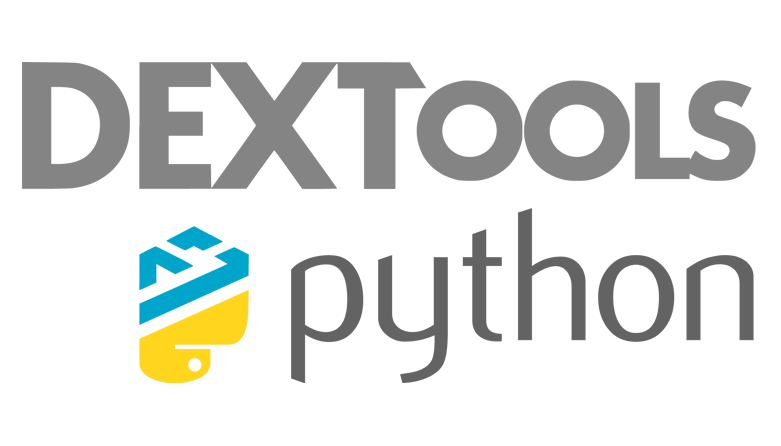
# DEXTools Python
[](https://github.com/alb2001/dextools-python/actions/workflows/python-app.yml)
[](https://pepy.tech/project/dextools-python)
[](https://opensource.org/licenses/MIT)
[](https://pypi.org/project/dextools-python/)
A simple Python API wrapper for DEXTools.
Supports [Dextools API v1](https://api.dextools.io/docs) and [Dextools API v2](https://developer.dextools.io/docs/start)
1. [Installation](#installation)
2. [Obtaining API Key](#obtaining-api-key)
3. [Getting Started](#getting-started)
1. [Version 1](#version-1)
2. [Version 2](#version-2)
1. [Available plans - Setting your plan](#available-plans---setting-your-plan)
4. [Version 1 Queries](#version-1-queries)
1. [Get pairs of a token](#get-pairs-of-a-token)
2. [Get token details](#get-token-details)
3. [Get chain list](#get-chain-list)
4. [Get exchange list](#get-exchange-list)
5. [Version 2 Queries](#version-2-queries)
1. [Blockchain](#blockchain)
1. [Get blockchain info](#get-blockchain-info)
2. [Get blockchains sorted by default settings](#get-blockchains-sorted-by-default-settings)
3. [Get blockchains sorted by default settings and descending order](#get-blockchains-sorted-by-default-settings-and-descending-order)
2. [Exchange](#exchange)
1. [Get dex factory info](#get-dex-factory-info)
2. [Get dexes on a specific chain](#get-dexes-on-a-specific-chain)
3. [Get dexes on a specific chain sorted by name and descending order](#get-dexes-on-a-specific-chain-sorted-by-name-and-descending-order)
3. [Pool](#pool)
1. [Get pool info](#get-pool-info)
2. [Get pool liquidity](#get-pool-liquidity)
3. [Get pool score](#get-pool-score)
4. [Get pool price](#get-pool-price)
5. [Get pools](#get-pools)
6. [Get pools sorted by `creationBlock` and descending order and providing block numbers instead](#get-pools-sorted-by-creationblock-and-descending-order-and-providing-block-numbers-instead)
4. [Token](#token)
1. [Get token](#get-token)
2. [Get token locks](#get-token-locks)
3. [Get token score](#get-token-score)
4. [Get token info](#get-token-info)
5. [Get token price](#get-token-price)
6. [Get tokens](#get-tokens)
7. [Get tokens sorted by `creationBlock` and descending order and providing block numbers instead in descending order](#get-tokens-sorted-by-creationblock-and-descending-order-and-providing-block-numbers-instead-in-descending-order)
8. [Get tokens sorted by `socialsInfoUpdated` and descending order and datetimes in descending order](#get-tokens-sorted-by-socialsinfoupdated-and-descending-order-and-datetimes-in-descending-order)
9. [Get token pools](#get-token-pools)
10. [Get token pools sorted by `creationBlock` and descending order and providing block numbers instead in descending order](#get-token-pools-sorted-by-creationblock-and-descending-order-and-providing-block-numbers-instead-in-descending-order)
5. [Rankings](#rankings)
1. [Get hot pools](#get-hot-pools)
2. [Get gainers](#get-gainers)
3. [Get losers](#get-losers)
6. [Page and PageSize arguments](#page-and-pagesize-arguments)
7. [Asynchronous support with asyncio](#asynchronous-support-with-asyncio)
8. [Examples](#examples)
9. [Testing](#testing)
10. [Supported Blockchains](#supported-blockchains)
11. [Authors](#authors)
12. [More information](#more-information)
## Installation
```
pip install dextools-python
```
## Obtaining API Key
To obtain an API key, head to the [Developer Portal](https://developer.dextools.io/) and choose your plan.
## Getting Started
There are 2 versions of the Dextools API. [Dextools API v1](https://api.dextools.io/docs) and [Dextools API v2](https://developer.dextools.io/docs/start)
### Version 1
To get started, import the package, and initiate a `DextoolsAPI` instance object by passing your API key:
```
from dextools_python import DextoolsAPI
dextools = DextoolsAPI(api_key)
```
You can also pass an optional user agent:
```
dextools = DextoolsAPI(api_key, useragent="User-Agent")
```
### Version 2
To get started, import the package, and initiate a `DextoolsAPIV2` instance object by passing your API key and your plan:
```
from dextools_python import DextoolsAPIV2
dextools = DextoolsAPIV2(api_key, plan="trial")
```
You can also pass an optional user agent:
```
dextools = DextoolsAPIV2(api_key, useragent="User-Agent", plan="trial")
```
If you don't specify any plan when instantiating the object, it will default to "partner" plan
#### Available plans - Setting your plan
You can setup your plan when setting the object instance by providing the `plan` argument in the constructor. If no `plan` is specified, it will default to "partner" plan
To set your plan after the object is created, you can use the `set_plan("your_plan")` method
```
dextools.set_plan("standard")
```
Available values: `"free"`, `"trial"`, `"standard"`, `"advanced"`, `"pro"`, and `"partner"`
## Version 1 Queries
Below are a set of queries supported by the [Dextools API v1](https://api.dextools.io/docs). All data is returned as a Python dictionary for easy data handling.
### Get pairs of a token
To get the pairs of a token, pass a `chain id` and a `pair address`:
```
pair = dextools.get_pair("ether", "0xa29fe6ef9592b5d408cca961d0fb9b1faf497d6d")
print(pair)
```
### Get token details
To get token details, pass a `chain id`, and a `token address`:
```
token = dextools.get_token("ether", "0xfb7b4564402e5500db5bb6d63ae671302777c75a")
print(token)
```
You can also pass the `page` and `pageSize` parameters:
```
token = dextools.get_token("ether", "0xfb7b4564402e5500db5bb6d63ae671302777c75a", 1, 50)
print(token)
```
### Get chain list
To get the chain list:
```
chain_list = dextools.get_chain_list()
print(chain_list)
```
You can also pass the `page` and `pageSize` parameters:
```
chain_list = dextools.get_chain_list(1, 50)
print(chain_list)
```
### Get exchange list
To get the exchange list, pass a `chain id`:
```
exchange_list = dextools.get_exchange_list("ether")
print(exchange_list)
```
You can also pass the `page` and `pageSize` parameters:
```
exchange_list = dextools.get_exchange_list("ether", 1, 50)
print(exchange_list)
```
## Version 2 Queries
Below are a set of queries supported by the [Dextools API v2](https://developer.dextools.io/docs/start). All data is returned as a Python dictionary for easy data handling.
### Blockchain
#### Get blockchain info
```
blockchain = dextools.get_blockchain("ether")
print(blockchain)
```
#### Get blockchains sorted by default settings
```
blockchains = dextools.get_blockchains()
print(blockchains)
```
#### Get blockchains sorted by default settings and descending order
```
blockchains = dextools.get_blockchains(sort="name", order="desc")
print(blockchains)
```
### Exchange
#### Get dex factory info
```
factory = dextools.get_dex_factory_info("ether", "0x5C69bEe701ef814a2B6a3EDD4B1652CB9cc5aA6f")
print(factory)
```
#### Get dexes on a specific chain
```
dexes = dextools.get_dexes("ether")
print(dexes)
```
#### Get dexes on a specific chain sorted by name and descending order
```
dexes = dextools.get_dexes("ether", sort="creationBlock", order="desc")
print(dexes)
```
### Pool
#### Get pool info
```
pool = dextools.get_pool("ether", "0xa29fe6ef9592b5d408cca961d0fb9b1faf497d6d")
print(pool)
```
#### Get pool liquidity
```
pool_liquidity = dextools.get_pool_liquidity("ether", "0xa29fe6ef9592b5d408cca961d0fb9b1faf497d6d")
print(pool_liquidity)
```
#### Get pool score
```
pool_score = dextools.get_pool_score("ether", "0xa29fe6ef9592b5d408cca961d0fb9b1faf497d6d")
print(pool_score)
```
#### Get pool price
```
pool_price = dextools.get_pool_price("ether", "0xa29fe6ef9592b5d408cca961d0fb9b1faf497d6d")
print(pool_price)
```
#### Get pools
```
pools = dextools.get_pools("ether", from_="2023-11-14T19:00:00", to="2023-11-14T23:00:00")
print(pools)
```
#### Get pools sorted by `creationBlock` and descending order and providing block numbers instead
```
pools = dextools.get_pools("ether", from_="12755070", to="12755071", sort="creationBlock", order="desc")
print(pools)
```
### Token
#### Get token
```
token = dextools.get_token("ether", "0xfb7b4564402e5500db5bb6d63ae671302777c75a")
print(token)
```
#### Get token locks
```
token_locks = dextools.get_token_locks("ether", "0xfb7b4564402e5500db5bb6d63ae671302777c75a")
print(token_locks)
```
#### Get token score
```
token_score = dextools.get_token_score("ether", "0xfb7b4564402e5500db5bb6d63ae671302777c75a")
print(token_score)
```
#### Get token info
```
token_info = dextools.get_token_info("ether", "0xfb7b4564402e5500db5bb6d63ae671302777c75a")
print(token_info)
```
#### Get token price
```
token_price = dextools.get_token_price("ether", "0xfb7b4564402e5500db5bb6d63ae671302777c75a")
print(token_price)
```
#### Get tokens
```
tokens = dextools.get_tokens("ether", from_="2023-11-14T19:00:00", to="2023-11-14T23:00:00")
print(tokens)
```
#### Get tokens sorted by `creationBlock` and descending order and providing block numbers instead in descending order
```
tokens = dextools.get_tokens("ether", from_="18570000", to="18570500", sort="creationBlock", order="desc")
print(tokens)
```
#### Get tokens sorted by `socialsInfoUpdated` and descending order and datetimes in descending order
```
tokens = dextools.get_tokens("ether", from_="2023-11-14T19:00:00", to="2023-11-14T23:00:00", sort="socialsInfoUpdated", order="desc")
print(tokens)
```
#### Get token pools
```
token_pools = dextools.get_token_pools("ether", "0xfb7b4564402e5500db5bb6d63ae671302777c75a", from_="2023-11-14T19:00:00", to="2023-11-14T23:00:00")
print(token_pools)
```
#### Get token pools sorted by `creationBlock` and descending order and providing block numbers instead in descending order
```
token_pools = dextools.get_token_pools("ether", "0xfb7b4564402e5500db5bb6d63ae671302777c75a", from_="18570000", to="18570500", sort="creationBlock", order="desc")
print(token_pools)
```
### Rankings
#### Get hot pools
```
hot_pools = dextools.get_ranking_hotpools("ether")
print(hot_pools)
```
#### Get gainers
```
gainers = dextools.get_ranking_gainers("ether")
print(gainers)
```
#### Get losers
```
losers = dextools.get_ranking_losers("ether")
print(losers)
```
## Page and PageSize arguments
Some methods support the `page` and `pageSize` arguments. Check out the API documentation for more information.
## Asynchronous support with asyncio
Asynchronous support has been added through [asyncio](https://docs.python.org/3/library/asyncio.html).
You can initiate an `AsyncDextoolsAPI` instance for API version 1, or an `AsyncDextoolsAPIV2` instance for API version 2.
Through a context manager (session will close automatically when the context manager ends):
```
import asyncio
from dextools_python import AsyncDextoolsAPIV2
api_key = "YOUR_API_KEY"
async def main():
async with AsyncDextoolsAPIV2(api_key, plan="partner") as dextools:
response = await dextools.get_blockchains()
print(response)
asyncio.run(main())
```
Creating an instance and then closing the session explicitly at the end:
```
import asyncio
from dextools_python import AsyncDextoolsAPIV2
api_key = "YOUR_API_KEY"
async def main():
dextools = AsyncDextoolsAPIV2(api_key, plan="partner")
response = await dextools.get_blockchains()
print(response)
await dextools.close()
asyncio.run(main())
```
## Examples
Check out the `examples` folder for some synchronous and asynchronous example scripts.
## Testing
A set of tests have been included inside `tests` folder. You will need to set an environment variable as `DextoolsAPIKey` using your API key.
## Supported Blockchains
Dextools adds support for new blockchains from time to time. `dextools.get_blockchains()` to get a list of supported blockchains and their IDs
## Authors
* [alb2001](https://github.com/alb2001)
## More information
* [dextools-python on PyPI](https://pypi.org/project/dextools-python)
* [DEXTools](https://www.dextools.io)
* [Dextools API v1](https://api.dextools.io/docs)
* [Dextools API v2](https://developer.dextools.io/docs/start)
* [Developer Portal](https://developer.dextools.io/)
* [asyncio](https://docs.python.org/3/library/asyncio.html)
Raw data
{
"_id": null,
"home_page": "https://github.com/alb2001/dextools-python",
"name": "dextools-python",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8,<4.0",
"maintainer_email": "",
"keywords": "dextools,api,wrapper,blockchain,trading,exchange,uniswap",
"author": "alb2001",
"author_email": "alb2001@outlook.es",
"download_url": "https://files.pythonhosted.org/packages/05/d0/a71392864b7e13de0afcfbf1025557a8d45c85d64d0a003df187117b435a/dextools_python-0.3.1.tar.gz",
"platform": null,
"description": "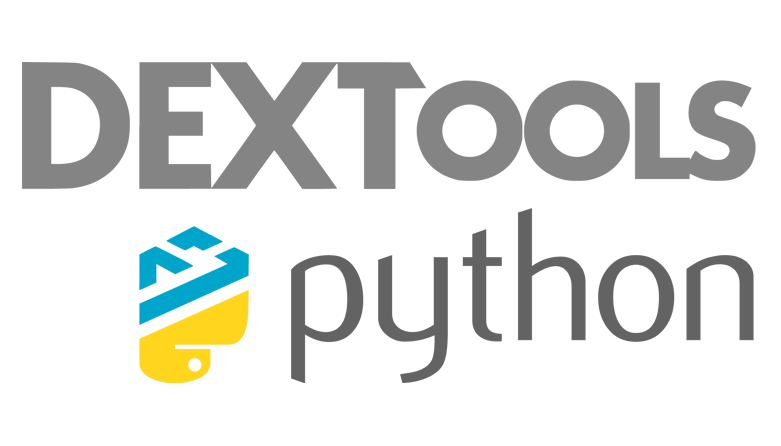\n\n# DEXTools Python\n[](https://github.com/alb2001/dextools-python/actions/workflows/python-app.yml)\n[](https://pepy.tech/project/dextools-python)\n[](https://opensource.org/licenses/MIT)\n[](https://pypi.org/project/dextools-python/)\n\nA simple Python API wrapper for DEXTools.\nSupports [Dextools API v1](https://api.dextools.io/docs) and [Dextools API v2](https://developer.dextools.io/docs/start)\n\n1. [Installation](#installation)\n2. [Obtaining API Key](#obtaining-api-key)\n3. [Getting Started](#getting-started)\n 1. [Version 1](#version-1)\n 2. [Version 2](#version-2)\n 1. [Available plans - Setting your plan](#available-plans---setting-your-plan)\n4. [Version 1 Queries](#version-1-queries)\n 1. [Get pairs of a token](#get-pairs-of-a-token)\n 2. [Get token details](#get-token-details)\n 3. [Get chain list](#get-chain-list)\n 4. [Get exchange list](#get-exchange-list)\n5. [Version 2 Queries](#version-2-queries)\n 1. [Blockchain](#blockchain)\n 1. [Get blockchain info](#get-blockchain-info)\n 2. [Get blockchains sorted by default settings](#get-blockchains-sorted-by-default-settings)\n 3. [Get blockchains sorted by default settings and descending order](#get-blockchains-sorted-by-default-settings-and-descending-order)\n 2. [Exchange](#exchange)\n 1. [Get dex factory info](#get-dex-factory-info)\n 2. [Get dexes on a specific chain](#get-dexes-on-a-specific-chain)\n 3. [Get dexes on a specific chain sorted by name and descending order](#get-dexes-on-a-specific-chain-sorted-by-name-and-descending-order)\n 3. [Pool](#pool)\n 1. [Get pool info](#get-pool-info)\n 2. [Get pool liquidity](#get-pool-liquidity)\n 3. [Get pool score](#get-pool-score)\n 4. [Get pool price](#get-pool-price)\n 5. [Get pools](#get-pools)\n 6. [Get pools sorted by `creationBlock` and descending order and providing block numbers instead](#get-pools-sorted-by-creationblock-and-descending-order-and-providing-block-numbers-instead)\n 4. [Token](#token)\n 1. [Get token](#get-token)\n 2. [Get token locks](#get-token-locks)\n 3. [Get token score](#get-token-score)\n 4. [Get token info](#get-token-info)\n 5. [Get token price](#get-token-price)\n 6. [Get tokens](#get-tokens)\n 7. [Get tokens sorted by `creationBlock` and descending order and providing block numbers instead in descending order](#get-tokens-sorted-by-creationblock-and-descending-order-and-providing-block-numbers-instead-in-descending-order)\n 8. [Get tokens sorted by `socialsInfoUpdated` and descending order and datetimes in descending order](#get-tokens-sorted-by-socialsinfoupdated-and-descending-order-and-datetimes-in-descending-order)\n 9. [Get token pools](#get-token-pools)\n 10. [Get token pools sorted by `creationBlock` and descending order and providing block numbers instead in descending order](#get-token-pools-sorted-by-creationblock-and-descending-order-and-providing-block-numbers-instead-in-descending-order)\n 5. [Rankings](#rankings)\n 1. [Get hot pools](#get-hot-pools)\n 2. [Get gainers](#get-gainers)\n 3. [Get losers](#get-losers)\n6. [Page and PageSize arguments](#page-and-pagesize-arguments)\n7. [Asynchronous support with asyncio](#asynchronous-support-with-asyncio)\n8. [Examples](#examples)\n9. [Testing](#testing)\n10. [Supported Blockchains](#supported-blockchains)\n11. [Authors](#authors)\n12. [More information](#more-information)\n\n\n## Installation\n\n```\npip install dextools-python\n```\n\n## Obtaining API Key\nTo obtain an API key, head to the [Developer Portal](https://developer.dextools.io/) and choose your plan.\n\n\n## Getting Started\nThere are 2 versions of the Dextools API. [Dextools API v1](https://api.dextools.io/docs) and [Dextools API v2](https://developer.dextools.io/docs/start)\n\n### Version 1\nTo get started, import the package, and initiate a `DextoolsAPI` instance object by passing your API key:\n```\nfrom dextools_python import DextoolsAPI\ndextools = DextoolsAPI(api_key)\n```\n\nYou can also pass an optional user agent:\n```\ndextools = DextoolsAPI(api_key, useragent=\"User-Agent\")\n```\n\n### Version 2\nTo get started, import the package, and initiate a `DextoolsAPIV2` instance object by passing your API key and your plan:\n```\nfrom dextools_python import DextoolsAPIV2\ndextools = DextoolsAPIV2(api_key, plan=\"trial\")\n```\n\nYou can also pass an optional user agent:\n```\ndextools = DextoolsAPIV2(api_key, useragent=\"User-Agent\", plan=\"trial\")\n```\n\nIf you don't specify any plan when instantiating the object, it will default to \"partner\" plan\n\n#### Available plans - Setting your plan\nYou can setup your plan when setting the object instance by providing the `plan` argument in the constructor. If no `plan` is specified, it will default to \"partner\" plan\n\nTo set your plan after the object is created, you can use the `set_plan(\"your_plan\")` method\n```\ndextools.set_plan(\"standard\")\n```\n\nAvailable values: `\"free\"`, `\"trial\"`, `\"standard\"`, `\"advanced\"`, `\"pro\"`, and `\"partner\"`\n\n\n## Version 1 Queries\nBelow are a set of queries supported by the [Dextools API v1](https://api.dextools.io/docs). All data is returned as a Python dictionary for easy data handling.\n\n### Get pairs of a token\nTo get the pairs of a token, pass a `chain id` and a `pair address`:\n```\npair = dextools.get_pair(\"ether\", \"0xa29fe6ef9592b5d408cca961d0fb9b1faf497d6d\")\nprint(pair)\n```\n\n### Get token details\nTo get token details, pass a `chain id`, and a `token address`:\n```\ntoken = dextools.get_token(\"ether\", \"0xfb7b4564402e5500db5bb6d63ae671302777c75a\")\nprint(token)\n```\n\nYou can also pass the `page` and `pageSize` parameters:\n```\ntoken = dextools.get_token(\"ether\", \"0xfb7b4564402e5500db5bb6d63ae671302777c75a\", 1, 50)\nprint(token)\n```\n\n### Get chain list\nTo get the chain list:\n```\nchain_list = dextools.get_chain_list()\nprint(chain_list)\n```\n\nYou can also pass the `page` and `pageSize` parameters:\n```\nchain_list = dextools.get_chain_list(1, 50)\nprint(chain_list)\n```\n\n### Get exchange list\nTo get the exchange list, pass a `chain id`:\n```\nexchange_list = dextools.get_exchange_list(\"ether\")\nprint(exchange_list)\n```\n\nYou can also pass the `page` and `pageSize` parameters:\n```\nexchange_list = dextools.get_exchange_list(\"ether\", 1, 50)\nprint(exchange_list)\n```\n\n## Version 2 Queries\nBelow are a set of queries supported by the [Dextools API v2](https://developer.dextools.io/docs/start). All data is returned as a Python dictionary for easy data handling.\n\n### Blockchain\n#### Get blockchain info\n```\nblockchain = dextools.get_blockchain(\"ether\")\nprint(blockchain)\n```\n\n#### Get blockchains sorted by default settings\n```\nblockchains = dextools.get_blockchains()\nprint(blockchains)\n```\n\n#### Get blockchains sorted by default settings and descending order\n```\nblockchains = dextools.get_blockchains(sort=\"name\", order=\"desc\")\nprint(blockchains)\n```\n\n### Exchange\n#### Get dex factory info\n```\nfactory = dextools.get_dex_factory_info(\"ether\", \"0x5C69bEe701ef814a2B6a3EDD4B1652CB9cc5aA6f\")\nprint(factory)\n```\n\n#### Get dexes on a specific chain\n```\ndexes = dextools.get_dexes(\"ether\")\nprint(dexes)\n```\n\n#### Get dexes on a specific chain sorted by name and descending order\n```\ndexes = dextools.get_dexes(\"ether\", sort=\"creationBlock\", order=\"desc\")\nprint(dexes)\n```\n\n### Pool\n#### Get pool info\n```\npool = dextools.get_pool(\"ether\", \"0xa29fe6ef9592b5d408cca961d0fb9b1faf497d6d\")\nprint(pool)\n```\n\n#### Get pool liquidity\n```\npool_liquidity = dextools.get_pool_liquidity(\"ether\", \"0xa29fe6ef9592b5d408cca961d0fb9b1faf497d6d\")\nprint(pool_liquidity)\n```\n\n#### Get pool score\n```\npool_score = dextools.get_pool_score(\"ether\", \"0xa29fe6ef9592b5d408cca961d0fb9b1faf497d6d\")\nprint(pool_score)\n```\n\n#### Get pool price\n```\npool_price = dextools.get_pool_price(\"ether\", \"0xa29fe6ef9592b5d408cca961d0fb9b1faf497d6d\")\nprint(pool_price)\n```\n\n#### Get pools\n```\npools = dextools.get_pools(\"ether\", from_=\"2023-11-14T19:00:00\", to=\"2023-11-14T23:00:00\")\nprint(pools)\n```\n\n#### Get pools sorted by `creationBlock` and descending order and providing block numbers instead\n```\npools = dextools.get_pools(\"ether\", from_=\"12755070\", to=\"12755071\", sort=\"creationBlock\", order=\"desc\")\nprint(pools)\n```\n\n### Token\n#### Get token\n```\ntoken = dextools.get_token(\"ether\", \"0xfb7b4564402e5500db5bb6d63ae671302777c75a\")\nprint(token)\n```\n\n#### Get token locks\n```\ntoken_locks = dextools.get_token_locks(\"ether\", \"0xfb7b4564402e5500db5bb6d63ae671302777c75a\")\nprint(token_locks)\n```\n\n#### Get token score\n```\ntoken_score = dextools.get_token_score(\"ether\", \"0xfb7b4564402e5500db5bb6d63ae671302777c75a\")\nprint(token_score)\n```\n\n#### Get token info\n```\ntoken_info = dextools.get_token_info(\"ether\", \"0xfb7b4564402e5500db5bb6d63ae671302777c75a\")\nprint(token_info)\n```\n\n#### Get token price\n```\ntoken_price = dextools.get_token_price(\"ether\", \"0xfb7b4564402e5500db5bb6d63ae671302777c75a\")\nprint(token_price)\n```\n\n#### Get tokens\n```\ntokens = dextools.get_tokens(\"ether\", from_=\"2023-11-14T19:00:00\", to=\"2023-11-14T23:00:00\")\nprint(tokens)\n```\n\n#### Get tokens sorted by `creationBlock` and descending order and providing block numbers instead in descending order\n```\ntokens = dextools.get_tokens(\"ether\", from_=\"18570000\", to=\"18570500\", sort=\"creationBlock\", order=\"desc\")\nprint(tokens)\n```\n\n#### Get tokens sorted by `socialsInfoUpdated` and descending order and datetimes in descending order\n```\ntokens = dextools.get_tokens(\"ether\", from_=\"2023-11-14T19:00:00\", to=\"2023-11-14T23:00:00\", sort=\"socialsInfoUpdated\", order=\"desc\")\nprint(tokens)\n```\n\n#### Get token pools\n```\ntoken_pools = dextools.get_token_pools(\"ether\", \"0xfb7b4564402e5500db5bb6d63ae671302777c75a\", from_=\"2023-11-14T19:00:00\", to=\"2023-11-14T23:00:00\")\nprint(token_pools)\n```\n\n#### Get token pools sorted by `creationBlock` and descending order and providing block numbers instead in descending order\n```\ntoken_pools = dextools.get_token_pools(\"ether\", \"0xfb7b4564402e5500db5bb6d63ae671302777c75a\", from_=\"18570000\", to=\"18570500\", sort=\"creationBlock\", order=\"desc\")\nprint(token_pools)\n```\n\n### Rankings\n#### Get hot pools\n```\nhot_pools = dextools.get_ranking_hotpools(\"ether\")\nprint(hot_pools)\n```\n\n#### Get gainers\n```\ngainers = dextools.get_ranking_gainers(\"ether\")\nprint(gainers)\n```\n\n#### Get losers\n```\nlosers = dextools.get_ranking_losers(\"ether\")\nprint(losers)\n```\n\n## Page and PageSize arguments\nSome methods support the `page` and `pageSize` arguments. Check out the API documentation for more information.\n\n## Asynchronous support with asyncio\nAsynchronous support has been added through [asyncio](https://docs.python.org/3/library/asyncio.html).\nYou can initiate an `AsyncDextoolsAPI` instance for API version 1, or an `AsyncDextoolsAPIV2` instance for API version 2.\n\nThrough a context manager (session will close automatically when the context manager ends):\n```\nimport asyncio\nfrom dextools_python import AsyncDextoolsAPIV2\n\napi_key = \"YOUR_API_KEY\"\n\nasync def main():\n async with AsyncDextoolsAPIV2(api_key, plan=\"partner\") as dextools:\n response = await dextools.get_blockchains()\n print(response)\n\nasyncio.run(main())\n```\n\nCreating an instance and then closing the session explicitly at the end:\n```\nimport asyncio\nfrom dextools_python import AsyncDextoolsAPIV2\n\napi_key = \"YOUR_API_KEY\"\n\nasync def main():\n dextools = AsyncDextoolsAPIV2(api_key, plan=\"partner\")\n\n response = await dextools.get_blockchains()\n print(response)\n\n await dextools.close()\n\nasyncio.run(main())\n```\n\n## Examples\nCheck out the `examples` folder for some synchronous and asynchronous example scripts.\n\n## Testing\nA set of tests have been included inside `tests` folder. You will need to set an environment variable as `DextoolsAPIKey` using your API key.\n\n## Supported Blockchains\nDextools adds support for new blockchains from time to time. `dextools.get_blockchains()` to get a list of supported blockchains and their IDs\n\n## Authors\n* [alb2001](https://github.com/alb2001)\n\n\n## More information\n* [dextools-python on PyPI](https://pypi.org/project/dextools-python)\n* [DEXTools](https://www.dextools.io)\n* [Dextools API v1](https://api.dextools.io/docs)\n* [Dextools API v2](https://developer.dextools.io/docs/start)\n* [Developer Portal](https://developer.dextools.io/)\n* [asyncio](https://docs.python.org/3/library/asyncio.html)",
"bugtrack_url": null,
"license": "MIT",
"summary": "A simple Python API wrapper for DEXTools",
"version": "0.3.1",
"project_urls": {
"Homepage": "https://github.com/alb2001/dextools-python",
"Repository": "https://github.com/alb2001/dextools-python"
},
"split_keywords": [
"dextools",
"api",
"wrapper",
"blockchain",
"trading",
"exchange",
"uniswap"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "1bfc3a6e525363735e55b7a8b6cf4c4869dd436bbd9901063f4e947897b378ab",
"md5": "141b3c42cb904e26a3398010120d1eb3",
"sha256": "95cfc396b1fd1e342e719db319ab0d6de8c06de6b9e422df1cf7ed24fb01539d"
},
"downloads": -1,
"filename": "dextools_python-0.3.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "141b3c42cb904e26a3398010120d1eb3",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8,<4.0",
"size": 9019,
"upload_time": "2024-03-11T16:14:49",
"upload_time_iso_8601": "2024-03-11T16:14:49.924530Z",
"url": "https://files.pythonhosted.org/packages/1b/fc/3a6e525363735e55b7a8b6cf4c4869dd436bbd9901063f4e947897b378ab/dextools_python-0.3.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "05d0a71392864b7e13de0afcfbf1025557a8d45c85d64d0a003df187117b435a",
"md5": "f12b932222632e28c0d8341ad4cdbe37",
"sha256": "78c478e2512353472000544c38a8c9e892100aa8b390628cff5d52de006f0214"
},
"downloads": -1,
"filename": "dextools_python-0.3.1.tar.gz",
"has_sig": false,
"md5_digest": "f12b932222632e28c0d8341ad4cdbe37",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8,<4.0",
"size": 9157,
"upload_time": "2024-03-11T16:14:51",
"upload_time_iso_8601": "2024-03-11T16:14:51.494997Z",
"url": "https://files.pythonhosted.org/packages/05/d0/a71392864b7e13de0afcfbf1025557a8d45c85d64d0a003df187117b435a/dextools_python-0.3.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-11 16:14:51",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "alb2001",
"github_project": "dextools-python",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "dextools-python"
}