Name | django-pictures JSON |
Version |
1.2.0
JSON |
| download |
home_page | |
Summary | Responsive cross-browser image library using modern codes like AVIF & WebP. |
upload_time | 2024-01-20 11:15:09 |
maintainer | |
docs_url | None |
author | |
requires_python | >=3.8 |
license | |
keywords |
pillow
django
image
pictures
webp
avif
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
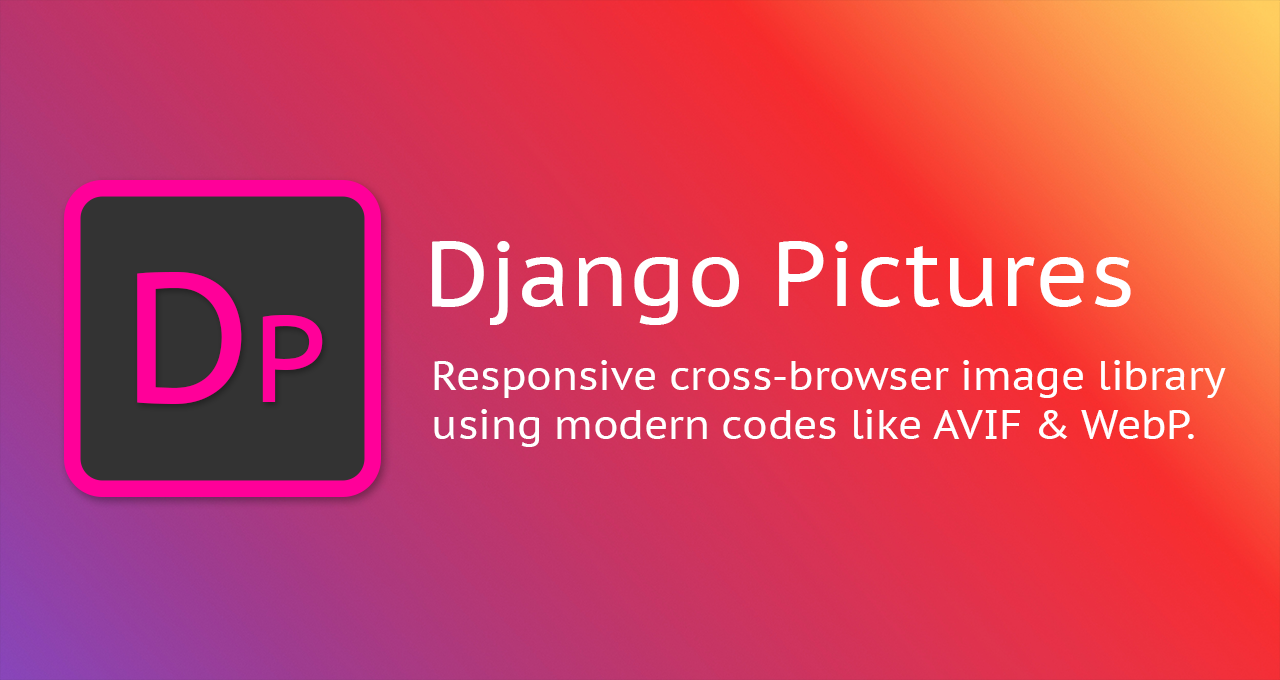
# Django Pictures
Responsive cross-browser image library using modern codes like AVIF & WebP.
* responsive web images using the [picture](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/picture) tag
* native grid system support
* serve files with or without a CDN
* placeholders for local development
* migration support
* async image processing for [Celery], [Dramatiq] or [Django RQ][django-rq]
* [DRF] support
[](https://pypi.python.org/pypi/django-pictures/)
[](https://codecov.io/gh/codingjoe/django-pictures)
[](https://raw.githubusercontent.com/codingjoe/django-pictures/master/LICENSE)
## Usage
Before you start, it can be a good idea to understand the fundamentals of
[responsive images](https://developer.mozilla.org/en-US/docs/Learn/HTML/Multimedia_and_embedding/Responsive_images).
Once you get a feeling how complicated things can get with all device types, you'll probably find
a new appreciation for this package and are ready to adopt in you project :)
```python
# models.py
from django.db import models
from pictures.models import PictureField
class Profile(models.Model):
title = models.CharField(max_length=255)
picture = PictureField(upload_to="avatars")
```
```html
<!-- template.html -->
{% load pictures %}
{% picture profile.picture img_alt="Spiderman" img_loading="lazy" picture_class="my-picture" m=6 l=4 %}
```
The keyword arguments `m=6 l=4` define the columns the image should take up in
a grid at a given breakpoint. So in this example, the image will take up
6 columns on medium screens and 4 columns on large screens. You can define your
grid and breakpoints as you want, refer to the [grid columns](#grid-columns) and
[breakpoints](#breakpoints) sections.
The template above will render into:
```html
<picture class="my-picture">
<source type="image/webp"
srcset="/media/testapp/profile/image/800w.webp 800w, /media/testapp/profile/image/100w.webp 100w, /media/testapp/profile/image/200w.webp 200w, /media/testapp/profile/image/300w.webp 300w, /media/testapp/profile/image/400w.webp 400w, /media/testapp/profile/image/500w.webp 500w, /media/testapp/profile/image/600w.webp 600w, /media/testapp/profile/image/700w.webp 700w"
sizes="(min-width: 0px) and (max-width: 991px) 100vw, (min-width: 992px) and (max-width: 1199px) 33vw, 600px">
<img src="/media/testapp/profile/image.jpg" alt="Spiderman" width="800" height="800" loading="lazy">
</picture>
```
Note that arbitrary attributes can be passed
to either the `<picture>` or `<img>` element
by prefixing parameters to the `{% picture %}` tag
with `picture_` or `img_` respectively.
## Setup
### Installation
```shell
python3 -m pip install django-pictures
```
### Settings
```python
# settings.py
INSTALLED_APPS = [
# ...
'pictures',
]
# the following are defaults, but you can override them
PICTURES = {
"BREAKPOINTS": {
"xs": 576,
"s": 768,
"m": 992,
"l": 1200,
"xl": 1400,
},
"GRID_COLUMNS": 12,
"CONTAINER_WIDTH": 1200,
"FILE_TYPES": ["WEBP"],
"PIXEL_DENSITIES": [1, 2],
"USE_PLACEHOLDERS": True,
"QUEUE_NAME": "pictures",
"PROCESSOR": "pictures.tasks.process_picture",
}
```
If you have either Dramatiq or Celery installed, we will default to async
image processing. You will need workers to listen to the `pictures` queue.
### Placeholders
This library comes with dynamically created placeholders to simplify local
development. To enable them, add the following to enable the
`PICTURES["USE_PLACEHOLDERS"]` setting and add the following URL configuration:
```python
# urls.py
from django.urls import include, path
from pictures.conf import get_settings
urlpatterns = [
# ...
]
if get_settings().USE_PLACEHOLDERS:
urlpatterns += [
path("_pictures/", include("pictures.urls")),
]
```
### Legacy use-cases (email)
Although the `picture`-tag is [adequate for most use-cases][caniuse-picture],
some remain, where a single `img` tag is necessary. Notably in email, where
[most clients do support WebP][caniemail-webp] but not [srcset][caniemail-srcset].
The template tag `img_url` returns a single size image URL.
In addition to the ratio you will need to define the `file_type`
as well as the `width` (absolute width in pixels).
```html
{% load pictures %}
<img src="{% img_url profile.picture ratio="3/2" file_type="webp" width=800 %}" alt="profile picture">
```
[caniuse-picture]: https://caniuse.com/picture
[caniemail-webp]: https://www.caniemail.com/features/image-webp/
[caniemail-srcset]: https://www.caniemail.com/features/html-srcset/
## Config
### Aspect ratios
You can specify the aspect ratios of your images. Images will be cropped to the
specified aspect ratio. Aspect ratios are specified as a string with a slash
between the width and height. For example, `16/9` will crop the image to 16:9.
```python
# models.py
from django.db import models
from pictures.models import PictureField
class Profile(models.Model):
title = models.CharField(max_length=255)
picture = PictureField(
upload_to="avatars",
aspect_ratios=[None, "1/1", "3/2", "16/9"],
)
```
```html
# template.html
{% load pictures %}
{% picture profile.picture img_alt="Spiderman" ratio="16/9" m=6 l=4 %}
```
If you don't specify an aspect ratio or None in your template, the image will be
served with the original aspect ratio of the file.
You may only use aspect ratios in templates, that have been defined on the model.
The model `aspect_ratios` will default to `[None]`, if other value is provided.
### Breakpoints
You may define your own breakpoints, they should be identical to the ones used
in your css library. Simply override the `PICTURES["BREAKPOINTS"]` setting.
### Grid columns
Grids are so common in web design, that they even made it into CSS.
We default to 12 columns, but you can override this setting, via the
`PICTURES["GRID_COLUMNS"]` setting.
### Container width
Containers are commonly used to limit the maximum width of layouts,
to promote better readability on larger screens. We default to `1200px`,
but you can override this setting, via the `PICTURES["CONTAINER_WIDTH"]` setting.
You may also set it to `None`, should you not use a container.
### File types
Unless you still services IE11 clients, you should be fine serving just
[WebP](https://caniuse.com/webp). Sadly, [AVIF](https://caniuse.com/avif)
(WebP's successor) is
[not yet supported by Pillow](https://github.com/python-pillow/Pillow/pull/5201).
If you are serving IE11 use add `JPEG` to the list. Beware though, that this may
drastically increase you storage needs.
### Pixel densities
Unless you really care that your images hold of if you hold your UHD phone very
close to your eyeballs, you should be fine, serving at the default `1x` and `2x`
densities.
### Async image processing
If you have either Dramatiq or Celery installed, we will default to async
image processing. You will need workers to listen to the `pictures` queue.
You can override the queue name, via the `PICTURES["QUEUE_NAME"]` setting.
You can also override the processor, via the `PICTURES["PROCESSOR"]` setting.
The default processor is `pictures.tasks.process_picture`. It takes a single
argument, the `PictureFileFile` instance. You can use this to override the
processor, should you need to do some custom processing.
## Migrations
Django doesn't support file field migrations, but we do.
You can simply auto create the migration and replace Django's
`AlterField` operation with `AlterPictureField`. That's it.
You can follow [the example][migration] in our test app, to see how it works.
[migration]: tests/testapp/migrations/0002_alter_profile_picture.py
## Contrib
### Django Rest Framework ([DRF])
We do ship with a read-only `PictureField` that can be used to include all
available picture sizes in a DRF serializer.
```python
from rest_framework import serializers
from pictures.contrib.rest_framework import PictureField
class PictureSerializer(serializers.Serializer):
picture = PictureField()
```
You may provide optional GET parameters to the serializer, to specify the aspect
ratio and breakpoints you want to include in the response. The parameters are
prefixed with the `fieldname_` to avoid conflicts with other fields.
```bash
curl http://localhost:8000/api/path/?picture_ratio=16%2F9&picture_m=6&picture_l=4
# %2F is the url encoded slash
```
```json
{
"other_fields": "…",
"picture": {
"url": "/path/to/image.jpg",
"width": 800,
"height": 800,
"ratios": {
"1/1": {
"sources": {
"image/webp": {
"100": "/path/to/image/1/100w.webp",
"200": "…"
}
},
"media": "(min-width: 0px) and (max-width: 991px) 100vw, (min-width: 992px) and (max-width: 1199px) 33vw, 25vw"
}
}
}
}
```
Note that the `media` keys are only included, if you have specified breakpoints.
### Django Cleanup
`PictureField` is compatible with [Django Cleanup](https://github.com/un1t/django-cleanup),
which automatically deletes its file and corresponding `SimplePicture` files.
[drf]: https://www.django-rest-framework.org/
[celery]: https://docs.celeryproject.org/en/stable/
[dramatiq]: https://dramatiq.io/
[django-rq]: https://github.com/rq/django-rq
Raw data
{
"_id": null,
"home_page": "",
"name": "django-pictures",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "",
"keywords": "pillow,Django,image,pictures,WebP,AVIF",
"author": "",
"author_email": "Johannes Maron <johannes@maron.family>",
"download_url": "https://files.pythonhosted.org/packages/1c/a2/fb2a0b070a268e3e2578447655abe1d279d51ec44ff2164a12dc6277dd8a/django_pictures-1.2.0.tar.gz",
"platform": null,
"description": "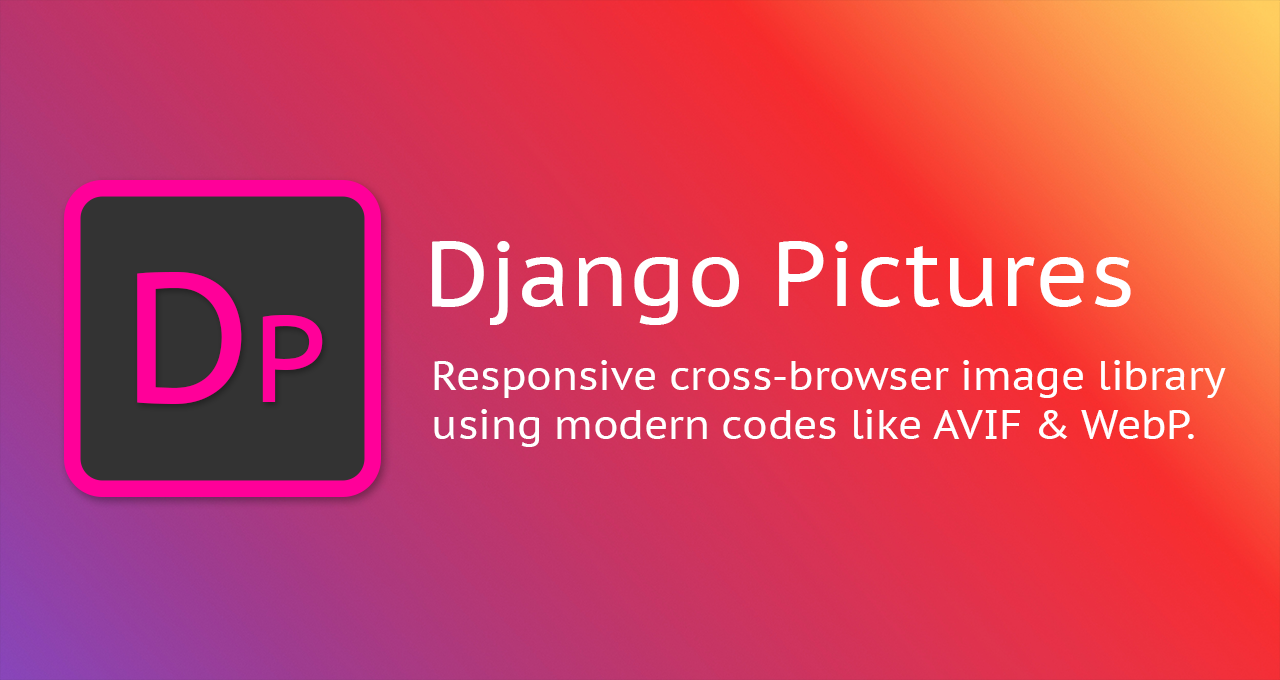\n\n# Django Pictures\n\nResponsive cross-browser image library using modern codes like AVIF & WebP.\n\n* responsive web images using the [picture](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/picture) tag\n* native grid system support\n* serve files with or without a CDN\n* placeholders for local development\n* migration support\n* async image processing for [Celery], [Dramatiq] or [Django RQ][django-rq]\n* [DRF] support\n\n[](https://pypi.python.org/pypi/django-pictures/)\n[](https://codecov.io/gh/codingjoe/django-pictures)\n[](https://raw.githubusercontent.com/codingjoe/django-pictures/master/LICENSE)\n\n## Usage\n\nBefore you start, it can be a good idea to understand the fundamentals of\n[responsive images](https://developer.mozilla.org/en-US/docs/Learn/HTML/Multimedia_and_embedding/Responsive_images).\n\nOnce you get a feeling how complicated things can get with all device types, you'll probably find\na new appreciation for this package and are ready to adopt in you project :)\n\n```python\n# models.py\nfrom django.db import models\nfrom pictures.models import PictureField\n\nclass Profile(models.Model):\n title = models.CharField(max_length=255)\n picture = PictureField(upload_to=\"avatars\")\n```\n\n```html\n<!-- template.html -->\n{% load pictures %}\n{% picture profile.picture img_alt=\"Spiderman\" img_loading=\"lazy\" picture_class=\"my-picture\" m=6 l=4 %}\n```\n\nThe keyword arguments `m=6 l=4` define the columns the image should take up in\na grid at a given breakpoint. So in this example, the image will take up\n6 columns on medium screens and 4 columns on large screens. You can define your\ngrid and breakpoints as you want, refer to the [grid columns](#grid-columns) and\n[breakpoints](#breakpoints) sections.\n\nThe template above will render into:\n```html\n<picture class=\"my-picture\">\n <source type=\"image/webp\"\n srcset=\"/media/testapp/profile/image/800w.webp 800w, /media/testapp/profile/image/100w.webp 100w, /media/testapp/profile/image/200w.webp 200w, /media/testapp/profile/image/300w.webp 300w, /media/testapp/profile/image/400w.webp 400w, /media/testapp/profile/image/500w.webp 500w, /media/testapp/profile/image/600w.webp 600w, /media/testapp/profile/image/700w.webp 700w\"\n sizes=\"(min-width: 0px) and (max-width: 991px) 100vw, (min-width: 992px) and (max-width: 1199px) 33vw, 600px\">\n <img src=\"/media/testapp/profile/image.jpg\" alt=\"Spiderman\" width=\"800\" height=\"800\" loading=\"lazy\">\n</picture>\n```\n\nNote that arbitrary attributes can be passed\nto either the `<picture>` or `<img>` element\nby prefixing parameters to the `{% picture %}` tag\nwith `picture_` or `img_` respectively.\n\n## Setup\n\n### Installation\n\n```shell\npython3 -m pip install django-pictures\n```\n\n### Settings\n\n```python\n# settings.py\nINSTALLED_APPS = [\n # ...\n 'pictures',\n]\n\n# the following are defaults, but you can override them\nPICTURES = {\n \"BREAKPOINTS\": {\n \"xs\": 576,\n \"s\": 768,\n \"m\": 992,\n \"l\": 1200,\n \"xl\": 1400,\n },\n \"GRID_COLUMNS\": 12,\n \"CONTAINER_WIDTH\": 1200,\n \"FILE_TYPES\": [\"WEBP\"],\n \"PIXEL_DENSITIES\": [1, 2],\n \"USE_PLACEHOLDERS\": True,\n \"QUEUE_NAME\": \"pictures\",\n \"PROCESSOR\": \"pictures.tasks.process_picture\",\n\n}\n```\n\nIf you have either Dramatiq or Celery installed, we will default to async\nimage processing. You will need workers to listen to the `pictures` queue.\n\n### Placeholders\n\nThis library comes with dynamically created placeholders to simplify local\ndevelopment. To enable them, add the following to enable the\n`PICTURES[\"USE_PLACEHOLDERS\"]` setting and add the following URL configuration:\n\n```python\n# urls.py\nfrom django.urls import include, path\nfrom pictures.conf import get_settings\n\nurlpatterns = [\n # ...\n]\n\nif get_settings().USE_PLACEHOLDERS:\n urlpatterns += [\n path(\"_pictures/\", include(\"pictures.urls\")),\n ]\n```\n\n### Legacy use-cases (email)\n\nAlthough the `picture`-tag is [adequate for most use-cases][caniuse-picture],\nsome remain, where a single `img` tag is necessary. Notably in email, where\n[most clients do support WebP][caniemail-webp] but not [srcset][caniemail-srcset].\nThe template tag `img_url` returns a single size image URL.\nIn addition to the ratio you will need to define the `file_type`\nas well as the `width` (absolute width in pixels).\n\n\n```html\n{% load pictures %}\n<img src=\"{% img_url profile.picture ratio=\"3/2\" file_type=\"webp\" width=800 %}\" alt=\"profile picture\">\n```\n\n[caniuse-picture]: https://caniuse.com/picture\n[caniemail-webp]: https://www.caniemail.com/features/image-webp/\n[caniemail-srcset]: https://www.caniemail.com/features/html-srcset/\n\n## Config\n\n### Aspect ratios\n\nYou can specify the aspect ratios of your images. Images will be cropped to the\nspecified aspect ratio. Aspect ratios are specified as a string with a slash\nbetween the width and height. For example, `16/9` will crop the image to 16:9.\n\n```python\n# models.py\nfrom django.db import models\nfrom pictures.models import PictureField\n\n\nclass Profile(models.Model):\n title = models.CharField(max_length=255)\n picture = PictureField(\n upload_to=\"avatars\",\n aspect_ratios=[None, \"1/1\", \"3/2\", \"16/9\"],\n )\n```\n\n```html\n# template.html\n{% load pictures %}\n{% picture profile.picture img_alt=\"Spiderman\" ratio=\"16/9\" m=6 l=4 %}\n```\n\nIf you don't specify an aspect ratio or None in your template, the image will be\nserved with the original aspect ratio of the file.\n\nYou may only use aspect ratios in templates, that have been defined on the model.\nThe model `aspect_ratios` will default to `[None]`, if other value is provided.\n\n### Breakpoints\n\nYou may define your own breakpoints, they should be identical to the ones used\nin your css library. Simply override the `PICTURES[\"BREAKPOINTS\"]` setting.\n\n### Grid columns\n\nGrids are so common in web design, that they even made it into CSS.\nWe default to 12 columns, but you can override this setting, via the\n`PICTURES[\"GRID_COLUMNS\"]` setting.\n\n### Container width\n\nContainers are commonly used to limit the maximum width of layouts,\nto promote better readability on larger screens. We default to `1200px`,\nbut you can override this setting, via the `PICTURES[\"CONTAINER_WIDTH\"]` setting.\n\nYou may also set it to `None`, should you not use a container.\n\n### File types\n\nUnless you still services IE11 clients, you should be fine serving just\n[WebP](https://caniuse.com/webp). Sadly, [AVIF](https://caniuse.com/avif)\n(WebP's successor) is\n[not yet supported by Pillow](https://github.com/python-pillow/Pillow/pull/5201).\n\nIf you are serving IE11 use add `JPEG` to the list. Beware though, that this may\ndrastically increase you storage needs.\n\n### Pixel densities\n\nUnless you really care that your images hold of if you hold your UHD phone very\nclose to your eyeballs, you should be fine, serving at the default `1x` and `2x`\ndensities.\n\n### Async image processing\n\nIf you have either Dramatiq or Celery installed, we will default to async\nimage processing. You will need workers to listen to the `pictures` queue.\nYou can override the queue name, via the `PICTURES[\"QUEUE_NAME\"]` setting.\n\nYou can also override the processor, via the `PICTURES[\"PROCESSOR\"]` setting.\nThe default processor is `pictures.tasks.process_picture`. It takes a single\nargument, the `PictureFileFile` instance. You can use this to override the\nprocessor, should you need to do some custom processing.\n\n## Migrations\n\nDjango doesn't support file field migrations, but we do.\nYou can simply auto create the migration and replace Django's\n`AlterField` operation with `AlterPictureField`. That's it.\n\nYou can follow [the example][migration] in our test app, to see how it works.\n\n[migration]: tests/testapp/migrations/0002_alter_profile_picture.py\n\n## Contrib\n\n### Django Rest Framework ([DRF])\n\nWe do ship with a read-only `PictureField` that can be used to include all\navailable picture sizes in a DRF serializer.\n\n```python\nfrom rest_framework import serializers\nfrom pictures.contrib.rest_framework import PictureField\n\nclass PictureSerializer(serializers.Serializer):\n picture = PictureField()\n```\n\nYou may provide optional GET parameters to the serializer, to specify the aspect\nratio and breakpoints you want to include in the response. The parameters are\nprefixed with the `fieldname_` to avoid conflicts with other fields.\n\n```bash\ncurl http://localhost:8000/api/path/?picture_ratio=16%2F9&picture_m=6&picture_l=4\n# %2F is the url encoded slash\n```\n\n```json\n{\n \"other_fields\": \"\u2026\",\n \"picture\": {\n \"url\": \"/path/to/image.jpg\",\n \"width\": 800,\n \"height\": 800,\n \"ratios\": {\n \"1/1\": {\n \"sources\": {\n \"image/webp\": {\n \"100\": \"/path/to/image/1/100w.webp\",\n \"200\": \"\u2026\"\n }\n },\n \"media\": \"(min-width: 0px) and (max-width: 991px) 100vw, (min-width: 992px) and (max-width: 1199px) 33vw, 25vw\"\n }\n }\n }\n}\n```\n\nNote that the `media` keys are only included, if you have specified breakpoints.\n\n### Django Cleanup\n\n`PictureField` is compatible with [Django Cleanup](https://github.com/un1t/django-cleanup),\nwhich automatically deletes its file and corresponding `SimplePicture` files.\n\n[drf]: https://www.django-rest-framework.org/\n[celery]: https://docs.celeryproject.org/en/stable/\n[dramatiq]: https://dramatiq.io/\n[django-rq]: https://github.com/rq/django-rq\n\n",
"bugtrack_url": null,
"license": "",
"summary": "Responsive cross-browser image library using modern codes like AVIF & WebP.",
"version": "1.2.0",
"project_urls": {
"Changelog": "https://github.com/codingjoe/django-pictures/releases",
"Documentation": "https://github.com/codingjoe/django-pictures#django-pictures",
"Issue-Tracker": "https://github.com/codingjoe/django-pictures/issues",
"Project-URL": "https://github.com/codingjoe/django-pictures",
"Source": "https://github.com/codingjoe/django-pictures"
},
"split_keywords": [
"pillow",
"django",
"image",
"pictures",
"webp",
"avif"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "b448f8284ea17bc340e33667d5430f70ea4aa410e0c7ed3238e25c8f12e7494e",
"md5": "36d8dc401715850c967b97e9aeb10eef",
"sha256": "265ba67168e10cbb4b89f4e950613b5ee0bb14b3f7066dc16e8bbe09431c6a42"
},
"downloads": -1,
"filename": "django_pictures-1.2.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "36d8dc401715850c967b97e9aeb10eef",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 20635,
"upload_time": "2024-01-20T11:15:07",
"upload_time_iso_8601": "2024-01-20T11:15:07.525600Z",
"url": "https://files.pythonhosted.org/packages/b4/48/f8284ea17bc340e33667d5430f70ea4aa410e0c7ed3238e25c8f12e7494e/django_pictures-1.2.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1ca2fb2a0b070a268e3e2578447655abe1d279d51ec44ff2164a12dc6277dd8a",
"md5": "472b9d19e5b191ab9fbb2a18923b41ab",
"sha256": "1205591ef970231e3fa62d193c778ee49cd4a60033a5edc6c197435dc1e34c0d"
},
"downloads": -1,
"filename": "django_pictures-1.2.0.tar.gz",
"has_sig": false,
"md5_digest": "472b9d19e5b191ab9fbb2a18923b41ab",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 19036,
"upload_time": "2024-01-20T11:15:09",
"upload_time_iso_8601": "2024-01-20T11:15:09.856422Z",
"url": "https://files.pythonhosted.org/packages/1c/a2/fb2a0b070a268e3e2578447655abe1d279d51ec44ff2164a12dc6277dd8a/django_pictures-1.2.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-01-20 11:15:09",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "codingjoe",
"github_project": "django-pictures",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "django-pictures"
}