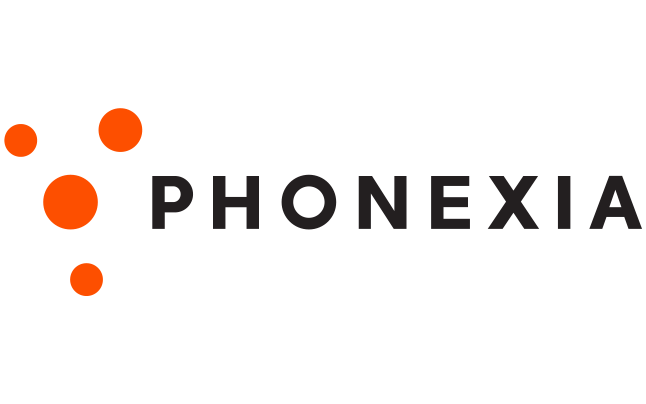
# Phonexia microservice communication via grpc library
This library contains defined interfaces for all microservices developed by [Phonexia](https://phonexia.com).
To use this library you will first need a running instance of any Phonexia microservice. If you don't yet have any running instance, don't hesitate to [contact our sales department](mailto:info@phonexia.com).
On [this page](https://docs.cloud.phonexia.com/docs/grpc/), you may find a gRPC API reference for all microservices.
## Example
Examples require microservice running on local machine at port 8080.
### Health check
You can invoke `health check` method of any microservice like this:
```python
import grpc
from phonexia.grpc.common.health_check_pb2 import HealthCheckRequest
from phonexia.grpc.common.health_check_pb2_grpc import HealthStub
def health_check(stub: HealthStub):
"""Create request and call the service.
Invoke Info method of the health stub.
Args:
stub (LicensingStub): Created stub for communication with service.
"""
request = HealthCheckRequest()
response = stub.Check(request)
print(response)
def main():
"""Create a gRPC channel and connect to the service."""
with grpc.insecure_channel(target="localhost:8080") as channel:
"""Create channel to the service.
The target parameter is the service address and port.
insecure channel is used for connection without TLS.
To use SSl/TLS see the grpc_secure_channel function.
"""
stub = HealthStub(channel)
health_check(stub)
if __name__ == "__main__":
main()
```
If the microservice is running, it should return: `status: SERVING`.
### Check license
Each microservice has licensed model which is required to run the service. You can fetch information about licensed models like this:
```python
import grpc
from phonexia.grpc.common.licensing_pb2 import LicensingInfoRequest
from phonexia.grpc.common.licensing_pb2_grpc import LicensingStub
def licensing_check(stub: LicensingStub):
"""Create request and call the service.
Invoke Info method of the Licensing stub.
Args:
stub (LicensingStub): Created stub for communication with service.
"""
request = LicensingInfoRequest()
response = stub.Info(request)
print(
f"The license for technology '{response.technology_name}' with model"
f" '{response.model_info.name}:{response.model_info.version}'"
f" {'is' if response.is_valid else 'was'} valid until {response.valid_until}."
)
def main():
"""Create a gRPC channel and connect to the service."""
with grpc.insecure_channel(target="localhost:8080") as channel:
"""Create channel to the service.
The target parameter is the service address and port.
insecure channel is used for connection without TLS.
To use SSl/TLS see the grpc_secure_channel function.
"""
stub = LicensingStub(channel=channel)
licensing_check(stub)
if __name__ == "__main__":
main()
```
Returned message should contain information about technology name, model name with version and time validity of license.
Raw data
{
"_id": null,
"home_page": null,
"name": "phonexia-grpc",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.8",
"maintainer_email": null,
"keywords": "grpc, voice, voice-biometry, speech, language, STT, whisper",
"author": "Phonexia s.r.o.",
"author_email": "info@phonexia.com",
"download_url": "https://files.pythonhosted.org/packages/2b/05/f9077efee5837dffd4692dd5c96f126cd385ed6bdd778a3ad1a7a0233537/phonexia_grpc-2.0.0.tar.gz",
"platform": null,
"description": "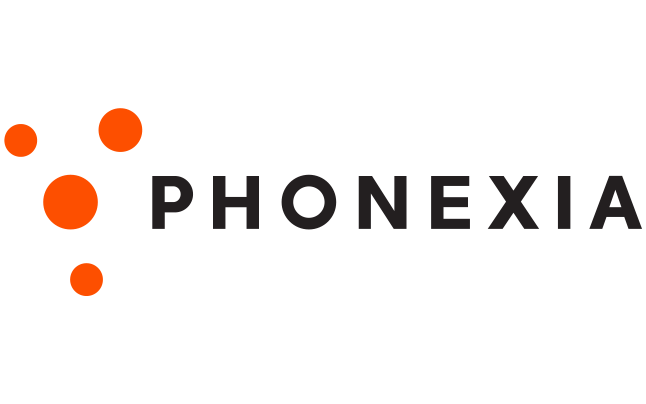\n\n# Phonexia microservice communication via grpc library\n\nThis library contains defined interfaces for all microservices developed by [Phonexia](https://phonexia.com).\n\nTo use this library you will first need a running instance of any Phonexia microservice. If you don't yet have any running instance, don't hesitate to [contact our sales department](mailto:info@phonexia.com).\n\nOn [this page](https://docs.cloud.phonexia.com/docs/grpc/), you may find a gRPC API reference for all microservices.\n\n## Example\n\nExamples require microservice running on local machine at port 8080.\n\n### Health check\n\nYou can invoke `health check` method of any microservice like this:\n\n```python\nimport grpc\n\nfrom phonexia.grpc.common.health_check_pb2 import HealthCheckRequest\nfrom phonexia.grpc.common.health_check_pb2_grpc import HealthStub\n\n\ndef health_check(stub: HealthStub):\n \"\"\"Create request and call the service.\n Invoke Info method of the health stub.\n Args:\n stub (LicensingStub): Created stub for communication with service.\n \"\"\"\n request = HealthCheckRequest()\n response = stub.Check(request)\n print(response)\n\n\ndef main():\n \"\"\"Create a gRPC channel and connect to the service.\"\"\"\n with grpc.insecure_channel(target=\"localhost:8080\") as channel:\n \"\"\"Create channel to the service.\n The target parameter is the service address and port.\n insecure channel is used for connection without TLS.\n To use SSl/TLS see the grpc_secure_channel function.\n \"\"\"\n stub = HealthStub(channel)\n health_check(stub)\n\n\nif __name__ == \"__main__\":\n main()\n\n```\n\nIf the microservice is running, it should return: `status: SERVING`.\n\n### Check license\n\nEach microservice has licensed model which is required to run the service. You can fetch information about licensed models like this:\n\n```python\nimport grpc\n\nfrom phonexia.grpc.common.licensing_pb2 import LicensingInfoRequest\nfrom phonexia.grpc.common.licensing_pb2_grpc import LicensingStub\n\n\ndef licensing_check(stub: LicensingStub):\n \"\"\"Create request and call the service.\n Invoke Info method of the Licensing stub.\n Args:\n stub (LicensingStub): Created stub for communication with service.\n \"\"\"\n request = LicensingInfoRequest()\n response = stub.Info(request)\n print(\n f\"The license for technology '{response.technology_name}' with model\"\n f\" '{response.model_info.name}:{response.model_info.version}'\"\n f\" {'is' if response.is_valid else 'was'} valid until {response.valid_until}.\"\n )\n\n\ndef main():\n \"\"\"Create a gRPC channel and connect to the service.\"\"\"\n with grpc.insecure_channel(target=\"localhost:8080\") as channel:\n \"\"\"Create channel to the service.\n The target parameter is the service address and port.\n insecure channel is used for connection without TLS.\n To use SSl/TLS see the grpc_secure_channel function.\n \"\"\"\n stub = LicensingStub(channel=channel)\n licensing_check(stub)\n\n\nif __name__ == \"__main__\":\n main()\n\n```\n\nReturned message should contain information about technology name, model name with version and time validity of license.\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "Library for communication with microservices developed by phonexia using grpc application interface.",
"version": "2.0.0",
"project_urls": {
"Changelog": "https://github.com/phonexia/protofiles/blob/main/CHANGELOG.md",
"Homepage": "https://phonexia.com",
"Issues": "https://phonexia.atlassian.net/servicedesk/customer/portal/15/group/20/create/40",
"Source": "https://github.com/phonexia/protofiles"
},
"split_keywords": [
"grpc",
" voice",
" voice-biometry",
" speech",
" language",
" stt",
" whisper"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "69851b8ac67dd8bd71920b32b017f1eadc89e256ac4114c207a2fe2f52b77855",
"md5": "694b29fb3859407b2f21844e15dd4110",
"sha256": "54a888fe43108bb7ffb4faf57ca57c19f9cbac7c79f7aa4893208e933bb8dd87"
},
"downloads": -1,
"filename": "phonexia_grpc-2.0.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "694b29fb3859407b2f21844e15dd4110",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.8",
"size": 38535,
"upload_time": "2024-04-25T12:36:45",
"upload_time_iso_8601": "2024-04-25T12:36:45.406751Z",
"url": "https://files.pythonhosted.org/packages/69/85/1b8ac67dd8bd71920b32b017f1eadc89e256ac4114c207a2fe2f52b77855/phonexia_grpc-2.0.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2b05f9077efee5837dffd4692dd5c96f126cd385ed6bdd778a3ad1a7a0233537",
"md5": "c108a65c107b94fd4cc9eed4385a6baa",
"sha256": "b58ccc5e84b6a6306c506615664e0606b2b5266beb13618dc03b67e40eff1252"
},
"downloads": -1,
"filename": "phonexia_grpc-2.0.0.tar.gz",
"has_sig": false,
"md5_digest": "c108a65c107b94fd4cc9eed4385a6baa",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.8",
"size": 20590,
"upload_time": "2024-04-25T12:36:47",
"upload_time_iso_8601": "2024-04-25T12:36:47.731459Z",
"url": "https://files.pythonhosted.org/packages/2b/05/f9077efee5837dffd4692dd5c96f126cd385ed6bdd778a3ad1a7a0233537/phonexia_grpc-2.0.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-04-25 12:36:47",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "phonexia",
"github_project": "protofiles",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "phonexia-grpc"
}