<p align="center">
<img src="https://raw.githubusercontent.com/genthalili/searoute-py/main/searoute/assets/searoute_logo.png" alt="Searoute-py" width=350>
</p>
<p align="center">
<a href="https://pypi.org/project/searoute" target="_blank">
<img src="https://img.shields.io/pypi/v/searoute.svg" alt="Package version">
</a>
<a href="https://pepy.tech/project/searoute" target="_blank">
<img src="https://static.pepy.tech/personalized-badge/searoute?period=total&units=international_system&left_color=grey&right_color=green&left_text=downloads" alt="Downloads">
</a>
<a href="https://pypi.org/project/searoute" target="_blank">
<img src="https://img.shields.io/pypi/pyversions/searoute.svg?color=%2334D058" alt="Supported Python versions">
</a>
</p>
---
## Searoute py
A python package for generating the shortest sea route between two points on Earth.
If points are on land, the function will attempt to find the nearest point on the sea and calculate the route from there.
**Not for routing purposes!** This library was developed to generate realistic-looking sea routes for visualizations of maritime routes, not for mariners to route their ships.
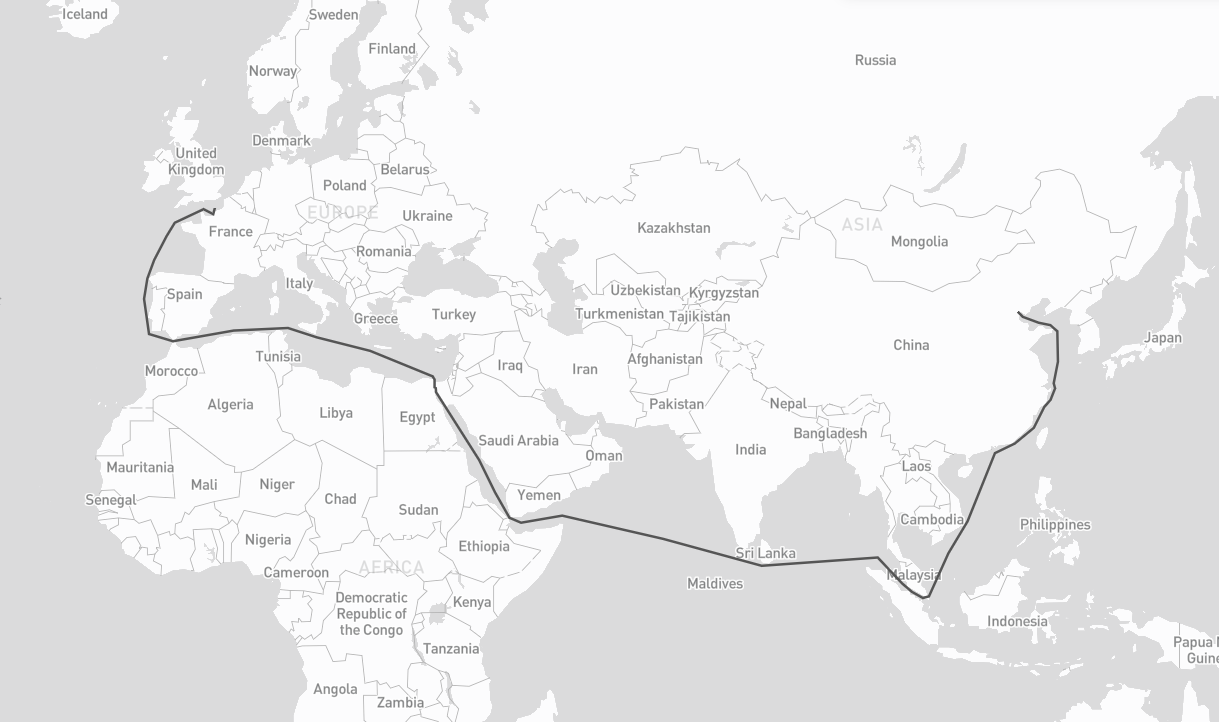
## Installation
~~~bash
pip install searoute
~~~
## Usage
~~~py
import searoute as sr
#Define origin and destination points:
origin = [0.3515625, 50.064191736659104]
destination = [117.42187500000001, 39.36827914916014]
route = sr.searoute(origin, destination)
# > Returns a GeoJSON LineString Feature
# show route distance with unit
print("{:.1f} {}".format(route.properties['length'], route.properties['units']))
# Optionally, define the units for the length calculation included in the properties object.
# Defaults to km, can be can be 'm' = meters 'mi = miles 'ft' = feet 'in' = inches 'deg' = degrees
# 'cen' = centimeters 'rad' = radians 'naut' = nautical 'yd' = yards
routeMiles = sr.searoute(origin, destination, units="mi")
~~~
### Bring your network :
```py
# using version >= 1.2.0
# nodes representing (1,2) of lon = 1, lat = 2
# required : 'x' for lon, 'y' for lat ; optional 'tt' for terminals (boolean or None)
my_nodes = {
(1, 2): {'x': 1, 'y': 2},
(2, 2): {'x': 2, 'y': 2}
}
# (1,2) -> (2,2) with weight, representing the distance, other attributes can be added
# recognized attributes are : `weight` (distance), `passage` (name of the passage to be restricted by restrictions)
my_edges = {
(1, 2): {
(2, 2): {"weight": 10, "other_attr": "some_value"}
}
}
# Marnet
myM = sr.from_nodes_edges_set(sr.Marnet(), my_nodes, my_edges)
# Ports
myP = sr.from_nodes_edges_set(sr.Ports(), my_nodes, None)
# get shortest with your ports
route_with_my_ports = sr.searoute(origin, destination, P = myP, include_ports=True)
# get shortest with your ports
route_with_my_ntw = sr.searoute(origin, destination, P = myP, M = myM )
```
### Nodes and Edges
#### Nodes
A node (or vertex) is a fundamental unit of which the graphs Ports and Marnet are formed.
In searoute, a node is represented by it's id as a ``tuple`` of lon,lat, and it's attributes:
- `x` : float ; required
- `y` : float ; required
- `t` : bool ; optional. default is `False`, will be used for filtering ports with `terminals`.
```py
{
(lon:float, lat:float): {'x': lon:float, 'y': lat:float, *args:any},
...
}
```
#### Edges
An edge is a line or connection between a note and an other. This connection can be represented by a set of node_id->node_id with it's attributes:
- `weight` : float ; required. Can be distance, cost etc... if not set will by default calculate the distance between nodes using [Haversine formula](https://en.wikipedia.org/wiki/Haversine_formula).
- `passage` : str ; optional. Can be one of Passage (searoute.classes.passages.Passage). If not not set, no restriction will be applied. If set make sure to update `Marnet().restrictions = [...]` with your list of passages to avoid.
```py
{
(lon:float, lat:float)->node_id: {
(lon:float, lat:float)->node_id: {
"weight": distance:float,
*args: any}
},
...
}
```
### Example with more parameters :
~~~py
## Using all parameters, wil include ports as well
route = sr.searoute(origin, destination, append_orig_dest=True, restrictions=['northwest'], include_ports=True, port_params={'only_terminals':True, 'country_pol': 'FR', 'country_pod' :'CN'})
~~~
returns :
~~~json
{
"geometry": {
"coordinates": [],
"type": "LineString"
},
"properties": {
"duration_hours": 461.88474412693756,
"length": 20529.85310695412,
"port_dest": {
"cty": "China",
"name": "Tianjin",
"port": "CNTSN",
"t": 1,
"x": 117.744852,
"y": 38.986802
},
"port_origin": {
"cty": "France",
"name": "Le Havre",
"port": "FRLEH",
"t": 1,
"x": 0.107054,
"y": 49.485998
},
"units": "km"
},
"type": "Feature"
}
~~~
## Parameters
`origin`
Mandatory. A tuple or array of 2 floats representing longitude and latitude i.e : `({lon}, {lat})`
`destination`
Mandatory. A tuple or array of 2 floats representing longitude and latitude i.e : `({lon}, {lat})`
`units`
Optional. Default to `km` = kilometers, can be `m` = meters `mi` = miles `ft` = feets `in` = inches `deg` = degrees `cen` = centimeters `rad` = radians `naut` = nauticals `yd` = yards
`speed_knot`
Optional. Speed of the boat, default 24 knots
`append_orig_dest`
Optional. If the origin and destination should be appended to the LineString, default is `False`
`restrictions`
Optional. List of passages to be restricted during calculations.
Possible values : `babalmandab`, `bosporus`, `gibraltar`, `suez`, `panama`, `ormuz`, `northwest`, `malacca`, `sunda`;
default is `['northwest']`
`include_ports`
Optional. If the port of load and discharge should be included, default is `False`
`port_params`
Optional. If `include_ports` is `True` then you can set port configuration for advanced filtering :
- `only_terminals` to include only terminal ports, default `False`
- `country_pol` country iso code (2 letters) for closest port of load, default `None`. When not set or not found, closest port is based on geography.
- `country_pod` country iso code (2 letters) for closest port of discharge, default `None`. When not set or not found, closest port is based on geography.
- `country_restricted` to filter out ports that have registered an argument key `to_cty`(a list) which indicates an existing route to the country same as in `country_pod`; default `False`
default is `{}`
### Returns
GeoJson Feature
## Credits
- [NetworkX](https://networkx.org/), a Python package for the creation, manipulation, and study of the structure, dynamics, and functions of complex networks.
- [GeoJson](https://github.com/jazzband/geojson), a python package for GeoJSON
- [turfpy](https://github.com/omanges/turfpy), a Python library for performing geo-spatial data analysis which reimplements turf.js. (up to version `searoute 1.1.0`)
- [OSMnx](https://github.com/gboeing/osmnx), for geo-spacial networks. (up to version `searoute 1.1.0`)
- Eurostat's [Searoute Java library](https://github.com/eurostat/searoute)
Change Log
==========
1.0.2 (30/09/2022)
------------------
- First Release, published module
1.0.3 (04/10/2022)
------------------
- changed the core of the calculation to osmnx library for better performance
- making 1.0.2 obsolete
- added duration measurement together with length in the LineString properties
1.0.4 (04/10/2022)
------------------
- fix requirements of the module
1.0.5 (04/10/2022)
------------------
- Adjusted network (.json) by connecting all LineStrings in order to return a route from any location to any location
1.0.6 (05/10/2022)
------------------
- Improved network connectivity
1.0.7 (13/10/2022)
------------------
- Improved network connectivity
- Connection points were not adjusted
1.0.8 (25/10/2022)
------------------
- Improved network connectivity
- Added append_orig_dest parameter as input to append origin and destination points to the LineString
- Updated README.md
1.0.9 (04/01/2023)
------------------
- Improved network connectivity
- Added restrictions parameter as input to control restrictiveness of connections due to different reasons
- Updated README.md
1.1.0 (23/05/2023)
------------------
- Improved network connectivity
- Added new feature : closest port of load and port of discharge
- Updated README.md
1.2.0 (24/08/2023)
------------------
- 35x Faster: Significantly accelerated compared to v1.1 by rethinking and reorganizing the code.
- Re-think Class Design: Re-structured and simplified class structure.
- Easy API Access
- Improved Network Connectivity: Enhanced network reliability.
- Updated README.md: Revised for the latest information.
1.2.1 (28/08/2023)
------------------
- Patch fixed issue when querying country with no port result
1.2.2 (08/09/2023)
------------------
- Patch fixed and simplified coords line normalization
1.2.3 (05/01/2024)
------------------
- Patch fixed and improved coords validation
1.3.0 (11/01/2024)
------------------
- Added in port_params additional parameter country_restricted
- Updated license to Apache 2.0
1.3.1 (27/01/2024)
------------------
- Enriched network with additional passages
Raw data
{
"_id": null,
"home_page": "",
"name": "searoute",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "searoute map sea route ocean ports",
"author": "Gent Halili",
"author_email": "genthalili@users.noreply.github.com",
"download_url": "https://files.pythonhosted.org/packages/90/c0/249e1fdc1ae392fd29f6d324b7783ccfea7c24da3c56e4674a47d336e7a6/searoute-1.3.1.tar.gz",
"platform": null,
"description": "<p align=\"center\">\n <img src=\"https://raw.githubusercontent.com/genthalili/searoute-py/main/searoute/assets/searoute_logo.png\" alt=\"Searoute-py\" width=350>\n</p>\n<p align=\"center\">\n<a href=\"https://pypi.org/project/searoute\" target=\"_blank\">\n <img src=\"https://img.shields.io/pypi/v/searoute.svg\" alt=\"Package version\">\n</a>\n<a href=\"https://pepy.tech/project/searoute\" target=\"_blank\">\n <img src=\"https://static.pepy.tech/personalized-badge/searoute?period=total&units=international_system&left_color=grey&right_color=green&left_text=downloads\" alt=\"Downloads\">\n</a>\n<a href=\"https://pypi.org/project/searoute\" target=\"_blank\">\n <img src=\"https://img.shields.io/pypi/pyversions/searoute.svg?color=%2334D058\" alt=\"Supported Python versions\">\n</a>\n</p>\n\n---\n## Searoute py\n\nA python package for generating the shortest sea route between two points on Earth. \n\nIf points are on land, the function will attempt to find the nearest point on the sea and calculate the route from there. \n\n**Not for routing purposes!** This library was developed to generate realistic-looking sea routes for visualizations of maritime routes, not for mariners to route their ships. \n\n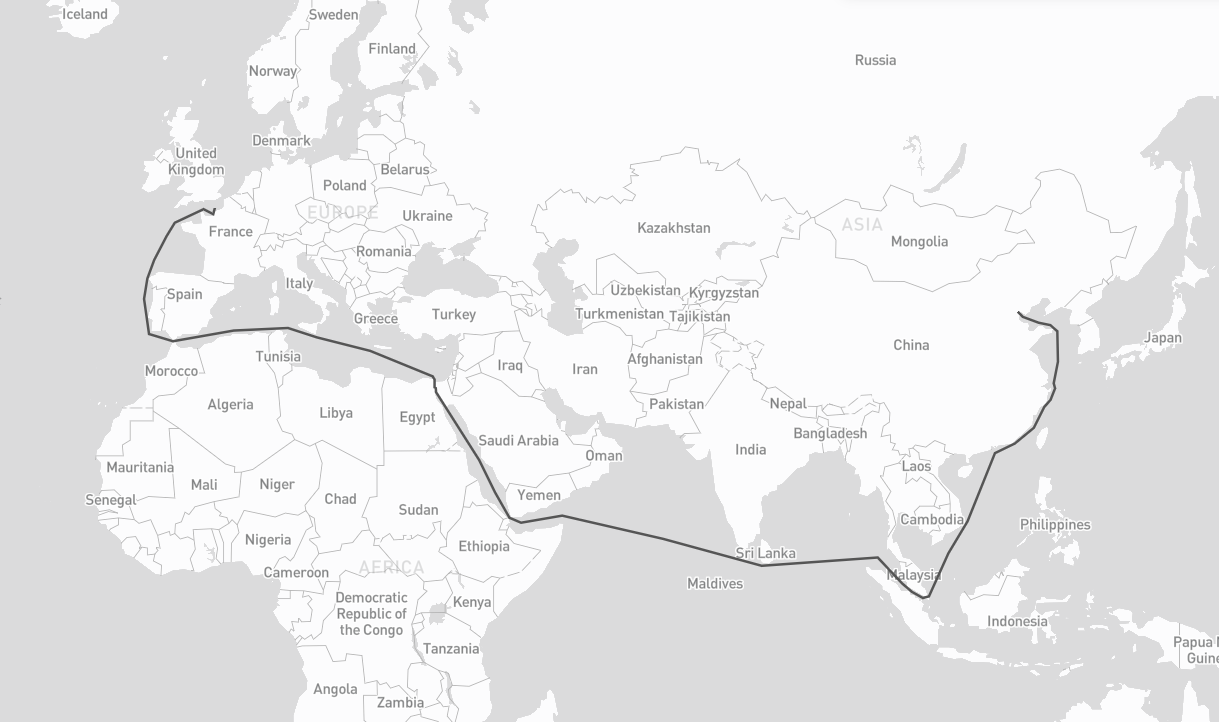\n\n## Installation\n\n~~~bash\npip install searoute\n~~~\n\n## Usage\n\n~~~py\nimport searoute as sr\n\n#Define origin and destination points:\norigin = [0.3515625, 50.064191736659104]\n\ndestination = [117.42187500000001, 39.36827914916014]\n\n\nroute = sr.searoute(origin, destination)\n# > Returns a GeoJSON LineString Feature\n# show route distance with unit\nprint(\"{:.1f} {}\".format(route.properties['length'], route.properties['units']))\n\n# Optionally, define the units for the length calculation included in the properties object.\n# Defaults to km, can be can be 'm' = meters 'mi = miles 'ft' = feet 'in' = inches 'deg' = degrees\n# 'cen' = centimeters 'rad' = radians 'naut' = nautical 'yd' = yards\nrouteMiles = sr.searoute(origin, destination, units=\"mi\")\n~~~\n### Bring your network :\n```py\n# using version >= 1.2.0\n\n# nodes representing (1,2) of lon = 1, lat = 2\n# required : 'x' for lon, 'y' for lat ; optional 'tt' for terminals (boolean or None)\nmy_nodes = {\n (1, 2): {'x': 1, 'y': 2},\n (2, 2): {'x': 2, 'y': 2}\n}\n# (1,2) -> (2,2) with weight, representing the distance, other attributes can be added\n# recognized attributes are : `weight` (distance), `passage` (name of the passage to be restricted by restrictions) \nmy_edges = {\n (1, 2): {\n (2, 2): {\"weight\": 10, \"other_attr\": \"some_value\"}\n }\n}\n\n# Marnet\nmyM = sr.from_nodes_edges_set(sr.Marnet(), my_nodes, my_edges)\n# Ports\nmyP = sr.from_nodes_edges_set(sr.Ports(), my_nodes, None) \n\n# get shortest with your ports\nroute_with_my_ports = sr.searoute(origin, destination, P = myP, include_ports=True)\n\n# get shortest with your ports\nroute_with_my_ntw = sr.searoute(origin, destination, P = myP, M = myM )\n\n```\n### Nodes and Edges\n#### Nodes \nA node (or vertex) is a fundamental unit of which the graphs Ports and Marnet are formed.\n\nIn searoute, a node is represented by it's id as a ``tuple`` of lon,lat, and it's attributes:\n- `x` : float ; required\n- `y` : float ; required\n- `t` : bool ; optional. default is `False`, will be used for filtering ports with `terminals`.\n```py\n{\n (lon:float, lat:float): {'x': lon:float, 'y': lat:float, *args:any},\n ...\n}\n```\n#### Edges \nAn edge is a line or connection between a note and an other. This connection can be represented by a set of node_id->node_id with it's attributes:\n- `weight` : float ; required. Can be distance, cost etc... if not set will by default calculate the distance between nodes using [Haversine formula](https://en.wikipedia.org/wiki/Haversine_formula).\n- `passage` : str ; optional. Can be one of Passage (searoute.classes.passages.Passage). If not not set, no restriction will be applied. If set make sure to update `Marnet().restrictions = [...]` with your list of passages to avoid.\n```py\n{\n (lon:float, lat:float)->node_id: {\n (lon:float, lat:float)->node_id: {\n \"weight\": distance:float, \n *args: any}\n },\n ...\n}\n```\n\n### Example with more parameters :\n~~~py\n## Using all parameters, wil include ports as well\nroute = sr.searoute(origin, destination, append_orig_dest=True, restrictions=['northwest'], include_ports=True, port_params={'only_terminals':True, 'country_pol': 'FR', 'country_pod' :'CN'})\n~~~\nreturns :\n~~~json\n{\n \"geometry\": {\n \"coordinates\": [],\n \"type\": \"LineString\"\n },\n \"properties\": {\n \"duration_hours\": 461.88474412693756,\n \"length\": 20529.85310695412,\n \"port_dest\": {\n \"cty\": \"China\",\n \"name\": \"Tianjin\",\n \"port\": \"CNTSN\",\n \"t\": 1,\n \"x\": 117.744852,\n \"y\": 38.986802\n },\n \"port_origin\": {\n \"cty\": \"France\",\n \"name\": \"Le Havre\",\n \"port\": \"FRLEH\",\n \"t\": 1,\n \"x\": 0.107054,\n \"y\": 49.485998\n },\n \"units\": \"km\"\n },\n \"type\": \"Feature\"\n}\n~~~\n\n## Parameters\n\n`origin` \nMandatory. A tuple or array of 2 floats representing longitude and latitude i.e : `({lon}, {lat})`\n\n`destination` \nMandatory. A tuple or array of 2 floats representing longitude and latitude i.e : `({lon}, {lat})`\n\n`units` \nOptional. Default to `km` = kilometers, can be `m` = meters `mi` = miles `ft` = feets `in` = inches `deg` = degrees `cen` = centimeters `rad` = radians `naut` = nauticals `yd` = yards\n\n`speed_knot` \nOptional. Speed of the boat, default 24 knots \n\n`append_orig_dest` \nOptional. If the origin and destination should be appended to the LineString, default is `False`\n\n`restrictions` \nOptional. List of passages to be restricted during calculations.\nPossible values : `babalmandab`, `bosporus`, `gibraltar`, `suez`, `panama`, `ormuz`, `northwest`, `malacca`, `sunda`;\ndefault is `['northwest']`\n\n`include_ports` \nOptional. If the port of load and discharge should be included, default is `False`\n\n`port_params` \nOptional. If `include_ports` is `True` then you can set port configuration for advanced filtering :\n- `only_terminals` to include only terminal ports, default `False`\n- `country_pol` country iso code (2 letters) for closest port of load, default `None`. When not set or not found, closest port is based on geography.\n- `country_pod` country iso code (2 letters) for closest port of discharge, default `None`. When not set or not found, closest port is based on geography.\n- `country_restricted` to filter out ports that have registered an argument key `to_cty`(a list) which indicates an existing route to the country same as in `country_pod`; default `False`\n\ndefault is `{}`\n\n### Returns\nGeoJson Feature\n## Credits\n\n- [NetworkX](https://networkx.org/), a Python package for the creation, manipulation, and study of the structure, dynamics, and functions of complex networks.\n- [GeoJson](https://github.com/jazzband/geojson), a python package for GeoJSON\n- [turfpy](https://github.com/omanges/turfpy), a Python library for performing geo-spatial data analysis which reimplements turf.js. (up to version `searoute 1.1.0`)\n- [OSMnx](https://github.com/gboeing/osmnx), for geo-spacial networks. (up to version `searoute 1.1.0`)\n- Eurostat's [Searoute Java library](https://github.com/eurostat/searoute)\n\nChange Log\n==========\n\n1.0.2 (30/09/2022)\n------------------\n- First Release, published module\n\n1.0.3 (04/10/2022)\n------------------\n- changed the core of the calculation to osmnx library for better performance\n- making 1.0.2 obsolete\n- added duration measurement together with length in the LineString properties\n\n1.0.4 (04/10/2022)\n------------------\n- fix requirements of the module\n\n1.0.5 (04/10/2022)\n------------------\n- Adjusted network (.json) by connecting all LineStrings in order to return a route from any location to any location\n\n1.0.6 (05/10/2022)\n------------------\n- Improved network connectivity\n\n1.0.7 (13/10/2022)\n------------------\n- Improved network connectivity\n- Connection points were not adjusted\n\n1.0.8 (25/10/2022)\n------------------\n- Improved network connectivity\n- Added append_orig_dest parameter as input to append origin and destination points to the LineString\n- Updated README.md\n\n1.0.9 (04/01/2023)\n------------------\n- Improved network connectivity\n- Added restrictions parameter as input to control restrictiveness of connections due to different reasons\n- Updated README.md\n\n1.1.0 (23/05/2023)\n------------------\n- Improved network connectivity\n- Added new feature : closest port of load and port of discharge\n- Updated README.md\n\n1.2.0 (24/08/2023)\n------------------\n- 35x Faster: Significantly accelerated compared to v1.1 by rethinking and reorganizing the code.\n- Re-think Class Design: Re-structured and simplified class structure.\n- Easy API Access\n- Improved Network Connectivity: Enhanced network reliability.\n- Updated README.md: Revised for the latest information.\n\n1.2.1 (28/08/2023)\n------------------\n- Patch fixed issue when querying country with no port result\n\n1.2.2 (08/09/2023)\n------------------\n- Patch fixed and simplified coords line normalization\n\n1.2.3 (05/01/2024)\n------------------\n- Patch fixed and improved coords validation\n\n1.3.0 (11/01/2024)\n------------------\n- Added in port_params additional parameter country_restricted\n- Updated license to Apache 2.0\n\n1.3.1 (27/01/2024)\n------------------\n- Enriched network with additional passages\n",
"bugtrack_url": null,
"license": "Apache License 2.0",
"summary": "A python package for generating the shortest sea route between two points on Earth.",
"version": "1.3.1",
"project_urls": {
"Documentation": "https://github.com/genthalili/searoute-py/blob/main/README.md",
"Source": "https://github.com/genthalili/searoute-py"
},
"split_keywords": [
"searoute",
"map",
"sea",
"route",
"ocean",
"ports"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "90c0249e1fdc1ae392fd29f6d324b7783ccfea7c24da3c56e4674a47d336e7a6",
"md5": "8f05e7067cdbbd6011edfe891bab3f4b",
"sha256": "975b5c3a655db8ced7043bcb414d8d4d9fbeb5653a90d5737dc4cffc056cbac2"
},
"downloads": -1,
"filename": "searoute-1.3.1.tar.gz",
"has_sig": false,
"md5_digest": "8f05e7067cdbbd6011edfe891bab3f4b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 1012262,
"upload_time": "2024-01-27T09:47:27",
"upload_time_iso_8601": "2024-01-27T09:47:27.869082Z",
"url": "https://files.pythonhosted.org/packages/90/c0/249e1fdc1ae392fd29f6d324b7783ccfea7c24da3c56e4674a47d336e7a6/searoute-1.3.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-01-27 09:47:27",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "genthalili",
"github_project": "searoute-py",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "searoute"
}