PyTweening
==========
A collection of tweening (aka easing) functions implemented in Python. You can learn more about it in this blog post: https://inventwithpython.com/blog/2024/02/20/make-lively-movement-animation-with-pytweenings-tweening-functions/ and in the Nordic Game Jam talk by Martin Jonasson and Petri Purho at https://youtu.be/Fy0aCDmgnxg?si=8pgITaxjJSKFyBuB&t=159
Example Usage
=============
All tweening functions are passed an argument of a float from 0.0 (the beginning of the path) to 1.0 (the end of the path) of the tween:
>>> pytweening.linear(0.5)
0.5
>>> pytweening.linear(0.75)
0.75
>>> pytweening.linear(1.0)
1.0
>>> pytweening.easeInQuad(0.5)
0.25
>>> pytweening.easeInQuad(0.75)
0.5625
>>> pytweening.easeInQuad(1.0)
1.0
>>> pytweening.easeInOutSine(0.75)
0.8535533905932737
>>> pytweening.easeInOutSine(1.0)
1.0
The getLine() function also provides a Bresenham line algorithm implementation:
>>> pytweening.getLine(0, 0, 5, 10)
[(0, 0), (0, 1), (1, 2), (1, 3), (2, 4), (2, 5), (3, 6), (3, 7), (4, 8), (4, 9), (5, 10)]
The getLinePoint() function finds (interpolates) a point on the given line (even if it extends before or past the start or end points):
>>> getLinePoint(0, 0, 5, 10, 0.0)
(0.0, 0.0)
>>> getLinePoint(0, 0, 5, 10, 0.25)
(1.25, 2.5)
>>> getLinePoint(0, 0, 5, 10, 0.5)
(2.5, 5.0)
>>> getLinePoint(0, 0, 5, 10, 0.75)
(3.75, 7.5)
>>> getLinePoint(0, 0, 5, 10, 1.0)
(5.0, 10.0)
PyTweening also provides iterators to get the XY coordinates in a for loop between two points (though some floating-point rounding errors naturally occur):
>>> import pytweening
>>> for x, y in pytweening.iterLinear(0, 0, 100, 150, 0.1): print(x, y)
...
0.0 0.0
10.0 15.0
20.0 30.0
30.000000000000004 45.00000000000001
40.0 60.0
50.0 75.0
60.0 90.0
70.0 105.0
80.0 119.99999999999999
89.99999999999999 135.0
100.0 150.0
>>> for x, y in pytweening.iterEaseOutQuad(0, 0, 100, 150, 0.1): print(x, y)
...
0.0 0.0
19.0 28.5
36.00000000000001 54.00000000000001
51.0 76.5
64.00000000000001 96.00000000000001
75.0 112.5
84.0 126.0
90.99999999999999 136.5
96.00000000000001 144.0
99.0 148.5
100.0 150.0
Tweens
======
pytweening.linear()
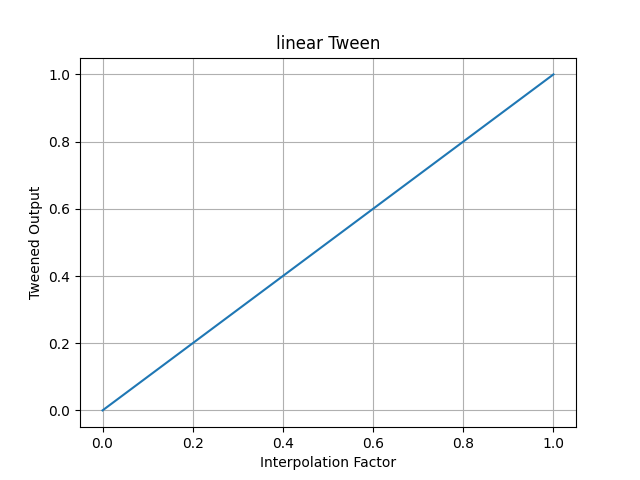
pytweening.easeInQuad()
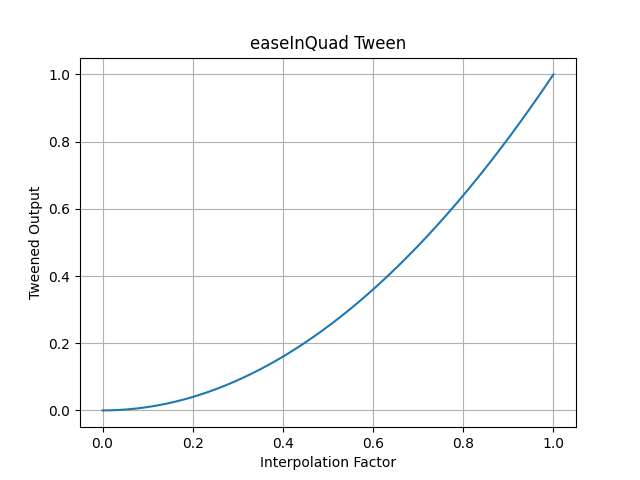
pytweening.easeOutQuad()
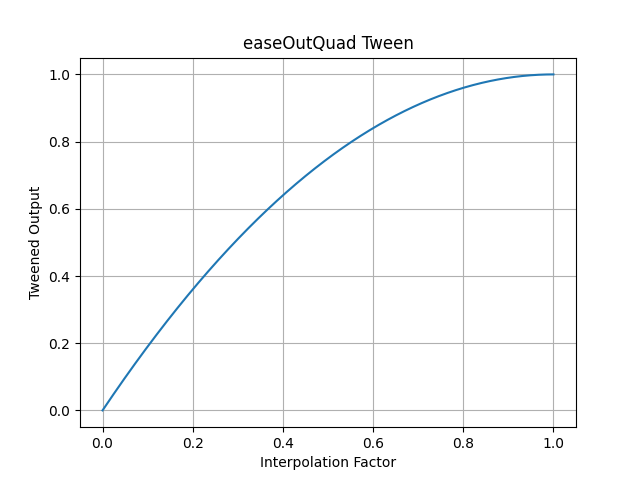
pytweening.easeInOutQuad()
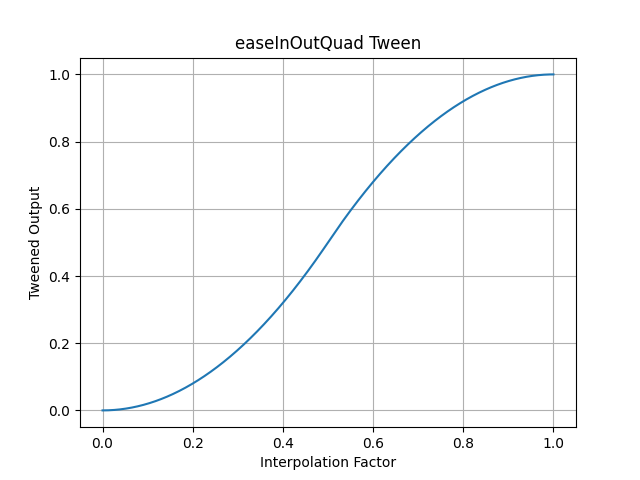
pytweening.easeInCubic()
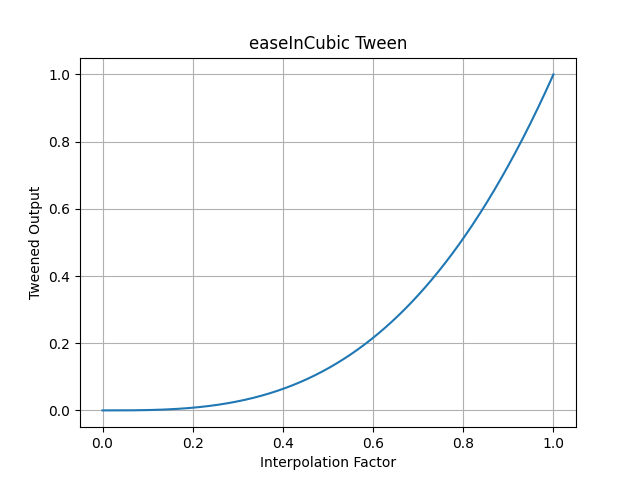
pytweening.easeOutCubic()
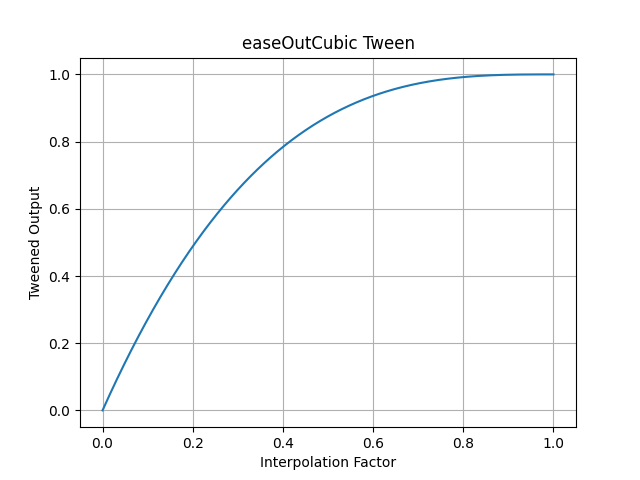
pytweening.easeInOutCubic()
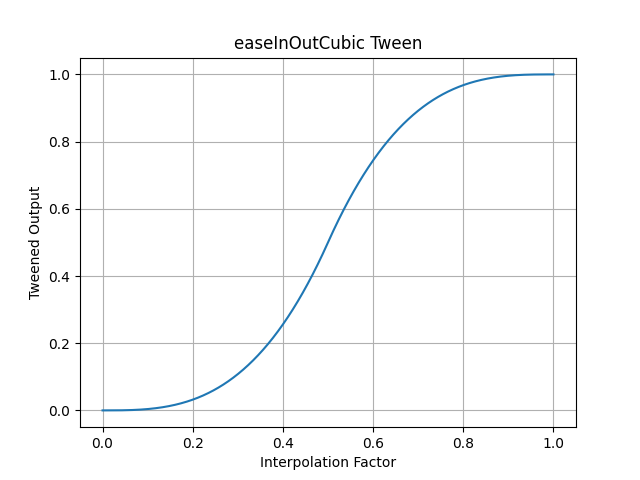
pytweening.easeInQuart()
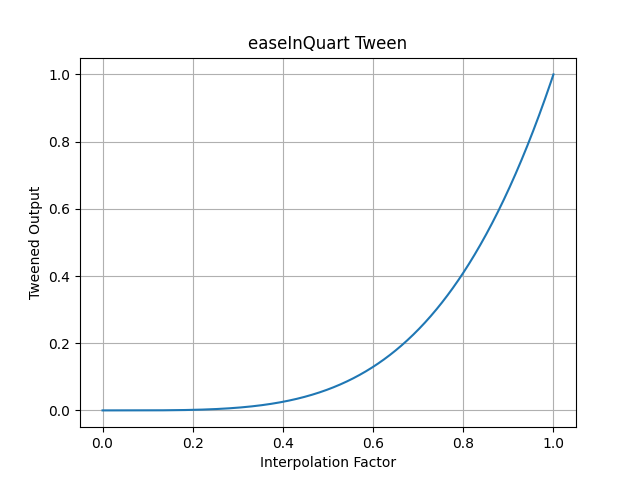
pytweening.easeOutQuart()
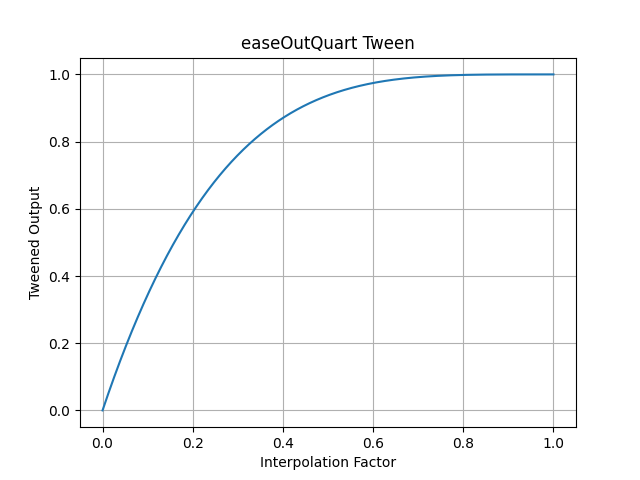
pytweening.easeInOutQuart()
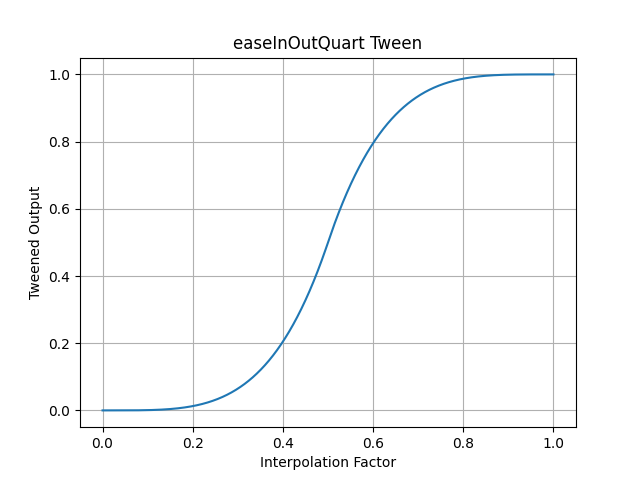
pytweening.easeInQuint()
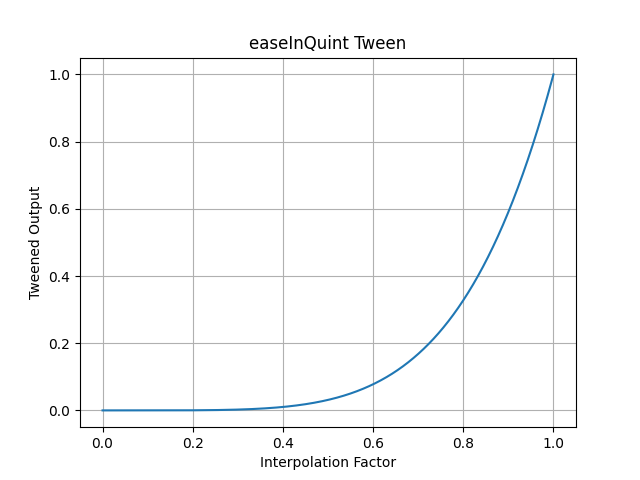
pytweening.easeOutQuint()
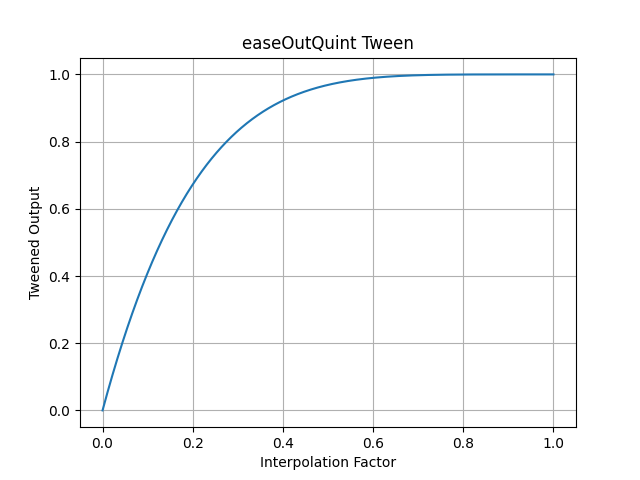
pytweening.easeInOutQuint()
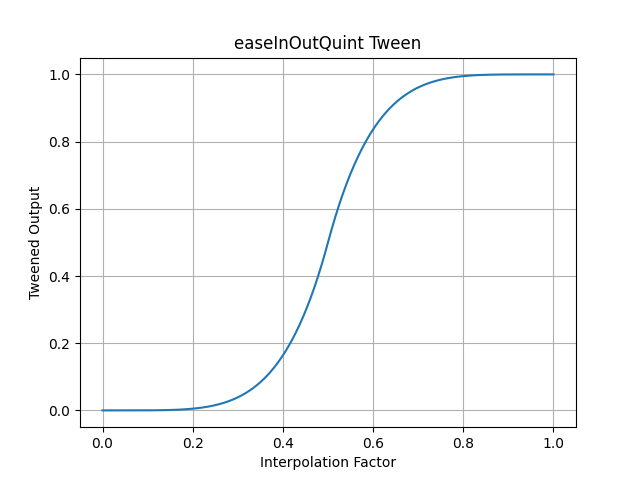
pytweening.easeInSine()
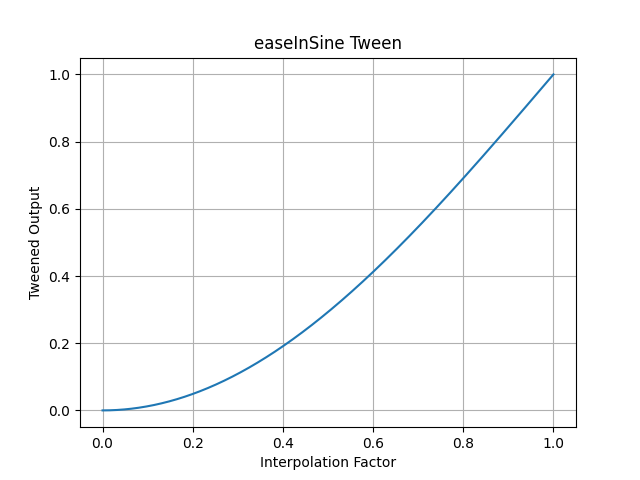
pytweening.easeOutSine()
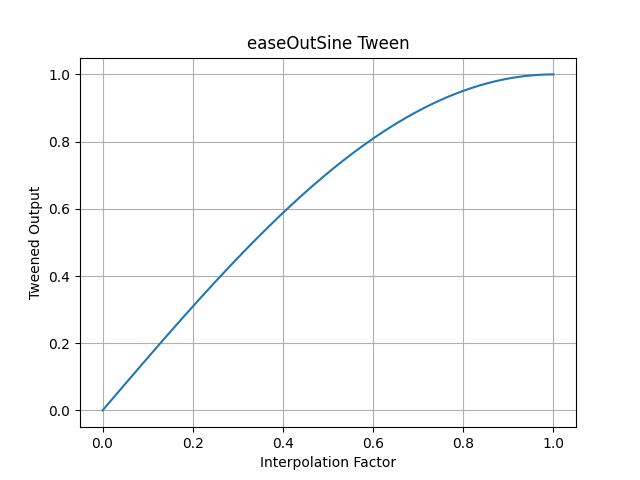
pytweening.easeInOutSine()
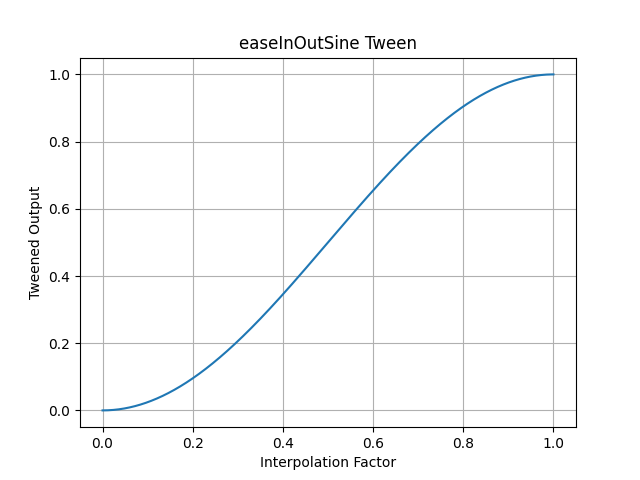
pytweening.easeInExpo()
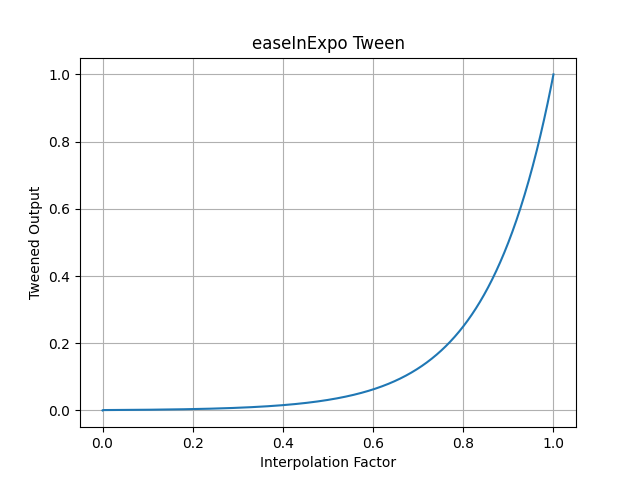
pytweening.easeOutExpo()
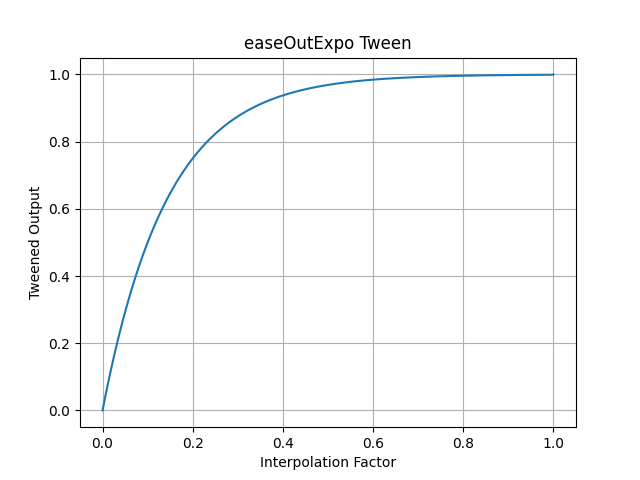
pytweening.easeInOutExpo()
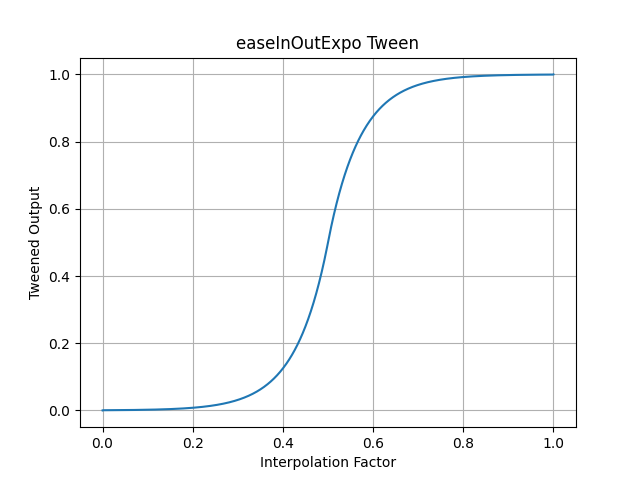
pytweening.easeInCirc()
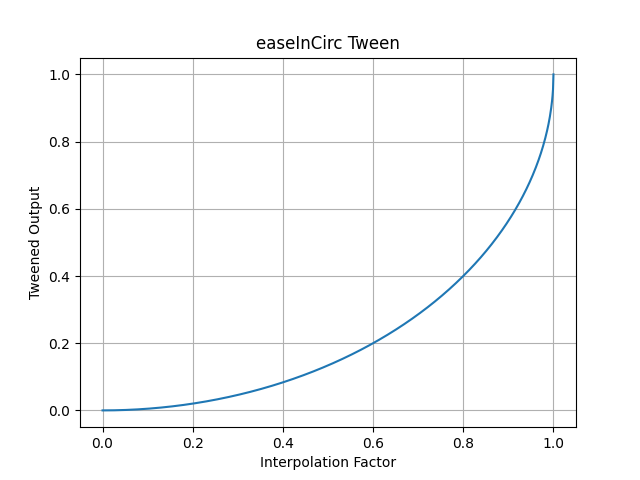
pytweening.easeOutCirc()
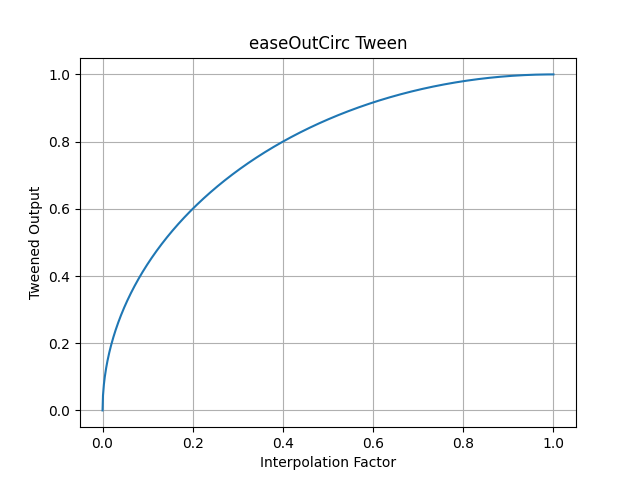
pytweening.easeInOutCirc()
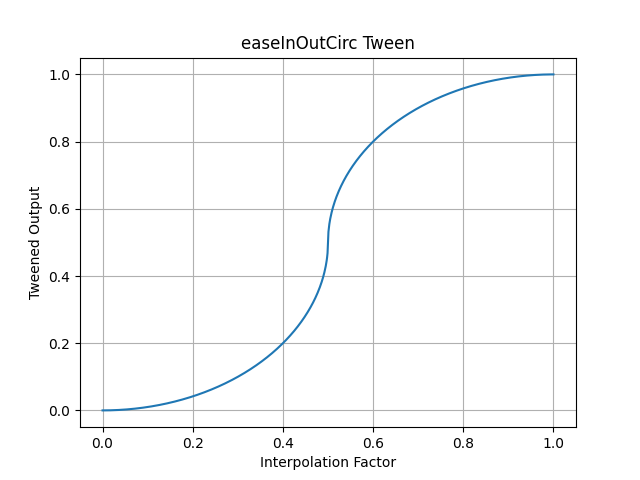
pytweening.easeInElastic()
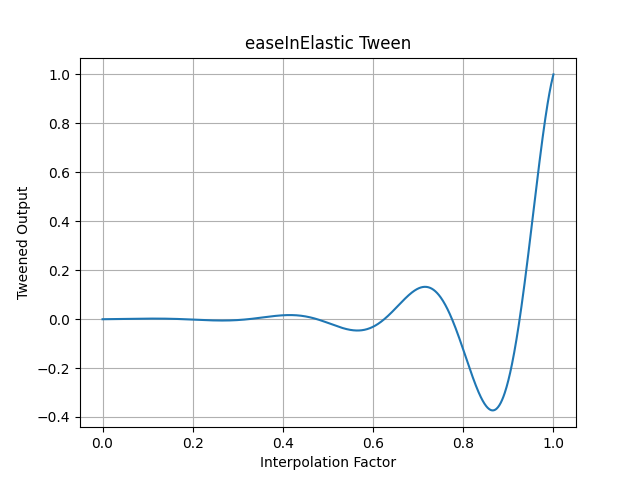
pytweening.easeOutElastic()
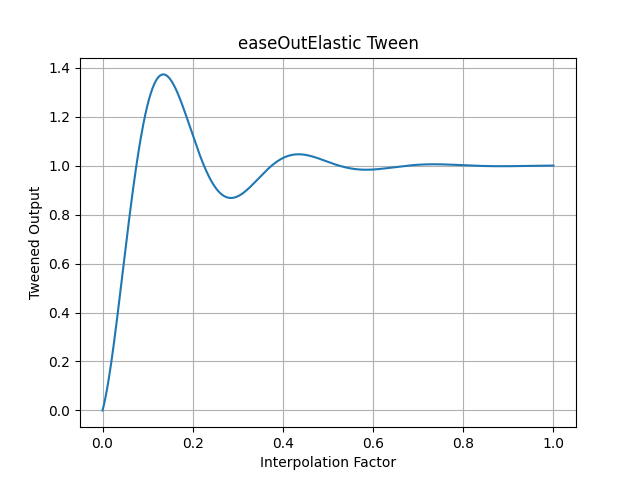
pytweening.easeInOutElastic()
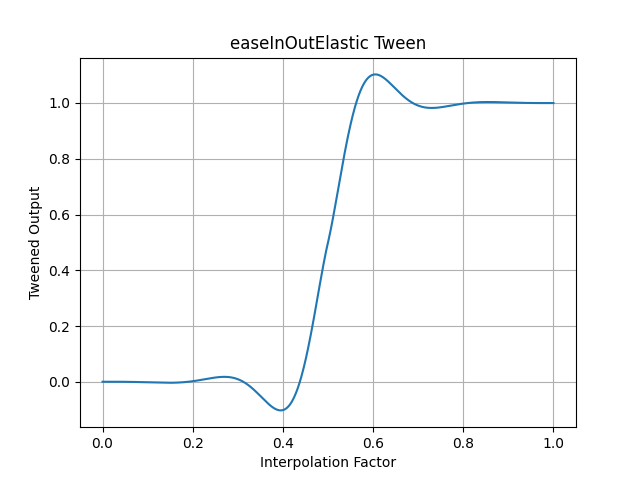
pytweening.easeInBack()
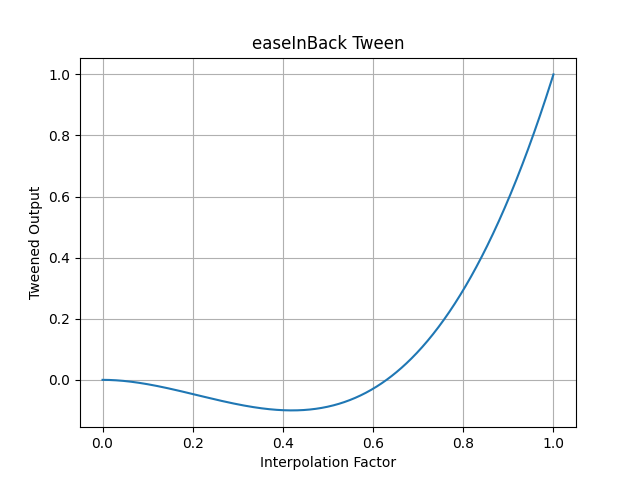
pytweening.easeOutBack()
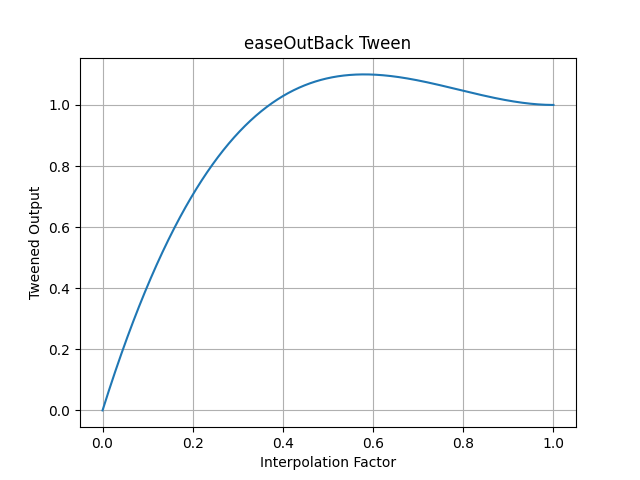
pytweening.easeInOutBack()
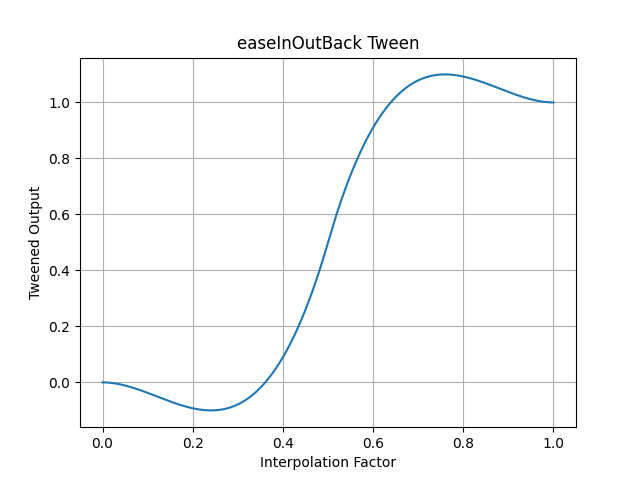
pytweening.easeInBounce()
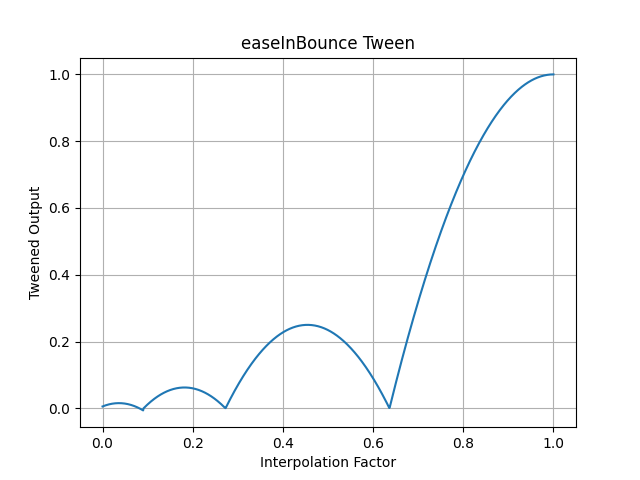
pytweening.easeOutBounce()
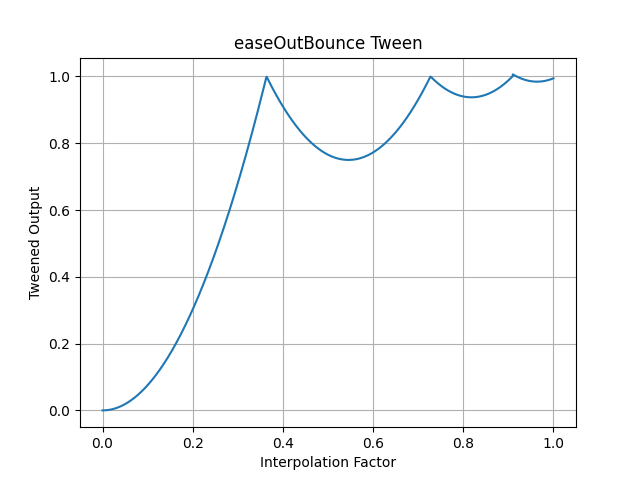
pytweening.easeInOutBounce()
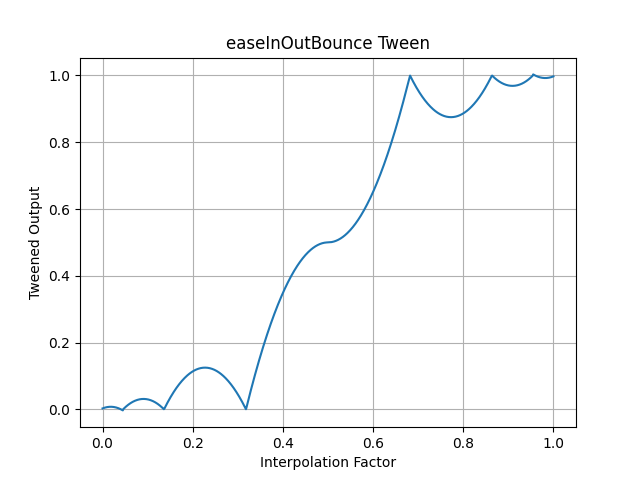
pytweening.easeInPoly() (default degree of 2)
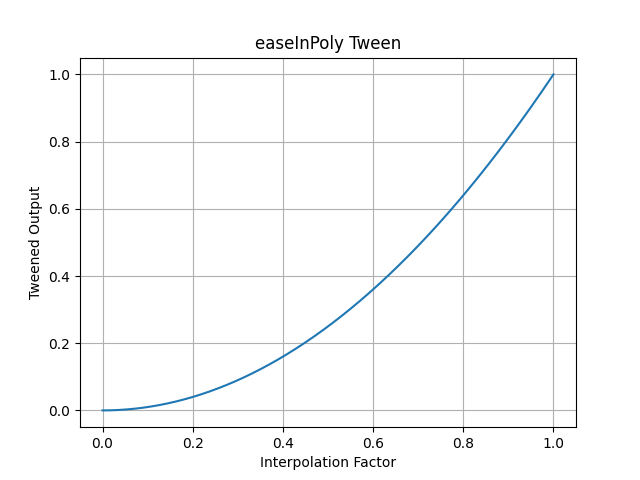
pytweening.easeOutPoly() (default degree of 2)
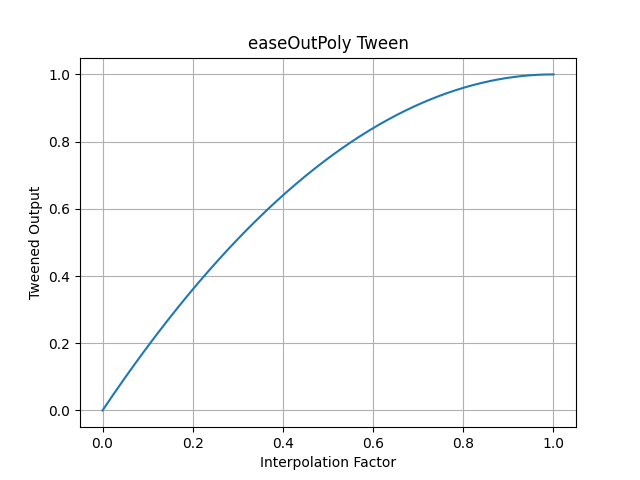
pytweening.easeInOutPoly() (default degree of 2)
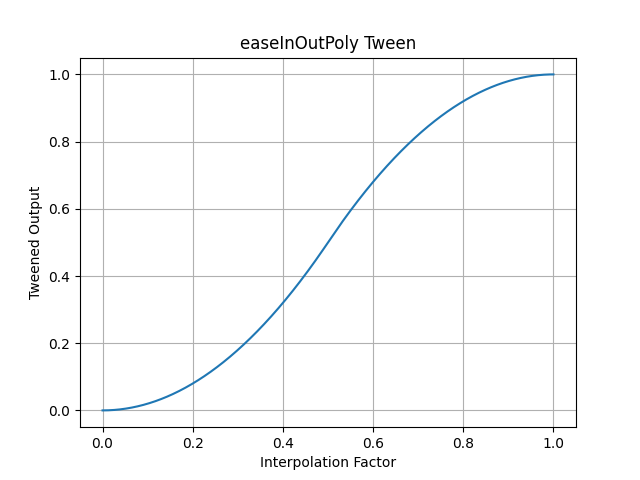
Support
-------
If you find this project helpful and would like to support its development, [consider donating to its creator on Patreon](https://www.patreon.com/AlSweigart).
Raw data
{
"_id": null,
"home_page": "https://github.com/asweigart/pytweening",
"name": "pytweening",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "2D animation tween tweening easing",
"author": "Al Sweigart",
"author_email": "al@inventwithpython.com",
"download_url": "https://files.pythonhosted.org/packages/79/0c/c16bc93ac2755bac0066a8ecbd2a2931a1735a6fffd99a2b9681c7e83e90/pytweening-1.2.0.tar.gz",
"platform": null,
"description": "PyTweening\r\n==========\r\n\r\nA collection of tweening (aka easing) functions implemented in Python. You can learn more about it in this blog post: https://inventwithpython.com/blog/2024/02/20/make-lively-movement-animation-with-pytweenings-tweening-functions/ and in the Nordic Game Jam talk by Martin Jonasson and Petri Purho at https://youtu.be/Fy0aCDmgnxg?si=8pgITaxjJSKFyBuB&t=159\r\n\r\nExample Usage\r\n=============\r\n\r\nAll tweening functions are passed an argument of a float from 0.0 (the beginning of the path) to 1.0 (the end of the path) of the tween:\r\n\r\n >>> pytweening.linear(0.5)\r\n 0.5\r\n >>> pytweening.linear(0.75)\r\n 0.75\r\n >>> pytweening.linear(1.0)\r\n 1.0\r\n >>> pytweening.easeInQuad(0.5)\r\n 0.25\r\n >>> pytweening.easeInQuad(0.75)\r\n 0.5625\r\n >>> pytweening.easeInQuad(1.0)\r\n 1.0\r\n >>> pytweening.easeInOutSine(0.75)\r\n 0.8535533905932737\r\n >>> pytweening.easeInOutSine(1.0)\r\n 1.0\r\n\r\nThe getLine() function also provides a Bresenham line algorithm implementation:\r\n\r\n >>> pytweening.getLine(0, 0, 5, 10)\r\n [(0, 0), (0, 1), (1, 2), (1, 3), (2, 4), (2, 5), (3, 6), (3, 7), (4, 8), (4, 9), (5, 10)]\r\n\r\nThe getLinePoint() function finds (interpolates) a point on the given line (even if it extends before or past the start or end points):\r\n\r\n >>> getLinePoint(0, 0, 5, 10, 0.0)\r\n (0.0, 0.0)\r\n >>> getLinePoint(0, 0, 5, 10, 0.25)\r\n (1.25, 2.5)\r\n >>> getLinePoint(0, 0, 5, 10, 0.5)\r\n (2.5, 5.0)\r\n >>> getLinePoint(0, 0, 5, 10, 0.75)\r\n (3.75, 7.5)\r\n >>> getLinePoint(0, 0, 5, 10, 1.0)\r\n (5.0, 10.0)\r\n\r\nPyTweening also provides iterators to get the XY coordinates in a for loop between two points (though some floating-point rounding errors naturally occur):\r\n\r\n >>> import pytweening\r\n >>> for x, y in pytweening.iterLinear(0, 0, 100, 150, 0.1): print(x, y)\r\n ...\r\n 0.0 0.0\r\n 10.0 15.0\r\n 20.0 30.0\r\n 30.000000000000004 45.00000000000001\r\n 40.0 60.0\r\n 50.0 75.0\r\n 60.0 90.0\r\n 70.0 105.0\r\n 80.0 119.99999999999999\r\n 89.99999999999999 135.0\r\n 100.0 150.0\r\n >>> for x, y in pytweening.iterEaseOutQuad(0, 0, 100, 150, 0.1): print(x, y)\r\n ...\r\n 0.0 0.0\r\n 19.0 28.5\r\n 36.00000000000001 54.00000000000001\r\n 51.0 76.5\r\n 64.00000000000001 96.00000000000001\r\n 75.0 112.5\r\n 84.0 126.0\r\n 90.99999999999999 136.5\r\n 96.00000000000001 144.0\r\n 99.0 148.5\r\n 100.0 150.0\r\n\r\n\r\nTweens\r\n======\r\n\r\npytweening.linear()\r\n\r\n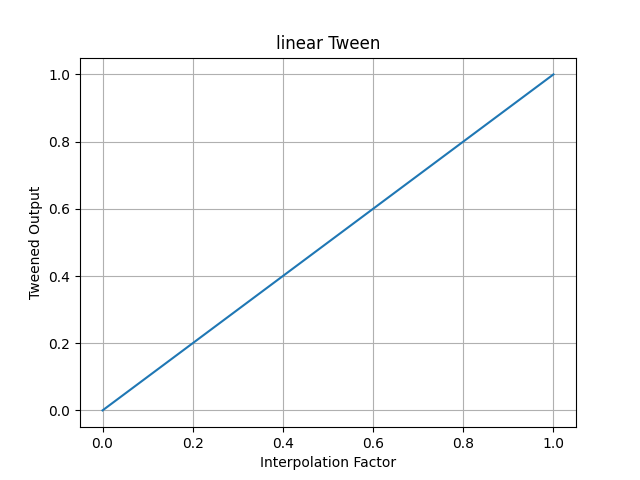\r\n\r\npytweening.easeInQuad()\r\n\r\n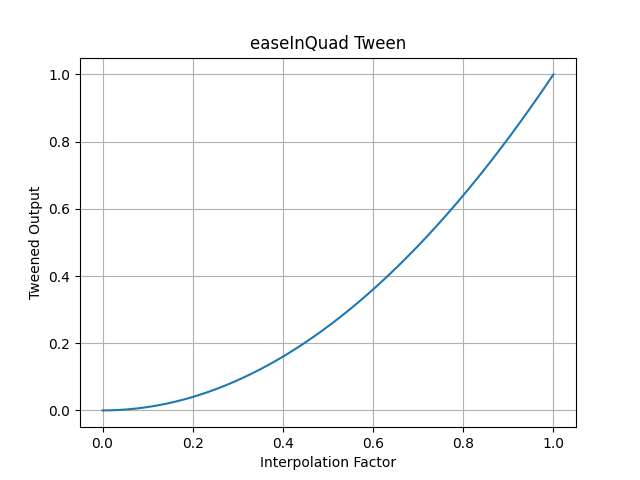\r\n\r\npytweening.easeOutQuad()\r\n\r\n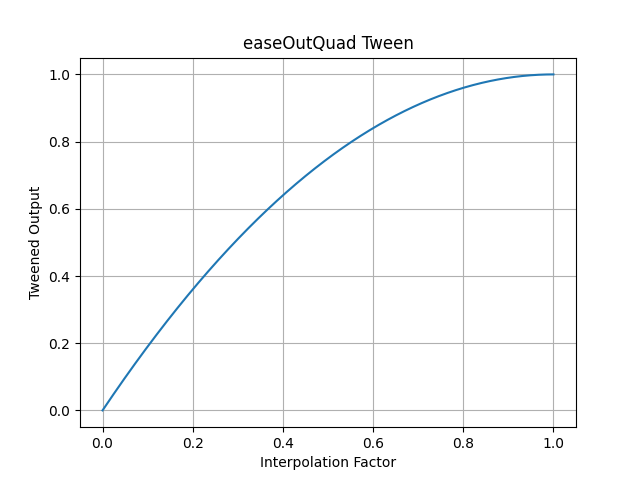\r\n\r\npytweening.easeInOutQuad()\r\n\r\n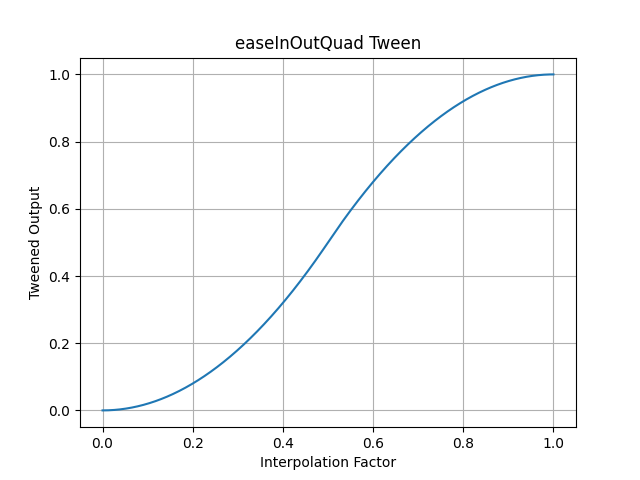\r\n\r\npytweening.easeInCubic()\r\n\r\n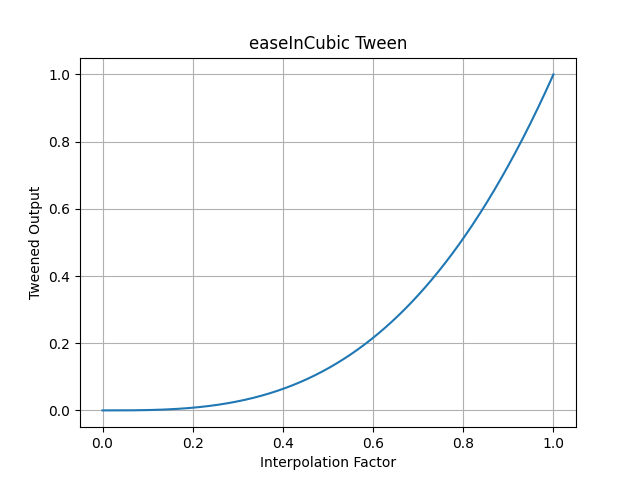\r\n\r\npytweening.easeOutCubic()\r\n\r\n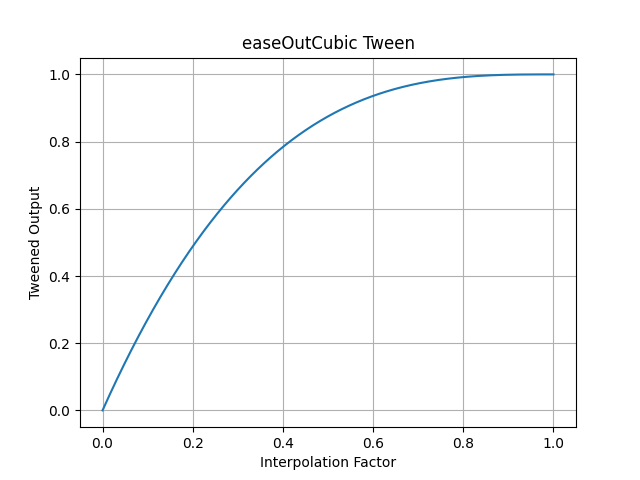\r\n\r\npytweening.easeInOutCubic()\r\n\r\n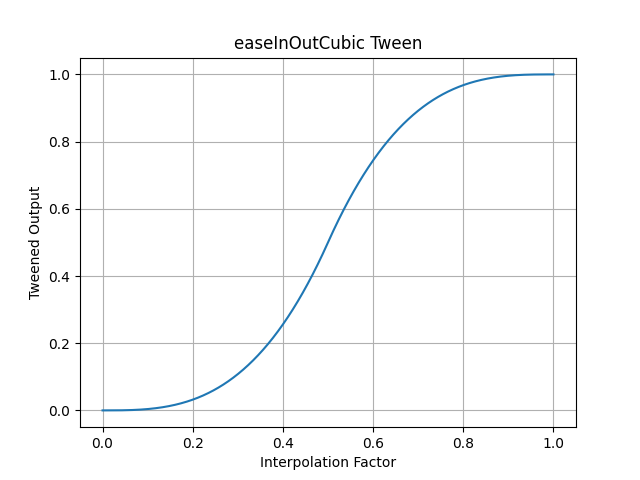\r\n\r\npytweening.easeInQuart()\r\n\r\n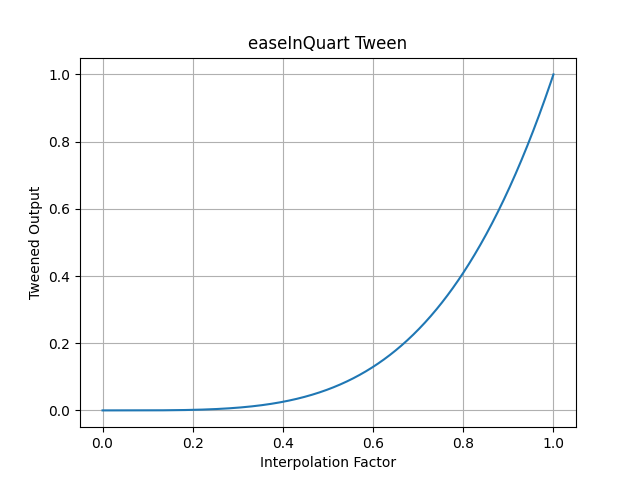\r\n\r\npytweening.easeOutQuart()\r\n\r\n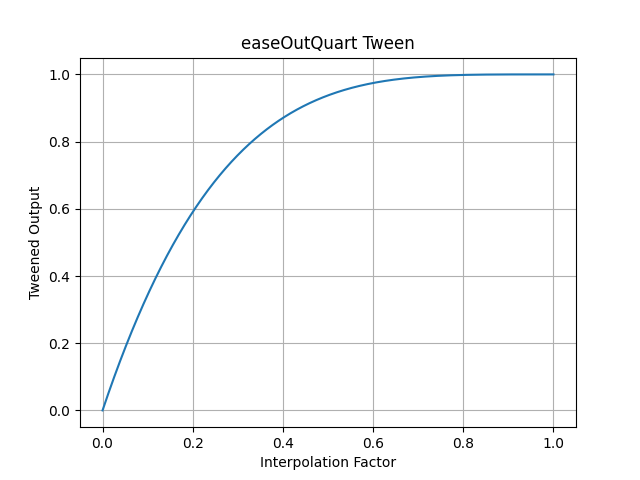\r\n\r\npytweening.easeInOutQuart()\r\n\r\n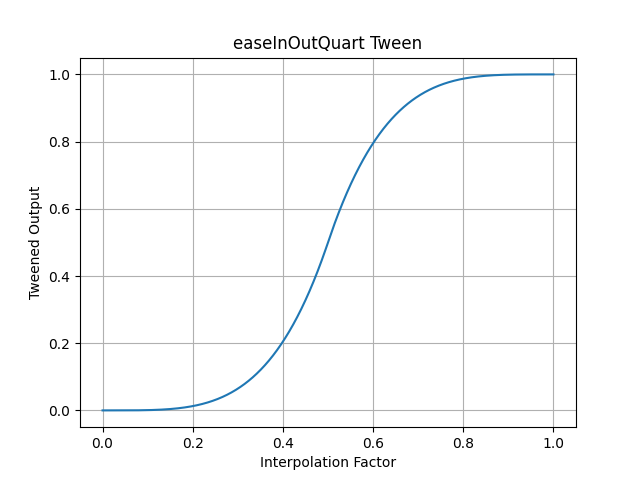\r\n\r\npytweening.easeInQuint()\r\n\r\n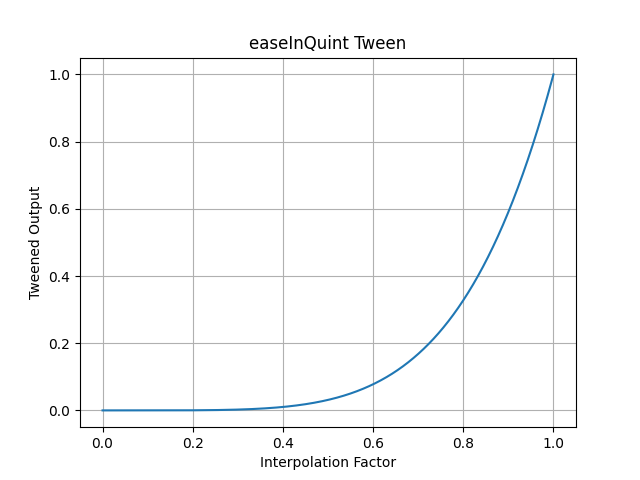\r\n\r\npytweening.easeOutQuint()\r\n\r\n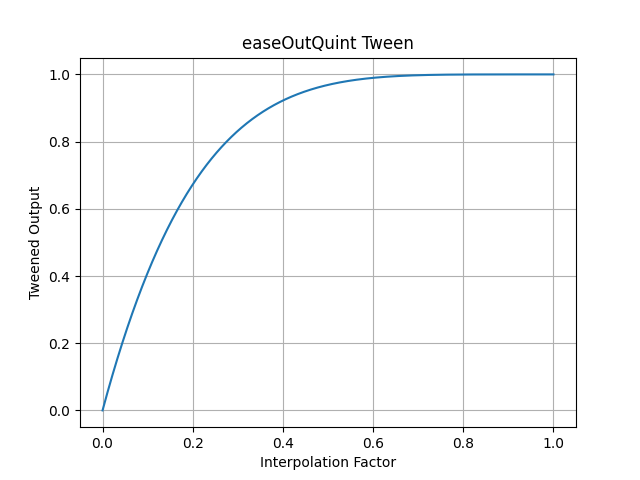\r\n\r\npytweening.easeInOutQuint()\r\n\r\n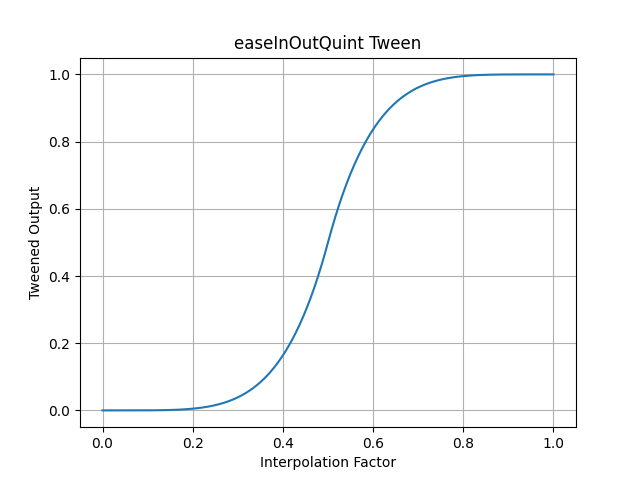\r\n\r\npytweening.easeInSine()\r\n\r\n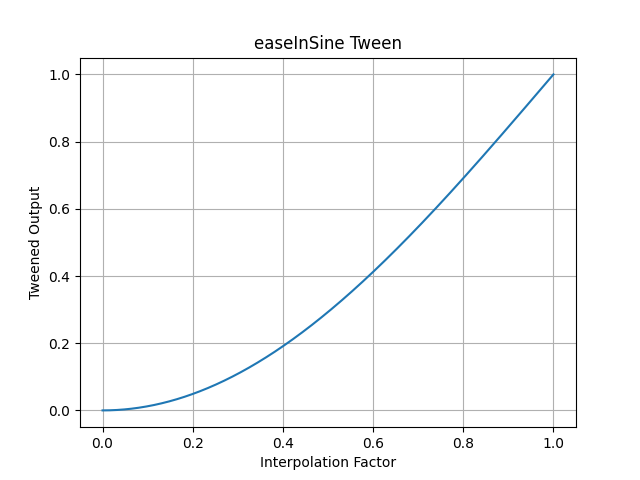\r\n\r\npytweening.easeOutSine()\r\n\r\n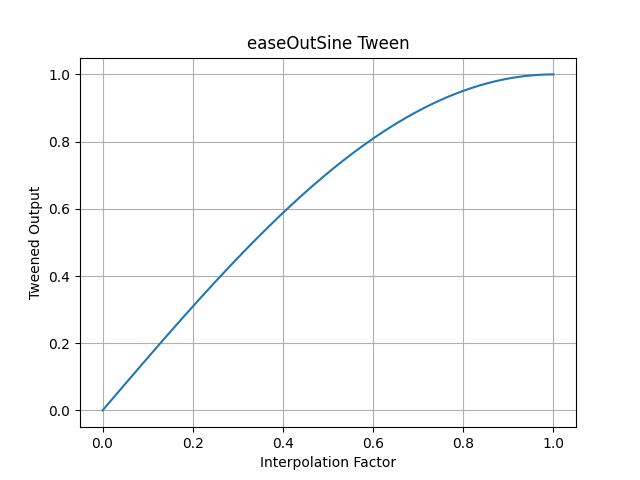\r\n\r\npytweening.easeInOutSine()\r\n\r\n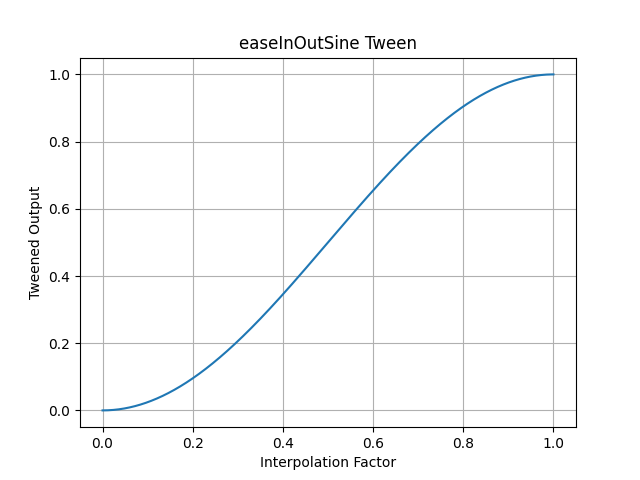\r\n\r\npytweening.easeInExpo()\r\n\r\n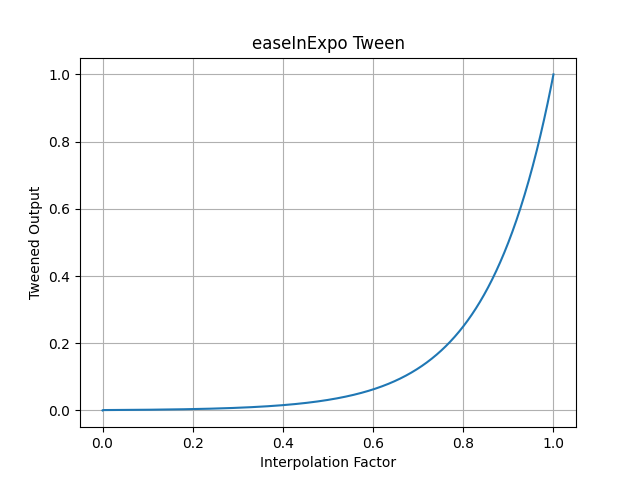\r\n\r\npytweening.easeOutExpo()\r\n\r\n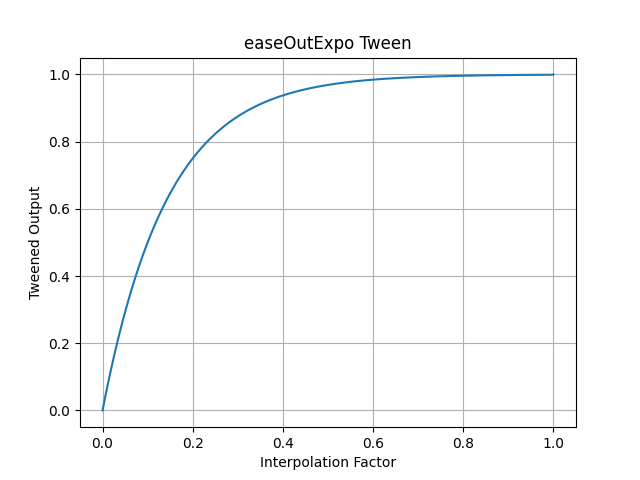\r\n\r\npytweening.easeInOutExpo()\r\n\r\n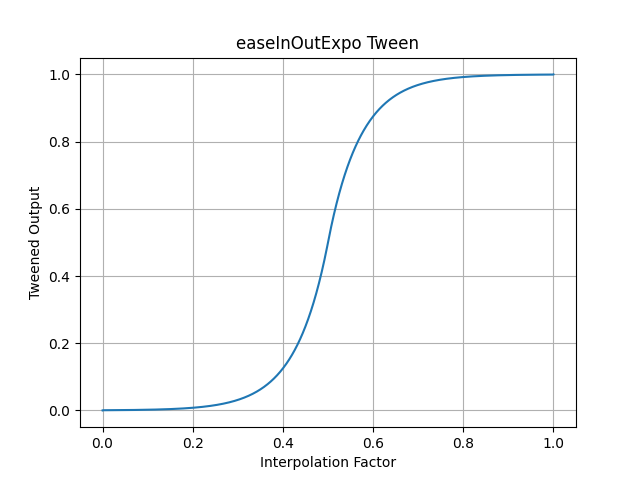\r\n\r\npytweening.easeInCirc()\r\n\r\n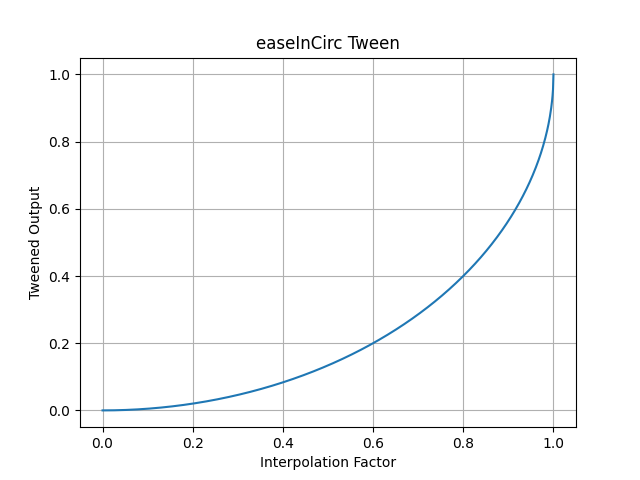\r\n\r\npytweening.easeOutCirc()\r\n\r\n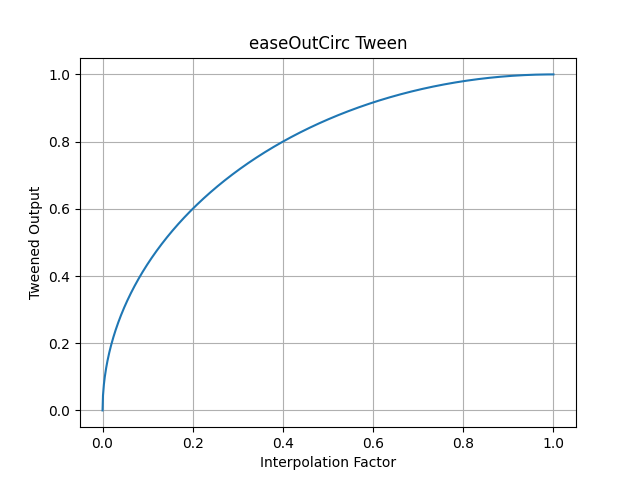\r\n\r\npytweening.easeInOutCirc()\r\n\r\n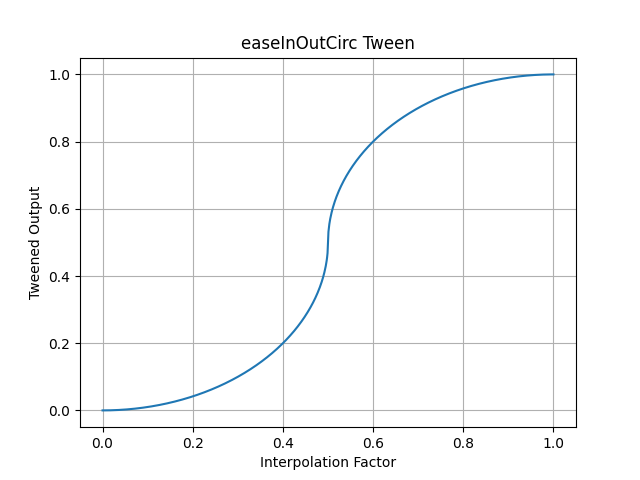\r\n\r\npytweening.easeInElastic()\r\n\r\n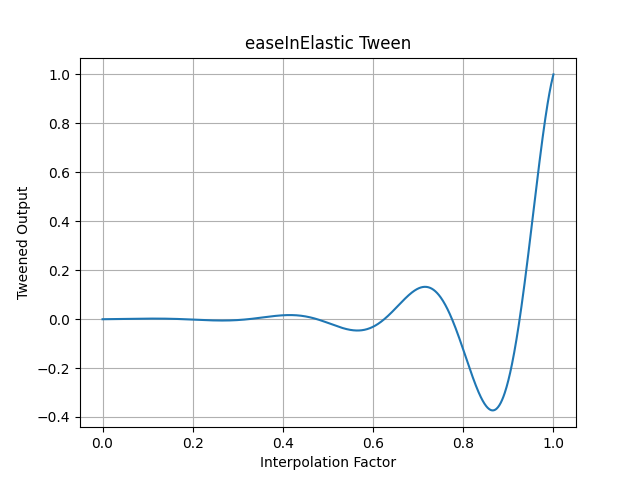\r\n\r\npytweening.easeOutElastic()\r\n\r\n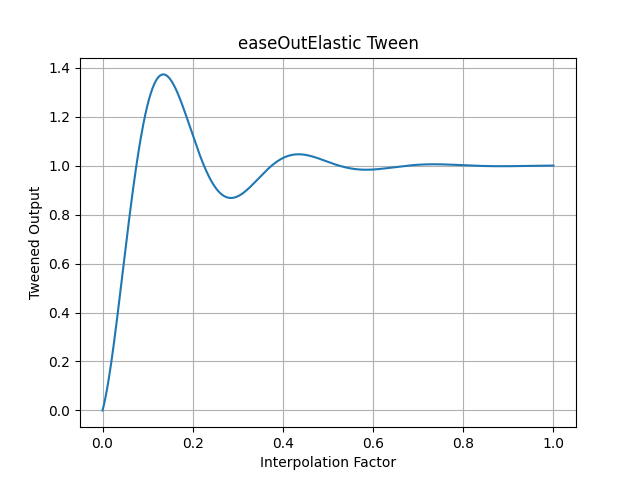\r\n\r\npytweening.easeInOutElastic()\r\n\r\n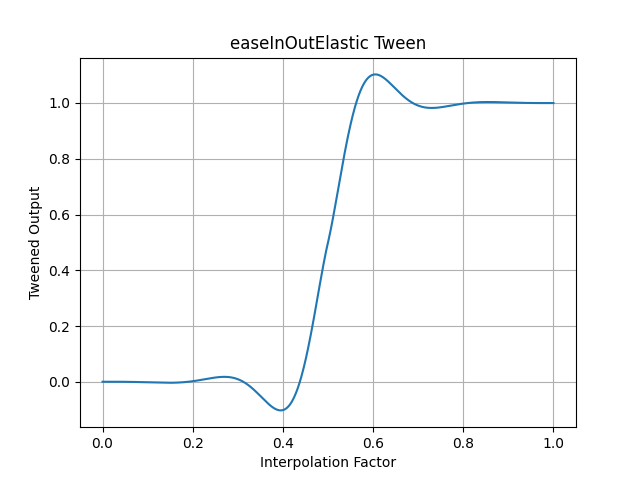\r\n\r\npytweening.easeInBack()\r\n\r\n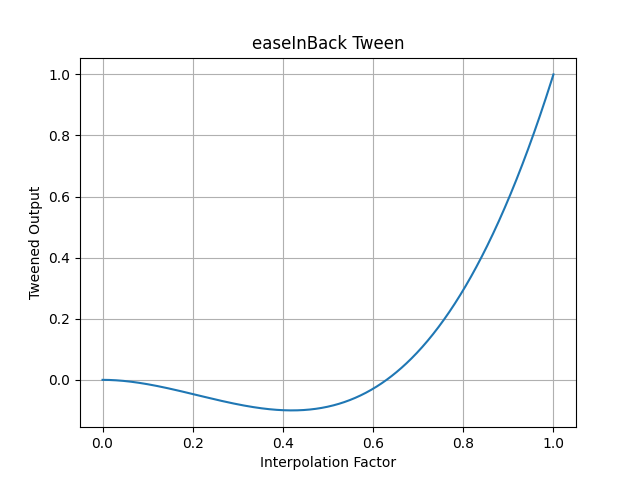\r\n\r\npytweening.easeOutBack()\r\n\r\n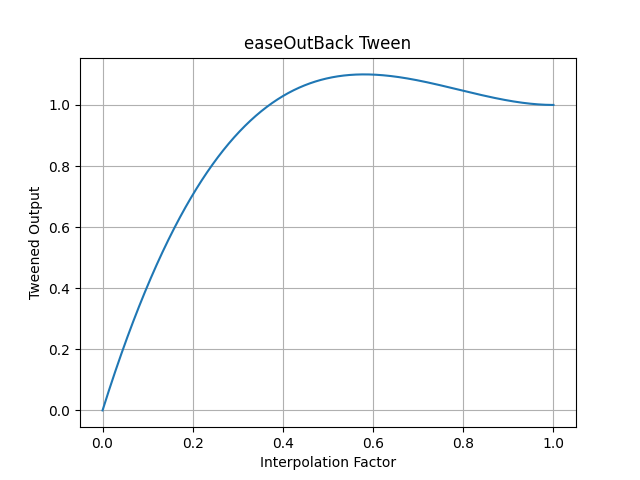\r\n\r\npytweening.easeInOutBack()\r\n\r\n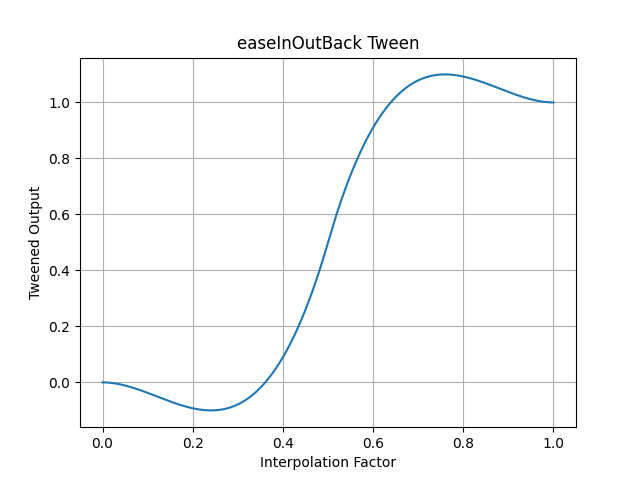\r\n\r\npytweening.easeInBounce()\r\n\r\n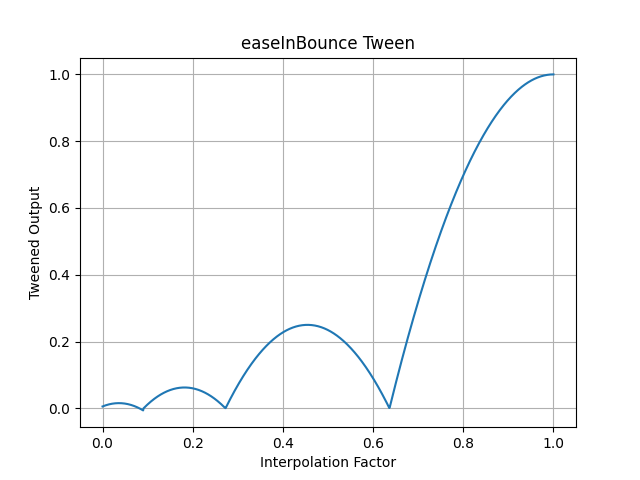\r\n\r\npytweening.easeOutBounce()\r\n\r\n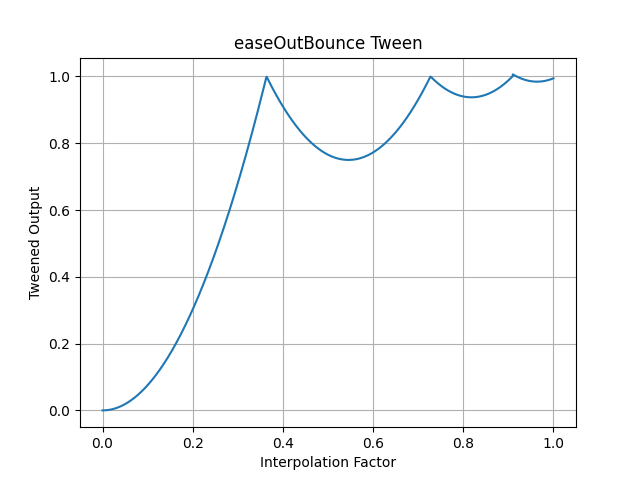\r\n\r\npytweening.easeInOutBounce()\r\n\r\n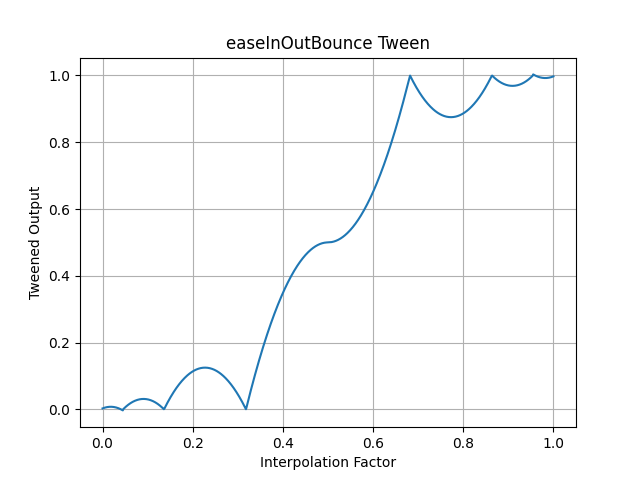\r\n\r\npytweening.easeInPoly() (default degree of 2)\r\n\r\n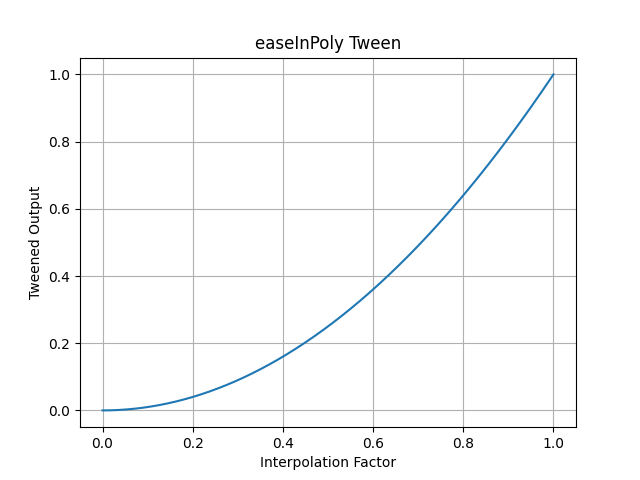\r\n\r\npytweening.easeOutPoly() (default degree of 2)\r\n\r\n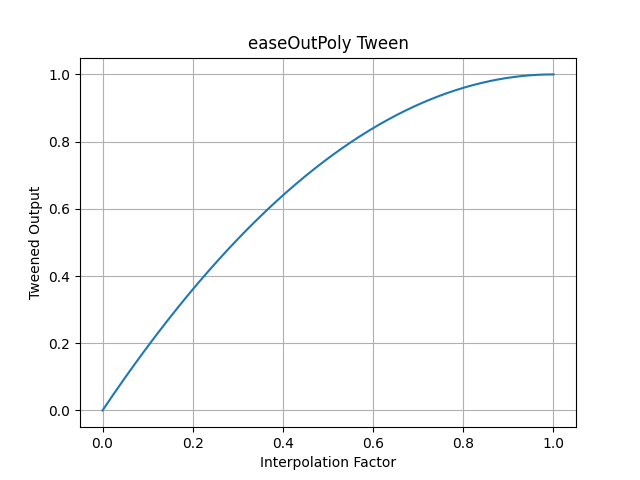\r\n\r\npytweening.easeInOutPoly() (default degree of 2)\r\n\r\n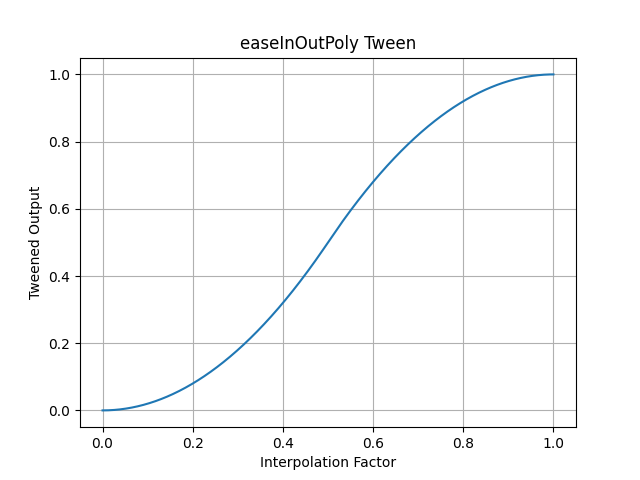\r\n\r\n\r\nSupport\r\n-------\r\n\r\nIf you find this project helpful and would like to support its development, [consider donating to its creator on Patreon](https://www.patreon.com/AlSweigart).\r\n\r\n\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A collection of tweening (aka easing) functions.",
"version": "1.2.0",
"project_urls": {
"Homepage": "https://github.com/asweigart/pytweening"
},
"split_keywords": [
"2d",
"animation",
"tween",
"tweening",
"easing"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "790cc16bc93ac2755bac0066a8ecbd2a2931a1735a6fffd99a2b9681c7e83e90",
"md5": "bc7a083daeefcaaf5d2a2ed31990bb0d",
"sha256": "243318b7736698066c5f362ec5c2b6434ecf4297c3c8e7caa8abfe6af4cac71b"
},
"downloads": -1,
"filename": "pytweening-1.2.0.tar.gz",
"has_sig": false,
"md5_digest": "bc7a083daeefcaaf5d2a2ed31990bb0d",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 171241,
"upload_time": "2024-02-20T03:37:56",
"upload_time_iso_8601": "2024-02-20T03:37:56.809919Z",
"url": "https://files.pythonhosted.org/packages/79/0c/c16bc93ac2755bac0066a8ecbd2a2931a1735a6fffd99a2b9681c7e83e90/pytweening-1.2.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-02-20 03:37:56",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "asweigart",
"github_project": "pytweening",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "pytweening"
}