# solarcell
Estimate IV- and PV-curves given photovoltaic cell datasheet parameters. Capable of generating combined curves for solar arrays with temperature and light intensity gradients. Assumes ideal bypass and blocking diodes for all cells.
[Available for download on PyPI.](https://pypi.org/project/solarcell/)
`pip install solarcell`
## Usage
See the examples contained within this repository. Please also consider reading the related blog post, ["How To Estimate Complex Solar Array Power Curves".](https://www.osborneee.com/solarcell/)
```python
import numpy as np
from matplotlib import pyplot as plt
from solarcell import solarcell
# Azur Space 3G30A triple-junction solar cells in a 24s12p configuration.
# Isc/Imp are specified in (A, A/C), Voc/Vmp are specified in (V, V/C).
# Temperature is specified in C. Intensity is unitless and scales Isc/Imp.
azur3g30a = solarcell(
isc=(0.5196, 0.00036), # short-circuit current, temp coefficient
voc=(2.690, -0.0062), # open-circuit voltage, temp coefficient
imp=(0.5029, 0.00024), # max-power current, temp coefficient
vmp=(2.409, -0.0067), # max-power voltage, temp coefficient
area=30.18, # solar cell area in square centimeters
t=28, # temperature at which the above parameters are specified
)
array = azur3g30a.array(t=np.full((24, 12), 80), g=np.ones((24, 12)))
print(array)
# Isc = 6.460 A, Voc = 56.82 V
# Imp = 6.201 A, Vmp = 49.33 V, Pmp = 305.9 W
fig, (ax0, ax1) = plt.subplots(nrows=2, sharex=True)
v = np.linspace(0, array.voc, 1000)
ax0.plot(v, array.iv(v)), ax0.grid()
ax1.plot(v, array.pv(v)), ax1.grid()
```
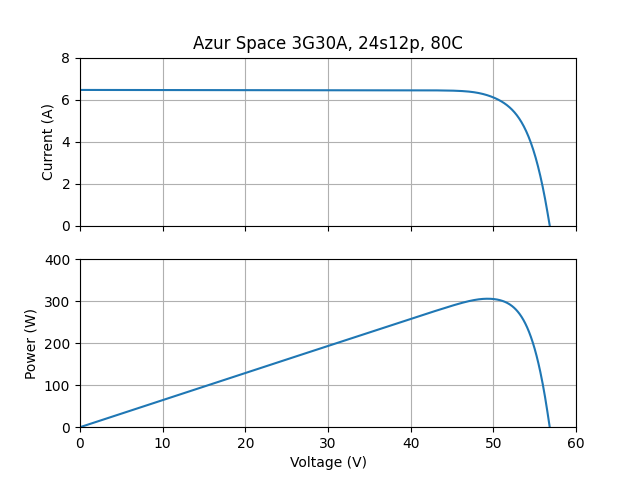
## Background
A numeric optimization procedure is used to best fit the classic photovoltaic cell single diode model equation to the datasheet parameters at the reference temperature. Curves at other temperatures are derived relative to this initial curve fit by way of a linear transformation (plus a sigmoid smoothing function) that maintains the characteristic shape of the curve. Combining cells in series/parallel with different IV-curves is done by linear interpolation.
When computing a curve, the provided temperatures and intensities are generally organized as follows: `cell(t, g)` accepts single values, `string(t, g)` accepts one-dimensional arrays, and `array(t, g)` accepts two-dimensional arrays (where strings make up the columns). A cache is implemented to increase speed for repeated computations; rounding by the user will yield better performance.
## References
1. Amit Jain, Avinashi Kapoor, Exact analytical solutions of the parameters of real solar cells using Lambert W-function, Solar Energy Materials and Solar Cells, Volume 81, Issue 2, 2004, Pages 269-277, ISSN 0927-0248, https://doi.org/10.1016/j.solmat.2003.11.018.
Raw data
{
"_id": null,
"home_page": "https://github.com/amosborne/solarcell",
"name": "solarcell",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.11,<4.0",
"maintainer_email": "",
"keywords": "solar,photovoltaic",
"author": "Alexander Osborne",
"author_email": "alex@osborneee.com",
"download_url": "https://files.pythonhosted.org/packages/ac/b8/a250f591a0facc888fdf0b50ddc0a4bcd91c7484de881625eb03108366e1/solarcell-0.2.0.tar.gz",
"platform": null,
"description": "# solarcell\nEstimate IV- and PV-curves given photovoltaic cell datasheet parameters. Capable of generating combined curves for solar arrays with temperature and light intensity gradients. Assumes ideal bypass and blocking diodes for all cells.\n\n[Available for download on PyPI.](https://pypi.org/project/solarcell/)\n\n`pip install solarcell`\n\n## Usage\n\nSee the examples contained within this repository. Please also consider reading the related blog post, [\"How To Estimate Complex Solar Array Power Curves\".](https://www.osborneee.com/solarcell/)\n\n```python\nimport numpy as np\nfrom matplotlib import pyplot as plt\nfrom solarcell import solarcell\n\n# Azur Space 3G30A triple-junction solar cells in a 24s12p configuration.\n# Isc/Imp are specified in (A, A/C), Voc/Vmp are specified in (V, V/C).\n# Temperature is specified in C. Intensity is unitless and scales Isc/Imp.\n\nazur3g30a = solarcell(\n isc=(0.5196, 0.00036), # short-circuit current, temp coefficient\n voc=(2.690, -0.0062), # open-circuit voltage, temp coefficient\n imp=(0.5029, 0.00024), # max-power current, temp coefficient\n vmp=(2.409, -0.0067), # max-power voltage, temp coefficient\n area=30.18, # solar cell area in square centimeters\n t=28, # temperature at which the above parameters are specified\n)\n\narray = azur3g30a.array(t=np.full((24, 12), 80), g=np.ones((24, 12)))\n\nprint(array)\n# Isc = 6.460 A, Voc = 56.82 V\n# Imp = 6.201 A, Vmp = 49.33 V, Pmp = 305.9 W\n\nfig, (ax0, ax1) = plt.subplots(nrows=2, sharex=True)\n\nv = np.linspace(0, array.voc, 1000)\nax0.plot(v, array.iv(v)), ax0.grid()\nax1.plot(v, array.pv(v)), ax1.grid()\n```\n\n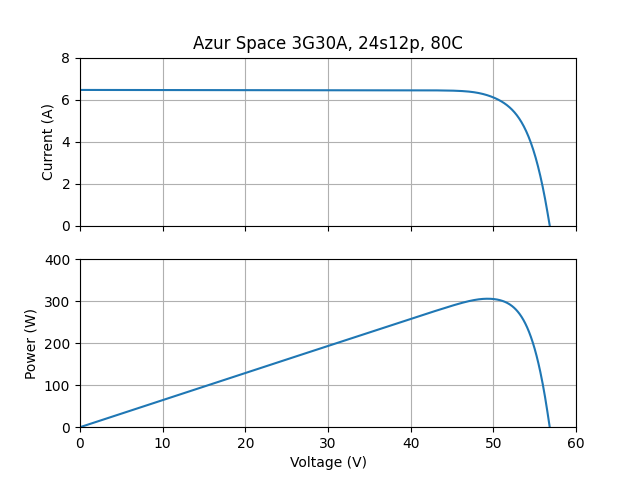\n\n## Background\n\nA numeric optimization procedure is used to best fit the classic photovoltaic cell single diode model equation to the datasheet parameters at the reference temperature. Curves at other temperatures are derived relative to this initial curve fit by way of a linear transformation (plus a sigmoid smoothing function) that maintains the characteristic shape of the curve. Combining cells in series/parallel with different IV-curves is done by linear interpolation.\n\nWhen computing a curve, the provided temperatures and intensities are generally organized as follows: `cell(t, g)` accepts single values, `string(t, g)` accepts one-dimensional arrays, and `array(t, g)` accepts two-dimensional arrays (where strings make up the columns). A cache is implemented to increase speed for repeated computations; rounding by the user will yield better performance.\n\n## References\n\n1. Amit Jain, Avinashi Kapoor, Exact analytical solutions of the parameters of real solar cells using Lambert W-function, Solar Energy Materials and Solar Cells, Volume 81, Issue 2, 2004, Pages 269-277, ISSN 0927-0248, https://doi.org/10.1016/j.solmat.2003.11.018.\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Estimate IV- and PV-curves given photovoltaic cell datasheet parameters.",
"version": "0.2.0",
"project_urls": {
"Homepage": "https://github.com/amosborne/solarcell",
"Repository": "https://github.com/amosborne/solarcell"
},
"split_keywords": [
"solar",
"photovoltaic"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "734996e9cb2175d70f002523cada4f55f359216d79142bf11c18954afc3feff7",
"md5": "6653d82e78a7882dae598cac3f102e38",
"sha256": "24d592964f75c0ed3f0a583aaf77f9c9723d7bf89bbc98a0d4de98de5d9cc06e"
},
"downloads": -1,
"filename": "solarcell-0.2.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "6653d82e78a7882dae598cac3f102e38",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.11,<4.0",
"size": 6686,
"upload_time": "2024-01-29T06:22:56",
"upload_time_iso_8601": "2024-01-29T06:22:56.730429Z",
"url": "https://files.pythonhosted.org/packages/73/49/96e9cb2175d70f002523cada4f55f359216d79142bf11c18954afc3feff7/solarcell-0.2.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "acb8a250f591a0facc888fdf0b50ddc0a4bcd91c7484de881625eb03108366e1",
"md5": "ceb29f1e349c41cf57d5c530bfcf9e6f",
"sha256": "386fb69514905951ef17cdf1b55abc359c5f530d34b66c1eb702ee8eec819e17"
},
"downloads": -1,
"filename": "solarcell-0.2.0.tar.gz",
"has_sig": false,
"md5_digest": "ceb29f1e349c41cf57d5c530bfcf9e6f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.11,<4.0",
"size": 6239,
"upload_time": "2024-01-29T06:22:57",
"upload_time_iso_8601": "2024-01-29T06:22:57.866570Z",
"url": "https://files.pythonhosted.org/packages/ac/b8/a250f591a0facc888fdf0b50ddc0a4bcd91c7484de881625eb03108366e1/solarcell-0.2.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-01-29 06:22:57",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "amosborne",
"github_project": "solarcell",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "solarcell"
}