Name | simmon JSON |
Version |
0.0.5
JSON |
| download |
home_page | |
Summary | A simple simulation monitor |
upload_time | 2023-05-20 08:19:20 |
maintainer | |
docs_url | None |
author | Roie Zemel |
requires_python | >=3.8 |
license | |
keywords |
simulation
data tracking
|
VCS |
|
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
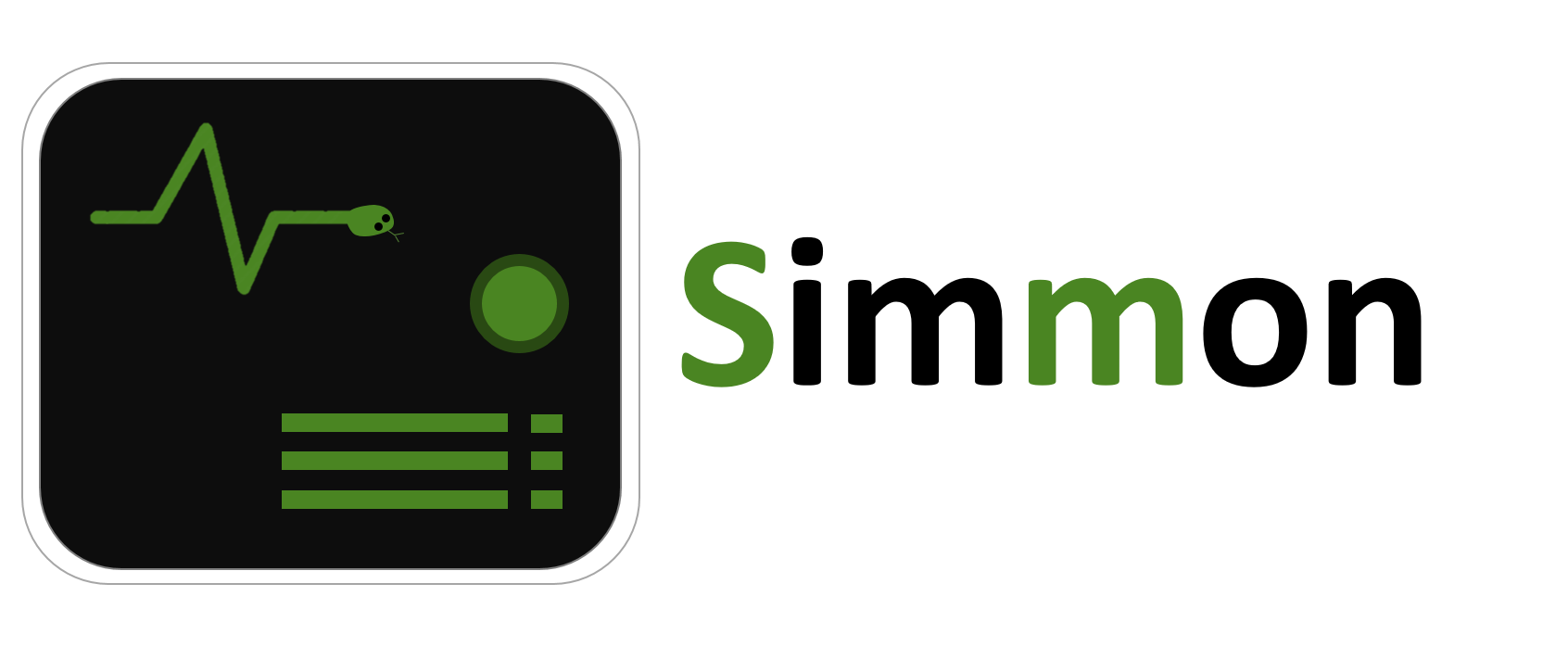
[](https://badge.fury.io/py/simmon)
[](http://choosealicense.com/licenses/mit/)
[](https://github.com/roiezemel/simmon)
[](https://simmon.readthedocs.io/en/latest/?badge=latest)
# A simple monitor for simulations and other numeric processes
## Overview
This project provides a simple tool for tracking simulations and
other numeric processes. It provides a Monitor class that has
all the following functionality:
* Tracking numeric variables.
* Plotting the data.
* An updating live view of the tracked variables.
* A window of convenience toggles to control the progress
of a long-running simulation.
* Automatically saving all the collected data in a single output directory, including:
* .csv files
* Plots
* A config file with configuration constants
* A summary text file
* Loading an output directory to continue working with a simulation that's been terminated.
* Preventing your computer from going into sleep mode (requires `pyautogui` module).
## Installation
Install with `pip`:
```commandline
pip install simmon
```
## Usage
You can find the documentation of this project [here](https://simmon.readthedocs.io/en/latest/?badge=latest).
Here's a quick example of the `Monitor` class used to track a moving object simulation:
```python
from simmon import Monitor
# create Monitor - this already opens an output directory and a toggle-buttons control window
mon = Monitor('Example Monitor')
# add a new 'tracker' to track variables
tr = mon.tracker('time', 'velocity')
# set simulation configuration constants
mon.its = 10000 # if declared as mon's attribute, the value will be
mon.dt = 0.1 # added to the config.txt file
mon.a = 1
# helper variables
time = 0
v = 0
# simulate
for i in range(mon.its):
# update tracker: provide time and velocity
tr.update(time, v)
# calculate next time step
time += mon.dt
v += mon.a * mon.dt
# finalize - saves all data and closes necessary processes and windows
mon.finalize()
```
A few notes:
- When creating a Monitor instance, an output directory is automatically created (unless disabled, see tip below).
- Also, a window of toggles opens to give the user some control over the progress of the simulation. One of the toggles
opens the live view of the data.
- Later, a 'tracker' is added to the Monitor to track velocity against time. Each tracker is associated with **one** independent variable,
and multiple dependent variables. When calling `update()` the number of values provided should be equal to the number of variable labels.
- Variables declared as attributes of the Monitor object (like `its` and `dt`) are considered configuration constants. These values will be
added to the config.txt file at the end.
- `finalize()` is an important method that closes all subprocesses that haven't been closed and saves all the data that hasn't been saved.
Tip: For a traceless Monitor with no output directory, use the
QuietMonitor class instead.
### About trackers
Each 'tracker' added to the `Monitor` is provided with a sequence of data labels.
As mentioned, a 'tracker' is associated with one independent variable and multiple dependent variables:
```python
tr = mon.tracker('x', 'y1', 'y2', 'y3') # 'y4', 'y5', ...
```
By default, the `update()` method of a tracker does not save the data into the output file.
To change that, set `autosave=` to True:
```python
tr = mon.tracker('time', 'force on body', autosave=True)
```
Sometimes, it might be helpful to give each tracker a title. This helps with the neat organization
of the data in the output directory. Trackers with the same title are plotted on the same
figure when `finalize()` is called. To give the tracker a title, set the `title=` keyword argument.
Use the `plot()` method of Monitor to show graphs of
the collected data.
`plot()` receives any number of arguments. Each argument can either be a
title, a tracker object, or an iterable of tracker objects:
```python
tr1 = mon.tracker('x', 'y1', title='Things against x')
tr2 = mon.tracker('x', 'y2', title='Things against x')
tr3 = mon.tracker('time', 'force')
...
# plot everything at once:
mon.plot('Things against x', tr3, [tr1, tr2, tr3])
```
### About toggles
The 'Toggles' window is opened from the moment a Monitor is created and until `finalize()` is called.
Some toggles are added by default. Custom toggles can be added in a very simple way:
```python
from simmon import Monitor
# after this, the toggles window is opened
mon = Monitor('Custom Toggles Example')
# add a toggle button that quits the simulation
quit_toggle = mon.add_toggle(desc='Quit simulation')
...
while not quit_toggle.toggled():
# simulate stuff
...
```
As you can see, new toggles can be added very easily. The `add_toggle()` method
returns a Toggle object, whose `toggled()` method returns True for every toggle made
by the user.
### Loading Monitor data
Finally, another cool feature of the Monitor class, is that all the data stored in
an output directory, can later be loaded into a Monitor object. This way, a simulation
that's been terminated can be resumed, and the data will keep streaming to the same place:
```python
from simmon import Monitor
mon = Monitor('Example Monitor')
mon.load_from_dir() # this loads all the data from the 'Example Monitor' output directory
# now all data has been loaded back to the Monitor
print(len(mon.trackers)) # a list of all trackers
mon.plot(mon.trackers[0]) # same data can be plotted
mon.plot('Things against x') # even the same titles
print(mon.dt, mon.its) # config variables are still there
```
## Contributing
Please report any bug you might come across. Contributions and enhancements are very welcome!
Take a look at the [documentation](https://simmon.readthedocs.io/en/latest/?badge=latest) of this project.
Raw data
{
"_id": null,
"home_page": "",
"name": "simmon",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "",
"keywords": "simulation, data tracking",
"author": "Roie Zemel",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/89/fe/a06b13a439d27b3f7d249226ce8a7c314b01f09de77c55fbd5c97f9564a2/simmon-0.0.5.tar.gz",
"platform": null,
"description": "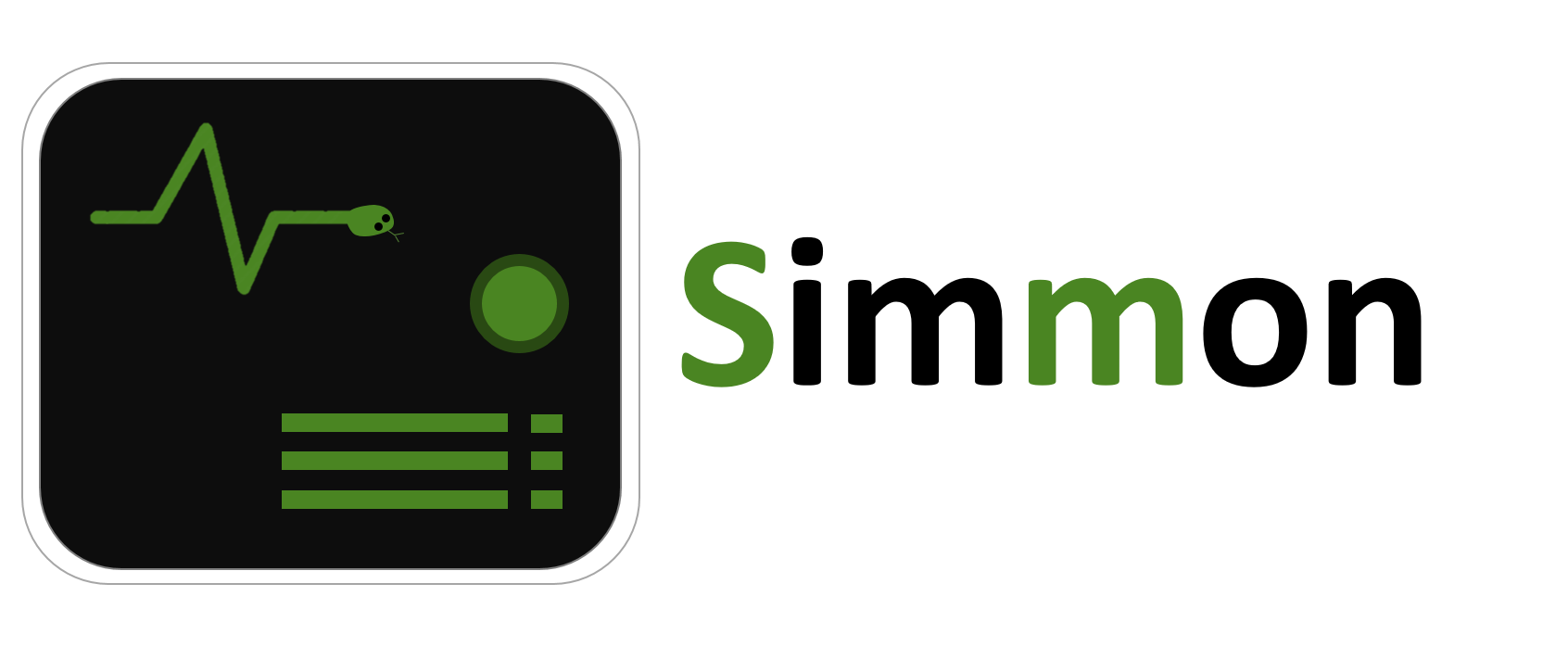\r\n\r\n[](https://badge.fury.io/py/simmon)\r\n[](http://choosealicense.com/licenses/mit/)\r\n[](https://github.com/roiezemel/simmon)\r\n[](https://simmon.readthedocs.io/en/latest/?badge=latest)\r\n\r\n# A simple monitor for simulations and other numeric processes\r\n\r\n## Overview\r\nThis project provides a simple tool for tracking simulations and \r\nother numeric processes. It provides a Monitor class that has \r\nall the following functionality:\r\n\r\n* Tracking numeric variables.\r\n* Plotting the data.\r\n* An updating live view of the tracked variables.\r\n* A window of convenience toggles to control the progress\r\nof a long-running simulation.\r\n* Automatically saving all the collected data in a single output directory, including:\r\n * .csv files\r\n * Plots\r\n * A config file with configuration constants\r\n * A summary text file\r\n* Loading an output directory to continue working with a simulation that's been terminated.\r\n* Preventing your computer from going into sleep mode (requires `pyautogui` module).\r\n\r\n## Installation\r\nInstall with `pip`:\r\n```commandline\r\npip install simmon\r\n```\r\n\r\n## Usage\r\nYou can find the documentation of this project [here](https://simmon.readthedocs.io/en/latest/?badge=latest).\r\nHere's a quick example of the `Monitor` class used to track a moving object simulation:\r\n\r\n```python\r\nfrom simmon import Monitor\r\n\r\n# create Monitor - this already opens an output directory and a toggle-buttons control window\r\nmon = Monitor('Example Monitor')\r\n\r\n# add a new 'tracker' to track variables\r\ntr = mon.tracker('time', 'velocity')\r\n\r\n# set simulation configuration constants\r\nmon.its = 10000 # if declared as mon's attribute, the value will be \r\nmon.dt = 0.1 # added to the config.txt file\r\nmon.a = 1\r\n\r\n# helper variables\r\ntime = 0\r\nv = 0\r\n\r\n# simulate\r\nfor i in range(mon.its):\r\n # update tracker: provide time and velocity\r\n tr.update(time, v)\r\n\r\n # calculate next time step\r\n time += mon.dt\r\n v += mon.a * mon.dt\r\n\r\n# finalize - saves all data and closes necessary processes and windows\r\nmon.finalize()\r\n```\r\nA few notes:\r\n- When creating a Monitor instance, an output directory is automatically created (unless disabled, see tip below).\r\n- Also, a window of toggles opens to give the user some control over the progress of the simulation. One of the toggles \r\nopens the live view of the data.\r\n- Later, a 'tracker' is added to the Monitor to track velocity against time. Each tracker is associated with **one** independent variable, \r\nand multiple dependent variables. When calling `update()` the number of values provided should be equal to the number of variable labels.\r\n- Variables declared as attributes of the Monitor object (like `its` and `dt`) are considered configuration constants. These values will be\r\nadded to the config.txt file at the end.\r\n- `finalize()` is an important method that closes all subprocesses that haven't been closed and saves all the data that hasn't been saved.\r\n\r\nTip: For a traceless Monitor with no output directory, use the \r\nQuietMonitor class instead.\r\n\r\n### About trackers\r\nEach 'tracker' added to the `Monitor` is provided with a sequence of data labels.\r\nAs mentioned, a 'tracker' is associated with one independent variable and multiple dependent variables:\r\n```python\r\ntr = mon.tracker('x', 'y1', 'y2', 'y3') # 'y4', 'y5', ...\r\n```\r\nBy default, the `update()` method of a tracker does not save the data into the output file.\r\nTo change that, set `autosave=` to True:\r\n```python\r\ntr = mon.tracker('time', 'force on body', autosave=True)\r\n```\r\nSometimes, it might be helpful to give each tracker a title. This helps with the neat organization \r\nof the data in the output directory. Trackers with the same title are plotted on the same \r\nfigure when `finalize()` is called. To give the tracker a title, set the `title=` keyword argument.\r\n\r\nUse the `plot()` method of Monitor to show graphs of\r\nthe collected data.\r\n`plot()` receives any number of arguments. Each argument can either be a \r\ntitle, a tracker object, or an iterable of tracker objects:\r\n```python\r\ntr1 = mon.tracker('x', 'y1', title='Things against x')\r\ntr2 = mon.tracker('x', 'y2', title='Things against x')\r\ntr3 = mon.tracker('time', 'force')\r\n\r\n...\r\n\r\n# plot everything at once:\r\nmon.plot('Things against x', tr3, [tr1, tr2, tr3])\r\n```\r\n\r\n### About toggles\r\nThe 'Toggles' window is opened from the moment a Monitor is created and until `finalize()` is called.\r\nSome toggles are added by default. Custom toggles can be added in a very simple way:\r\n\r\n```python\r\nfrom simmon import Monitor\r\n\r\n# after this, the toggles window is opened\r\nmon = Monitor('Custom Toggles Example')\r\n\r\n# add a toggle button that quits the simulation\r\nquit_toggle = mon.add_toggle(desc='Quit simulation')\r\n\r\n...\r\n\r\nwhile not quit_toggle.toggled():\r\n# simulate stuff\r\n\r\n...\r\n```\r\n\r\nAs you can see, new toggles can be added very easily. The `add_toggle()` method\r\nreturns a Toggle object, whose `toggled()` method returns True for every toggle made \r\nby the user.\r\n\r\n\r\n### Loading Monitor data\r\nFinally, another cool feature of the Monitor class, is that all the data stored in \r\nan output directory, can later be loaded into a Monitor object. This way, a simulation \r\nthat's been terminated can be resumed, and the data will keep streaming to the same place:\r\n\r\n```python\r\nfrom simmon import Monitor\r\n\r\nmon = Monitor('Example Monitor')\r\nmon.load_from_dir() # this loads all the data from the 'Example Monitor' output directory\r\n\r\n# now all data has been loaded back to the Monitor\r\nprint(len(mon.trackers)) # a list of all trackers\r\nmon.plot(mon.trackers[0]) # same data can be plotted\r\nmon.plot('Things against x') # even the same titles\r\nprint(mon.dt, mon.its) # config variables are still there\r\n```\r\n\r\n## Contributing\r\nPlease report any bug you might come across. Contributions and enhancements are very welcome!\r\nTake a look at the [documentation](https://simmon.readthedocs.io/en/latest/?badge=latest) of this project.\r\n",
"bugtrack_url": null,
"license": "",
"summary": "A simple simulation monitor",
"version": "0.0.5",
"project_urls": null,
"split_keywords": [
"simulation",
" data tracking"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "57aab02273fc2b1742a8e756a935a998f20ce005d2bc549295ebae4f5806a4be",
"md5": "61c8523ef13439d464afce5bfdc0ab08",
"sha256": "5ad40f444209fea59fd2aa902ebe39e4c3f7342dafa0263c1ea6097e40ca39e1"
},
"downloads": -1,
"filename": "simmon-0.0.5-py3-none-any.whl",
"has_sig": false,
"md5_digest": "61c8523ef13439d464afce5bfdc0ab08",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 17260,
"upload_time": "2023-05-20T08:19:17",
"upload_time_iso_8601": "2023-05-20T08:19:17.746701Z",
"url": "https://files.pythonhosted.org/packages/57/aa/b02273fc2b1742a8e756a935a998f20ce005d2bc549295ebae4f5806a4be/simmon-0.0.5-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "89fea06b13a439d27b3f7d249226ce8a7c314b01f09de77c55fbd5c97f9564a2",
"md5": "6091a8535df356ef178123c1e7dbf4f5",
"sha256": "a3a9dd1829c9f9582557abe22c61b7d2a4dc77d67fd317f00f0caad7932fad46"
},
"downloads": -1,
"filename": "simmon-0.0.5.tar.gz",
"has_sig": false,
"md5_digest": "6091a8535df356ef178123c1e7dbf4f5",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 17023,
"upload_time": "2023-05-20T08:19:20",
"upload_time_iso_8601": "2023-05-20T08:19:20.075239Z",
"url": "https://files.pythonhosted.org/packages/89/fe/a06b13a439d27b3f7d249226ce8a7c314b01f09de77c55fbd5c97f9564a2/simmon-0.0.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-05-20 08:19:20",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "simmon"
}