<div align="center">
<p>
<a align="center" href="" target="https://supervision.roboflow.com">
<img
width="100%"
src="https://media.roboflow.com/open-source/supervision/rf-supervision-banner.png?updatedAt=1678995927529"
>
</a>
</p>
<br>
[notebooks](https://github.com/roboflow/notebooks) | [inference](https://github.com/roboflow/inference) | [autodistill](https://github.com/autodistill/autodistill) | [maestro](https://github.com/roboflow/multimodal-maestro)
<br>
[](https://badge.fury.io/py/supervision)
[](https://pypistats.org/packages/supervision)
[](https://github.com/roboflow/supervision/blob/main/LICENSE.md)
[](https://badge.fury.io/py/supervision)
[](https://colab.research.google.com/github/roboflow/supervision/blob/main/demo.ipynb)
[](https://huggingface.co/spaces/Roboflow/Annotators)
[](https://discord.gg/GbfgXGJ8Bk)
[](https://squidfunk.github.io/mkdocs-material/)
</div>
## 👋 hello
**We write your reusable computer vision tools.** Whether you need to load your dataset from your hard drive, draw detections on an image or video, or count how many detections are in a zone. You can count on us! 🤝
[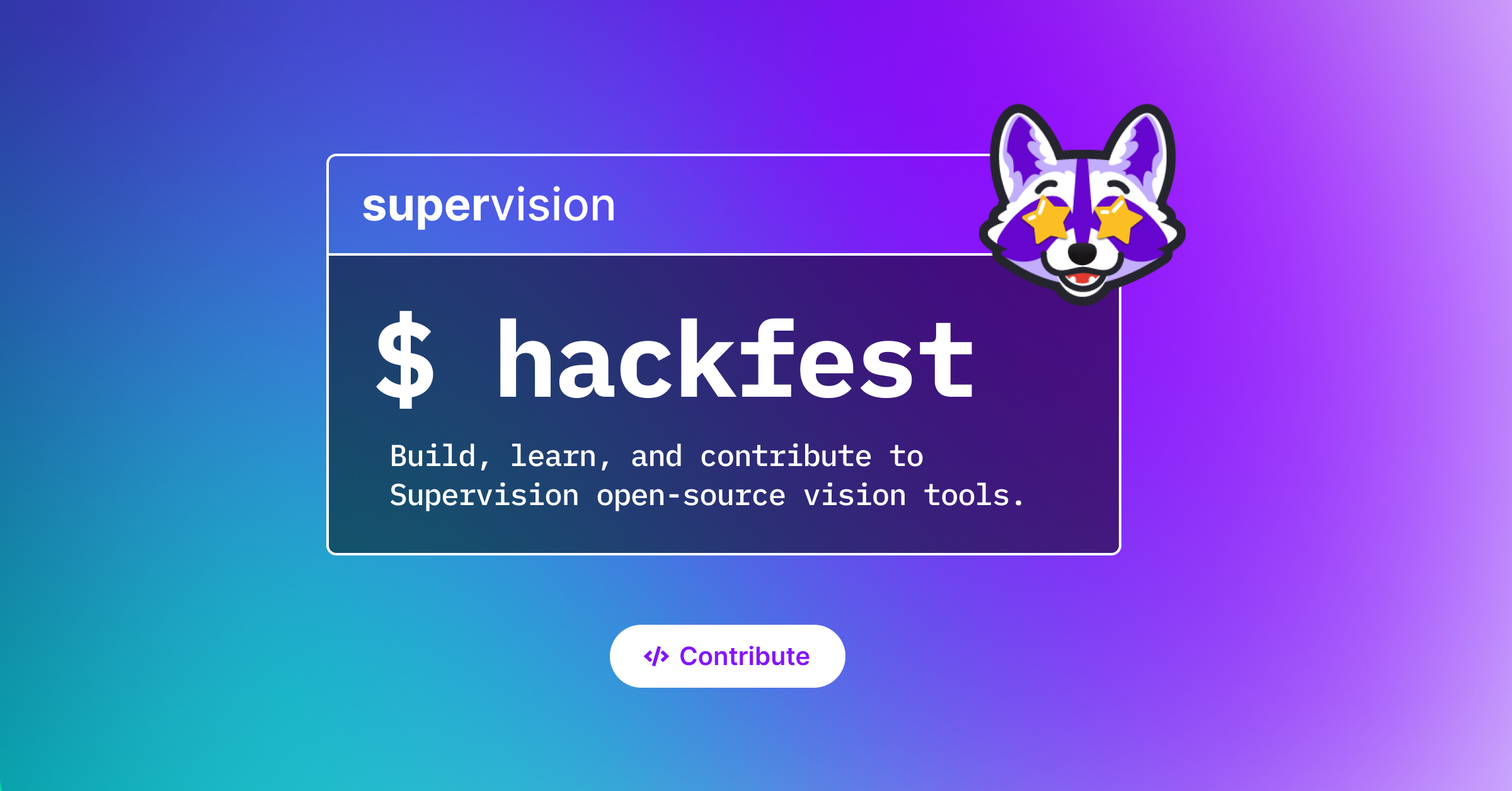](https://github.com/orgs/roboflow/projects/10)
## 💻 install
Pip install the supervision package in a
[**Python>=3.8**](https://www.python.org/) environment.
```bash
pip install supervision
```
Read more about conda, mamba, and installing from source in our [guide](https://roboflow.github.io/supervision/).
## 🔥 quickstart
### models
Supervision was designed to be model agnostic. Just plug in any classification, detection, or segmentation model. For your convenience, we have created [connectors](https://supervision.roboflow.com/latest/detection/core/#detections) for the most popular libraries like Ultralytics, Transformers, or MMDetection.
```python
import cv2
import supervision as sv
from ultralytics import YOLO
image = cv2.imread(...)
model = YOLO('yolov8s.pt')
result = model(image)[0]
detections = sv.Detections.from_ultralytics(result)
len(detections)
# 5
```
<details>
<summary>👉 more model connectors</summary>
- inference
Running with [Inference](https://github.com/roboflow/inference) requires a [Roboflow API KEY](https://docs.roboflow.com/api-reference/authentication#retrieve-an-api-key).
```python
import cv2
import supervision as sv
from inference import get_model
image = cv2.imread(...)
model = get_model(model_id="yolov8s-640", api_key=<ROBOFLOW API KEY>)
result = model.infer(image)[0]
detections = sv.Detections.from_inference(result)
len(detections)
# 5
```
</details>
### annotators
Supervision offers a wide range of highly customizable [annotators](https://supervision.roboflow.com/latest/annotators/), allowing you to compose the perfect visualization for your use case.
```python
import cv2
import supervision as sv
image = cv2.imread(...)
detections = sv.Detections(...)
bounding_box_annotator = sv.BoundingBoxAnnotator()
annotated_frame = bounding_box_annotator.annotate(
scene=image.copy(),
detections=detections
)
```
https://github.com/roboflow/supervision/assets/26109316/691e219c-0565-4403-9218-ab5644f39bce
### datasets
Supervision provides a set of [utils](https://supervision.roboflow.com/latest/datasets/) that allow you to load, split, merge, and save datasets in one of the supported formats.
```python
import supervision as sv
dataset = sv.DetectionDataset.from_yolo(
images_directory_path=...,
annotations_directory_path=...,
data_yaml_path=...
)
dataset.classes
['dog', 'person']
len(dataset)
# 1000
```
<details close>
<summary>👉 more dataset utils</summary>
- load
```python
dataset = sv.DetectionDataset.from_yolo(
images_directory_path=...,
annotations_directory_path=...,
data_yaml_path=...
)
dataset = sv.DetectionDataset.from_pascal_voc(
images_directory_path=...,
annotations_directory_path=...
)
dataset = sv.DetectionDataset.from_coco(
images_directory_path=...,
annotations_path=...
)
```
- split
```python
train_dataset, test_dataset = dataset.split(split_ratio=0.7)
test_dataset, valid_dataset = test_dataset.split(split_ratio=0.5)
len(train_dataset), len(test_dataset), len(valid_dataset)
# (700, 150, 150)
```
- merge
```python
ds_1 = sv.DetectionDataset(...)
len(ds_1)
# 100
ds_1.classes
# ['dog', 'person']
ds_2 = sv.DetectionDataset(...)
len(ds_2)
# 200
ds_2.classes
# ['cat']
ds_merged = sv.DetectionDataset.merge([ds_1, ds_2])
len(ds_merged)
# 300
ds_merged.classes
# ['cat', 'dog', 'person']
```
- save
```python
dataset.as_yolo(
images_directory_path=...,
annotations_directory_path=...,
data_yaml_path=...
)
dataset.as_pascal_voc(
images_directory_path=...,
annotations_directory_path=...
)
dataset.as_coco(
images_directory_path=...,
annotations_path=...
)
```
- convert
```python
sv.DetectionDataset.from_yolo(
images_directory_path=...,
annotations_directory_path=...,
data_yaml_path=...
).as_pascal_voc(
images_directory_path=...,
annotations_directory_path=...
)
```
</details>
## 🎬 tutorials
Want to learn how to use Supervision? Explore our [how-to guides](https://supervision.roboflow.com/develop/how_to/detect_and_annotate/), [end-to-end examples](https://github.com/roboflow/supervision/tree/develop/examples), and [cookbooks](https://supervision.roboflow.com/develop/cookbooks/)!
<br/>
<p align="left">
<a href="https://youtu.be/hAWpsIuem10" title="Dwell Time Analysis with Computer Vision | Real-Time Stream Processing"><img src="https://github.com/SkalskiP/SkalskiP/assets/26109316/a742823d-c158-407d-b30f-063a5d11b4e1" alt="Dwell Time Analysis with Computer Vision | Real-Time Stream Processing" width="300px" align="left" /></a>
<a href="https://youtu.be/hAWpsIuem10" title="Dwell Time Analysis with Computer Vision | Real-Time Stream Processing"><strong>Dwell Time Analysis with Computer Vision | Real-Time Stream Processing</strong></a>
<div><strong>Created: 5 Apr 2024</strong></div>
<br/>Learn how to use computer vision to analyze wait times and optimize processes. This tutorial covers object detection, tracking, and calculating time spent in designated zones. Use these techniques to improve customer experience in retail, traffic management, or other scenarios.</p>
<br/>
<p align="left">
<a href="https://youtu.be/uWP6UjDeZvY" title="Speed Estimation & Vehicle Tracking | Computer Vision | Open Source"><img src="https://github.com/SkalskiP/SkalskiP/assets/26109316/61a444c8-b135-48ce-b979-2a5ab47c5a91" alt="Speed Estimation & Vehicle Tracking | Computer Vision | Open Source" width="300px" align="left" /></a>
<a href="https://youtu.be/uWP6UjDeZvY" title="Speed Estimation & Vehicle Tracking | Computer Vision | Open Source"><strong>Speed Estimation & Vehicle Tracking | Computer Vision | Open Source</strong></a>
<div><strong>Created: 11 Jan 2024</strong></div>
<br/>Learn how to track and estimate the speed of vehicles using YOLO, ByteTrack, and Roboflow Inference. This comprehensive tutorial covers object detection, multi-object tracking, filtering detections, perspective transformation, speed estimation, visualization improvements, and more.</p>
## 💜 built with supervision
Did you build something cool using supervision? [Let us know!](https://github.com/roboflow/supervision/discussions/categories/built-with-supervision)
https://user-images.githubusercontent.com/26109316/207858600-ee862b22-0353-440b-ad85-caa0c4777904.mp4
https://github.com/roboflow/supervision/assets/26109316/c9436828-9fbf-4c25-ae8c-60e9c81b3900
https://github.com/roboflow/supervision/assets/26109316/3ac6982f-4943-4108-9b7f-51787ef1a69f
## 📚 documentation
Visit our [documentation](https://roboflow.github.io/supervision) page to learn how supervision can help you build computer vision applications faster and more reliably.
## 🏆 contribution
We love your input! Please see our [contributing guide](https://github.com/roboflow/supervision/blob/main/CONTRIBUTING.md) to get started. Thank you 🙏 to all our contributors!
<p align="center">
<a href="https://github.com/roboflow/supervision/graphs/contributors">
<img src="https://contrib.rocks/image?repo=roboflow/supervision" />
</a>
</p>
<br>
<div align="center">
<div align="center">
<a href="https://youtube.com/roboflow">
<img
src="https://media.roboflow.com/notebooks/template/icons/purple/youtube.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949634652"
width="3%"
/>
</a>
<img src="https://raw.githubusercontent.com/ultralytics/assets/main/social/logo-transparent.png" width="3%"/>
<a href="https://roboflow.com">
<img
src="https://media.roboflow.com/notebooks/template/icons/purple/roboflow-app.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949746649"
width="3%"
/>
</a>
<img src="https://raw.githubusercontent.com/ultralytics/assets/main/social/logo-transparent.png" width="3%"/>
<a href="https://www.linkedin.com/company/roboflow-ai/">
<img
src="https://media.roboflow.com/notebooks/template/icons/purple/linkedin.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949633691"
width="3%"
/>
</a>
<img src="https://raw.githubusercontent.com/ultralytics/assets/main/social/logo-transparent.png" width="3%"/>
<a href="https://docs.roboflow.com">
<img
src="https://media.roboflow.com/notebooks/template/icons/purple/knowledge.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949634511"
width="3%"
/>
</a>
<img src="https://raw.githubusercontent.com/ultralytics/assets/main/social/logo-transparent.png" width="3%"/>
<a href="https://disuss.roboflow.com">
<img
src="https://media.roboflow.com/notebooks/template/icons/purple/forum.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949633584"
width="3%"
/>
<img src="https://raw.githubusercontent.com/ultralytics/assets/main/social/logo-transparent.png" width="3%"/>
<a href="https://blog.roboflow.com">
<img
src="https://media.roboflow.com/notebooks/template/icons/purple/blog.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949633605"
width="3%"
/>
</a>
</a>
</div>
</div>
Raw data
{
"_id": null,
"home_page": "https://github.com/roboflow/supervision",
"name": "supervision",
"maintainer": "Piotr Skalski",
"docs_url": null,
"requires_python": "<4.0,>=3.8",
"maintainer_email": "piotr.skalski92@gmail.com",
"keywords": "machine-learning, deep-learning, vision, ML, DL, AI, Roboflow",
"author": "Piotr Skalski",
"author_email": "piotr.skalski92@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/5a/60/10cb5bed70a1cc54c2d4686fb3262ffa3c3611be1ef118f6780cb386bbb1/supervision-0.20.0.tar.gz",
"platform": null,
"description": "<div align=\"center\">\n <p>\n <a align=\"center\" href=\"\" target=\"https://supervision.roboflow.com\">\n <img\n width=\"100%\"\n src=\"https://media.roboflow.com/open-source/supervision/rf-supervision-banner.png?updatedAt=1678995927529\"\n >\n </a>\n </p>\n\n <br>\n\n[notebooks](https://github.com/roboflow/notebooks) | [inference](https://github.com/roboflow/inference) | [autodistill](https://github.com/autodistill/autodistill) | [maestro](https://github.com/roboflow/multimodal-maestro)\n\n <br>\n\n[](https://badge.fury.io/py/supervision)\n[](https://pypistats.org/packages/supervision)\n[](https://github.com/roboflow/supervision/blob/main/LICENSE.md)\n[](https://badge.fury.io/py/supervision)\n[](https://colab.research.google.com/github/roboflow/supervision/blob/main/demo.ipynb)\n[](https://huggingface.co/spaces/Roboflow/Annotators)\n[](https://discord.gg/GbfgXGJ8Bk)\n[](https://squidfunk.github.io/mkdocs-material/)\n</div>\n\n## \ud83d\udc4b hello\n\n**We write your reusable computer vision tools.** Whether you need to load your dataset from your hard drive, draw detections on an image or video, or count how many detections are in a zone. You can count on us! \ud83e\udd1d\n\n[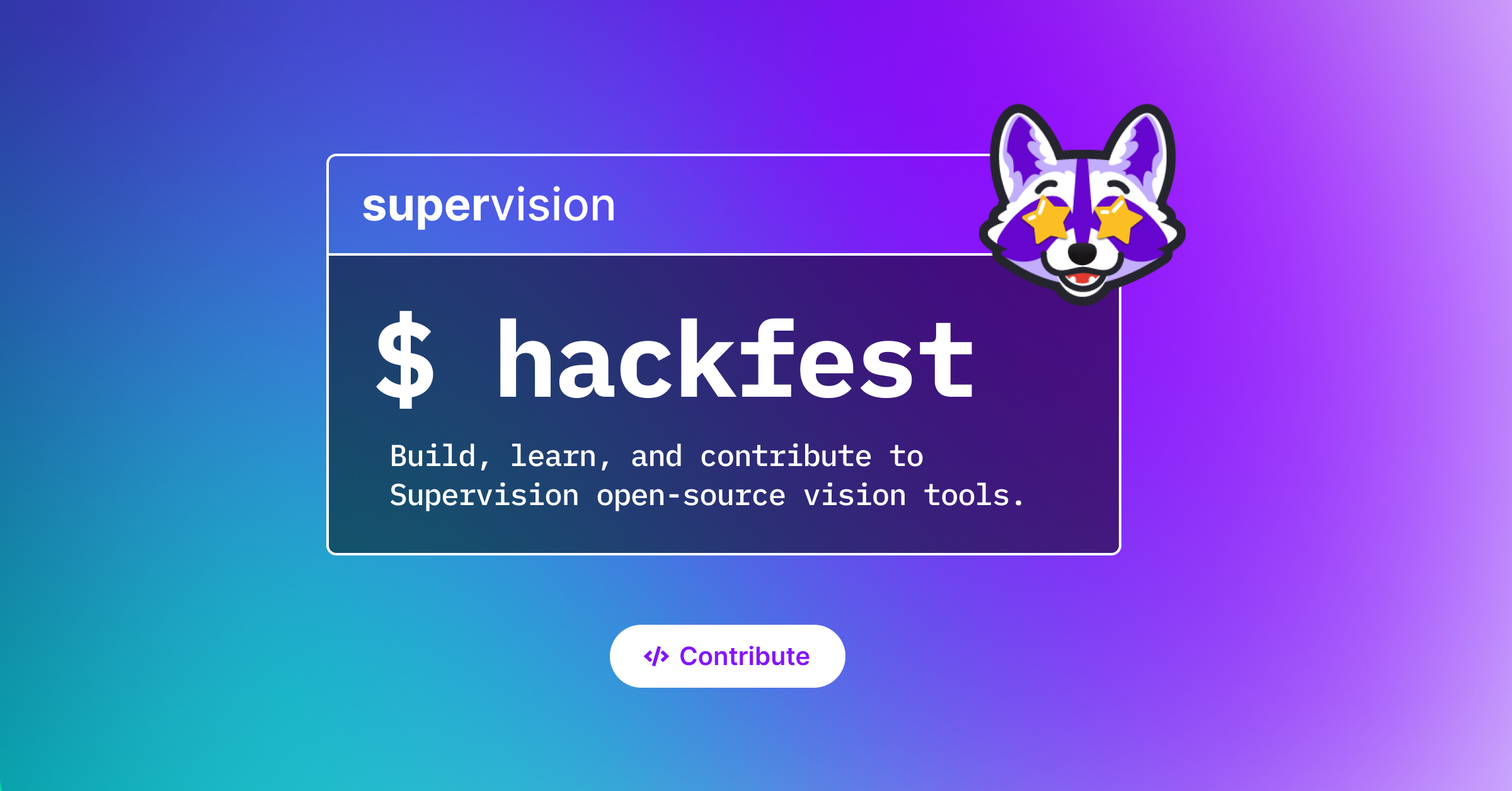](https://github.com/orgs/roboflow/projects/10)\n\n## \ud83d\udcbb install\n\nPip install the supervision package in a\n[**Python>=3.8**](https://www.python.org/) environment.\n\n```bash\npip install supervision\n```\n\nRead more about conda, mamba, and installing from source in our [guide](https://roboflow.github.io/supervision/).\n\n## \ud83d\udd25 quickstart\n\n### models\n\nSupervision was designed to be model agnostic. Just plug in any classification, detection, or segmentation model. For your convenience, we have created [connectors](https://supervision.roboflow.com/latest/detection/core/#detections) for the most popular libraries like Ultralytics, Transformers, or MMDetection.\n\n```python\nimport cv2\nimport supervision as sv\nfrom ultralytics import YOLO\n\nimage = cv2.imread(...)\nmodel = YOLO('yolov8s.pt')\nresult = model(image)[0]\ndetections = sv.Detections.from_ultralytics(result)\n\nlen(detections)\n# 5\n```\n\n<details>\n<summary>\ud83d\udc49 more model connectors</summary>\n\n- inference\n\n Running with [Inference](https://github.com/roboflow/inference) requires a [Roboflow API KEY](https://docs.roboflow.com/api-reference/authentication#retrieve-an-api-key).\n\n ```python\n import cv2\n import supervision as sv\n from inference import get_model\n\n image = cv2.imread(...)\n model = get_model(model_id=\"yolov8s-640\", api_key=<ROBOFLOW API KEY>)\n result = model.infer(image)[0]\n detections = sv.Detections.from_inference(result)\n\n len(detections)\n # 5\n ```\n\n</details>\n\n### annotators\n\nSupervision offers a wide range of highly customizable [annotators](https://supervision.roboflow.com/latest/annotators/), allowing you to compose the perfect visualization for your use case.\n\n```python\nimport cv2\nimport supervision as sv\n\nimage = cv2.imread(...)\ndetections = sv.Detections(...)\n\nbounding_box_annotator = sv.BoundingBoxAnnotator()\nannotated_frame = bounding_box_annotator.annotate(\n scene=image.copy(),\n detections=detections\n)\n```\n\nhttps://github.com/roboflow/supervision/assets/26109316/691e219c-0565-4403-9218-ab5644f39bce\n\n### datasets\n\nSupervision provides a set of [utils](https://supervision.roboflow.com/latest/datasets/) that allow you to load, split, merge, and save datasets in one of the supported formats.\n\n```python\nimport supervision as sv\n\ndataset = sv.DetectionDataset.from_yolo(\n images_directory_path=...,\n annotations_directory_path=...,\n data_yaml_path=...\n)\n\ndataset.classes\n['dog', 'person']\n\nlen(dataset)\n#\u00a01000\n```\n\n<details close>\n<summary>\ud83d\udc49 more dataset utils</summary>\n\n- load\n\n ```python\n dataset = sv.DetectionDataset.from_yolo(\n images_directory_path=...,\n annotations_directory_path=...,\n data_yaml_path=...\n )\n\n dataset = sv.DetectionDataset.from_pascal_voc(\n images_directory_path=...,\n annotations_directory_path=...\n )\n\n dataset = sv.DetectionDataset.from_coco(\n images_directory_path=...,\n annotations_path=...\n )\n ```\n\n- split\n\n ```python\n train_dataset, test_dataset = dataset.split(split_ratio=0.7)\n test_dataset, valid_dataset = test_dataset.split(split_ratio=0.5)\n\n len(train_dataset), len(test_dataset), len(valid_dataset)\n #\u00a0(700, 150, 150)\n ```\n\n- merge\n\n ```python\n ds_1 = sv.DetectionDataset(...)\n len(ds_1)\n #\u00a0100\n ds_1.classes\n #\u00a0['dog', 'person']\n\n ds_2 = sv.DetectionDataset(...)\n len(ds_2)\n # 200\n ds_2.classes\n #\u00a0['cat']\n\n ds_merged = sv.DetectionDataset.merge([ds_1, ds_2])\n len(ds_merged)\n #\u00a0300\n ds_merged.classes\n #\u00a0['cat', 'dog', 'person']\n ```\n\n- save\n\n ```python\n dataset.as_yolo(\n images_directory_path=...,\n annotations_directory_path=...,\n data_yaml_path=...\n )\n\n dataset.as_pascal_voc(\n images_directory_path=...,\n annotations_directory_path=...\n )\n\n dataset.as_coco(\n images_directory_path=...,\n annotations_path=...\n )\n ```\n\n- convert\n\n ```python\n sv.DetectionDataset.from_yolo(\n images_directory_path=...,\n annotations_directory_path=...,\n data_yaml_path=...\n ).as_pascal_voc(\n images_directory_path=...,\n annotations_directory_path=...\n )\n ```\n\n</details>\n\n## \ud83c\udfac tutorials\n\nWant to learn how to use Supervision? Explore our [how-to guides](https://supervision.roboflow.com/develop/how_to/detect_and_annotate/), [end-to-end examples](https://github.com/roboflow/supervision/tree/develop/examples), and [cookbooks](https://supervision.roboflow.com/develop/cookbooks/)!\n\n<br/>\n\n<p align=\"left\">\n<a href=\"https://youtu.be/hAWpsIuem10\" title=\"Dwell Time Analysis with Computer Vision | Real-Time Stream Processing\"><img src=\"https://github.com/SkalskiP/SkalskiP/assets/26109316/a742823d-c158-407d-b30f-063a5d11b4e1\" alt=\"Dwell Time Analysis with Computer Vision | Real-Time Stream Processing\" width=\"300px\" align=\"left\" /></a>\n<a href=\"https://youtu.be/hAWpsIuem10\" title=\"Dwell Time Analysis with Computer Vision | Real-Time Stream Processing\"><strong>Dwell Time Analysis with Computer Vision | Real-Time Stream Processing</strong></a>\n<div><strong>Created: 5 Apr 2024</strong></div>\n<br/>Learn how to use computer vision to analyze wait times and optimize processes. This tutorial covers object detection, tracking, and calculating time spent in designated zones. Use these techniques to improve customer experience in retail, traffic management, or other scenarios.</p>\n\n<br/>\n\n<p align=\"left\">\n<a href=\"https://youtu.be/uWP6UjDeZvY\" title=\"Speed Estimation & Vehicle Tracking | Computer Vision | Open Source\"><img src=\"https://github.com/SkalskiP/SkalskiP/assets/26109316/61a444c8-b135-48ce-b979-2a5ab47c5a91\" alt=\"Speed Estimation & Vehicle Tracking | Computer Vision | Open Source\" width=\"300px\" align=\"left\" /></a>\n<a href=\"https://youtu.be/uWP6UjDeZvY\" title=\"Speed Estimation & Vehicle Tracking | Computer Vision | Open Source\"><strong>Speed Estimation & Vehicle Tracking | Computer Vision | Open Source</strong></a>\n<div><strong>Created: 11 Jan 2024</strong></div>\n<br/>Learn how to track and estimate the speed of vehicles using YOLO, ByteTrack, and Roboflow Inference. This comprehensive tutorial covers object detection, multi-object tracking, filtering detections, perspective transformation, speed estimation, visualization improvements, and more.</p>\n\n## \ud83d\udc9c built with supervision\n\nDid you build something cool using supervision? [Let us know!](https://github.com/roboflow/supervision/discussions/categories/built-with-supervision)\n\nhttps://user-images.githubusercontent.com/26109316/207858600-ee862b22-0353-440b-ad85-caa0c4777904.mp4\n\nhttps://github.com/roboflow/supervision/assets/26109316/c9436828-9fbf-4c25-ae8c-60e9c81b3900\n\nhttps://github.com/roboflow/supervision/assets/26109316/3ac6982f-4943-4108-9b7f-51787ef1a69f\n\n## \ud83d\udcda documentation\n\nVisit our [documentation](https://roboflow.github.io/supervision) page to learn how supervision can help you build computer vision applications faster and more reliably.\n\n## \ud83c\udfc6 contribution\n\nWe love your input! Please see our [contributing guide](https://github.com/roboflow/supervision/blob/main/CONTRIBUTING.md) to get started. Thank you \ud83d\ude4f to all our contributors!\n\n<p align=\"center\">\n <a href=\"https://github.com/roboflow/supervision/graphs/contributors\">\n <img src=\"https://contrib.rocks/image?repo=roboflow/supervision\" />\n </a>\n</p>\n\n<br>\n\n<div align=\"center\">\n\n <div align=\"center\">\n <a href=\"https://youtube.com/roboflow\">\n <img\n src=\"https://media.roboflow.com/notebooks/template/icons/purple/youtube.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949634652\"\n width=\"3%\"\n />\n </a>\n <img src=\"https://raw.githubusercontent.com/ultralytics/assets/main/social/logo-transparent.png\" width=\"3%\"/>\n <a href=\"https://roboflow.com\">\n <img\n src=\"https://media.roboflow.com/notebooks/template/icons/purple/roboflow-app.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949746649\"\n width=\"3%\"\n />\n </a>\n <img src=\"https://raw.githubusercontent.com/ultralytics/assets/main/social/logo-transparent.png\" width=\"3%\"/>\n <a href=\"https://www.linkedin.com/company/roboflow-ai/\">\n <img\n src=\"https://media.roboflow.com/notebooks/template/icons/purple/linkedin.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949633691\"\n width=\"3%\"\n />\n </a>\n <img src=\"https://raw.githubusercontent.com/ultralytics/assets/main/social/logo-transparent.png\" width=\"3%\"/>\n <a href=\"https://docs.roboflow.com\">\n <img\n src=\"https://media.roboflow.com/notebooks/template/icons/purple/knowledge.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949634511\"\n width=\"3%\"\n />\n </a>\n <img src=\"https://raw.githubusercontent.com/ultralytics/assets/main/social/logo-transparent.png\" width=\"3%\"/>\n <a href=\"https://disuss.roboflow.com\">\n <img\n src=\"https://media.roboflow.com/notebooks/template/icons/purple/forum.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949633584\"\n width=\"3%\"\n />\n <img src=\"https://raw.githubusercontent.com/ultralytics/assets/main/social/logo-transparent.png\" width=\"3%\"/>\n <a href=\"https://blog.roboflow.com\">\n <img\n src=\"https://media.roboflow.com/notebooks/template/icons/purple/blog.png?ik-sdk-version=javascript-1.4.3&updatedAt=1672949633605\"\n width=\"3%\"\n />\n </a>\n </a>\n </div>\n</div>\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A set of easy-to-use utils that will come in handy in any Computer Vision project",
"version": "0.20.0",
"project_urls": {
"Documentation": "https://github.com/roboflow/supervision/blob/main/README.md",
"Homepage": "https://github.com/roboflow/supervision",
"Repository": "https://github.com/roboflow/supervision"
},
"split_keywords": [
"machine-learning",
" deep-learning",
" vision",
" ml",
" dl",
" ai",
" roboflow"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "501a971a415d67011854c2c59716c49b3de151d54b422c6dd0a6489e0152b42e",
"md5": "0f39ffb7eac8c4731883ba453fc35e3b",
"sha256": "58a91375b65fe05222ce1dc10a2c06095a6700b87bad5d8b52c333b90b330dff"
},
"downloads": -1,
"filename": "supervision-0.20.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "0f39ffb7eac8c4731883ba453fc35e3b",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.8",
"size": 110951,
"upload_time": "2024-04-24T17:38:38",
"upload_time_iso_8601": "2024-04-24T17:38:38.742623Z",
"url": "https://files.pythonhosted.org/packages/50/1a/971a415d67011854c2c59716c49b3de151d54b422c6dd0a6489e0152b42e/supervision-0.20.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5a6010cb5bed70a1cc54c2d4686fb3262ffa3c3611be1ef118f6780cb386bbb1",
"md5": "f4dffcb090683bb3d6fa54c72b376c1c",
"sha256": "2e8ca93b5bf854e8f0e01813f8789dc76251267032f745de90654f2d900213c2"
},
"downloads": -1,
"filename": "supervision-0.20.0.tar.gz",
"has_sig": false,
"md5_digest": "f4dffcb090683bb3d6fa54c72b376c1c",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.8",
"size": 94410,
"upload_time": "2024-04-24T17:38:40",
"upload_time_iso_8601": "2024-04-24T17:38:40.775632Z",
"url": "https://files.pythonhosted.org/packages/5a/60/10cb5bed70a1cc54c2d4686fb3262ffa3c3611be1ef118f6780cb386bbb1/supervision-0.20.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-04-24 17:38:40",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "roboflow",
"github_project": "supervision",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"tox": true,
"lcname": "supervision"
}