# strawberry-jwt-auth
A JWT auth library based on Django and strawberry.
## About
Auth is a flexible, drop-in solution to add authentication and authorization services to your applications. Your team and organization can avoid the cost, time, and risk that come with building your own solution to authenticate and authorize users.
## Features
- **JWT**: The library uses JSON Web Tokens to send information about an authenticated user. This information can be used to authorize access to resources without the need to query the database again.
- **Refresh Tokens**: The library also provides a refresh token to allow users to request a new access token without having to re-authenticate.
- **Blacklisting**: Refresh tokens can be blacklisted to prevent them from being used again. This is useful for logging out users or preventing them from accessing resources after a password change.
- **Token Revocation**: Refresh tokens can be revoked to prevent them from being used again. This is useful for logging out users or preventing them from accessing resources after a password change.
- **Token Verification**: The library provides a decorator to verify that a token is valid and has not been tampered with.
- **Fresh Login Requirement**: The library provides a decorator to verify that a user has logged in recently. This is useful for sensitive actions like changing a password or adding a new device to your account.
- **User Identity Lookup**: The library provides a method to look up a user's identity (userID) from the access token.
- **Cookie Storage**: The library stores the JWT in an HTTP-only cookie to prevent it from being accessed by JavaScript. This is useful for SPAs that use cookies for authentication.
## Installation
1. Install the package from PyPI:
```bash
pip install strawberry-jwt-auth
```
2. Add `strawberry_jwt_auth` to your `INSTALLED_APPS`:
```python
INSTALLED_APPS = [
...
"strawberry_jwt_auth",
]
```
3. Migrate the database:
```bash
python manage.py migrate
```
4. Add `strawberry_jwt_auth.extensions.JWTAuthExtension` to your strawberry schema extensions:-
```python
from strawberry_jwt_auth.extension import JWTExtension
schema = strawberry.Schema(
query=Query,
mutation=Mutation,
extensions = [
...
JWTExtension,
]
)
```
5. Wrap your Strawberry GraphQL view with `strawberry_jwt_auth.view.auth_enabled_view`:
```python
from strawberry_jwt_auth.views import strawberry_auth_view
urlpatterns = [
...
path('graphql/', strawberry_auth_view(GraphQLView.as_view(schema=schema))),
),
]
```
6. Add Attributes to your login mutation:
```python
@strawberry.mutation
def login(self, info, email: str, password: str) -> bool:
# Your Authentication logic goes here
setattr(info.context, "userID", user.id)
setattr(info.context.request, "issueNewTokens", True)
setattr(info.context.request, "clientID", user.id)
return True
```
7. Add Attributes to your logout mutation:
```python
@strawberry.mutation
def logout(self, info) -> bool:
# Your logout logic goes here
setattr(info.context.request, "revokeTokens", True)
return True
```
8. ( Optional ) Add login_required decorator to your mutations:
```python
from strawberry_jwt_auth.decorator import login_required
@strawberry.mutation
@login_required
def change_password(self, info, old_password: str, new_password: str) -> bool:
# Your change password logic goes here
return True
```
9. ( Optional ) Add Authentication backend to your settings.py:
```python
# Use this backend to authenticate users using their email and password
AUTHENTICATION_BACKENDS = ['strawberry_jwt_auth.utils.authentication.AuthenticationBackend']
```
## Working
Read about Auth0 [here](https://auth0.com/docs)
## Working Examples
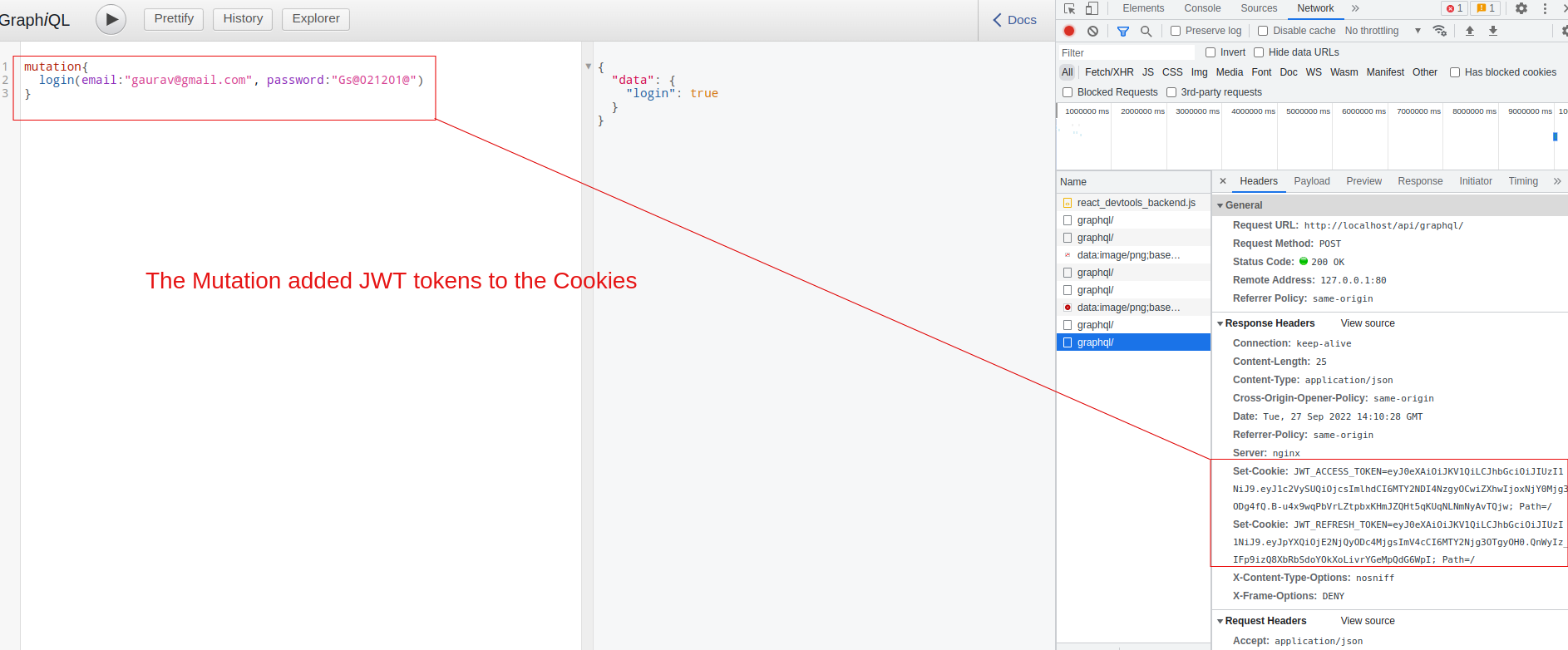
## Future Plans
- [ ] Add support for customizing fields like cookies name, token expiry, etc.
- [ ] Add support for customizing the refresh token model.
- [ ] Add support for more decorators like `fresh_login_required` and `roles_required`.
- [ ] Add support for customizing the user identity lookup.
Raw data
{
"_id": null,
"home_page": "",
"name": "strawberry-jwt-auth",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "python,jwt,auth,strawberry,graphql,Authentication",
"author": "grvsh02 (Gaurav Sharma)",
"author_email": "<gaurav021201@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/f0/e6/8ef84ab205f0ddadd126cf21442905e0400afa4d5b11ca9d76044c65a183/strawberry_jwt_auth-0.2.3.tar.gz",
"platform": null,
"description": "\n# strawberry-jwt-auth\n\nA JWT auth library based on Django and strawberry.\n\n## About\nAuth is a flexible, drop-in solution to add authentication and authorization services to your applications. Your team and organization can avoid the cost, time, and risk that come with building your own solution to authenticate and authorize users.\n\n## Features\n- **JWT**: The library uses JSON Web Tokens to send information about an authenticated user. This information can be used to authorize access to resources without the need to query the database again.\n- **Refresh Tokens**: The library also provides a refresh token to allow users to request a new access token without having to re-authenticate.\n- **Blacklisting**: Refresh tokens can be blacklisted to prevent them from being used again. This is useful for logging out users or preventing them from accessing resources after a password change.\n- **Token Revocation**: Refresh tokens can be revoked to prevent them from being used again. This is useful for logging out users or preventing them from accessing resources after a password change.\n- **Token Verification**: The library provides a decorator to verify that a token is valid and has not been tampered with.\n- **Fresh Login Requirement**: The library provides a decorator to verify that a user has logged in recently. This is useful for sensitive actions like changing a password or adding a new device to your account.\n- **User Identity Lookup**: The library provides a method to look up a user's identity (userID) from the access token.\n- **Cookie Storage**: The library stores the JWT in an HTTP-only cookie to prevent it from being accessed by JavaScript. This is useful for SPAs that use cookies for authentication.\n\n## Installation\n1. Install the package from PyPI:\n```bash\npip install strawberry-jwt-auth\n```\n\n2. Add `strawberry_jwt_auth` to your `INSTALLED_APPS`:\n```python\nINSTALLED_APPS = [\n ...\n \"strawberry_jwt_auth\",\n]\n```\n\n3. Migrate the database:\n```bash\npython manage.py migrate\n```\n\n4. Add `strawberry_jwt_auth.extensions.JWTAuthExtension` to your strawberry schema extensions:-\n```python\nfrom strawberry_jwt_auth.extension import JWTExtension\n\nschema = strawberry.Schema(\n query=Query,\n mutation=Mutation,\n extensions = [\n ...\n JWTExtension,\n ]\n)\n```\n\n5. Wrap your Strawberry GraphQL view with `strawberry_jwt_auth.view.auth_enabled_view`:\n```python\nfrom strawberry_jwt_auth.views import strawberry_auth_view \n\nurlpatterns = [\n ...\n path('graphql/', strawberry_auth_view(GraphQLView.as_view(schema=schema))),\n ),\n]\n```\n\n6. Add Attributes to your login mutation:\n```python\n @strawberry.mutation\n def login(self, info, email: str, password: str) -> bool:\n \n # Your Authentication logic goes here\n \n setattr(info.context, \"userID\", user.id)\n setattr(info.context.request, \"issueNewTokens\", True)\n setattr(info.context.request, \"clientID\", user.id)\n return True\n```\n\n7. Add Attributes to your logout mutation:\n```python\n @strawberry.mutation\n def logout(self, info) -> bool:\n \n # Your logout logic goes here\n \n setattr(info.context.request, \"revokeTokens\", True)\n return True\n```\n\n8. ( Optional ) Add login_required decorator to your mutations:\n```python\n from strawberry_jwt_auth.decorator import login_required\n\n @strawberry.mutation\n @login_required\n def change_password(self, info, old_password: str, new_password: str) -> bool:\n \n # Your change password logic goes here\n \n return True\n```\n\n9. ( Optional ) Add Authentication backend to your settings.py:\n```python\n# Use this backend to authenticate users using their email and password\nAUTHENTICATION_BACKENDS = ['strawberry_jwt_auth.utils.authentication.AuthenticationBackend']\n```\n## Working\n Read about Auth0 [here](https://auth0.com/docs)\n\n## Working Examples\n\n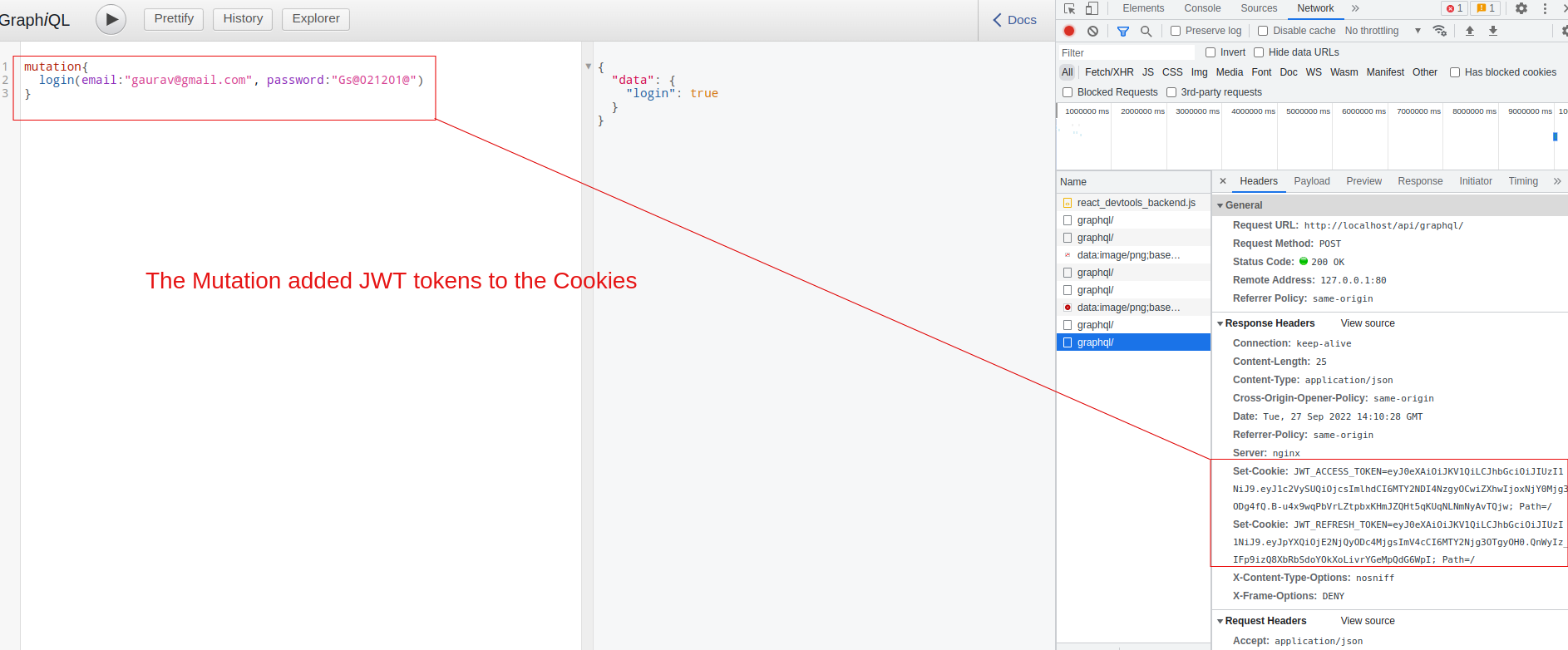\n\n## Future Plans\n- [ ] Add support for customizing fields like cookies name, token expiry, etc.\n- [ ] Add support for customizing the refresh token model.\n- [ ] Add support for more decorators like `fresh_login_required` and `roles_required`.\n- [ ] Add support for customizing the user identity lookup.\n\n\n\n",
"bugtrack_url": null,
"license": "",
"summary": "A JWT auth library based on Django and strawberry",
"version": "0.2.3",
"split_keywords": [
"python",
"jwt",
"auth",
"strawberry",
"graphql",
"authentication"
],
"urls": [
{
"comment_text": "",
"digests": {
"md5": "4439280864f99aadcc6374457bc92596",
"sha256": "c53e62cc02fe9563107ea0ae07e63a80d835fabe9298d525d1163c6c116afe9f"
},
"downloads": -1,
"filename": "strawberry_jwt_auth-0.2.3.tar.gz",
"has_sig": false,
"md5_digest": "4439280864f99aadcc6374457bc92596",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 7255,
"upload_time": "2022-12-09T16:08:13",
"upload_time_iso_8601": "2022-12-09T16:08:13.654580Z",
"url": "https://files.pythonhosted.org/packages/f0/e6/8ef84ab205f0ddadd126cf21442905e0400afa4d5b11ca9d76044c65a183/strawberry_jwt_auth-0.2.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2022-12-09 16:08:13",
"github": false,
"gitlab": false,
"bitbucket": false,
"lcname": "strawberry-jwt-auth"
}