# Package Description
[](https://dl.circleci.com/status-badge/redirect/gh/sfkleach/lazychains/tree/main) [](https://lazychains.readthedocs.io/en/latest/?badge=latest)
A Python library to provide "chains", which are Lisp-like singly linked lists
that support the lazy expansion of iterators. For example, we can construct a
Chain of three characters from the iterable "abc" and it initially starts as
unexpanded, shown by the three dots:
```py
>>> from lazychains import lazychain
>>> c = lazychain( "abc")
>>> c
chain([...])
```
We can force the expansion of *c* by performing (say) a lookup or by forcing the whole
chain of items by calling expand:
```py
>>> c[1] # Force the expansion of the next 2 elements.
True
>>> c
chain(['a','b',...])
>>> c.expand() # Force the expansion of the whole chain.
chain(['a','b','c'])
```
Chain are typically a lot less efficient than using ordinary arrays. So,
almost all the time you should carry on using ordinary arrays and/or tuples.
But Chains have a couple of special features that makes them the
perfect choice for some problems.
* Chains are immutable and hence can safely share their trailing segments.
* Chains can make it easy to work with extremely large (or infinite)
sequences.
Expanded or Unexpanded
----------------------
When you construct a chain from an iterator, you can choose whether or not
it should be immediately expanded by calling chain rather than lazychain.
The difference between the two is pictured below. First we can see what happens
in the example given above where we create the chain using lazychain on
"abc".

By contrast, we would immediately go to a fully expanded chain if we were to
simply apply chain:
```py
>>> from lazychains import chain
>>> c = chain( "abc" )
>>> c
chain(['a','b','c'])
>>>
```
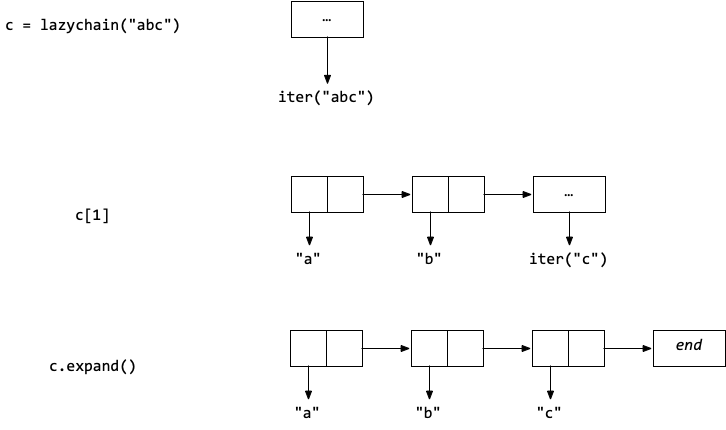
Raw data
{
"_id": null,
"home_page": "https://lazychains.readthedocs.io/en/latest/",
"name": "lazychains",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.10",
"maintainer_email": null,
"keywords": "iterators, linked list, single, lazy",
"author": "Stephen Leach",
"author_email": "sfkleach@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/90/74/e21b013a0ab2c2bf31ba355fca7c9fc70e8d9be4798ebe450fe13975d8cc/lazychains-0.2.12.tar.gz",
"platform": null,
"description": "# Package Description\n\n[](https://dl.circleci.com/status-badge/redirect/gh/sfkleach/lazychains/tree/main) [](https://lazychains.readthedocs.io/en/latest/?badge=latest)\n\nA Python library to provide \"chains\", which are Lisp-like singly linked lists \nthat support the lazy expansion of iterators. For example, we can construct a \nChain of three characters from the iterable \"abc\" and it initially starts as \nunexpanded, shown by the three dots:\n\n```py\n>>> from lazychains import lazychain\n>>> c = lazychain( \"abc\")\n>>> c\nchain([...])\n```\n\nWe can force the expansion of *c* by performing (say) a lookup or by forcing the whole\nchain of items by calling expand:\n\n```py\n>>> c[1] # Force the expansion of the next 2 elements.\nTrue\n>>> c\nchain(['a','b',...])\n>>> c.expand() # Force the expansion of the whole chain.\nchain(['a','b','c'])\n```\n\nChain are typically a lot less efficient than using ordinary arrays. So,\nalmost all the time you should carry on using ordinary arrays and/or tuples.\nBut Chains have a couple of special features that makes them the \nperfect choice for some problems.\n\n * Chains are immutable and hence can safely share their trailing segments.\n * Chains can make it easy to work with extremely large (or infinite) \n sequences.\n\nExpanded or Unexpanded\n----------------------\n\nWhen you construct a chain from an iterator, you can choose whether or not\nit should be immediately expanded by calling chain rather than lazychain.\nThe difference between the two is pictured below. First we can see what happens\nin the example given above where we create the chain using lazychain on \n\"abc\".\n\n\n\nBy contrast, we would immediately go to a fully expanded chain if we were to\nsimply apply chain:\n\n```py\n>>> from lazychains import chain\n>>> c = chain( \"abc\" )\n>>> c\nchain(['a','b','c'])\n>>> \n```\n\n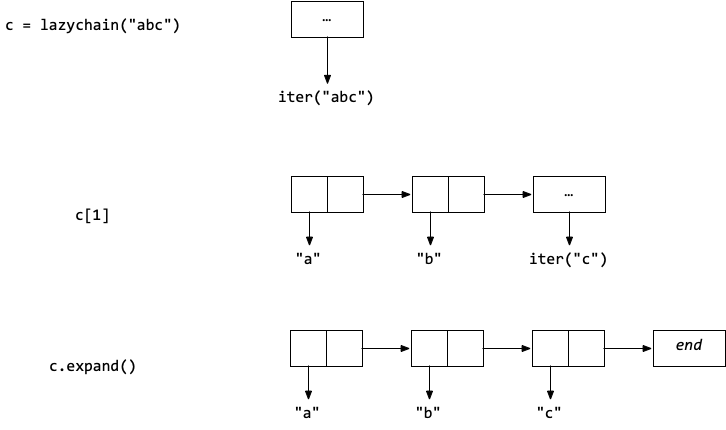\n\n",
"bugtrack_url": null,
"license": "GPL-3.0-or-later",
"summary": "Singly linked lists with incremental instantiation of iterators",
"version": "0.2.12",
"project_urls": {
"Documentation": "https://lazychains.readthedocs.io/en/latest/",
"Homepage": "https://lazychains.readthedocs.io/en/latest/",
"Repository": "https://github.com/sfkleach/lazychains"
},
"split_keywords": [
"iterators",
" linked list",
" single",
" lazy"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "25b8d450fdce3cc19c490e47c27d040725d7c0f8084524776b0c3c521cbb243f",
"md5": "e32477e06cdd980127f4ee12b64b9a33",
"sha256": "d5964d4eaec4d7e48ce13bec5235c325f4998b90a57d1a0b6635492f568d7139"
},
"downloads": -1,
"filename": "lazychains-0.2.12-py3-none-any.whl",
"has_sig": false,
"md5_digest": "e32477e06cdd980127f4ee12b64b9a33",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.10",
"size": 18603,
"upload_time": "2024-08-13T19:29:38",
"upload_time_iso_8601": "2024-08-13T19:29:38.684327Z",
"url": "https://files.pythonhosted.org/packages/25/b8/d450fdce3cc19c490e47c27d040725d7c0f8084524776b0c3c521cbb243f/lazychains-0.2.12-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9074e21b013a0ab2c2bf31ba355fca7c9fc70e8d9be4798ebe450fe13975d8cc",
"md5": "c56276b6d27510700ee626a13def43a4",
"sha256": "77c75a9dca5e03e00a660cf71aacce99acd4ec90e4d60c24286a4fab7f0d5907"
},
"downloads": -1,
"filename": "lazychains-0.2.12.tar.gz",
"has_sig": false,
"md5_digest": "c56276b6d27510700ee626a13def43a4",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.10",
"size": 17841,
"upload_time": "2024-08-13T19:29:40",
"upload_time_iso_8601": "2024-08-13T19:29:40.071062Z",
"url": "https://files.pythonhosted.org/packages/90/74/e21b013a0ab2c2bf31ba355fca7c9fc70e8d9be4798ebe450fe13975d8cc/lazychains-0.2.12.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-13 19:29:40",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "sfkleach",
"github_project": "lazychains",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"circle": true,
"lcname": "lazychains"
}