Name | kornia-rs JSON |
Version |
0.1.2
JSON |
| download |
home_page | None |
Summary | Low level implementations for computer vision in Rust |
upload_time | 2024-03-17 17:24:13 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.8 |
license | None |
keywords |
computer vision
rust
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# kornia-rs: low level computer vision library in Rust

[](https://badge.fury.io/py/kornia-rs)
[](https://docs.rs/kornia-rs)
[](LICENCE)
[](https://join.slack.com/t/kornia/shared_invite/zt-csobk21g-CnydWe5fmvkcktIeRFGCEQ)
The `kornia-rs` crate is a low level library for Computer Vision written in [Rust](https://www.rust-lang.org/) π¦
Use the library to perform image I/O, visualisation and other low level operations in your machine learning and data-science projects in a thread-safe and efficient way.
## Getting Started
`cargo run --example hello_world`
```rust
use kornia_rs::image::Image;
use kornia_rs::io::functional as F;
fn main() -> Result<(), Box<dyn std::error::Error>> {
// read the image
let image_path = std::path::Path::new("tests/data/dog.jpeg");
let image: Image<u8, 3> = F::read_image_jpeg(image_path)?;
println!("Hello, world!");
println!("Loaded Image size: {:?}", image.size());
println!("\nGoodbyte!");
Ok(())
}
```
```bash
Hello, world!
Loaded Image size: ImageSize { width: 258, height: 195 }
Goodbyte!
```
## Features
- π¦The library is primarly written in [Rust](https://www.rust-lang.org/).
- π Multi-threaded and efficient image I/O, image processing and advanced computer vision operators.
- π’ The n-dimensional backend is based on the [`ndarray`](https://crates.io/crates/ndarray) crate.
- π Pthon bindings are created with [PyO3/Maturin](https://github.com/PyO3/maturin).
- π¦ We package with support for Linux [amd64/arm64], Macos and WIndows.
- Supported Python versions are 3.7/3.8/3.9/3.10/3.11
### Supported image formats
- Read images from AVIF, BMP, DDS, Farbeld, GIF, HDR, ICO, JPEG (libjpeg-turbo), OpenEXR, PNG, PNM, TGA, TIFF, WebP.
### Image processing
- Convert images to grayscale, resize, crop, rotate, flip, pad, normalize, denormalize, and other image processing operations.
## π οΈ Installation
### >_ System dependencies
You need to install the following dependencies in your system:
```bash
sudo apt-get install nasm
```
### π¦ Rust
Add the following to your `Cargo.toml`:
```toml
[dependencies]
kornia-rs = "0.1.0"
```
Alternatively, you can use the `cargo` command to add the dependency:
```bash
cargo add kornia-rs
```
### π Python
```bash
pip install kornia-rs
```
## Examples: Image processing
The following example shows how to read an image, convert it to grayscale and resize it. The image is then logged to a [`rerun`](https://github.com/rerun-io/rerun) recording stream.
Checkout all the examples in the [`examples`](https://github.com/kornia/kornia-rs/tree/main/examples) directory to see more use cases.
```rust
use kornia_rs::image::Image;
use kornia_rs::io::functional as F;
fn main() -> Result<(), Box<dyn std::error::Error>> {
// read the image
let image_path = std::path::Path::new("tests/data/dog.jpeg");
let image: Image<u8, 3> = F::read_image_jpeg(image_path)?;
let image_viz = image.clone();
let image_f32: Image<f32, 3> = image.cast_and_scale::<f32>(1.0 / 255.0)?;
// convert the image to grayscale
let gray: Image<f32, 1> = kornia_rs::color::gray_from_rgb(&image_f32)?;
let gray_resize: Image<f32, 1> = kornia_rs::resize::resize_native(
&gray,
kornia_rs::image::ImageSize {
width: 128,
height: 128,
},
kornia_rs::resize::InterpolationMode::Bilinear,
)?;
println!("gray_resize: {:?}", gray_resize.size());
// create a Rerun recording stream
let rec = rerun::RecordingStreamBuilder::new("Kornia App").connect()?;
// log the images
let _ = rec.log("image", &rerun::Image::try_from(image_viz.data)?);
let _ = rec.log("gray", &rerun::Image::try_from(gray.data)?);
let _ = rec.log("gray_resize", &rerun::Image::try_from(gray_resize.data)?);
Ok(())
}
```
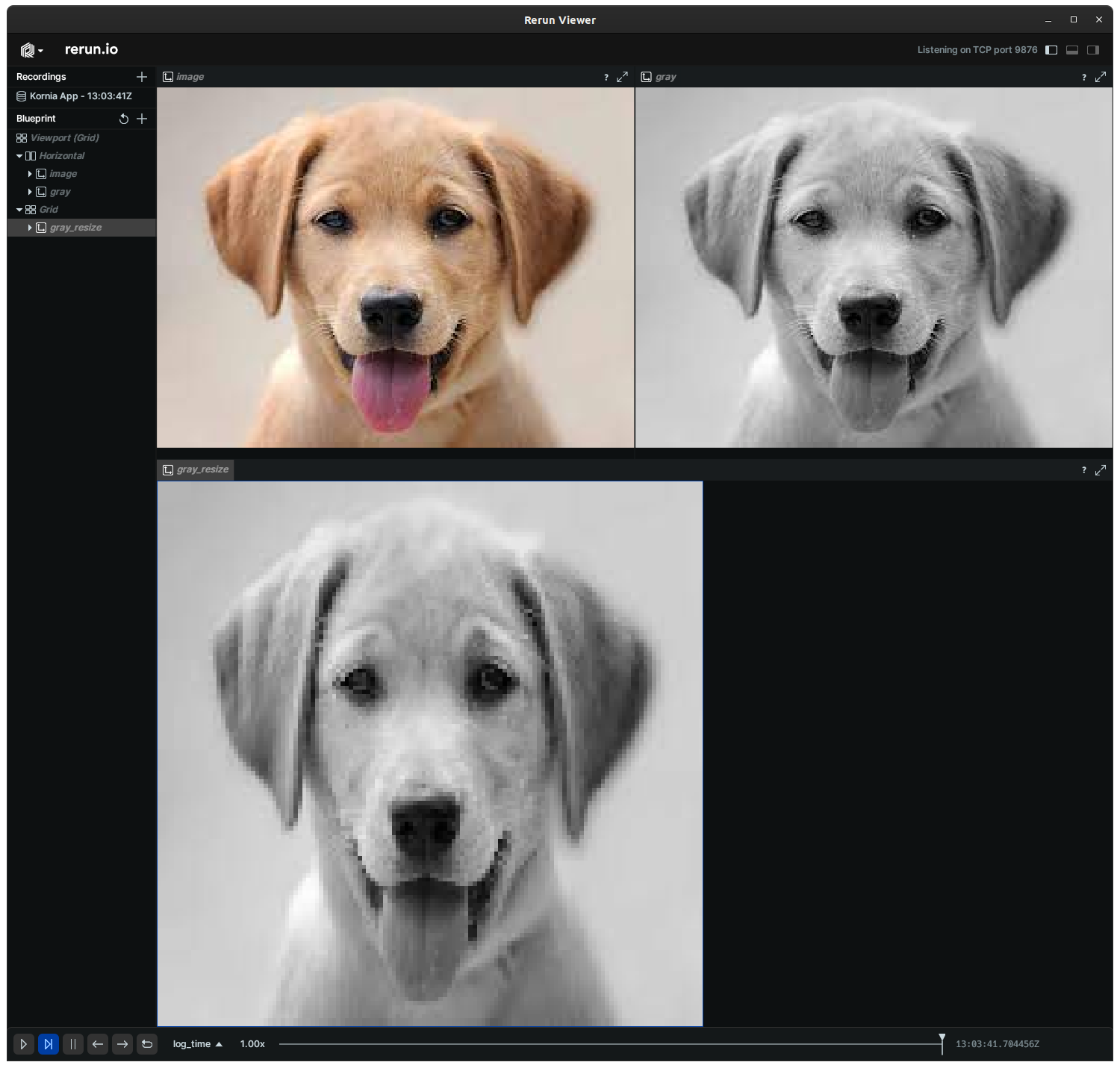
## Python usage
Load an image, that is converted directly to a numpy array to ease the integration with other libraries.
```python
import kornia_rs as K
import numpy as np
# load an image with using libjpeg-turbo
img: np.ndarray = K.read_image_jpeg("dog.jpeg")
# alternatively, load other formats
# img: np.ndarray = K.read_image_any("dog.png")
assert img.shape == (195, 258, 3)
# convert to dlpack to import to torch
img_t = torch.from_dlpack(img)
assert img_t.shape == (195, 258, 3)
```
Write an image to disk
```python
import kornia_rs as K
import numpy as np
# load an image with using libjpeg-turbo
img: np.ndarray = K.read_image_jpeg("dog.jpeg")
# write the image to disk
K.write_image_jpeg("dog_copy.jpeg", img)
```
Encode or decode image streams using the `turbojpeg` backend
```python
import kornia_rs as K
# load image with kornia-rs
img = K.read_image_jpeg("dog.jpeg")
# encode the image with jpeg
image_encoder = K.ImageEncoder()
image_encoder.set_quality(95) # set the encoding quality
# get the encoded stream
img_encoded: list[int] = image_encoder.encode(img)
# decode back the image
image_decoder = K.ImageDecoder()
decoded_img: np.ndarray = image_decoder.decode(bytes(image_encoded))
```
Resize an image using the `kornia-rs` backend with SIMD acceleration
```python
import kornia_rs as K
# load image with kornia-rs
img = K.read_image_jpeg("dog.jpeg")
# resize the image
resized_img = K.resize(img, (128, 128), interpolation="bilinear")
assert resized_img.shape == (128, 128, 3)
```
## π§βπ» Development
Pre-requisites: install `rust` and `python3` in your system.
```bash
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
```
Clone the repository in your local directory
```bash
git clone https://github.com/kornia/kornia-rs.git
```
### π¦ Rust
Compile the project and run the tests
```bash
cargo test
```
For specific tests, you can run the following command:
```bash
cargo test image
```
### π Python
To build the Python wheels, we use the `maturin` package. Use the following command to build the wheels:
```bash
make build-python
```
To run the tests, use the following command:
```bash
make test-python
```
## π Contributing
This is a child project of [Kornia](https://github.com/kornia/kornia). Join the community to get in touch with us, or just sponsor the project: https://opencollective.com/kornia
Raw data
{
"_id": null,
"home_page": null,
"name": "kornia-rs",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "Edgar Riba <edgar@kornia.org>",
"keywords": "computer vision,rust",
"author": null,
"author_email": "Edgar Riba <edgar@kornia.org>",
"download_url": "https://files.pythonhosted.org/packages/09/74/3ce6a94c9ed22c93ad6f62baec9c70081da4eb6047ad54348e3c7368fad6/kornia_rs-0.1.2.tar.gz",
"platform": null,
"description": "# kornia-rs: low level computer vision library in Rust\n\n\n[](https://badge.fury.io/py/kornia-rs)\n[](https://docs.rs/kornia-rs)\n[](LICENCE)\n[](https://join.slack.com/t/kornia/shared_invite/zt-csobk21g-CnydWe5fmvkcktIeRFGCEQ)\n\nThe `kornia-rs` crate is a low level library for Computer Vision written in [Rust](https://www.rust-lang.org/) \ud83e\udd80\n\nUse the library to perform image I/O, visualisation and other low level operations in your machine learning and data-science projects in a thread-safe and efficient way.\n\n## Getting Started\n\n`cargo run --example hello_world`\n\n```rust\nuse kornia_rs::image::Image;\nuse kornia_rs::io::functional as F;\n\nfn main() -> Result<(), Box<dyn std::error::Error>> {\n // read the image\n let image_path = std::path::Path::new(\"tests/data/dog.jpeg\");\n let image: Image<u8, 3> = F::read_image_jpeg(image_path)?;\n\n println!(\"Hello, world!\");\n println!(\"Loaded Image size: {:?}\", image.size());\n println!(\"\\nGoodbyte!\");\n\n Ok(())\n}\n```\n\n```bash\nHello, world!\nLoaded Image size: ImageSize { width: 258, height: 195 }\n\nGoodbyte!\n```\n\n## Features\n\n- \ud83e\udd80The library is primarly written in [Rust](https://www.rust-lang.org/).\n- \ud83d\ude80 Multi-threaded and efficient image I/O, image processing and advanced computer vision operators.\n- \ud83d\udd22 The n-dimensional backend is based on the [`ndarray`](https://crates.io/crates/ndarray) crate.\n- \ud83d\udc0d Pthon bindings are created with [PyO3/Maturin](https://github.com/PyO3/maturin).\n- \ud83d\udce6 We package with support for Linux [amd64/arm64], Macos and WIndows.\n- Supported Python versions are 3.7/3.8/3.9/3.10/3.11\n\n### Supported image formats\n\n- Read images from AVIF, BMP, DDS, Farbeld, GIF, HDR, ICO, JPEG (libjpeg-turbo), OpenEXR, PNG, PNM, TGA, TIFF, WebP.\n\n### Image processing\n\n- Convert images to grayscale, resize, crop, rotate, flip, pad, normalize, denormalize, and other image processing operations.\n\n## \ud83d\udee0\ufe0f Installation\n\n### >_ System dependencies\n\nYou need to install the following dependencies in your system:\n\n```bash\nsudo apt-get install nasm\n```\n\n### \ud83e\udd80 Rust\n\nAdd the following to your `Cargo.toml`:\n\n```toml\n[dependencies]\nkornia-rs = \"0.1.0\"\n```\n\nAlternatively, you can use the `cargo` command to add the dependency:\n\n```bash\ncargo add kornia-rs\n```\n\n### \ud83d\udc0d Python\n\n```bash\npip install kornia-rs\n```\n\n## Examples: Image processing\n\nThe following example shows how to read an image, convert it to grayscale and resize it. The image is then logged to a [`rerun`](https://github.com/rerun-io/rerun) recording stream.\n\nCheckout all the examples in the [`examples`](https://github.com/kornia/kornia-rs/tree/main/examples) directory to see more use cases.\n\n```rust\nuse kornia_rs::image::Image;\nuse kornia_rs::io::functional as F;\n\nfn main() -> Result<(), Box<dyn std::error::Error>> {\n // read the image\n let image_path = std::path::Path::new(\"tests/data/dog.jpeg\");\n let image: Image<u8, 3> = F::read_image_jpeg(image_path)?;\n let image_viz = image.clone();\n\n let image_f32: Image<f32, 3> = image.cast_and_scale::<f32>(1.0 / 255.0)?;\n\n // convert the image to grayscale\n let gray: Image<f32, 1> = kornia_rs::color::gray_from_rgb(&image_f32)?;\n\n let gray_resize: Image<f32, 1> = kornia_rs::resize::resize_native(\n &gray,\n kornia_rs::image::ImageSize {\n width: 128,\n height: 128,\n },\n kornia_rs::resize::InterpolationMode::Bilinear,\n )?;\n\n println!(\"gray_resize: {:?}\", gray_resize.size());\n\n // create a Rerun recording stream\n let rec = rerun::RecordingStreamBuilder::new(\"Kornia App\").connect()?;\n\n // log the images\n let _ = rec.log(\"image\", &rerun::Image::try_from(image_viz.data)?);\n let _ = rec.log(\"gray\", &rerun::Image::try_from(gray.data)?);\n let _ = rec.log(\"gray_resize\", &rerun::Image::try_from(gray_resize.data)?);\n\n Ok(())\n}\n```\n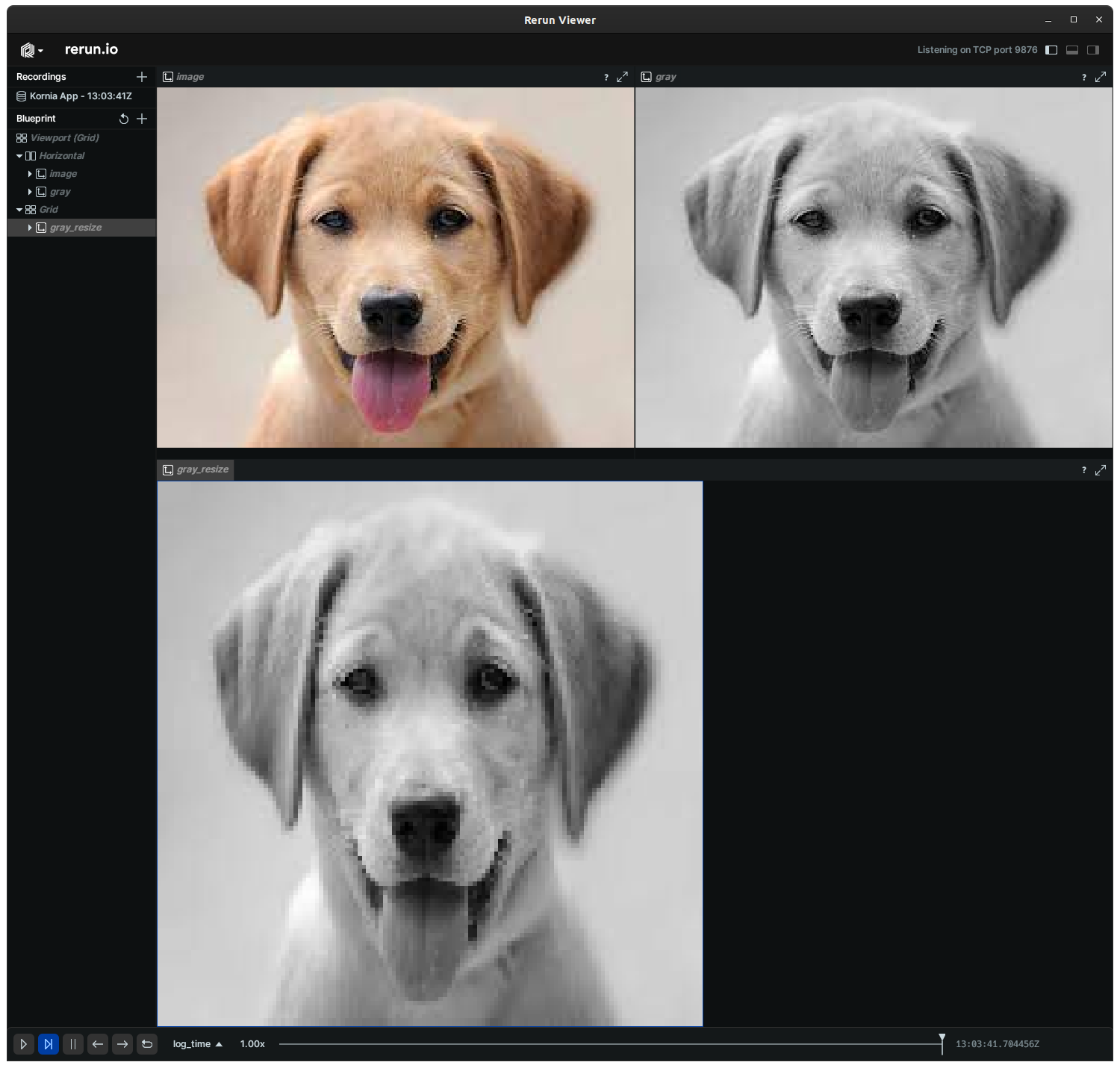\n\n## Python usage\n\nLoad an image, that is converted directly to a numpy array to ease the integration with other libraries.\n\n```python\n import kornia_rs as K\n import numpy as np\n\n # load an image with using libjpeg-turbo\n img: np.ndarray = K.read_image_jpeg(\"dog.jpeg\")\n\n # alternatively, load other formats\n # img: np.ndarray = K.read_image_any(\"dog.png\")\n\n assert img.shape == (195, 258, 3)\n\n # convert to dlpack to import to torch\n img_t = torch.from_dlpack(img)\n assert img_t.shape == (195, 258, 3)\n```\n\nWrite an image to disk\n\n```python\n import kornia_rs as K\n import numpy as np\n\n # load an image with using libjpeg-turbo\n img: np.ndarray = K.read_image_jpeg(\"dog.jpeg\")\n\n # write the image to disk\n K.write_image_jpeg(\"dog_copy.jpeg\", img)\n```\n\nEncode or decode image streams using the `turbojpeg` backend\n\n```python\nimport kornia_rs as K\n\n# load image with kornia-rs\nimg = K.read_image_jpeg(\"dog.jpeg\")\n\n# encode the image with jpeg\nimage_encoder = K.ImageEncoder()\nimage_encoder.set_quality(95) # set the encoding quality\n\n# get the encoded stream\nimg_encoded: list[int] = image_encoder.encode(img)\n\n# decode back the image\nimage_decoder = K.ImageDecoder()\n\ndecoded_img: np.ndarray = image_decoder.decode(bytes(image_encoded))\n```\n\nResize an image using the `kornia-rs` backend with SIMD acceleration\n\n```python\nimport kornia_rs as K\n\n# load image with kornia-rs\nimg = K.read_image_jpeg(\"dog.jpeg\")\n\n# resize the image\nresized_img = K.resize(img, (128, 128), interpolation=\"bilinear\")\n\nassert resized_img.shape == (128, 128, 3)\n```\n\n## \ud83e\uddd1\u200d\ud83d\udcbb Development\n\nPre-requisites: install `rust` and `python3` in your system.\n\n```bash\ncurl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh\n```\n\nClone the repository in your local directory\n\n```bash\ngit clone https://github.com/kornia/kornia-rs.git\n```\n\n### \ud83e\udd80 Rust\n\nCompile the project and run the tests\n\n```bash\ncargo test\n```\n\nFor specific tests, you can run the following command:\n\n```bash\ncargo test image\n```\n\n### \ud83d\udc0d Python\n\nTo build the Python wheels, we use the `maturin` package. Use the following command to build the wheels:\n\n```bash\nmake build-python\n```\n\nTo run the tests, use the following command:\n\n```bash\nmake test-python\n```\n\n## \ud83d\udc9c Contributing\n\nThis is a child project of [Kornia](https://github.com/kornia/kornia). Join the community to get in touch with us, or just sponsor the project: https://opencollective.com/kornia\n\n",
"bugtrack_url": null,
"license": null,
"summary": "Low level implementations for computer vision in Rust",
"version": "0.1.2",
"project_urls": {
"documentation": "https://kornia.readthedocs.io",
"homepage": "http://www.kornia.org",
"repository": "https://github.com/kornia/kornia-rs"
},
"split_keywords": [
"computer vision",
"rust"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "c6b4bc31a8f35d5ca53e364c17bf8816eb5684d4f96b7112d977ac16271ee2b5",
"md5": "7c5a6b7ea7b7bb4471636d926f933198",
"sha256": "83f1d2b4e2201a2d0cb635cd9e2cadb63272f61593c2b6b3efa1985d67001ae1"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp310-cp310-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "7c5a6b7ea7b7bb4471636d926f933198",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1501272,
"upload_time": "2024-03-17T17:23:19",
"upload_time_iso_8601": "2024-03-17T17:23:19.102295Z",
"url": "https://files.pythonhosted.org/packages/c6/b4/bc31a8f35d5ca53e364c17bf8816eb5684d4f96b7112d977ac16271ee2b5/kornia_rs-0.1.2-cp310-cp310-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "1897f852e026793a3fcbcfb5eda5b540447c6b68ad71ff4824a244922b2c7fd0",
"md5": "9528aea0d23daa21aaf68c42e4c84c92",
"sha256": "256747d123613ec0c7d7876b89c89f1d19d07e40aae01fbef136552cd18ced78"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp310-cp310-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "9528aea0d23daa21aaf68c42e4c84c92",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1312157,
"upload_time": "2024-03-17T17:23:21",
"upload_time_iso_8601": "2024-03-17T17:23:21.263130Z",
"url": "https://files.pythonhosted.org/packages/18/97/f852e026793a3fcbcfb5eda5b540447c6b68ad71ff4824a244922b2c7fd0/kornia_rs-0.1.2-cp310-cp310-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "d4a8d6ad7a6b90e33541fbd7e02c86ab8e382fff7cb56b1debefaa1589d305d5",
"md5": "7845a849a08d3aa19163b3dce468642d",
"sha256": "15bfc18b27e976c5c23b7ff0c1de04342595db2f149e83b1f75b7d6066668667"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "7845a849a08d3aa19163b3dce468642d",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 2222240,
"upload_time": "2024-03-17T17:23:22",
"upload_time_iso_8601": "2024-03-17T17:23:22.605686Z",
"url": "https://files.pythonhosted.org/packages/d4/a8/d6ad7a6b90e33541fbd7e02c86ab8e382fff7cb56b1debefaa1589d305d5/kornia_rs-0.1.2-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "7befeec16e87bc8893f608a42c96739ad0c35e30877b0f64bd19d95971534cef",
"md5": "541c49edf30a5d45bb6759f057184600",
"sha256": "eb627590ccfabcfb0fdc39cf186d4abf5eee017c74a9bdf1903ca0bc6b4192f0"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "541c49edf30a5d45bb6759f057184600",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 2363352,
"upload_time": "2024-03-17T17:23:24",
"upload_time_iso_8601": "2024-03-17T17:23:24.151750Z",
"url": "https://files.pythonhosted.org/packages/7b/ef/eec16e87bc8893f608a42c96739ad0c35e30877b0f64bd19d95971534cef/kornia_rs-0.1.2-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "29cfcdce4a1d424532d244fa8908241154f4a23f4e36a135bc88e160f369f150",
"md5": "511ff47a90a679a41959d546b3e5c77b",
"sha256": "0b0b4a0a44c2eb1050af1e64cd887ee654f1b4b101c3cad718413515d7b152a0"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp310-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "511ff47a90a679a41959d546b3e5c77b",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1196222,
"upload_time": "2024-03-17T17:23:26",
"upload_time_iso_8601": "2024-03-17T17:23:26.114516Z",
"url": "https://files.pythonhosted.org/packages/29/cf/cdce4a1d424532d244fa8908241154f4a23f4e36a135bc88e160f369f150/kornia_rs-0.1.2-cp310-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "177050f398bfa5e83d81a4f6f14a9b5ccb8864fbfffbc38e524ee41d377c5893",
"md5": "7f95c7e322379247cc11a2aaa2ed4c6f",
"sha256": "7bb6265532a3012a6818c4b4ec1e617cb47f259e1a9aae9a6c7a8987deaefc8e"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp311-cp311-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "7f95c7e322379247cc11a2aaa2ed4c6f",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1501270,
"upload_time": "2024-03-17T17:23:28",
"upload_time_iso_8601": "2024-03-17T17:23:28.302553Z",
"url": "https://files.pythonhosted.org/packages/17/70/50f398bfa5e83d81a4f6f14a9b5ccb8864fbfffbc38e524ee41d377c5893/kornia_rs-0.1.2-cp311-cp311-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "11bc44f42e780cdda8420839af34ca0b2bf54dfe622b75b4053bfafe77ffafcf",
"md5": "b5dfcc1663f5454dee9940464a67aa59",
"sha256": "9a0ebaa37642a37f228f435b3382656981fc2e5c8f7187f74361d5e2d595bf55"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp311-cp311-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "b5dfcc1663f5454dee9940464a67aa59",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1312062,
"upload_time": "2024-03-17T17:23:30",
"upload_time_iso_8601": "2024-03-17T17:23:30.314754Z",
"url": "https://files.pythonhosted.org/packages/11/bc/44f42e780cdda8420839af34ca0b2bf54dfe622b75b4053bfafe77ffafcf/kornia_rs-0.1.2-cp311-cp311-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "2e0390366c791e495670162497092e901272d70ee6fb712a75c42e21f8b42011",
"md5": "d2560ce09c78fc6345ae6452a9d8ba65",
"sha256": "5c323ccb05518d965fd96b079aea05298ceb3631fdea4fcf3de8f16a6bb3aa34"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "d2560ce09c78fc6345ae6452a9d8ba65",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 2222281,
"upload_time": "2024-03-17T17:23:31",
"upload_time_iso_8601": "2024-03-17T17:23:31.800814Z",
"url": "https://files.pythonhosted.org/packages/2e/03/90366c791e495670162497092e901272d70ee6fb712a75c42e21f8b42011/kornia_rs-0.1.2-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "d007f531801e3a1b26ced41c579c93c509715051585823f6a0ec1148da03902e",
"md5": "f8451e7683b52c833b2f1576596f8471",
"sha256": "f5edafa835abcdfa6cf8b94684eaef8c5df8ea13949bfe9048ddd0a901b2d200"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "f8451e7683b52c833b2f1576596f8471",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 2363211,
"upload_time": "2024-03-17T17:23:33",
"upload_time_iso_8601": "2024-03-17T17:23:33.219463Z",
"url": "https://files.pythonhosted.org/packages/d0/07/f531801e3a1b26ced41c579c93c509715051585823f6a0ec1148da03902e/kornia_rs-0.1.2-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "16e61c29f436e882fcd15d7e2ebd13d178197fbb4f6e2885147cf1d44d75bcf2",
"md5": "3493e9daded517cf7a0ad0d4d7911002",
"sha256": "4de0688535c3cb0ab4a93df5511a6dc7b7bd24eb3a5c842665e4e60d25997511"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp311-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "3493e9daded517cf7a0ad0d4d7911002",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1196179,
"upload_time": "2024-03-17T17:23:35",
"upload_time_iso_8601": "2024-03-17T17:23:35.454825Z",
"url": "https://files.pythonhosted.org/packages/16/e6/1c29f436e882fcd15d7e2ebd13d178197fbb4f6e2885147cf1d44d75bcf2/kornia_rs-0.1.2-cp311-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "d27aac19acf00bb3bf9c60c86646646894612cf7166a3eddc7a9d93140b706e8",
"md5": "3f9bf1eed706370062345932cad94a13",
"sha256": "c96ba400223b3bc863f494ada1b65bfd30783f8665d54899c7c673abaee842be"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp312-cp312-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "3f9bf1eed706370062345932cad94a13",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1497433,
"upload_time": "2024-03-17T17:23:37",
"upload_time_iso_8601": "2024-03-17T17:23:37.454722Z",
"url": "https://files.pythonhosted.org/packages/d2/7a/ac19acf00bb3bf9c60c86646646894612cf7166a3eddc7a9d93140b706e8/kornia_rs-0.1.2-cp312-cp312-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "1becce3743fdece9cf3a239011a212b2f9ed6b053cabcd53fe143c4106a33fb8",
"md5": "11015e9d81ad1f16e16bf1e99807042f",
"sha256": "7cb2f36b5b4048146fd5d73c5bd536a99dd7ab1a7b276c8b432038fd262d5908"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp312-cp312-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "11015e9d81ad1f16e16bf1e99807042f",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1309218,
"upload_time": "2024-03-17T17:23:39",
"upload_time_iso_8601": "2024-03-17T17:23:39.732485Z",
"url": "https://files.pythonhosted.org/packages/1b/ec/ce3743fdece9cf3a239011a212b2f9ed6b053cabcd53fe143c4106a33fb8/kornia_rs-0.1.2-cp312-cp312-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "d5e5ffac64637823884962ea618ecb0079da4df2e81e15657d8316a59d3ead23",
"md5": "2bf347ad41142c452ce252d3d5a4d796",
"sha256": "586aff2e2ce596936c64ab9b02d654e11166f0912282b95c02a15ab63a985cb7"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "2bf347ad41142c452ce252d3d5a4d796",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 2219789,
"upload_time": "2024-03-17T17:23:41",
"upload_time_iso_8601": "2024-03-17T17:23:41.301044Z",
"url": "https://files.pythonhosted.org/packages/d5/e5/ffac64637823884962ea618ecb0079da4df2e81e15657d8316a59d3ead23/kornia_rs-0.1.2-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "e1d8a3ee42bacf62c94ba2754174bdf5b0d07fc5a8dca6cf226e5bf10ed13a9c",
"md5": "43a2844c3cc4cbec5b391edd48748c86",
"sha256": "f2ddac3f7c71ef6750da3b90393c52cd873047a56af96e0cde8cf6de377aa3c0"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "43a2844c3cc4cbec5b391edd48748c86",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 2360941,
"upload_time": "2024-03-17T17:23:42",
"upload_time_iso_8601": "2024-03-17T17:23:42.616119Z",
"url": "https://files.pythonhosted.org/packages/e1/d8/a3ee42bacf62c94ba2754174bdf5b0d07fc5a8dca6cf226e5bf10ed13a9c/kornia_rs-0.1.2-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "19c6a9e925787a061af43f49083f8335e186a5f3495bdea0f3971f833b21050a",
"md5": "cccaf19442d2b666d08f2cd59ca1aee4",
"sha256": "8a8f0135bb002edf72214dbd249ed7ccdcd9eb8c303332fdb2b190f37c2087ba"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp312-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "cccaf19442d2b666d08f2cd59ca1aee4",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1194538,
"upload_time": "2024-03-17T17:23:44",
"upload_time_iso_8601": "2024-03-17T17:23:44.975806Z",
"url": "https://files.pythonhosted.org/packages/19/c6/a9e925787a061af43f49083f8335e186a5f3495bdea0f3971f833b21050a/kornia_rs-0.1.2-cp312-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "61d9423a525815b7a91337c3dab45e40f8bab0c4821b58d7ce7bb4ad4ec8cd73",
"md5": "76ffebec02ff3bdeb1487266c807bf14",
"sha256": "ac85497c891ff9293d56fa01cc64e48cafed0524edc3c34ad0c51856eb0b589c"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp37-cp37m-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "76ffebec02ff3bdeb1487266c807bf14",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": ">=3.8",
"size": 1502009,
"upload_time": "2024-03-17T17:23:46",
"upload_time_iso_8601": "2024-03-17T17:23:46.423042Z",
"url": "https://files.pythonhosted.org/packages/61/d9/423a525815b7a91337c3dab45e40f8bab0c4821b58d7ce7bb4ad4ec8cd73/kornia_rs-0.1.2-cp37-cp37m-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "afe280e835f6adc0e29c163f3f477d612034f2f18dbd6c6c25c1c086e7be534a",
"md5": "0bc048c14c5927495dbb059ff04ceb17",
"sha256": "1d5a16960134bd0f0cfd973207d432a76ee8b938bf13eef200e42eaa47bcd09f"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp37-cp37m-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "0bc048c14c5927495dbb059ff04ceb17",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": ">=3.8",
"size": 1313023,
"upload_time": "2024-03-17T17:23:49",
"upload_time_iso_8601": "2024-03-17T17:23:49.033564Z",
"url": "https://files.pythonhosted.org/packages/af/e2/80e835f6adc0e29c163f3f477d612034f2f18dbd6c6c25c1c086e7be534a/kornia_rs-0.1.2-cp37-cp37m-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "c4b709e2cf59b9ef523a8c8635de5498458a0c0983c6c20af96ddb89fc19830a",
"md5": "45b75e680fc79a8211069fbc5c5053f5",
"sha256": "e4e904c62b594aac8705c42385ad2a6a8bab1ceed5ee29d0f9edd2729883d7bf"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp37-cp37m-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "45b75e680fc79a8211069fbc5c5053f5",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": ">=3.8",
"size": 2222367,
"upload_time": "2024-03-17T17:23:50",
"upload_time_iso_8601": "2024-03-17T17:23:50.496561Z",
"url": "https://files.pythonhosted.org/packages/c4/b7/09e2cf59b9ef523a8c8635de5498458a0c0983c6c20af96ddb89fc19830a/kornia_rs-0.1.2-cp37-cp37m-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "4a19cbb7c8fa0a05bdce2fc2f894f0edfba2719936c0ae53a1fd7ccd15974f01",
"md5": "2ed7e36c8c9b51a5ac5497d24c919595",
"sha256": "6cc20f575437c83060fe83997a9778a931f26a6e2b6de302dac21246436419e7"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "2ed7e36c8c9b51a5ac5497d24c919595",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": ">=3.8",
"size": 2363939,
"upload_time": "2024-03-17T17:23:53",
"upload_time_iso_8601": "2024-03-17T17:23:53.452724Z",
"url": "https://files.pythonhosted.org/packages/4a/19/cbb7c8fa0a05bdce2fc2f894f0edfba2719936c0ae53a1fd7ccd15974f01/kornia_rs-0.1.2-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "226a0552062395fd7a042b24d40af96b838ed049bed614e49cc4583c1311992d",
"md5": "2a398cc682ec5c8fceb464c2182934ba",
"sha256": "88fcddc24fb000739b7360d3adcda12c7745fb678c0da42bbcfedd5eb69dfc22"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp37-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "2a398cc682ec5c8fceb464c2182934ba",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": ">=3.8",
"size": 1196121,
"upload_time": "2024-03-17T17:23:55",
"upload_time_iso_8601": "2024-03-17T17:23:55.618079Z",
"url": "https://files.pythonhosted.org/packages/22/6a/0552062395fd7a042b24d40af96b838ed049bed614e49cc4583c1311992d/kornia_rs-0.1.2-cp37-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "e628fe41a8e20010176f57f3606b194209f5fa542aadfb41e806557200887c08",
"md5": "7f2542e3c1487d5cf210ba1492df734b",
"sha256": "df5e65732e8aa1c3540c83a5661f97d648ba282cc5723e1b10d1fda25984f170"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp38-cp38-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "7f2542e3c1487d5cf210ba1492df734b",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1501935,
"upload_time": "2024-03-17T17:23:58",
"upload_time_iso_8601": "2024-03-17T17:23:58.060639Z",
"url": "https://files.pythonhosted.org/packages/e6/28/fe41a8e20010176f57f3606b194209f5fa542aadfb41e806557200887c08/kornia_rs-0.1.2-cp38-cp38-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "7edc5672b4e93b955d1a83d79b596e402730a67e94300e2cdb20c74638b0cc96",
"md5": "d90bd55bde1a29883402318736bc1667",
"sha256": "0d5a65aa3034d1dbb6d04f8f5613eb0e779187db729b70fe27cbb765fd4c0b91"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp38-cp38-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "d90bd55bde1a29883402318736bc1667",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1312807,
"upload_time": "2024-03-17T17:23:59",
"upload_time_iso_8601": "2024-03-17T17:23:59.456128Z",
"url": "https://files.pythonhosted.org/packages/7e/dc/5672b4e93b955d1a83d79b596e402730a67e94300e2cdb20c74638b0cc96/kornia_rs-0.1.2-cp38-cp38-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "504b54c98eb427f28ceb0a27927d9e90a2e23e5aa003a51681e3a8b334f890dd",
"md5": "571a6dca43d0d4a60c2d5dfbb1093156",
"sha256": "3dd7c8e71f1e80a3f034ef99f8892287d2ea9e248b0ad666795131fbd17eb6b7"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "571a6dca43d0d4a60c2d5dfbb1093156",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 2222298,
"upload_time": "2024-03-17T17:24:00",
"upload_time_iso_8601": "2024-03-17T17:24:00.923604Z",
"url": "https://files.pythonhosted.org/packages/50/4b/54c98eb427f28ceb0a27927d9e90a2e23e5aa003a51681e3a8b334f890dd/kornia_rs-0.1.2-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "010aefbf59b0a6140153e05ff2576b2980637cf7b3d7a3587623e766c835c700",
"md5": "8342de950aa0fe0626153b1de289bfba",
"sha256": "e76dbdb7e40b611e7000b087a54202869e568389915bff18702f80f6f95d6123"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "8342de950aa0fe0626153b1de289bfba",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 2363896,
"upload_time": "2024-03-17T17:24:02",
"upload_time_iso_8601": "2024-03-17T17:24:02.516886Z",
"url": "https://files.pythonhosted.org/packages/01/0a/efbf59b0a6140153e05ff2576b2980637cf7b3d7a3587623e766c835c700/kornia_rs-0.1.2-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "b455dac234b2e46ccf240659c782adc434a148ac497fb9ef4efb61f4801b39d4",
"md5": "81e1c66f67b242a778911ed1093707ad",
"sha256": "a706f99fea3f183612559071a29f032eeba2706871d23dee891c520f0d53c4d5"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp38-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "81e1c66f67b242a778911ed1093707ad",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1196149,
"upload_time": "2024-03-17T17:24:04",
"upload_time_iso_8601": "2024-03-17T17:24:04.054765Z",
"url": "https://files.pythonhosted.org/packages/b4/55/dac234b2e46ccf240659c782adc434a148ac497fb9ef4efb61f4801b39d4/kornia_rs-0.1.2-cp38-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "021e9c12171ae6689dce406c179abd681173dae9fb9cc13e048c5f3948164423",
"md5": "2c18681d412383e0e62055ea53077f1c",
"sha256": "481fbf61af2ae9a15d9de5d2bd6969afcc5f6db63a3f6e02b264c95106f3134e"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp39-cp39-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "2c18681d412383e0e62055ea53077f1c",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1501916,
"upload_time": "2024-03-17T17:24:05",
"upload_time_iso_8601": "2024-03-17T17:24:05.510281Z",
"url": "https://files.pythonhosted.org/packages/02/1e/9c12171ae6689dce406c179abd681173dae9fb9cc13e048c5f3948164423/kornia_rs-0.1.2-cp39-cp39-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "e1df0796bc3f28e2f9e43e132f2e4383e90c27a73d70f39c718dab0e1c006e92",
"md5": "3cd036473bf4a25c8bf72b98e6766f3a",
"sha256": "16422daf545630778866f66a51361e50ce9e89a5e8197a25f2450ceada2d11a3"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp39-cp39-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "3cd036473bf4a25c8bf72b98e6766f3a",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1312803,
"upload_time": "2024-03-17T17:24:06",
"upload_time_iso_8601": "2024-03-17T17:24:06.981441Z",
"url": "https://files.pythonhosted.org/packages/e1/df/0796bc3f28e2f9e43e132f2e4383e90c27a73d70f39c718dab0e1c006e92/kornia_rs-0.1.2-cp39-cp39-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "944a2387a112ca38a1f86fbe32a7c38d48a315a8c922229754c5e69f696c373c",
"md5": "1b94a6b2aa68108056fc0f8909bfee31",
"sha256": "0f3777ca772efcf892ec17a4930c47b86dbc9079b46773b54dd03d7887eb29e2"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "1b94a6b2aa68108056fc0f8909bfee31",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 2221796,
"upload_time": "2024-03-17T17:24:08",
"upload_time_iso_8601": "2024-03-17T17:24:08.299784Z",
"url": "https://files.pythonhosted.org/packages/94/4a/2387a112ca38a1f86fbe32a7c38d48a315a8c922229754c5e69f696c373c/kornia_rs-0.1.2-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "78e58e26aad206d276125a843bd63937f6a00e7fe720d400c21eeba241e69ca3",
"md5": "a0aa38aa178773af0693a8e325df4855",
"sha256": "4b1c45cfcc006b06b9a5a2284bc743a38cf297faea087d18e866b093f0800cdf"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "a0aa38aa178773af0693a8e325df4855",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 2363737,
"upload_time": "2024-03-17T17:24:10",
"upload_time_iso_8601": "2024-03-17T17:24:10.347460Z",
"url": "https://files.pythonhosted.org/packages/78/e5/8e26aad206d276125a843bd63937f6a00e7fe720d400c21eeba241e69ca3/kornia_rs-0.1.2-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "06cf1e1016c4b946c839a3cd9100ec4c67e55bddc85f10d921bb5857cff0e360",
"md5": "7c1092c45401201c46281c6d76166986",
"sha256": "1e626f4e4e77adf26ad238da1ef978f87427a93c1c54f3a7b2c0ee2d81865cf2"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2-cp39-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "7c1092c45401201c46281c6d76166986",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1196084,
"upload_time": "2024-03-17T17:24:11",
"upload_time_iso_8601": "2024-03-17T17:24:11.635460Z",
"url": "https://files.pythonhosted.org/packages/06/cf/1e1016c4b946c839a3cd9100ec4c67e55bddc85f10d921bb5857cff0e360/kornia_rs-0.1.2-cp39-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "09743ce6a94c9ed22c93ad6f62baec9c70081da4eb6047ad54348e3c7368fad6",
"md5": "47739c37ba503a45b0ae599d76b535a8",
"sha256": "c24cfe72e9400c7cf4616629b43b927d51e7cde91e770e4b0e2942a348515ab8"
},
"downloads": -1,
"filename": "kornia_rs-0.1.2.tar.gz",
"has_sig": false,
"md5_digest": "47739c37ba503a45b0ae599d76b535a8",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 106873,
"upload_time": "2024-03-17T17:24:13",
"upload_time_iso_8601": "2024-03-17T17:24:13.069434Z",
"url": "https://files.pythonhosted.org/packages/09/74/3ce6a94c9ed22c93ad6f62baec9c70081da4eb6047ad54348e3c7368fad6/kornia_rs-0.1.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-17 17:24:13",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "kornia",
"github_project": "kornia-rs",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "kornia-rs"
}