[//]: # (<p align="center"> )
[//]: # (<img src="./partitura/assets/partitura_logo_final.jpg" height="200">)
[//]: # (</p>)
<p align="center">
<img src="partitura/assets/partitura_logo_black.png#gh-light-mode-only" height="200">
<img align="center" src="./partitura/assets/partitura_logo_white.png#gh-dark-mode-only" height="200">
</p>
[](https://github.com/cpjku/partitura/releases)
[](https://badge.fury.io/py/partitura)
[](https://github.com/CPJKU/partitura/actions?query=workflow%3A%22Partitura+Unittests%22)
[](https://codecov.io/gh/CPJKU/partitura)
[](CODE_OF_CONDUCT.md)
**Partitura** is a Python package for handling symbolic musical information. It
supports loading from and exporting to *MusicXML* and *MIDI* files. It also supports loading from _Humdrum *kern*_ and *MEI*.
The full documentation for `partitura` is available online at [readthedocs.org](https://partitura.readthedocs.io/en/latest/index.html).
User Installation
==========
The easiest way to install the package is via `pip` from the [PyPI (Python
Package Index)](https://pypi.python.org/pypi>):
```shell
pip install partitura
```
This will install the latest release of the package and will install all dependencies automatically.
Quickstart
==========
A detailed tutorial with some hands-on MIR applications is available [here](https://cpjku.github.io/partitura_tutorial/index.html).
The following code loads the contents of an example MusicXML file included in
the package:
```python
import partitura as pt
my_xml_file = pt.EXAMPLE_MUSICXML
score = pt.load_score(my_xml_file)
```
The partitura `load_score` function will import any score format, i.e. (Musicxml, Kern, MIDI or MEI) to a `partitura.Score` object.
The score object will contain all the information in the score, including the score parts.
The following shows the contents of the first part of the score:
```python
part = score.parts[0]
print(part.pretty())
```
Output:
```shell
Part id="P1" name="Piano"
│
├─ TimePoint t=0 quarter=12
│ │
│ └─ starting objects
│ │
│ ├─ 0--48 Measure number=1
│ ├─ 0--48 Note id=n01 voice=1 staff=2 type=whole pitch=A4
│ ├─ 0--48 Page number=1
│ ├─ 0--24 Rest id=r01 voice=2 staff=1 type=half
│ ├─ 0--48 System number=1
│ └─ 0-- TimeSignature 4/4
│
├─ TimePoint t=24 quarter=12
│ │
│ ├─ ending objects
│ │ │
│ │ └─ 0--24 Rest id=r01 voice=2 staff=1 type=half
│ │
│ └─ starting objects
│ │
│ ├─ 24--48 Note id=n02 voice=2 staff=1 type=half pitch=C5
│ └─ 24--48 Note id=n03 voice=2 staff=1 type=half pitch=E5
│
└─ TimePoint t=48 quarter=12
│
└─ ending objects
│
├─ 0--48 Measure number=1
├─ 0--48 Note id=n01 voice=1 staff=2 type=whole pitch=A4
├─ 24--48 Note id=n02 voice=2 staff=1 type=half pitch=C5
├─ 24--48 Note id=n03 voice=2 staff=1 type=half pitch=E5
├─ 0--48 Page number=1
└─ 0--48 System number=1
```
If `lilypond` or `MuseScore` are installed on the system, the following command
renders the part to an image and displays it:
```python
pt.render(part)
```
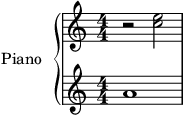
The notes in this part can be accessed through the property
`part.notes`:
```python
part.notes
> [<partitura.score.Note object at 0x...>, <partitura.score.Note object at 0x...>,
> <partitura.score.Note object at 0x...>]
```
The following code stores the start, end, and midi pitch of the notes in a numpy
array:
```python
import numpy as np
pianoroll = np.array([(n.start.t, n.end.t, n.midi_pitch) for n in part.notes])
print(pianoroll)
> [[ 0 48 69]
> [24 48 72]
> [24 48 76]]
```
The note start and end times are in the units specified by the
`divisions` element of the MusicXML file. This element specifies the
duration of a quarter note. The `divisions` value can vary within an
MusicXML file, so it is generally better to work with musical time in
beats.
The part object has a property :`part.beat_map` that converts timeline
times into beat times:
```python
beat_map = part.beat_map
print(beat_map(pianoroll[:, 0]))
> [0. 2. 2.]
print(beat_map(pianoroll[:, 1]))
> [4. 4. 4.]
```
The following commands save the part to MIDI and MusicXML, or export it as a WAV file (using [additive synthesis](https://en.wikipedia.org/wiki/Additive_synthesis)), respectively:
```python
# Save Score MIDI to file.
pt.save_score_midi(part, 'mypart.mid')
# Save Score MusicXML to file.
pt.save_musicxml(part, 'mypart.musicxml')
# Save as audio file using additive synthesis
pt.save_wav(part, 'mypart.wav')
```
More elaborate examples can be found in the `documentation
<https://partitura.readthedocs.io/en/latest/index.html>`_.
Import other formats
====================
For **MusicXML** files do:
```python
import partitura as pt
my_xml_file = pt.EXAMPLE_MUSICXML
score = pt.load_musicxml(my_xml_file)
```
For **Kern** files do:
```python
import partitura as pt
my_kern_file = pt.EXAMPLE_KERN
score = pt.load_kern(my_kern_file)
```
For **MEI** files do:
```python
import partitura as pt
my_mei_file = pt.EXAMPLE_MEI
score = pt.load_mei(my_mei_file)
```
One can also import any of the above formats by just using:
```python
import partitura as pt
any_score_format_path = pt.EXAMPLE_MUSICXML
score = pt.load_score(any_score_format_path)
```
License
=======
The code in this package is licensed under the Apache 2.0 License. For details,
please see the [LICENSE](LICENSE) file.
Citing Partitura
================
If you find Partitura useful, we would appreciate if you could cite us!
```
@inproceedings{partitura_mec,
title={{Partitura: A Python Package for Symbolic Music Processing}},
author={Cancino-Chac\'{o}n, Carlos Eduardo and Peter, Silvan David and Karystinaios, Emmanouil and Foscarin, Francesco and Grachten, Maarten and Widmer, Gerhard},
booktitle={{Proceedings of the Music Encoding Conference (MEC2022)}},
address={Halifax, Canada},
year={2022}
}
```
[//]: # ( | `Grachten, M. <https://maarten.grachten.eu>`__, `Cancino-Chacón, C. <http://www.carloscancinochacon.com>`__ and `Gadermaier, T. <https://www.jku.at/en/institute-of-computational-perception/about-us/people/thassilo-gadermaier/>`__)
[//]: # ( | "`partitura: A Python Package for Handling Symbolic Musical Data <http://carloscancinochacon.com/documents/extended_abstracts/GrachtenEtAl-ISMIR2019-LBD-ext-abstract.pdf>`__\ ".)
[//]: # ( | Late Breaking/Demo Session at the 20th International Society for)
[//]: # ( Music Information Retrieval Conference, Delft, The Netherlands,)
[//]: # ( 2019.)
Acknowledgments
===============
This project receives funding from the European Research Council (ERC) under
the European Union's Horizon 2020 research and innovation programme under grant
agreement No 101019375 ["Whither Music?"](https://www.jku.at/en/institute-of-computational-perception/research/projects/whither-music/).
This work has received support from the European Research Council (ERC) under
the European Union’s Horizon 2020 research and innovation programme under grant
agreement No. 670035 project ["Con Espressione"](https://www.jku.at/en/institute-of-computational-perception/research/projects/con-espressione/)
and the Austrian Science Fund (FWF) under grant P 29840-G26 (project
["Computer-assisted Analysis of Herbert von Karajan's Musical Conducting Style"](https://karajan-research.org/programs/musical-interpretation-karajan))
<p align="center">
<img src="docs/source/images/aknowledge_logo.png#gh-light-mode-only" height="200">
<img src="docs/source/images/aknowledge_logo_negative.png#gh-dark-mode-only" height="200">
</p>
[//]: # ()
[//]: # (.. image:: https://raw.githubusercontent.com/CPJKU/partitura/master/docs/images/erc_fwf_logos.jpg)
[//]: # ( :width: 600 px)
[//]: # ( :scale: 1%)
[//]: # ( :align: center)
Raw data
{
"_id": null,
"home_page": "https://github.com/CPJKU/partitura",
"name": "partitura",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "",
"keywords": "music notation musicxml midi",
"author": "Maarten Grachten, Carlos Cancino-Chac\u00f3n, Silvan Peter, Emmanouil Karystinaios, Francesco Foscarin, Thassilo Gadermaier, Patricia Hu",
"author_email": "partitura-users@googlegroups.com",
"download_url": "https://files.pythonhosted.org/packages/53/cf/e741160c687617138f5243714f7cb34247702e6eba10c6e05d9a4927d744/partitura-1.4.1.tar.gz",
"platform": null,
"description": "\n[//]: # (<p align=\"center\"> )\n\n[//]: # (<img src=\"./partitura/assets/partitura_logo_final.jpg\" height=\"200\">)\n\n[//]: # (</p>)\n<p align=\"center\">\n <img src=\"partitura/assets/partitura_logo_black.png#gh-light-mode-only\" height=\"200\">\n <img align=\"center\" src=\"./partitura/assets/partitura_logo_white.png#gh-dark-mode-only\" height=\"200\">\n</p>\n\n[](https://github.com/cpjku/partitura/releases)\n[](https://badge.fury.io/py/partitura)\n[](https://github.com/CPJKU/partitura/actions?query=workflow%3A%22Partitura+Unittests%22)\n[](https://codecov.io/gh/CPJKU/partitura)\n[](CODE_OF_CONDUCT.md)\n\n\n\n**Partitura** is a Python package for handling symbolic musical information. It\nsupports loading from and exporting to *MusicXML* and *MIDI* files. It also supports loading from _Humdrum *kern*_ and *MEI*.\n\nThe full documentation for `partitura` is available online at [readthedocs.org](https://partitura.readthedocs.io/en/latest/index.html).\n\nUser Installation\n==========\n\nThe easiest way to install the package is via `pip` from the [PyPI (Python\nPackage Index)](https://pypi.python.org/pypi>):\n```shell\npip install partitura\n```\nThis will install the latest release of the package and will install all dependencies automatically.\n\n\nQuickstart\n==========\nA detailed tutorial with some hands-on MIR applications is available [here](https://cpjku.github.io/partitura_tutorial/index.html).\n\nThe following code loads the contents of an example MusicXML file included in\nthe package:\n```python\nimport partitura as pt\nmy_xml_file = pt.EXAMPLE_MUSICXML\nscore = pt.load_score(my_xml_file)\n```\nThe partitura `load_score` function will import any score format, i.e. (Musicxml, Kern, MIDI or MEI) to a `partitura.Score` object.\nThe score object will contain all the information in the score, including the score parts.\nThe following shows the contents of the first part of the score:\n\n```python\npart = score.parts[0]\nprint(part.pretty())\n```\nOutput:\n```shell\nPart id=\"P1\" name=\"Piano\"\n \u2502\n \u251c\u2500 TimePoint t=0 quarter=12\n \u2502 \u2502\n \u2502 \u2514\u2500 starting objects\n \u2502 \u2502\n \u2502 \u251c\u2500 0--48 Measure number=1\n \u2502 \u251c\u2500 0--48 Note id=n01 voice=1 staff=2 type=whole pitch=A4\n \u2502 \u251c\u2500 0--48 Page number=1\n \u2502 \u251c\u2500 0--24 Rest id=r01 voice=2 staff=1 type=half\n \u2502 \u251c\u2500 0--48 System number=1\n \u2502 \u2514\u2500 0-- TimeSignature 4/4\n \u2502\n \u251c\u2500 TimePoint t=24 quarter=12\n \u2502 \u2502\n \u2502 \u251c\u2500 ending objects\n \u2502 \u2502 \u2502\n \u2502 \u2502 \u2514\u2500 0--24 Rest id=r01 voice=2 staff=1 type=half\n \u2502 \u2502\n \u2502 \u2514\u2500 starting objects\n \u2502 \u2502\n \u2502 \u251c\u2500 24--48 Note id=n02 voice=2 staff=1 type=half pitch=C5\n \u2502 \u2514\u2500 24--48 Note id=n03 voice=2 staff=1 type=half pitch=E5\n \u2502\n \u2514\u2500 TimePoint t=48 quarter=12\n \u2502\n \u2514\u2500 ending objects\n \u2502\n \u251c\u2500 0--48 Measure number=1\n \u251c\u2500 0--48 Note id=n01 voice=1 staff=2 type=whole pitch=A4\n \u251c\u2500 24--48 Note id=n02 voice=2 staff=1 type=half pitch=C5\n \u251c\u2500 24--48 Note id=n03 voice=2 staff=1 type=half pitch=E5\n \u251c\u2500 0--48 Page number=1\n \u2514\u2500 0--48 System number=1\n \n```\nIf `lilypond` or `MuseScore` are installed on the system, the following command\nrenders the part to an image and displays it:\n\n```python\npt.render(part)\n```\n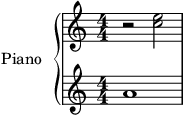\n\n\nThe notes in this part can be accessed through the property\n`part.notes`:\n\n```python\npart.notes\n> [<partitura.score.Note object at 0x...>, <partitura.score.Note object at 0x...>, \n> <partitura.score.Note object at 0x...>]\n\n```\n\n\nThe following code stores the start, end, and midi pitch of the notes in a numpy\narray:\n\n```python\nimport numpy as np\npianoroll = np.array([(n.start.t, n.end.t, n.midi_pitch) for n in part.notes])\nprint(pianoroll)\n> [[ 0 48 69]\n> [24 48 72]\n> [24 48 76]]\n```\n\n\nThe note start and end times are in the units specified by the\n`divisions` element of the MusicXML file. This element specifies the\nduration of a quarter note. The `divisions` value can vary within an\nMusicXML file, so it is generally better to work with musical time in\nbeats.\n\nThe part object has a property :`part.beat_map` that converts timeline\ntimes into beat times:\n\n```python\nbeat_map = part.beat_map\nprint(beat_map(pianoroll[:, 0]))\n> [0. 2. 2.]\nprint(beat_map(pianoroll[:, 1]))\n> [4. 4. 4.]\n```\n\n\nThe following commands save the part to MIDI and MusicXML, or export it as a WAV file (using [additive synthesis](https://en.wikipedia.org/wiki/Additive_synthesis)), respectively:\n\n```python\n# Save Score MIDI to file.\npt.save_score_midi(part, 'mypart.mid')\n\n# Save Score MusicXML to file.\npt.save_musicxml(part, 'mypart.musicxml')\n\n# Save as audio file using additive synthesis\npt.save_wav(part, 'mypart.wav')\n```\n\n\nMore elaborate examples can be found in the `documentation\n<https://partitura.readthedocs.io/en/latest/index.html>`_.\n\nImport other formats\n====================\nFor **MusicXML** files do:\n\n```python\nimport partitura as pt\nmy_xml_file = pt.EXAMPLE_MUSICXML\nscore = pt.load_musicxml(my_xml_file)\n```\n\nFor **Kern** files do:\n\n```python\nimport partitura as pt\nmy_kern_file = pt.EXAMPLE_KERN\nscore = pt.load_kern(my_kern_file)\n```\n\nFor **MEI** files do:\n\n```python\nimport partitura as pt\nmy_mei_file = pt.EXAMPLE_MEI\nscore = pt.load_mei(my_mei_file)\n```\n\n\nOne can also import any of the above formats by just using:\n\n```python\nimport partitura as pt\nany_score_format_path = pt.EXAMPLE_MUSICXML\nscore = pt.load_score(any_score_format_path)\n```\n\n\nLicense\n=======\n\nThe code in this package is licensed under the Apache 2.0 License. For details,\nplease see the [LICENSE](LICENSE) file.\n\n\nCiting Partitura\n================\n\nIf you find Partitura useful, we would appreciate if you could cite us!\n\n```\n@inproceedings{partitura_mec,\n title={{Partitura: A Python Package for Symbolic Music Processing}},\n author={Cancino-Chac\\'{o}n, Carlos Eduardo and Peter, Silvan David and Karystinaios, Emmanouil and Foscarin, Francesco and Grachten, Maarten and Widmer, Gerhard},\n booktitle={{Proceedings of the Music Encoding Conference (MEC2022)}},\n address={Halifax, Canada},\n year={2022}\n}\n```\n\n[//]: # ( | `Grachten, M. <https://maarten.grachten.eu>`__, `Cancino-Chac\u00f3n, C. <http://www.carloscancinochacon.com>`__ and `Gadermaier, T. <https://www.jku.at/en/institute-of-computational-perception/about-us/people/thassilo-gadermaier/>`__)\n\n[//]: # ( | \"`partitura: A Python Package for Handling Symbolic Musical Data <http://carloscancinochacon.com/documents/extended_abstracts/GrachtenEtAl-ISMIR2019-LBD-ext-abstract.pdf>`__\\ \".)\n\n[//]: # ( | Late Breaking/Demo Session at the 20th International Society for)\n\n[//]: # ( Music Information Retrieval Conference, Delft, The Netherlands,)\n\n[//]: # ( 2019.)\n\n\n\nAcknowledgments\n===============\n\nThis project receives funding from the European Research Council (ERC) under \nthe European Union's Horizon 2020 research and innovation programme under grant \nagreement No 101019375 [\"Whither Music?\"](https://www.jku.at/en/institute-of-computational-perception/research/projects/whither-music/).\n\n\n\nThis work has received support from the European Research Council (ERC) under\nthe European Union\u2019s Horizon 2020 research and innovation programme under grant\nagreement No. 670035 project [\"Con Espressione\"](https://www.jku.at/en/institute-of-computational-perception/research/projects/con-espressione/)\nand the Austrian Science Fund (FWF) under grant P 29840-G26 (project\n[\"Computer-assisted Analysis of Herbert von Karajan's Musical Conducting Style\"](https://karajan-research.org/programs/musical-interpretation-karajan))\n<p align=\"center\">\n <img src=\"docs/source/images/aknowledge_logo.png#gh-light-mode-only\" height=\"200\">\n <img src=\"docs/source/images/aknowledge_logo_negative.png#gh-dark-mode-only\" height=\"200\">\n</p>\n\n[//]: # ()\n[//]: # (.. image:: https://raw.githubusercontent.com/CPJKU/partitura/master/docs/images/erc_fwf_logos.jpg)\n\n[//]: # ( :width: 600 px)\n\n[//]: # ( :scale: 1%)\n\n[//]: # ( :align: center)\n",
"bugtrack_url": null,
"license": "Apache 2.0",
"summary": "A package for handling symbolic musical information",
"version": "1.4.1",
"project_urls": {
"Homepage": "https://github.com/CPJKU/partitura"
},
"split_keywords": [
"music",
"notation",
"musicxml",
"midi"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "b78a2071abd3602918d25de408b05f6f0c55459d3b1a747810326712afb28942",
"md5": "dfa1b61b6a6596eb8c62857f31b6c265",
"sha256": "3ead9f96b080816dfab90ff25289d222b47aaa8899b26a08b3d2228ac54a8997"
},
"downloads": -1,
"filename": "partitura-1.4.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "dfa1b61b6a6596eb8c62857f31b6c265",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 323270,
"upload_time": "2023-10-25T12:28:54",
"upload_time_iso_8601": "2023-10-25T12:28:54.492424Z",
"url": "https://files.pythonhosted.org/packages/b7/8a/2071abd3602918d25de408b05f6f0c55459d3b1a747810326712afb28942/partitura-1.4.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "53cfe741160c687617138f5243714f7cb34247702e6eba10c6e05d9a4927d744",
"md5": "a3c558b21f7ce4323e94fd021e2db8e8",
"sha256": "0412c27d872721719e8fc89fe9d0ad7c8869b6c9f1119f6ff8fe67f07b71d7f0"
},
"downloads": -1,
"filename": "partitura-1.4.1.tar.gz",
"has_sig": false,
"md5_digest": "a3c558b21f7ce4323e94fd021e2db8e8",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 349967,
"upload_time": "2023-10-25T12:28:56",
"upload_time_iso_8601": "2023-10-25T12:28:56.095304Z",
"url": "https://files.pythonhosted.org/packages/53/cf/e741160c687617138f5243714f7cb34247702e6eba10c6e05d9a4927d744/partitura-1.4.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-10-25 12:28:56",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "CPJKU",
"github_project": "partitura",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "partitura"
}