Name | probreg JSON |
Version |
0.3.8
JSON |
| download |
home_page | https://github.com/neka-nat/probreg |
Summary | Probablistic point cloud resitration algorithms |
upload_time | 2024-05-11 06:42:17 |
maintainer | None |
docs_url | None |
author | neka-nat |
requires_python | None |
license | None |
keywords |
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# 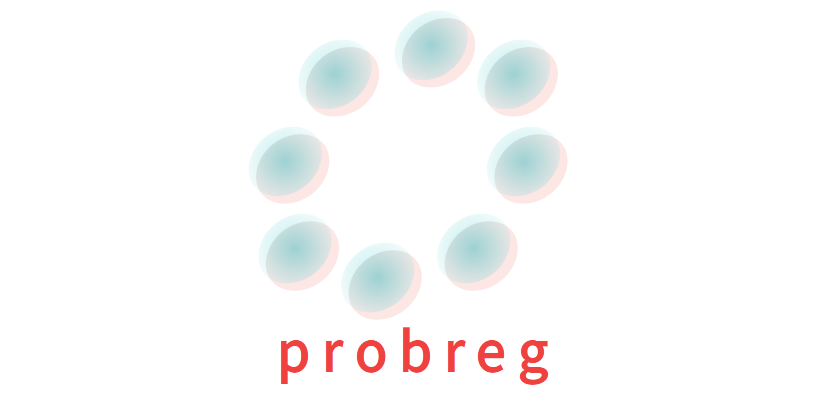
[](https://github.com/neka-nat/probreg/actions/workflows/build-and-test.yaml/badge.svg)
[](https://badge.fury.io/py/probreg)
[](LICENSE)
[](https://probreg.readthedocs.io/en/latest/?badge=latest)
[](https://pepy.tech/project/probreg)
Probreg is a library that implements point cloud **reg**istration algorithms with **prob**ablistic model.
The point set registration algorithms using stochastic model are more robust than ICP(Iterative Closest Point).
This package implements several algorithms using stochastic models and provides a simple interface with [Open3D](http://www.open3d.org/).
## Core features
* Open3D interface
* Rigid and non-rigid transformation
## Algorithms
* Maximum likelihood when the target or source point cloud is observation data
* [Coherent Point Drift (2010)](https://arxiv.org/pdf/0905.2635.pdf)
* [Extended Coherent Point Drift (2016)](https://ieeexplore.ieee.org/abstract/document/7477719) (add correspondence priors to CPD)
* [Color Coherent Point Drift (2018)](https://arxiv.org/pdf/1802.01516)
* [FilterReg (CVPR2019)](https://arxiv.org/pdf/1811.10136.pdf)
* Variational Bayesian inference
* [Bayesian Coherent Point Drift (2020)](https://ieeexplore.ieee.org/stamp/stamp.jsp?arnumber=8985307)
* Distance minimization of two probabilistic distributions
* [GMMReg (2011)](https://ieeexplore.ieee.org/document/5674050)
* [Support Vector Registration (2015)](https://arxiv.org/pdf/1511.04240.pdf)
* Hierarchical Stocastic model
* [GMMTree (ECCV2018)](https://arxiv.org/pdf/1807.02587.pdf)
### Transformations
| type | CPD | SVR, GMMReg | GMMTree | FilterReg | BCPD (experimental) |
|------|-----|-------------|---------|-----------|---------------------|
|Rigid | **Scale + 6D pose** | **6D pose** | **6D pose** | **6D pose** </br> (Point-to-point,</br> Point-to-plane,</br> FPFH-based)| - |
|NonRigid | **Affine**, **MCT** | **TPS** | - | **Deformable Kinematic** </br> (experimental) | **Combined model** </br> (Rigid + Scale + NonRigid-term)
### CUDA support
You need to install cupy.
```
pip install cupy
```
* [Rigid CPD](https://github.com/neka-nat/probreg/blob/master/examples/cpd_rigid_cuda.py)
* [Affine CPD](https://github.com/neka-nat/probreg/blob/master/examples/cpd_affine3d_cuda.py)
## Installation
You can install probreg using `pip`.
```
pip install probreg
```
Or install probreg from source.
```
git clone https://github.com/neka-nat/probreg.git --recursive
cd probreg
pip install -e .
```
## Getting Started
This is a sample code that reads a PCD file and calls CPD registration.
You can easily execute registrations from Open3D point cloud object and draw the results.
```py
import copy
import numpy as np
import open3d as o3
from probreg import cpd
# load source and target point cloud
source = o3.io.read_point_cloud('bunny.pcd')
source.remove_non_finite_points()
target = copy.deepcopy(source)
# transform target point cloud
th = np.deg2rad(30.0)
target.transform(np.array([[np.cos(th), -np.sin(th), 0.0, 0.0],
[np.sin(th), np.cos(th), 0.0, 0.0],
[0.0, 0.0, 1.0, 0.0],
[0.0, 0.0, 0.0, 1.0]]))
source = source.voxel_down_sample(voxel_size=0.005)
target = target.voxel_down_sample(voxel_size=0.005)
# compute cpd registration
tf_param, _, _ = cpd.registration_cpd(source, target)
result = copy.deepcopy(source)
result.points = tf_param.transform(result.points)
# draw result
source.paint_uniform_color([1, 0, 0])
target.paint_uniform_color([0, 1, 0])
result.paint_uniform_color([0, 0, 1])
o3.visualization.draw_geometries([source, target, result])
```
## Resources
* [Documentation](https://probreg.readthedocs.io/en/latest/?badge=latest)
## Results
### Compare algorithms
| CPD | SVR | GMMTree | FilterReg |
|-----|-----|---------|-----------|
| <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/cpd_rigid.gif" width="640"> | <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/svr_rigid.gif" width="640"> | <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/gmmtree_rigid.gif" width="640"> | <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/filterreg_rigid.gif" width="640"> |
### Noise test
| ICP(Open3D) | CPD | FilterReg |
|-------------|-----|-----------|
| <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/icp_noise.gif" width="640"> | <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/cpd_noise.gif" width="640"> | <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/filterreg_noise.gif" width="640"> |
### Non rigid registration
| CPD | SVR | Filterreg | BCPD |
|-----|-----|-----------|------|
| <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/cpd_nonrigid.gif" width="640"> | <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/svr_nonrigid.gif" width="640"> | <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/filterreg_deformable.gif" width="640"> | <img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/bcpd_nonrigid.gif" width="640"> |
### Feature based registration
| FPFH FilterReg |
|----------------|
|<img src="https://raw.githubusercontent.com/neka-nat/probreg/master/images/filterreg_fpfh.gif" width="640"> |
### Time measurement
Execute an example script for measuring time.
```
OMP_NUM_THREADS=1 python time_measurement.py
# Results [s]
# ICP(Open3D): 0.0014092829951550812
# CPD: 0.038112225010991096
# SVR: 0.036476270004641265
# GMMTree: 0.10535842599347234
# FilterReg: 0.005098833993542939
```
## Citing
```
@software{probreg,
author = {{Kenta-Tanaka et al.}},
title = {probreg},
url = {https://probreg.readthedocs.io/en/latest/},
version = {0.1.6},
date = {2019-9-29},
}
```
Raw data
{
"_id": null,
"home_page": "https://github.com/neka-nat/probreg",
"name": "probreg",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": null,
"author": "neka-nat",
"author_email": "nekanat.stock@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/ed/f5/4cda3ac2e064013c293f7b405a9ed2e55f80ef802f2e7811eb6e9ef6b094/probreg-0.3.8.tar.gz",
"platform": null,
"description": "# 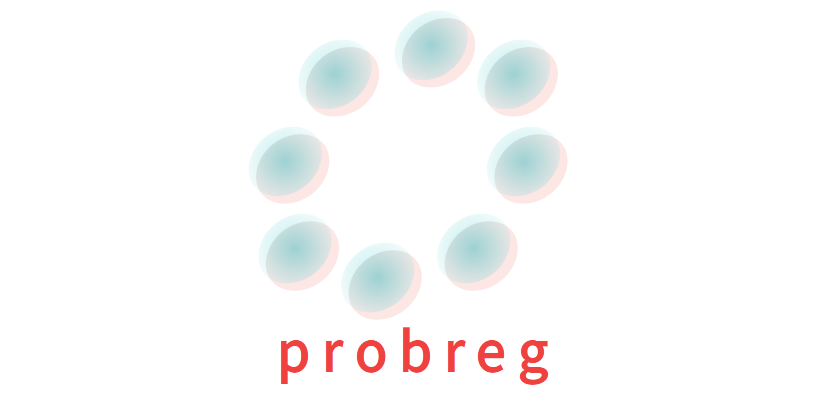\n[](https://github.com/neka-nat/probreg/actions/workflows/build-and-test.yaml/badge.svg)\n[](https://badge.fury.io/py/probreg)\n[](LICENSE)\n[](https://probreg.readthedocs.io/en/latest/?badge=latest)\n[](https://pepy.tech/project/probreg)\n\nProbreg is a library that implements point cloud **reg**istration algorithms with **prob**ablistic model.\n\nThe point set registration algorithms using stochastic model are more robust than ICP(Iterative Closest Point).\nThis package implements several algorithms using stochastic models and provides a simple interface with [Open3D](http://www.open3d.org/).\n\n## Core features\n\n* Open3D interface\n* Rigid and non-rigid transformation\n\n## Algorithms\n\n* Maximum likelihood when the target or source point cloud is observation data\n * [Coherent Point Drift (2010)](https://arxiv.org/pdf/0905.2635.pdf)\n * [Extended Coherent Point Drift (2016)](https://ieeexplore.ieee.org/abstract/document/7477719) (add correspondence priors to CPD)\n * [Color Coherent Point Drift (2018)](https://arxiv.org/pdf/1802.01516)\n * [FilterReg (CVPR2019)](https://arxiv.org/pdf/1811.10136.pdf)\n* Variational Bayesian inference\n * [Bayesian Coherent Point Drift (2020)](https://ieeexplore.ieee.org/stamp/stamp.jsp?arnumber=8985307)\n* Distance minimization of two probabilistic distributions\n * [GMMReg (2011)](https://ieeexplore.ieee.org/document/5674050)\n * [Support Vector Registration (2015)](https://arxiv.org/pdf/1511.04240.pdf)\n* Hierarchical Stocastic model\n * [GMMTree (ECCV2018)](https://arxiv.org/pdf/1807.02587.pdf)\n\n### Transformations\n\n| type | CPD | SVR, GMMReg | GMMTree | FilterReg | BCPD (experimental) |\n|------|-----|-------------|---------|-----------|---------------------|\n|Rigid | **Scale + 6D pose** | **6D pose** | **6D pose** | **6D pose** </br> (Point-to-point,</br> Point-to-plane,</br> FPFH-based)| - |\n|NonRigid | **Affine**, **MCT** | **TPS** | - | **Deformable Kinematic** </br> (experimental) | **Combined model** </br> (Rigid + Scale + NonRigid-term)\n\n### CUDA support\nYou need to install cupy.\n\n```\npip install cupy\n```\n\n* [Rigid CPD](https://github.com/neka-nat/probreg/blob/master/examples/cpd_rigid_cuda.py)\n* [Affine CPD](https://github.com/neka-nat/probreg/blob/master/examples/cpd_affine3d_cuda.py)\n\n## Installation\n\nYou can install probreg using `pip`.\n\n```\npip install probreg\n```\n\nOr install probreg from source.\n\n```\ngit clone https://github.com/neka-nat/probreg.git --recursive\ncd probreg\npip install -e .\n```\n\n## Getting Started\n\nThis is a sample code that reads a PCD file and calls CPD registration.\nYou can easily execute registrations from Open3D point cloud object and draw the results.\n\n```py\nimport copy\nimport numpy as np\nimport open3d as o3\nfrom probreg import cpd\n\n# load source and target point cloud\nsource = o3.io.read_point_cloud('bunny.pcd')\nsource.remove_non_finite_points()\ntarget = copy.deepcopy(source)\n# transform target point cloud\nth = np.deg2rad(30.0)\ntarget.transform(np.array([[np.cos(th), -np.sin(th), 0.0, 0.0],\n [np.sin(th), np.cos(th), 0.0, 0.0],\n [0.0, 0.0, 1.0, 0.0],\n [0.0, 0.0, 0.0, 1.0]]))\nsource = source.voxel_down_sample(voxel_size=0.005)\ntarget = target.voxel_down_sample(voxel_size=0.005)\n\n# compute cpd registration\ntf_param, _, _ = cpd.registration_cpd(source, target)\nresult = copy.deepcopy(source)\nresult.points = tf_param.transform(result.points)\n\n# draw result\nsource.paint_uniform_color([1, 0, 0])\ntarget.paint_uniform_color([0, 1, 0])\nresult.paint_uniform_color([0, 0, 1])\no3.visualization.draw_geometries([source, target, result])\n```\n\n## Resources\n\n* [Documentation](https://probreg.readthedocs.io/en/latest/?badge=latest)\n\n## Results\n\n### Compare algorithms\n\n| CPD | SVR | GMMTree | FilterReg |\n|-----|-----|---------|-----------|\n| <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/cpd_rigid.gif\" width=\"640\"> | <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/svr_rigid.gif\" width=\"640\"> | <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/gmmtree_rigid.gif\" width=\"640\"> | <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/filterreg_rigid.gif\" width=\"640\"> |\n\n### Noise test\n\n| ICP(Open3D) | CPD | FilterReg |\n|-------------|-----|-----------|\n| <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/icp_noise.gif\" width=\"640\"> | <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/cpd_noise.gif\" width=\"640\"> | <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/filterreg_noise.gif\" width=\"640\"> |\n\n### Non rigid registration\n\n| CPD | SVR | Filterreg | BCPD |\n|-----|-----|-----------|------|\n| <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/cpd_nonrigid.gif\" width=\"640\"> | <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/svr_nonrigid.gif\" width=\"640\"> | <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/filterreg_deformable.gif\" width=\"640\"> | <img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/bcpd_nonrigid.gif\" width=\"640\"> |\n\n### Feature based registration\n\n| FPFH FilterReg |\n|----------------|\n|<img src=\"https://raw.githubusercontent.com/neka-nat/probreg/master/images/filterreg_fpfh.gif\" width=\"640\"> |\n\n### Time measurement\n\nExecute an example script for measuring time.\n\n```\nOMP_NUM_THREADS=1 python time_measurement.py\n\n# Results [s]\n# ICP(Open3D): 0.0014092829951550812\n# CPD: 0.038112225010991096\n# SVR: 0.036476270004641265\n# GMMTree: 0.10535842599347234\n# FilterReg: 0.005098833993542939\n```\n\n## Citing\n\n```\n@software{probreg,\n author = {{Kenta-Tanaka et al.}},\n title = {probreg},\n url = {https://probreg.readthedocs.io/en/latest/},\n version = {0.1.6},\n date = {2019-9-29},\n}\n```",
"bugtrack_url": null,
"license": null,
"summary": "Probablistic point cloud resitration algorithms",
"version": "0.3.8",
"project_urls": {
"Homepage": "https://github.com/neka-nat/probreg"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "edf54cda3ac2e064013c293f7b405a9ed2e55f80ef802f2e7811eb6e9ef6b094",
"md5": "8877127558e279094fffa87ac6d0c269",
"sha256": "35558e43ddc68db999692818702c688a53fffb5168d535872edba890e9c6b5f8"
},
"downloads": -1,
"filename": "probreg-0.3.8.tar.gz",
"has_sig": false,
"md5_digest": "8877127558e279094fffa87ac6d0c269",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 1040035,
"upload_time": "2024-05-11T06:42:17",
"upload_time_iso_8601": "2024-05-11T06:42:17.043478Z",
"url": "https://files.pythonhosted.org/packages/ed/f5/4cda3ac2e064013c293f7b405a9ed2e55f80ef802f2e7811eb6e9ef6b094/probreg-0.3.8.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-05-11 06:42:17",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "neka-nat",
"github_project": "probreg",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "probreg"
}