[](https://travis-ci.com/ig248/pyampd)
[](https://codecov.io/gh/ig248/pyampdgit)
# AMPD algorithm in Python
Implements a function `find_peaks` based on the Automatic Multi-scale
Peak Detection algorithm proposed by Felix Scholkmann et al. in
"An Efficient Algorithm for Automatic Peak Detection in
Noisy Periodic and Quasi-Periodic Signals", Algorithms 2012,
5, 588-603
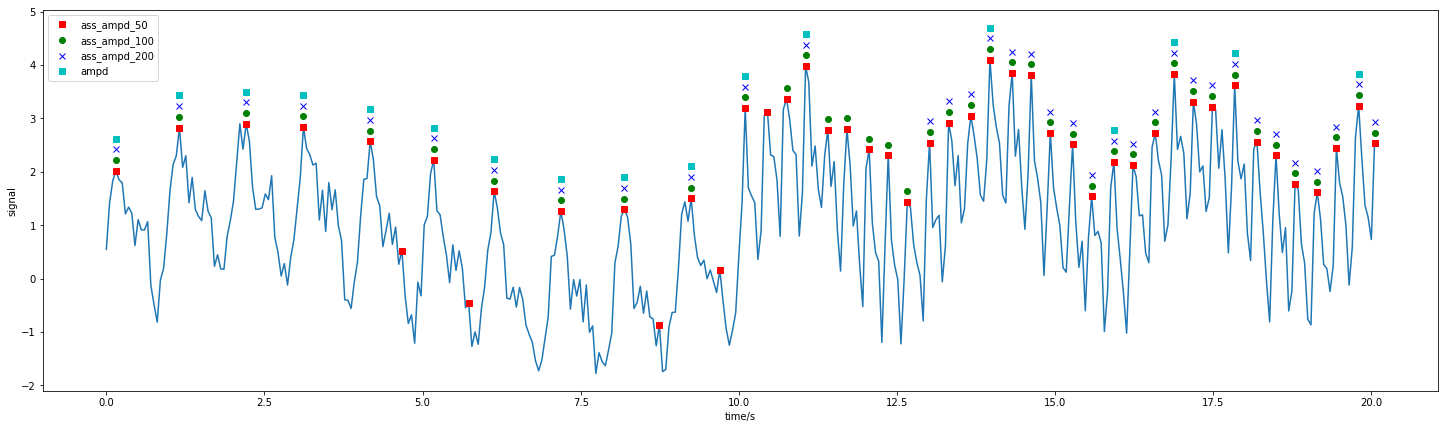
## Usage
Install from PyPI:
```
pip install pyampd
```
Or install from source:
```
pip install git+https://github.com/ig248/pyampd
```
Import function:
```python
from pyampd.ampd import find_peaks
```
See `notebooks/ampd.ipynb` for usage examples.
### Specifying maximum scale
To improve run-time on large time-series, it is possible to specify the maximum scale to consider:
```python
peaks = find_peaks(x, scale=100)
```
will only consider windows up to +-100 point either side of peak candidates.
### Adaptive Scale Selection
If the characteristic scale of the signal changes over time, a new algorithm called
Adaptive Scale Selection can track the changes in optimal scales and detect peaks accordingly:
```python
peaks = find_peaks_adaptive(x, window=200)
```
will select the optimal scale at each point using a 200-point running window.
### Original implementation
`find_peaks` is not identical to the algorithm proposed in the original paper (especially near start and end of time series).
A performance-optimized version of the original implementation is provided in `find_peaks_original`.
## Tests
Run
```bash
pytest
```
## Other implementations
- R: https://cran.r-project.org/web/packages/ampd/index.html
- MATLAB: https://github.com/mathouse/AMPD-algorithm
- Python: https://github.com/LucaCerina/ampdLib
## Improvements
This Python implementation provides significant speed-ups in two areas:
1. Efficient tracking of local minima without using random numbers
2. Introduction of maximum window size, reducing algorithm run-time from
quadratic to linear in the number of samples.
3. Better handling of peaks near start/end of the series
4. Addition of new Adaptive Scale Selection
## ToDo
- It may be possible to avoid repeated comparisons, and reduce worst-case
runtime from `O(n^2)` to `O(n log(n))`.
- `find_peaks_adaptive` could benefit from specifying both `window` and `max_scale`
## References
Original paper: https://doi.org/10.1109/ICRERA.2016.7884365
Raw data
{
"_id": null,
"home_page": "https://github.com/ig248/pyampd",
"name": "pyampd",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "",
"author": "Igor Gotlibovych",
"author_email": "igor.gotlibovych@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/51/97/6a56d7ed7d1ed5cb25c4643cb327a1903438587991fe364ac6042c2b837d/pyampd-0.0.1.tar.gz",
"platform": "",
"description": "[](https://travis-ci.com/ig248/pyampd)\n[](https://codecov.io/gh/ig248/pyampdgit)\n\n# AMPD algorithm in Python\nImplements a function `find_peaks` based on the Automatic Multi-scale\nPeak Detection algorithm proposed by Felix Scholkmann et al. in\n\"An Efficient Algorithm for Automatic Peak Detection in\nNoisy Periodic and Quasi-Periodic Signals\", Algorithms 2012,\n 5, 588-603\n\n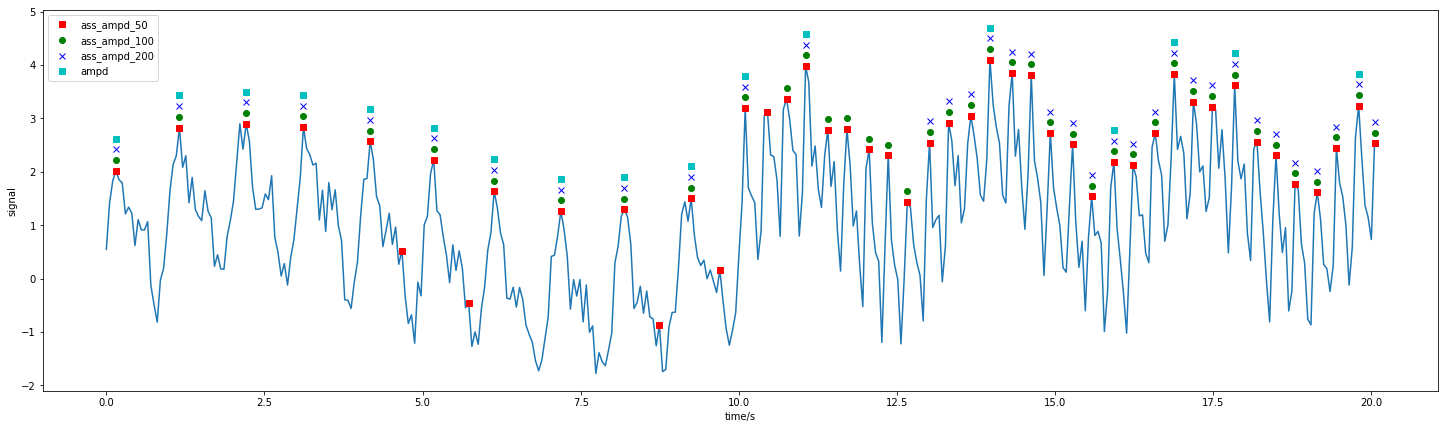\n\n## Usage\nInstall from PyPI:\n\n```\npip install pyampd\n```\n\nOr install from source:\n\n```\npip install git+https://github.com/ig248/pyampd\n```\n\nImport function:\n\n```python\nfrom pyampd.ampd import find_peaks\n```\n\nSee `notebooks/ampd.ipynb` for usage examples.\n\n### Specifying maximum scale\nTo improve run-time on large time-series, it is possible to specify the maximum scale to consider:\n```python\npeaks = find_peaks(x, scale=100)\n```\nwill only consider windows up to +-100 point either side of peak candidates.\n\n### Adaptive Scale Selection\nIf the characteristic scale of the signal changes over time, a new algorithm called\nAdaptive Scale Selection can track the changes in optimal scales and detect peaks accordingly:\n```python\npeaks = find_peaks_adaptive(x, window=200)\n```\nwill select the optimal scale at each point using a 200-point running window.\n\n\n### Original implementation\n`find_peaks` is not identical to the algorithm proposed in the original paper (especially near start and end of time series).\n A performance-optimized version of the original implementation is provided in `find_peaks_original`.\n\n\n## Tests\nRun\n```bash\npytest\n```\n\n## Other implementations\n- R: https://cran.r-project.org/web/packages/ampd/index.html\n- MATLAB: https://github.com/mathouse/AMPD-algorithm\n- Python: https://github.com/LucaCerina/ampdLib\n\n## Improvements\nThis Python implementation provides significant speed-ups in two areas:\n1. Efficient tracking of local minima without using random numbers\n2. Introduction of maximum window size, reducing algorithm run-time from\nquadratic to linear in the number of samples.\n3. Better handling of peaks near start/end of the series\n4. Addition of new Adaptive Scale Selection \n\n## ToDo\n- It may be possible to avoid repeated comparisons, and reduce worst-case\nruntime from `O(n^2)` to `O(n log(n))`.\n- `find_peaks_adaptive` could benefit from specifying both `window` and `max_scale`\n\n## References\nOriginal paper: https://doi.org/10.1109/ICRERA.2016.7884365\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Peak detection using AMPD and ASS-AMPD algorithms",
"version": "0.0.1",
"project_urls": {
"Homepage": "https://github.com/ig248/pyampd"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "53b5587d52d43aa803c20743fd23e2bcbb454a06694c5645de741311678c8750",
"md5": "89da3b6ba286573eec3062f713b83c6b",
"sha256": "d923a4372308d447b98cd80f935a059e29323777dd26c0ac131945ad84f5f89c"
},
"downloads": -1,
"filename": "pyampd-0.0.1-py2.py3-none-any.whl",
"has_sig": false,
"md5_digest": "89da3b6ba286573eec3062f713b83c6b",
"packagetype": "bdist_wheel",
"python_version": "py2.py3",
"requires_python": null,
"size": 5106,
"upload_time": "2018-12-25T20:25:49",
"upload_time_iso_8601": "2018-12-25T20:25:49.761201Z",
"url": "https://files.pythonhosted.org/packages/53/b5/587d52d43aa803c20743fd23e2bcbb454a06694c5645de741311678c8750/pyampd-0.0.1-py2.py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "51976a56d7ed7d1ed5cb25c4643cb327a1903438587991fe364ac6042c2b837d",
"md5": "48da763e6ab7df22347212859589d051",
"sha256": "e333d00b61d2844b0ed0383b010394e0a0cb384a036dd83960cdc647cf71a755"
},
"downloads": -1,
"filename": "pyampd-0.0.1.tar.gz",
"has_sig": false,
"md5_digest": "48da763e6ab7df22347212859589d051",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 4612,
"upload_time": "2018-12-25T20:25:52",
"upload_time_iso_8601": "2018-12-25T20:25:52.050467Z",
"url": "https://files.pythonhosted.org/packages/51/97/6a56d7ed7d1ed5cb25c4643cb327a1903438587991fe364ac6042c2b837d/pyampd-0.0.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2018-12-25 20:25:52",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "ig248",
"github_project": "pyampd",
"travis_ci": true,
"coveralls": false,
"github_actions": false,
"lcname": "pyampd"
}