# Fastapi CRUD Template Generator
[](https://coveralls.io/github/LuisLuii/fastapi-crud-template-generator?branch=main)
[](https://www.codacy.com/gh/LuisLuii/fastapi-crud-template-generator/dashboard?utm_source=github.com&utm_medium=referral&utm_content=LuisLuii/fastapi-crud-template-generator&utm_campaign=Badge_Grade)
[](https://badge.fury.io/py/fastapi-crud-code-generator)
[](https://pypi.org/project/fastapi-crud-code-generator)
[](https://github.com/LuisLuii/fastapi-crud-template-generator/actions/workflows/ci.yml)
## Introducing FastAPI CRUD Template Generator
FastAPI CRUD Template Generator is a tool that helps developers quickly scaffold FastAPI projects with CRUD operations for their SQLAlchemy databases. It can provide a starting point for your project and can save time and effort by providing pre-written code of the CRUD operations and validation model, and a pre-defined project structureIt. The generated code can be customized to add business logic and extend the functionality of the FastAPI project. The tool supports the following APIs:
>- Get one
>- Get many
>- Insert one
>- Insert many
>- Update one
>- Update many
>- Patch one
>- Patch many
>- Delete one
>- Delete many
FastAPI CRUD Generator is the tool for building efficient and scalable APIs with FastAPI and SQLAlchemy. Try it now and start building your next great project.
## DEMO
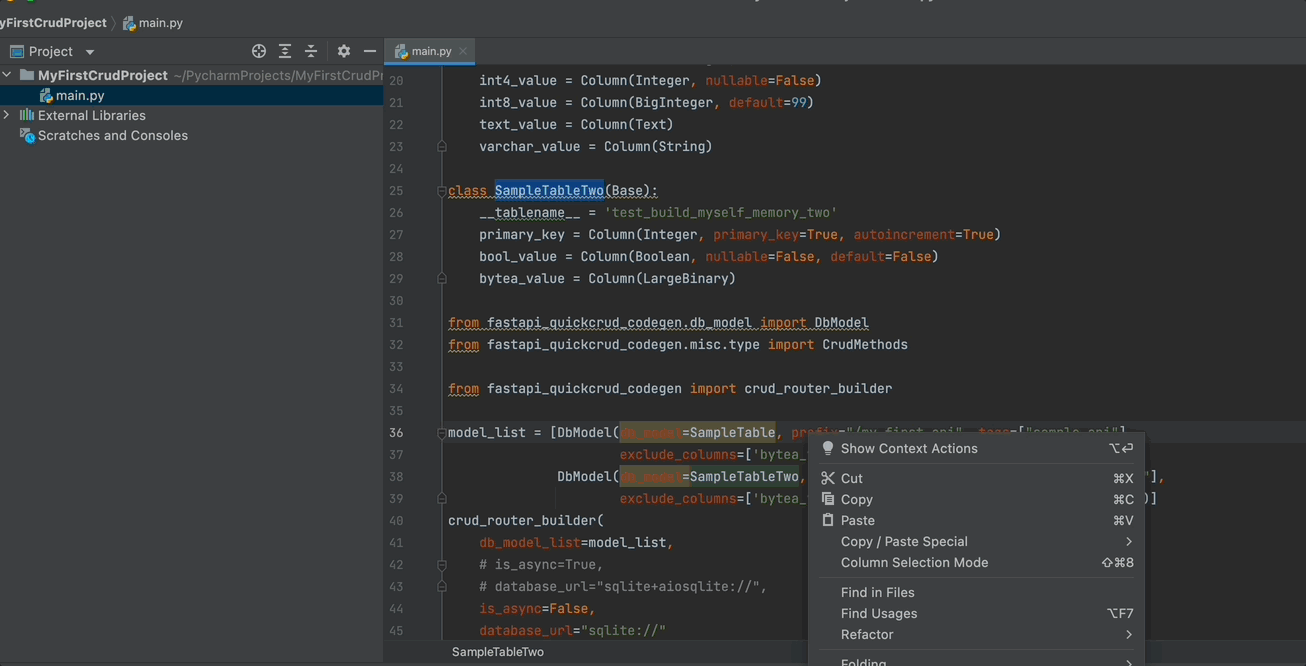
## Features
* CRUD router code generation from SQLAlchemy declarative class definitions
* API validation model code generation
* Support for SQLAlchemy 1.4
* Pagination support for APIs
* Flexible API request handling
## Quick Start with in-memory DB (or see the other ([example](https://github.com/LuisLuii/fastapi-crud-template-generator/tree/feature/fix_code_style/tutorial))
### Install
To get started with FastAPI CRUD Template Generator, you will need to install the package using pip:
```python
pip install fastapi-crud-code-generator
```
### Prepare your Sqlalchemy schema
Next, you will need to prepare your SQLAlchemy schema by defining your database tables as declarative classes. You can use the [sqlacodegen](https://github.com/agronholm/sqlacodegen) tool to automatically generate these classes from your database.
```
from sqlalchemy import *
from sqlalchemy.orm import declarative_base
Base = declarative_base()
metadata = Base.metadata
class SampleTable(Base):
__tablename__ = 'test_build_myself_memory'
__table_args__ = (
UniqueConstraint('primary_key', 'int4_value', 'float4_value'),
)
primary_key = Column(Integer, primary_key=True, autoincrement=True)
bool_value = Column(Boolean, nullable=False, default=False)
bytea_value = Column(LargeBinary)
char_value = Column(CHAR(10, collation='NOCASE'))
date_value = Column(Date)
float4_value = Column(Float, nullable=False)
float8_value = Column(Float(53), nullable=False, default=10.10)
int2_value = Column(SmallInteger, nullable=False)
int4_value = Column(Integer, nullable=False)
int8_value = Column(BigInteger, default=99)
text_value = Column(Text)
varchar_value = Column(String)
class SampleTableTwo(Base):
__tablename__ = 'test_build_myself_memory_two'
primary_key = Column(Integer, primary_key=True, autoincrement=True)
bool_value = Column(Boolean, nullable=False, default=False)
bytea_value = Column(LargeBinary)
```
### Generate template
Once you have your SQLAlchemy schema defined, you can use the `crud_router_builder` method to generate CRUD router code for your API. This method takes the declarative class definitions as input and generates code that defines the endpoints and validation models for each CRUD operation.
Here is an example of how to use the `crud_router_builder` method to generate CRUD router code:
```python
from fastapi_quickcrud_codegen.db_model import DbModel
from fastapi_quickcrud_codegen.misc.type import CrudMethods
from fastapi_quickcrud_codegen import crud_router_builder
model_list = [DbModel(db_model=SampleTable, prefix="/my_first_api", tags=["sample api"],
exclude_columns=['bytea_value']),
DbModel(db_model=SampleTableTwo, prefix="/my_second_api", tags=["sample api"],
exclude_columns=['bytea_value'],crud_methods=[CrudMethods.FIND_ONE])]
crud_router_builder(
db_model_list=model_list,
# is_async=True,
# database_url="sqlite+aiosqlite://",
is_async=False,
database_url="sqlite://"
)
```
<img width="1292" alt="image" src="https://user-images.githubusercontent.com/31765235/200206184-a16a8622-7db6-4c62-bb56-99cdee126493.png">
**crud_router_builder args**
- db_model_list `[Required[List[DbModel]]] `
> Model list of dict for code generate
- `List[]`
- `DbModel`
- db_model `[Required[DeclarativeMeta]]`
- prefix `[Required[str]]`
> prefix for Fastapi's end point
- tags `[Required[List[str]]]`
> list of tag for Fastapi's end point
- exclude_columns `[Optional[List[str]]]`
> set the columns that not to be operated but the columns should nullable or set the default value)
- crud_methods `[Opional[List[CrudMethods]]]`
> - Create the following apis for that model, but default: [CrudMethods.FIND_MANY, CrudMethods.FIND_ONE, CrudMethods.CREATE_MANY, CrudMethods.PATCH_ONE, CrudMethods.PATCH_MANY, CrudMethods.PATCH_ONE, CrudMethods.UPDATE_MANY, CrudMethods.UPDATE_ONE, CrudMethods.DELETE_MANY, CrudMethods.DELETE_ONE]
```
- CrudMethods.FIND_ONE
- CrudMethods.FIND_MANY
- CrudMethods.UPDATE_ONE
- CrudMethods.UPDATE_MANY
- CrudMethods.PATCH_ONE
- CrudMethods.PATCH_MANY
- CrudMethods.CREATE_ONE
- CrudMethods.CREATE_MANY
- CrudMethods.DELETE_ONE
- CrudMethods.DELETE_MANY
```
- is_async `[Required]`
> True for async; False for sync
- database_url `[Optional (str)]`
> A database URL. The URL is passed directly to SQLAlchemy's create_engine() method so please refer to SQLAlchemy's documentation for instructions on how to construct a proper URL.
# Known limitations
* ❌ Please use composite unique constraints instead of multiple unique constraints
* ❌ Composite primary key is not supported
* ❌ Sqlalchemy table type model schema is not supported
# Design:
The model generation part and api router part refer to my another [project](https://github.com/LuisLuii/FastAPIQuickCRUD); The code generation part is using Jinja
## How to contribute more apis?
It will be super excited if you have any idea with api router/ model template. then you can follow the step as below to try to contribute.
1. Model generation
1. Prepare Jinja template to `fastapi-crud-project-generator/src/fastapi_quickcrud_codegen/model/template`
2. Prepare model code generation method from `fastapi_quickcrud_codegen.utils.schema_builder.ApiParameterSchemaBuilder`
3. Generate the code from `fastapi_quickcrud_codegen/model/model_builder.py`
2. CRUD Method Generation
1. After the model generation, you can try to build your own api by your own model
2. Modify `fastapi_quickcrud_codegen.utils.sqlalchemy_to_pydantic.sqlalchemy_to_pydantic`, `fastapi_quickcrud_codegen.misc.type.CrudMethods` and `fastapi_quickcrud_codegen.misc.crud_model.CRUDModel` to let project supports your api
3. Prepare your api router Jinja template from `src/fastapi_quickcrud_codegen/model/template/route`
4. Generate the code from `fastapi_quickcrud_codegen/model/crud_builder.py`
5. Update the api_register from `src/fastapi_quickcrud_codegen/crud_generator.py`
* use Sqlalchemy's sql.expression instead custom statement when building sql statement for your api
# Road map
#### Current State: Stable
#### Will do:
>- Support foreign tree in find api
>- Support foreign tree in insert api
>- Support foreign tree in update api
>- Support foreign tree in delete api
#### Good to have:
>- Support Sqlalchemy Table type model
Raw data
{
"_id": null,
"home_page": "https://github.com/LuisLuii/fastapi-crud-template-generator",
"name": "fastapi-crud-code-generator",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "",
"keywords": "fastapi,crud,restful,routing,SQLAlchemy,generator,crudrouter,postgresql,builder",
"author": "Luis Lui",
"author_email": "luis11235178@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/d8/d2/91ed0fc3c9fd5e45ed23402c044bc2ae9e22e40ca58db694204648765121/fastapi-crud-code-generator-0.0.31.tar.gz",
"platform": null,
"description": "# Fastapi CRUD Template Generator\n[](https://coveralls.io/github/LuisLuii/fastapi-crud-template-generator?branch=main)\n[](https://www.codacy.com/gh/LuisLuii/fastapi-crud-template-generator/dashboard?utm_source=github.com&utm_medium=referral&utm_content=LuisLuii/fastapi-crud-template-generator&utm_campaign=Badge_Grade)\n[](https://badge.fury.io/py/fastapi-crud-code-generator)\n[](https://pypi.org/project/fastapi-crud-code-generator)\n[](https://github.com/LuisLuii/fastapi-crud-template-generator/actions/workflows/ci.yml)\n\n## Introducing FastAPI CRUD Template Generator\nFastAPI CRUD Template Generator is a tool that helps developers quickly scaffold FastAPI projects with CRUD operations for their SQLAlchemy databases. It can provide a starting point for your project and can save time and effort by providing pre-written code of the CRUD operations and validation model, and a pre-defined project structureIt. The generated code can be customized to add business logic and extend the functionality of the FastAPI project. The tool supports the following APIs:\n>- Get one\n>- Get many\n>- Insert one\n>- Insert many\n>- Update one\n>- Update many\n>- Patch one\n>- Patch many\n>- Delete one\n>- Delete many\n\nFastAPI CRUD Generator is the tool for building efficient and scalable APIs with FastAPI and SQLAlchemy. Try it now and start building your next great project.\n\n## DEMO\n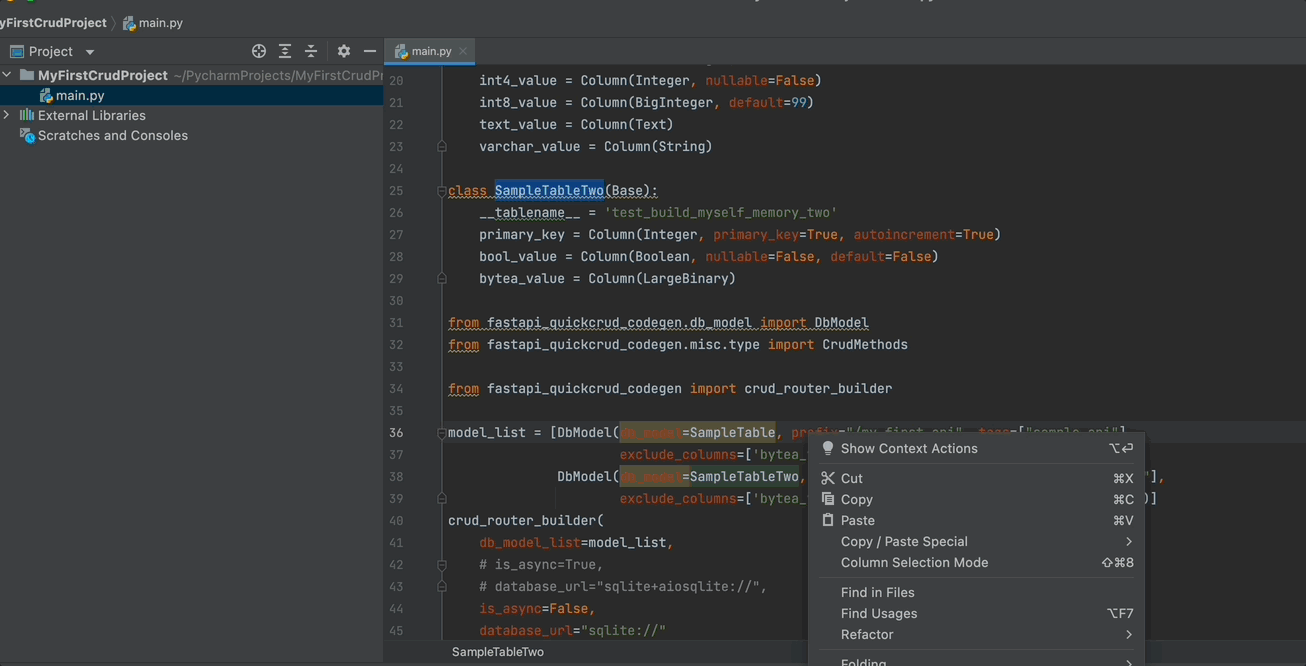\n\n## Features\n* CRUD router code generation from SQLAlchemy declarative class definitions\n* API validation model code generation\n* Support for SQLAlchemy 1.4\n* Pagination support for APIs\n* Flexible API request handling\n\n## Quick Start with in-memory DB (or see the other ([example](https://github.com/LuisLuii/fastapi-crud-template-generator/tree/feature/fix_code_style/tutorial))\n\n\n### Install\nTo get started with FastAPI CRUD Template Generator, you will need to install the package using pip:\n```python\npip install fastapi-crud-code-generator\n```\n### Prepare your Sqlalchemy schema\nNext, you will need to prepare your SQLAlchemy schema by defining your database tables as declarative classes. You can use the [sqlacodegen](https://github.com/agronholm/sqlacodegen) tool to automatically generate these classes from your database.\n\n```\nfrom sqlalchemy import *\nfrom sqlalchemy.orm import declarative_base\n\nBase = declarative_base()\nmetadata = Base.metadata\n\nclass SampleTable(Base):\n __tablename__ = 'test_build_myself_memory'\n __table_args__ = (\n UniqueConstraint('primary_key', 'int4_value', 'float4_value'),\n )\n primary_key = Column(Integer, primary_key=True, autoincrement=True)\n bool_value = Column(Boolean, nullable=False, default=False)\n bytea_value = Column(LargeBinary)\n char_value = Column(CHAR(10, collation='NOCASE'))\n date_value = Column(Date)\n float4_value = Column(Float, nullable=False)\n float8_value = Column(Float(53), nullable=False, default=10.10)\n int2_value = Column(SmallInteger, nullable=False)\n int4_value = Column(Integer, nullable=False)\n int8_value = Column(BigInteger, default=99)\n text_value = Column(Text)\n varchar_value = Column(String)\n\nclass SampleTableTwo(Base):\n __tablename__ = 'test_build_myself_memory_two'\n primary_key = Column(Integer, primary_key=True, autoincrement=True)\n bool_value = Column(Boolean, nullable=False, default=False)\n bytea_value = Column(LargeBinary)\n```\n\n### Generate template\n\nOnce you have your SQLAlchemy schema defined, you can use the `crud_router_builder` method to generate CRUD router code for your API. This method takes the declarative class definitions as input and generates code that defines the endpoints and validation models for each CRUD operation.\n\nHere is an example of how to use the `crud_router_builder` method to generate CRUD router code:\n```python\nfrom fastapi_quickcrud_codegen.db_model import DbModel\nfrom fastapi_quickcrud_codegen.misc.type import CrudMethods\n\nfrom fastapi_quickcrud_codegen import crud_router_builder\n\nmodel_list = [DbModel(db_model=SampleTable, prefix=\"/my_first_api\", tags=[\"sample api\"],\n exclude_columns=['bytea_value']),\n DbModel(db_model=SampleTableTwo, prefix=\"/my_second_api\", tags=[\"sample api\"],\n exclude_columns=['bytea_value'],crud_methods=[CrudMethods.FIND_ONE])]\ncrud_router_builder(\n db_model_list=model_list,\n # is_async=True,\n # database_url=\"sqlite+aiosqlite://\",\n is_async=False,\n database_url=\"sqlite://\"\n)\n\n```\n<img width=\"1292\" alt=\"image\" src=\"https://user-images.githubusercontent.com/31765235/200206184-a16a8622-7db6-4c62-bb56-99cdee126493.png\">\n\n\n**crud_router_builder args**\n- db_model_list `[Required[List[DbModel]]] `\n > Model list of dict for code generate\n - `List[]`\n - `DbModel`\n - db_model `[Required[DeclarativeMeta]]`\n - prefix `[Required[str]]`\n > prefix for Fastapi's end point \n - tags `[Required[List[str]]]`\n > list of tag for Fastapi's end point \n - exclude_columns `[Optional[List[str]]]`\n > set the columns that not to be operated but the columns should nullable or set the default value)\n - crud_methods `[Opional[List[CrudMethods]]]`\n > - Create the following apis for that model, but default: [CrudMethods.FIND_MANY, CrudMethods.FIND_ONE, CrudMethods.CREATE_MANY, CrudMethods.PATCH_ONE, CrudMethods.PATCH_MANY, CrudMethods.PATCH_ONE, CrudMethods.UPDATE_MANY, CrudMethods.UPDATE_ONE, CrudMethods.DELETE_MANY, CrudMethods.DELETE_ONE] \n ```\n - CrudMethods.FIND_ONE\n - CrudMethods.FIND_MANY\n - CrudMethods.UPDATE_ONE\n - CrudMethods.UPDATE_MANY\n - CrudMethods.PATCH_ONE\n - CrudMethods.PATCH_MANY\n - CrudMethods.CREATE_ONE\n - CrudMethods.CREATE_MANY\n - CrudMethods.DELETE_ONE\n - CrudMethods.DELETE_MANY\n ```\n \n- is_async `[Required]`\n \n > True for async; False for sync\n \n- database_url `[Optional (str)]`\n > A database URL. The URL is passed directly to SQLAlchemy's create_engine() method so please refer to SQLAlchemy's documentation for instructions on how to construct a proper URL.\n \n# Known limitations\n* \u274c Please use composite unique constraints instead of multiple unique constraints\n* \u274c Composite primary key is not supported\n* \u274c Sqlalchemy table type model schema is not supported\n\n# Design:\nThe model generation part and api router part refer to my another [project](https://github.com/LuisLuii/FastAPIQuickCRUD); The code generation part is using Jinja\n\n## How to contribute more apis?\nIt will be super excited if you have any idea with api router/ model template. then you can follow the step as below to try to contribute.\n1. Model generation\n 1. Prepare Jinja template to `fastapi-crud-project-generator/src/fastapi_quickcrud_codegen/model/template`\n 2. Prepare model code generation method from `fastapi_quickcrud_codegen.utils.schema_builder.ApiParameterSchemaBuilder`\n 3. Generate the code from `fastapi_quickcrud_codegen/model/model_builder.py` \n2. CRUD Method Generation\n 1. After the model generation, you can try to build your own api by your own model\n 2. Modify `fastapi_quickcrud_codegen.utils.sqlalchemy_to_pydantic.sqlalchemy_to_pydantic`, `fastapi_quickcrud_codegen.misc.type.CrudMethods` and `fastapi_quickcrud_codegen.misc.crud_model.CRUDModel` to let project supports your api\n 3. Prepare your api router Jinja template from `src/fastapi_quickcrud_codegen/model/template/route`\n 4. Generate the code from `fastapi_quickcrud_codegen/model/crud_builder.py` \n 5. Update the api_register from `src/fastapi_quickcrud_codegen/crud_generator.py`\n* use Sqlalchemy's sql.expression instead custom statement when building sql statement for your api\n# Road map\n#### Current State: Stable\n#### Will do:\n>- Support foreign tree in find api\n>- Support foreign tree in insert api\n>- Support foreign tree in update api\n>- Support foreign tree in delete api\n#### Good to have:\n>- Support Sqlalchemy Table type model\n",
"bugtrack_url": null,
"license": "MIT License",
"summary": "FastaAPI's CRUD project generator for SQLALchemy.",
"version": "0.0.31",
"project_urls": {
"Homepage": "https://github.com/LuisLuii/fastapi-crud-template-generator"
},
"split_keywords": [
"fastapi",
"crud",
"restful",
"routing",
"sqlalchemy",
"generator",
"crudrouter",
"postgresql",
"builder"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "ed81a4f95d73796e39fc5666b9fa4e74d14b1c55ff63122866661709d3c62af1",
"md5": "cb5974f56388d2b030cee47780128400",
"sha256": "4116d7e012776f4e448cfb78cf4648925745f80dfd57ca41939b76916dc02a24"
},
"downloads": -1,
"filename": "fastapi_crud_code_generator-0.0.31-py3-none-any.whl",
"has_sig": false,
"md5_digest": "cb5974f56388d2b030cee47780128400",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 46311,
"upload_time": "2024-01-07T15:01:36",
"upload_time_iso_8601": "2024-01-07T15:01:36.229840Z",
"url": "https://files.pythonhosted.org/packages/ed/81/a4f95d73796e39fc5666b9fa4e74d14b1c55ff63122866661709d3c62af1/fastapi_crud_code_generator-0.0.31-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d8d291ed0fc3c9fd5e45ed23402c044bc2ae9e22e40ca58db694204648765121",
"md5": "3142da95f9a64394eb70fc0b967ce94b",
"sha256": "67595fbd0a67d1d9e6ced1f19ea92ec43af47ab81a0ab8ce6667f7e8fa1ec22a"
},
"downloads": -1,
"filename": "fastapi-crud-code-generator-0.0.31.tar.gz",
"has_sig": false,
"md5_digest": "3142da95f9a64394eb70fc0b967ce94b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 60854,
"upload_time": "2024-01-07T15:01:37",
"upload_time_iso_8601": "2024-01-07T15:01:37.856247Z",
"url": "https://files.pythonhosted.org/packages/d8/d2/91ed0fc3c9fd5e45ed23402c044bc2ae9e22e40ca58db694204648765121/fastapi-crud-code-generator-0.0.31.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-01-07 15:01:37",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "LuisLuii",
"github_project": "fastapi-crud-template-generator",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"requirements": [],
"lcname": "fastapi-crud-code-generator"
}