[](https://www.rust-lang.org/)
[](https://github.com/Anexen/pyxirr/blob/master/LICENSE)
[](https://pypi.org/project/pyxirr/)
[](https://pypi.org/project/pyxirr/)
# PyXIRR
Rust-powered collection of financial functions.
PyXIRR stands for "Python XIRR" (for historical reasons), but contains many other financial functions such as IRR, FV, NPV, etc.
Features:
- correct
- supports different day count conventions (e.g. ACT/360, 30E/360, etc.)
- works with different input data types (iterators, numpy arrays, pandas DataFrames)
- no external dependencies
- type annotations
- blazingly fast
# Installation
```
pip install pyxirr
```
> WASM wheels for [pyodide](https://github.com/pyodide/pyodide) are also available,
> but unfortunately are [not supported by PyPI](https://github.com/pypi/warehouse/issues/10416).
> You can find them on the [GitHub Releases](https://github.com/Anexen/pyxirr/releases) page.
# Benchmarks
Rust implementation has been tested against existing [xirr](https://pypi.org/project/xirr/) package
(uses [scipy.optimize](https://docs.scipy.org/doc/scipy/reference/generated/scipy.optimize.newton.html) under the hood)
and the [implementation from the Stack Overflow](https://stackoverflow.com/a/11503492) (pure python).
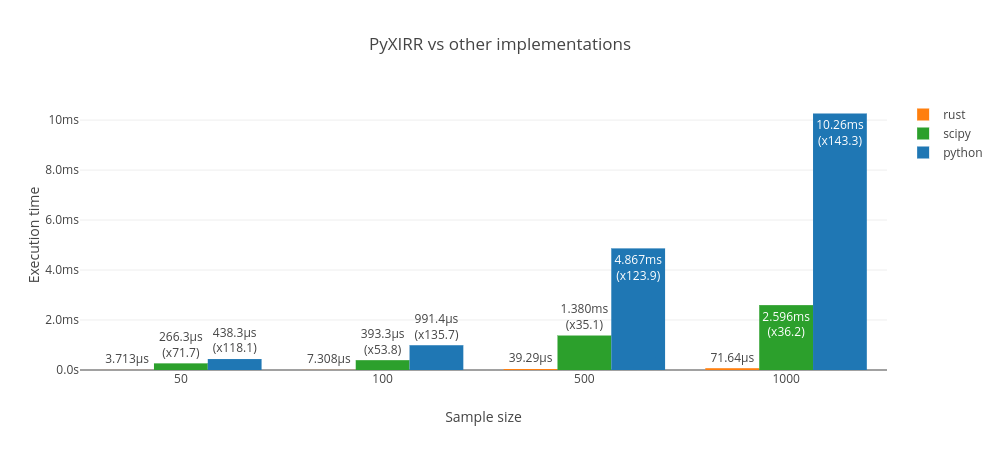
PyXIRR is much faster than the other implementations.
Powered by [github-action-benchmark](https://github.com/benchmark-action/github-action-benchmark) and [plotly.js](https://github.com/plotly/plotly.js).
Live benchmarks are hosted on [Github Pages](https://anexen.github.io/pyxirr/bench).
# Example
```python
from datetime import date
from pyxirr import xirr
dates = [date(2020, 1, 1), date(2021, 1, 1), date(2022, 1, 1)]
amounts = [-1000, 750, 500]
# feed columnar data
xirr(dates, amounts)
# feed iterators
xirr(iter(dates), (x / 2 for x in amounts))
# feed an iterable of tuples
xirr(zip(dates, amounts))
# feed a dictionary
xirr(dict(zip(dates, amounts)))
# dates as strings
xirr(['2020-01-01', '2021-01-01'], [-1000, 1200])
```
# Multiple IRR problem
The Multiple IRR problem occurs when the signs of cash flows change more than
once. In this case, we say that the project has non-conventional cash flows.
This leads to situation, where it can have more the one IRR or have no IRR at all.
PyXIRR addresses the Multiple IRR problem as follows:
1. It looks for positive result around 0.1 (the same as Excel with the default guess=0.1).
2. If it can't find a result, it uses several other attempts and selects the lowest IRR to be conservative.
Here is an example illustrating how to identify multiple IRRs:
```python
import numpy as np
import pyxirr
# load cash flow:
cf = pd.read_csv("tests/samples/30-22.csv", names=["date", "amount"])
# check whether the cash flow is conventional:
print(pyxirr.is_conventional_cash_flow(cf["amount"])) # false
# build NPV profile:
# calculate 50 NPV values for different rates
rates = np.linspace(-0.5, 0.5, 50)
# any iterable, any rates, e.g.
# rates = [-0.5, -0.3, -0.1, 0.1, -0.6]
values = pyxirr.xnpv(rates, cf)
# print NPV profile:
# NPV changes sign two times:
# 1) between -0.316 and -0.295
# 2) between -0.03 and -0.01
print("NPV profile:")
for rate, value in zip(rates, values):
print(rate, value)
# plot NPV profile
import pandas as pd
series = pd.Series(values, index=rates)
pd.DataFrame(series[series > -1e6]).assign(zero=0).plot()
# find points where NPV function crosses zero
indexes = pyxirr.zero_crossing_points(values)
print("Zero crossing points:")
for idx in indexes:
print("between", rates[idx], "and", rates[idx+1])
# XIRR has two results:
# -0.31540826742734207
# -0.028668460065441048
for i, idx in enumerate(indexes, start=1):
rate = pyxirr.xirr(cf, guess=rates[idx])
npv = pyxirr.xnpv(rate, cf)
print(f"{i}) {rate}; XNPV = {npv}")
```
# More Examples
### Numpy and Pandas
```python
import numpy as np
import pandas as pd
# feed numpy array
xirr(np.array([dates, amounts]))
xirr(np.array(dates), np.array(amounts))
# feed DataFrame (columns names doesn't matter; ordering matters)
xirr(pd.DataFrame({"a": dates, "b": amounts}))
# feed Series with DatetimeIndex
xirr(pd.Series(amounts, index=pd.to_datetime(dates)))
# bonus: apply xirr to a DataFrame with DatetimeIndex:
df = pd.DataFrame(
index=pd.date_range("2021", "2022", freq="MS", inclusive="left"),
data={
"one": [-100] + [20] * 11,
"two": [-80] + [19] * 11,
},
)
df.apply(xirr) # Series(index=["one", "two"], data=[5.09623547168478, 8.780801977141174])
```
### Day count conventions
Check out the available options on the [docs/day-count-conventions](https://anexen.github.io/pyxirr/functions.html#day-count-conventions).
```python
from pyxirr import DayCount
xirr(dates, amounts, day_count=DayCount.ACT_360)
# parse day count from string
xirr(dates, amounts, day_count="30E/360")
```
### Private equity performance metrics
```python
from pyxirr import pe
pe.pme_plus([-20, 15, 0], index=[100, 115, 130], nav=20)
pe.direct_alpha([-20, 15, 0], index=[100, 115, 130], nav=20)
```
[Docs](https://anexen.github.io/pyxirr/private_equity.html)
### Other financial functions
```python
import pyxirr
# Future Value
pyxirr.fv(0.05/12, 10*12, -100, -100)
# Net Present Value
pyxirr.npv(0, [-40_000, 5_000, 8_000, 12_000, 30_000])
# IRR
pyxirr.irr([-100, 39, 59, 55, 20])
# ... and more! Check out the docs.
```
[Docs](https://anexen.github.io/pyxirr/functions.html)
### Vectorization
PyXIRR supports numpy-like vectorization.
If all input is scalar, returns a scalar float. If any input is array_like,
returns values for each input element. If multiple inputs are
array_like, performs broadcasting and returns values for each element.
```python
import pyxirr
# feed list
pyxirr.fv([0.05/12, 0.06/12], 10*12, -100, -100)
pyxirr.fv([0.05/12, 0.06/12], [10*12, 9*12], [-100, -200], -100)
# feed numpy array
import numpy as np
rates = np.array([0.05, 0.06, 0.07])/12
pyxirr.fv(rates, 10*12, -100, -100)
# feed any iterable!
pyxirr.fv(
np.linspace(0.01, 0.2, 10),
(x + 1 for x in range(10)),
range(-100, -1100, -100),
tuple(range(-100, -200, -10))
)
# 2d, 3d, 4d, and more!
rates = [[[[[[0.01], [0.02]]]]]]
pyxirr.fv(rates, 10*12, -100, -100)
```
# API reference
See the [docs](https://anexen.github.io/pyxirr)
# Roadmap
- [x] Implement all functions from [numpy-financial](https://numpy.org/numpy-financial/latest/index.html)
- [x] Improve docs, add more tests
- [x] Type hints
- [x] Vectorized versions of numpy-financial functions.
- [ ] Compile library for rust/javascript/python
# Development
Running tests with pyo3 is a bit tricky. In short, you need to compile your tests without `extension-module` feature to avoid linking errors.
See the following issues for the details: [#341](https://github.com/PyO3/pyo3/issues/341), [#771](https://github.com/PyO3/pyo3/issues/771).
If you are using `pyenv`, make sure you have the shared library installed (check for `${PYENV_ROOT}/versions/<version>/lib/libpython3.so` file).
```bash
$ PYTHON_CONFIGURE_OPTS="--enable-shared" pyenv install <version>
```
Install dev-requirements
```bash
$ pip install -r dev-requirements.txt
```
### Building
```bash
$ maturin develop
```
### Testing
```bash
$ LD_LIBRARY_PATH=${PYENV_ROOT}/versions/3.10.8/lib cargo test
```
### Benchmarks
```bash
$ pip install -r bench-requirements.txt
$ LD_LIBRARY_PATH=${PYENV_ROOT}/versions/3.10.8/lib cargo +nightly bench
```
# Building and distribution
This library uses [maturin](https://github.com/PyO3/maturin) to build and distribute python wheels.
```bash
$ docker run --rm -v $(pwd):/io ghcr.io/pyo3/maturin build --release --manylinux 2010 --strip
$ maturin upload target/wheels/pyxirr-${version}*
```
Raw data
{
"_id": null,
"home_page": "https://github.com/Anexen/pyxirr",
"name": "pyxirr",
"maintainer": null,
"docs_url": null,
"requires_python": "<3.14,>=3.7",
"maintainer_email": null,
"keywords": "python, fast, financial, xirr, cashflow, day count convention, PME",
"author": "Anexen",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/c5/4a/3d2dc1bf565605a4f0e3ae6d5592518bb26698f4d929140d3ea16a4bb478/pyxirr-0.10.6.tar.gz",
"platform": null,
"description": "[](https://www.rust-lang.org/)\n[](https://github.com/Anexen/pyxirr/blob/master/LICENSE)\n[](https://pypi.org/project/pyxirr/)\n[](https://pypi.org/project/pyxirr/)\n\n# PyXIRR\n\nRust-powered collection of financial functions.\n\nPyXIRR stands for \"Python XIRR\" (for historical reasons), but contains many other financial functions such as IRR, FV, NPV, etc.\n\nFeatures:\n\n- correct\n- supports different day count conventions (e.g. ACT/360, 30E/360, etc.)\n- works with different input data types (iterators, numpy arrays, pandas DataFrames)\n- no external dependencies\n- type annotations\n- blazingly fast\n\n# Installation\n\n```\npip install pyxirr\n```\n\n> WASM wheels for [pyodide](https://github.com/pyodide/pyodide) are also available,\n> but unfortunately are [not supported by PyPI](https://github.com/pypi/warehouse/issues/10416).\n> You can find them on the [GitHub Releases](https://github.com/Anexen/pyxirr/releases) page.\n\n# Benchmarks\n\nRust implementation has been tested against existing [xirr](https://pypi.org/project/xirr/) package\n(uses [scipy.optimize](https://docs.scipy.org/doc/scipy/reference/generated/scipy.optimize.newton.html) under the hood)\nand the [implementation from the Stack Overflow](https://stackoverflow.com/a/11503492) (pure python).\n\n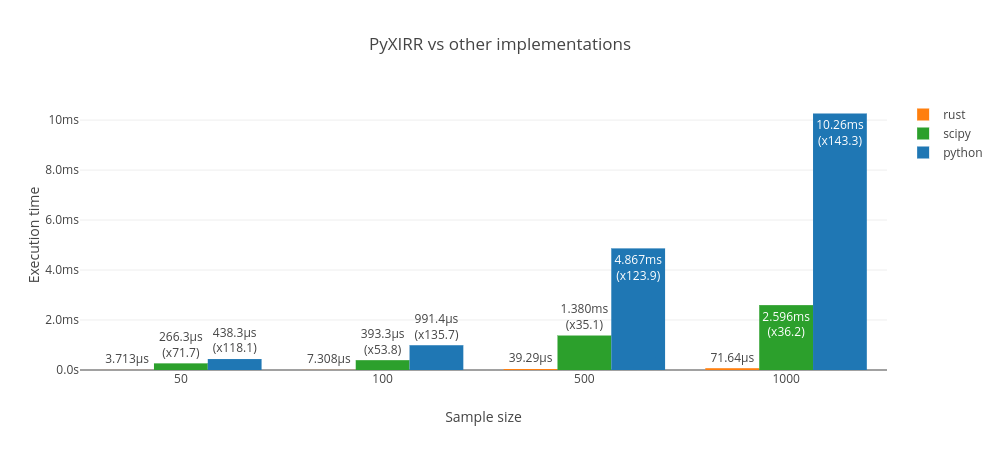\n\nPyXIRR is much faster than the other implementations.\n\nPowered by [github-action-benchmark](https://github.com/benchmark-action/github-action-benchmark) and [plotly.js](https://github.com/plotly/plotly.js).\n\nLive benchmarks are hosted on [Github Pages](https://anexen.github.io/pyxirr/bench).\n\n# Example\n\n```python\nfrom datetime import date\nfrom pyxirr import xirr\n\ndates = [date(2020, 1, 1), date(2021, 1, 1), date(2022, 1, 1)]\namounts = [-1000, 750, 500]\n\n# feed columnar data\nxirr(dates, amounts)\n# feed iterators\nxirr(iter(dates), (x / 2 for x in amounts))\n# feed an iterable of tuples\nxirr(zip(dates, amounts))\n# feed a dictionary\nxirr(dict(zip(dates, amounts)))\n# dates as strings\nxirr(['2020-01-01', '2021-01-01'], [-1000, 1200])\n```\n\n# Multiple IRR problem\n\nThe Multiple IRR problem occurs when the signs of cash flows change more than\nonce. In this case, we say that the project has non-conventional cash flows.\nThis leads to situation, where it can have more the one IRR or have no IRR at all.\n\nPyXIRR addresses the Multiple IRR problem as follows:\n\n1. It looks for positive result around 0.1 (the same as Excel with the default guess=0.1).\n2. If it can't find a result, it uses several other attempts and selects the lowest IRR to be conservative.\n\nHere is an example illustrating how to identify multiple IRRs:\n\n```python\nimport numpy as np\nimport pyxirr\n\n# load cash flow:\ncf = pd.read_csv(\"tests/samples/30-22.csv\", names=[\"date\", \"amount\"])\n# check whether the cash flow is conventional:\nprint(pyxirr.is_conventional_cash_flow(cf[\"amount\"])) # false\n\n# build NPV profile:\n# calculate 50 NPV values for different rates\nrates = np.linspace(-0.5, 0.5, 50)\n# any iterable, any rates, e.g.\n# rates = [-0.5, -0.3, -0.1, 0.1, -0.6]\nvalues = pyxirr.xnpv(rates, cf)\n\n# print NPV profile:\n# NPV changes sign two times:\n# 1) between -0.316 and -0.295\n# 2) between -0.03 and -0.01\nprint(\"NPV profile:\")\nfor rate, value in zip(rates, values):\n print(rate, value)\n\n# plot NPV profile\nimport pandas as pd\nseries = pd.Series(values, index=rates)\npd.DataFrame(series[series > -1e6]).assign(zero=0).plot()\n\n# find points where NPV function crosses zero\nindexes = pyxirr.zero_crossing_points(values)\n\nprint(\"Zero crossing points:\")\nfor idx in indexes:\n print(\"between\", rates[idx], \"and\", rates[idx+1])\n\n# XIRR has two results:\n# -0.31540826742734207\n# -0.028668460065441048\nfor i, idx in enumerate(indexes, start=1):\n rate = pyxirr.xirr(cf, guess=rates[idx])\n npv = pyxirr.xnpv(rate, cf)\n print(f\"{i}) {rate}; XNPV = {npv}\")\n```\n\n# More Examples\n\n### Numpy and Pandas\n\n```python\nimport numpy as np\nimport pandas as pd\n\n# feed numpy array\nxirr(np.array([dates, amounts]))\nxirr(np.array(dates), np.array(amounts))\n\n# feed DataFrame (columns names doesn't matter; ordering matters)\nxirr(pd.DataFrame({\"a\": dates, \"b\": amounts}))\n\n# feed Series with DatetimeIndex\nxirr(pd.Series(amounts, index=pd.to_datetime(dates)))\n\n# bonus: apply xirr to a DataFrame with DatetimeIndex:\ndf = pd.DataFrame(\n index=pd.date_range(\"2021\", \"2022\", freq=\"MS\", inclusive=\"left\"),\n data={\n \"one\": [-100] + [20] * 11,\n \"two\": [-80] + [19] * 11,\n },\n)\ndf.apply(xirr) # Series(index=[\"one\", \"two\"], data=[5.09623547168478, 8.780801977141174])\n```\n\n### Day count conventions\n\nCheck out the available options on the [docs/day-count-conventions](https://anexen.github.io/pyxirr/functions.html#day-count-conventions).\n\n```python\nfrom pyxirr import DayCount\n\nxirr(dates, amounts, day_count=DayCount.ACT_360)\n\n# parse day count from string\nxirr(dates, amounts, day_count=\"30E/360\")\n```\n\n### Private equity performance metrics\n\n```python\nfrom pyxirr import pe\n\npe.pme_plus([-20, 15, 0], index=[100, 115, 130], nav=20)\n\npe.direct_alpha([-20, 15, 0], index=[100, 115, 130], nav=20)\n```\n\n[Docs](https://anexen.github.io/pyxirr/private_equity.html)\n\n### Other financial functions\n\n```python\nimport pyxirr\n\n# Future Value\npyxirr.fv(0.05/12, 10*12, -100, -100)\n\n# Net Present Value\npyxirr.npv(0, [-40_000, 5_000, 8_000, 12_000, 30_000])\n\n# IRR\npyxirr.irr([-100, 39, 59, 55, 20])\n\n# ... and more! Check out the docs.\n```\n\n[Docs](https://anexen.github.io/pyxirr/functions.html)\n\n### Vectorization\n\nPyXIRR supports numpy-like vectorization.\n\nIf all input is scalar, returns a scalar float. If any input is array_like,\nreturns values for each input element. If multiple inputs are\narray_like, performs broadcasting and returns values for each element.\n\n```python\nimport pyxirr\n\n# feed list\npyxirr.fv([0.05/12, 0.06/12], 10*12, -100, -100)\npyxirr.fv([0.05/12, 0.06/12], [10*12, 9*12], [-100, -200], -100)\n\n# feed numpy array\nimport numpy as np\nrates = np.array([0.05, 0.06, 0.07])/12\npyxirr.fv(rates, 10*12, -100, -100)\n\n# feed any iterable!\npyxirr.fv(\n np.linspace(0.01, 0.2, 10),\n (x + 1 for x in range(10)),\n range(-100, -1100, -100),\n tuple(range(-100, -200, -10))\n)\n\n# 2d, 3d, 4d, and more!\nrates = [[[[[[0.01], [0.02]]]]]]\npyxirr.fv(rates, 10*12, -100, -100)\n```\n\n# API reference\n\nSee the [docs](https://anexen.github.io/pyxirr)\n\n# Roadmap\n\n- [x] Implement all functions from [numpy-financial](https://numpy.org/numpy-financial/latest/index.html)\n- [x] Improve docs, add more tests\n- [x] Type hints\n- [x] Vectorized versions of numpy-financial functions.\n- [ ] Compile library for rust/javascript/python\n\n# Development\n\nRunning tests with pyo3 is a bit tricky. In short, you need to compile your tests without `extension-module` feature to avoid linking errors.\nSee the following issues for the details: [#341](https://github.com/PyO3/pyo3/issues/341), [#771](https://github.com/PyO3/pyo3/issues/771).\n\nIf you are using `pyenv`, make sure you have the shared library installed (check for `${PYENV_ROOT}/versions/<version>/lib/libpython3.so` file).\n\n```bash\n$ PYTHON_CONFIGURE_OPTS=\"--enable-shared\" pyenv install <version>\n```\n\nInstall dev-requirements\n\n```bash\n$ pip install -r dev-requirements.txt\n```\n\n### Building\n\n```bash\n$ maturin develop\n```\n\n### Testing\n\n```bash\n$ LD_LIBRARY_PATH=${PYENV_ROOT}/versions/3.10.8/lib cargo test\n```\n\n### Benchmarks\n\n```bash\n$ pip install -r bench-requirements.txt\n$ LD_LIBRARY_PATH=${PYENV_ROOT}/versions/3.10.8/lib cargo +nightly bench\n```\n\n# Building and distribution\n\nThis library uses [maturin](https://github.com/PyO3/maturin) to build and distribute python wheels.\n\n```bash\n$ docker run --rm -v $(pwd):/io ghcr.io/pyo3/maturin build --release --manylinux 2010 --strip\n$ maturin upload target/wheels/pyxirr-${version}*\n```\n\n",
"bugtrack_url": null,
"license": "Unlicense",
"summary": "Rust-powered collection of financial functions for Python.",
"version": "0.10.6",
"project_urls": {
"Homepage": "https://github.com/Anexen/pyxirr"
},
"split_keywords": [
"python",
" fast",
" financial",
" xirr",
" cashflow",
" day count convention",
" pme"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "be640e780fb147d3a8d38097a96e3ead4147cef7d37ad6a1815f80ab08887c5a",
"md5": "4eb99901420457d5197432d591fdd72d",
"sha256": "106b6a647a72a42435d560ca5525ce67594df5c749496c4d8607beab6b719a53"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"has_sig": false,
"md5_digest": "4eb99901420457d5197432d591fdd72d",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 983363,
"upload_time": "2024-10-13T20:50:13",
"upload_time_iso_8601": "2024-10-13T20:50:13.198418Z",
"url": "https://files.pythonhosted.org/packages/be/64/0e780fb147d3a8d38097a96e3ead4147cef7d37ad6a1815f80ab08887c5a/pyxirr-0.10.6-cp310-cp310-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "666e5e80ec676d7eca601f86ab989c1ed1577bcd8d45564640fd292fff5df86f",
"md5": "4b117a1429742808608348bfa86a4e93",
"sha256": "2eaa3b7e4b7a3873737ae41b6d37ba9689cb7c7e474344b0a1e9dde5f64f1309"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "4b117a1429742808608348bfa86a4e93",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 473869,
"upload_time": "2024-10-13T20:49:23",
"upload_time_iso_8601": "2024-10-13T20:49:23.763511Z",
"url": "https://files.pythonhosted.org/packages/66/6e/5e80ec676d7eca601f86ab989c1ed1577bcd8d45564640fd292fff5df86f/pyxirr-0.10.6-cp310-cp310-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3f55c5987d501d686bf8a165ef3886ca10089ffe1d4a347147df09438792c87a",
"md5": "1f54ed39840cad89ca34c1e6ef9663e6",
"sha256": "1eb8612c459ea2582bbec10210b73baf0aee0e0b919b2d0421b0c63e465c02f1"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "1f54ed39840cad89ca34c1e6ef9663e6",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 512206,
"upload_time": "2024-10-13T20:50:06",
"upload_time_iso_8601": "2024-10-13T20:50:06.131411Z",
"url": "https://files.pythonhosted.org/packages/3f/55/c5987d501d686bf8a165ef3886ca10089ffe1d4a347147df09438792c87a/pyxirr-0.10.6-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "fc73876ab272781f06eaf9bc2127e615cc7ee96588b7eaaebb6eb1b685b4d610",
"md5": "2e500c0ad7ad59a778be064736f389dd",
"sha256": "543534d7bbe6e0540b3490d2c66fe9c4beb37627f7dcef04d30a3aebe3bda5d7"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"has_sig": false,
"md5_digest": "2e500c0ad7ad59a778be064736f389dd",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 508247,
"upload_time": "2024-10-13T20:49:37",
"upload_time_iso_8601": "2024-10-13T20:49:37.481871Z",
"url": "https://files.pythonhosted.org/packages/fc/73/876ab272781f06eaf9bc2127e615cc7ee96588b7eaaebb6eb1b685b4d610/pyxirr-0.10.6-cp310-cp310-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "7b00ca41547bf8ea43b1f51f0c18ccc51dd4f2367f599ceccf588848eb44683d",
"md5": "7aa98cfbc2e384d10929e373259e60b7",
"sha256": "4623115ef6c710fd5a1aba5e79b9760698d9c3bf27caadc456b7624cef8a574f"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"has_sig": false,
"md5_digest": "7aa98cfbc2e384d10929e373259e60b7",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 640213,
"upload_time": "2024-10-13T20:49:50",
"upload_time_iso_8601": "2024-10-13T20:49:50.993679Z",
"url": "https://files.pythonhosted.org/packages/7b/00/ca41547bf8ea43b1f51f0c18ccc51dd4f2367f599ceccf588848eb44683d/pyxirr-0.10.6-cp310-cp310-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "fe9fad590332d66a098507e040a616333e99ac5e04080f3be61f85167bef598d",
"md5": "2e3ed0c4c71e6503bf5e4041ce488df2",
"sha256": "440b6349db7a3fdf3b2a5eaf12cf1ab0d553ad74eaef19b073d10869961d6a1f"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"has_sig": false,
"md5_digest": "2e3ed0c4c71e6503bf5e4041ce488df2",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 541021,
"upload_time": "2024-10-13T20:50:19",
"upload_time_iso_8601": "2024-10-13T20:50:19.888542Z",
"url": "https://files.pythonhosted.org/packages/fe/9f/ad590332d66a098507e040a616333e99ac5e04080f3be61f85167bef598d/pyxirr-0.10.6-cp310-cp310-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "eb611fbf452ad5ef6562a62249dd03a904812318f374652edba19319a8e68787",
"md5": "da907df800ac90835ed02340d2ed702a",
"sha256": "a474f9f5116f9ace9f9b344f06f4b629b3abf4caed3f106e81086e6083df5938"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "da907df800ac90835ed02340d2ed702a",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 885141,
"upload_time": "2024-10-13T20:50:08",
"upload_time_iso_8601": "2024-10-13T20:50:08.631831Z",
"url": "https://files.pythonhosted.org/packages/eb/61/1fbf452ad5ef6562a62249dd03a904812318f374652edba19319a8e68787/pyxirr-0.10.6-cp310-cp310-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f1d36244f012feb6d3e392a4c70fface1df1a9fda58408b0410e7069b3de8c1b",
"md5": "c411f69dc3a68e202e67e10083102258",
"sha256": "05ee52665fe1bebbd18075416d17ef22a4ff70d3ba0f09a8947c8636ea170bd3"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "c411f69dc3a68e202e67e10083102258",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 546699,
"upload_time": "2024-10-13T20:48:39",
"upload_time_iso_8601": "2024-10-13T20:48:39.400064Z",
"url": "https://files.pythonhosted.org/packages/f1/d3/6244f012feb6d3e392a4c70fface1df1a9fda58408b0410e7069b3de8c1b/pyxirr-0.10.6-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "877baf60e746a9416c4398238df4357d79bd7ee5485715bdae87f15eceb7d6c4",
"md5": "69ce0d0b938b6a575d89678f5d8f3b5c",
"sha256": "547caced83c91f4a96ea1c104da18891813e964b2e12845cbc503f46fb099883"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-musllinux_1_2_aarch64.whl",
"has_sig": false,
"md5_digest": "69ce0d0b938b6a575d89678f5d8f3b5c",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 682924,
"upload_time": "2024-10-13T20:48:24",
"upload_time_iso_8601": "2024-10-13T20:48:24.333063Z",
"url": "https://files.pythonhosted.org/packages/87/7b/af60e746a9416c4398238df4357d79bd7ee5485715bdae87f15eceb7d6c4/pyxirr-0.10.6-cp310-cp310-musllinux_1_2_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "52653e74aa13d7d282a151dd37d8e8221323c389c2a9c0c51034f8ef7270ff16",
"md5": "a6541a688239d507422cd88e1e07acf4",
"sha256": "4a39e7b8e4dbe07658472c5da7ba3f0b122fb4e77a4d635c41f59644e3e5ac6a"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-musllinux_1_2_armv7l.whl",
"has_sig": false,
"md5_digest": "a6541a688239d507422cd88e1e07acf4",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 756294,
"upload_time": "2024-10-13T20:48:47",
"upload_time_iso_8601": "2024-10-13T20:48:47.783785Z",
"url": "https://files.pythonhosted.org/packages/52/65/3e74aa13d7d282a151dd37d8e8221323c389c2a9c0c51034f8ef7270ff16/pyxirr-0.10.6-cp310-cp310-musllinux_1_2_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "ebe6f5bc90d41c6a891e13fa027956edd926c1ed3212a41371ed1e5154df0526",
"md5": "3b0ca7a42a27e8772926044f1f8d99cc",
"sha256": "b3862e7f2311f4d88b636bbd9db715176e16f1ca55c3c92b6ab04c17c497752a"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-musllinux_1_2_i686.whl",
"has_sig": false,
"md5_digest": "3b0ca7a42a27e8772926044f1f8d99cc",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 703929,
"upload_time": "2024-10-13T20:50:21",
"upload_time_iso_8601": "2024-10-13T20:50:21.122240Z",
"url": "https://files.pythonhosted.org/packages/eb/e6/f5bc90d41c6a891e13fa027956edd926c1ed3212a41371ed1e5154df0526/pyxirr-0.10.6-cp310-cp310-musllinux_1_2_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "6af5380528b65357dcc81260d1e0d6724d9a92787f8f939aacefa494ecfe866f",
"md5": "2a7f5ec2779cb81520b95ec4a4dfc28f",
"sha256": "581b50bead9c5b0d9f558d3bde5865a294480a3aa1e9533e02208ff681687f55"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-cp310-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "2a7f5ec2779cb81520b95ec4a4dfc28f",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 708002,
"upload_time": "2024-10-13T20:49:45",
"upload_time_iso_8601": "2024-10-13T20:49:45.162631Z",
"url": "https://files.pythonhosted.org/packages/6a/f5/380528b65357dcc81260d1e0d6724d9a92787f8f939aacefa494ecfe866f/pyxirr-0.10.6-cp310-cp310-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "4ea6aff4948725dcfe89017d675e2f3b45e766ddf981489b573118b1eb63afeb",
"md5": "fe99b0b892860a1d63270cceacd74ff8",
"sha256": "edb85743b3e6084a8e72b30c657efdf3a34c728b82cd2ec1618ad6187792ab3f"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp310-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "fe99b0b892860a1d63270cceacd74ff8",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.14,>=3.7",
"size": 448246,
"upload_time": "2024-10-13T20:50:14",
"upload_time_iso_8601": "2024-10-13T20:50:14.490383Z",
"url": "https://files.pythonhosted.org/packages/4e/a6/aff4948725dcfe89017d675e2f3b45e766ddf981489b573118b1eb63afeb/pyxirr-0.10.6-cp310-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "6e9f2d3aba71e763b03d2965dfe82970609eb035a9ca737caf3b17f53914f041",
"md5": "255948cb856e7f2a3904c274432eb1f2",
"sha256": "00c4cedf0925337cb98b6ea10ba6adf120d1578636852a01235c17ffc55cee23"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"has_sig": false,
"md5_digest": "255948cb856e7f2a3904c274432eb1f2",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 983466,
"upload_time": "2024-10-13T20:49:25",
"upload_time_iso_8601": "2024-10-13T20:49:25.005319Z",
"url": "https://files.pythonhosted.org/packages/6e/9f/2d3aba71e763b03d2965dfe82970609eb035a9ca737caf3b17f53914f041/pyxirr-0.10.6-cp311-cp311-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "fb6a11565c0049f2163dfe895182df79bbc13821f475a5c0fddd16ae21fa95ec",
"md5": "27c4fd2c7c213f5067410565d63ebaeb",
"sha256": "285a57e23885af4beb3a5a9b7dbff4c21bf27e294dacc66e9d0df2b11a6a9555"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "27c4fd2c7c213f5067410565d63ebaeb",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 473879,
"upload_time": "2024-10-13T20:49:34",
"upload_time_iso_8601": "2024-10-13T20:49:34.941522Z",
"url": "https://files.pythonhosted.org/packages/fb/6a/11565c0049f2163dfe895182df79bbc13821f475a5c0fddd16ae21fa95ec/pyxirr-0.10.6-cp311-cp311-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "06fdd9868ea9665e2eb06019df2957a5986b535bf5b992bd2bc6120036916c54",
"md5": "f443ef5c3ad299ba29f61885b4d54a2a",
"sha256": "636577b5f19499765dd93a95fd5be1ac684dec4ec59caf7e6e185543ea08fb2c"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "f443ef5c3ad299ba29f61885b4d54a2a",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 512266,
"upload_time": "2024-10-13T20:48:31",
"upload_time_iso_8601": "2024-10-13T20:48:31.302212Z",
"url": "https://files.pythonhosted.org/packages/06/fd/d9868ea9665e2eb06019df2957a5986b535bf5b992bd2bc6120036916c54/pyxirr-0.10.6-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "ffdf3915e8599c066e6c0b61c567c46442dda33d177c9ef06d56574e38f89a43",
"md5": "81147c464ae5597b9f9ee87303a9088b",
"sha256": "4413bfd84d5d82fba97027f4cee546f2cfd434b8aa625ae2f58fe1497f8a74e6"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"has_sig": false,
"md5_digest": "81147c464ae5597b9f9ee87303a9088b",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 508363,
"upload_time": "2024-10-13T20:48:28",
"upload_time_iso_8601": "2024-10-13T20:48:28.686329Z",
"url": "https://files.pythonhosted.org/packages/ff/df/3915e8599c066e6c0b61c567c46442dda33d177c9ef06d56574e38f89a43/pyxirr-0.10.6-cp311-cp311-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f5deadadc0d611b89fd031f85dde68fc1c8b940e06d2f00131efbca5b5eece4d",
"md5": "28ab7968693b629c455e0b073a39c6a7",
"sha256": "825c9007dfe7f7cd10dbf020d40528275df20707d5c9e4f661aef90392f961b8"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"has_sig": false,
"md5_digest": "28ab7968693b629c455e0b073a39c6a7",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 640285,
"upload_time": "2024-10-13T20:48:51",
"upload_time_iso_8601": "2024-10-13T20:48:51.859307Z",
"url": "https://files.pythonhosted.org/packages/f5/de/adadc0d611b89fd031f85dde68fc1c8b940e06d2f00131efbca5b5eece4d/pyxirr-0.10.6-cp311-cp311-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "cd9b7417f9a998cacbd010e94fd69bdf4274978b9a864f8531fec2d20d7c1c3b",
"md5": "1fb0dd894c99518b3c5a9a2d7d6f3244",
"sha256": "44f691906a570e7ba52152d7e2d58c973827c870c58cecf6f3bc638cd1568161"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"has_sig": false,
"md5_digest": "1fb0dd894c99518b3c5a9a2d7d6f3244",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 541020,
"upload_time": "2024-10-13T20:48:57",
"upload_time_iso_8601": "2024-10-13T20:48:57.955237Z",
"url": "https://files.pythonhosted.org/packages/cd/9b/7417f9a998cacbd010e94fd69bdf4274978b9a864f8531fec2d20d7c1c3b/pyxirr-0.10.6-cp311-cp311-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "bec114e98fbe48f13f3450d51c97f9fedf27270d170fdd0e3680679cac262b73",
"md5": "87b307453956d1be4bbcf80ad2f7fde4",
"sha256": "9d790fcd3370fcf70c4544de4db90ff35949197ba407a2bf40256bcb9c79a5ab"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "87b307453956d1be4bbcf80ad2f7fde4",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 885109,
"upload_time": "2024-10-13T20:49:13",
"upload_time_iso_8601": "2024-10-13T20:49:13.893821Z",
"url": "https://files.pythonhosted.org/packages/be/c1/14e98fbe48f13f3450d51c97f9fedf27270d170fdd0e3680679cac262b73/pyxirr-0.10.6-cp311-cp311-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "24a015df6fc0ce74d428d52c445553e76e7e3bc3732bb05d18b12f3fa2323cbc",
"md5": "80cfaae0ef22444d3ffc13032675f32b",
"sha256": "b627004b280df4159cf613ab21c9f8c7f26d05c7e449484c4377c11e1c39c794"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "80cfaae0ef22444d3ffc13032675f32b",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 546820,
"upload_time": "2024-10-13T20:49:36",
"upload_time_iso_8601": "2024-10-13T20:49:36.209576Z",
"url": "https://files.pythonhosted.org/packages/24/a0/15df6fc0ce74d428d52c445553e76e7e3bc3732bb05d18b12f3fa2323cbc/pyxirr-0.10.6-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "5ffaa976ebe09aaba79c9cdace1cff8c4d30d0206a4fa63937f1b3b6eaac76e8",
"md5": "f5296199213086ab2400dc1cadf833c2",
"sha256": "82c609c554ab369ef2c435e770f1d32e8e7e35bf78857d4c79e511f002f80004"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-musllinux_1_2_aarch64.whl",
"has_sig": false,
"md5_digest": "f5296199213086ab2400dc1cadf833c2",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 682955,
"upload_time": "2024-10-13T20:49:08",
"upload_time_iso_8601": "2024-10-13T20:49:08.379876Z",
"url": "https://files.pythonhosted.org/packages/5f/fa/a976ebe09aaba79c9cdace1cff8c4d30d0206a4fa63937f1b3b6eaac76e8/pyxirr-0.10.6-cp311-cp311-musllinux_1_2_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3462da811621ba0f83e66669d957d84c5bbb1e39f92b7bfd08df87c47e2ebef4",
"md5": "ffd77cc65797353a58584ebf08c46dde",
"sha256": "0357665310ec5b4740ef7cfa6e4780026f3d601dda4058ceef651d9ea72dc474"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-musllinux_1_2_armv7l.whl",
"has_sig": false,
"md5_digest": "ffd77cc65797353a58584ebf08c46dde",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 756366,
"upload_time": "2024-10-13T20:49:38",
"upload_time_iso_8601": "2024-10-13T20:49:38.712633Z",
"url": "https://files.pythonhosted.org/packages/34/62/da811621ba0f83e66669d957d84c5bbb1e39f92b7bfd08df87c47e2ebef4/pyxirr-0.10.6-cp311-cp311-musllinux_1_2_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "81ca19337447ba03f9132d1048071dddc557859e0c1eeb43103d3bc93db19c70",
"md5": "2662e0dfc649625b5c0fc405d70367c4",
"sha256": "782b0fd6e53a8c34e6a40472baff587a088121d14dd6aae5bb66ced22514e5cb"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-musllinux_1_2_i686.whl",
"has_sig": false,
"md5_digest": "2662e0dfc649625b5c0fc405d70367c4",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 703951,
"upload_time": "2024-10-13T20:48:32",
"upload_time_iso_8601": "2024-10-13T20:48:32.479671Z",
"url": "https://files.pythonhosted.org/packages/81/ca/19337447ba03f9132d1048071dddc557859e0c1eeb43103d3bc93db19c70/pyxirr-0.10.6-cp311-cp311-musllinux_1_2_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "1e7be6821fb5d09eba45c8e4565f348dd48c1c5719dae94a81fc6a2fe1b8165b",
"md5": "dddb2cd50f0a5116585270ad6413fce8",
"sha256": "7f170c8bcde7fa0b667968b961777b8f945c31f87a397ad291f6c470dcb140bf"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-cp311-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "dddb2cd50f0a5116585270ad6413fce8",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 708141,
"upload_time": "2024-10-13T20:48:53",
"upload_time_iso_8601": "2024-10-13T20:48:53.685563Z",
"url": "https://files.pythonhosted.org/packages/1e/7b/e6821fb5d09eba45c8e4565f348dd48c1c5719dae94a81fc6a2fe1b8165b/pyxirr-0.10.6-cp311-cp311-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3813835317dd3b9f0db60d6298474f24dd62168d90b610b02392871449a5a5ec",
"md5": "38a253d5cf828c859e2d3c7c9c754862",
"sha256": "a1326fcc5a2f279cc5aae5286d077c184ed150229036780986ea20c33e1f0f7c"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp311-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "38a253d5cf828c859e2d3c7c9c754862",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.14,>=3.7",
"size": 448277,
"upload_time": "2024-10-13T20:49:43",
"upload_time_iso_8601": "2024-10-13T20:49:43.919937Z",
"url": "https://files.pythonhosted.org/packages/38/13/835317dd3b9f0db60d6298474f24dd62168d90b610b02392871449a5a5ec/pyxirr-0.10.6-cp311-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3cc8641fcf451e2a45f411106d8dbe534a59fbd204ca5c72b1ede01964214d5c",
"md5": "5426f7ff5048f4f989e2b62cb033218d",
"sha256": "5315fcba78fba77cee652054c4e54221f3dc12706cacf14571c5c50d79245752"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"has_sig": false,
"md5_digest": "5426f7ff5048f4f989e2b62cb033218d",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 984070,
"upload_time": "2024-10-13T20:50:11",
"upload_time_iso_8601": "2024-10-13T20:50:11.586927Z",
"url": "https://files.pythonhosted.org/packages/3c/c8/641fcf451e2a45f411106d8dbe534a59fbd204ca5c72b1ede01964214d5c/pyxirr-0.10.6-cp312-cp312-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "0d2d5fd26ccd022546ac67862215cda79cd2a89675673ed0d1d8691e8a1a22da",
"md5": "a26868c31fda6e45074ae59b857014ff",
"sha256": "a7a723647326398f35d0068ed62a78f21a2ccbab0ff8833f8127a6e13d439946"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "a26868c31fda6e45074ae59b857014ff",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 474118,
"upload_time": "2024-10-13T20:49:59",
"upload_time_iso_8601": "2024-10-13T20:49:59.998144Z",
"url": "https://files.pythonhosted.org/packages/0d/2d/5fd26ccd022546ac67862215cda79cd2a89675673ed0d1d8691e8a1a22da/pyxirr-0.10.6-cp312-cp312-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f45a2a278c34c10dfad28bd3fff1baef9f05bc83231f2c3caf0bf81bb5c9ecaa",
"md5": "6aee78627a593ef892dbf806f328e251",
"sha256": "8b34f4e10bdc87ae33ddcd83dfff43707d67d24341b2f4ee9a31f0ac1bb220b6"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "6aee78627a593ef892dbf806f328e251",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 512612,
"upload_time": "2024-10-13T20:48:26",
"upload_time_iso_8601": "2024-10-13T20:48:26.038734Z",
"url": "https://files.pythonhosted.org/packages/f4/5a/2a278c34c10dfad28bd3fff1baef9f05bc83231f2c3caf0bf81bb5c9ecaa/pyxirr-0.10.6-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "670c16252ae2af1f2e49293ba7d2b89c848040df44107a823fcaca3f6f40784d",
"md5": "776e043f857a912d899204ac34508ed5",
"sha256": "ede65da069ba4560340dbb599ef827c8f3216beba8ce228870d0f26ca70aa38d"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"has_sig": false,
"md5_digest": "776e043f857a912d899204ac34508ed5",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 509017,
"upload_time": "2024-10-13T20:50:02",
"upload_time_iso_8601": "2024-10-13T20:50:02.821511Z",
"url": "https://files.pythonhosted.org/packages/67/0c/16252ae2af1f2e49293ba7d2b89c848040df44107a823fcaca3f6f40784d/pyxirr-0.10.6-cp312-cp312-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "95841d84432f5fbdebb362f401f78cbcd6885d17c425020a9c226690f8d67fff",
"md5": "b714845c308d2a49a3efe8f3590f5220",
"sha256": "ca127523dbf39d09eda08e724ca20627298fb01048301a0aa9d8841d3639e2a0"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"has_sig": false,
"md5_digest": "b714845c308d2a49a3efe8f3590f5220",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 640107,
"upload_time": "2024-10-13T20:49:53",
"upload_time_iso_8601": "2024-10-13T20:49:53.523068Z",
"url": "https://files.pythonhosted.org/packages/95/84/1d84432f5fbdebb362f401f78cbcd6885d17c425020a9c226690f8d67fff/pyxirr-0.10.6-cp312-cp312-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "ba40cea42d9eb752101a4e22ed8c4245e45e9b6ca90e39e45ad116adb9a11bbd",
"md5": "8ecb365462adadb6d84fa1fb0f608692",
"sha256": "4613554b41e24d465b7c1f136c84e884e2b227d816671ef702422aaf0d801ea8"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"has_sig": false,
"md5_digest": "8ecb365462adadb6d84fa1fb0f608692",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 540065,
"upload_time": "2024-10-13T20:48:42",
"upload_time_iso_8601": "2024-10-13T20:48:42.026886Z",
"url": "https://files.pythonhosted.org/packages/ba/40/cea42d9eb752101a4e22ed8c4245e45e9b6ca90e39e45ad116adb9a11bbd/pyxirr-0.10.6-cp312-cp312-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "fd93c4311aacc971b341000496c2ef901acc9708ac90e6afa56f2eb27b1055f3",
"md5": "14b0ed4d5a47c3d0996c351b18f08c50",
"sha256": "0b4bd3d7e111b7e91b53622e5d601deb22de9ef53d87a3c54a8e4551e9524054"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "14b0ed4d5a47c3d0996c351b18f08c50",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 883510,
"upload_time": "2024-10-13T20:49:58",
"upload_time_iso_8601": "2024-10-13T20:49:58.676904Z",
"url": "https://files.pythonhosted.org/packages/fd/93/c4311aacc971b341000496c2ef901acc9708ac90e6afa56f2eb27b1055f3/pyxirr-0.10.6-cp312-cp312-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "a290844265597a59df4c28088317a7a5a05bb5a37f41403b4adc828cb4612013",
"md5": "157ec2a5886a06569dfcb6254329fc65",
"sha256": "f7745cf488aa6a8daba2669b748d0f2712f3c257db4de71c20acf46fd6115b2f"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "157ec2a5886a06569dfcb6254329fc65",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 548026,
"upload_time": "2024-10-13T20:50:07",
"upload_time_iso_8601": "2024-10-13T20:50:07.416040Z",
"url": "https://files.pythonhosted.org/packages/a2/90/844265597a59df4c28088317a7a5a05bb5a37f41403b4adc828cb4612013/pyxirr-0.10.6-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "a86cbfe8405798977cce1eb026fc475c1a4de61bf73586d2788dbe8fd8d914a6",
"md5": "50328b556041e683ae3503277a52c64c",
"sha256": "75a5487c6944d89144699bef295c7e8f7e60423fb379069586ec05cc94318cda"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-musllinux_1_2_aarch64.whl",
"has_sig": false,
"md5_digest": "50328b556041e683ae3503277a52c64c",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 683253,
"upload_time": "2024-10-13T20:48:34",
"upload_time_iso_8601": "2024-10-13T20:48:34.994412Z",
"url": "https://files.pythonhosted.org/packages/a8/6c/bfe8405798977cce1eb026fc475c1a4de61bf73586d2788dbe8fd8d914a6/pyxirr-0.10.6-cp312-cp312-musllinux_1_2_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "e10c885ddaeeb9e838a1eb40ea3c5d99a3a25250f0794b83b7e2a9a368e6182c",
"md5": "8ed8b9b7d857c6a5e8ba9ccc425e799b",
"sha256": "706cd21905390362631add1ad8e87c48a5d68c6bc4251130e6a9e5013a10bfd6"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-musllinux_1_2_armv7l.whl",
"has_sig": false,
"md5_digest": "8ed8b9b7d857c6a5e8ba9ccc425e799b",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 756833,
"upload_time": "2024-10-13T20:49:16",
"upload_time_iso_8601": "2024-10-13T20:49:16.308729Z",
"url": "https://files.pythonhosted.org/packages/e1/0c/885ddaeeb9e838a1eb40ea3c5d99a3a25250f0794b83b7e2a9a368e6182c/pyxirr-0.10.6-cp312-cp312-musllinux_1_2_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "2ae5bc967d09edcef069c1837a8d18d7dc26ffd81e769053eb05cafa2ac2c46b",
"md5": "130ab1c76a127393ef6809b0a9eb0356",
"sha256": "ee2e1b1b3ea9793da6337e395f8c493c7a676fbc1a85b6d03773d91abbf34498"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-musllinux_1_2_i686.whl",
"has_sig": false,
"md5_digest": "130ab1c76a127393ef6809b0a9eb0356",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 704906,
"upload_time": "2024-10-13T20:48:15",
"upload_time_iso_8601": "2024-10-13T20:48:15.471537Z",
"url": "https://files.pythonhosted.org/packages/2a/e5/bc967d09edcef069c1837a8d18d7dc26ffd81e769053eb05cafa2ac2c46b/pyxirr-0.10.6-cp312-cp312-musllinux_1_2_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "440615b346c53e3f88ee77c55e4ef27cef6c495a8125169cfece71899b655fbe",
"md5": "9c24402fd40f293243750778b822619f",
"sha256": "0703ab5a7137aa221ecbe7bc5c9e0c99a370a625220750a973469b92c215fc01"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-cp312-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "9c24402fd40f293243750778b822619f",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 709045,
"upload_time": "2024-10-13T20:49:46",
"upload_time_iso_8601": "2024-10-13T20:49:46.736455Z",
"url": "https://files.pythonhosted.org/packages/44/06/15b346c53e3f88ee77c55e4ef27cef6c495a8125169cfece71899b655fbe/pyxirr-0.10.6-cp312-cp312-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "0fe528f889be53384e613a8f6ccff12bf03de9df99b3fab420758bdcb393f65a",
"md5": "c74dd15e039f3dc70f930ce5d9a73f7c",
"sha256": "d5bead5a69cade163f65bacfd28e1b304c19a6f7509565f124f3187ad13c017a"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp312-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "c74dd15e039f3dc70f930ce5d9a73f7c",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": "<3.14,>=3.7",
"size": 445210,
"upload_time": "2024-10-13T20:49:29",
"upload_time_iso_8601": "2024-10-13T20:49:29.996486Z",
"url": "https://files.pythonhosted.org/packages/0f/e5/28f889be53384e613a8f6ccff12bf03de9df99b3fab420758bdcb393f65a/pyxirr-0.10.6-cp312-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3810bddf5887c01a897364b2f9864d4ac43606d9e210301d1a4af250ac766870",
"md5": "77df2b5e30b93bb0ef108dfb4528b8a6",
"sha256": "9d79bc8563c16987c1382a6956061bf2802d70bf01537a2009a86969c1f3d800"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"has_sig": false,
"md5_digest": "77df2b5e30b93bb0ef108dfb4528b8a6",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 984072,
"upload_time": "2024-10-13T20:49:32",
"upload_time_iso_8601": "2024-10-13T20:49:32.423078Z",
"url": "https://files.pythonhosted.org/packages/38/10/bddf5887c01a897364b2f9864d4ac43606d9e210301d1a4af250ac766870/pyxirr-0.10.6-cp313-cp313-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "287e45cef0f13d6fa0125360c98e3160ee63aeb5074d2513c4d2f1e3e573d29a",
"md5": "272d905eacb82b68b5e60f6df134a69b",
"sha256": "ed4b7a89a8d06bc5c56aa08c9498ce0bd28c1350110c9cdefbc73a8c5a69cd8c"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "272d905eacb82b68b5e60f6df134a69b",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 474117,
"upload_time": "2024-10-13T20:49:57",
"upload_time_iso_8601": "2024-10-13T20:49:57.299478Z",
"url": "https://files.pythonhosted.org/packages/28/7e/45cef0f13d6fa0125360c98e3160ee63aeb5074d2513c4d2f1e3e573d29a/pyxirr-0.10.6-cp313-cp313-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "120030c807a6974a51d635d4dc5248e0fc9453a8b271e866f6c7591458d8edf7",
"md5": "ff5380ca3a79bd57c2a70e8873e53335",
"sha256": "536a534b4fc69dbeb9f30dbc11fc5d0806f3143483dc3bb5cdcc0275b7138e6e"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "ff5380ca3a79bd57c2a70e8873e53335",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 512613,
"upload_time": "2024-10-13T20:48:13",
"upload_time_iso_8601": "2024-10-13T20:48:13.971012Z",
"url": "https://files.pythonhosted.org/packages/12/00/30c807a6974a51d635d4dc5248e0fc9453a8b271e866f6c7591458d8edf7/pyxirr-0.10.6-cp313-cp313-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "90861438f093c6e733b0f89d63931742c87657528fc6e1c4a0b95556dcd074c4",
"md5": "0f5403e2504828a53bb8affc0a0e6137",
"sha256": "2b1390e8a5d5a5917b26916d715b24618493a50777042c306fe871e694a672ba"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"has_sig": false,
"md5_digest": "0f5403e2504828a53bb8affc0a0e6137",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 509017,
"upload_time": "2024-10-13T20:48:56",
"upload_time_iso_8601": "2024-10-13T20:48:56.324922Z",
"url": "https://files.pythonhosted.org/packages/90/86/1438f093c6e733b0f89d63931742c87657528fc6e1c4a0b95556dcd074c4/pyxirr-0.10.6-cp313-cp313-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "b1b8bde63b913341a26b0a8430ac224d7a3ea204dde80669d7ad5ff43306f3c1",
"md5": "d678eb84831a46578231ae9f6f1e4661",
"sha256": "6d2c72ad9495e01b99e086a1f2e7bf180858887df9344dbb62f59c6fe0fb2acc"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"has_sig": false,
"md5_digest": "d678eb84831a46578231ae9f6f1e4661",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 640108,
"upload_time": "2024-10-13T20:48:40",
"upload_time_iso_8601": "2024-10-13T20:48:40.903212Z",
"url": "https://files.pythonhosted.org/packages/b1/b8/bde63b913341a26b0a8430ac224d7a3ea204dde80669d7ad5ff43306f3c1/pyxirr-0.10.6-cp313-cp313-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "855cd83f43de51a087b108803666d12cdb8d5d57a6de30a52f26a536b9fbf0c6",
"md5": "b0408175cad0273265a232ca3071b2fa",
"sha256": "cbe16ba89b477a5f87140d2522c06c7d5c88d56a7d314beec3aea03238c15e74"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"has_sig": false,
"md5_digest": "b0408175cad0273265a232ca3071b2fa",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 540065,
"upload_time": "2024-10-13T20:48:33",
"upload_time_iso_8601": "2024-10-13T20:48:33.875584Z",
"url": "https://files.pythonhosted.org/packages/85/5c/d83f43de51a087b108803666d12cdb8d5d57a6de30a52f26a536b9fbf0c6/pyxirr-0.10.6-cp313-cp313-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "35eea255c33c8c6eb08ee9ec7447b2a8d44b957cf26f94416be68ce2cd17c693",
"md5": "4e88ee743d8da07a1fb021b93c6d6318",
"sha256": "7488d9c711cb83990ec96270d142be78e85ad726af8ed5f39906d80671ed1cdf"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "4e88ee743d8da07a1fb021b93c6d6318",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 883510,
"upload_time": "2024-10-13T20:49:27",
"upload_time_iso_8601": "2024-10-13T20:49:27.561286Z",
"url": "https://files.pythonhosted.org/packages/35/ee/a255c33c8c6eb08ee9ec7447b2a8d44b957cf26f94416be68ce2cd17c693/pyxirr-0.10.6-cp313-cp313-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "56df494df5b576485d8ced1c618aa8ca0695f8ef15fd48b25fb824814c78533f",
"md5": "e1586f66811d430f37f52acda507e92a",
"sha256": "10bf67d176720ffc78ce182fd70e5c712a002d4fcf799f6e53d1f31e24bd6743"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "e1586f66811d430f37f52acda507e92a",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 548025,
"upload_time": "2024-10-13T20:49:11",
"upload_time_iso_8601": "2024-10-13T20:49:11.405917Z",
"url": "https://files.pythonhosted.org/packages/56/df/494df5b576485d8ced1c618aa8ca0695f8ef15fd48b25fb824814c78533f/pyxirr-0.10.6-cp313-cp313-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "306ee105813911224dcc822d75cc0b05fd399c42822793d486464278013d3ccf",
"md5": "43fdd1bcbcce3d377abe821b41513953",
"sha256": "179a7fe7da0692f3668c9e230a7159e69475858197965c612c7519a99ae61669"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-musllinux_1_2_aarch64.whl",
"has_sig": false,
"md5_digest": "43fdd1bcbcce3d377abe821b41513953",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 683253,
"upload_time": "2024-10-13T20:49:41",
"upload_time_iso_8601": "2024-10-13T20:49:41.220849Z",
"url": "https://files.pythonhosted.org/packages/30/6e/e105813911224dcc822d75cc0b05fd399c42822793d486464278013d3ccf/pyxirr-0.10.6-cp313-cp313-musllinux_1_2_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "fcac79d09ac2e82341c940d034e8c812c906e09f4798b3bd5d2013cafedf7cfd",
"md5": "1ae2265f324d14307a307ee4dc469ac5",
"sha256": "40ce8fb1bcf57941854580d849cfc261d70b075fe555a1211c5f484e7a659312"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-musllinux_1_2_armv7l.whl",
"has_sig": false,
"md5_digest": "1ae2265f324d14307a307ee4dc469ac5",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 756833,
"upload_time": "2024-10-13T20:48:37",
"upload_time_iso_8601": "2024-10-13T20:48:37.822358Z",
"url": "https://files.pythonhosted.org/packages/fc/ac/79d09ac2e82341c940d034e8c812c906e09f4798b3bd5d2013cafedf7cfd/pyxirr-0.10.6-cp313-cp313-musllinux_1_2_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "d91313541a35a43e93e37a65c5f4f647f0e221be9ec41ac5b7e546595e2cb747",
"md5": "cc4016a75dcd8de0c0dffa6385dfcef2",
"sha256": "a4b7d7dd7b65e56268b55e56598d53c6c6a624f2adaca3665ce76533edec92e1"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-musllinux_1_2_i686.whl",
"has_sig": false,
"md5_digest": "cc4016a75dcd8de0c0dffa6385dfcef2",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 704905,
"upload_time": "2024-10-13T20:48:54",
"upload_time_iso_8601": "2024-10-13T20:48:54.853800Z",
"url": "https://files.pythonhosted.org/packages/d9/13/13541a35a43e93e37a65c5f4f647f0e221be9ec41ac5b7e546595e2cb747/pyxirr-0.10.6-cp313-cp313-musllinux_1_2_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "6d2a79541be5d4d7febb886f47192d84ad29212e424d5a86ed51a1d0cdc02ec4",
"md5": "5399d63e9abad2d2d199406e1caa32c8",
"sha256": "2c77f392139a871feb723a58a49ca028b0b623c4429ae987d74d5068a8d0f2ff"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-cp313-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "5399d63e9abad2d2d199406e1caa32c8",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 709045,
"upload_time": "2024-10-13T20:49:54",
"upload_time_iso_8601": "2024-10-13T20:49:54.764649Z",
"url": "https://files.pythonhosted.org/packages/6d/2a/79541be5d4d7febb886f47192d84ad29212e424d5a86ed51a1d0cdc02ec4/pyxirr-0.10.6-cp313-cp313-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "afc569f576c6f97e598a8c553a8f1e843355d81aa910cfa77a15e09a5ca9cac0",
"md5": "d856cae8b481f265644bed1207465ac6",
"sha256": "7828cfed1dd81a663a4b3075dadd8bd691afa8ac969afb30ed6f5346de02bafa"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp313-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "d856cae8b481f265644bed1207465ac6",
"packagetype": "bdist_wheel",
"python_version": "cp313",
"requires_python": "<3.14,>=3.7",
"size": 445212,
"upload_time": "2024-10-13T20:49:31",
"upload_time_iso_8601": "2024-10-13T20:49:31.221136Z",
"url": "https://files.pythonhosted.org/packages/af/c5/69f576c6f97e598a8c553a8f1e843355d81aa910cfa77a15e09a5ca9cac0/pyxirr-0.10.6-cp313-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3642338b3b1e51365a19d0e1cbd74840da915a40fc3c04dc8c8aa736331cdb32",
"md5": "e6ac04e76c7a9f0e5e6a0ffaa946c17a",
"sha256": "463c9f23e360b14a440f36c010c78076cb93e052a6fe33f37023ac89d4e434ee"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"has_sig": false,
"md5_digest": "e6ac04e76c7a9f0e5e6a0ffaa946c17a",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 984581,
"upload_time": "2024-10-13T20:48:46",
"upload_time_iso_8601": "2024-10-13T20:48:46.178593Z",
"url": "https://files.pythonhosted.org/packages/36/42/338b3b1e51365a19d0e1cbd74840da915a40fc3c04dc8c8aa736331cdb32/pyxirr-0.10.6-cp37-cp37m-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f0d5b2d68e9c1248f472c29acd3e4dee65f2b202bcca64d91d5856a793c84fc8",
"md5": "30b66b788a02d8637687e6c440dff3cc",
"sha256": "06f6b494c629812e123500d24ef49ae43cbbc6abb4c7204ba60243db2a24861c"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "30b66b788a02d8637687e6c440dff3cc",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 474441,
"upload_time": "2024-10-13T20:48:30",
"upload_time_iso_8601": "2024-10-13T20:48:30.170322Z",
"url": "https://files.pythonhosted.org/packages/f0/d5/b2d68e9c1248f472c29acd3e4dee65f2b202bcca64d91d5856a793c84fc8/pyxirr-0.10.6-cp37-cp37m-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "607a0c3d6972520d3eeaa417c0f42cb86e1341bee8ea17359c095cfc7e83944a",
"md5": "2517bac6c803fa12b1a7dfa6d1e33fb9",
"sha256": "1dd38c75386ff65ab7ae70e4e6064e7960bd12e5001f4e4657579ac2cdfd584b"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "2517bac6c803fa12b1a7dfa6d1e33fb9",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 513028,
"upload_time": "2024-10-13T20:49:19",
"upload_time_iso_8601": "2024-10-13T20:49:19.895729Z",
"url": "https://files.pythonhosted.org/packages/60/7a/0c3d6972520d3eeaa417c0f42cb86e1341bee8ea17359c095cfc7e83944a/pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "8fc3ada929d6eb38cfc942dfe231e65894f66f84dcb45bc77a0939c0453811df",
"md5": "eb0fa53513f51adce5c69a9cda561f2c",
"sha256": "fe9621056d9dd169515d2bc7194d911c3157b3101eb64636f5e1714ea2f3a02a"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"has_sig": false,
"md5_digest": "eb0fa53513f51adce5c69a9cda561f2c",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 509162,
"upload_time": "2024-10-13T20:50:17",
"upload_time_iso_8601": "2024-10-13T20:50:17.354934Z",
"url": "https://files.pythonhosted.org/packages/8f/c3/ada929d6eb38cfc942dfe231e65894f66f84dcb45bc77a0939c0453811df/pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "ab6f2acd0e4931b4cbc754181b8d56365ee5188630cb6bb91e068571ac721c0c",
"md5": "e24263eccb81c12a5a90aaebf76b39c2",
"sha256": "d0168cc0f814c440f5ebf10ff5fcd886dc36ca781321fc4d484a1b02b847da0b"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"has_sig": false,
"md5_digest": "e24263eccb81c12a5a90aaebf76b39c2",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 640967,
"upload_time": "2024-10-13T20:50:18",
"upload_time_iso_8601": "2024-10-13T20:50:18.645935Z",
"url": "https://files.pythonhosted.org/packages/ab/6f/2acd0e4931b4cbc754181b8d56365ee5188630cb6bb91e068571ac721c0c/pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "bc024f386f37ea558d317d4d0327cbaefe0bac8da11973100ba7553fc5319f41",
"md5": "32bfcae9c19660356a8320027c7d72ed",
"sha256": "83834a5c11b030ee69f430dfe1027d50e176dcfffdf6ef54364463b320a8be90"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"has_sig": false,
"md5_digest": "32bfcae9c19660356a8320027c7d72ed",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 542024,
"upload_time": "2024-10-13T20:48:59",
"upload_time_iso_8601": "2024-10-13T20:48:59.112974Z",
"url": "https://files.pythonhosted.org/packages/bc/02/4f386f37ea558d317d4d0327cbaefe0bac8da11973100ba7553fc5319f41/pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "8f73e34157972317ae07d7cb2c2853a97a189e9afc4c3cbcec3ea9fb439e4f7c",
"md5": "f6b414a7aa915a11ca93ac4b4e6b5dfb",
"sha256": "8a0855624b9d0b7e9a08168c0f7091683c28b6b89ef74df1af604ae89ab6d256"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "f6b414a7aa915a11ca93ac4b4e6b5dfb",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 885790,
"upload_time": "2024-10-13T20:48:36",
"upload_time_iso_8601": "2024-10-13T20:48:36.337622Z",
"url": "https://files.pythonhosted.org/packages/8f/73/e34157972317ae07d7cb2c2853a97a189e9afc4c3cbcec3ea9fb439e4f7c/pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "399bd0d2ff6c42cc7bb223703905ffdee066af454e9af00bc434233627170c09",
"md5": "8a038bf1f37b81479478931ef77bd89a",
"sha256": "1e9105bb7d4826f724c9245e99725bb21a43ca9033103399d3123fb76e542219"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "8a038bf1f37b81479478931ef77bd89a",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 547261,
"upload_time": "2024-10-13T20:50:04",
"upload_time_iso_8601": "2024-10-13T20:50:04.725852Z",
"url": "https://files.pythonhosted.org/packages/39/9b/d0d2ff6c42cc7bb223703905ffdee066af454e9af00bc434233627170c09/pyxirr-0.10.6-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "dcad4d2403e6f3e4ee40cb90103dee8284111eec49fc836e2f3ca792b22a8bd5",
"md5": "087b4526b30342656ccf5e6a53b31d1d",
"sha256": "04b7c20481ac017044fdf80d893588e55dc6ea26e31ec4ac2a95b9388ba45bd9"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-musllinux_1_2_aarch64.whl",
"has_sig": false,
"md5_digest": "087b4526b30342656ccf5e6a53b31d1d",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 683415,
"upload_time": "2024-10-13T20:48:43",
"upload_time_iso_8601": "2024-10-13T20:48:43.559442Z",
"url": "https://files.pythonhosted.org/packages/dc/ad/4d2403e6f3e4ee40cb90103dee8284111eec49fc836e2f3ca792b22a8bd5/pyxirr-0.10.6-cp37-cp37m-musllinux_1_2_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "fa9e5c538165e7b01157f1efaec658136f6e35df1192a8cd5145585d9aad36c6",
"md5": "cc88eb7b4e9ad1e4577d5ee6cad81770",
"sha256": "d8989e544169c833ed180d9792a10af1a806ccea4133b61f5f9d0d350dec14b9"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-musllinux_1_2_armv7l.whl",
"has_sig": false,
"md5_digest": "cc88eb7b4e9ad1e4577d5ee6cad81770",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 756837,
"upload_time": "2024-10-13T20:50:16",
"upload_time_iso_8601": "2024-10-13T20:50:16.044950Z",
"url": "https://files.pythonhosted.org/packages/fa/9e/5c538165e7b01157f1efaec658136f6e35df1192a8cd5145585d9aad36c6/pyxirr-0.10.6-cp37-cp37m-musllinux_1_2_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "062729fe5b04cc8ac9f788aad4f4d9018c43c664def6b763c073542c9c108230",
"md5": "9b0ecb2aeb11ad20a5c9ea4cdb6182e5",
"sha256": "483063048c15f6aa408db7aecd8b41e58fa77ab82bc9b14d7285cd77214d7882"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-musllinux_1_2_i686.whl",
"has_sig": false,
"md5_digest": "9b0ecb2aeb11ad20a5c9ea4cdb6182e5",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 704581,
"upload_time": "2024-10-13T20:48:50",
"upload_time_iso_8601": "2024-10-13T20:48:50.111506Z",
"url": "https://files.pythonhosted.org/packages/06/27/29fe5b04cc8ac9f788aad4f4d9018c43c664def6b763c073542c9c108230/pyxirr-0.10.6-cp37-cp37m-musllinux_1_2_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "92551184f193b1a21be5380cf67c6595514e022b9c9dd57c41dd1a20f134a995",
"md5": "faef9929f3aca61e643842eb777a2ca6",
"sha256": "0fdf2a339048d05e145e68e3b0921a9d0dd60a472b9225e848ee7f7f78c838f2"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-cp37m-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "faef9929f3aca61e643842eb777a2ca6",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 708749,
"upload_time": "2024-10-13T20:49:17",
"upload_time_iso_8601": "2024-10-13T20:49:17.500585Z",
"url": "https://files.pythonhosted.org/packages/92/55/1184f193b1a21be5380cf67c6595514e022b9c9dd57c41dd1a20f134a995/pyxirr-0.10.6-cp37-cp37m-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "720c57a10ed87a440ebfa582daf066ce525493dc14874ce4f0048ee8f3be3ec6",
"md5": "a1cfd7c1b3c6dca840e9743aefafc56f",
"sha256": "2a96e17650debf7651f3ba6b87445d9f05198cf96f7a28258e5bed751729ceaa"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp37-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "a1cfd7c1b3c6dca840e9743aefafc56f",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": "<3.14,>=3.7",
"size": 448648,
"upload_time": "2024-10-13T20:50:10",
"upload_time_iso_8601": "2024-10-13T20:50:10.341360Z",
"url": "https://files.pythonhosted.org/packages/72/0c/57a10ed87a440ebfa582daf066ce525493dc14874ce4f0048ee8f3be3ec6/pyxirr-0.10.6-cp37-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "21c324d562ceb89d0f5e696154d7b51c64459d17f443b19eeed30c53cd2d7472",
"md5": "2fddd4cd3843bd1ae7174ec6c88f95e7",
"sha256": "a839849ebb2d621e8d1d8073d91871e5317ea4dfcce9fb8de24b474f979b9b66"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"has_sig": false,
"md5_digest": "2fddd4cd3843bd1ae7174ec6c88f95e7",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 984443,
"upload_time": "2024-10-13T20:49:39",
"upload_time_iso_8601": "2024-10-13T20:49:39.966201Z",
"url": "https://files.pythonhosted.org/packages/21/c3/24d562ceb89d0f5e696154d7b51c64459d17f443b19eeed30c53cd2d7472/pyxirr-0.10.6-cp38-cp38-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "a1b45141d374279c2145e324214f282f3de33a0b76d19bb2d217a47e72a0fb1b",
"md5": "5f15abe5ed7cb7504728cc52d52941c1",
"sha256": "9022970aeac0110d8997b6c3b99d7c83e5173c377edab50f4a150f5715dc2607"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "5f15abe5ed7cb7504728cc52d52941c1",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 474294,
"upload_time": "2024-10-13T20:49:52",
"upload_time_iso_8601": "2024-10-13T20:49:52.316726Z",
"url": "https://files.pythonhosted.org/packages/a1/b4/5141d374279c2145e324214f282f3de33a0b76d19bb2d217a47e72a0fb1b/pyxirr-0.10.6-cp38-cp38-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "e710e28c39b6eefcf5b3260c6181de8e30f3b43685790c9fff9010105cbc626d",
"md5": "bb41e81d00254e067b666975abc40787",
"sha256": "c3268d2b5cdc4ca8ea8d3723408ca1558afd820ee58de774e13204f34ee6ec75"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "bb41e81d00254e067b666975abc40787",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 512918,
"upload_time": "2024-10-13T20:49:22",
"upload_time_iso_8601": "2024-10-13T20:49:22.552881Z",
"url": "https://files.pythonhosted.org/packages/e7/10/e28c39b6eefcf5b3260c6181de8e30f3b43685790c9fff9010105cbc626d/pyxirr-0.10.6-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "ff4cc56f159dc6090401d4f7c6913d583bb6f0d9ecf02c3cea0709fff97e5433",
"md5": "0a86312248dd26f555640559c8a35832",
"sha256": "6e3d836b0f3e781776a36625c74a8d071b58238069beaccc690ea44b96c9ed6c"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"has_sig": false,
"md5_digest": "0a86312248dd26f555640559c8a35832",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 509158,
"upload_time": "2024-10-13T20:49:42",
"upload_time_iso_8601": "2024-10-13T20:49:42.742850Z",
"url": "https://files.pythonhosted.org/packages/ff/4c/c56f159dc6090401d4f7c6913d583bb6f0d9ecf02c3cea0709fff97e5433/pyxirr-0.10.6-cp38-cp38-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f00439f27ce32270c157d9a53149bed464136c91da617e7d3da865b17be4dad9",
"md5": "490994488bc962e1c11d41f5a9929593",
"sha256": "3f9ebc01883994d932b24a2717f6497ce7e922348e4f040d135541dd6c783298"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"has_sig": false,
"md5_digest": "490994488bc962e1c11d41f5a9929593",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 640887,
"upload_time": "2024-10-13T20:48:12",
"upload_time_iso_8601": "2024-10-13T20:48:12.531441Z",
"url": "https://files.pythonhosted.org/packages/f0/04/39f27ce32270c157d9a53149bed464136c91da617e7d3da865b17be4dad9/pyxirr-0.10.6-cp38-cp38-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "b9da0612470a7bd8d947609282ce261344d390bd1468f482f0d84c9232941e25",
"md5": "c362041ad8d218d4e21e8bbb8abc2cd5",
"sha256": "6093c8b201d43aafe7aa3fa8077d19aa00cfff173a21da84ad27a5c4efd1166f"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"has_sig": false,
"md5_digest": "c362041ad8d218d4e21e8bbb8abc2cd5",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 541986,
"upload_time": "2024-10-13T20:48:48",
"upload_time_iso_8601": "2024-10-13T20:48:48.928261Z",
"url": "https://files.pythonhosted.org/packages/b9/da/0612470a7bd8d947609282ce261344d390bd1468f482f0d84c9232941e25/pyxirr-0.10.6-cp38-cp38-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "ef74a694965303f768f557667eb106a626dd538cc10d0922286ed06cb9fa9bda",
"md5": "e23513c51fd6ebb4832607be25c5ea1e",
"sha256": "f4f0cafa8d02eeb0237c741f590c83221c2bde23fdbac1107f987d8f80c9d7e1"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "e23513c51fd6ebb4832607be25c5ea1e",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 885844,
"upload_time": "2024-10-13T20:49:09",
"upload_time_iso_8601": "2024-10-13T20:49:09.786926Z",
"url": "https://files.pythonhosted.org/packages/ef/74/a694965303f768f557667eb106a626dd538cc10d0922286ed06cb9fa9bda/pyxirr-0.10.6-cp38-cp38-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "4ec9d68dc301fe993215754ee5ab0c96cdc4db28d2b5a3550090cac40b6e5b1f",
"md5": "4dba4ca2cc86174cbb69e2d0404d7162",
"sha256": "8c229140d9efe2e8563a7d3b9d29d0691e7aeb2b6a66ba50072894597db9f708"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "4dba4ca2cc86174cbb69e2d0404d7162",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 547328,
"upload_time": "2024-10-13T20:48:27",
"upload_time_iso_8601": "2024-10-13T20:48:27.202997Z",
"url": "https://files.pythonhosted.org/packages/4e/c9/d68dc301fe993215754ee5ab0c96cdc4db28d2b5a3550090cac40b6e5b1f/pyxirr-0.10.6-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "5eace5002c9a2be03ffe26d96e3f1c6e42b1c0f6e3ab08606c1aa8862d279f84",
"md5": "1e79d7ab97ba9f5d6b3778e3b09873ca",
"sha256": "3e75a56cdc25caf80b6263db151cfb3fa2d3123c34bcfec87f220875a2207744"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-musllinux_1_2_aarch64.whl",
"has_sig": false,
"md5_digest": "1e79d7ab97ba9f5d6b3778e3b09873ca",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 683428,
"upload_time": "2024-10-13T20:49:15",
"upload_time_iso_8601": "2024-10-13T20:49:15.094600Z",
"url": "https://files.pythonhosted.org/packages/5e/ac/e5002c9a2be03ffe26d96e3f1c6e42b1c0f6e3ab08606c1aa8862d279f84/pyxirr-0.10.6-cp38-cp38-musllinux_1_2_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "977ab7c6b2837ba61d2f1f1c514c840de44c10e0482d5149f306ae4a5243b718",
"md5": "921bcca0a26e2c8910355f94361ea5a6",
"sha256": "9869e3bf7bf22dc0a49efc9de78d9f39ab9a5636025e3953812846867a3e8c85"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-musllinux_1_2_armv7l.whl",
"has_sig": false,
"md5_digest": "921bcca0a26e2c8910355f94361ea5a6",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 756770,
"upload_time": "2024-10-13T20:48:21",
"upload_time_iso_8601": "2024-10-13T20:48:21.019742Z",
"url": "https://files.pythonhosted.org/packages/97/7a/b7c6b2837ba61d2f1f1c514c840de44c10e0482d5149f306ae4a5243b718/pyxirr-0.10.6-cp38-cp38-musllinux_1_2_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "796fc556769bc4d02dd0f3b3ce73a5b95725158123c9b5944b4248e46da86ede",
"md5": "705a9bda58e584558d7e37e87c9a5c37",
"sha256": "51bbd6b61c07638a6278860374c74850fa92a46d3bba7012af1e94756188e63e"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-musllinux_1_2_i686.whl",
"has_sig": false,
"md5_digest": "705a9bda58e584558d7e37e87c9a5c37",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 704475,
"upload_time": "2024-10-13T20:48:19",
"upload_time_iso_8601": "2024-10-13T20:48:19.520707Z",
"url": "https://files.pythonhosted.org/packages/79/6f/c556769bc4d02dd0f3b3ce73a5b95725158123c9b5944b4248e46da86ede/pyxirr-0.10.6-cp38-cp38-musllinux_1_2_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "6ff9975b40d768004f9eef33dc7fb996c1b6f3960ea8306f166845409cab9416",
"md5": "51f2e11b366981cf8f829538f7d9787c",
"sha256": "ec1e5a8ebb8de7adaab6907fc6bc237cf588c4fae32611e7aa0df6136ac09cac"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-cp38-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "51f2e11b366981cf8f829538f7d9787c",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 708787,
"upload_time": "2024-10-13T20:49:33",
"upload_time_iso_8601": "2024-10-13T20:49:33.687752Z",
"url": "https://files.pythonhosted.org/packages/6f/f9/975b40d768004f9eef33dc7fb996c1b6f3960ea8306f166845409cab9416/pyxirr-0.10.6-cp38-cp38-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "75e1b62548782436ee75a6a8c27c85ca13cc7931d831e3be17b0ec0cf29a0c3a",
"md5": "ee630c70a409087a3c9bbb2fa037a5d5",
"sha256": "11926c16bee9ad22b5a3c1f8c26641a0ecb2957c5353575be69dbb96f09edf0c"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp38-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "ee630c70a409087a3c9bbb2fa037a5d5",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.14,>=3.7",
"size": 448715,
"upload_time": "2024-10-13T20:49:18",
"upload_time_iso_8601": "2024-10-13T20:49:18.750602Z",
"url": "https://files.pythonhosted.org/packages/75/e1/b62548782436ee75a6a8c27c85ca13cc7931d831e3be17b0ec0cf29a0c3a/pyxirr-0.10.6-cp38-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "99121a2466776705c8132e567bf4a6ca775dc7ae55fea953b07474748a95568f",
"md5": "79bbc56f3f4143b64d447a98e41dc47e",
"sha256": "a04aab4b73d239a6fbdec647b33b09f182d40b510d89c98ff86159938aa758e7"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"has_sig": false,
"md5_digest": "79bbc56f3f4143b64d447a98e41dc47e",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 983994,
"upload_time": "2024-10-13T20:48:17",
"upload_time_iso_8601": "2024-10-13T20:48:17.730616Z",
"url": "https://files.pythonhosted.org/packages/99/12/1a2466776705c8132e567bf4a6ca775dc7ae55fea953b07474748a95568f/pyxirr-0.10.6-cp39-cp39-macosx_10_12_x86_64.macosx_11_0_arm64.macosx_10_12_universal2.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3682cb1230aace926cc91eec64a4a7add37152039568009bb8de7de8e13558b4",
"md5": "c62142f7b457437af6d0508e3060a0cf",
"sha256": "62fb161b9df2ee245d70597957265198a310c3dedf0c5892943d5441e2561a42"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "c62142f7b457437af6d0508e3060a0cf",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 474151,
"upload_time": "2024-10-13T20:48:10",
"upload_time_iso_8601": "2024-10-13T20:48:10.352563Z",
"url": "https://files.pythonhosted.org/packages/36/82/cb1230aace926cc91eec64a4a7add37152039568009bb8de7de8e13558b4/pyxirr-0.10.6-cp39-cp39-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "130693feb00f15e8ed8ab1cad9e067b7de136d4c9fad1432a3060e0b1b01efbc",
"md5": "3bdbb6b433bcc027340f737e3dfd1d73",
"sha256": "b02489336ae789c481bb112b1d7bcdd314149440c20ec0288c40e36840bc99fe"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "3bdbb6b433bcc027340f737e3dfd1d73",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 512647,
"upload_time": "2024-10-13T20:50:01",
"upload_time_iso_8601": "2024-10-13T20:50:01.589296Z",
"url": "https://files.pythonhosted.org/packages/13/06/93feb00f15e8ed8ab1cad9e067b7de136d4c9fad1432a3060e0b1b01efbc/pyxirr-0.10.6-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "5a4bcb263549247f4307190a661e26d91cb6ac2403454c6b2e0505cf19da7a37",
"md5": "8b17ba4231d996d24859a4adf543f2e6",
"sha256": "551e7d020bf4936390f99718f051586c48e46d4b8c19190f7ef1dfa41c2bb62b"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"has_sig": false,
"md5_digest": "8b17ba4231d996d24859a4adf543f2e6",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 508786,
"upload_time": "2024-10-13T20:50:22",
"upload_time_iso_8601": "2024-10-13T20:50:22.811515Z",
"url": "https://files.pythonhosted.org/packages/5a/4b/cb263549247f4307190a661e26d91cb6ac2403454c6b2e0505cf19da7a37/pyxirr-0.10.6-cp39-cp39-manylinux_2_17_armv7l.manylinux2014_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "2bb37fc4cc32658e6285eeb37812252c0d72a29e0f8efeac310603f0dd34c407",
"md5": "d3c5744ab13f5c5edf61ee8c71398bd0",
"sha256": "d8c0add86800977d934d316a63f65a28992b77a72371273b8db5bcd2e5cf6b36"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"has_sig": false,
"md5_digest": "d3c5744ab13f5c5edf61ee8c71398bd0",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 640606,
"upload_time": "2024-10-13T20:49:21",
"upload_time_iso_8601": "2024-10-13T20:49:21.116352Z",
"url": "https://files.pythonhosted.org/packages/2b/b3/7fc4cc32658e6285eeb37812252c0d72a29e0f8efeac310603f0dd34c407/pyxirr-0.10.6-cp39-cp39-manylinux_2_17_ppc64le.manylinux2014_ppc64le.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "77ba5b07d121af20e358556b6bacd07ccf10113dac8779675852c6d032d9031d",
"md5": "5a9a83af00414078ad1f5cd6549f34a9",
"sha256": "64588a06ef2f1c54608b84e4211e27a7a8ad4530004f30cb71745e50cda4dc78"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"has_sig": false,
"md5_digest": "5a9a83af00414078ad1f5cd6549f34a9",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 541736,
"upload_time": "2024-10-13T20:49:28",
"upload_time_iso_8601": "2024-10-13T20:49:28.806806Z",
"url": "https://files.pythonhosted.org/packages/77/ba/5b07d121af20e358556b6bacd07ccf10113dac8779675852c6d032d9031d/pyxirr-0.10.6-cp39-cp39-manylinux_2_17_ppc64.manylinux2014_ppc64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "80e6801ae7fc1af601b18fe5977a748146608738259d9790b8c41482788fbf8a",
"md5": "9de0fa14be741ba1e320846346e82804",
"sha256": "6da486fece2b4d6c744ae33de0c9a236e7b663349567e4d54e28b4385a3136b0"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "9de0fa14be741ba1e320846346e82804",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 885458,
"upload_time": "2024-10-13T20:49:12",
"upload_time_iso_8601": "2024-10-13T20:49:12.643145Z",
"url": "https://files.pythonhosted.org/packages/80/e6/801ae7fc1af601b18fe5977a748146608738259d9790b8c41482788fbf8a/pyxirr-0.10.6-cp39-cp39-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "28ba271b2ef1b85f69c09352340108448d4bf40f93d8876ac55405b4fc0b0d0e",
"md5": "8ce321609febed4f74d1a0898f7543cd",
"sha256": "0c2efa5a03fac27cb6d58965afa5ca4c8ad9e65d26cb438c8cedc0991c1e5c29"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "8ce321609febed4f74d1a0898f7543cd",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 547005,
"upload_time": "2024-10-13T20:49:06",
"upload_time_iso_8601": "2024-10-13T20:49:06.815951Z",
"url": "https://files.pythonhosted.org/packages/28/ba/271b2ef1b85f69c09352340108448d4bf40f93d8876ac55405b4fc0b0d0e/pyxirr-0.10.6-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "8494d78a591afd47dbe0d0b7bb917ff65f261c7a55894f097f3d2613f57ca67d",
"md5": "502aa3491e5e6df12e5c4b99beefaa96",
"sha256": "34f5a6e79d91af6f36b9f0c2cc79b23b476d1a2451803800c11a80162caa77ae"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-musllinux_1_2_aarch64.whl",
"has_sig": false,
"md5_digest": "502aa3491e5e6df12e5c4b99beefaa96",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 683244,
"upload_time": "2024-10-13T20:48:22",
"upload_time_iso_8601": "2024-10-13T20:48:22.506459Z",
"url": "https://files.pythonhosted.org/packages/84/94/d78a591afd47dbe0d0b7bb917ff65f261c7a55894f097f3d2613f57ca67d/pyxirr-0.10.6-cp39-cp39-musllinux_1_2_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f799ce52d51006264dedfa53b999da7a4a5315be6184fe119aec012de85abeb2",
"md5": "3a5c3503218a115c95a0ccf85e2a1e33",
"sha256": "b999dee3cefadc4b9d64a14f9aacd5541dc0a4b1a480fe9f03bd996303518852"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-musllinux_1_2_armv7l.whl",
"has_sig": false,
"md5_digest": "3a5c3503218a115c95a0ccf85e2a1e33",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 756613,
"upload_time": "2024-10-13T20:48:44",
"upload_time_iso_8601": "2024-10-13T20:48:44.793929Z",
"url": "https://files.pythonhosted.org/packages/f7/99/ce52d51006264dedfa53b999da7a4a5315be6184fe119aec012de85abeb2/pyxirr-0.10.6-cp39-cp39-musllinux_1_2_armv7l.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "47842a582de6f5c613b451f8e7ec69ebfdc64f66bf4917c15f1259b4a1a942a1",
"md5": "c05ae868100779eb482a756f69779a9b",
"sha256": "574aa2aa46c73ef8e4ea06ba69339405b366c6b233eefe4687fddc5889350a6a"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-musllinux_1_2_i686.whl",
"has_sig": false,
"md5_digest": "c05ae868100779eb482a756f69779a9b",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 704327,
"upload_time": "2024-10-13T20:49:49",
"upload_time_iso_8601": "2024-10-13T20:49:49.840529Z",
"url": "https://files.pythonhosted.org/packages/47/84/2a582de6f5c613b451f8e7ec69ebfdc64f66bf4917c15f1259b4a1a942a1/pyxirr-0.10.6-cp39-cp39-musllinux_1_2_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "faa34319ff96af7b2d871db6c6f9079cb50df6bba4ac601404ffa67656d96edf",
"md5": "baf514e74f18754b9e8ac522933ad59e",
"sha256": "a29f895a1abaa8157f81f32b09d478b12926408a4a12e3f95464293886cd3c46"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-cp39-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "baf514e74f18754b9e8ac522933ad59e",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 708398,
"upload_time": "2024-10-13T20:49:26",
"upload_time_iso_8601": "2024-10-13T20:49:26.306842Z",
"url": "https://files.pythonhosted.org/packages/fa/a3/4319ff96af7b2d871db6c6f9079cb50df6bba4ac601404ffa67656d96edf/pyxirr-0.10.6-cp39-cp39-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3a2a1f7f2eabd960358e0e83780ba7f1918cae06b29eb261440967b933738014",
"md5": "f5f2edb1e833d593127656a44077cb13",
"sha256": "a299a57ebb69146130ad38305e800aa9c4549c445004b1b23a298c232c620d49"
},
"downloads": -1,
"filename": "pyxirr-0.10.6-cp39-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "f5f2edb1e833d593127656a44077cb13",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.14,>=3.7",
"size": 448533,
"upload_time": "2024-10-13T20:49:48",
"upload_time_iso_8601": "2024-10-13T20:49:48.359791Z",
"url": "https://files.pythonhosted.org/packages/3a/2a/1f7f2eabd960358e0e83780ba7f1918cae06b29eb261440967b933738014/pyxirr-0.10.6-cp39-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "c54a3d2dc1bf565605a4f0e3ae6d5592518bb26698f4d929140d3ea16a4bb478",
"md5": "9975eacc32c19f8bcd579383dd10eda8",
"sha256": "f0ed1fc8607f0769c238ffaf85f0bd3ddac7d383c125f1d9ff16d3a4b86a3cdf"
},
"downloads": -1,
"filename": "pyxirr-0.10.6.tar.gz",
"has_sig": false,
"md5_digest": "9975eacc32c19f8bcd579383dd10eda8",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<3.14,>=3.7",
"size": 40452,
"upload_time": "2024-10-13T20:49:56",
"upload_time_iso_8601": "2024-10-13T20:49:56.412845Z",
"url": "https://files.pythonhosted.org/packages/c5/4a/3d2dc1bf565605a4f0e3ae6d5592518bb26698f4d929140d3ea16a4bb478/pyxirr-0.10.6.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-13 20:49:56",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Anexen",
"github_project": "pyxirr",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "pyxirr"
}