# FastAPI Quick CRUD

[](https://www.codacy.com/gh/LuisLuii/FastAPIQuickCRUD/dashboard?utm_source=github.com&utm_medium=referral&utm_content=LuisLuii/FastAPIQuickCRUD&utm_campaign=Badge_Grade)
[](https://coveralls.io/github/LuisLuii/FastAPIQuickCRUD?branch=main)
[](https://github.com/LuisLuii/FastAPIQuickCRUD/actions/workflows/ci.yml)
[](https://pypi.org/project/fastapi-quickcrud)
[](https://pypi.org/project/fastapi-quickcrud)
[](https://badge.fury.io/py/fastapi-quickcrud)
---
## [If you need apply business logic or add on some code, you can use my another open source project which supports CRUD router code generator](https://github.com/LuisLuii/fastapi-crud-template-generator)
- [Introduction](#introduction)
- [Advantages](#advantages)
- [Limitations](#limitations)
- [Getting started](#getting-started)
- [Installation](#installation)
- [Usage](#usage)
- [Design](#design)
- [Path Parameter](#path-parameter)
- [Query Parameter](#query-parameter)
- [Request Body](#request-body)
- [Foreign Tree](#foreign-tree)
- [Upsert](#upsert)
- [Add description into docs](#add-description-into-docs)
- [Relationship](#relationship)
- [FastAPI_quickcrud Response Status Code standard](#fastapi_quickcrud-response-status-code-standard)
# Introduction
I believe that many people who work with FastApi to build RESTful CRUD services end up wasting time writing repitive boilerplate code.
`FastAPI Quick CRUD` can generate CRUD methods in FastApi from an SQLAlchemy schema:
- Get one
- Get one with foreign key
- Get many
- Get many with foreign key
- Update one
- Update many
- Patch one
- Patch many
- Create/Upsert one
- Create/Upsert many
- Delete One
- Delete Many
- Post Redirect Get
`FastAPI Quick CRUD`is developed based on SQLAlchemy `1.4.23` version and supports sync and async.
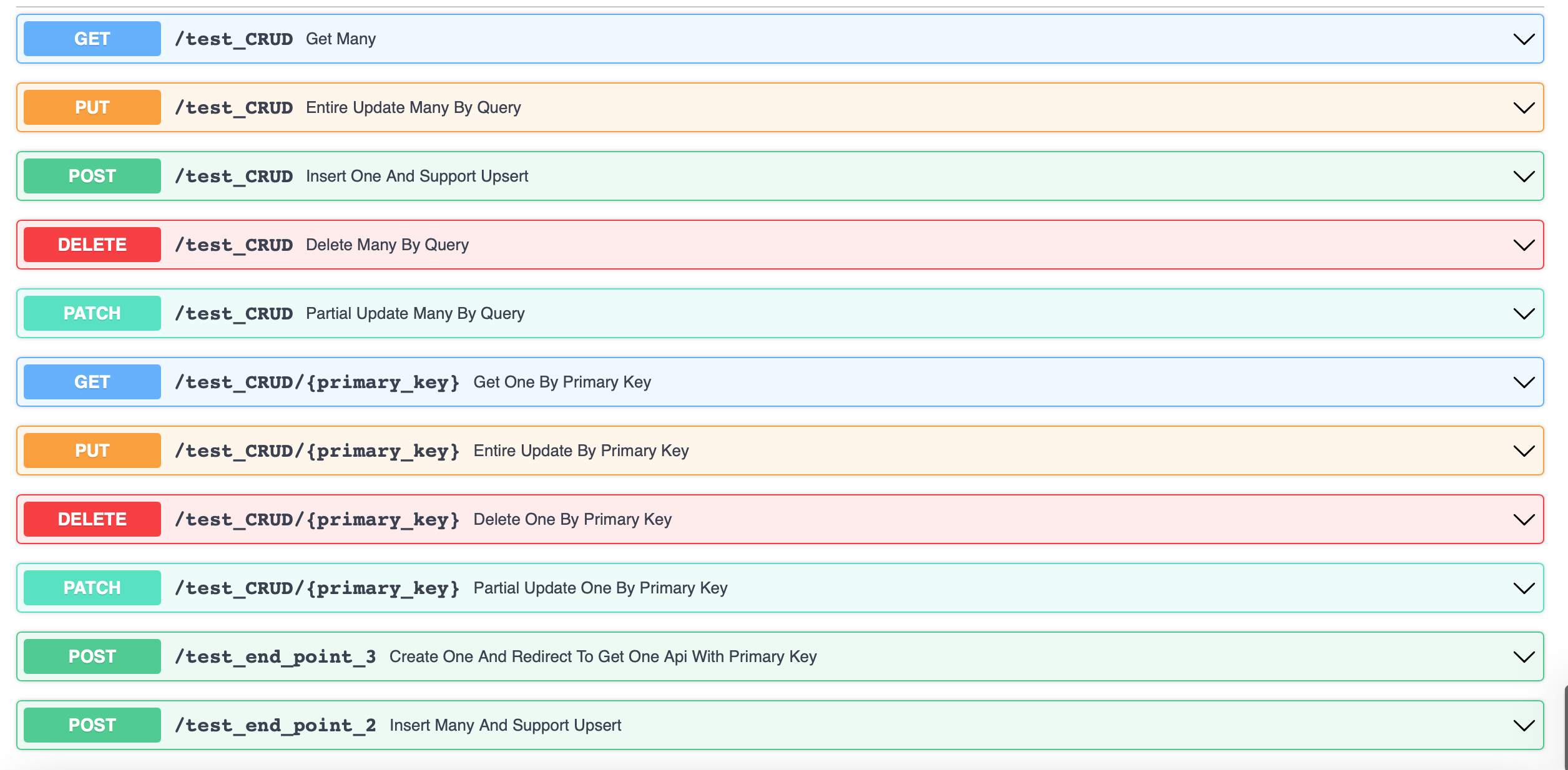
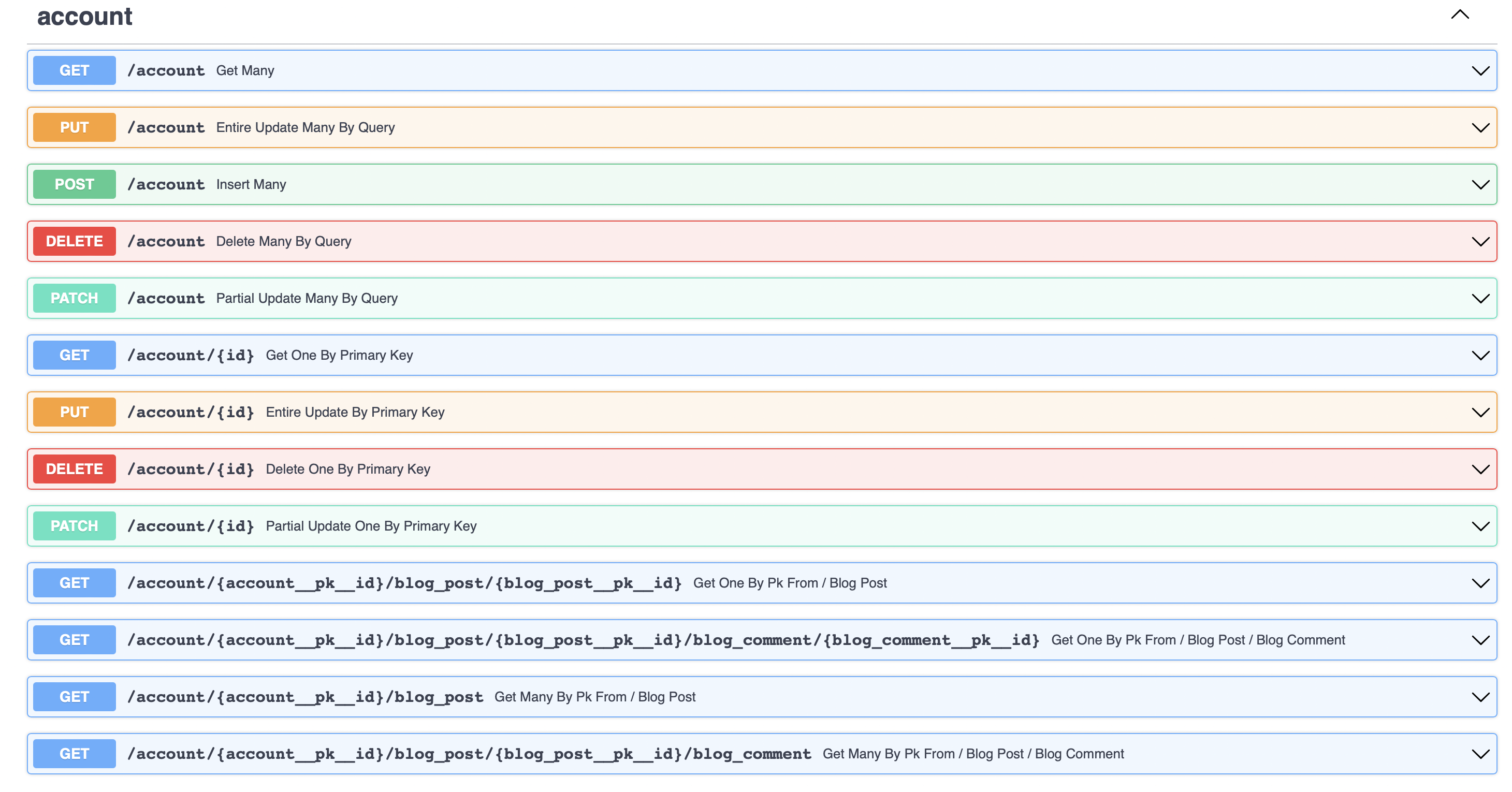
## Advantages
- [x] **Support SQLAlchemy 1.4** - Allows you build a fully asynchronous or synchronous python service
- [x] **Full SQLAlchemy DBAPI Support** - Support different SQL for SQLAlchemy
- [x] **Support Pagination** - `Get many` API support `order by` `offset` `limit` field in API
- [x] **Rich FastAPI CRUD router generation** - Many operations of CRUD are implemented to complete the development and coverage of all aspects of basic CRUD.
- [x] **CRUD route automatically generated** - Support Declarative class definitions and Imperative table
- [x] **Flexible API request** - `UPDATE ONE/MANY` `FIND ONE/MANY` `PATCH ONE/MANY` `DELETE ONE/MANY` supports Path Parameters (primary key) and Query Parameters as a command to the resource to filter and limit the scope of the scope of data in request.
- [x] **SQL Relationship** - `FIND ONE/MANY` supports Path get data with relationship
## Limitations
- ❌ If there are multiple **unique constraints**, please use **composite unique constraints** instead
- ❌ **Composite primary key** is not supported
- ❌ Unsupported API requests with on resources `xxx/{primary key}` for tables without a primary key;
- `UPDATE ONE`
- `FIND ONE`
- `PATCH ONE`
- `DELETE ONE`
# Getting started
##
I try to update the version dependencies as soon as possible to ensure that the core dependencies of this project have the highest version possible.
```bash
fastapi<=0.68.2
pydantic<=1.8.2
SQLAlchemy<=1.4.30
starlette==0.14.2
```
## Installation
```bash
pip install fastapi-quickcrud
```
I suggest the following library if you try to connect to PostgreSQL
```bash
pip install psycopg2
pip install asyncpg
```
## Usage
run and go to http://127.0.0.1:port/docs and see the auto-generated API
### Simple Code (or see the longer ([example](https://github.com/LuisLuii/FastAPIQuickCRUD/blob/main/tutorial/sample.py))
```python
from fastapi import FastAPI
from sqlalchemy import Column, Integer, \
String, Table, ForeignKey, orm
from fastapi_quickcrud import crud_router_builder
Base = orm.declarative_base()
class User(Base):
__tablename__ = 'test_users'
id = Column(Integer, primary_key=True, autoincrement=True, unique=True)
name = Column(String, nullable=False)
email = Column(String, nullable=False)
friend = Table(
'test_friend', Base.metadata,
Column('id', ForeignKey('test_users.id', ondelete='CASCADE', onupdate='CASCADE'), nullable=False),
Column('friend_name', String, nullable=False)
)
crud_route_1 = crud_router_builder(db_model=User,
prefix="/user",
tags=["User"],
async_mode=True
)
crud_route_2 = crud_router_builder(db_model=friend,
prefix="/friend",
tags=["friend"],
async_mode=True
)
app = FastAPI()
app.include_router(crud_route_1)
app.include_router(crud_route_2)
```
### Foreign Tree With Relationship
```python
from fastapi import FastAPI
from fastapi_quickcrud import crud_router_builder
from sqlalchemy import *
from sqlalchemy.orm import *
from fastapi_quickcrud.crud_router import generic_sql_crud_router_builder
Base = declarative_base()
class Account(Base):
__tablename__ = "account"
id = Column(Integer, primary_key=True, autoincrement=True)
blog_post = relationship("BlogPost", back_populates="account")
class BlogPost(Base):
__tablename__ = "blog_post"
id = Column(Integer, primary_key=True, autoincrement=True)
account_id = Column(Integer, ForeignKey("account.id"), nullable=False)
account = relationship("Account", back_populates="blog_post")
blog_comment = relationship("BlogComment", back_populates="blog_post")
class BlogComment(Base):
__tablename__ = "blog_comment"
id = Column(Integer, primary_key=True, autoincrement=True)
blog_id = Column(Integer, ForeignKey("blog_post.id"), nullable=False)
blog_post = relationship("BlogPost", back_populates="blog_comment")
crud_route_parent = crud_router_builder(
db_model=Account,
prefix="/account",
tags=["account"],
foreign_include=[BlogComment, BlogPost]
)
crud_route_child1 = generic_sql_crud_router_builder(
db_model=BlogPost,
prefix="/blog_post",
tags=["blog_post"],
foreign_include=[BlogComment]
)
crud_route_child2 = generic_sql_crud_router_builder(
db_model=BlogComment,
prefix="/blog_comment",
tags=["blog_comment"]
)
app = FastAPI()
[app.include_router(i) for i in [crud_route_parent, crud_route_child1, crud_route_child2]]
```
### SQLAlchemy to Pydantic Model Converter And Build your own API([example](https://github.com/LuisLuii/FastAPIQuickCRUD/blob/main/tutorial/basic_usage/quick_usage_with_async_SQLALchemy_Base.py))
```python
import uvicorn
from fastapi import FastAPI, Depends
from fastapi_quickcrud import CrudMethods
from fastapi_quickcrud import sqlalchemy_to_pydantic
from fastapi_quickcrud.misc.memory_sql import sync_memory_db
from sqlalchemy import CHAR, Column, Integer
from sqlalchemy.ext.declarative import declarative_base
app = FastAPI()
Base = declarative_base()
metadata = Base.metadata
class Child(Base):
__tablename__ = 'right'
id = Column(Integer, primary_key=True)
name = Column(CHAR, nullable=True)
friend_model_set = sqlalchemy_to_pydantic(db_model=Child,
crud_methods=[
CrudMethods.FIND_MANY,
CrudMethods.UPSERT_MANY,
CrudMethods.UPDATE_MANY,
CrudMethods.DELETE_MANY,
CrudMethods.CREATE_ONE,
CrudMethods.PATCH_MANY,
],
exclude_columns=[])
post_model = friend_model_set.POST[CrudMethods.CREATE_ONE]
sync_memory_db.create_memory_table(Child)
@app.post("/hello",
status_code=201,
tags=["Child"],
response_model=post_model.responseModel,
dependencies=[])
async def my_api(
query: post_model.requestBodyModel = Depends(post_model.requestBodyModel),
session=Depends(sync_memory_db.get_memory_db_session)
):
db_item = Child(**query.__dict__)
session.add(db_item)
session.commit()
session.refresh(db_item)
return db_item.__dict__
uvicorn.run(app, host="0.0.0.0", port=8000, debug=False)
```
* Note:
you can use [sqlacodegen](https://github.com/agronholm/sqlacodegen) to generate SQLAlchemy models for your table. This project is based on the model development and testing generated by sqlacodegen
### Main module
#### Generate CRUD router
**crud_router_builder args**
- db_session [Optional] `execute session generator`
- default using in-memory db with create table automatically
- example:
- sync SQLALchemy:
```python
from sqlalchemy.orm import sessionmaker
def get_transaction_session():
try:
db = sessionmaker(...)
yield db
except Exception as e:
db.rollback()
raise e
finally:
db.close()
```
- Async SQLALchemy
```python
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.asyncio import AsyncSession
async_session = sessionmaker(autocommit=False,
autoflush=False,
bind=engine,
class_=AsyncSession)
async def get_transaction_session() -> AsyncSession:
async with async_session() as session:
async with session.begin():
yield session
```
- db_model [Require] `SQLALchemy Declarative Base Class or Table`
> **Note**: There are some constraint in the SQLALchemy Schema
- async_mode [Optional (auto set by db_session)] `bool`: if your db session is async
> **Note**: require async session generator if True
- autocommit [Optional (default True)] `bool`: if you don't need to commit by your self
> **Note**: require handle the commit in your async session generator if False
- dependencies [Optional]: API dependency injection of fastapi
> **Note**: Get the example usage in `./example`
- crud_methods: ```CrudMethods```
> - CrudMethods.FIND_ONE
> - CrudMethods.FIND_MANY
> - CrudMethods.UPDATE_ONE
> - CrudMethods.UPDATE_MANY
> - CrudMethods.PATCH_ONE
> - CrudMethods.PATCH_MANY
> - CrudMethods.UPSERT_ONE (only support postgresql yet)
> - CrudMethods.UPSERT_MANY (only support postgresql yet)
> - CrudMethods.CREATE_ONE
> - CrudMethods.CREATE_MANY
> - CrudMethods.DELETE_ONE
> - CrudMethods.DELETE_MANY
> - CrudMethods.POST_REDIRECT_GET
- exclude_columns: `list`
> set the columns that not to be operated but the columns should nullable or set the default value)
- foreign_include: `list[declarative_base()]`
> add the SqlAlchemy models here, and build the foreign tree get one/many api (don't support SqlAlchemy table)
- dynamic argument (prefix, tags): extra argument for APIRouter() of fastapi
# Design
In `PUT` `DELETE` `PATCH`, user can use Path Parameters and Query Parameters to limit the scope of the data affected by the operation, and the Query Parameters is same with `FIND` API
## Path Parameter
In the design of this tool, **Path Parameters** should be a primary key of table, that why limited primary key can only be one.
## Query Parameter
- Query Operation will look like that when python type of column is
<details>
<summary>string</summary>
- **support Approximate String Matching that require this**
- (<column_name>____str, <column_name>____str_____matching_pattern)
- **support In-place Operation, get the value of column in the list of input**
- (<column_name>____list, <column_name>____list____comparison_operator)
- **preview**
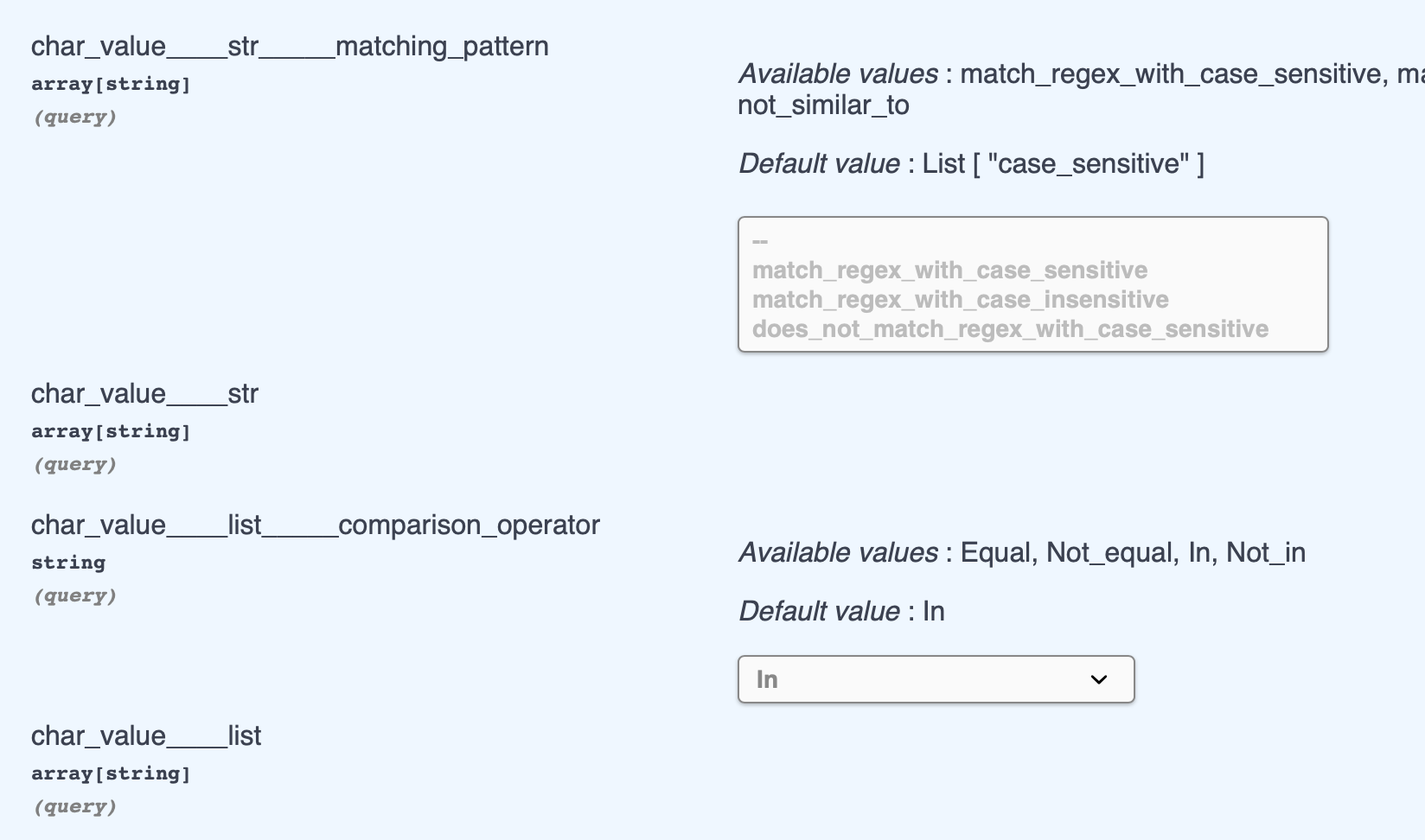
</details>
<details>
<summary>numeric or datetime</summary>
- **support Range Searching from and to**
- (<column_name>____from, <column_name>____from_____comparison_operator)
- (<column_name>____to, <column_name>____to_____comparison_operator)
- **support In-place Operation, get the value of column in the list of input**
- (<column_name>____list, <column_name>____list____comparison_operator)
- **preview**
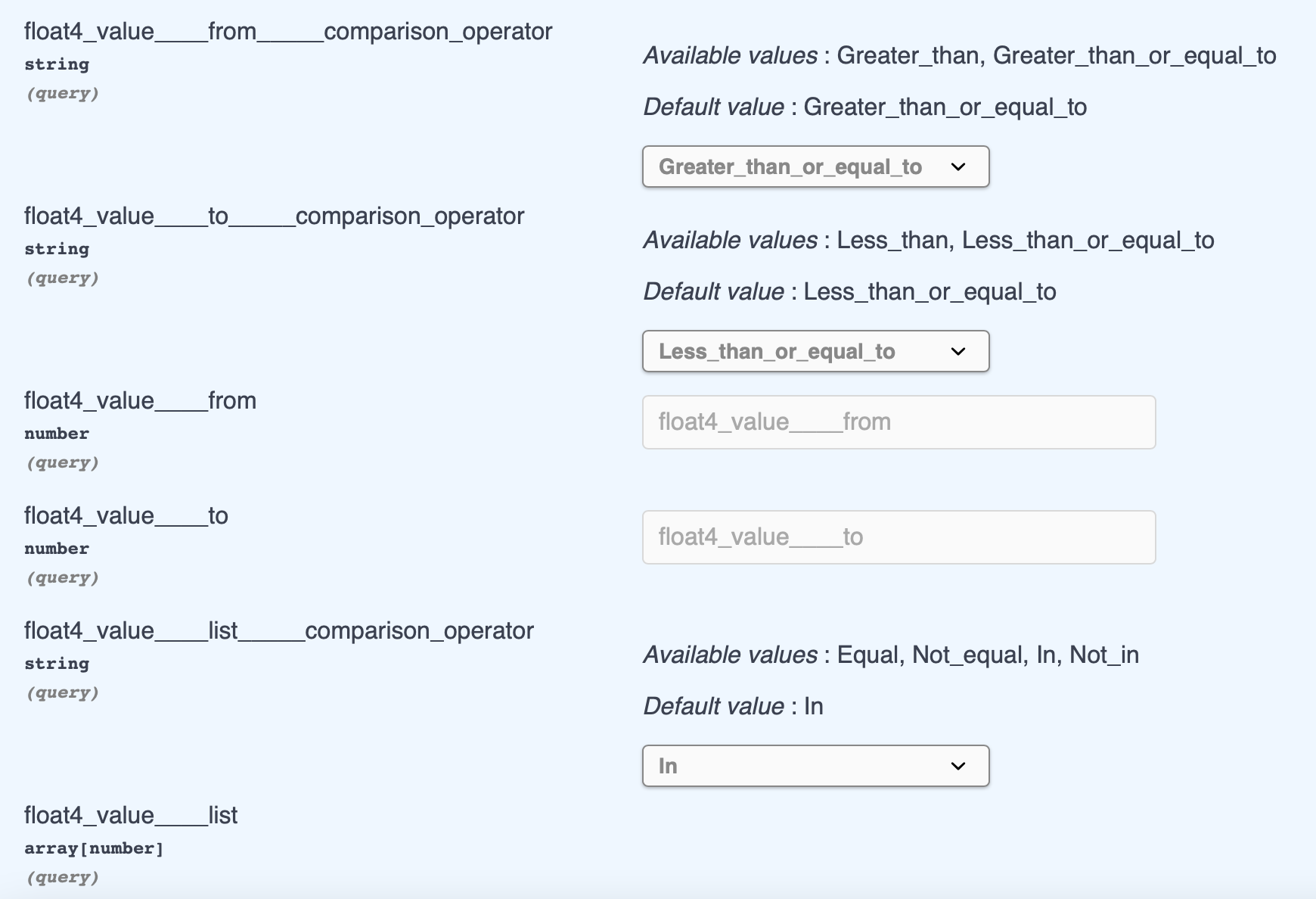
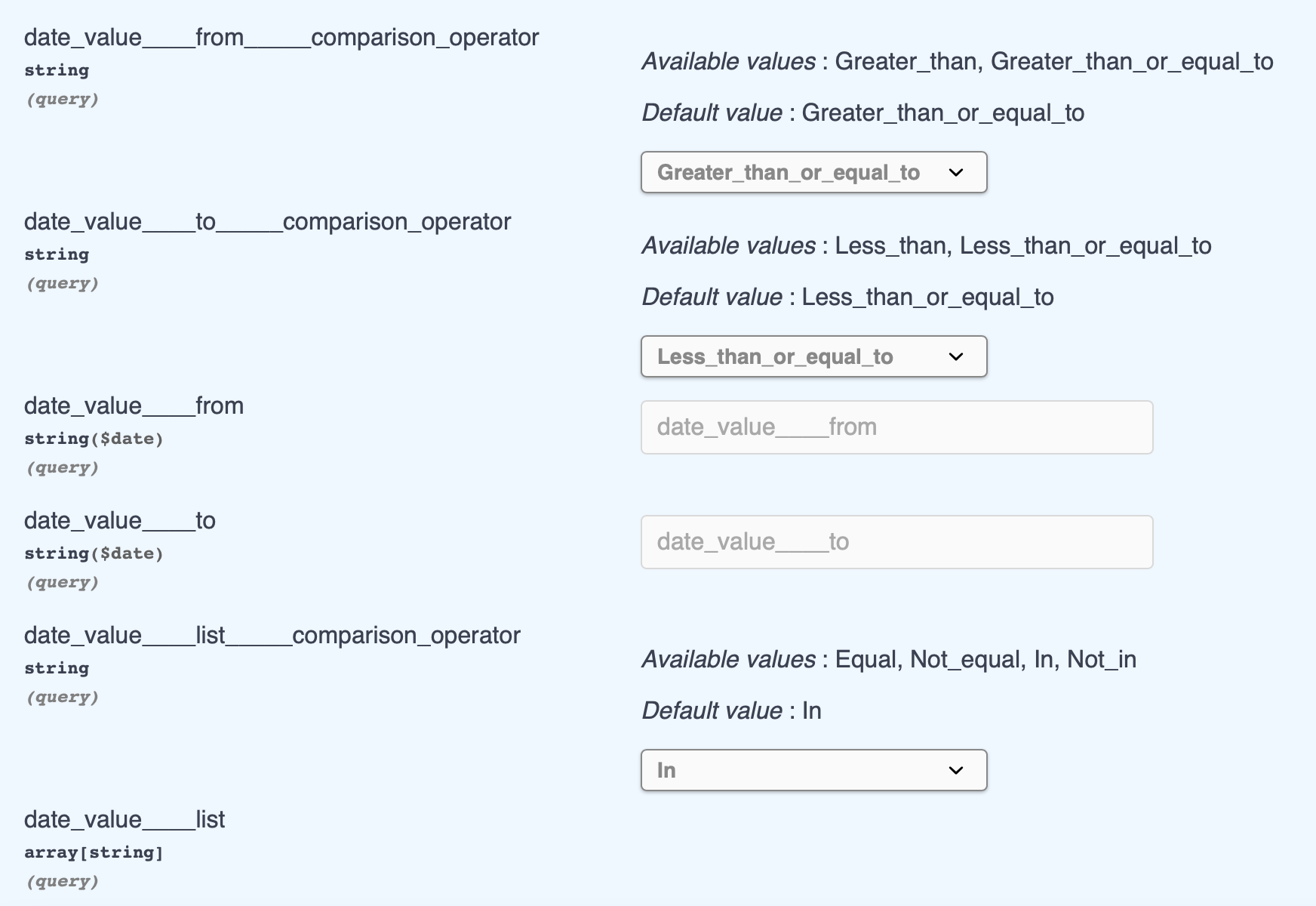
</details>
<details>
<summary>uuid</summary>
uuid supports In-place Operation only
- **support In-place Operation, get the value of column in the list of input**
- (<column_name>____list, <column_name>____list____comparison_operator)
</details>
- EXTRA query parameter for `GET_MANY`:
<details>
<summary>Pagination</summary>
- **limit**
- **offset**
- **order by**
- **preview**
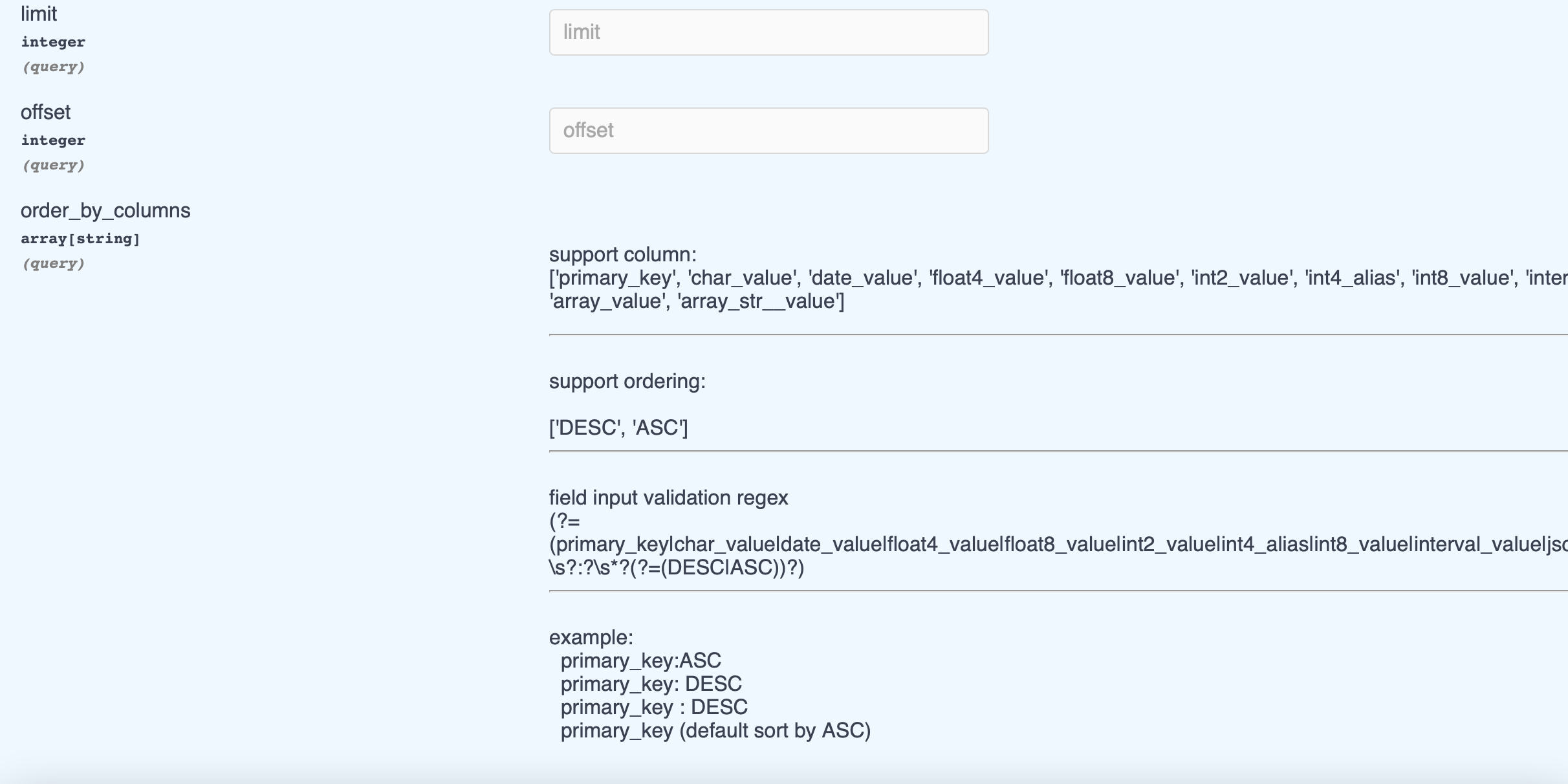
</details>
### Query to SQL statement example
- [**Approximate String Matching**](https://www.postgresql.org/docs/9.3/functions-matching.html)
<details>
<summary>example</summary>
- request url
```text
/test_CRUD?
char_value____str_____matching_pattern=match_regex_with_case_sensitive&
char_value____str_____matching_pattern=does_not_match_regex_with_case_insensitive&
char_value____str_____matching_pattern=case_sensitive&
char_value____str_____matching_pattern=not_case_insensitive&
char_value____str=a&
char_value____str=b
```
- generated sql
```sql
SELECT *
FROM untitled_table_256
WHERE (untitled_table_256.char_value ~ 'a') OR
(untitled_table_256.char_value ~ 'b' OR
(untitled_table_256.char_value !~* 'a') OR
(untitled_table_256.char_value !~* 'b' OR
untitled_table_256.char_value LIKE 'a' OR
untitled_table_256.char_value LIKE 'b' OR
untitled_table_256.char_value NOT ILIKE 'a'
OR untitled_table_256.char_value NOT ILIKE 'b'
```
</details>
- **In-place Operation**
<details>
<summary>example</summary>
- In-place support the following operation
- generated sql if user select Equal operation and input True and False
- preview
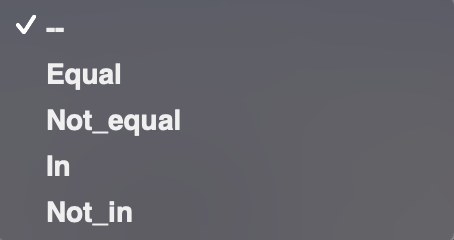
- generated sql
```sql
select * FROM untitled_table_256
WHERE untitled_table_256.bool_value = true OR
untitled_table_256.bool_value = false
```
</details>
- **Range Searching**
<details>
<summary>example</summary>
- Range Searching support the following operation
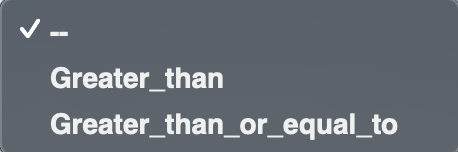
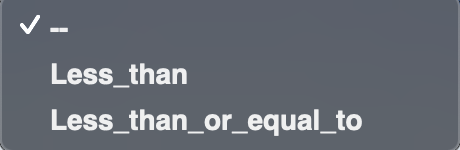
- generated sql
```sql
select * from untitled_table_256
WHERE untitled_table_256.date_value > %(date_value_1)s
```
```sql
select * from untitled_table_256
WHERE untitled_table_256.date_value < %(date_value_1)s
```
</details>
- Also support your custom dependency for each api(there is a example in `./example`)
### Request Body
In the design of this tool, the columns of the table will be used as the fields of request body.
In the basic request body in the api generated by this tool, some fields are optional if :
* [x] it is primary key with `autoincrement` is True or the `server_default` or `default` is True
* [x] it is not a primary key, but the `server_default` or `default` is True
* [x] The field is nullable
### Foreign Tree
TBC
## Upsert
** Upsert supports PosgreSQL only yet
POST API will perform the data insertion action with using the basic [Request Body](#request-body),
In addition, it also supports upsert(insert on conflict do)
The operation will use upsert instead if the unique column in the inserted row that is being inserted already exists in the table
The tool uses `unique columns` in the table as a parameter of on conflict , and you can define which column will be updated
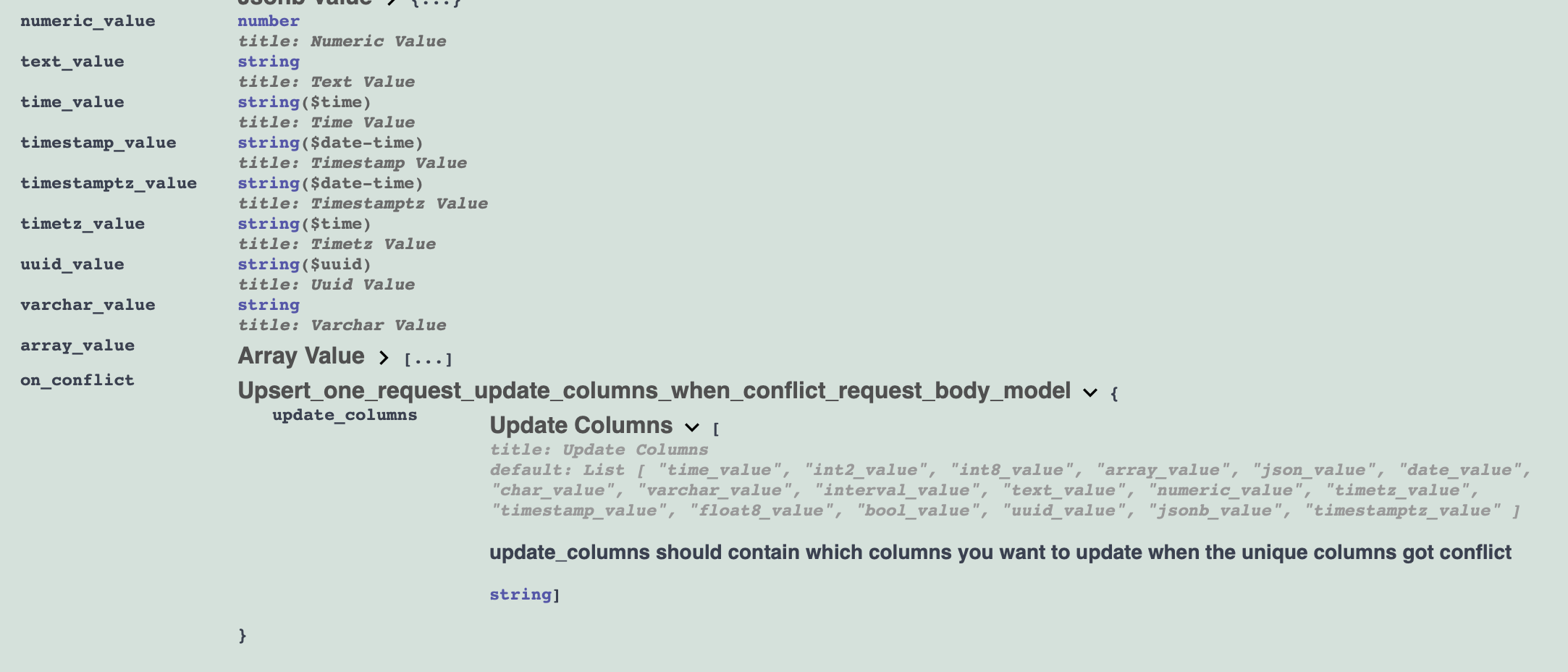
## Add description into docs
You can declare `comment` argument for `sqlalchemy.Column` to configure the description of column
example:
```python
class Parent(Base):
__tablename__ = 'parent_o2o'
id = Column(Integer, primary_key=True,comment='parent_test')
# one-to-many collection
children = relationship("Child", back_populates="parent")
class Child(Base):
__tablename__ = 'child_o2o'
id = Column(Integer, primary_key=True,comment='child_pk_test')
parent_id = Column(Integer, ForeignKey('parent_o2o.id'),info=({'description':'child_parent_id_test'}))
# many-to-one scalar
parent = relationship("Parent", back_populates="children")
```
## Relationship
Now, `FIND_ONE` and `FIND_MANY` are supporting select data with join operation
```python
class Parent(Base):
__tablename__ = 'parent_o2o'
id = Column(Integer, primary_key=True)
children = relationship("Child", back_populates="parent")
class Child(Base):
__tablename__ = 'child_o2o'
id = Column(Integer, primary_key=True)
parent_id = Column(Integer, ForeignKey('parent_o2o.id'))
parent = relationship("Parent", back_populates="children")
```
there is a relationship with using back_populates between Parent table and Child table, the `parent_id` in `Child` will refer to `id` column in `Parent`.
`FastApi Quick CRUD` will generate an api with a `join_foreign_table` field, and get api will respond to your selection of the reference data row of the corresponding table in `join_foreign_table` field,

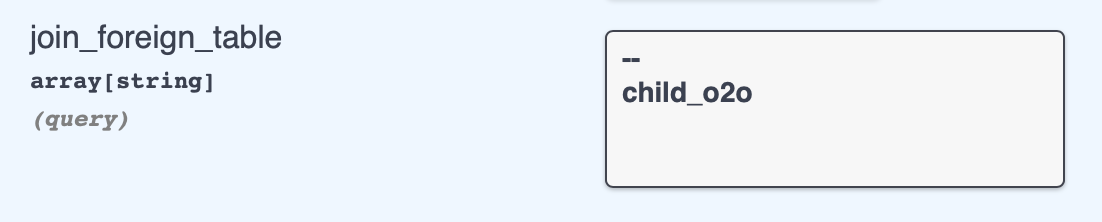
* Try Request
now there are some data in these two table

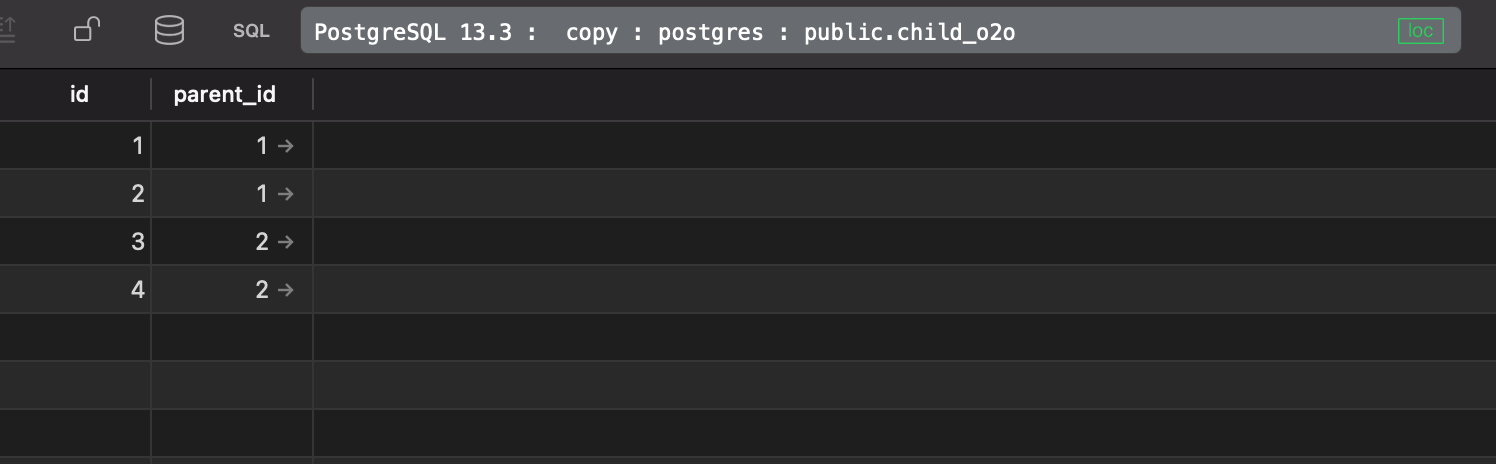
when i request
* Case One
```commandline
curl -X 'GET' \
'http://0.0.0.0:8000/parent?join_foreign_table=child_o2o' \
-H 'accept: application/json'
```
Response data
```json
[
{
"id_foreign": [
{
"id": 1,
"parent_id": 1
},
{
"id": 2,
"parent_id": 1
}
],
"id": 1
},
{
"id_foreign": [
{
"id": 3,
"parent_id": 2
},
{
"id": 4,
"parent_id": 2
}
],
"id": 2
}
]
```
Response headers
```text
x-total-count: 4
```
There are response 4 data, response data will be grouped by the parent row, if the child refer to the same parent row
* Case Two
```commandline
curl -X 'GET' \
'http://0.0.0.0:8000/child?join_foreign_table=parent_o2o' \
-H 'accept: application/json'
```
Response data
```json
[
{
"parent_id_foreign": [
{
"id": 1
}
],
"id": 1,
"parent_id": 1
},
{
"parent_id_foreign": [
{
"id": 1
}
],
"id": 2,
"parent_id": 1
},
{
"parent_id_foreign": [
{
"id": 2
}
],
"id": 3,
"parent_id": 2
},
{
"parent_id_foreign": [
{
"id": 2
}
],
"id": 4,
"parent_id": 2
}
]
```
Response Header
```text
x-total-count: 4
```
#### FastAPI_quickcrud Response Status Code standard
When you ask for a specific resource, say a user or with query param, and the user doesn't exist
```GET: get one : https://0.0.0.0:8080/api/:userid?xx=xx```
```UPDATE: update one : https://0.0.0.0:8080/api/:userid?xx=xx```
```PATCH: patch one : https://0.0.0.0:8080/api/:userid?xx=xx```
```DELETE: delete one : https://0.0.0.0:8080/api/:userid?xx=xx```
then fastapi-qucikcrud should return 404. In this case, the client requested a resource that doesn't exist.
----
In the other case, you have an api that operate data on batch in the system using the following url:
```GET: get many : https://0.0.0.0:8080/api/user?xx=xx```
```UPDATE: update many : https://0.0.0.0:8080/api/user?xx=xx```
```DELETE: delete many : https://0.0.0.0:8080/api/user?xx=xx```
```PATCH: patch many : https://0.0.0.0:8080/api/user?xx=xx```
If there are no users in the system, then, in this case, you should return 204.
### TODO
[milestones](https://github.com/LuisLuii/FastAPIQuickCRUD/milestones)
Raw data
{
"_id": null,
"home_page": "https://github.com/LuisLuii/FastAPIQuickCRUD",
"name": "fastapi-quickcrud",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "",
"keywords": "fastapi,crud,restful,routing,SQLAlchemy,generator,crudrouter,postgresql,builder",
"author": "Luis Lui",
"author_email": "luis11235178@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/27/37/0ddb85d685095c431b318a5044761ecf9a46dabfa2a09ce339e4ad8914ce/fastapi_quickcrud-0.2.8.tar.gz",
"platform": null,
"description": "# FastAPI Quick CRUD\n\n\n\n[](https://www.codacy.com/gh/LuisLuii/FastAPIQuickCRUD/dashboard?utm_source=github.com&utm_medium=referral&utm_content=LuisLuii/FastAPIQuickCRUD&utm_campaign=Badge_Grade)\n[](https://coveralls.io/github/LuisLuii/FastAPIQuickCRUD?branch=main)\n[](https://github.com/LuisLuii/FastAPIQuickCRUD/actions/workflows/ci.yml)\n[](https://pypi.org/project/fastapi-quickcrud)\n[](https://pypi.org/project/fastapi-quickcrud)\n[](https://badge.fury.io/py/fastapi-quickcrud)\n\n\n---\n\n## [If you need apply business logic or add on some code, you can use my another open source project which supports CRUD router code generator](https://github.com/LuisLuii/fastapi-crud-template-generator)\n\n\n- [Introduction](#introduction)\n - [Advantages](#advantages)\n - [Limitations](#limitations)\n- [Getting started](#getting-started)\n - [Installation](#installation)\n - [Usage](#usage)\n- [Design](#design)\n - [Path Parameter](#path-parameter)\n - [Query Parameter](#query-parameter)\n - [Request Body](#request-body)\n - [Foreign Tree](#foreign-tree)\n - [Upsert](#upsert)\n - [Add description into docs](#add-description-into-docs)\n - [Relationship](#relationship)\n - [FastAPI_quickcrud Response Status Code standard](#fastapi_quickcrud-response-status-code-standard)\n \n\n\n# Introduction\n\nI believe that many people who work with FastApi to build RESTful CRUD services end up wasting time writing repitive boilerplate code.\n\n`FastAPI Quick CRUD` can generate CRUD methods in FastApi from an SQLAlchemy schema:\n\n- Get one\n- Get one with foreign key \n- Get many\n- Get many with foreign key\n- Update one\n- Update many\n- Patch one\n- Patch many\n- Create/Upsert one\n- Create/Upsert many\n- Delete One\n- Delete Many\n- Post Redirect Get\n\n`FastAPI Quick CRUD`is developed based on SQLAlchemy `1.4.23` version and supports sync and async.\n\n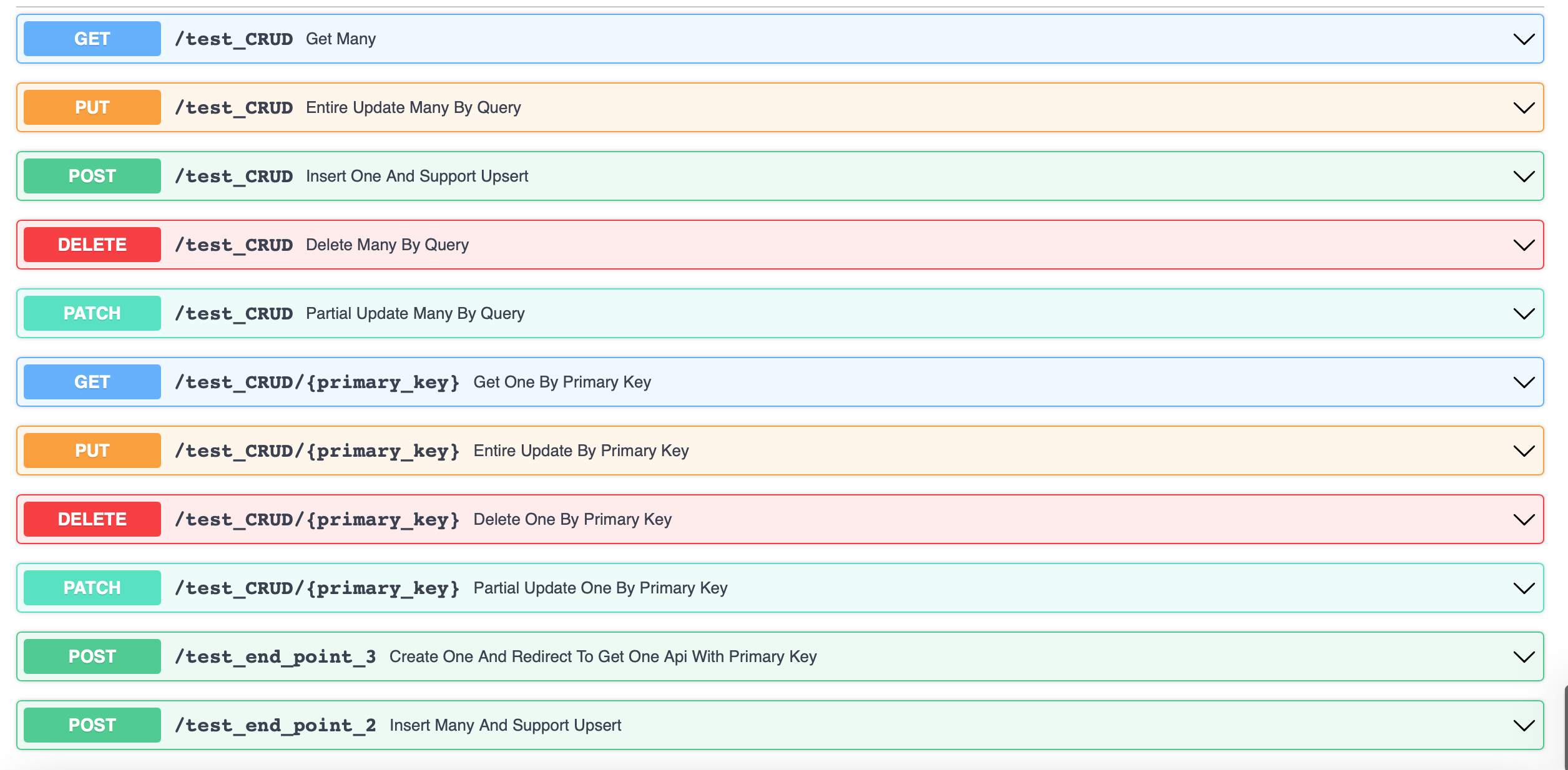\n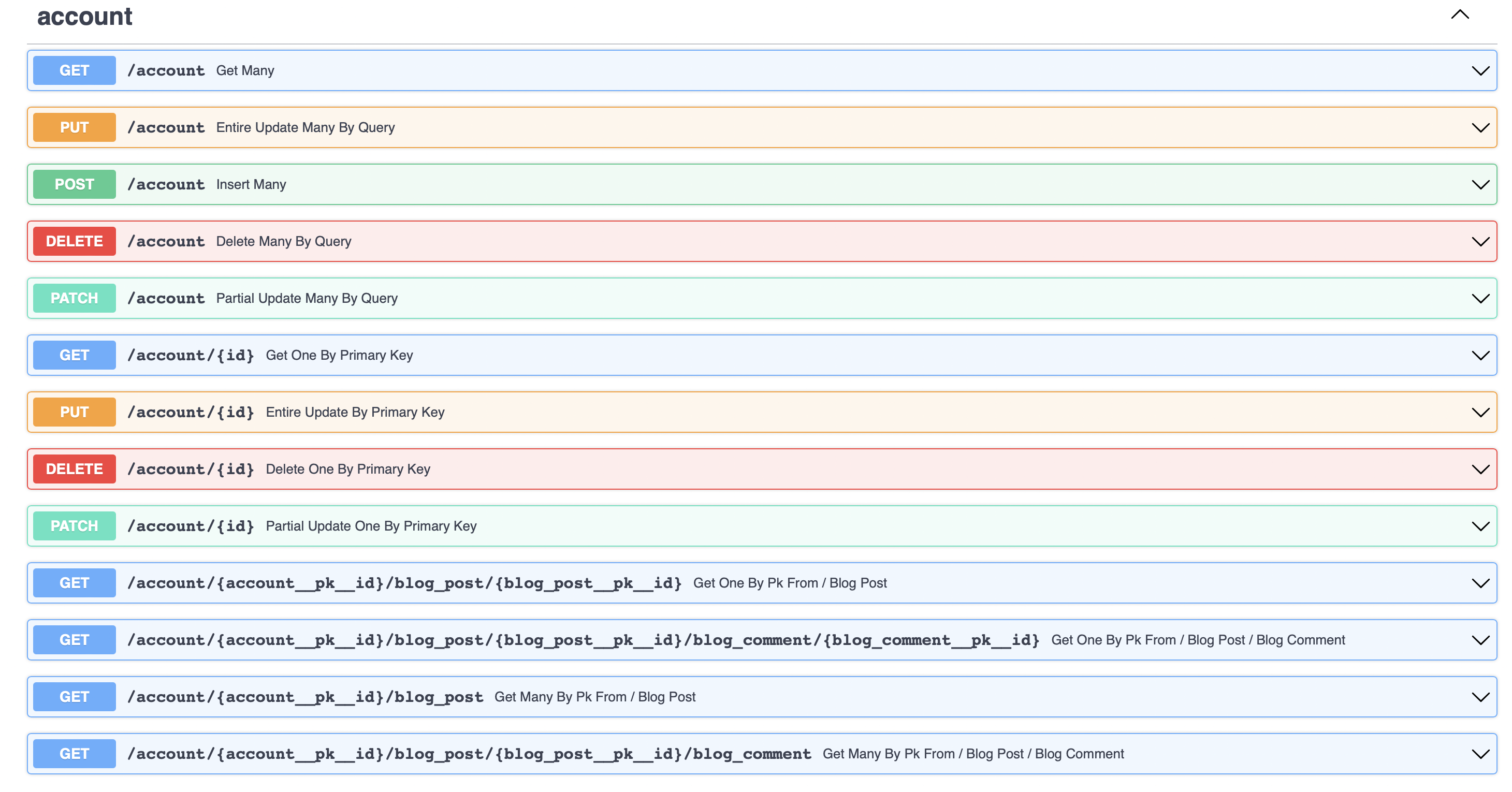\n\n\n\n## Advantages\n\n - [x] **Support SQLAlchemy 1.4** - Allows you build a fully asynchronous or synchronous python service\n \n - [x] **Full SQLAlchemy DBAPI Support** - Support different SQL for SQLAlchemy\n \n - [x] **Support Pagination** - `Get many` API support `order by` `offset` `limit` field in API\n\n - [x] **Rich FastAPI CRUD router generation** - Many operations of CRUD are implemented to complete the development and coverage of all aspects of basic CRUD.\n\n - [x] **CRUD route automatically generated** - Support Declarative class definitions and Imperative table\n \n - [x] **Flexible API request** - `UPDATE ONE/MANY` `FIND ONE/MANY` `PATCH ONE/MANY` `DELETE ONE/MANY` supports Path Parameters (primary key) and Query Parameters as a command to the resource to filter and limit the scope of the scope of data in request.\n \n - [x] **SQL Relationship** - `FIND ONE/MANY` supports Path get data with relationship\n \n## Limitations\n \n - \u274c If there are multiple **unique constraints**, please use **composite unique constraints** instead\n - \u274c **Composite primary key** is not supported\n - \u274c Unsupported API requests with on resources `xxx/{primary key}` for tables without a primary key; \n - `UPDATE ONE`\n - `FIND ONE`\n - `PATCH ONE` \n - `DELETE ONE` \n \n\n# Getting started\n\n##\nI try to update the version dependencies as soon as possible to ensure that the core dependencies of this project have the highest version possible.\n```bash\nfastapi<=0.68.2\npydantic<=1.8.2\nSQLAlchemy<=1.4.30\nstarlette==0.14.2\n```\n\n## Installation\n\n```bash\npip install fastapi-quickcrud\n```\n\nI suggest the following library if you try to connect to PostgreSQL \n```bash\npip install psycopg2\npip install asyncpg\n```\n\n## Usage\nrun and go to http://127.0.0.1:port/docs and see the auto-generated API\n### Simple Code (or see the longer ([example](https://github.com/LuisLuii/FastAPIQuickCRUD/blob/main/tutorial/sample.py))\n\n\n```python\nfrom fastapi import FastAPI\nfrom sqlalchemy import Column, Integer, \\\n String, Table, ForeignKey, orm\nfrom fastapi_quickcrud import crud_router_builder\n\nBase = orm.declarative_base()\n\n\nclass User(Base):\n __tablename__ = 'test_users'\n id = Column(Integer, primary_key=True, autoincrement=True, unique=True)\n name = Column(String, nullable=False)\n email = Column(String, nullable=False)\n\n\nfriend = Table(\n 'test_friend', Base.metadata,\n Column('id', ForeignKey('test_users.id', ondelete='CASCADE', onupdate='CASCADE'), nullable=False),\n Column('friend_name', String, nullable=False)\n)\n\ncrud_route_1 = crud_router_builder(db_model=User,\n prefix=\"/user\",\n tags=[\"User\"],\n async_mode=True\n )\ncrud_route_2 = crud_router_builder(db_model=friend,\n prefix=\"/friend\",\n tags=[\"friend\"],\n async_mode=True\n )\n\napp = FastAPI()\napp.include_router(crud_route_1)\napp.include_router(crud_route_2)\n```\n\n### Foreign Tree With Relationship\n```python\nfrom fastapi import FastAPI\nfrom fastapi_quickcrud import crud_router_builder\nfrom sqlalchemy import *\nfrom sqlalchemy.orm import *\nfrom fastapi_quickcrud.crud_router import generic_sql_crud_router_builder\n\nBase = declarative_base()\n\nclass Account(Base):\n __tablename__ = \"account\"\n id = Column(Integer, primary_key=True, autoincrement=True)\n blog_post = relationship(\"BlogPost\", back_populates=\"account\")\n\n\nclass BlogPost(Base):\n __tablename__ = \"blog_post\"\n id = Column(Integer, primary_key=True, autoincrement=True)\n account_id = Column(Integer, ForeignKey(\"account.id\"), nullable=False)\n account = relationship(\"Account\", back_populates=\"blog_post\")\n blog_comment = relationship(\"BlogComment\", back_populates=\"blog_post\")\n\n\nclass BlogComment(Base):\n __tablename__ = \"blog_comment\"\n id = Column(Integer, primary_key=True, autoincrement=True)\n blog_id = Column(Integer, ForeignKey(\"blog_post.id\"), nullable=False)\n blog_post = relationship(\"BlogPost\", back_populates=\"blog_comment\")\n\n\ncrud_route_parent = crud_router_builder(\n db_model=Account,\n prefix=\"/account\",\n tags=[\"account\"],\n foreign_include=[BlogComment, BlogPost]\n\n)\ncrud_route_child1 = generic_sql_crud_router_builder(\n db_model=BlogPost,\n prefix=\"/blog_post\",\n tags=[\"blog_post\"],\n foreign_include=[BlogComment]\n\n)\ncrud_route_child2 = generic_sql_crud_router_builder(\n db_model=BlogComment,\n prefix=\"/blog_comment\",\n tags=[\"blog_comment\"]\n\n)\n\napp = FastAPI()\n[app.include_router(i) for i in [crud_route_parent, crud_route_child1, crud_route_child2]]\n\n```\n\n### SQLAlchemy to Pydantic Model Converter And Build your own API([example](https://github.com/LuisLuii/FastAPIQuickCRUD/blob/main/tutorial/basic_usage/quick_usage_with_async_SQLALchemy_Base.py))\n```python\nimport uvicorn\nfrom fastapi import FastAPI, Depends\nfrom fastapi_quickcrud import CrudMethods\nfrom fastapi_quickcrud import sqlalchemy_to_pydantic\nfrom fastapi_quickcrud.misc.memory_sql import sync_memory_db\n\nfrom sqlalchemy import CHAR, Column, Integer\nfrom sqlalchemy.ext.declarative import declarative_base\n\napp = FastAPI()\n\nBase = declarative_base()\nmetadata = Base.metadata\n\n\nclass Child(Base):\n __tablename__ = 'right'\n id = Column(Integer, primary_key=True)\n name = Column(CHAR, nullable=True)\n\n\nfriend_model_set = sqlalchemy_to_pydantic(db_model=Child,\n crud_methods=[\n CrudMethods.FIND_MANY,\n CrudMethods.UPSERT_MANY,\n CrudMethods.UPDATE_MANY,\n CrudMethods.DELETE_MANY,\n CrudMethods.CREATE_ONE,\n CrudMethods.PATCH_MANY,\n\n ],\n exclude_columns=[])\n\n\npost_model = friend_model_set.POST[CrudMethods.CREATE_ONE]\nsync_memory_db.create_memory_table(Child)\n\n@app.post(\"/hello\",\n status_code=201,\n tags=[\"Child\"],\n response_model=post_model.responseModel,\n dependencies=[])\nasync def my_api(\n query: post_model.requestBodyModel = Depends(post_model.requestBodyModel),\n session=Depends(sync_memory_db.get_memory_db_session)\n):\n db_item = Child(**query.__dict__)\n session.add(db_item)\n session.commit()\n session.refresh(db_item)\n return db_item.__dict__\n\n\n\nuvicorn.run(app, host=\"0.0.0.0\", port=8000, debug=False)\n\n```\n\n* Note:\n you can use [sqlacodegen](https://github.com/agronholm/sqlacodegen) to generate SQLAlchemy models for your table. This project is based on the model development and testing generated by sqlacodegen\n\n### Main module\n\n#### Generate CRUD router\n\n**crud_router_builder args**\n- db_session [Optional] `execute session generator` \n - default using in-memory db with create table automatically\n - example:\n - sync SQLALchemy:\n ```python\n from sqlalchemy.orm import sessionmaker\n def get_transaction_session():\n try:\n db = sessionmaker(...)\n yield db\n except Exception as e:\n db.rollback()\n raise e\n finally:\n db.close()\n ```\n - Async SQLALchemy\n ```python\n from sqlalchemy.orm import sessionmaker\n from sqlalchemy.ext.asyncio import AsyncSession\n async_session = sessionmaker(autocommit=False,\n autoflush=False,\n bind=engine,\n class_=AsyncSession)\n\n async def get_transaction_session() -> AsyncSession:\n async with async_session() as session:\n async with session.begin():\n yield session\n ```\n- db_model [Require] `SQLALchemy Declarative Base Class or Table`\n \n > **Note**: There are some constraint in the SQLALchemy Schema\n \n- async_mode [Optional (auto set by db_session)] `bool`: if your db session is async\n \n > **Note**: require async session generator if True\n \n- autocommit [Optional (default True)] `bool`: if you don't need to commit by your self \n \n > **Note**: require handle the commit in your async session generator if False\n \n- dependencies [Optional]: API dependency injection of fastapi\n \n > **Note**: Get the example usage in `./example` \n\n- crud_methods: ```CrudMethods```\n > - CrudMethods.FIND_ONE\n > - CrudMethods.FIND_MANY\n > - CrudMethods.UPDATE_ONE\n > - CrudMethods.UPDATE_MANY\n > - CrudMethods.PATCH_ONE\n > - CrudMethods.PATCH_MANY\n > - CrudMethods.UPSERT_ONE (only support postgresql yet)\n > - CrudMethods.UPSERT_MANY (only support postgresql yet)\n > - CrudMethods.CREATE_ONE\n > - CrudMethods.CREATE_MANY\n > - CrudMethods.DELETE_ONE\n > - CrudMethods.DELETE_MANY\n > - CrudMethods.POST_REDIRECT_GET\n\n- exclude_columns: `list` \n > set the columns that not to be operated but the columns should nullable or set the default value)\n\n- foreign_include: `list[declarative_base()]` \n > add the SqlAlchemy models here, and build the foreign tree get one/many api (don't support SqlAlchemy table)\n\n\n- dynamic argument (prefix, tags): extra argument for APIRouter() of fastapi\n\n\n# Design\n\nIn `PUT` `DELETE` `PATCH`, user can use Path Parameters and Query Parameters to limit the scope of the data affected by the operation, and the Query Parameters is same with `FIND` API\n\n## Path Parameter\n\nIn the design of this tool, **Path Parameters** should be a primary key of table, that why limited primary key can only be one.\n\n## Query Parameter\n\n- Query Operation will look like that when python type of column is \n <details>\n <summary>string</summary>\n \n - **support Approximate String Matching that require this** \n - (<column_name>____str, <column_name>____str_____matching_pattern)\n - **support In-place Operation, get the value of column in the list of input**\n - (<column_name>____list, <column_name>____list____comparison_operator)\n - **preview**\n 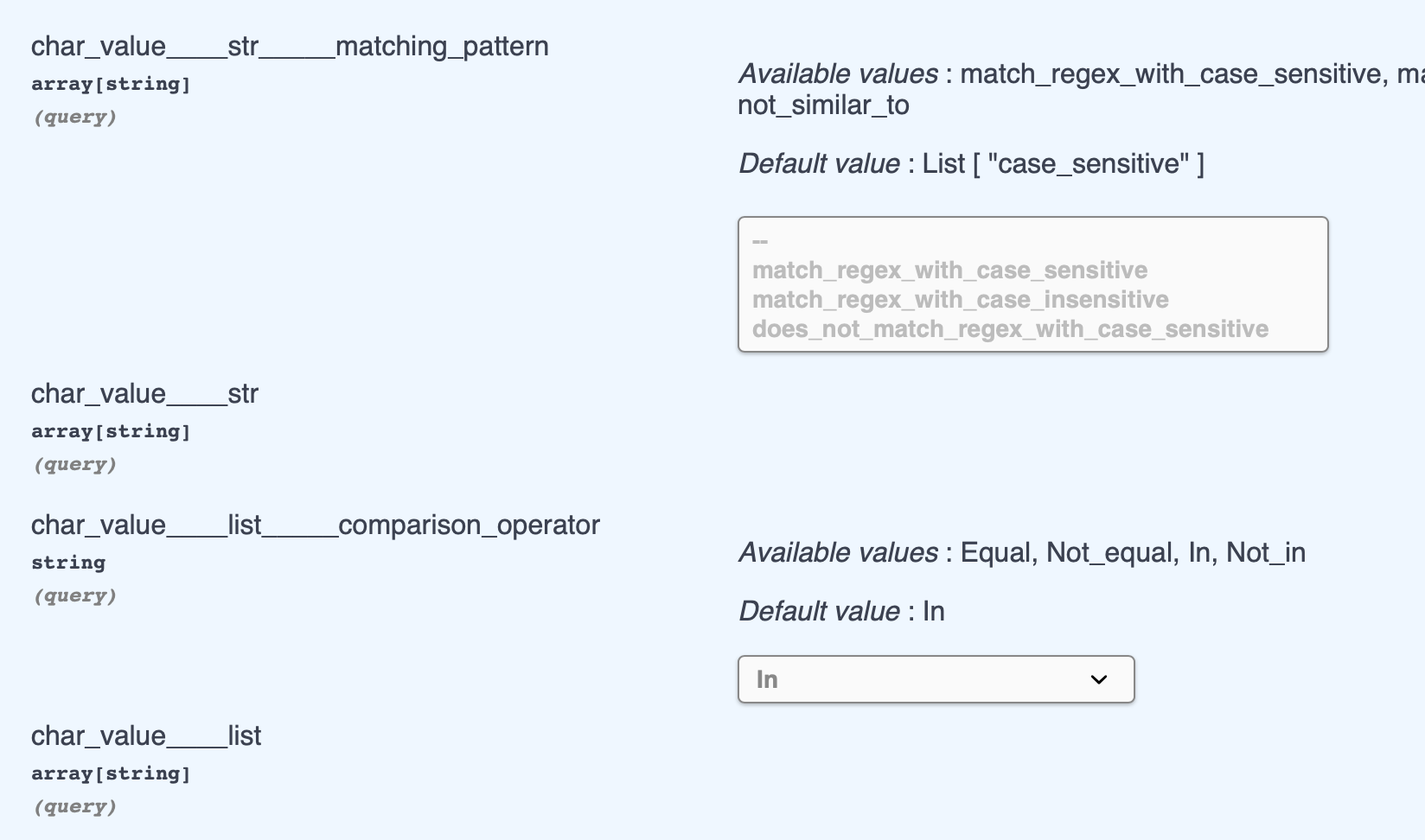\n </details>\n \n <details>\n <summary>numeric or datetime</summary>\n \n - **support Range Searching from and to**\n - (<column_name>____from, <column_name>____from_____comparison_operator)\n - (<column_name>____to, <column_name>____to_____comparison_operator)\n - **support In-place Operation, get the value of column in the list of input**\n - (<column_name>____list, <column_name>____list____comparison_operator)\n - **preview**\n 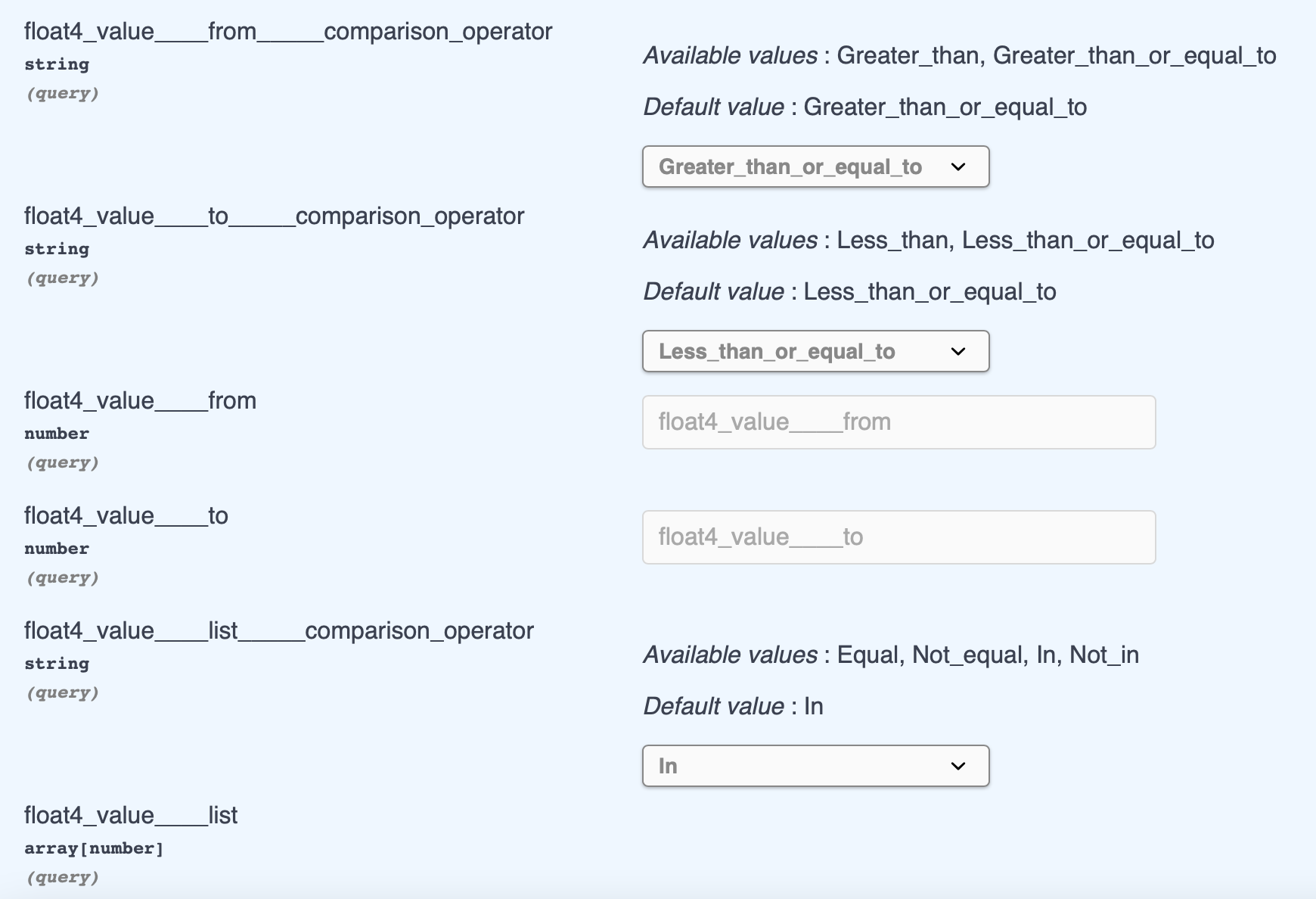\n 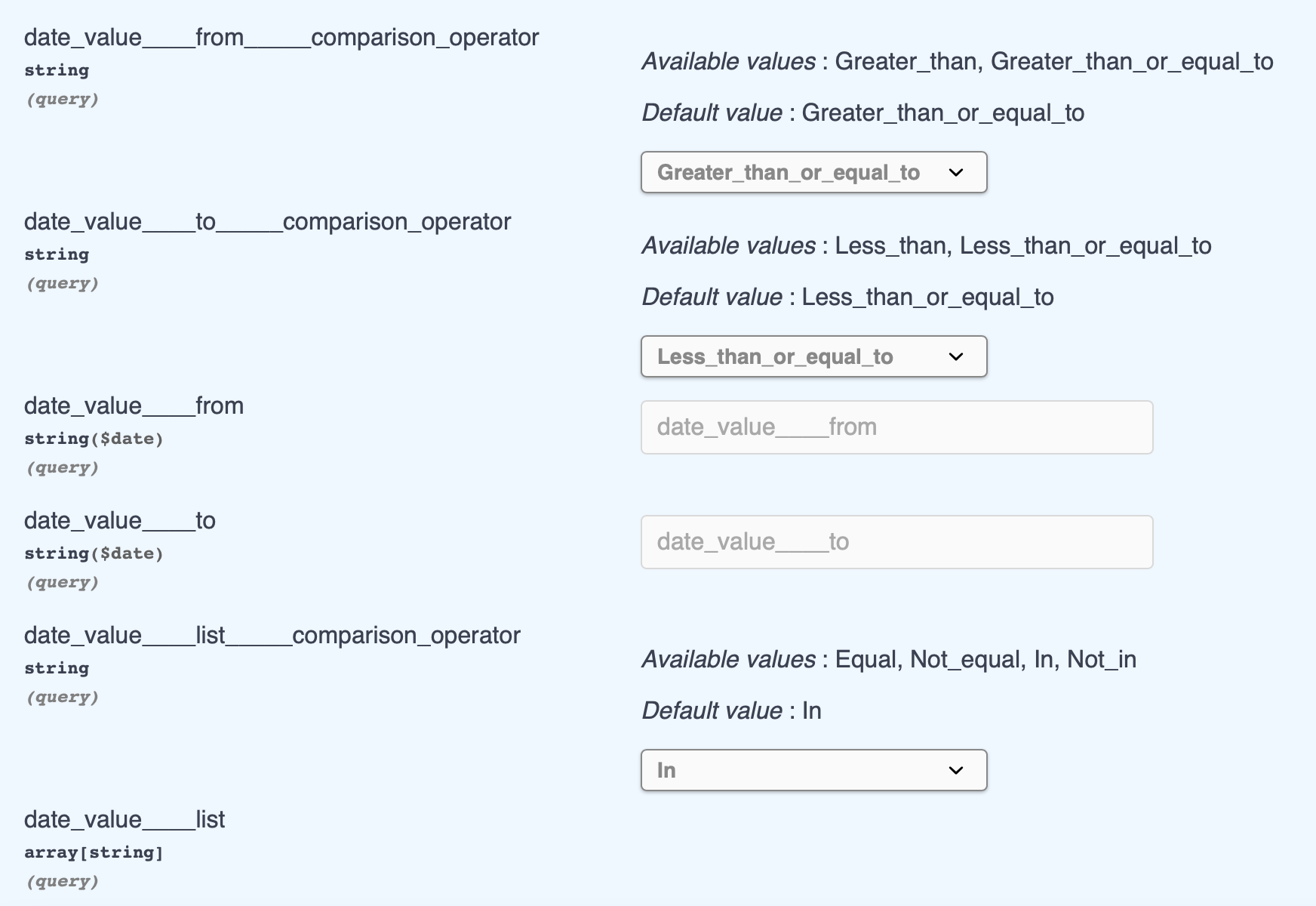\n </details>\n\n <details>\n <summary>uuid</summary>\n \n uuid supports In-place Operation only\n - **support In-place Operation, get the value of column in the list of input**\n - (<column_name>____list, <column_name>____list____comparison_operator)\n </details>\n\n\n- EXTRA query parameter for `GET_MANY`: \n <details>\n <summary>Pagination</summary>\n \n - **limit**\n - **offset**\n - **order by**\n - **preview**\n 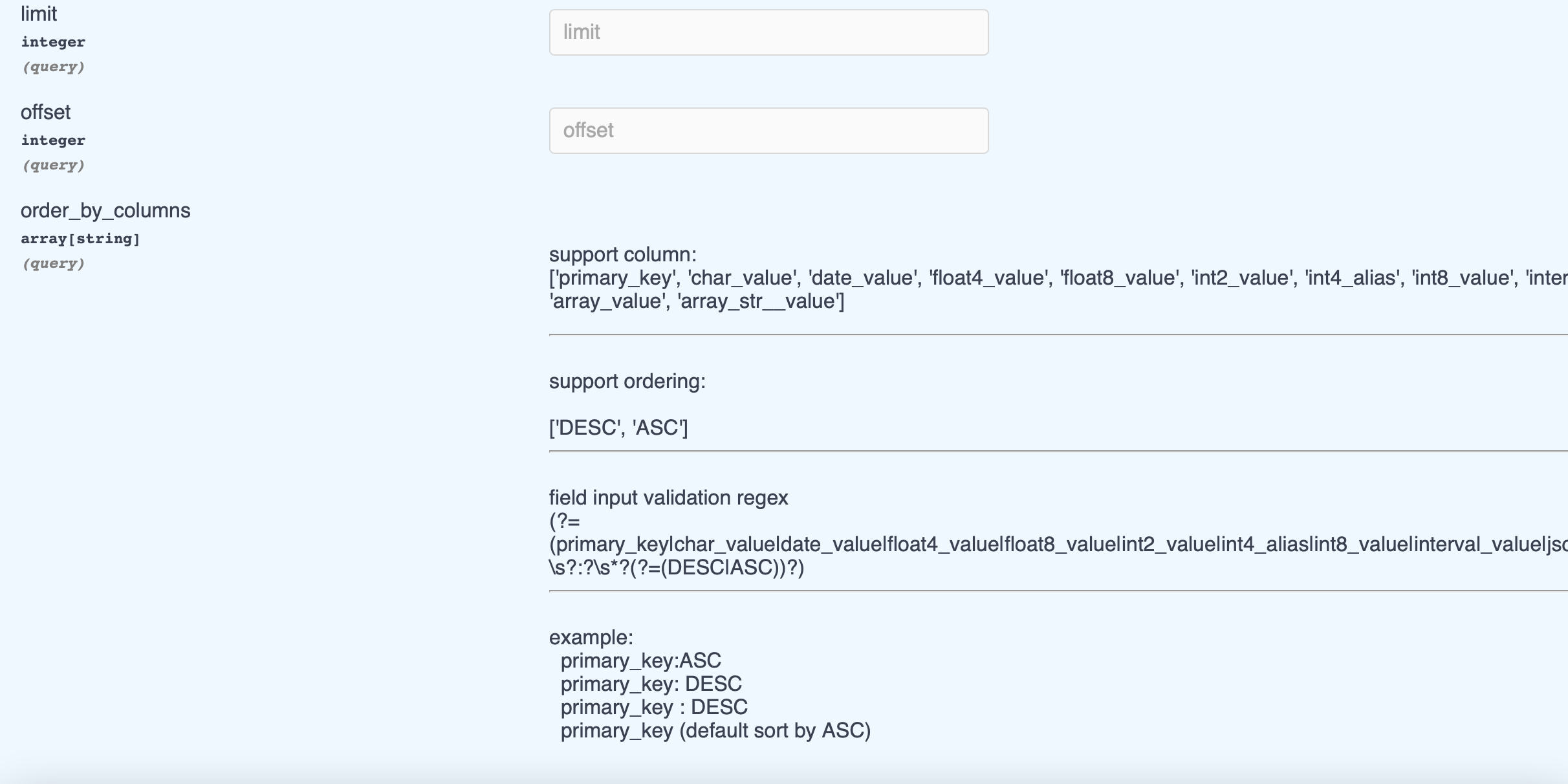 \n\n </details>\n\n \n### Query to SQL statement example\n\n- [**Approximate String Matching**](https://www.postgresql.org/docs/9.3/functions-matching.html)\n <details>\n <summary>example</summary>\n \n - request url\n ```text\n /test_CRUD?\n char_value____str_____matching_pattern=match_regex_with_case_sensitive&\n char_value____str_____matching_pattern=does_not_match_regex_with_case_insensitive&\n char_value____str_____matching_pattern=case_sensitive&\n char_value____str_____matching_pattern=not_case_insensitive&\n char_value____str=a&\n char_value____str=b\n ```\n - generated sql\n ```sql\n SELECT *\n FROM untitled_table_256 \n WHERE (untitled_table_256.char_value ~ 'a') OR \n (untitled_table_256.char_value ~ 'b' OR \n (untitled_table_256.char_value !~* 'a') OR \n (untitled_table_256.char_value !~* 'b' OR \n untitled_table_256.char_value LIKE 'a' OR \n untitled_table_256.char_value LIKE 'b' OR \n untitled_table_256.char_value NOT ILIKE 'a' \n OR untitled_table_256.char_value NOT ILIKE 'b'\n ```\n </details>\n\n \n- **In-place Operation**\n <details>\n <summary>example</summary>\n \n - In-place support the following operation\n - generated sql if user select Equal operation and input True and False\n - preview\n 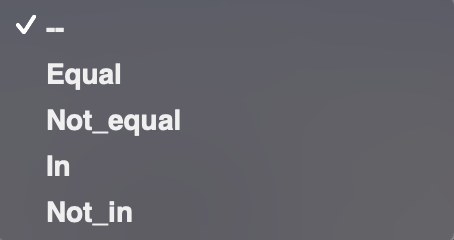 \n\n - generated sql\n ```sql \n select * FROM untitled_table_256 \n WHERE untitled_table_256.bool_value = true OR \n untitled_table_256.bool_value = false\n ``` \n \n </details> \n\n\n- **Range Searching**\n <details>\n <summary>example</summary>\n \n - Range Searching support the following operation\n \n 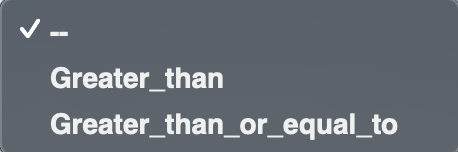 \n \n 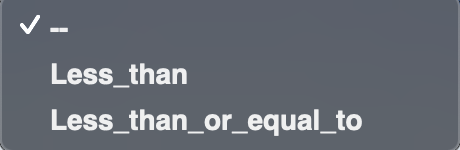\n - generated sql \n ```sql\n select * from untitled_table_256\n WHERE untitled_table_256.date_value > %(date_value_1)s \n ```\n ```sql\n select * from untitled_table_256\n WHERE untitled_table_256.date_value < %(date_value_1)s \n ```\n \n </details>\n \n\n- Also support your custom dependency for each api(there is a example in `./example`)\n\n\n### Request Body\n\nIn the design of this tool, the columns of the table will be used as the fields of request body.\n\nIn the basic request body in the api generated by this tool, some fields are optional if :\n\n* [x] it is primary key with `autoincrement` is True or the `server_default` or `default` is True\n* [x] it is not a primary key, but the `server_default` or `default` is True\n* [x] The field is nullable\n\n\n### Foreign Tree\nTBC\n\n\n\n## Upsert\n\n** Upsert supports PosgreSQL only yet\n\nPOST API will perform the data insertion action with using the basic [Request Body](#request-body),\nIn addition, it also supports upsert(insert on conflict do)\n\nThe operation will use upsert instead if the unique column in the inserted row that is being inserted already exists in the table \n\nThe tool uses `unique columns` in the table as a parameter of on conflict , and you can define which column will be updated \n\n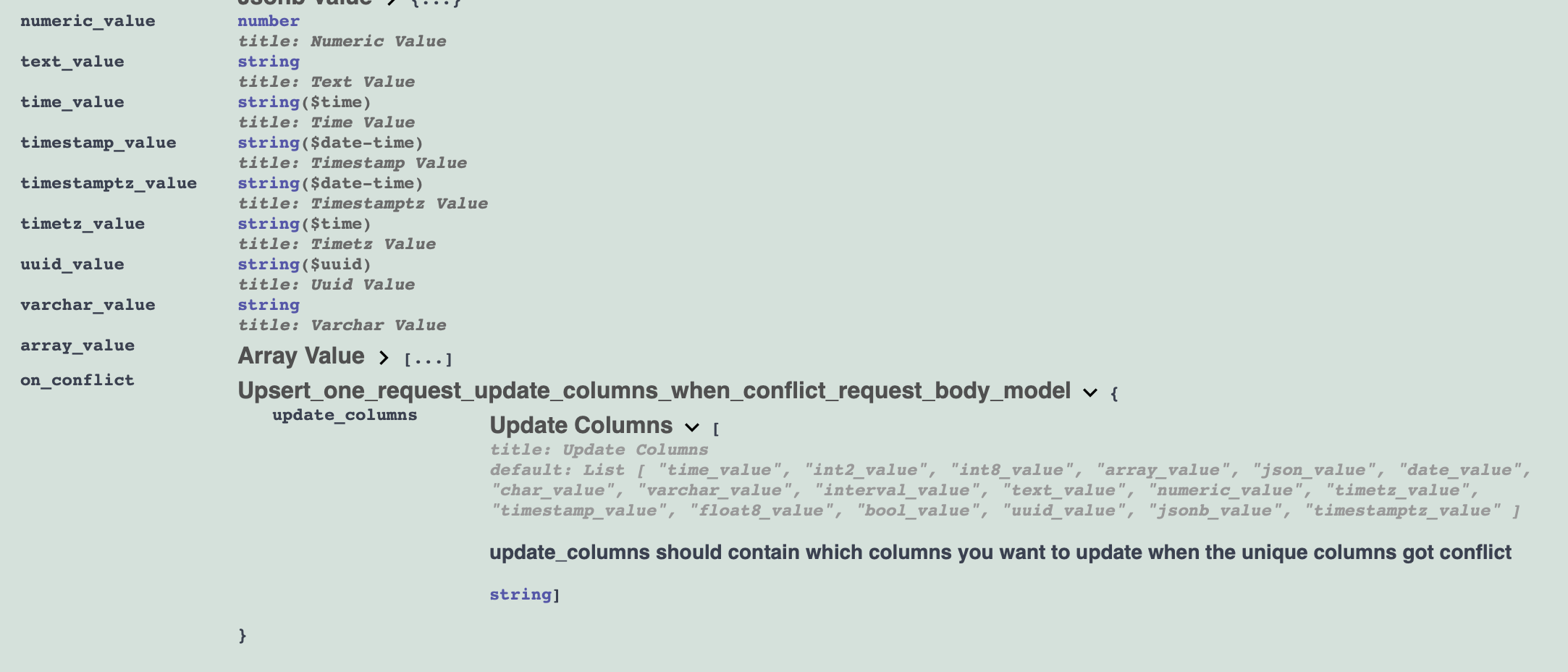\n\n## Add description into docs\n\nYou can declare `comment` argument for `sqlalchemy.Column` to configure the description of column\n\nexample:\n\n```python\n\nclass Parent(Base):\n __tablename__ = 'parent_o2o'\n id = Column(Integer, primary_key=True,comment='parent_test')\n\n # one-to-many collection\n children = relationship(\"Child\", back_populates=\"parent\")\n\nclass Child(Base):\n __tablename__ = 'child_o2o'\n id = Column(Integer, primary_key=True,comment='child_pk_test')\n parent_id = Column(Integer, ForeignKey('parent_o2o.id'),info=({'description':'child_parent_id_test'}))\n\n # many-to-one scalar\n parent = relationship(\"Parent\", back_populates=\"children\")\n```\n\n\n## Relationship\n\nNow, `FIND_ONE` and `FIND_MANY` are supporting select data with join operation\n```python\n\nclass Parent(Base):\n __tablename__ = 'parent_o2o'\n id = Column(Integer, primary_key=True)\n children = relationship(\"Child\", back_populates=\"parent\")\n\nclass Child(Base):\n __tablename__ = 'child_o2o'\n id = Column(Integer, primary_key=True)\n parent_id = Column(Integer, ForeignKey('parent_o2o.id'))\n parent = relationship(\"Parent\", back_populates=\"children\")\n```\nthere is a relationship with using back_populates between Parent table and Child table, the `parent_id` in `Child` will refer to `id` column in `Parent`.\n\n`FastApi Quick CRUD` will generate an api with a `join_foreign_table` field, and get api will respond to your selection of the reference data row of the corresponding table in `join_foreign_table` field, \n\n\n\n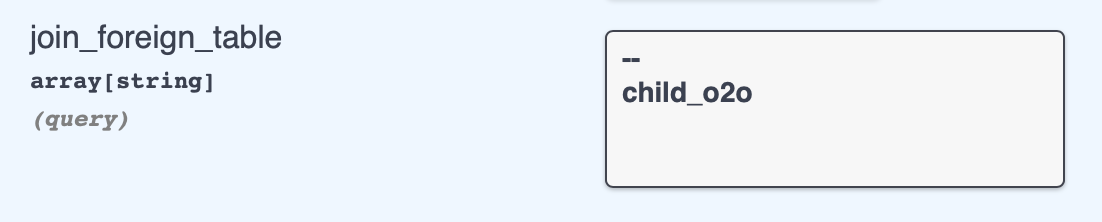\n\n* Try Request\nnow there are some data in these two table\n \n\n\n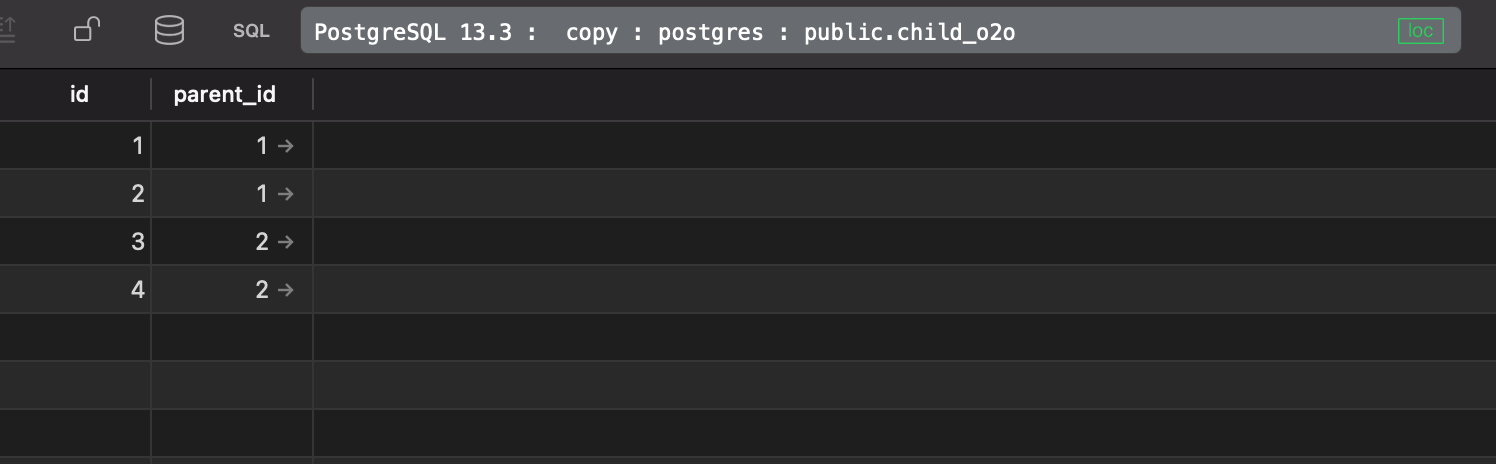\n\nwhen i request\n* Case One\n ```commandline\n curl -X 'GET' \\\n 'http://0.0.0.0:8000/parent?join_foreign_table=child_o2o' \\\n -H 'accept: application/json'\n ```\n Response data\n ```json\n [\n {\n \"id_foreign\": [\n {\n \"id\": 1,\n \"parent_id\": 1\n },\n {\n \"id\": 2,\n \"parent_id\": 1\n }\n ],\n \"id\": 1\n },\n {\n \"id_foreign\": [\n {\n \"id\": 3,\n \"parent_id\": 2\n },\n {\n \"id\": 4,\n \"parent_id\": 2\n }\n ],\n \"id\": 2\n }\n ]\n ```\n Response headers\n ```text\n x-total-count: 4 \n ```\n \n There are response 4 data, response data will be grouped by the parent row, if the child refer to the same parent row\n \n* Case Two\n ```commandline\n curl -X 'GET' \\\n 'http://0.0.0.0:8000/child?join_foreign_table=parent_o2o' \\\n -H 'accept: application/json'\n ```\n Response data\n ```json\n [\n {\n \"parent_id_foreign\": [\n {\n \"id\": 1\n }\n ],\n \"id\": 1,\n \"parent_id\": 1\n },\n {\n \"parent_id_foreign\": [\n {\n \"id\": 1\n }\n ],\n \"id\": 2,\n \"parent_id\": 1\n },\n {\n \"parent_id_foreign\": [\n {\n \"id\": 2\n }\n ],\n \"id\": 3,\n \"parent_id\": 2\n },\n {\n \"parent_id_foreign\": [\n {\n \"id\": 2\n }\n ],\n \"id\": 4,\n \"parent_id\": 2\n }\n ]\n ```\n Response Header\n ```text\n x-total-count: 4 \n ```\n\n#### FastAPI_quickcrud Response Status Code standard \n\n\nWhen you ask for a specific resource, say a user or with query param, and the user doesn't exist\n\n ```GET: get one : https://0.0.0.0:8080/api/:userid?xx=xx```\n\n ```UPDATE: update one : https://0.0.0.0:8080/api/:userid?xx=xx```\n\n ```PATCH: patch one : https://0.0.0.0:8080/api/:userid?xx=xx```\n\n ```DELETE: delete one : https://0.0.0.0:8080/api/:userid?xx=xx```\n\n \nthen fastapi-qucikcrud should return 404. In this case, the client requested a resource that doesn't exist.\n\n----\n\nIn the other case, you have an api that operate data on batch in the system using the following url:\n\n ```GET: get many : https://0.0.0.0:8080/api/user?xx=xx```\n\n ```UPDATE: update many : https://0.0.0.0:8080/api/user?xx=xx```\n\n ```DELETE: delete many : https://0.0.0.0:8080/api/user?xx=xx```\n\n ```PATCH: patch many : https://0.0.0.0:8080/api/user?xx=xx```\n\nIf there are no users in the system, then, in this case, you should return 204.\n\n\n### TODO\n[milestones](https://github.com/LuisLuii/FastAPIQuickCRUD/milestones)\n\n \n\n",
"bugtrack_url": null,
"license": "MIT License",
"summary": "A comprehensive FastaAPI's CRUD router generator for SQLALchemy.",
"version": "0.2.8",
"project_urls": {
"Homepage": "https://github.com/LuisLuii/FastAPIQuickCRUD"
},
"split_keywords": [
"fastapi",
"crud",
"restful",
"routing",
"sqlalchemy",
"generator",
"crudrouter",
"postgresql",
"builder"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "e9110bd60a3a59050deae2468688424e80d109ddfea92ceaa8e09ba78938ae28",
"md5": "7e20aafbd762fc23a0f0b160856c1591",
"sha256": "b7ead10b8a2aa5955dbf9e9604ae916e6076166c25239dc6d2845d0ee4bde3aa"
},
"downloads": -1,
"filename": "fastapi_quickcrud-0.2.8-py3-none-any.whl",
"has_sig": false,
"md5_digest": "7e20aafbd762fc23a0f0b160856c1591",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 40561,
"upload_time": "2024-01-07T00:22:42",
"upload_time_iso_8601": "2024-01-07T00:22:42.423981Z",
"url": "https://files.pythonhosted.org/packages/e9/11/0bd60a3a59050deae2468688424e80d109ddfea92ceaa8e09ba78938ae28/fastapi_quickcrud-0.2.8-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "27370ddb85d685095c431b318a5044761ecf9a46dabfa2a09ce339e4ad8914ce",
"md5": "76d60900004cd17ece3b4408f651a557",
"sha256": "7d297e524611020256e4b2ffb31f185559485b8612a5ca140acaae6df68d9e6c"
},
"downloads": -1,
"filename": "fastapi_quickcrud-0.2.8.tar.gz",
"has_sig": false,
"md5_digest": "76d60900004cd17ece3b4408f651a557",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 42837,
"upload_time": "2024-01-07T00:22:50",
"upload_time_iso_8601": "2024-01-07T00:22:50.137466Z",
"url": "https://files.pythonhosted.org/packages/27/37/0ddb85d685095c431b318a5044761ecf9a46dabfa2a09ce339e4ad8914ce/fastapi_quickcrud-0.2.8.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-01-07 00:22:50",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "LuisLuii",
"github_project": "FastAPIQuickCRUD",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"requirements": [],
"lcname": "fastapi-quickcrud"
}