[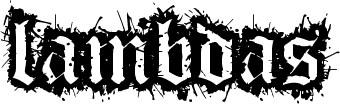](https://github.com/dry-python/lambdas)
-----
[](https://github.com/dry-python/lambdas/actions?query=workflow%3Atest)
[](https://codecov.io/gh/dry-python/lambdas)
[](https://lambdas.readthedocs.io/en/latest/?badge=latest)
[](https://pypi.org/project/lambdas/)
[](https://github.com/wemake-services/wemake-python-styleguide)
[](https://t.me/drypython)
-----
Write short and fully-typed `lambda`s where you need them.
## Features
- Allows to write `lambda`s as `_`
- Fully typed with annotations and checked with `mypy`, [PEP561 compatible](https://www.python.org/dev/peps/pep-0561/)
- Has a bunch of helpers for better composition
- Easy to start: has lots of docs, tests, and tutorials
## Installation
```bash
pip install lambdas
```
You also need to configure `mypy` correctly and install our plugin:
```ini
# In setup.cfg or mypy.ini:
[mypy]
plugins =
lambdas.contrib.mypy.lambdas_plugin
```
We recommend to use the same `mypy` settings [we use](https://github.com/wemake-services/wemake-python-styleguide/blob/master/styles/mypy.toml).
## Examples
Imagine that you need to sort an array of dictionaries like so:
```python
>>> scores = [
... {'name': 'Nikita', 'score': 2},
... {'name': 'Oleg', 'score': 1},
... {'name': 'Pavel', 'score': 4},
... ]
>>> print(sorted(scores, key=lambda item: item['score']))
[{'name': 'Oleg', 'score': 1}, {'name': 'Nikita', 'score': 2}, {'name': 'Pavel', 'score': 4}]
```
And it works perfectly fine.
Except, that you have to do a lot of typing for such a simple operation.
That's where `lambdas` helper steps in:
```python
>>> from lambdas import _
>>> scores = [
... {'name': 'Nikita', 'score': 2},
... {'name': 'Oleg', 'score': 1},
... {'name': 'Pavel', 'score': 4},
... ]
>>> print(sorted(scores, key=_['score']))
[{'name': 'Oleg', 'score': 1}, {'name': 'Nikita', 'score': 2}, {'name': 'Pavel', 'score': 4}]
```
It might really save you a lot of effort,
when you use a lot of `lambda` functions.
Like when using [`returns`](https://github.com/dry-python/returns) library.
We can easily create math expressions:
```python
>>> from lambdas import _
>>> math_expression = _ * 2 + 1
>>> print(math_expression(10))
21
>>> complex_math_expression = 50 / (_ ** 2) * 2
>>> print(complex_math_expression(5))
100.0
```
Work in progress:
- `_.method()` is not supported yet for the same reason
- `TypedDict`s are not tested with `__getitem__`
- `__getitem__` does not work with list and tuples (collections), only dicts (mappings)
For now you will have to use regular `lamdba`s in these cases.
Raw data
{
"_id": null,
"home_page": "https://lambdas.readthedocs.io",
"name": "lambdas",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8.1,<4",
"maintainer_email": "",
"keywords": "functional programming,fp,lambda,lambdas,composition,type-safety,mypy,stubs",
"author": "sobolevn",
"author_email": "mail@sobolevn.me",
"download_url": "https://files.pythonhosted.org/packages/db/13/dcc0a750047115dafdcd6f40f5af339567b985d99f6a14a91f6884ae2b37/lambdas-0.2.0.tar.gz",
"platform": null,
"description": "[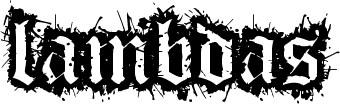](https://github.com/dry-python/lambdas)\n\n-----\n\n[](https://github.com/dry-python/lambdas/actions?query=workflow%3Atest)\n[](https://codecov.io/gh/dry-python/lambdas)\n[](https://lambdas.readthedocs.io/en/latest/?badge=latest)\n[](https://pypi.org/project/lambdas/)\n[](https://github.com/wemake-services/wemake-python-styleguide)\n[](https://t.me/drypython)\n\n-----\n\nWrite short and fully-typed `lambda`s where you need them.\n\n\n## Features\n\n- Allows to write `lambda`s as `_`\n- Fully typed with annotations and checked with `mypy`, [PEP561 compatible](https://www.python.org/dev/peps/pep-0561/)\n- Has a bunch of helpers for better composition\n- Easy to start: has lots of docs, tests, and tutorials\n\n\n## Installation\n\n```bash\npip install lambdas\n```\n\nYou also need to configure `mypy` correctly and install our plugin:\n\n```ini\n# In setup.cfg or mypy.ini:\n[mypy]\nplugins =\n lambdas.contrib.mypy.lambdas_plugin\n```\n\nWe recommend to use the same `mypy` settings [we use](https://github.com/wemake-services/wemake-python-styleguide/blob/master/styles/mypy.toml).\n\n\n## Examples\n\nImagine that you need to sort an array of dictionaries like so:\n\n```python\n>>> scores = [\n... {'name': 'Nikita', 'score': 2},\n... {'name': 'Oleg', 'score': 1},\n... {'name': 'Pavel', 'score': 4},\n... ]\n\n>>> print(sorted(scores, key=lambda item: item['score']))\n[{'name': 'Oleg', 'score': 1}, {'name': 'Nikita', 'score': 2}, {'name': 'Pavel', 'score': 4}]\n```\n\nAnd it works perfectly fine.\nExcept, that you have to do a lot of typing for such a simple operation.\n\nThat's where `lambdas` helper steps in:\n\n```python\n>>> from lambdas import _\n\n>>> scores = [\n... {'name': 'Nikita', 'score': 2},\n... {'name': 'Oleg', 'score': 1},\n... {'name': 'Pavel', 'score': 4},\n... ]\n\n>>> print(sorted(scores, key=_['score']))\n[{'name': 'Oleg', 'score': 1}, {'name': 'Nikita', 'score': 2}, {'name': 'Pavel', 'score': 4}]\n```\n\nIt might really save you a lot of effort,\nwhen you use a lot of `lambda` functions.\nLike when using [`returns`](https://github.com/dry-python/returns) library.\n\nWe can easily create math expressions:\n\n```python\n>>> from lambdas import _\n\n>>> math_expression = _ * 2 + 1\n>>> print(math_expression(10))\n21\n>>> complex_math_expression = 50 / (_ ** 2) * 2\n>>> print(complex_math_expression(5))\n100.0\n```\n\nWork in progress:\n\n- `_.method()` is not supported yet for the same reason\n- `TypedDict`s are not tested with `__getitem__`\n- `__getitem__` does not work with list and tuples (collections), only dicts (mappings)\n\nFor now you will have to use regular `lamdba`s in these cases.\n",
"bugtrack_url": null,
"license": "BSD-2-Clause",
"summary": "Typed lambdas that are short and readable",
"version": "0.2.0",
"project_urls": {
"Homepage": "https://lambdas.readthedocs.io",
"Repository": "https://github.com/dry-python/lambdas"
},
"split_keywords": [
"functional programming",
"fp",
"lambda",
"lambdas",
"composition",
"type-safety",
"mypy",
"stubs"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "daea52296bff57c194bc74d3367e88e26dc253991d50a54de8f48b0dbb2293db",
"md5": "7688d7fb6ce480c0b7badb74eee31e3e",
"sha256": "4cd3e6f10966951c5810b019d1693a0c0881d7646b2bbad28f87e4f43f8a053e"
},
"downloads": -1,
"filename": "lambdas-0.2.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "7688d7fb6ce480c0b7badb74eee31e3e",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8.1,<4",
"size": 6592,
"upload_time": "2023-09-14T01:47:01",
"upload_time_iso_8601": "2023-09-14T01:47:01.293029Z",
"url": "https://files.pythonhosted.org/packages/da/ea/52296bff57c194bc74d3367e88e26dc253991d50a54de8f48b0dbb2293db/lambdas-0.2.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "db13dcc0a750047115dafdcd6f40f5af339567b985d99f6a14a91f6884ae2b37",
"md5": "61c44c9cb9a5a337670cc2040a50010f",
"sha256": "b9ddeb7d10f6f6abe665fe4a21e7441890696a42b406b9c3ef3de8fac256ebe8"
},
"downloads": -1,
"filename": "lambdas-0.2.0.tar.gz",
"has_sig": false,
"md5_digest": "61c44c9cb9a5a337670cc2040a50010f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8.1,<4",
"size": 5712,
"upload_time": "2023-09-14T01:47:03",
"upload_time_iso_8601": "2023-09-14T01:47:03.256980Z",
"url": "https://files.pythonhosted.org/packages/db/13/dcc0a750047115dafdcd6f40f5af339567b985d99f6a14a91f6884ae2b37/lambdas-0.2.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-09-14 01:47:03",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "dry-python",
"github_project": "lambdas",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "lambdas"
}