Name | psd-export JSON |
Version |
1.0.33
JSON |
| download |
home_page | None |
Summary | Fast exporting of PSDs with [tagged] layers for variants. |
upload_time | 2025-01-01 18:34:44 |
maintainer | None |
docs_url | None |
author | cromachina |
requires_python | >=3.11.2 |
license | MIT License Copyright (c) 2022 cromachina Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
exporter
psd
art
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# psd-export
- Fast multi-threaded exporting of PSDs with `[tagged]` layers for variants.
- Special named layers can be used to apply filters like mosaic or gaussian blur, or whatever else you can hook in.
- This tool is primarily meant to be compatible with PSDs exported from `PaintTool SAIv2` and `Clip Studio Paint`.
---
### Why
For my art workflow, I typically make a bunch variation layers and also need to apply mosaics to all of them. As a manual process, exporting every variant can take several minutes, with lots of clicking everywhere to turn layers on and off (potentially missing layers by accident) and then adding mosaics can be another 10 or 20 minutes of extra work. If I find I need to change something in my pictures and export again, I have to repeat this whole ordeal. This script puts this export process on the order of seconds, saving me a lot of time and pain.
---
### Installation
- Install python: https://www.python.org/downloads/
- Run `pip install psd-export` to install the script.
- Run `psd-export` to export any PSD files in the current directory.
- Run `psd-export --help` for more command line arguments.
---
### Building from source
- Install a C compiler (like MSVC, GCC, Clang)
- Install extra dependencies: `pip install setuptools wheel cython numpy`
- Install this repository locally: `pip install -e .`
- If you modify a Cython file (.pyx), then rebuild it with: `python setup.py build_ext --inplace`
---
### Setting up the PSD
#### Automatic Exporting
##### Primary tags
- Add tags surrounded by `[]` to the names of layers that you want as part of an exported picture.
- A layer name can contain anything else outside of tags: `scene [1]`, which will be ignored.
- Because whitespace is used to delimit arguments to filters, a tag's name will not include anything after a space, for example:
- `[blur 50]` the tag name will be `blur`, and `50` is an argument to `blur`.
- Each set of primary tagged layers will be turned on and off and then exported in turn.
- That means multiple layers can have the same tag, so you can toggle layers in your foreground and background together, for example:
- A layer named `foreground [1]` and a separate layer named `background [1]`
- A layer can have multiple tags in the name: `scene [1][2]`
- This will export with this layer visible for both tags.
- A primary tag is not necessary. If no primary tag is provided, then the whole picture is exported as is.
##### Secondary tags
- Tags with an `@` in the name will be treated as secondary tags.
- Text before the `@` is the tag name, and text after the `@` is the exclusion group.
- The exclusion group can be empty.
- Valid secondary tag names: `[jp@]`, `[jp@text]`
- These tags will be exported in combination with primary tags, for example:
- If you have layers tagged `[1]`, `[thing@]` `[jp@text]`, and `[en@text]`, then what will be exported is `1`, `1-thing`, `1-thing-jp`, `1-thing-en`, `1-jp`, `1-en`
- Because `[jp@text]` and `[en@text]` share the same exclusion group `text`, they will never be enabled together.
- If there was a second primary tag `[2]` for example, the whole set of combinations would be exported again but with `2` instead of `1`
#### Image filters
- Tags with special names will be treated as filters on the colors below them.
- Filters can double as export tags too.
- If you want a filter to not be treated as an export tag, you can preceed it with `#` to set the ignore flag, for example `[#censor]`
- Filters can have arguments as well to control their behavior, separated by spaces, for example `[#censor 50]`
- If you want the filter to apply to layers outside of the group it is in, then the group blend mode should be set to `pass-through`, otherwise it may blend with transparent black pixels if the filter is over a transparent part of a group. The blur and motion blur filters apply to alpha as well, so they should behave as expected in isolated groups.
- If multiple filters are enabled in one layer, they will be applied from left to right on top of each result, example:
- `[#censor][#blur]` will apply a mosaic, and then a blur on top of that mosaic.
- Blend modes and clipping layers applied directly to filter layers are ignored.
##### Available default filters:
- `[censor mosaic_factor apply_to_alpha]`
- If the `mosaic_factor` argument is omitted, then it is defaulted to 100, which means 1/100th the smallest dimension, (or 4 pixels, whichever is larger) in order to be Pixiv compliant.
- `apply_to_alpha` defaults to False. Any value is treated as True.
- Typically you will want this filter to be a secondary tag, for example: `[censor@]`, so you can have censored and uncensored outputs.
- `[blur size]`
- The `size` argument defaults to 50 if omitted.
- This filter is best used to create a non-destructive blur, such as for a background layer. You can fill an entire layer and set it to `[#blur 8]` for example.
- `[motion-blur angle size]`
- `angle` is in degrees, starting from horizontal to the right; Default 0.
- `size` defaults to 50.
- Best used for non-destructive blur: `[#motion-blur 45 20]`
##### Adding a new filter:
In your own script:
```py
# my-export.py
from psd_export import (export, filters, util, blendfuncs)
import numpy as np
my_arg1_default = 1.0
# Register the filter with this decorator:
@filters.filter('my-filter')
# Only positional arguments work right now. The result of this function replaces the destination color and alpha.
def some_filter(color_dst, color_src, alpha_dst, alpha_src, arg1=None, arg2=100, *_):
# Cast arguments to your desired types, as they will come in as strings.
if arg1 is None:
arg1 = my_arg1_default
arg1 = float(arg1)
# Manipulate color and alpha numpy arrays, in-place if you want.
color = np.subtract(arg1, color, out=color)
color = blendfuncs.lerp(color_dst, color, alpha_src)
# Always return the same shaped arrays as a tuple:
return color, alpha
if __name__ == '__main__':
# Add your own command line arguments if needed.
export.arg_parser.add_argument('--my-arg1', default=my_arg1_default, type=float,
help='Set the arg1 default parameter.')
args = export.arg_parser.parse_args()
my_arg1_default = args.my_arg1
export.main()
```
Apply it to a layer in your PSD, for example:
`[my-filter 20.4]` or `[#my-filter]`, etc.
---
### Examples
In the layers below, there are 2 primary tags and 3 secondary tags (with two unique exclusion groups):
Primary tags: `1`, `2`
Secondary tags: `jp`, `en`, `censor`
Exclusion groups: `text`, `<empty>`
Groups with primary tags will be exported again even if the secondary tag does not exist under that group! This keeps the exported folders uniformly sized for publishing, so different folders may appear to have duplicate outputs.
Example layer configuration in SAI:
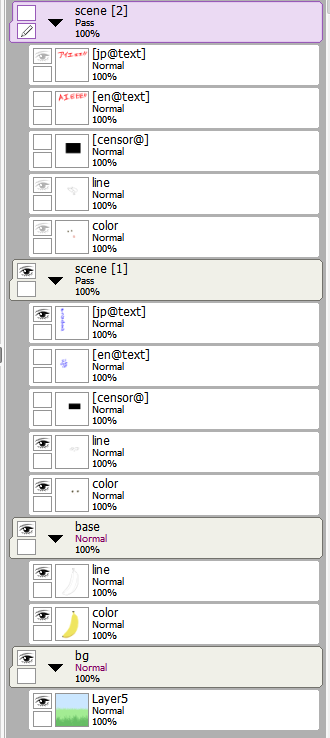
Example output from script, showing every valid combination:
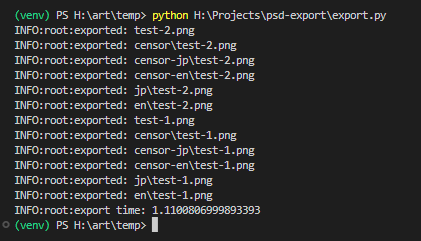
Folder after exporting everything:
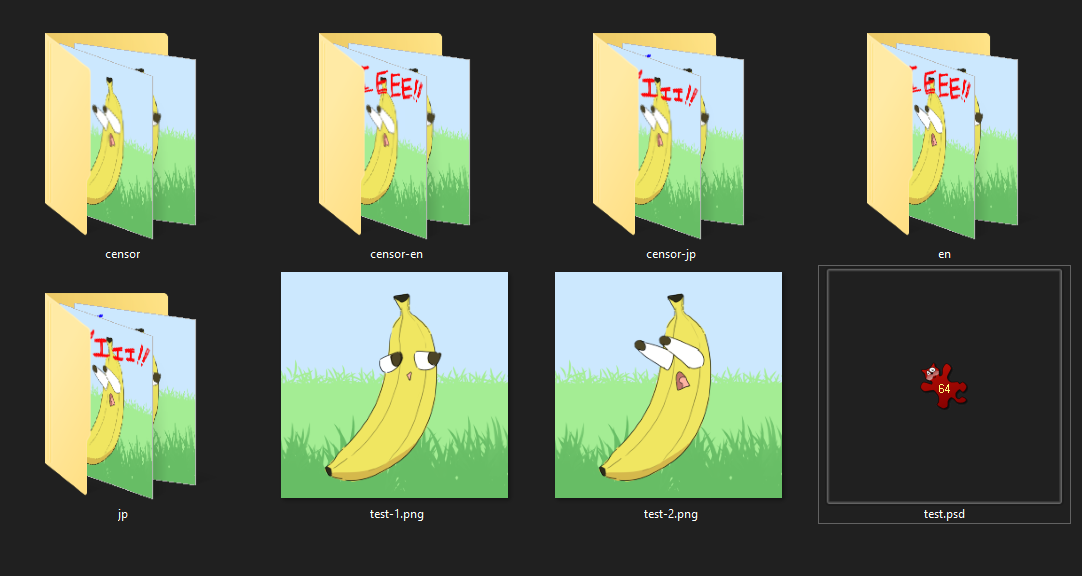
Example of a layer with the tag `[censor@]` (the layer does not need to be set to visible before exporting):
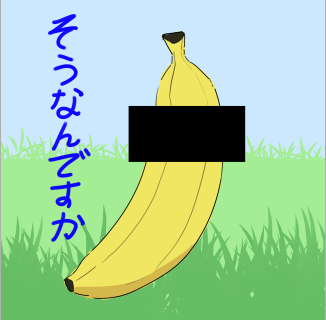
After exporting:
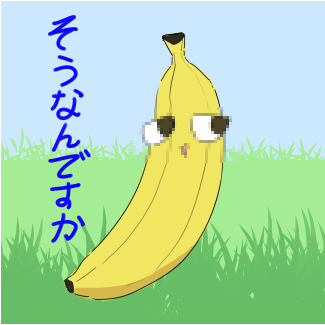
---
### Blendmode status:
| Blendmode | Status |
| - | - |
| Normal | Pass |
| Multiply | Pass |
| Screen | Pass |
| Overlay | Pass |
| Linear Burn (Shade) | Pass |
| Linear Dodge (Shine) | Pass |
| Linear Light (Shade/Shine) | Pass |
| Color Burn (Burn) | Pass |
| Color Dodge (Dodge) | Pass |
| Vivid Light (Burn/Dodge) | Pass |
| Soft Light | Pass |
| Hard Light | Pass |
| Pin Light | Pass |
| Hard Mix | Small precision error for near-black colors, can look slightly different from SAI |
| Darken | Pass |
| Lighten | Pass |
| Darken Color | Pass |
| Lighten Color | Pass |
| Difference | Pass |
| Exclude | Pass |
| Subtract | Pass |
| Divide | Pass |
| Hue | Pass |
| Saturation | Pass |
| Color | Pass |
| Luminosity | Pass |
| [TS] Linear Burn (Shade) | Pass |
| [TS] Linear Dodge (Shine) | Pass |
| [TS] Linear Light (Shade/Shine) | Pass |
| [TS] Color Burn (Burn) | Small precision error for near-black colors, can look slightly different from SAI |
| [TS] Color Dodge (Dodge) | Pass |
| [TS] Vivid Light (Burn/Dodge) | Pass |
| [TS] Hard Mix | Small precision error for near-black colors, can look slightly different from SAI |
| [TS] Difference | Pass |
### Missing PSD features:
- Other things that are not implemented (also not implemented in `psd-tools`):
- Adjustment layers (gradient map, color balance, etc.)
- Layer effects (shadow, glow, overlay, strokes, etc.)
- Font rendering
- Probably some other things I'm unaware of.
---
### TODO:
- Fix blend modes that don't quite work properly. This is low priority because I hardly use these modes myself or merge the results when painting.
- Binary package export
Raw data
{
"_id": null,
"home_page": null,
"name": "psd-export",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.11.2",
"maintainer_email": null,
"keywords": "exporter, psd, art",
"author": "cromachina",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/d2/17/f73aecc883c9759404a3d7b870061c28c0573028a599c2412675f30c9b6d/psd_export-1.0.33.tar.gz",
"platform": null,
"description": "# psd-export\n\n- Fast multi-threaded exporting of PSDs with `[tagged]` layers for variants.\n- Special named layers can be used to apply filters like mosaic or gaussian blur, or whatever else you can hook in.\n- This tool is primarily meant to be compatible with PSDs exported from `PaintTool SAIv2` and `Clip Studio Paint`.\n\n---\n### Why\nFor my art workflow, I typically make a bunch variation layers and also need to apply mosaics to all of them. As a manual process, exporting every variant can take several minutes, with lots of clicking everywhere to turn layers on and off (potentially missing layers by accident) and then adding mosaics can be another 10 or 20 minutes of extra work. If I find I need to change something in my pictures and export again, I have to repeat this whole ordeal. This script puts this export process on the order of seconds, saving me a lot of time and pain.\n\n---\n### Installation\n- Install python: https://www.python.org/downloads/\n- Run `pip install psd-export` to install the script.\n- Run `psd-export` to export any PSD files in the current directory.\n- Run `psd-export --help` for more command line arguments.\n\n---\n### Building from source\n- Install a C compiler (like MSVC, GCC, Clang)\n- Install extra dependencies: `pip install setuptools wheel cython numpy`\n- Install this repository locally: `pip install -e .`\n- If you modify a Cython file (.pyx), then rebuild it with: `python setup.py build_ext --inplace`\n\n---\n### Setting up the PSD\n\n#### Automatic Exporting\n\n##### Primary tags\n- Add tags surrounded by `[]` to the names of layers that you want as part of an exported picture.\n - A layer name can contain anything else outside of tags: `scene [1]`, which will be ignored.\n- Because whitespace is used to delimit arguments to filters, a tag's name will not include anything after a space, for example:\n - `[blur 50]` the tag name will be `blur`, and `50` is an argument to `blur`.\n- Each set of primary tagged layers will be turned on and off and then exported in turn.\n - That means multiple layers can have the same tag, so you can toggle layers in your foreground and background together, for example:\n - A layer named `foreground [1]` and a separate layer named `background [1]`\n- A layer can have multiple tags in the name: `scene [1][2]`\n - This will export with this layer visible for both tags.\n- A primary tag is not necessary. If no primary tag is provided, then the whole picture is exported as is.\n\n##### Secondary tags\n- Tags with an `@` in the name will be treated as secondary tags.\n- Text before the `@` is the tag name, and text after the `@` is the exclusion group.\n - The exclusion group can be empty.\n - Valid secondary tag names: `[jp@]`, `[jp@text]`\n- These tags will be exported in combination with primary tags, for example:\n - If you have layers tagged `[1]`, `[thing@]` `[jp@text]`, and `[en@text]`, then what will be exported is `1`, `1-thing`, `1-thing-jp`, `1-thing-en`, `1-jp`, `1-en`\n - Because `[jp@text]` and `[en@text]` share the same exclusion group `text`, they will never be enabled together.\n - If there was a second primary tag `[2]` for example, the whole set of combinations would be exported again but with `2` instead of `1`\n\n#### Image filters\n- Tags with special names will be treated as filters on the colors below them.\n- Filters can double as export tags too.\n- If you want a filter to not be treated as an export tag, you can preceed it with `#` to set the ignore flag, for example `[#censor]`\n- Filters can have arguments as well to control their behavior, separated by spaces, for example `[#censor 50]`\n- If you want the filter to apply to layers outside of the group it is in, then the group blend mode should be set to `pass-through`, otherwise it may blend with transparent black pixels if the filter is over a transparent part of a group. The blur and motion blur filters apply to alpha as well, so they should behave as expected in isolated groups.\n- If multiple filters are enabled in one layer, they will be applied from left to right on top of each result, example:\n - `[#censor][#blur]` will apply a mosaic, and then a blur on top of that mosaic.\n- Blend modes and clipping layers applied directly to filter layers are ignored.\n\n##### Available default filters:\n- `[censor mosaic_factor apply_to_alpha]`\n - If the `mosaic_factor` argument is omitted, then it is defaulted to 100, which means 1/100th the smallest dimension, (or 4 pixels, whichever is larger) in order to be Pixiv compliant.\n - `apply_to_alpha` defaults to False. Any value is treated as True.\n - Typically you will want this filter to be a secondary tag, for example: `[censor@]`, so you can have censored and uncensored outputs.\n- `[blur size]`\n - The `size` argument defaults to 50 if omitted.\n - This filter is best used to create a non-destructive blur, such as for a background layer. You can fill an entire layer and set it to `[#blur 8]` for example.\n- `[motion-blur angle size]`\n - `angle` is in degrees, starting from horizontal to the right; Default 0.\n - `size` defaults to 50.\n - Best used for non-destructive blur: `[#motion-blur 45 20]`\n\n##### Adding a new filter:\nIn your own script:\n```py\n# my-export.py\nfrom psd_export import (export, filters, util, blendfuncs)\nimport numpy as np\n\nmy_arg1_default = 1.0\n\n# Register the filter with this decorator:\n@filters.filter('my-filter')\n# Only positional arguments work right now. The result of this function replaces the destination color and alpha.\ndef some_filter(color_dst, color_src, alpha_dst, alpha_src, arg1=None, arg2=100, *_):\n # Cast arguments to your desired types, as they will come in as strings.\n if arg1 is None:\n arg1 = my_arg1_default\n arg1 = float(arg1)\n # Manipulate color and alpha numpy arrays, in-place if you want.\n color = np.subtract(arg1, color, out=color)\n color = blendfuncs.lerp(color_dst, color, alpha_src)\n # Always return the same shaped arrays as a tuple:\n return color, alpha\n\nif __name__ == '__main__':\n # Add your own command line arguments if needed.\n export.arg_parser.add_argument('--my-arg1', default=my_arg1_default, type=float,\n help='Set the arg1 default parameter.')\n args = export.arg_parser.parse_args()\n my_arg1_default = args.my_arg1\n\n export.main()\n```\n\nApply it to a layer in your PSD, for example:\n`[my-filter 20.4]` or `[#my-filter]`, etc.\n\n---\n### Examples\nIn the layers below, there are 2 primary tags and 3 secondary tags (with two unique exclusion groups):\n\nPrimary tags: `1`, `2`\n\nSecondary tags: `jp`, `en`, `censor`\n\nExclusion groups: `text`, `<empty>`\n\nGroups with primary tags will be exported again even if the secondary tag does not exist under that group! This keeps the exported folders uniformly sized for publishing, so different folders may appear to have duplicate outputs.\n\nExample layer configuration in SAI:\n\n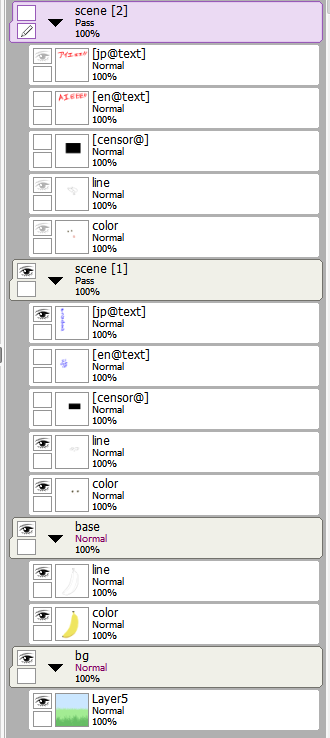\n\nExample output from script, showing every valid combination:\n\n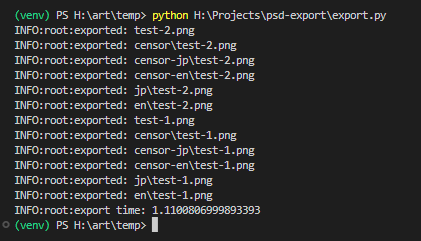\n\nFolder after exporting everything:\n\n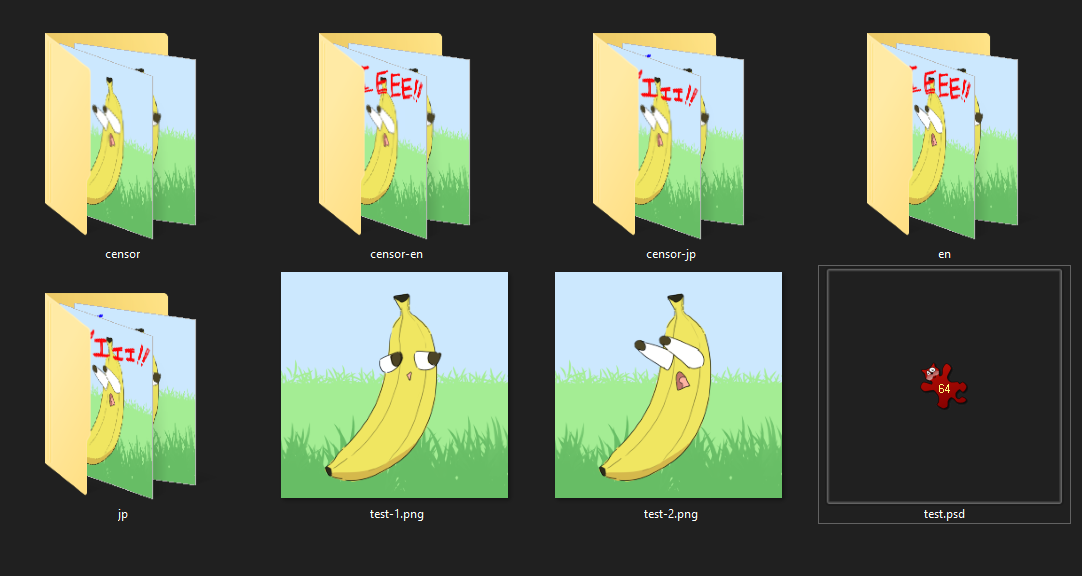\n\nExample of a layer with the tag `[censor@]` (the layer does not need to be set to visible before exporting):\n\n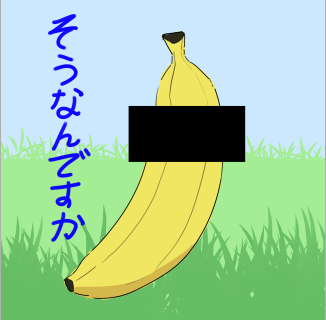\n\nAfter exporting:\n\n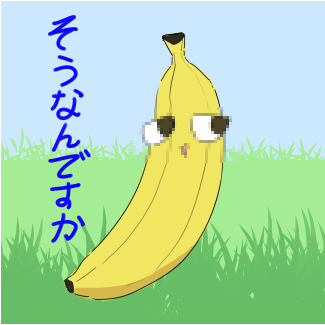\n\n---\n### Blendmode status:\n| Blendmode | Status |\n| - | - |\n| Normal | Pass |\n| Multiply | Pass |\n| Screen | Pass |\n| Overlay | Pass |\n| Linear Burn (Shade) | Pass |\n| Linear Dodge (Shine) | Pass |\n| Linear Light (Shade/Shine) | Pass |\n| Color Burn (Burn) | Pass |\n| Color Dodge (Dodge) | Pass |\n| Vivid Light (Burn/Dodge) | Pass |\n| Soft Light | Pass |\n| Hard Light | Pass |\n| Pin Light | Pass |\n| Hard Mix | Small precision error for near-black colors, can look slightly different from SAI |\n| Darken | Pass |\n| Lighten | Pass |\n| Darken Color | Pass |\n| Lighten Color | Pass |\n| Difference | Pass |\n| Exclude | Pass |\n| Subtract | Pass |\n| Divide | Pass |\n| Hue | Pass |\n| Saturation | Pass |\n| Color | Pass |\n| Luminosity | Pass |\n| [TS] Linear Burn (Shade) | Pass |\n| [TS] Linear Dodge (Shine) | Pass |\n| [TS] Linear Light (Shade/Shine) | Pass |\n| [TS] Color Burn (Burn) | Small precision error for near-black colors, can look slightly different from SAI |\n| [TS] Color Dodge (Dodge) | Pass |\n| [TS] Vivid Light (Burn/Dodge) | Pass |\n| [TS] Hard Mix | Small precision error for near-black colors, can look slightly different from SAI |\n| [TS] Difference | Pass |\n\n### Missing PSD features:\n- Other things that are not implemented (also not implemented in `psd-tools`):\n - Adjustment layers (gradient map, color balance, etc.)\n - Layer effects (shadow, glow, overlay, strokes, etc.)\n - Font rendering\n - Probably some other things I'm unaware of.\n\n---\n### TODO:\n- Fix blend modes that don't quite work properly. This is low priority because I hardly use these modes myself or merge the results when painting.\n- Binary package export\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2022 cromachina Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ",
"summary": "Fast exporting of PSDs with [tagged] layers for variants.",
"version": "1.0.33",
"project_urls": {
"Homepage": "https://github.com/cromachina/psd-export"
},
"split_keywords": [
"exporter",
" psd",
" art"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "55eae728b5b200f9f3c82872d043d6d4251d81f66f33257522587a255ba91c39",
"md5": "37c0d8ae3d078fd5cec56b112a3cd295",
"sha256": "9bcab353901f3fba68b6a4801c6712198da41eecb223b21b2e2c6d223070fa77"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp311-cp311-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "37c0d8ae3d078fd5cec56b112a3cd295",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.11.2",
"size": 397358,
"upload_time": "2025-01-01T18:33:47",
"upload_time_iso_8601": "2025-01-01T18:33:47.803105Z",
"url": "https://files.pythonhosted.org/packages/55/ea/e728b5b200f9f3c82872d043d6d4251d81f66f33257522587a255ba91c39/psd_export-1.0.33-cp311-cp311-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "364fe75809aec4d1c74dfece0f521d771e4ba530f62e254e3ade8120945e5bd0",
"md5": "bfe2602496117c0f4b37143e6160d99a",
"sha256": "e7442e9b573bc9a20b75a9731bb6057f9c1c6c0f2edef641bfdd5f5828dea541"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp311-cp311-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "bfe2602496117c0f4b37143e6160d99a",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.11.2",
"size": 384033,
"upload_time": "2025-01-01T18:33:49",
"upload_time_iso_8601": "2025-01-01T18:33:49.203776Z",
"url": "https://files.pythonhosted.org/packages/36/4f/e75809aec4d1c74dfece0f521d771e4ba530f62e254e3ade8120945e5bd0/psd_export-1.0.33-cp311-cp311-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d2186e6a7978e50e959816c1493301531737dc086733cdf83de6a28535e174de",
"md5": "7fe280ce70ed969038523232147a5403",
"sha256": "2166af0c6448bb5c5dbbd33a53b3abf927c6c571bd5f16821e272e3518013bdd"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "7fe280ce70ed969038523232147a5403",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.11.2",
"size": 1079578,
"upload_time": "2025-01-01T18:33:50",
"upload_time_iso_8601": "2025-01-01T18:33:50.587428Z",
"url": "https://files.pythonhosted.org/packages/d2/18/6e6a7978e50e959816c1493301531737dc086733cdf83de6a28535e174de/psd_export-1.0.33-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "f492c9410c203de5ca0e3fd5b28a3f0ffaaeea2123531f013ebfe85f2af8e544",
"md5": "eef3d71e1d6203fa5856d7d6256e107e",
"sha256": "04eb457a1a2db82d105fdd0b53aebb56087ec599874f727e64f65723d09dfadf"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "eef3d71e1d6203fa5856d7d6256e107e",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.11.2",
"size": 1092746,
"upload_time": "2025-01-01T18:33:53",
"upload_time_iso_8601": "2025-01-01T18:33:53.132998Z",
"url": "https://files.pythonhosted.org/packages/f4/92/c9410c203de5ca0e3fd5b28a3f0ffaaeea2123531f013ebfe85f2af8e544/psd_export-1.0.33-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4f0dc560be20c779ee2ce6c94d53d75aad105a5732cfbaca6caa79fa27893bf7",
"md5": "e2c21f74ed03d899150309b8b1a94cfc",
"sha256": "dadf772787bbdbdc482ff91bf139cf8443c73bffc8d1794230b9561f8f249114"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp311-cp311-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl",
"has_sig": false,
"md5_digest": "e2c21f74ed03d899150309b8b1a94cfc",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.11.2",
"size": 1050968,
"upload_time": "2025-01-01T18:33:56",
"upload_time_iso_8601": "2025-01-01T18:33:56.178244Z",
"url": "https://files.pythonhosted.org/packages/4f/0d/c560be20c779ee2ce6c94d53d75aad105a5732cfbaca6caa79fa27893bf7/psd_export-1.0.33-cp311-cp311-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1d05898e57a39e89ba172bad94f15162abad53856313003551ab71156ad2f6c5",
"md5": "edb5b6f203cf6037c752519feabdf00a",
"sha256": "f588d32279bf998b61d8f0d454c37c97dcedcc66abcb233afd3b28a76053ca7b"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp311-cp311-musllinux_1_2_aarch64.whl",
"has_sig": false,
"md5_digest": "edb5b6f203cf6037c752519feabdf00a",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.11.2",
"size": 1089721,
"upload_time": "2025-01-01T18:33:57",
"upload_time_iso_8601": "2025-01-01T18:33:57.493388Z",
"url": "https://files.pythonhosted.org/packages/1d/05/898e57a39e89ba172bad94f15162abad53856313003551ab71156ad2f6c5/psd_export-1.0.33-cp311-cp311-musllinux_1_2_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d2894afa59c31d2092e826656cf2e2b96facdd818c30e63df929d58b6a868211",
"md5": "efb492a16fcfad2ddff8d9a34dd03177",
"sha256": "2f39b9037590c3615815aec241cd357f629f02eaa40acf385cd07783f3ff5016"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp311-cp311-musllinux_1_2_i686.whl",
"has_sig": false,
"md5_digest": "efb492a16fcfad2ddff8d9a34dd03177",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.11.2",
"size": 1094096,
"upload_time": "2025-01-01T18:33:58",
"upload_time_iso_8601": "2025-01-01T18:33:58.849158Z",
"url": "https://files.pythonhosted.org/packages/d2/89/4afa59c31d2092e826656cf2e2b96facdd818c30e63df929d58b6a868211/psd_export-1.0.33-cp311-cp311-musllinux_1_2_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "cfc2e66f37df8127d9047c31735f56e76d312861ba60dd4d38f17f5c505dc778",
"md5": "b37aa2c5b49be1b982dba8d17a474787",
"sha256": "e00fcb76efc3c2e784f1cd690548de58931ce758677f2ab63efb2d2de650a102"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp311-cp311-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "b37aa2c5b49be1b982dba8d17a474787",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.11.2",
"size": 1126952,
"upload_time": "2025-01-01T18:34:00",
"upload_time_iso_8601": "2025-01-01T18:34:00.208690Z",
"url": "https://files.pythonhosted.org/packages/cf/c2/e66f37df8127d9047c31735f56e76d312861ba60dd4d38f17f5c505dc778/psd_export-1.0.33-cp311-cp311-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "00e9277ecb2fed6437b4aabe67496354a2ca631ba5eaec10a1a49284ec7f593f",
"md5": "bbde9f6e8674db8a7861c7a0f4bccfc6",
"sha256": "d67dcd349614f65233b0d84b0c4731d6cddf8e6d2c5f0af08def8886b2776ab6"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp311-cp311-win32.whl",
"has_sig": false,
"md5_digest": "bbde9f6e8674db8a7861c7a0f4bccfc6",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.11.2",
"size": 366376,
"upload_time": "2025-01-01T18:34:01",
"upload_time_iso_8601": "2025-01-01T18:34:01.427553Z",
"url": "https://files.pythonhosted.org/packages/00/e9/277ecb2fed6437b4aabe67496354a2ca631ba5eaec10a1a49284ec7f593f/psd_export-1.0.33-cp311-cp311-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1cbe18fba42c20fadccce8820c8998b34f9f811ef9d26852a8b470633b835e65",
"md5": "9ffd4534813a28174630a5dd3c59140c",
"sha256": "1b72a0fd7bd1b72862416167f63aefc486c0301cb0b54414e91f12feba08cdf4"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp311-cp311-win_amd64.whl",
"has_sig": false,
"md5_digest": "9ffd4534813a28174630a5dd3c59140c",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.11.2",
"size": 383985,
"upload_time": "2025-01-01T18:34:03",
"upload_time_iso_8601": "2025-01-01T18:34:03.757046Z",
"url": "https://files.pythonhosted.org/packages/1c/be/18fba42c20fadccce8820c8998b34f9f811ef9d26852a8b470633b835e65/psd_export-1.0.33-cp311-cp311-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d8c48c8788cca9b77b9cbeaf12d7a5d929df640f93d7b78051fe27aa352bc31d",
"md5": "292d047ec2d949a775704e73247271c3",
"sha256": "e72c19b240a36d77114c7ffe4ffd46f76efd5c1c6ee54938b1e351c74aae7c25"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp312-cp312-macosx_10_13_x86_64.whl",
"has_sig": false,
"md5_digest": "292d047ec2d949a775704e73247271c3",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.11.2",
"size": 393164,
"upload_time": "2025-01-01T18:34:04",
"upload_time_iso_8601": "2025-01-01T18:34:04.910809Z",
"url": "https://files.pythonhosted.org/packages/d8/c4/8c8788cca9b77b9cbeaf12d7a5d929df640f93d7b78051fe27aa352bc31d/psd_export-1.0.33-cp312-cp312-macosx_10_13_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "aea2e3ed1346712653185093570e73c331d189579d7baf55256c01ddd16f34bc",
"md5": "0734c13cf8abd93e00cff8352b3ab348",
"sha256": "aab405d4a6efa946e909ec35f6f14f940e3b0e8108a93875972a2d6109149cd1"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp312-cp312-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "0734c13cf8abd93e00cff8352b3ab348",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.11.2",
"size": 382937,
"upload_time": "2025-01-01T18:34:06",
"upload_time_iso_8601": "2025-01-01T18:34:06.058499Z",
"url": "https://files.pythonhosted.org/packages/ae/a2/e3ed1346712653185093570e73c331d189579d7baf55256c01ddd16f34bc/psd_export-1.0.33-cp312-cp312-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "3148d4b0d775dc3f60e18219e9f48e3f44b6a79431a615ab1259da8f6f590e76",
"md5": "b760ae12fbeb5c3a63d23faf4025ab4c",
"sha256": "0bd8d6efa2ff706bf089b8c0597d769daa7a190ac75d77b47998f011e522a8f1"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "b760ae12fbeb5c3a63d23faf4025ab4c",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.11.2",
"size": 1062947,
"upload_time": "2025-01-01T18:34:08",
"upload_time_iso_8601": "2025-01-01T18:34:08.457650Z",
"url": "https://files.pythonhosted.org/packages/31/48/d4b0d775dc3f60e18219e9f48e3f44b6a79431a615ab1259da8f6f590e76/psd_export-1.0.33-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "e0655bfff8915c09161c8b6be2ce4f21a7c785b57ba9d49fd7a1b8cbce42d8da",
"md5": "fa5433fa1afef6094349eb6df6f0ea58",
"sha256": "f6f05d73d567ea3b7fc1b1d33ed6285b5685b8ea0ed17e544b9e932ae8dfd941"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "fa5433fa1afef6094349eb6df6f0ea58",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.11.2",
"size": 1084409,
"upload_time": "2025-01-01T18:34:10",
"upload_time_iso_8601": "2025-01-01T18:34:10.957706Z",
"url": "https://files.pythonhosted.org/packages/e0/65/5bfff8915c09161c8b6be2ce4f21a7c785b57ba9d49fd7a1b8cbce42d8da/psd_export-1.0.33-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "68d1af332d9f2c0353c34d3a787a2342a86ec4c3b5febd560c69c3d8ae100ce9",
"md5": "ccf974815bb14f7b5ddaf1ccac3b1705",
"sha256": "ee998e0b07e5c225577d6569221207ee8f4580693ad3082c5aff57018c78bfbe"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp312-cp312-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl",
"has_sig": false,
"md5_digest": "ccf974815bb14f7b5ddaf1ccac3b1705",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.11.2",
"size": 1041030,
"upload_time": "2025-01-01T18:34:13",
"upload_time_iso_8601": "2025-01-01T18:34:13.098455Z",
"url": "https://files.pythonhosted.org/packages/68/d1/af332d9f2c0353c34d3a787a2342a86ec4c3b5febd560c69c3d8ae100ce9/psd_export-1.0.33-cp312-cp312-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9eb723e789063248c04f50768136f6ec5fc2bea07f203a70e08d0638a31b6588",
"md5": "e03c71db654235d4e86e6d606d8c94c9",
"sha256": "b94d8cb6eb4346bd55dd16fd421b286c7300ebbfb097422c0bb06443dfe9ede6"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp312-cp312-musllinux_1_2_aarch64.whl",
"has_sig": false,
"md5_digest": "e03c71db654235d4e86e6d606d8c94c9",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.11.2",
"size": 1073281,
"upload_time": "2025-01-01T18:34:15",
"upload_time_iso_8601": "2025-01-01T18:34:15.620310Z",
"url": "https://files.pythonhosted.org/packages/9e/b7/23e789063248c04f50768136f6ec5fc2bea07f203a70e08d0638a31b6588/psd_export-1.0.33-cp312-cp312-musllinux_1_2_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "355b428d04edf9a9a21f00c9a037c9998d51bbba88c2332ace0b7d1af67200af",
"md5": "c80bddcfc9e89db6f1f7e458905b8c46",
"sha256": "d1d883fc2af910ad1bd9fb66110b4d4ad48b2fc98631fdbbdb883b5e2b52084b"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp312-cp312-musllinux_1_2_i686.whl",
"has_sig": false,
"md5_digest": "c80bddcfc9e89db6f1f7e458905b8c46",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.11.2",
"size": 1090552,
"upload_time": "2025-01-01T18:34:17",
"upload_time_iso_8601": "2025-01-01T18:34:17.080771Z",
"url": "https://files.pythonhosted.org/packages/35/5b/428d04edf9a9a21f00c9a037c9998d51bbba88c2332ace0b7d1af67200af/psd_export-1.0.33-cp312-cp312-musllinux_1_2_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "cb0430c07cfca62976206e73fe1c9c0c7a667805b447454a711364ed35cde672",
"md5": "6663816516713a3e62376e8882ecf1bd",
"sha256": "553c27ee023b7df4470472bcce4da44c18abedaa0ad7588716233683386d50d7"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp312-cp312-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "6663816516713a3e62376e8882ecf1bd",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.11.2",
"size": 1116300,
"upload_time": "2025-01-01T18:34:19",
"upload_time_iso_8601": "2025-01-01T18:34:19.601654Z",
"url": "https://files.pythonhosted.org/packages/cb/04/30c07cfca62976206e73fe1c9c0c7a667805b447454a711364ed35cde672/psd_export-1.0.33-cp312-cp312-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6db1ea896b313c7e057772538b4c8451b3b985d8f41b38a104ef0b651066aa5a",
"md5": "84f22b845ca5f0381858c84946989633",
"sha256": "b5057cc61f9fa752b56f21d453e6a2148fdf8e4a14d7f71bbe65c42377c2517d"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp312-cp312-win32.whl",
"has_sig": false,
"md5_digest": "84f22b845ca5f0381858c84946989633",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.11.2",
"size": 362274,
"upload_time": "2025-01-01T18:34:24",
"upload_time_iso_8601": "2025-01-01T18:34:24.086124Z",
"url": "https://files.pythonhosted.org/packages/6d/b1/ea896b313c7e057772538b4c8451b3b985d8f41b38a104ef0b651066aa5a/psd_export-1.0.33-cp312-cp312-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "c4b67704e4ebf49794fb13572fb93c64d6c0d380f20a76d9254217f7b7b9fc93",
"md5": "4f41dda68974e1bb39b3a23f066f5499",
"sha256": "880c288fcd1957a2d52181856dff62cc3feddd9d445a25b91a5cd8b9834b98c4"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp312-cp312-win_amd64.whl",
"has_sig": false,
"md5_digest": "4f41dda68974e1bb39b3a23f066f5499",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.11.2",
"size": 380551,
"upload_time": "2025-01-01T18:34:26",
"upload_time_iso_8601": "2025-01-01T18:34:26.353283Z",
"url": "https://files.pythonhosted.org/packages/c4/b6/7704e4ebf49794fb13572fb93c64d6c0d380f20a76d9254217f7b7b9fc93/psd_export-1.0.33-cp312-cp312-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "85c700137b97cbd7bf0ed0307e8fa07e5b5269818cdfc8ebefe0636cdd09853f",
"md5": "b14bc65a0e1a85ccb9d033e06db4d4e3",
"sha256": "d2f7d8cbbf0c5b3745e5d0b15f37be35c0cdabc0307cb717030aecdfc448b649"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp313-cp313-macosx_10_13_x86_64.whl",
"has_sig": false,
"md5_digest": "b14bc65a0e1a85ccb9d033e06db4d4e3",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": ">=3.11.2",
"size": 391092,
"upload_time": "2025-01-01T18:34:28",
"upload_time_iso_8601": "2025-01-01T18:34:28.017508Z",
"url": "https://files.pythonhosted.org/packages/85/c7/00137b97cbd7bf0ed0307e8fa07e5b5269818cdfc8ebefe0636cdd09853f/psd_export-1.0.33-cp313-cp313-macosx_10_13_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "f7082aa82bb5f19b08882eefdfac7dcbf67fb7151025da1d3e814b9ee3889739",
"md5": "bf9d04a88db0d9e4c6b4923f24bb9069",
"sha256": "9f1c24942a368c6c2dfc80539d85c28b4c6cf6dd0f7e011b54b19eb9a167a522"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp313-cp313-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "bf9d04a88db0d9e4c6b4923f24bb9069",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": ">=3.11.2",
"size": 380834,
"upload_time": "2025-01-01T18:34:30",
"upload_time_iso_8601": "2025-01-01T18:34:30.325368Z",
"url": "https://files.pythonhosted.org/packages/f7/08/2aa82bb5f19b08882eefdfac7dcbf67fb7151025da1d3e814b9ee3889739/psd_export-1.0.33-cp313-cp313-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4926ba92ae2f415d2c724c6e8aec424830b29b06faee0e2e552ab13a719db9b0",
"md5": "219b64aa6686d5dadb8221646a02588e",
"sha256": "f6be37aa0a78356a56a285257824853c9a77b65d45f25f8d7e86b67c9d1ba508"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp313-cp313-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "219b64aa6686d5dadb8221646a02588e",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": ">=3.11.2",
"size": 1062072,
"upload_time": "2025-01-01T18:34:31",
"upload_time_iso_8601": "2025-01-01T18:34:31.660893Z",
"url": "https://files.pythonhosted.org/packages/49/26/ba92ae2f415d2c724c6e8aec424830b29b06faee0e2e552ab13a719db9b0/psd_export-1.0.33-cp313-cp313-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1f406d37e359aef8f89961bacb8ec35a508cd566aaa3f697cb82896933ea15a1",
"md5": "d37ad08f6806e17bc817e10e38ed43c6",
"sha256": "c35e55459dad29e715eaba12cf4285d3d2b408cc8744872b5e0fc0eaf5db048b"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp313-cp313-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "d37ad08f6806e17bc817e10e38ed43c6",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": ">=3.11.2",
"size": 1080547,
"upload_time": "2025-01-01T18:34:33",
"upload_time_iso_8601": "2025-01-01T18:34:33.958303Z",
"url": "https://files.pythonhosted.org/packages/1f/40/6d37e359aef8f89961bacb8ec35a508cd566aaa3f697cb82896933ea15a1/psd_export-1.0.33-cp313-cp313-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6c4ac6527f9d398bdcb07e2de8ce1b1178331e11033e62a9e2f0b1893e7a1e95",
"md5": "2a6ad52658c0920e87221c3ae3187e31",
"sha256": "e13800e189e312f22075e8f49d2054fd08df31d555e117e39ad0734791142b66"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp313-cp313-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl",
"has_sig": false,
"md5_digest": "2a6ad52658c0920e87221c3ae3187e31",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": ">=3.11.2",
"size": 1039293,
"upload_time": "2025-01-01T18:34:35",
"upload_time_iso_8601": "2025-01-01T18:34:35.239072Z",
"url": "https://files.pythonhosted.org/packages/6c/4a/c6527f9d398bdcb07e2de8ce1b1178331e11033e62a9e2f0b1893e7a1e95/psd_export-1.0.33-cp313-cp313-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6d80088e83853d9c3313b4d01e512d18b5603db83e7e60b9300c5aed7365342d",
"md5": "cb524db44767276a94354b16fc068c99",
"sha256": "c035487907524de3879d0c9ea68d1b093b1de3c6892a2a0ccfa6ca59aeca0be1"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp313-cp313-musllinux_1_2_aarch64.whl",
"has_sig": false,
"md5_digest": "cb524db44767276a94354b16fc068c99",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": ">=3.11.2",
"size": 1070504,
"upload_time": "2025-01-01T18:34:36",
"upload_time_iso_8601": "2025-01-01T18:34:36.565269Z",
"url": "https://files.pythonhosted.org/packages/6d/80/088e83853d9c3313b4d01e512d18b5603db83e7e60b9300c5aed7365342d/psd_export-1.0.33-cp313-cp313-musllinux_1_2_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7e075453b3f457d00764064c8e0efe6457e60b61505c9a444e9873cc59b20179",
"md5": "2ae821cef1180b6104fc84b7009785a5",
"sha256": "73f1462514b58549a37fc3a11675eedbc1dbf0b64d2e74a64d0104dcc19b1571"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp313-cp313-musllinux_1_2_i686.whl",
"has_sig": false,
"md5_digest": "2ae821cef1180b6104fc84b7009785a5",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": ">=3.11.2",
"size": 1079924,
"upload_time": "2025-01-01T18:34:37",
"upload_time_iso_8601": "2025-01-01T18:34:37.962788Z",
"url": "https://files.pythonhosted.org/packages/7e/07/5453b3f457d00764064c8e0efe6457e60b61505c9a444e9873cc59b20179/psd_export-1.0.33-cp313-cp313-musllinux_1_2_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8a950a1000fdf39c87a7799677309983d78be87be9541e994135ee19770c87b2",
"md5": "b8e20e58b2b09f03bd37897ba12c8103",
"sha256": "de15d8ec49dc29bd46f952775a06b1da8d50840dcf4fbf5c330d348cb4507282"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp313-cp313-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "b8e20e58b2b09f03bd37897ba12c8103",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": ">=3.11.2",
"size": 1109451,
"upload_time": "2025-01-01T18:34:39",
"upload_time_iso_8601": "2025-01-01T18:34:39.453600Z",
"url": "https://files.pythonhosted.org/packages/8a/95/0a1000fdf39c87a7799677309983d78be87be9541e994135ee19770c87b2/psd_export-1.0.33-cp313-cp313-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5c816f36a77962032f3556a2b395b7b3083c72b97ca5aeec88187efbf546e707",
"md5": "dbd35b521d8855ce938e165baa2ef60a",
"sha256": "c45dad9ebe279436d580dd6ec1f2bdfb33547bd471e4e33417b8e1404a22b837"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp313-cp313-win32.whl",
"has_sig": false,
"md5_digest": "dbd35b521d8855ce938e165baa2ef60a",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": ">=3.11.2",
"size": 361508,
"upload_time": "2025-01-01T18:34:40",
"upload_time_iso_8601": "2025-01-01T18:34:40.752073Z",
"url": "https://files.pythonhosted.org/packages/5c/81/6f36a77962032f3556a2b395b7b3083c72b97ca5aeec88187efbf546e707/psd_export-1.0.33-cp313-cp313-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d19d8ddb804d18c8ff01f9db6735d90f715e843fbd5a1904fe792aa52e751d24",
"md5": "fea853a054120a2891f5f091208e5fb9",
"sha256": "8dc31cc6dd800d29696e19308593c285aa9f2baafe213646bde789c57354be9c"
},
"downloads": -1,
"filename": "psd_export-1.0.33-cp313-cp313-win_amd64.whl",
"has_sig": false,
"md5_digest": "fea853a054120a2891f5f091208e5fb9",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": ">=3.11.2",
"size": 379462,
"upload_time": "2025-01-01T18:34:42",
"upload_time_iso_8601": "2025-01-01T18:34:42.185320Z",
"url": "https://files.pythonhosted.org/packages/d1/9d/8ddb804d18c8ff01f9db6735d90f715e843fbd5a1904fe792aa52e751d24/psd_export-1.0.33-cp313-cp313-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d217f73aecc883c9759404a3d7b870061c28c0573028a599c2412675f30c9b6d",
"md5": "c66ac5577723af5cdf275ad8aaf25e50",
"sha256": "c359a62faa838ecf7fda8403600d8fe8526a3b58a779e5a7a3278a58d51a6ea2"
},
"downloads": -1,
"filename": "psd_export-1.0.33.tar.gz",
"has_sig": false,
"md5_digest": "c66ac5577723af5cdf275ad8aaf25e50",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.11.2",
"size": 258221,
"upload_time": "2025-01-01T18:34:44",
"upload_time_iso_8601": "2025-01-01T18:34:44.681523Z",
"url": "https://files.pythonhosted.org/packages/d2/17/f73aecc883c9759404a3d7b870061c28c0573028a599c2412675f30c9b6d/psd_export-1.0.33.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-01-01 18:34:44",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "cromachina",
"github_project": "psd-export",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "psd-export"
}