# Code Analyzer

[](https://badge.fury.io/py/python-code-analyzer)
[](https://github.com/josephedradan/code_analyzer/actions/workflows/test.yml)


### What is this?
A python code analyzer that allows you to visually see how your code works after executing code.
Alternatively, you can think of it as a python debugger that prints all the lines executed.
### Why would you use this?
Let's say you don't want to use a python debugger and you want to see how your code runs line by line printed out. You can import this module and add a few lines of code to initialize the analyzer and run your code, and
you will get a simple analysis of the code executed in your terminal or exported to a txt or html file.
It is not advised to use this analyzer in a big project as the output won't fit in your terminal; though, using an export
method call to see the code in a file might be more useful/helpful.
### Requirements:
python>=3.7
rich
colorama
pandas
### Example:
from code_analyzer import CodeAnalyzer
code_analyzer = CodeAnalyzer() # Initialize analyzer
code_analyzer.start()
# Comment that will be displayed on the next line
code_analyzer.record_comment_for_interpretable_next("Function definition here!")
def recursive(depth: int) -> int:
# Comment that will be displayed on the previous line
code_analyzer.record_comment_for_interpretable_previous({"__depth": depth})
if depth <= 0:
code_analyzer.record_comment_for_interpretable_next({"Final depth": depth})
return depth
return recursive(depth - 1)
code_analyzer.record_comment_for_interpretable_next("This is where the fun begins")
recursive(5)
code_analyzer.stop()
code_analyzer.print()
# code_analyzer.get_code_analyzer_printer().print_debug()
code_analyzer.get_code_analyzer_printer().export_to_txt()
# code_analyzer.get_code_analyzer_printer().print_rich() # export_rich_to_html prints to console by default
code_analyzer.get_code_analyzer_printer().export_rich_to_html()
Or just look at the other examples in examples folder.
### Output
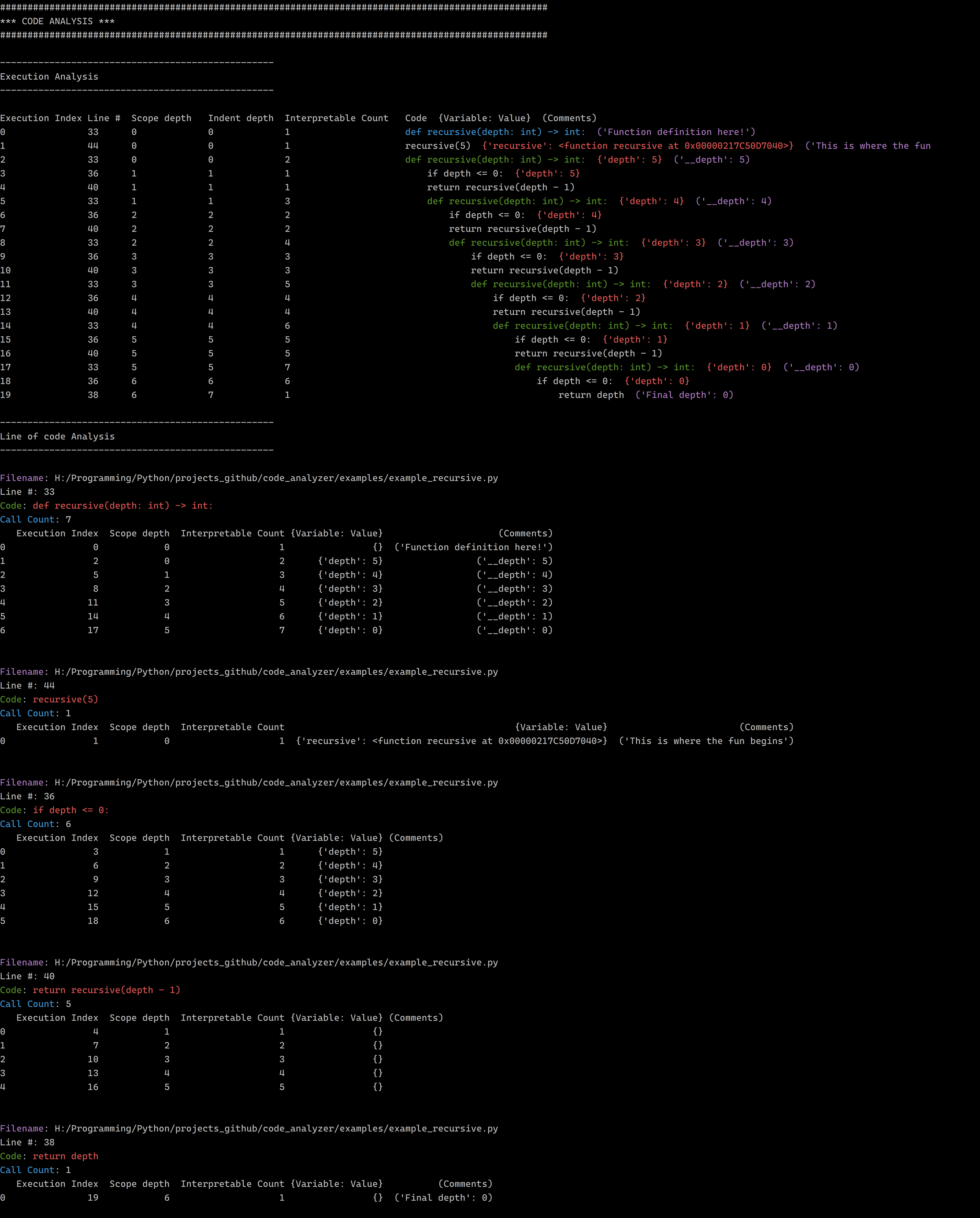
## [Rich output html](https://htmlpreview.github.io/?https://github.com/josephedradan/code_analyzer/blob/main/examples/example_recursive_code_analysis_rich.html)
### Notes
In the output of a print (Such as in the image above):
* Blue foreground code is a callable's definition.
* Green foreground code is a callable being executed.
* Red foreground text are {Variable: value} pairs found in between the `{}` brackets that are new to the current interpretable relative to its scope.
* Purple foreground text are (arguments) found in between the `()` brackets passed to the method calls below:
* .record_comment_for_interpretable_next(...)
* .record_comment_for_interpretable_previous(...)
In the output of a rich export (such as the .html files in the examples folder):
* Blue background code is a callable's definition.
* Green background code is a callable being executed.
* Orange foreground text are {Variable: value} pairs found in between the {} brackets that are new to the current interpretable relative to its scope.
* Red foreground text are (arguments) found in between the () brackets passed to the method calls below:
* .record_comment_for_interpretable_next(...)
* .record_comment_for_interpretable_previous(...)
__IF YOU SEE CODE THAT DOESN'T SEEM TO BE CODE THAT YOU SHOULD BE ANALYZING WHEN USING TYPE HINTING, ADD THE
IMPORT BELOW TO THE TOP OF THE FILE TO POSSIBLY REMOVE IT__
from __future__ import annotations
### Installation
pip install python-code-analyzer
### [pypi](https://pypi.org/project/python-code-analyzer/)
__TODO:__
* Fancy visualizer
* Memory usage?
* Timing code?
* profiler (Time and calls amount)
Raw data
{
"_id": null,
"home_page": "https://github.com/josephedradan/code_analyzer",
"name": "python-code-analyzer",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "",
"keywords": "python,analyzer,debugger,python code analyzer,python analyzer",
"author": "Joseph Edradan",
"author_email": "edradanjoseph245@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/0c/fc/f49befa1e84fec22f581657488d1f36399cc3abb37210385e0f2e391d5dd/python_code_analyzer-1.0.13.tar.gz",
"platform": null,
"description": "\n# Code Analyzer\n\n\n[](https://badge.fury.io/py/python-code-analyzer)\n[](https://github.com/josephedradan/code_analyzer/actions/workflows/test.yml)\n\n\n\n### What is this?\nA python code analyzer that allows you to visually see how your code works after executing code.\nAlternatively, you can think of it as a python debugger that prints all the lines executed.\n\n### Why would you use this?\nLet's say you don't want to use a python debugger and you want to see how your code runs line by line printed out. You can import this module and add a few lines of code to initialize the analyzer and run your code, and\nyou will get a simple analysis of the code executed in your terminal or exported to a txt or html file. \n\nIt is not advised to use this analyzer in a big project as the output won't fit in your terminal; though, using an export\nmethod call to see the code in a file might be more useful/helpful.\n\n### Requirements:\n python>=3.7\n rich\n colorama\n pandas\n\n### Example:\n\n from code_analyzer import CodeAnalyzer\n \n code_analyzer = CodeAnalyzer() # Initialize analyzer\n code_analyzer.start()\n \n # Comment that will be displayed on the next line\n code_analyzer.record_comment_for_interpretable_next(\"Function definition here!\")\n \n \n def recursive(depth: int) -> int:\n # Comment that will be displayed on the previous line\n code_analyzer.record_comment_for_interpretable_previous({\"__depth\": depth})\n if depth <= 0:\n code_analyzer.record_comment_for_interpretable_next({\"Final depth\": depth})\n return depth\n \n return recursive(depth - 1)\n \n \n code_analyzer.record_comment_for_interpretable_next(\"This is where the fun begins\")\n recursive(5)\n \n code_analyzer.stop()\n code_analyzer.print()\n \n # code_analyzer.get_code_analyzer_printer().print_debug()\n code_analyzer.get_code_analyzer_printer().export_to_txt()\n \n # code_analyzer.get_code_analyzer_printer().print_rich() # export_rich_to_html prints to console by default\n code_analyzer.get_code_analyzer_printer().export_rich_to_html()\n \n Or just look at the other examples in examples folder.\n\n\n### Output\n\n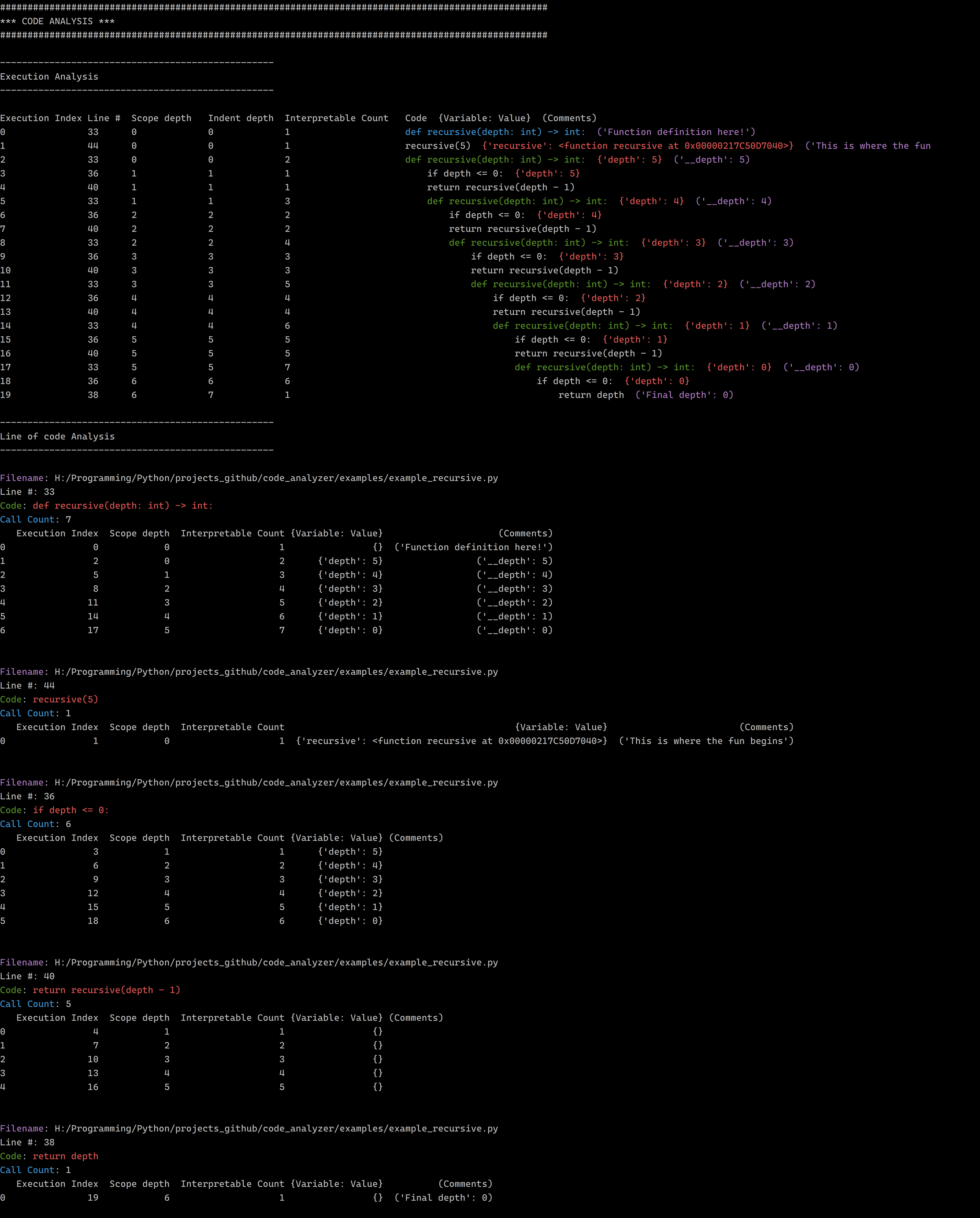\n\n## [Rich output html](https://htmlpreview.github.io/?https://github.com/josephedradan/code_analyzer/blob/main/examples/example_recursive_code_analysis_rich.html)\n### Notes\n\nIn the output of a print (Such as in the image above):\n\n* Blue foreground code is a callable's definition.\n* Green foreground code is a callable being executed.\n* Red foreground text are {Variable: value} pairs found in between the `{}` brackets that are new to the current interpretable relative to its scope. \n* Purple foreground text are (arguments) found in between the `()` brackets passed to the method calls below:\n * .record_comment_for_interpretable_next(...) \n * .record_comment_for_interpretable_previous(...)\n \nIn the output of a rich export (such as the .html files in the examples folder):\n\n* Blue background code is a callable's definition.\n* Green background code is a callable being executed.\n* Orange foreground text are {Variable: value} pairs found in between the {} brackets that are new to the current interpretable relative to its scope. \n* Red foreground text are (arguments) found in between the () brackets passed to the method calls below:\n * .record_comment_for_interpretable_next(...) \n * .record_comment_for_interpretable_previous(...)\n\n__IF YOU SEE CODE THAT DOESN'T SEEM TO BE CODE THAT YOU SHOULD BE ANALYZING WHEN USING TYPE HINTING, ADD THE \nIMPORT BELOW TO THE TOP OF THE FILE TO POSSIBLY REMOVE IT__\n\n from __future__ import annotations\n\n### Installation\n pip install python-code-analyzer\n\n### [pypi](https://pypi.org/project/python-code-analyzer/)\n\n__TODO:__\n* Fancy visualizer\n* Memory usage?\n* Timing code?\n* profiler (Time and calls amount)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A python code analyzer that high jacks the current settrace function to analyze executed code.",
"version": "1.0.13",
"split_keywords": [
"python",
"analyzer",
"debugger",
"python code analyzer",
"python analyzer"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "27eda6d0bddbe09996acd855ad27cb432c514ba28004004b20eda48f7727de53",
"md5": "cc69dd376d92e47bcd582f2484a98445",
"sha256": "0295d4533f241e9c767c26a453e79d0f09cb5df0837ac7ae5adc30a944672cfb"
},
"downloads": -1,
"filename": "python_code_analyzer-1.0.13-py3-none-any.whl",
"has_sig": false,
"md5_digest": "cc69dd376d92e47bcd582f2484a98445",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 36526,
"upload_time": "2023-01-09T06:56:22",
"upload_time_iso_8601": "2023-01-09T06:56:22.490707Z",
"url": "https://files.pythonhosted.org/packages/27/ed/a6d0bddbe09996acd855ad27cb432c514ba28004004b20eda48f7727de53/python_code_analyzer-1.0.13-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0cfcf49befa1e84fec22f581657488d1f36399cc3abb37210385e0f2e391d5dd",
"md5": "57faa0b526fc3989b756e18b00a5790f",
"sha256": "e8b7f53a30b11dfd78fc87084ef6cb7b7771da82be17c0b46e2af32a7b04e0dc"
},
"downloads": -1,
"filename": "python_code_analyzer-1.0.13.tar.gz",
"has_sig": false,
"md5_digest": "57faa0b526fc3989b756e18b00a5790f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 34259,
"upload_time": "2023-01-09T06:56:24",
"upload_time_iso_8601": "2023-01-09T06:56:24.036488Z",
"url": "https://files.pythonhosted.org/packages/0c/fc/f49befa1e84fec22f581657488d1f36399cc3abb37210385e0f2e391d5dd/python_code_analyzer-1.0.13.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-01-09 06:56:24",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "josephedradan",
"github_project": "code_analyzer",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"tox": true,
"lcname": "python-code-analyzer"
}