Name | prettier-prints JSON |
Version |
2.0.2
JSON |
| download |
home_page | |
Summary | Lightweight library for prettier terminal outputs and debugging similar to chalk for Javascript |
upload_time | 2023-09-22 03:17:18 |
maintainer | |
docs_url | None |
author | Dylan Stocking |
requires_python | |
license | MIT |
keywords |
prints
chalk
output
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# Prettier_prints
Lightweight library for prettier terminal outputs similar to [chalk](https://github.com/chalk/chalk) for JavaScript.
Helping make Python outputs easier to read and styled to your desires.
### Install
```python
pip install prettier-prints
```
Examples:
out()
```python
from prettier_prints.prettier_prints import PrettierPrints
pp = PrettierPrints()
pp.style = 'red;underline' # <-- Can optionally predefine styling and can be overwritten
print(pp.out(msg="Lets test the output"))
print(pp.out(msg="Lets override the styling here", style="blue;bold"))
print(pp.out(json_out={'msg': 'For those who prefer a JSON object param, this works too'}))
print(pp.out(json_out={'msg': 'Can also overwrite this way', 'style': 'yellow;highlight'}))
print(f'Works great for output messages as well -> {pp.out(msg="See :)", style="magenta")}')
```

json()
```python
from prettier_prints.prettier_prints import PrettierPrints
pp = PrettierPrints()
json_obj = {'test': 'cool', 'test_two': 'cool_two', 'dict_check': {'test': 'hello'},
'list_check': [
'test',
'test two',
{
'test': 'hello',
'test_two': 'bloop'
},
{
'test': 'hello',
'test_two': 'bloop'
}
]}
print(prettier_prints.json(json_obj=json_obj, style='list=blue;underline&dict=red;bold&string=green;'))
```
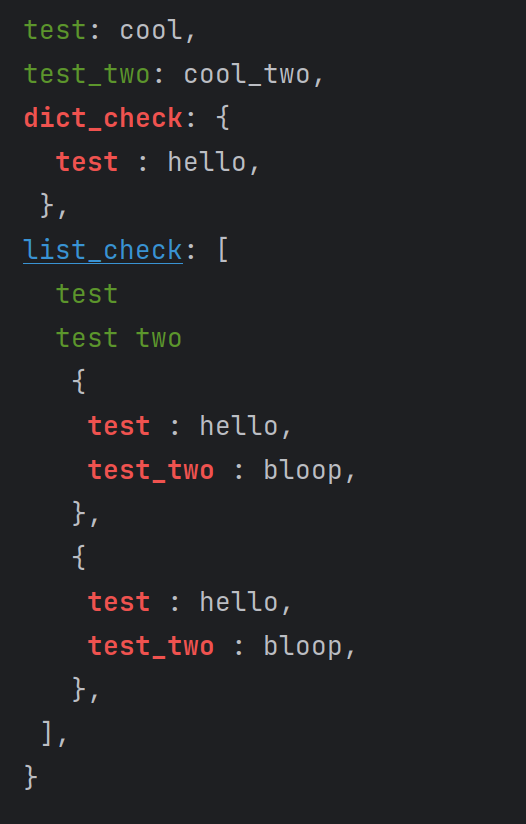
### Current functions:
- out()
- json()
### Function parameters:
- out()
- msg: str Output / display message[required]
- style: str Styling to apply to the message[optional]
- json_out: dict JSON object containing the message and styling[optional]
- json()
- style: str Styling to apply to the message[optional]
- json_obj: dict JSON object containing the message and styling[required]
### Styling Examples By Function:
- out()
- style: 'red;underline'
- json_out: {'msg': 'Lets test the output', 'style': 'blue;bold'}
- json()
- style: 'list=red;underline&dict=blue;bold&str=yellow;highlight' # <- Break up the styling by type (list, dict, str)
### Current available styling:
| Modifiers | Colors / Background Colors |
|:----------|:--------------------------:|
| Bold | Red |
| Underline | Green |
| Highlight | Yellow |
| | Blue |
| | Magenta |
| | Cyan |
| | White |
| | Bright Red |
| | Bright Green |
| | Bright Yellow |
| | Bright Blue |
| | Bright Magenta |
| | Bright Cyan |
| | Bright White |
### Contributors / Thanks:
- [SheepDogg586](https://github.com/SheepDogg586)
Raw data
{
"_id": null,
"home_page": "",
"name": "prettier-prints",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "prints,chalk,output",
"author": "Dylan Stocking",
"author_email": "phantomleak@protonmail.com",
"download_url": "https://files.pythonhosted.org/packages/11/8d/4c72426069966af76a2c3c67cd0f9b430e50ff112425c14fac927e4bd037/prettier_prints-2.0.2.tar.gz",
"platform": null,
"description": "# Prettier_prints\n\nLightweight library for prettier terminal outputs similar to [chalk](https://github.com/chalk/chalk) for JavaScript. \nHelping make Python outputs easier to read and styled to your desires.\n\n### Install\n```python\npip install prettier-prints\n```\n\nExamples:\nout() \n```python\n from prettier_prints.prettier_prints import PrettierPrints\n pp = PrettierPrints()\n pp.style = 'red;underline' # <-- Can optionally predefine styling and can be overwritten\n\n print(pp.out(msg=\"Lets test the output\"))\n print(pp.out(msg=\"Lets override the styling here\", style=\"blue;bold\"))\n print(pp.out(json_out={'msg': 'For those who prefer a JSON object param, this works too'}))\n print(pp.out(json_out={'msg': 'Can also overwrite this way', 'style': 'yellow;highlight'}))\n print(f'Works great for output messages as well -> {pp.out(msg=\"See :)\", style=\"magenta\")}')\n```\n\n\njson()\n```python\n from prettier_prints.prettier_prints import PrettierPrints\n pp = PrettierPrints()\n json_obj = {'test': 'cool', 'test_two': 'cool_two', 'dict_check': {'test': 'hello'},\n 'list_check': [\n 'test',\n 'test two',\n {\n 'test': 'hello',\n 'test_two': 'bloop'\n },\n {\n 'test': 'hello',\n 'test_two': 'bloop'\n }\n ]}\n print(prettier_prints.json(json_obj=json_obj, style='list=blue;underline&dict=red;bold&string=green;'))\n```\n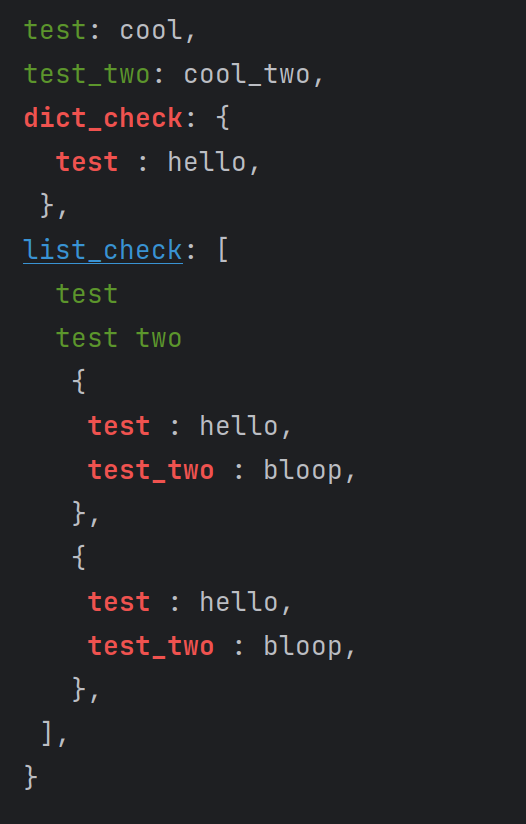\n\n### Current functions:\n - out()\n - json()\n\n### Function parameters:\n - out()\n - msg: str Output / display message[required]\n - style: str Styling to apply to the message[optional]\n - json_out: dict JSON object containing the message and styling[optional]\n\n - json()\n - style: str Styling to apply to the message[optional]\n - json_obj: dict JSON object containing the message and styling[required]\n \n### Styling Examples By Function:\n - out()\n - style: 'red;underline'\n - json_out: {'msg': 'Lets test the output', 'style': 'blue;bold'}\n - json()\n - style: 'list=red;underline&dict=blue;bold&str=yellow;highlight' # <- Break up the styling by type (list, dict, str)\n\n### Current available styling:\n| Modifiers | Colors / Background Colors | \n|:----------|:--------------------------:|\n| Bold | Red |\n| Underline | Green | \n| Highlight | Yellow |\n| | Blue |\n| | Magenta |\n| | Cyan |\n| | White |\n| | Bright Red |\n| | Bright Green |\n| | Bright Yellow |\n| | Bright Blue |\n| | Bright Magenta |\n| | Bright Cyan |\n| | Bright White |\n\n### Contributors / Thanks:\n - [SheepDogg586](https://github.com/SheepDogg586)\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Lightweight library for prettier terminal outputs and debugging similar to chalk for Javascript",
"version": "2.0.2",
"project_urls": {
"Bug Tracker": "https://github.com/PhantomLeak/prettier_prints/issues",
"Code Repo": "https://github.com/PhantomLeak/prettier_prints"
},
"split_keywords": [
"prints",
"chalk",
"output"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "4158cf166a9c37bb5b74441118e478ff6f5a9cd447cf23775a31f61f93a17a40",
"md5": "ff9f7bdf8d514b570af7cec1413d1591",
"sha256": "1b559e651229891748d594ad912b743f7c03b220769044a1333759a16ee10874"
},
"downloads": -1,
"filename": "prettier_prints-2.0.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "ff9f7bdf8d514b570af7cec1413d1591",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 5303,
"upload_time": "2023-09-22T03:17:16",
"upload_time_iso_8601": "2023-09-22T03:17:16.783891Z",
"url": "https://files.pythonhosted.org/packages/41/58/cf166a9c37bb5b74441118e478ff6f5a9cd447cf23775a31f61f93a17a40/prettier_prints-2.0.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "118d4c72426069966af76a2c3c67cd0f9b430e50ff112425c14fac927e4bd037",
"md5": "a39acc50121903cfdb5259db48a882d4",
"sha256": "cba9a81a0333433f7c99259e7e1c8c06cb96c438b6fa3ed564e964b5b6314cb9"
},
"downloads": -1,
"filename": "prettier_prints-2.0.2.tar.gz",
"has_sig": false,
"md5_digest": "a39acc50121903cfdb5259db48a882d4",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 4836,
"upload_time": "2023-09-22T03:17:18",
"upload_time_iso_8601": "2023-09-22T03:17:18.202898Z",
"url": "https://files.pythonhosted.org/packages/11/8d/4c72426069966af76a2c3c67cd0f9b430e50ff112425c14fac927e4bd037/prettier_prints-2.0.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-09-22 03:17:18",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "PhantomLeak",
"github_project": "prettier_prints",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "prettier-prints"
}