# The claydates Package
The claydates package serves to clean and restructure financial data retrieved from the Twelve Data API. Secondarily, it allows for the user to nicely plot time series data using numerous cleaning and restructuring methodologies. Additionally, it provides the user with the option to store information on data quality during usage, which may later provide insight into changes in data quality over time for certain tickers.
**The program is comprised of 4 main classes:**
```
1. SingleTickerProcessor
- Used for gathering and cleaning time series data.
- Determines where there might be any missing dates coming in from the API call.
- Also can be used for logging the quality of data received from the API call to a CSV file, to be reviewed at a later date.
- Also used to iteratively construct one or more time series objects of the SingleTickerProcessor class.
- It is the parent class of the SingleTickerPlotter class, which is the parent of the multiTickerPlotter class.
2. SingleTickerPlotter
- Used for plotting time series data.
- Also can be used for logging the quality of data received from the API call to a csv file, to be reviewed at a later date.
- It is also used to iteratively construct one or more time series objects of the SingleTickerPlotter class.
- It is the child class of SingleTickerProcessor, and the parent class of multiTickerPlotter.
3. MultiTickerProcessor
- Used for gathering and processing time series data for one or more ticker symbols.
- Creates a list of SingleTickerProcessor class objects, and then organizes data in accordance with the various arguments passed or not-passed to methods belonging to the SingleTickerProcessor class.
- Also can be used for logging the quality of data received from the API call to a CSV file, to be reviewed at a later date.
- It is the parent class of MultiTickerPlotter.
4. MultiTickerPlotter
- Used for gathering and processing time series data for one or more ticker symbols.
- Creates a list of SingleTickerPlotter class objects, and then organizes data in accordance with the various arguments passed or not passed to the various methods belonging to the SingleTickerPlotter class.
- Also can be used for logging the quality of data received from the API call to a CSV file, to be reviewed at a later date.
- It is the child class of MultiTickerProcessor and SingleTickerPlotter.
```
## Directory Tree
```
claydatesRootDirectory
│ README.md
│ setup.py
│ requirements.txt
│ LICENSE
│ .gitignore
│
└───claydates
│ │ __init__.py
│ │
│ └───processors
│ │ __init__.py
│ │ singleTickerProcessor.py
│ │ multiTickerProcessor.py
│ │
│ └───plotters
│ │ __init__.py
│ │ singleTickerPlotter.py
│ │ multiTickerPlotter.py
│ │
│ └───datasets
│ │ currencyPairs.txt
│ │ exampleSet.csv
│ │ key.txt
│ │ missingDataLog.csv
│ │
│ └───examples
│ __init__.py
│ examplesSingleTickerProcessor.py
│ examplesMultiTickerProcessor.py
│ examplesSingleTickerPlotter.py
│ examplesMultiTickerPlotter.py
│
└───tests
__init__.py
testSingleTickerProcessor.py
testMultiTickerProcessor.py
testSingleTickerPlotter.py
testMultiTickerPlotter.py
```
## Quick Usage Examples
**For additional descriptions of arguments and methods, refer to the examples folder, which further details each quick usage example provided here.**
### SingleTickerProcessor
```
1.) from claydates import SingleTickerProcessor
1a.) singleTickerProcessor = SingleTickerProcessor('QQQ', '1min', 1170)
1b.) singleTickerPlotter = SingleTickerPlotter(tickerSymbol = 'QQQ', tickInterval = '1min', numberOfUnits = 1170,
percentageChange = True, timeZone = 'America/New_York', quoteCurrency = 'USD',
logMissingData = True, mockResponse = False, spacingFactor = 14,
seriesType = 'Close', scalerRange = (0,1), binningFactor = 10
figureSize = [14.275,9.525], labelsize = 16, color = 'black')
```
```
2.) from claydates import SingleTickerProcessor
2a.) singleTickerPlotter.datetimeHandler('missingDataIncludedInFrame')
2b.) singleTickerPlotter.datetimeHandler('missingPercentage')
2c.) singleTickerPlotter.datetimeHandler('lagTime')
```
```
3.) from claydates import SingleTickerProcessor
3a.) singleTickerProcessor.unalteredFrameGetter()
```
### MultiTickerProcessor
```
1.) from claydates import MultiTickerProcessor
1a.) multiTickerProcessor = MultiTickerProcessor(['QQQ','SPY','IWM','DIA'], '1min', 390)
1b.) multiTickerProcessor = MultiTickerProcessor(tickerSymbols = ['QQQ','SPY','IWM','DIA'], tickInterval = '1min', numberOfUnits = 1170,
percentageChange = True, timeZone = 'America/New_York', quoteCurrency = 'USD',
logMissingData = True, mockResponse = False)
```
```
2.) from claydates import MultiTickerProcessor
2a.) multiTickerProcessor.dateMatcher(dropMissing = True)
```
```
3.) from claydates import MultiTickerProcessor
3a.) multiTickerProcessor.unalteredFrames(dataType = 'pandas')
```
```
4.) from claydates import MultiTickerProcessor
4a.) multiTickerProcessor.missingUnitsIncluded(dataType = 'pandas', interpolationMethod = None, matchDates = False)
```
```
5.) from claydates import MultiTickerProcessor
5a.) multiTickerProcessor.missingUnitsExcluded(dataType = 'pandas', matchDates = True)
```
```
6.) from claydates import MultiTickerProcessor
6a.) multiTickerProcessor.missingPercentages(onlyPrint = True)
```
### SingleTickerPlotter
```
1.) from claydates import SingleTickerPlotter
1a.) singleTickerPlotter = SingleTickerPlotter('QQQ', '1min', 1170)
1b.) singleTickerPlotter = SingleTickerPlotter(tickerSymbol = 'QQQ', tickInterval = '1min', numberOfUnits = 1170,
percentageChange = True, timeZone = 'America/New_York', quoteCurrency = 'USD',
logMissingData = True, mockResponse = False, spacingFactor = 14,
seriesType = 'Close', scalerRange = (0,1), binningFactor = 10,
figureSize = [14.275,9.525], labelsize = 16, color = 'black')
```
```
2.) from claydates import SingleTickerPlotter
2a.) singleTickerPlotter.datetimeHandler('missingDataIncludedInFrame')
2b.) singleTickerPlotter.datetimeHandler('missingDataIncludedInFrame')
2c.) singleTickerPlotter.datetimeHandler('missingDataIncludedInFrame')
```
```
3.) from claydates import SingleTickerPlotter
3a.) singleTickerPlotter.unalteredFrameGetter()
```
```
4.) from claydates import SingleTickerPlotter
4a.) singleTickerPlotter.standardSinglePlot()
```
```
5.) from claydates import SingleTickerPlotter
5a.) singleTickerPlotter.missingDataPlot()
```
```
6.) from claydates import SingleTickerPlotter
6a.) singleTickerPlotter.interpolatedSinglePlot(methodology = 'linear')
6b.) singleTickerPlotter.interpolatedSinglePlot(methodology = 'cubic')
```
```
7.) from claydates import SingleTickerPlotter
7a.) singleTickerPlotter.profileProcessor(numberOfBins = 10, methodology = 'count', interpolation = 'linear')
```
```
8.) from claydates import SingleTickerPlotter
8a.) singleTickerPlotter.singleProfilePlot(seriesType = 'standard', binningType = 'standard', methodology = 'price',
numberOfBins = None, scaledX = True, scaledY = False, interpolation = None)
```
```
9.) from claydates import SingleTickerPlotter
9a.) singleTickerPlotter.externalWindowSinglePlot()
```
```
10.) from claydates import SingleTickerPlotter
10a.) singleTickerPlotter.liveSinglePlot(numberOfUpdates = 14400, interactiveExternalWindow = False, secondsToSleep = 55)
```
### MultiTickerPlotter
```
1.) from claydates import MultiTickerPlotter
1a.) multiTickerPlotter = MultiTickerPlotter(['QQQ','SPY','IWM','DIA'], '1min', 390)
1b.) multiTickerPlotter = MultiTickerPlotter(tickerSymbols = ['QQQ','SPY','IWM','DIA'], tickInterval = '1min', numberOfUnits = 1170,
percentageChange = True, timeZone = 'America/New_York', quoteCurrency = 'USD',
logMissingData = True, mockResponse = False, spacingFactor = 14,
seriesType = 'Close', scalerRange = (0,1), binningFactor = 10,
figureSize = [14.275,9.525], labelsize = 16, color = 'black')
```
```
2.) from claydates import MultiTickerPlotter
2a.) multiTickerPlotter.standardMultiPlot(method = multiTickerPlotter.missingUnitsExcluded, matchDates = True, interpolationMethod = None,
interactiveExternalWindow = False, scaled = True, plotTitle = 'Example Plot')
```
```
3.) from claydates import MultiTickerPlotter
3a.) multiTickerPlotter.cyclePlot(method = multiTickerPlotter.missingUnitsExcluded, matchDates = True, interpolationMethod = None,
interactiveExternalWindow = False, scaled = True, secondsToPauseFor = 15)
```
```
4.) from claydates import MultiTickerPlotter
4a.) multiTickerPlotter.profileCyclerPlot('standard','standard', methodology = 'price', numberOfBins = None,
scaledX = True, scaledY = True, interpolation = None)
```
```
5.) from claydates import MultiTickerPlotter
5a.) multiTickerPlotter.multipleExternalWindowsPlot(method = multiTickerPlotter.missingUnitsExcluded, matchDates = True, interpolationMethod = None,
interactiveExternalWindow = False, scaled = True)
```
```
6.) from claydates import MultiTickerPlotter
6a.) multiTickerPlotter.liveMultiPlot(method = multiTickerPlotter.missingUnitsExcluded, matchDates = True, interpolationMethod = None,
interactiveExternalWindow = False, scaled = True, numberOfUpdates = 14400, secondsToSleepFor = 55)
```
## History
### version 1.0.6.
* Something went wrong with the datasets folder in versions 1.0.4. and 1.0.5. Fixed this.
* December, 2022.
* [https://pypi.org/project/claydates/1.0.6/](https://pypi.org/project/claydates/1.0.6/)
### version 1.0.5.
* Removed Table of Contents.
* December, 2022.
* [https://pypi.org/project/claydates/1.0.5/](https://pypi.org/project/claydates/1.0.5/)
### version 1.0.4.
* Added Table of Contents.
* Fixed typo in singleTickerProcessor.py.
* December, 2022.
* [https://pypi.org/project/claydates/1.0.4/](https://pypi.org/project/claydates/1.0.4/)
### version 1.0.3.
* Fixed typo in README.md.
* December, 2022.
* [https://pypi.org/project/claydates/1.0.3/](https://pypi.org/project/claydates/1.0.3/)
### version 1.0.2.
* Added datasets folder to install.
* December, 2022.
* [https://pypi.org/project/claydates/1.0.2/](https://pypi.org/project/claydates/1.0.2/)
### version 1.0.1.
* Fixed installation issue.
* December, 2022.
* [https://pypi.org/project/claydates/1.0.1/](https://pypi.org/project/claydates/1.0.1/)
### version 1.0.0.
* Developed and published.
* December, 2022.
* [https://pypi.org/project/claydates/1.0.0/](https://pypi.org/project/claydates/1.0.0/)
## Gallery
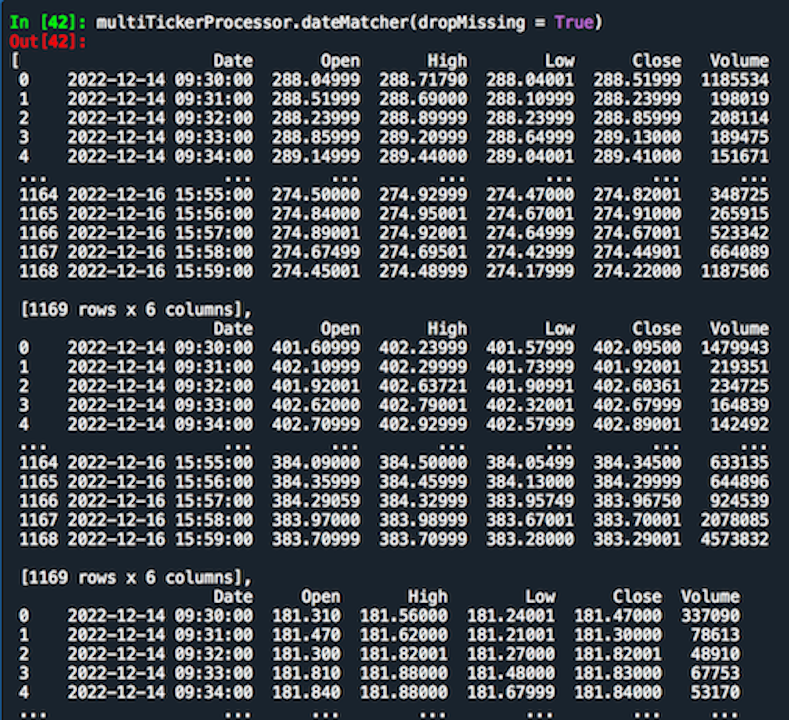
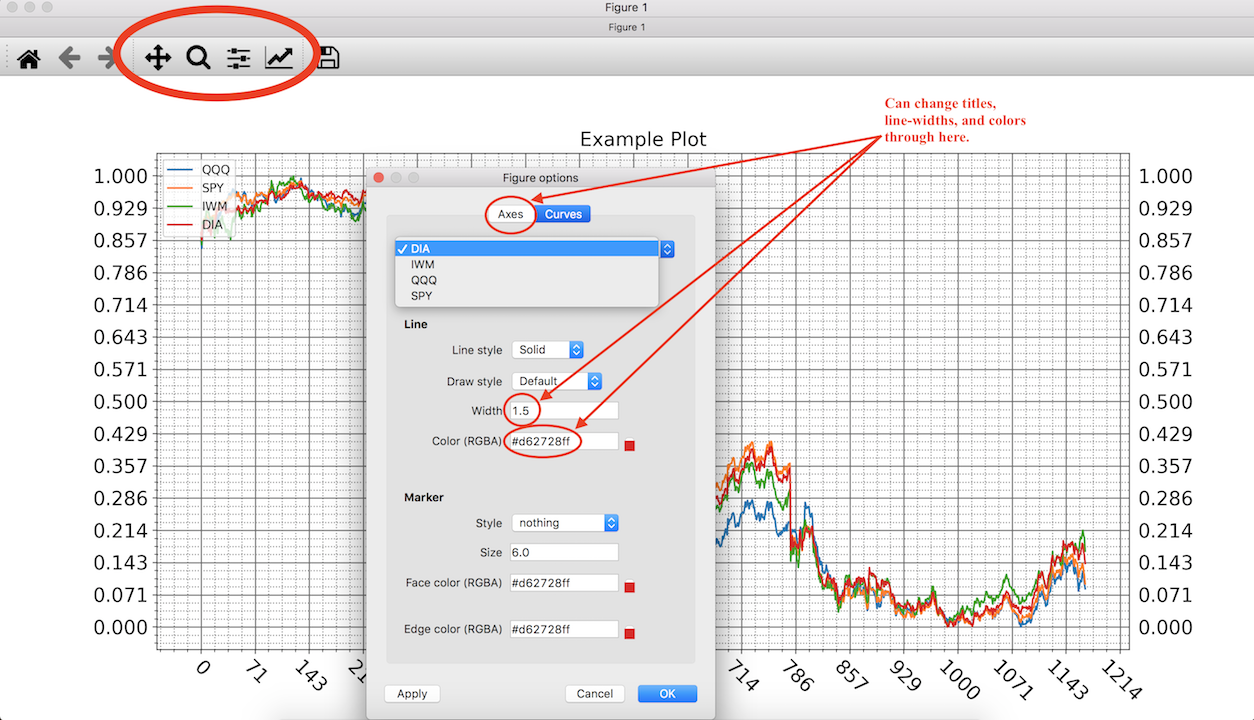
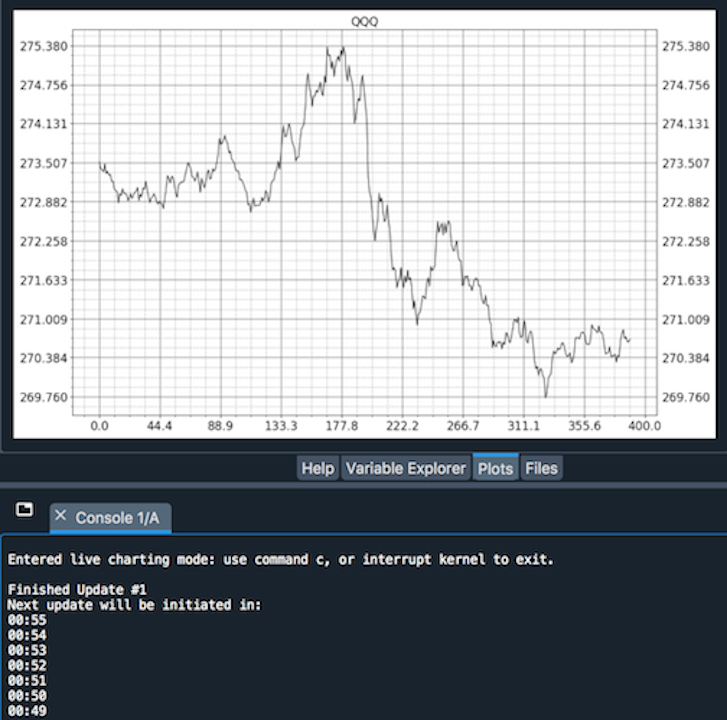
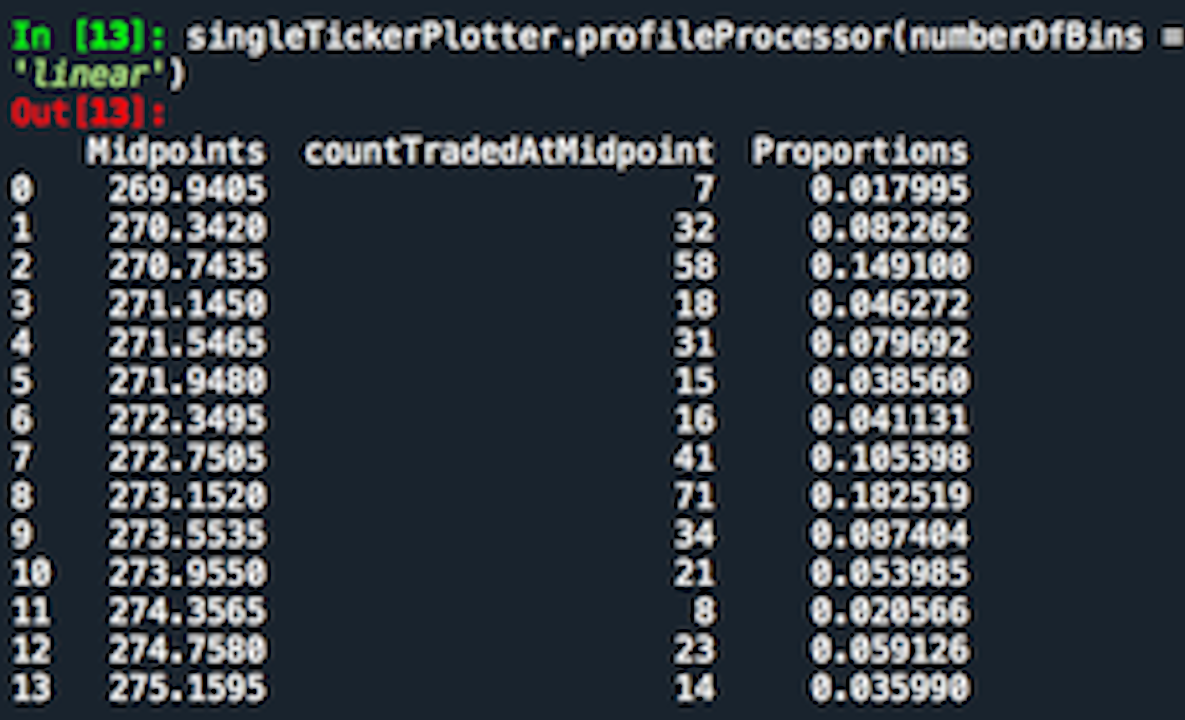
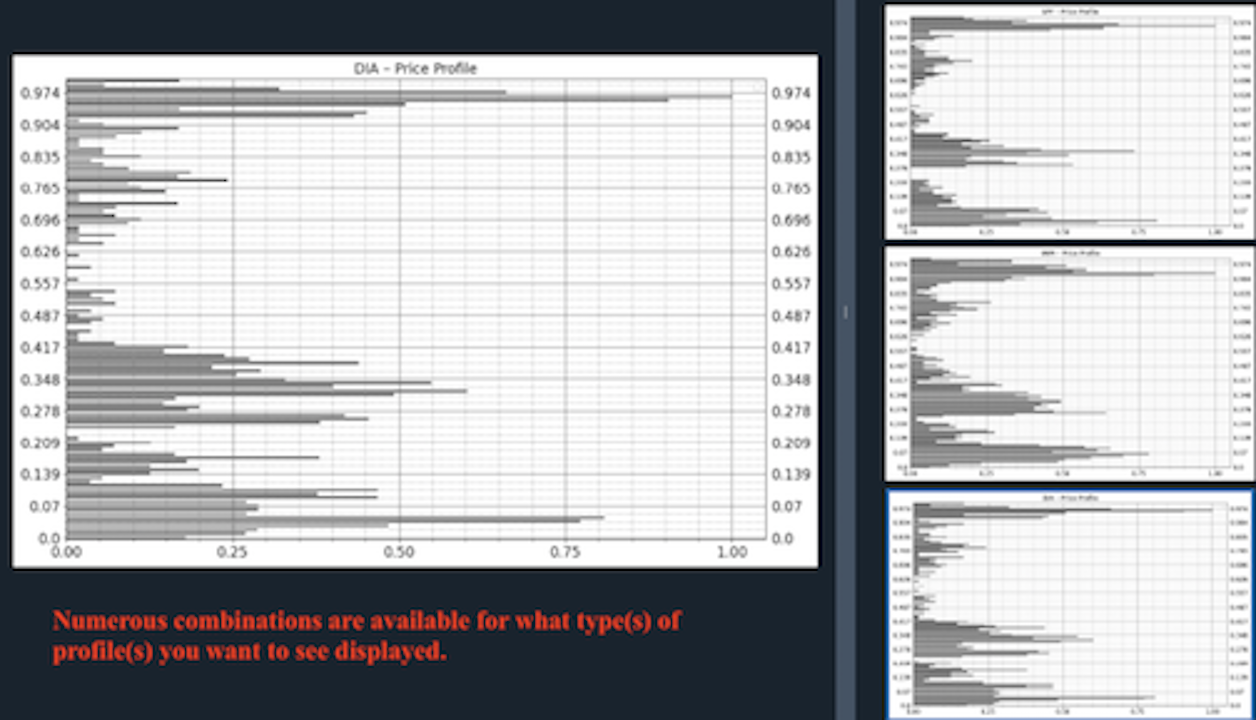
<br/>
Raw data
{
"_id": null,
"home_page": "https://github.com/ClaytonDuffin/claydates",
"name": "claydates",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "twelvedata API,restructuring,cleaning,plotting,financial data",
"author": "Clayton Duffin",
"author_email": "clayduffin@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/b7/c0/00b443552deec669619cc8f74682e9476b44df2a7d412030433e6619d074/claydates-1.0.6.tar.gz",
"platform": null,
"description": "\ufeff# The claydates Package\n\n\nThe claydates package serves to clean and restructure financial data retrieved from the Twelve Data API. Secondarily, it allows for the user to nicely plot time series data using numerous cleaning and restructuring methodologies. Additionally, it provides the user with the option to store information on data quality during usage, which may later provide insight into changes in data quality over time for certain tickers.\n\n\n**The program is comprised of 4 main classes:**\n```\n 1. SingleTickerProcessor\n - Used for gathering and cleaning time series data. \n - Determines where there might be any missing dates coming in from the API call.\n - Also can be used for logging the quality of data received from the API call to a CSV file, to be reviewed at a later date.\n - Also used to iteratively construct one or more time series objects of the SingleTickerProcessor class.\n - It is the parent class of the SingleTickerPlotter class, which is the parent of the multiTickerPlotter class.\n \n 2. SingleTickerPlotter\n - Used for plotting time series data. \n - Also can be used for logging the quality of data received from the API call to a csv file, to be reviewed at a later date.\n - It is also used to iteratively construct one or more time series objects of the SingleTickerPlotter class.\n - It is the child class of SingleTickerProcessor, and the parent class of multiTickerPlotter.\n \n 3. MultiTickerProcessor\n - Used for gathering and processing time series data for one or more ticker symbols. \n - Creates a list of SingleTickerProcessor class objects, and then organizes data in accordance with the various arguments passed or not-passed to methods belonging to the SingleTickerProcessor class.\n - Also can be used for logging the quality of data received from the API call to a CSV file, to be reviewed at a later date.\n - It is the parent class of MultiTickerPlotter.\n \n 4. MultiTickerPlotter\n - Used for gathering and processing time series data for one or more ticker symbols. \n - Creates a list of SingleTickerPlotter class objects, and then organizes data in accordance with the various arguments passed or not passed to the various methods belonging to the SingleTickerPlotter class.\n - Also can be used for logging the quality of data received from the API call to a CSV file, to be reviewed at a later date.\n - It is the child class of MultiTickerProcessor and SingleTickerPlotter.\n```\n\n## Directory Tree \n```\n claydatesRootDirectory\n \u2502 README.md\n \u2502 setup.py\n \u2502 requirements.txt\n \u2502 LICENSE\n \u2502 .gitignore\n \u2502\n \u2514\u2500\u2500\u2500claydates\n \u2502 \u2502 __init__.py\n \u2502 \u2502\n \u2502 \u2514\u2500\u2500\u2500processors\n \u2502 \u2502 __init__.py\n \u2502 \u2502 singleTickerProcessor.py\n \u2502 \u2502 multiTickerProcessor.py\n \u2502 \u2502\n \u2502 \u2514\u2500\u2500\u2500plotters\n \u2502 \u2502 __init__.py\n \u2502 \u2502 singleTickerPlotter.py\n \u2502 \u2502 multiTickerPlotter.py\n \u2502 \u2502\n \u2502 \u2514\u2500\u2500\u2500datasets\n \u2502 \u2502 currencyPairs.txt\n \u2502 \u2502 exampleSet.csv\n \u2502 \u2502 key.txt\n \u2502 \u2502 missingDataLog.csv\n \u2502 \u2502\n \u2502 \u2514\u2500\u2500\u2500examples\n \u2502 __init__.py\n \u2502 examplesSingleTickerProcessor.py\n \u2502 examplesMultiTickerProcessor.py\n \u2502 examplesSingleTickerPlotter.py\n \u2502 examplesMultiTickerPlotter.py\n \u2502\n \u2514\u2500\u2500\u2500tests\n __init__.py\n testSingleTickerProcessor.py\n testMultiTickerProcessor.py\n testSingleTickerPlotter.py\n testMultiTickerPlotter.py\n```\n\n## Quick Usage Examples\n\n**For additional descriptions of arguments and methods, refer to the examples folder, which further details each quick usage example provided here.**\n\n### SingleTickerProcessor\n```\n1.) from claydates import SingleTickerProcessor\n\n 1a.) singleTickerProcessor = SingleTickerProcessor('QQQ', '1min', 1170)\n 1b.) singleTickerPlotter = SingleTickerPlotter(tickerSymbol = 'QQQ', tickInterval = '1min', numberOfUnits = 1170,\n percentageChange = True, timeZone = 'America/New_York', quoteCurrency = 'USD',\n logMissingData = True, mockResponse = False, spacingFactor = 14, \n seriesType = 'Close', scalerRange = (0,1), binningFactor = 10\n figureSize = [14.275,9.525], labelsize = 16, color = 'black')\n```\n```\n2.) from claydates import SingleTickerProcessor\n\n 2a.) singleTickerPlotter.datetimeHandler('missingDataIncludedInFrame') \n 2b.) singleTickerPlotter.datetimeHandler('missingPercentage') \n 2c.) singleTickerPlotter.datetimeHandler('lagTime') \n```\n```\n3.) from claydates import SingleTickerProcessor\n\n 3a.) singleTickerProcessor.unalteredFrameGetter()\n```\n### MultiTickerProcessor\n```\n1.) from claydates import MultiTickerProcessor\n \n 1a.) multiTickerProcessor = MultiTickerProcessor(['QQQ','SPY','IWM','DIA'], '1min', 390)\n 1b.) multiTickerProcessor = MultiTickerProcessor(tickerSymbols = ['QQQ','SPY','IWM','DIA'], tickInterval = '1min', numberOfUnits = 1170,\n percentageChange = True, timeZone = 'America/New_York', quoteCurrency = 'USD',\n logMissingData = True, mockResponse = False)\n```\n```\n2.) from claydates import MultiTickerProcessor\n\n 2a.) multiTickerProcessor.dateMatcher(dropMissing = True)\n```\n```\n3.) from claydates import MultiTickerProcessor\n\n 3a.) multiTickerProcessor.unalteredFrames(dataType = 'pandas')\n```\n```\n4.) from claydates import MultiTickerProcessor\n\n 4a.) multiTickerProcessor.missingUnitsIncluded(dataType = 'pandas', interpolationMethod = None, matchDates = False)\n```\n```\n5.) from claydates import MultiTickerProcessor\n\n 5a.) multiTickerProcessor.missingUnitsExcluded(dataType = 'pandas', matchDates = True)\n```\n```\n6.) from claydates import MultiTickerProcessor\n\n 6a.) multiTickerProcessor.missingPercentages(onlyPrint = True)\n```\n### SingleTickerPlotter\n```\n1.) from claydates import SingleTickerPlotter\n\n 1a.) singleTickerPlotter = SingleTickerPlotter('QQQ', '1min', 1170)\n 1b.) singleTickerPlotter = SingleTickerPlotter(tickerSymbol = 'QQQ', tickInterval = '1min', numberOfUnits = 1170,\n percentageChange = True, timeZone = 'America/New_York', quoteCurrency = 'USD',\n logMissingData = True, mockResponse = False, spacingFactor = 14, \n seriesType = 'Close', scalerRange = (0,1), binningFactor = 10,\n figureSize = [14.275,9.525], labelsize = 16, color = 'black')\n```\n```\n2.) from claydates import SingleTickerPlotter\n\n 2a.) singleTickerPlotter.datetimeHandler('missingDataIncludedInFrame') \n 2b.) singleTickerPlotter.datetimeHandler('missingDataIncludedInFrame') \n 2c.) singleTickerPlotter.datetimeHandler('missingDataIncludedInFrame') \n```\n```\n3.) from claydates import SingleTickerPlotter\n\n 3a.) singleTickerPlotter.unalteredFrameGetter()\n```\n```\n4.) from claydates import SingleTickerPlotter\n\n 4a.) singleTickerPlotter.standardSinglePlot()\n```\n```\n5.) from claydates import SingleTickerPlotter\n\n 5a.) singleTickerPlotter.missingDataPlot()\n```\n```\n6.) from claydates import SingleTickerPlotter\n\n 6a.) singleTickerPlotter.interpolatedSinglePlot(methodology = 'linear')\n 6b.) singleTickerPlotter.interpolatedSinglePlot(methodology = 'cubic')\n\n```\n```\n7.) from claydates import SingleTickerPlotter\n\n 7a.) singleTickerPlotter.profileProcessor(numberOfBins = 10, methodology = 'count', interpolation = 'linear')\n\n```\n```\n8.) from claydates import SingleTickerPlotter\n\n 8a.) singleTickerPlotter.singleProfilePlot(seriesType = 'standard', binningType = 'standard', methodology = 'price',\n numberOfBins = None, scaledX = True, scaledY = False, interpolation = None)\n```\n```\n9.) from claydates import SingleTickerPlotter\n\n 9a.) singleTickerPlotter.externalWindowSinglePlot()\n```\n```\n10.) from claydates import SingleTickerPlotter\n\n 10a.) singleTickerPlotter.liveSinglePlot(numberOfUpdates = 14400, interactiveExternalWindow = False, secondsToSleep = 55)\n```\n### MultiTickerPlotter\n```\n1.) from claydates import MultiTickerPlotter\n \n 1a.) multiTickerPlotter = MultiTickerPlotter(['QQQ','SPY','IWM','DIA'], '1min', 390)\n 1b.) multiTickerPlotter = MultiTickerPlotter(tickerSymbols = ['QQQ','SPY','IWM','DIA'], tickInterval = '1min', numberOfUnits = 1170,\n percentageChange = True, timeZone = 'America/New_York', quoteCurrency = 'USD',\n logMissingData = True, mockResponse = False, spacingFactor = 14, \n seriesType = 'Close', scalerRange = (0,1), binningFactor = 10,\n figureSize = [14.275,9.525], labelsize = 16, color = 'black')\n```\n```\n2.) from claydates import MultiTickerPlotter\n\n 2a.) multiTickerPlotter.standardMultiPlot(method = multiTickerPlotter.missingUnitsExcluded, matchDates = True, interpolationMethod = None,\n interactiveExternalWindow = False, scaled = True, plotTitle = 'Example Plot')\n```\n```\n3.) from claydates import MultiTickerPlotter\n\n 3a.) multiTickerPlotter.cyclePlot(method = multiTickerPlotter.missingUnitsExcluded, matchDates = True, interpolationMethod = None, \n interactiveExternalWindow = False, scaled = True, secondsToPauseFor = 15)\n\n```\n```\n4.) from claydates import MultiTickerPlotter\n\n 4a.) multiTickerPlotter.profileCyclerPlot('standard','standard', methodology = 'price', numberOfBins = None,\n scaledX = True, scaledY = True, interpolation = None)\n```\n```\n5.) from claydates import MultiTickerPlotter\n\n 5a.) multiTickerPlotter.multipleExternalWindowsPlot(method = multiTickerPlotter.missingUnitsExcluded, matchDates = True, interpolationMethod = None,\n interactiveExternalWindow = False, scaled = True)\n\n```\n```\n6.) from claydates import MultiTickerPlotter\n\n 6a.) multiTickerPlotter.liveMultiPlot(method = multiTickerPlotter.missingUnitsExcluded, matchDates = True, interpolationMethod = None,\n interactiveExternalWindow = False, scaled = True, numberOfUpdates = 14400, secondsToSleepFor = 55)\n```\n## History \n\n### version 1.0.6.\n* Something went wrong with the datasets folder in versions 1.0.4. and 1.0.5. Fixed this.\n* December, 2022.\n* [https://pypi.org/project/claydates/1.0.6/](https://pypi.org/project/claydates/1.0.6/)\n\n### version 1.0.5.\n* Removed Table of Contents.\n* December, 2022.\n* [https://pypi.org/project/claydates/1.0.5/](https://pypi.org/project/claydates/1.0.5/)\n\n### version 1.0.4.\n* Added Table of Contents.\n* Fixed typo in singleTickerProcessor.py.\n* December, 2022.\n* [https://pypi.org/project/claydates/1.0.4/](https://pypi.org/project/claydates/1.0.4/)\n\n### version 1.0.3.\n* Fixed typo in README.md.\n* December, 2022.\n* [https://pypi.org/project/claydates/1.0.3/](https://pypi.org/project/claydates/1.0.3/)\n\n### version 1.0.2.\n* Added datasets folder to install.\n* December, 2022.\n* [https://pypi.org/project/claydates/1.0.2/](https://pypi.org/project/claydates/1.0.2/)\n\n### version 1.0.1.\n* Fixed installation issue.\n* December, 2022.\n* [https://pypi.org/project/claydates/1.0.1/](https://pypi.org/project/claydates/1.0.1/)\n\n### version 1.0.0.\n* Developed and published.\n* December, 2022.\n* [https://pypi.org/project/claydates/1.0.0/](https://pypi.org/project/claydates/1.0.0/)\n\n\n## Gallery \n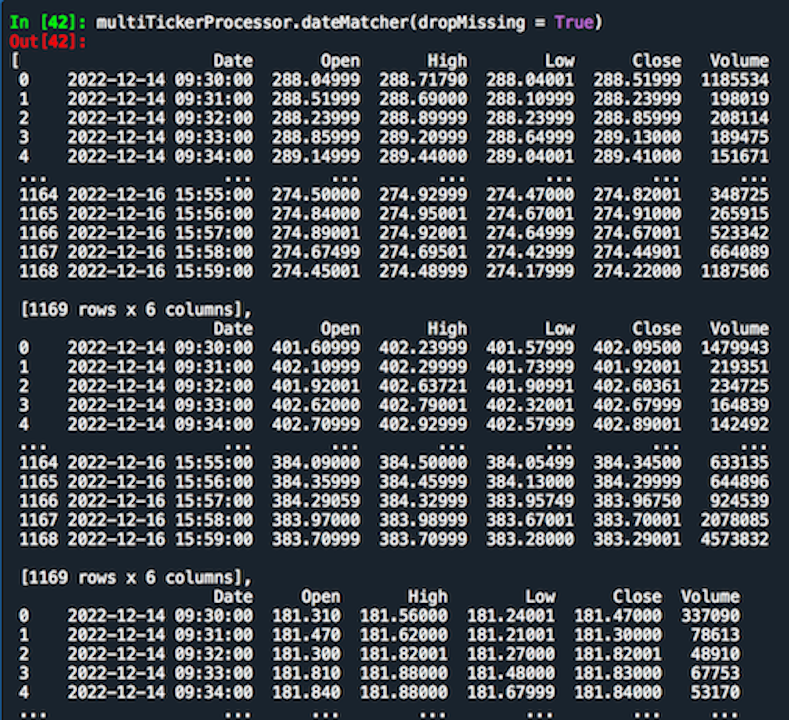\n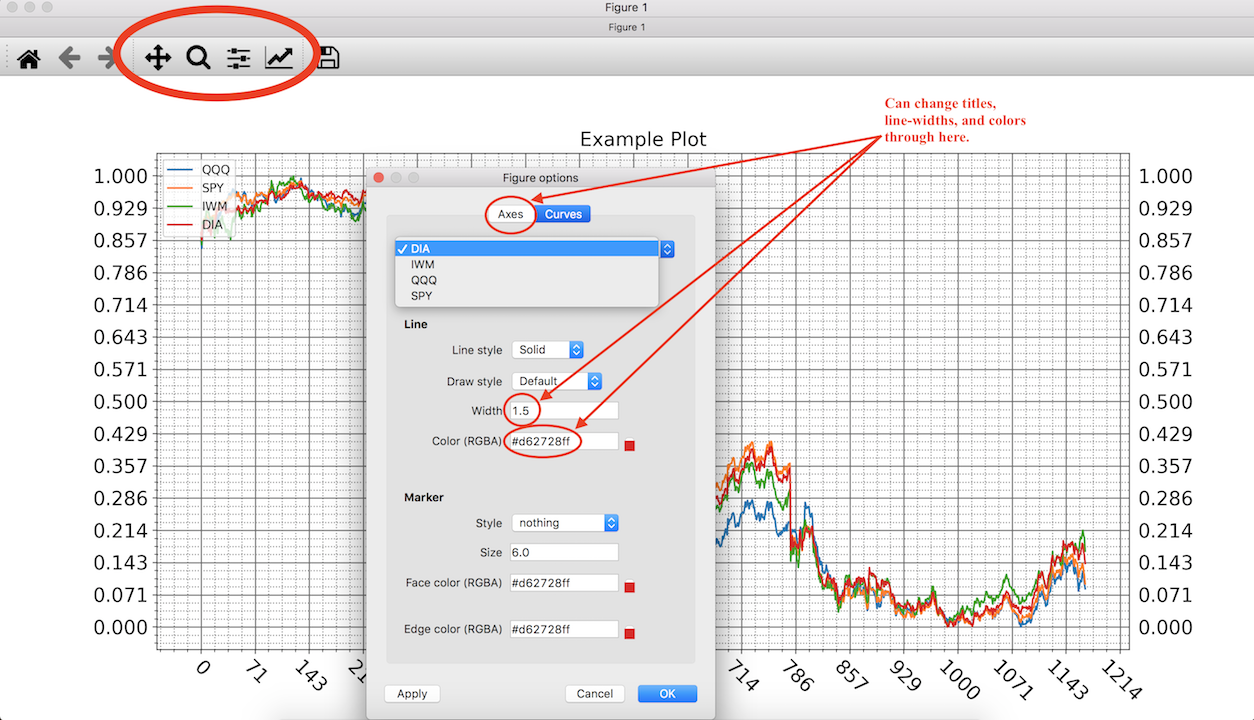\n\n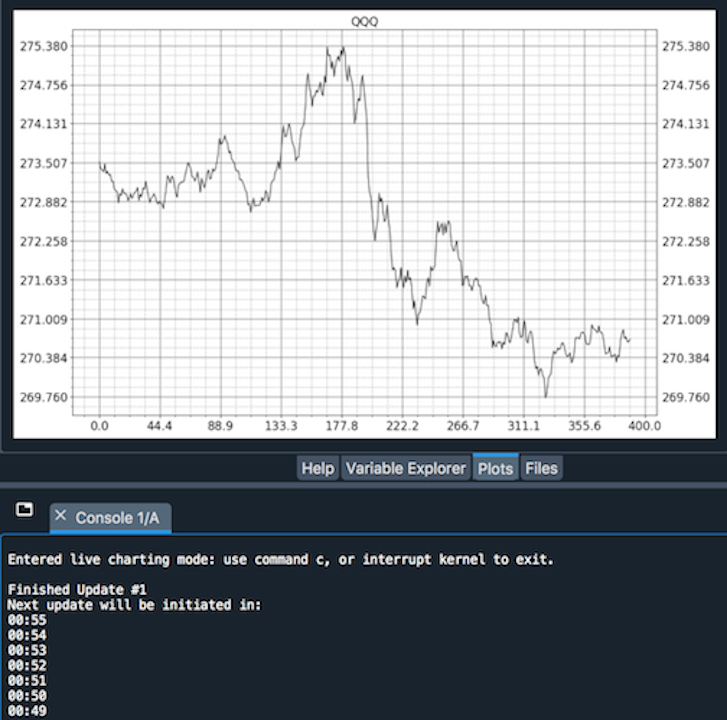\n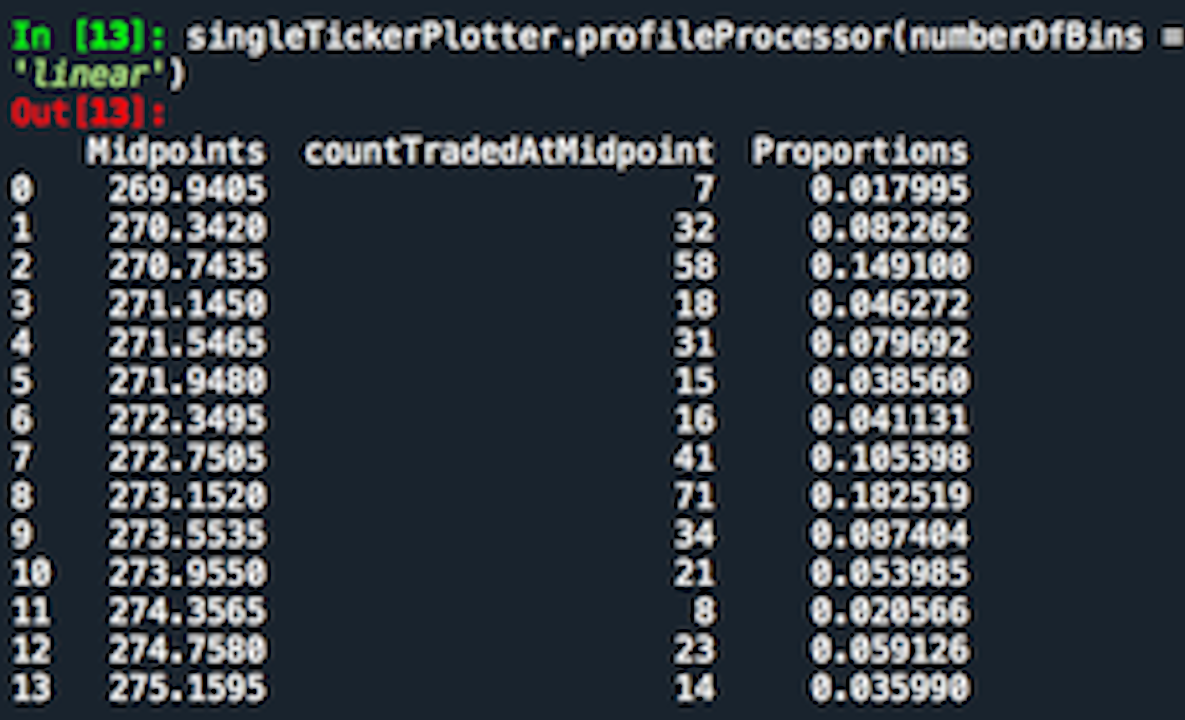\n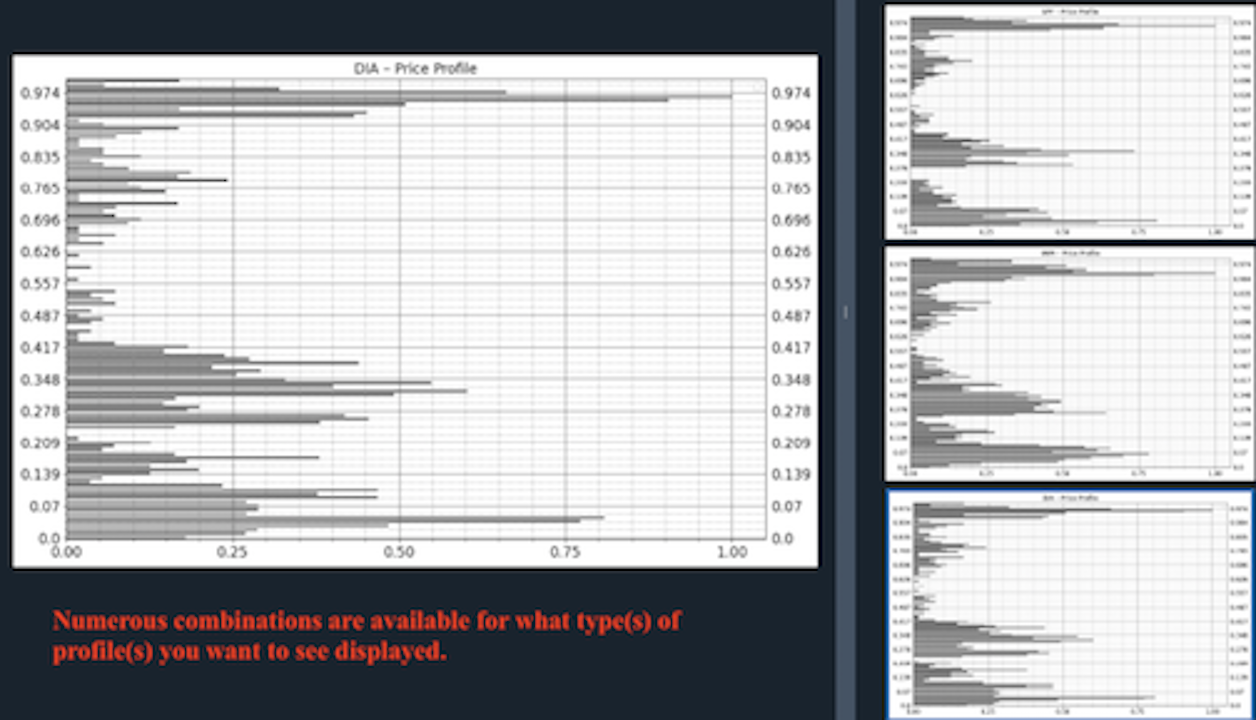\n\n<br/>\n",
"bugtrack_url": null,
"license": "",
"summary": "Package used for cleaning, restructuring, logging, and plotting of financial data retrieved from the Twelve Data API.",
"version": "1.0.6",
"split_keywords": [
"twelvedata api",
"restructuring",
"cleaning",
"plotting",
"financial data"
],
"urls": [
{
"comment_text": "",
"digests": {
"md5": "f051bd5e051450a17ca596cc9a8b5fd7",
"sha256": "184304587f7f020d18a164b99454efaa3cdf63ebcc10400ea9330edd7f75e21d"
},
"downloads": -1,
"filename": "claydates-1.0.6-py3-none-any.whl",
"has_sig": false,
"md5_digest": "f051bd5e051450a17ca596cc9a8b5fd7",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 37753,
"upload_time": "2022-12-21T23:27:10",
"upload_time_iso_8601": "2022-12-21T23:27:10.798942Z",
"url": "https://files.pythonhosted.org/packages/00/e2/77b84f620151a5bb953130858f2b393b7fe5a674b8fd3c583b7a63305589/claydates-1.0.6-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "d95cef21070081b314743ca360ce2808",
"sha256": "d3fae21034753aca514f5745040411d79f7af0f93f722d3f978f22b7ab1d3340"
},
"downloads": -1,
"filename": "claydates-1.0.6.tar.gz",
"has_sig": false,
"md5_digest": "d95cef21070081b314743ca360ce2808",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 28177,
"upload_time": "2022-12-21T23:27:12",
"upload_time_iso_8601": "2022-12-21T23:27:12.132704Z",
"url": "https://files.pythonhosted.org/packages/b7/c0/00b443552deec669619cc8f74682e9476b44df2a7d412030433e6619d074/claydates-1.0.6.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2022-12-21 23:27:12",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "ClaytonDuffin",
"github_project": "claydates",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "claydates"
}