetan@omkar.cloud",
"download_url": "https://files.pythonhosted.org/packages/38/0e/3ceb28980d397150c059413d02d1da79f0e95ffa4779f27341f0d2b4cea3/botasaurus_driver-4.0.66.tar.gz",
"platform": null,
"description": "# Botasaurus Driver\n\nBotasaurus Driver is a powerful Driver Automation Python library that offers the following benefits:\n\n\n- It is really humane; it looks and works exactly like a real browser, allowing it to access any website.\n- Compared to Selenium and Playwright, it is super fast to launch and use.\n- The API is designed by and for web scrapers, and you will love it.\n\n## Installation\n```bash\npip install botasaurus-driver\n```\n\n## Bypassing Bot Detection: Code Example\n\n```python\nfrom botasaurus_driver import Driver\n\ndriver = Driver()\ndriver.google_get(\"https://www.g2.com/products/github/reviews.html?page=5&product_id=github\", bypass_cloudflare=True)\ndriver.prompt()\nheading = driver.get_text('.product-head__title [itemprop=\"name\"]')\nprint(heading)\n```\n\n**Result**\n\n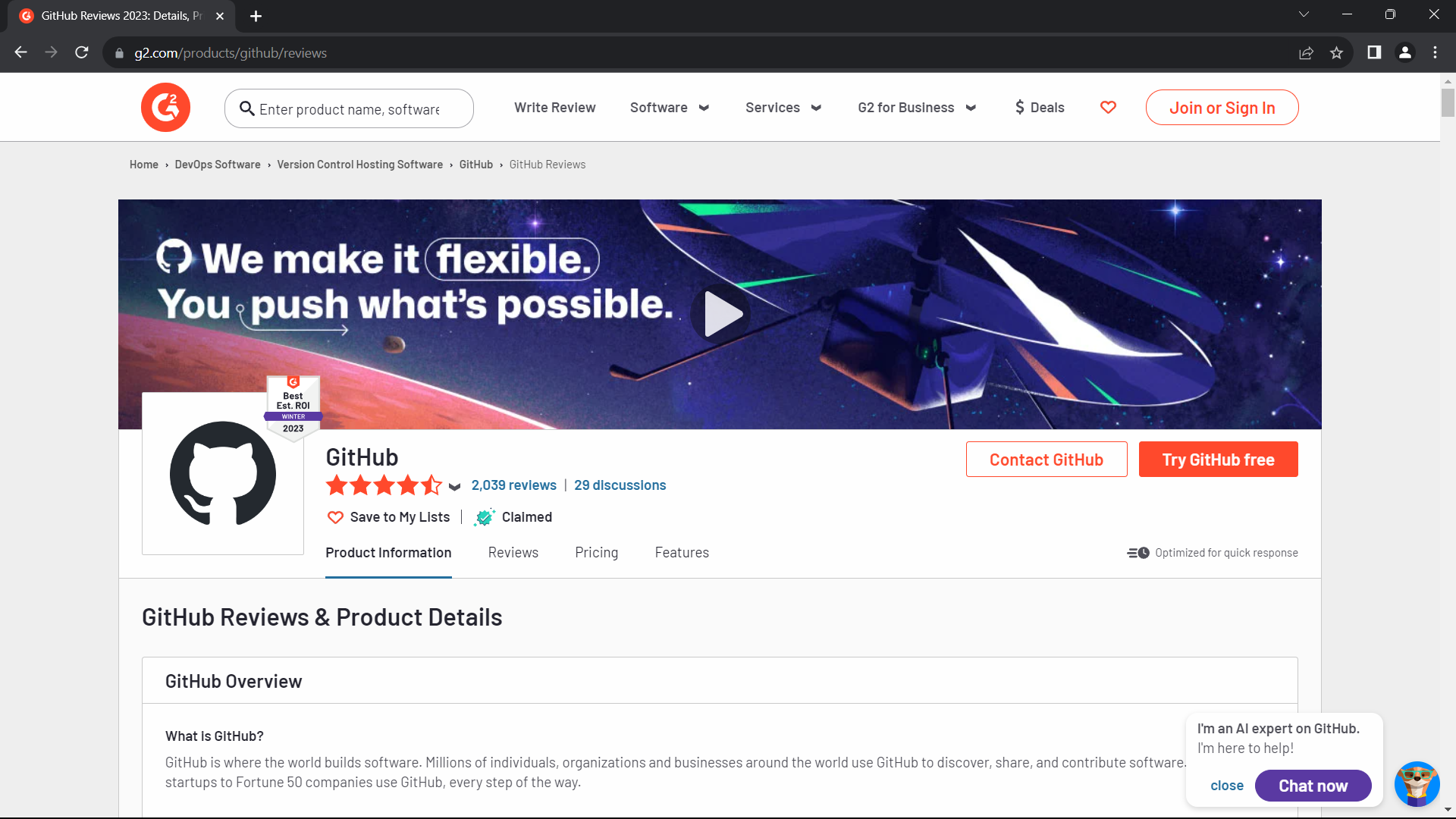\n\n## API\nBotasaurus Driver provides several handy methods for web automation tasks such as:\n\n- Navigate to URLs:\n ```python\n driver.get(\"https://www.example.com\")\n driver.google_get(\"https://www.example.com\") # Use Google as the referer [Recommended]\n driver.get_via(\"https://www.example.com\", referer=\"https://duckduckgo.com/\") # Use custom referer\n driver.get_via_this_page(\"https://www.example.com\") # Use current page as referer\n ```\n\n- For finding elements:\n ```python\n from botasaurus.browser import Wait\n search_results = driver.select(\".search-results\", wait=Wait.SHORT) # Wait for up to 4 seconds for the element to be present, return None if not found\n search_results = driver.wait_for_element(\".search-results\", wait=Wait.LONG) # Wait for up to 8 seconds for the element to be present, raise exception if not found\n hello_mom = driver.get_element_with_exact_text(\"Hello Mom\", wait=Wait.VERY_LONG) # Wait for up to 16 seconds for an element having the exact text \"Hello Mom\"\n ```\n\n- Interact with elements:\n ```python\n driver.type(\"input[name='username']\", \"john_doe\") # Type into an input field\n driver.click(\"button.submit\") # Clicks an element\n element = driver.select(\"button.submit\")\n element.click() # Click on an element\n ```\n\n- Retrieve element properties:\n ```python\n header_text = driver.get_text(\"h1\") # Get text content\n error_message = driver.get_element_containing_text(\"Error: Invalid input\")\n image_url = driver.select(\"img.logo\").get_attribute(\"src\") # Get attribute value\n ```\n\n- Work with parent-child elements:\n ```python\n parent_element = driver.select(\".parent\")\n child_element = parent_element.select(\".child\")\n child_element.click() # Click child element\n ```\n\n- Execute JavaScript:\n ```python\n result = driver.run_js(\"return document.title\")\n text_content = element.run_js(\"(el) => el.textContent\")\n ```\n\n- Working with iframes:\n ```python\n driver.get(\"https://www.freecodecamp.org/news/using-entity-framework-core-with-mongodb/\")\n iframe = driver.get_iframe_by_link(\"www.youtube.com/embed\") \n # OR following works as well\n # iframe = driver.select(\".embed-wrapper iframe\") \n freecodecamp_youtube_subscribers_count = iframe.select(\".ytp-title-expanded-subtitle\").text\n ```\n\n- Miscellaneous:\n ```python\n form.type(\"input[name='password']\", \"secret_password\") # Type into a form field\n container.is_element_present(\".button\") # Check element presence\n page_html = driver.page_html # Current page HTML\n driver.select(\".footer\").scroll_into_view() # Scroll element into view\n driver.close() # Close the browser\n ```\n\n## Love It? [Star It \u2b50!](https://github.com/omkarcloud/botasaurus-driver)\n\nBecome one of our amazing stargazers by giving us a star \u2b50 on GitHub!\n\nIt's just one click, but it means the world to me.\n\n[](https://github.com/omkarcloud/botasaurus-driver/stargazers)\n\n## Made with \u2764\ufe0f using [Botasaurus Web Scraping Framework](https://github.com/omkarcloud/botasaurus)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Super Fast, Super Anti-Detect, and Super Intuitive Web Driver",
"version": "4.0.66",
"project_urls": {
"Bug Reports": "https://github.com/omkarcloud/botasaurus-driver/issues",
"Homepage": "https://github.com/omkarcloud/botasaurus-driver",
"Source": "https://github.com/omkarcloud/botasaurus-driver"
},
"split_keywords": [
"webdriver",
" browser",
" captcha",
" web-scraping",
" selenium",
" navigator",
" python3",
" cloudflare",
" anti-delect",
" anti-bot",
" bot-detection",
" cloudflare-bypass",
" distil",
" anti-detection"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "380e3ceb28980d397150c059413d02d1da79f0e95ffa4779f27341f0d2b4cea3",
"md5": "2341a48838e5ece15ec8ac101b9be517",
"sha256": "af0d070846f7f816f1bcc58ddfb316ee6d166d9c5768b14ef3698ae49395e3e1"
},
"downloads": -1,
"filename": "botasaurus_driver-4.0.66.tar.gz",
"has_sig": false,
"md5_digest": "2341a48838e5ece15ec8ac101b9be517",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.5",
"size": 263941,
"upload_time": "2024-10-26T11:49:15",
"upload_time_iso_8601": "2024-10-26T11:49:15.121538Z",
"url": "https://files.pythonhosted.org/packages/38/0e/3ceb28980d397150c059413d02d1da79f0e95ffa4779f27341f0d2b4cea3/botasaurus_driver-4.0.66.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-26 11:49:15",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "omkarcloud",
"github_project": "botasaurus-driver",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "botasaurus-driver"
}