[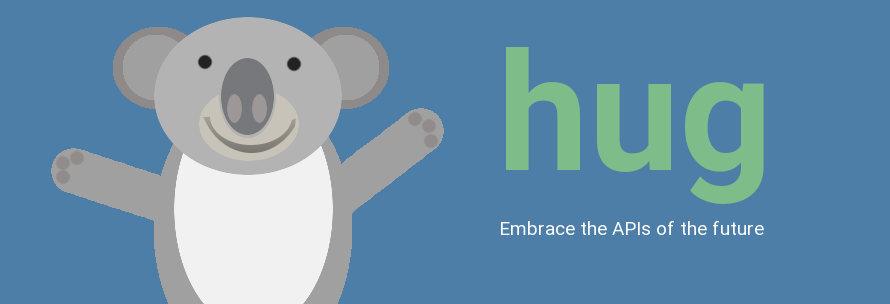](http://hug.rest)
===================
[](http://badge.fury.io/py/hug)
[](https://travis-ci.org/hugapi/hug)
[](https://ci.appveyor.com/project/TimothyCrosley/hug)
[](https://coveralls.io/github/hugapi/hug?branch=master)
[](https://pypi.python.org/pypi/hug/)
[](https://gitter.im/timothycrosley/hug?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
_________________
[Read Latest Documentation](https://hugapi.github.io/hug/) - [Browse GitHub Code Repository](https://github.com/hugapi/hug)
_________________
hug aims to make developing Python driven APIs as simple as possible, but no simpler. As a result, it drastically simplifies Python API development.
hug's Design Objectives:
- Make developing a Python driven API as succinct as a written definition.
- The framework should encourage code that self-documents.
- It should be fast. A developer should never feel the need to look somewhere else for performance reasons.
- Writing tests for APIs written on-top of hug should be easy and intuitive.
- Magic done once, in an API framework, is better than pushing the problem set to the user of the API framework.
- Be the basis for next generation Python APIs, embracing the latest technology.
As a result of these goals, hug is Python 3+ only and built upon [Falcon's](https://github.com/falconry/falcon) high performance HTTP library
[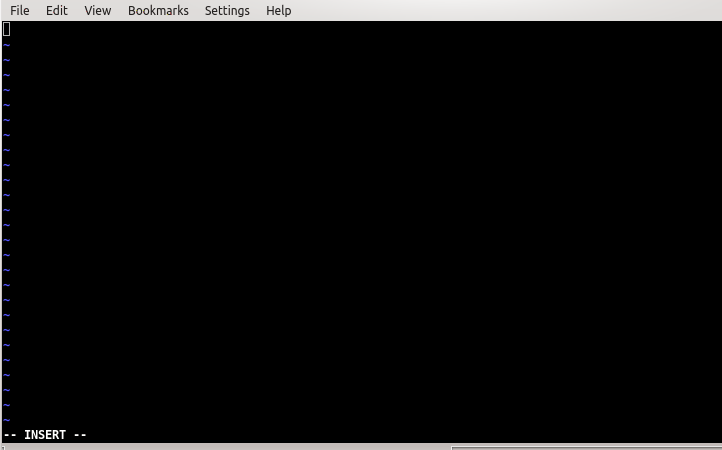](https://github.com/hugapi/hug/blob/develop/examples/hello_world.py)
Supporting hug development
===================
[Get professionally supported hug with the Tidelift Subscription](https://tidelift.com/subscription/pkg/pypi-hug?utm_source=pypi-hug&utm_medium=referral&utm_campaign=readme)
Professional support for hug is available as part of the [Tidelift
Subscription](https://tidelift.com/subscription/pkg/pypi-hug?utm_source=pypi-hug&utm_medium=referral&utm_campaign=readme).
Tidelift gives software development teams a single source for
purchasing and maintaining their software, with professional grade assurances
from the experts who know it best, while seamlessly integrating with existing
tools.
Installing hug
===================
Installing hug is as simple as:
```bash
pip3 install hug --upgrade
```
Ideally, within a [virtual environment](http://docs.python-guide.org/en/latest/dev/virtualenvs/).
Getting Started
===================
Build an example API with a simple endpoint in just a few lines.
```py
# filename: happy_birthday.py
"""A basic (single function) API written using hug"""
import hug
@hug.get('/happy_birthday')
def happy_birthday(name, age:hug.types.number=1):
"""Says happy birthday to a user"""
return "Happy {age} Birthday {name}!".format(**locals())
```
To run, from the command line type:
```bash
hug -f happy_birthday.py
```
You can access the example in your browser at:
`localhost:8000/happy_birthday?name=hug&age=1`. Then check out the
documentation for your API at `localhost:8000/documentation`
Parameters can also be encoded in the URL (check
out [`happy_birthday.py`](examples/happy_birthday.py) for the whole
example).
```py
@hug.get('/greet/{event}')
def greet(event: str):
"""Greets appropriately (from http://blog.ketchum.com/how-to-write-10-common-holiday-greetings/) """
greetings = "Happy"
if event == "Christmas":
greetings = "Merry"
if event == "Kwanzaa":
greetings = "Joyous"
if event == "wishes":
greetings = "Warm"
return "{greetings} {event}!".format(**locals())
```
Which, once you are running the server as above, you can use this way:
```
curl http://localhost:8000/greet/wishes
"Warm wishes!"
```
Versioning with hug
===================
```py
# filename: versioning_example.py
"""A simple example of a hug API call with versioning"""
import hug
@hug.get('/echo', versions=1)
def echo(text):
return text
@hug.get('/echo', versions=range(2, 5))
def echo(text):
return "Echo: {text}".format(**locals())
```
To run the example:
```bash
hug -f versioning_example.py
```
Then you can access the example from `localhost:8000/v1/echo?text=Hi` / `localhost:8000/v2/echo?text=Hi` Or access the documentation for your API from `localhost:8000`
Note: versioning in hug automatically supports both the version header as well as direct URL based specification.
Testing hug APIs
===================
hug's `http` method decorators don't modify your original functions. This makes testing hug APIs as simple as testing any other Python functions. Additionally, this means interacting with your API functions in other Python code is as straight forward as calling Python only API functions. hug makes it easy to test the full Python stack of your API by using the `hug.test` module:
```python
import hug
import happy_birthday
hug.test.get(happy_birthday, 'happy_birthday', {'name': 'Timothy', 'age': 25}) # Returns a Response object
```
You can use this `Response` object for test assertions (check
out [`test_happy_birthday.py`](examples/test_happy_birthday.py) ):
```python
def tests_happy_birthday():
response = hug.test.get(happy_birthday, 'happy_birthday', {'name': 'Timothy', 'age': 25})
assert response.status == HTTP_200
assert response.data is not None
```
Running hug with other WSGI based servers
===================
hug exposes a `__hug_wsgi__` magic method on every API module automatically. Running your hug based API on any standard wsgi server should be as simple as pointing it to `module_name`: `__hug_wsgi__`.
For Example:
```bash
uwsgi --http 0.0.0.0:8000 --wsgi-file examples/hello_world.py --callable __hug_wsgi__
```
To run the hello world hug example API.
Building Blocks of a hug API
===================
When building an API using the hug framework you'll use the following concepts:
**METHOD Decorators** `get`, `post`, `update`, etc HTTP method decorators that expose your Python function as an API while keeping your Python method unchanged
```py
@hug.get() # <- Is the hug METHOD decorator
def hello_world():
return "Hello"
```
hug uses the structure of the function you decorate to automatically generate documentation for users of your API. hug always passes a request, response, and api_version variable to your function if they are defined params in your function definition.
**Type Annotations** functions that optionally are attached to your methods arguments to specify how the argument is validated and converted into a Python type
```py
@hug.get()
def math(number_1:int, number_2:int): #The :int after both arguments is the Type Annotation
return number_1 + number_2
```
Type annotations also feed into `hug`'s automatic documentation
generation to let users of your API know what data to supply.
**Directives** functions that get executed with the request / response data based on being requested as an argument in your api_function.
These apply as input parameters only, and can not be applied currently as output formats or transformations.
```py
@hug.get()
def test_time(hug_timer):
return {'time_taken': float(hug_timer)}
```
Directives may be accessed via an argument with a `hug_` prefix, or by using Python 3 type annotations. The latter is the more modern approach, and is recommended. Directives declared in a module can be accessed by using their fully qualified name as the type annotation (ex: `module.directive_name`).
Aside from the obvious input transformation use case, directives can be used to pipe data into your API functions, even if they are not present in the request query string, POST body, etc. For an example of how to use directives in this way, see the authentication example in the examples folder.
Adding your own directives is straight forward:
```py
@hug.directive()
def square(value=1, **kwargs):
'''Returns passed in parameter multiplied by itself'''
return value * value
@hug.get()
@hug.local()
def tester(value: square=10):
return value
tester() == 100
```
For completeness, here is an example of accessing the directive via the magic name approach:
```py
@hug.directive()
def multiply(value=1, **kwargs):
'''Returns passed in parameter multiplied by itself'''
return value * value
@hug.get()
@hug.local()
def tester(hug_multiply=10):
return hug_multiply
tester() == 100
```
**Output Formatters** a function that takes the output of your API function and formats it for transport to the user of the API.
```py
@hug.default_output_format()
def my_output_formatter(data):
return "STRING:{0}".format(data)
@hug.get(output=hug.output_format.json)
def hello():
return {'hello': 'world'}
```
as shown, you can easily change the output format for both an entire API as well as an individual API call
**Input Formatters** a function that takes the body of data given from a user of your API and formats it for handling.
```py
@hug.default_input_format("application/json")
def my_input_formatter(data):
return ('Results', hug.input_format.json(data))
```
Input formatters are mapped based on the `content_type` of the request data, and only perform basic parsing. More detailed parsing should be done by the Type Annotations present on your `api_function`
**Middleware** functions that get called for every request a hug API processes
```py
@hug.request_middleware()
def process_data(request, response):
request.env['SERVER_NAME'] = 'changed'
@hug.response_middleware()
def process_data(request, response, resource):
response.set_header('MyHeader', 'Value')
```
You can also easily add any Falcon style middleware using:
```py
__hug__.http.add_middleware(MiddlewareObject())
```
**Parameter mapping** can be used to override inferred parameter names, eg. for reserved keywords:
```py
import marshmallow.fields as fields
...
@hug.get('/foo', map_params={'from': 'from_date'}) # API call uses 'from'
def get_foo_by_date(from_date: fields.DateTime()):
return find_foo(from_date)
```
Input formatters are mapped based on the `content_type` of the request data, and only perform basic parsing. More detailed parsing should be done by the Type Annotations present on your `api_function`
Splitting APIs over multiple files
===================
hug enables you to organize large projects in any manner you see fit. You can import any module that contains hug decorated functions (request handling, directives, type handlers, etc) and extend your base API with that module.
For example:
`something.py`
```py
import hug
@hug.get('/')
def say_hi():
return 'hello from something'
```
Can be imported into the main API file:
`__init__.py`
```py
import hug
from . import something
@hug.get('/')
def say_hi():
return "Hi from root"
@hug.extend_api('/something')
def something_api():
return [something]
```
Or alternatively - for cases like this - where only one module is being included per a URL route:
```py
#alternatively
hug.API(__name__).extend(something, '/something')
```
Configuring hug 404
===================
By default, hug returns an auto generated API spec when a user tries to access an endpoint that isn't defined. If you would not like to return this spec you can turn off 404 documentation:
From the command line application:
```bash
hug -nd -f {file} #nd flag tells hug not to generate documentation on 404
```
Additionally, you can easily create a custom 404 handler using the `hug.not_found` decorator:
```py
@hug.not_found()
def not_found_handler():
return "Not Found"
```
This decorator works in the same manner as the hug HTTP method decorators, and is even version aware:
```py
@hug.not_found(versions=1)
def not_found_handler():
return ""
@hug.not_found(versions=2)
def not_found_handler():
return "Not Found"
```
Asyncio support
===============
When using the `get` and `cli` method decorator on coroutines, hug will schedule
the execution of the coroutine.
Using asyncio coroutine decorator
```py
@hug.get()
@asyncio.coroutine
def hello_world():
return "Hello"
```
Using Python 3.5 async keyword.
```py
@hug.get()
async def hello_world():
return "Hello"
```
NOTE: Hug is running on top Falcon which is not an asynchronous server. Even if using
asyncio, requests will still be processed synchronously.
Using Docker
===================
If you like to develop in Docker and keep your system clean, you can do that but you'll need to first install [Docker Compose](https://docs.docker.com/compose/install/).
Once you've done that, you'll need to `cd` into the `docker` directory and run the web server (Gunicorn) specified in `./docker/gunicorn/Dockerfile`, after which you can preview the output of your API in the browser on your host machine.
```bash
$ cd ./docker
# This will run Gunicorn on port 8000 of the Docker container.
$ docker-compose up gunicorn
# From the host machine, find your Dockers IP address.
# For Windows & Mac:
$ docker-machine ip default
# For Linux:
$ ifconfig docker0 | grep 'inet' | cut -d: -f2 | awk '{ print $1}' | head -n1
```
By default, the IP is 172.17.0.1. Assuming that's the IP you see, as well, you would then go to `http://172.17.0.1:8000/` in your browser to view your API.
You can also log into a Docker container that you can consider your work space. This workspace has Python and Pip installed so you can use those tools within Docker. If you need to test the CLI interface, for example, you would use this.
```bash
$ docker-compose run workspace bash
```
On your Docker `workspace` container, the `./docker/templates` directory on your host computer is mounted to `/src` in the Docker container. This is specified under `services` > `app` of `./docker/docker-compose.yml`.
```bash
bash-4.3# cd /src
bash-4.3# tree
.
├── __init__.py
└── handlers
├── birthday.py
└── hello.py
1 directory, 3 files
```
Security contact information
===================
hug takes security and quality seriously. This focus is why we depend only on thoroughly tested components and utilize static analysis tools (such as bandit and safety) to verify the security of our code base.
If you find or encounter any potential security issues, please let us know right away so we can resolve them.
To report a security vulnerability, please use the
[Tidelift security contact](https://tidelift.com/security).
Tidelift will coordinate the fix and disclosure.
Why hug?
===================
HUG simply stands for Hopefully Useful Guide. This represents the project's goal to help guide developers into creating well written and intuitive APIs.
--------------------------------------------
Thanks and I hope you find *this* hug helpful as you develop your next Python API!
~Timothy Crosley
Raw data
{
"_id": null,
"home_page": "https://github.com/hugapi/hug",
"name": "hug",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.5",
"maintainer_email": "",
"keywords": "Web,Python,Python3,Refactoring,REST,Framework,RPC",
"author": "Timothy Crosley",
"author_email": "timothy.crosley@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/9e/b2/0549ffd2f6bdeb37e5cbee7119830e0928ca0df12c16ab8ed68078c69239/hug-2.6.1.tar.gz",
"platform": "",
"description": "[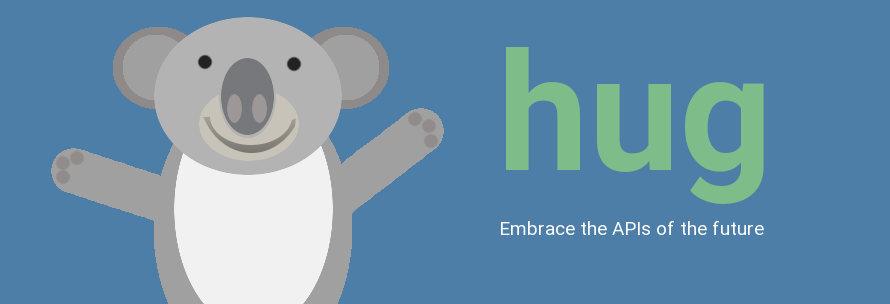](http://hug.rest)\n===================\n\n[](http://badge.fury.io/py/hug)\n[](https://travis-ci.org/hugapi/hug)\n[](https://ci.appveyor.com/project/TimothyCrosley/hug)\n[](https://coveralls.io/github/hugapi/hug?branch=master)\n[](https://pypi.python.org/pypi/hug/)\n[](https://gitter.im/timothycrosley/hug?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)\n\n_________________\n\n[Read Latest Documentation](https://hugapi.github.io/hug/) - [Browse GitHub Code Repository](https://github.com/hugapi/hug)\n_________________\n\nhug aims to make developing Python driven APIs as simple as possible, but no simpler. As a result, it drastically simplifies Python API development.\n\nhug's Design Objectives:\n\n- Make developing a Python driven API as succinct as a written definition.\n- The framework should encourage code that self-documents.\n- It should be fast. A developer should never feel the need to look somewhere else for performance reasons.\n- Writing tests for APIs written on-top of hug should be easy and intuitive.\n- Magic done once, in an API framework, is better than pushing the problem set to the user of the API framework.\n- Be the basis for next generation Python APIs, embracing the latest technology.\n\nAs a result of these goals, hug is Python 3+ only and built upon [Falcon's](https://github.com/falconry/falcon) high performance HTTP library\n\n[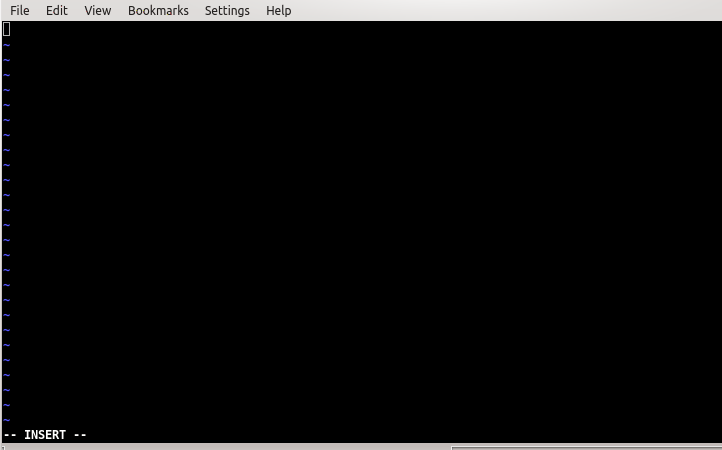](https://github.com/hugapi/hug/blob/develop/examples/hello_world.py)\n\nSupporting hug development\n===================\n[Get professionally supported hug with the Tidelift Subscription](https://tidelift.com/subscription/pkg/pypi-hug?utm_source=pypi-hug&utm_medium=referral&utm_campaign=readme)\n\nProfessional support for hug is available as part of the [Tidelift\nSubscription](https://tidelift.com/subscription/pkg/pypi-hug?utm_source=pypi-hug&utm_medium=referral&utm_campaign=readme).\nTidelift gives software development teams a single source for\npurchasing and maintaining their software, with professional grade assurances\nfrom the experts who know it best, while seamlessly integrating with existing\ntools.\n\nInstalling hug\n===================\n\nInstalling hug is as simple as:\n\n```bash\npip3 install hug --upgrade\n```\n\nIdeally, within a [virtual environment](http://docs.python-guide.org/en/latest/dev/virtualenvs/).\n\nGetting Started\n===================\n\nBuild an example API with a simple endpoint in just a few lines.\n\n```py\n# filename: happy_birthday.py\n\"\"\"A basic (single function) API written using hug\"\"\"\nimport hug\n\n\n@hug.get('/happy_birthday')\ndef happy_birthday(name, age:hug.types.number=1):\n \u00a0 \u00a0\"\"\"Says happy birthday to a user\"\"\"\n return \"Happy {age} Birthday {name}!\".format(**locals())\n```\n\nTo run, from the command line type:\n\n```bash\nhug -f happy_birthday.py\n```\n\nYou can access the example in your browser at:\n`localhost:8000/happy_birthday?name=hug&age=1`. Then check out the\ndocumentation for your API at `localhost:8000/documentation`\n\nParameters can also be encoded in the URL (check\nout [`happy_birthday.py`](examples/happy_birthday.py) for the whole\nexample).\n\n```py\n@hug.get('/greet/{event}')\ndef greet(event: str):\n \"\"\"Greets appropriately (from http://blog.ketchum.com/how-to-write-10-common-holiday-greetings/) \"\"\"\n greetings = \"Happy\"\n if event == \"Christmas\":\n greetings = \"Merry\"\n if event == \"Kwanzaa\":\n greetings = \"Joyous\"\n if event == \"wishes\":\n greetings = \"Warm\"\n\n return \"{greetings} {event}!\".format(**locals())\n```\n\nWhich, once you are running the server as above, you can use this way:\n\n```\ncurl http://localhost:8000/greet/wishes\n\"Warm wishes!\"\n```\n\nVersioning with hug\n===================\n\n```py\n# filename: versioning_example.py\n\"\"\"A simple example of a hug API call with versioning\"\"\"\nimport hug\n\n@hug.get('/echo', versions=1)\ndef echo(text):\n return text\n\n\n@hug.get('/echo', versions=range(2, 5))\ndef echo(text):\n return \"Echo: {text}\".format(**locals())\n```\n\nTo run the example:\n\n```bash\nhug -f versioning_example.py\n```\n\nThen you can access the example from `localhost:8000/v1/echo?text=Hi` / `localhost:8000/v2/echo?text=Hi` Or access the documentation for your API from `localhost:8000`\n\nNote: versioning in hug automatically supports both the version header as well as direct URL based specification.\n\nTesting hug APIs\n===================\n\nhug's `http` method decorators don't modify your original functions. This makes testing hug APIs as simple as testing any other Python functions. Additionally, this means interacting with your API functions in other Python code is as straight forward as calling Python only API functions. hug makes it easy to test the full Python stack of your API by using the `hug.test` module:\n\n```python\nimport hug\nimport happy_birthday\n\nhug.test.get(happy_birthday, 'happy_birthday', {'name': 'Timothy', 'age': 25}) # Returns a Response object\n```\n\nYou can use this `Response` object for test assertions (check\nout [`test_happy_birthday.py`](examples/test_happy_birthday.py) ):\n\n```python\ndef tests_happy_birthday():\n response = hug.test.get(happy_birthday, 'happy_birthday', {'name': 'Timothy', 'age': 25})\n assert response.status == HTTP_200\n assert response.data is not None\n```\n\nRunning hug with other WSGI based servers\n===================\n\nhug exposes a `__hug_wsgi__` magic method on every API module automatically. Running your hug based API on any standard wsgi server should be as simple as pointing it to `module_name`: `__hug_wsgi__`.\n\nFor Example:\n\n```bash\nuwsgi --http 0.0.0.0:8000 --wsgi-file examples/hello_world.py --callable __hug_wsgi__\n```\n\nTo run the hello world hug example API.\n\nBuilding Blocks of a hug API\n===================\n\nWhen building an API using the hug framework you'll use the following concepts:\n\n**METHOD Decorators** `get`, `post`, `update`, etc HTTP method decorators that expose your Python function as an API while keeping your Python method unchanged\n\n```py\n@hug.get() # <- Is the hug METHOD decorator\ndef hello_world():\n return \"Hello\"\n```\n\nhug uses the structure of the function you decorate to automatically generate documentation for users of your API. hug always passes a request, response, and api_version variable to your function if they are defined params in your function definition.\n\n**Type Annotations** functions that optionally are attached to your methods arguments to specify how the argument is validated and converted into a Python type\n\n```py\n@hug.get()\ndef math(number_1:int, number_2:int): #The :int after both arguments is the Type Annotation\n return number_1 + number_2\n```\n\nType annotations also feed into `hug`'s automatic documentation\ngeneration to let users of your API know what data to supply.\n\n**Directives** functions that get executed with the request / response data based on being requested as an argument in your api_function.\nThese apply as input parameters only, and can not be applied currently as output formats or transformations.\n\n```py\n@hug.get()\ndef test_time(hug_timer):\n return {'time_taken': float(hug_timer)}\n```\n\nDirectives may be accessed via an argument with a `hug_` prefix, or by using Python 3 type annotations. The latter is the more modern approach, and is recommended. Directives declared in a module can be accessed by using their fully qualified name as the type annotation (ex: `module.directive_name`).\n\nAside from the obvious input transformation use case, directives can be used to pipe data into your API functions, even if they are not present in the request query string, POST body, etc. For an example of how to use directives in this way, see the authentication example in the examples folder.\n\nAdding your own directives is straight forward:\n\n```py\n@hug.directive()\ndef square(value=1, **kwargs):\n '''Returns passed in parameter multiplied by itself'''\n return value * value\n\n@hug.get()\n@hug.local()\ndef tester(value: square=10):\n return value\n\ntester() == 100\n```\n\nFor completeness, here is an example of accessing the directive via the magic name approach:\n\n```py\n@hug.directive()\ndef multiply(value=1, **kwargs):\n '''Returns passed in parameter multiplied by itself'''\n return value * value\n\n@hug.get()\n@hug.local()\ndef tester(hug_multiply=10):\n return hug_multiply\n\ntester() == 100\n```\n\n**Output Formatters** a function that takes the output of your API function and formats it for transport to the user of the API.\n\n```py\n@hug.default_output_format()\ndef my_output_formatter(data):\n return \"STRING:{0}\".format(data)\n\n@hug.get(output=hug.output_format.json)\ndef hello():\n return {'hello': 'world'}\n```\n\nas shown, you can easily change the output format for both an entire API as well as an individual API call\n\n**Input Formatters** a function that takes the body of data given from a user of your API and formats it for handling.\n\n```py\n@hug.default_input_format(\"application/json\")\ndef my_input_formatter(data):\n return ('Results', hug.input_format.json(data))\n```\n\nInput formatters are mapped based on the `content_type` of the request data, and only perform basic parsing. More detailed parsing should be done by the Type Annotations present on your `api_function`\n\n**Middleware** functions that get called for every request a hug API processes\n\n```py\n@hug.request_middleware()\ndef process_data(request, response):\n request.env['SERVER_NAME'] = 'changed'\n\n@hug.response_middleware()\ndef process_data(request, response, resource):\n response.set_header('MyHeader', 'Value')\n```\n\nYou can also easily add any Falcon style middleware using:\n\n```py\n__hug__.http.add_middleware(MiddlewareObject())\n```\n\n**Parameter mapping** can be used to override inferred parameter names, eg. for reserved keywords:\n\n```py\nimport marshmallow.fields as fields\n...\n\n@hug.get('/foo', map_params={'from': 'from_date'}) # API call uses 'from'\ndef get_foo_by_date(from_date: fields.DateTime()):\n return find_foo(from_date)\n```\n\nInput formatters are mapped based on the `content_type` of the request data, and only perform basic parsing. More detailed parsing should be done by the Type Annotations present on your `api_function`\n\nSplitting APIs over multiple files\n===================\n\nhug enables you to organize large projects in any manner you see fit. You can import any module that contains hug decorated functions (request handling, directives, type handlers, etc) and extend your base API with that module.\n\nFor example:\n\n`something.py`\n\n```py\nimport hug\n\n@hug.get('/')\ndef say_hi():\n return 'hello from something'\n```\n\nCan be imported into the main API file:\n\n`__init__.py`\n\n```py\nimport hug\nfrom . import something\n\n@hug.get('/')\ndef say_hi():\n return \"Hi from root\"\n\n@hug.extend_api('/something')\ndef something_api():\n return [something]\n```\n\nOr alternatively - for cases like this - where only one module is being included per a URL route:\n\n```py\n#alternatively\nhug.API(__name__).extend(something, '/something')\n```\n\nConfiguring hug 404\n===================\n\nBy default, hug returns an auto generated API spec when a user tries to access an endpoint that isn't defined. If you would not like to return this spec you can turn off 404 documentation:\n\nFrom the command line application:\n\n```bash\nhug -nd -f {file} #nd flag tells hug not to generate documentation on 404\n```\n\nAdditionally, you can easily create a custom 404 handler using the `hug.not_found` decorator:\n\n```py\n@hug.not_found()\ndef not_found_handler():\n return \"Not Found\"\n```\n\nThis decorator works in the same manner as the hug HTTP method decorators, and is even version aware:\n\n```py\n@hug.not_found(versions=1)\ndef not_found_handler():\n return \"\"\n\n@hug.not_found(versions=2)\ndef not_found_handler():\n return \"Not Found\"\n```\n\nAsyncio support\n===============\n\nWhen using the `get` and `cli` method decorator on coroutines, hug will schedule\nthe execution of the coroutine.\n\nUsing asyncio coroutine decorator\n\n```py\n@hug.get()\n@asyncio.coroutine\ndef hello_world():\n return \"Hello\"\n```\n\nUsing Python 3.5 async keyword.\n\n```py\n@hug.get()\nasync def hello_world():\n return \"Hello\"\n```\n\nNOTE: Hug is running on top Falcon which is not an asynchronous server. Even if using\nasyncio, requests will still be processed synchronously.\n\nUsing Docker\n===================\n\nIf you like to develop in Docker and keep your system clean, you can do that but you'll need to first install [Docker Compose](https://docs.docker.com/compose/install/).\n\nOnce you've done that, you'll need to `cd` into the `docker` directory and run the web server (Gunicorn) specified in `./docker/gunicorn/Dockerfile`, after which you can preview the output of your API in the browser on your host machine.\n\n```bash\n$ cd ./docker\n# This will run Gunicorn on port 8000 of the Docker container.\n$ docker-compose up gunicorn\n\n# From the host machine, find your Dockers IP address.\n# For Windows & Mac:\n$ docker-machine ip default\n\n# For Linux:\n$ ifconfig docker0 | grep 'inet' | cut -d: -f2 | awk '{ print $1}' | head -n1\n```\n\nBy default, the IP is 172.17.0.1. Assuming that's the IP you see, as well, you would then go to `http://172.17.0.1:8000/` in your browser to view your API.\n\nYou can also log into a Docker container that you can consider your work space. This workspace has Python and Pip installed so you can use those tools within Docker. If you need to test the CLI interface, for example, you would use this.\n\n```bash\n$ docker-compose run workspace bash\n```\n\nOn your Docker `workspace` container, the `./docker/templates` directory on your host computer is mounted to `/src` in the Docker container. This is specified under `services` > `app` of `./docker/docker-compose.yml`.\n\n```bash\nbash-4.3# cd /src\nbash-4.3# tree\n.\n\u251c\u2500\u2500 __init__.py\n\u2514\u2500\u2500 handlers\n \u251c\u2500\u2500 birthday.py\n \u2514\u2500\u2500 hello.py\n\n1 directory, 3 files\n```\n\nSecurity contact information\n===================\n\nhug takes security and quality seriously. This focus is why we depend only on thoroughly tested components and utilize static analysis tools (such as bandit and safety) to verify the security of our code base.\nIf you find or encounter any potential security issues, please let us know right away so we can resolve them.\n\nTo report a security vulnerability, please use the\n[Tidelift security contact](https://tidelift.com/security).\nTidelift will coordinate the fix and disclosure.\n\nWhy hug?\n===================\n\nHUG simply stands for Hopefully Useful Guide. This represents the project's goal to help guide developers into creating well written and intuitive APIs.\n\n--------------------------------------------\n\nThanks and I hope you find *this* hug helpful as you develop your next Python API!\n\n~Timothy Crosley\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A Python framework that makes developing APIs as simple as possible, but no simpler.",
"version": "2.6.1",
"split_keywords": [
"web",
"python",
"python3",
"refactoring",
"rest",
"framework",
"rpc"
],
"urls": [
{
"comment_text": "",
"digests": {
"md5": "535d174bc1b2cccc92cfd4193e40743a",
"sha256": "31c8fc284f81377278629a4b94cbb619ae9ce829cdc2da9564ccc66a121046b4"
},
"downloads": -1,
"filename": "hug-2.6.1-py2.py3-none-any.whl",
"has_sig": false,
"md5_digest": "535d174bc1b2cccc92cfd4193e40743a",
"packagetype": "bdist_wheel",
"python_version": "py2.py3",
"requires_python": ">=3.5",
"size": 75085,
"upload_time": "2020-02-06T18:13:37",
"upload_time_iso_8601": "2020-02-06T18:13:37.987522Z",
"url": "https://files.pythonhosted.org/packages/93/01/ee254f209868b12c5327948bd193641beaad526fc34db44923c054a016fc/hug-2.6.1-py2.py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "3355631e28ccab572630277360a4a2c3",
"sha256": "b0edace2acb618873779c9ce6ecf9165db54fef95c22262f5700fcdd9febaec9"
},
"downloads": -1,
"filename": "hug-2.6.1.tar.gz",
"has_sig": false,
"md5_digest": "3355631e28ccab572630277360a4a2c3",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.5",
"size": 76270,
"upload_time": "2020-02-06T18:13:39",
"upload_time_iso_8601": "2020-02-06T18:13:39.954196Z",
"url": "https://files.pythonhosted.org/packages/9e/b2/0549ffd2f6bdeb37e5cbee7119830e0928ca0df12c16ab8ed68078c69239/hug-2.6.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2020-02-06 18:13:39",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "hugapi",
"github_project": "hug",
"travis_ci": true,
"coveralls": true,
"github_actions": false,
"appveyor": true,
"tox": true,
"lcname": "hug"
}