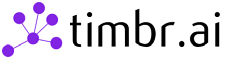
# timbr Python connector sample file
This is a sample repository for how to connect to timbr using SQLAlchemy and Python.
## Dependencies
- Access to a timbr-server
- Python from 3.7.13 or newer
- Support SQLAlchemy 1.4.36 or newer but not version 2.x yet.
## Installation
- Install as clone repository:
- Install Python: https://www.python.org/downloads/release/python-3713/
- Run the following command to install the Python dependencies: `pip install -r requirements.txt`
- Install using pip and git:
- `pip install git+https://github.com/WPSemantix/timbr_python_SQLAlchemy`
- Install using pip:
- `pip install pytimbr-sqla`
## Known issues
If you encounter a problem installing `PyHive` with sasl dependencies on windows, install the following wheel (for 64bit Windows) by running:
`pip install https://download.lfd.uci.edu/pythonlibs/archived/cp37/sasl-0.3.1-cp37-cp37m-win_amd64.whl`
For Python 3.9:
`pip install https://download.lfd.uci.edu/pythonlibs/archived/sasl-0.3.1-cp39-cp39-win_amd64.whl`
## Sample usage
- For an example of how to use the Python connector for timbr, follow this [example file](example.py)
## Connect options
### Connect using 'pytimbr_sqla' and 'SQLAlchemy' packages
```python
from sqlalchemy import create_engine
# Connection protocol can be 'http' or 'https'
protocol = 'http'
# Use 'token' as the username when connecting using a Timbr token, otherwise use the user name
user_name = 'token'
# If using a token as a username then the pass is the token value, otherwise its the user's password.
user_pass = '<token_value_or_user_password>'
# The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).
hostname = '<timbr_server_host>'
# The port to connect to in the Timbr server. Timbr's default port is 11000
port = '<timbr_server_port>'
# The name of the ontology (knowledge graph) to connect
ontology = '<ontology_name>'
# Create new sqlalchemy connection
engine = create_engine(f"timbr+{protocol}://{user_name}@{ontology}:{user_pass}@{hostname}:{port}")
conn = engine.connect()
# Use the connection to execute a query
query = "SHOW CONCEPTS"
concepts = conn.execute(query).fetchall()
for concept in concepts:
print(concept)
```
### Attention:
### timbr works only as async when running a query, if you want to use standard PyHive you have two options
### Connect using 'PyHive' and 'SQLAlchemy' packages
#### Connect using PyHive Async Query
```python
from sqlalchemy import create_engine
from TCLIService.ttypes import TOperationState
# Connection protocol can be 'http' or 'https'
protocol = 'http'
# Use 'token' as the username when connecting using a Timbr token, otherwise use the user name
user_name = 'token'
# If using a token as a username then the pass is the token value, otherwise its the user's password.
user_pass = '<token_value_or_user_password>'
# The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).
hostname = '<timbr_server_host>'
# The port to connect to in the Timbr server. Timbr's default port is 11000
port = '<timbr_server_port>'
# The name of the ontology (knowledge graph) to connect
ontology = '<ontology_name>'
# Create new sqlalchemy connection
engine = create_engine(f"hive+{protocol}://{user_name}@{ontology}:{user_pass}@{hostname}:{port}", connect_args={'configuration': {'set:hiveconf:hiveMetadata': 'true'}})
conn = engine.connect()
dbapi_conn = engine.raw_connection()
cursor = dbapi_conn.cursor()
# Use the connection to execute a query
query = "SHOW CONCEPTS"
cursor.execute(query)
# Check the status of this execution
status = cursor.poll().operationState
while status in (TOperationState.INITIALIZED_STATE, TOperationState.RUNNING_STATE):
status = cursor.poll().operationState
results = cursor.fetchall()
print(results)
```
#### Connect using PyHive Sync Query
```python
from sqlalchemy import create_engine
from TCLIService.ttypes import TOperationState
# Connection protocol can be 'http' or 'https'
protocol = 'http'
# Use 'token' as the username when connecting using a Timbr token, otherwise use the user name
user_name = 'token'
# If using a token as a username then the pass is the token value, otherwise its the user's password.
user_pass = '<token_value_or_user_password>'
# The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).
hostname = '<timbr_server_host>'
# The port to connect to in the Timbr server. Timbr's default port is 11000
port = '<timbr_server_port>'
# The name of the ontology (knowledge graph) to connect
ontology = '<ontology_name>'
# Create new sqlalchemy connection
engine = create_engine(f"hive+{protocol}://{user_name}@{ontology}:{user_pass}@{hostname}:{port}", connect_args={'configuration': {'set:hiveconf:async': 'false', 'set:hiveconf:hiveMetadata': 'true'}})
conn = engine.connect()
# Use the connection to execute a query
query = "SHOW CONCEPTS"
results = conn.execute(query).fetchall()
print(results)
```
Raw data
{
"_id": null,
"home_page": "https://github.com/WPSemantix/timbr_python_SQLAlchemy",
"name": "pytimbr-sqla",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "timbr,timbr-python,timbr-connector,python-connector,PyTimbr,pytimbr,py-timbr,Py-Timbr,pytimbr_sqla,pytimbr_Sqla,PyTimbr_Sqla,pytimbr_SQla,PyTimbr_SQla,pytimbr_SQLa,PyTimbr_SQLa,pytimbr_SQlA,PyTimbr_SQLA,pytimbrsqlalchemy,PyTimbrSqlalchemy,PyTimbrSQlalchemy,PyTimbrSQLalchemy,PyTimbrSQLAlchemy,Py-TimbrSQLAlchemy,py-timbrsqlalchemy,Py-Timbr-SQLAlchemy,py-timbr-sqlalchemy",
"author": "timbr",
"author_email": "contact@timbr.ai",
"download_url": "https://files.pythonhosted.org/packages/76/7e/736fbd3b6ba9d65bd4780da4cf47b000d13e2975e0fc54fefb9c1c11d549/pytimbr_sqla-1.0.3.tar.gz",
"platform": null,
"description": "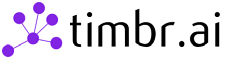\r\n\r\n# timbr Python connector sample file\r\nThis is a sample repository for how to connect to timbr using SQLAlchemy and Python.\r\n\r\n## Dependencies\r\n- Access to a timbr-server\r\n- Python from 3.7.13 or newer\r\n- Support SQLAlchemy 1.4.36 or newer but not version 2.x yet.\r\n\r\n## Installation\r\n- Install as clone repository:\r\n - Install Python: https://www.python.org/downloads/release/python-3713/\r\n - Run the following command to install the Python dependencies: `pip install -r requirements.txt`\r\n\r\n- Install using pip and git:\r\n - `pip install git+https://github.com/WPSemantix/timbr_python_SQLAlchemy`\r\n\r\n- Install using pip:\r\n - `pip install pytimbr-sqla`\r\n\r\n## Known issues\r\nIf you encounter a problem installing `PyHive` with sasl dependencies on windows, install the following wheel (for 64bit Windows) by running:\r\n\r\n`pip install https://download.lfd.uci.edu/pythonlibs/archived/cp37/sasl-0.3.1-cp37-cp37m-win_amd64.whl`\r\n\r\nFor Python 3.9:\r\n\r\n`pip install https://download.lfd.uci.edu/pythonlibs/archived/sasl-0.3.1-cp39-cp39-win_amd64.whl`\r\n\r\n## Sample usage\r\n- For an example of how to use the Python connector for timbr, follow this [example file](example.py)\r\n\r\n## Connect options\r\n\r\n### Connect using 'pytimbr_sqla' and 'SQLAlchemy' packages\r\n```python\r\n from sqlalchemy import create_engine\r\n\r\n # Connection protocol can be 'http' or 'https'\r\n protocol = 'http'\r\n # Use 'token' as the username when connecting using a Timbr token, otherwise use the user name\r\n user_name = 'token'\r\n # If using a token as a username then the pass is the token value, otherwise its the user's password.\r\n user_pass = '<token_value_or_user_password>'\r\n # The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).\r\n hostname = '<timbr_server_host>'\r\n # The port to connect to in the Timbr server. Timbr's default port is 11000\r\n port = '<timbr_server_port>'\r\n # The name of the ontology (knowledge graph) to connect\r\n ontology = '<ontology_name>'\r\n \r\n # Create new sqlalchemy connection\r\n engine = create_engine(f\"timbr+{protocol}://{user_name}@{ontology}:{user_pass}@{hostname}:{port}\")\r\n conn = engine.connect()\r\n\r\n # Use the connection to execute a query\r\n query = \"SHOW CONCEPTS\"\r\n concepts = conn.execute(query).fetchall()\r\n for concept in concepts:\r\n print(concept)\r\n```\r\n### Attention:\r\n### timbr works only as async when running a query, if you want to use standard PyHive you have two options\r\n\r\n### Connect using 'PyHive' and 'SQLAlchemy' packages\r\n\r\n#### Connect using PyHive Async Query\r\n```python\r\n from sqlalchemy import create_engine\r\n from TCLIService.ttypes import TOperationState\r\n\r\n # Connection protocol can be 'http' or 'https'\r\n protocol = 'http'\r\n # Use 'token' as the username when connecting using a Timbr token, otherwise use the user name\r\n user_name = 'token'\r\n # If using a token as a username then the pass is the token value, otherwise its the user's password.\r\n user_pass = '<token_value_or_user_password>'\r\n # The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).\r\n hostname = '<timbr_server_host>'\r\n # The port to connect to in the Timbr server. Timbr's default port is 11000\r\n port = '<timbr_server_port>'\r\n # The name of the ontology (knowledge graph) to connect\r\n ontology = '<ontology_name>'\r\n \r\n # Create new sqlalchemy connection\r\n engine = create_engine(f\"hive+{protocol}://{user_name}@{ontology}:{user_pass}@{hostname}:{port}\", connect_args={'configuration': {'set:hiveconf:hiveMetadata': 'true'}})\r\n conn = engine.connect()\r\n dbapi_conn = engine.raw_connection()\r\n cursor = dbapi_conn.cursor()\r\n\r\n # Use the connection to execute a query\r\n query = \"SHOW CONCEPTS\"\r\n cursor.execute(query)\r\n\r\n # Check the status of this execution\r\n status = cursor.poll().operationState\r\n while status in (TOperationState.INITIALIZED_STATE, TOperationState.RUNNING_STATE):\r\n status = cursor.poll().operationState\r\n\r\n results = cursor.fetchall()\r\n print(results)\r\n```\r\n\r\n#### Connect using PyHive Sync Query\r\n```python\r\n from sqlalchemy import create_engine\r\n from TCLIService.ttypes import TOperationState\r\n\r\n # Connection protocol can be 'http' or 'https'\r\n protocol = 'http'\r\n # Use 'token' as the username when connecting using a Timbr token, otherwise use the user name\r\n user_name = 'token'\r\n # If using a token as a username then the pass is the token value, otherwise its the user's password.\r\n user_pass = '<token_value_or_user_password>'\r\n # The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).\r\n hostname = '<timbr_server_host>'\r\n # The port to connect to in the Timbr server. Timbr's default port is 11000\r\n port = '<timbr_server_port>'\r\n # The name of the ontology (knowledge graph) to connect\r\n ontology = '<ontology_name>'\r\n \r\n # Create new sqlalchemy connection\r\n engine = create_engine(f\"hive+{protocol}://{user_name}@{ontology}:{user_pass}@{hostname}:{port}\", connect_args={'configuration': {'set:hiveconf:async': 'false', 'set:hiveconf:hiveMetadata': 'true'}})\r\n conn = engine.connect()\r\n\r\n # Use the connection to execute a query\r\n query = \"SHOW CONCEPTS\"\r\n results = conn.execute(query).fetchall()\r\n print(results)\r\n```\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Timbr Python connector",
"version": "1.0.3",
"project_urls": {
"Bug Tracker": "https://github.com/WPSemantix/timbr_python_SQLAlchemy/issues",
"Download": "https://github.com/WPSemantix/timbr_python_SQLAlchemy/archive/refs/tags/v1.0.3.tar.gz",
"Homepage": "https://github.com/WPSemantix/timbr_python_SQLAlchemy"
},
"split_keywords": [
"timbr",
"timbr-python",
"timbr-connector",
"python-connector",
"pytimbr",
"pytimbr",
"py-timbr",
"py-timbr",
"pytimbr_sqla",
"pytimbr_sqla",
"pytimbr_sqla",
"pytimbr_sqla",
"pytimbr_sqla",
"pytimbr_sqla",
"pytimbr_sqla",
"pytimbr_sqla",
"pytimbr_sqla",
"pytimbrsqlalchemy",
"pytimbrsqlalchemy",
"pytimbrsqlalchemy",
"pytimbrsqlalchemy",
"pytimbrsqlalchemy",
"py-timbrsqlalchemy",
"py-timbrsqlalchemy",
"py-timbr-sqlalchemy",
"py-timbr-sqlalchemy"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "767e736fbd3b6ba9d65bd4780da4cf47b000d13e2975e0fc54fefb9c1c11d549",
"md5": "68e7746d8871b2c24a488b2c8280ed4c",
"sha256": "66e5b5035cd57160aa12889d513c72a34f1b63175c86fddc18b445e9f8e9174b"
},
"downloads": -1,
"filename": "pytimbr_sqla-1.0.3.tar.gz",
"has_sig": false,
"md5_digest": "68e7746d8871b2c24a488b2c8280ed4c",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 40071,
"upload_time": "2023-12-20T13:21:11",
"upload_time_iso_8601": "2023-12-20T13:21:11.300498Z",
"url": "https://files.pythonhosted.org/packages/76/7e/736fbd3b6ba9d65bd4780da4cf47b000d13e2975e0fc54fefb9c1c11d549/pytimbr_sqla-1.0.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-12-20 13:21:11",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "WPSemantix",
"github_project": "timbr_python_SQLAlchemy",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "pytimbr-sqla"
}