Name | table2ascii JSON |
Version |
1.1.3
JSON |
| download |
home_page | |
Summary | Convert 2D Python lists into Unicode/ASCII tables |
upload_time | 2023-10-20 16:46:43 |
maintainer | |
docs_url | None |
author | |
requires_python | >=3.7 |
license | MIT License Copyright (c) 2021 Jonah Lawrence Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
table
ascii
unicode
formatter
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# table2ascii
[](https://github.com/DenverCoder1/table2ascii/actions/workflows/python-app.yml)
[](https://pypi.org/project/table2ascii/)
[](https://pepy.tech/project/table2ascii)
[](https://github.com/DenverCoder1/table2ascii/blob/main/LICENSE)
[](https://discord.gg/fPrdqh3Zfu)
An intuitive and type-safe library for converting 2D Python lists to fancy ASCII/Unicode tables
Documentation and examples are available at [table2ascii.rtfd.io](https://table2ascii.readthedocs.io/)
## ๐ฅ Installation
`pip install -U table2ascii`
**Requirements:** `Python 3.7+`
## ๐งโ๐ป Usage
### ๐ Convert lists to ASCII tables
```py
from table2ascii import table2ascii
output = table2ascii(
header=["#", "G", "H", "R", "S"],
body=[["1", "30", "40", "35", "30"], ["2", "30", "40", "35", "30"]],
footer=["SUM", "130", "140", "135", "130"],
)
print(output)
"""
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ
โ # G H R S โ
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโข
โ 1 30 40 35 30 โ
โ 2 30 40 35 30 โ
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโข
โ SUM 130 140 135 130 โ
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ
"""
```
### ๐ Set first or last column headings
```py
from table2ascii import table2ascii
output = table2ascii(
body=[["Assignment", "30", "40", "35", "30"], ["Bonus", "10", "20", "5", "10"]],
first_col_heading=True,
)
print(output)
"""
โโโโโโโโโโโโโโฆโโโโโโโโโโโโโโโโโโโโ
โ Assignment โ 30 40 35 30 โ
โ Bonus โ 10 20 5 10 โ
โโโโโโโโโโโโโโฉโโโโโโโโโโโโโโโโโโโโ
"""
```
### ๐ฐ Set column widths and alignments
```py
from table2ascii import table2ascii, Alignment
output = table2ascii(
header=["Product", "Category", "Price", "Rating"],
body=[
["Milk", "Dairy", "$2.99", "6.283"],
["Cheese", "Dairy", "$10.99", "8.2"],
["Apples", "Produce", "$0.99", "10.00"],
],
column_widths=[12, 12, 12, 12],
alignments=[Alignment.LEFT, Alignment.CENTER, Alignment.RIGHT, Alignment.DECIMAL],
)
print(output)
"""
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ
โ Product Category Price Rating โ
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโข
โ Milk Dairy $2.99 6.283 โ
โ Cheese Dairy $10.99 8.2 โ
โ Apples Produce $0.99 10.00 โ
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ
"""
```
### ๐จ Use a preset style
See a list of 30+ preset styles [here](https://table2ascii.readthedocs.io/en/latest/styles.html).
```py
from table2ascii import table2ascii, Alignment, PresetStyle
output = table2ascii(
header=["First", "Second", "Third", "Fourth"],
body=[["10", "30", "40", "35"], ["20", "10", "20", "5"]],
column_widths=[10, 10, 10, 10],
style=PresetStyle.ascii_box
)
print(output)
"""
+----------+----------+----------+----------+
| First | Second | Third | Fourth |
+----------+----------+----------+----------+
| 10 | 30 | 40 | 35 |
+----------+----------+----------+----------+
| 20 | 10 | 20 | 5 |
+----------+----------+----------+----------+
"""
output = table2ascii(
header=["First", "Second", "Third", "Fourth"],
body=[["10", "30", "40", "35"], ["20", "10", "20", "5"]],
style=PresetStyle.plain,
cell_padding=0,
alignments=Alignment.LEFT,
)
print(output)
"""
First Second Third Fourth
10 30 40 35
20 10 20 5
"""
```
### ๐ฒ Define a custom style
Check [`TableStyle`](https://github.com/DenverCoder1/table2ascii/blob/main/table2ascii/table_style.py) for more info and [`PresetStyle`](https://github.com/DenverCoder1/table2ascii/blob/main/table2ascii/preset_style.py) for examples.
```py
from table2ascii import table2ascii, TableStyle
my_style = TableStyle.from_string("*-..*||:+-+:+ *''*")
output = table2ascii(
header=["First", "Second", "Third"],
body=[["10", "30", "40"], ["20", "10", "20"], ["30", "20", "30"]],
style=my_style
)
print(output)
"""
*-------.--------.-------*
| First : Second : Third |
+-------:--------:-------+
| 10 : 30 : 40 |
| 20 : 10 : 20 |
| 30 : 20 : 30 |
*-------'--------'-------*
"""
```
### ๐ช Merge adjacent cells
```py
from table2ascii import table2ascii, Merge, PresetStyle
output = table2ascii(
header=["#", "G", "Merge", Merge.LEFT, "S"],
body=[
[1, 5, 6, 200, Merge.LEFT],
[2, "E", "Long cell", Merge.LEFT, Merge.LEFT],
["Bonus", Merge.LEFT, Merge.LEFT, "F", "G"],
],
footer=["SUM", "100", "200", Merge.LEFT, "300"],
style=PresetStyle.double_thin_box,
first_col_heading=True,
)
print(output)
"""
โโโโโโโฆโโโโโโคโโโโโโโโคโโโโโโ
โ # โ G โ Merge โ S โ
โ โโโโโโฌโโโโโโชโโโโคโโโโงโโโโโโฃ
โ 1 โ 5 โ 6 โ 200 โ
โโโโโโโซโโโโโโผโโโโดโโโโโโโโโโข
โ 2 โ E โ Long cell โ
โโโโโโโจโโโโโโดโโโโฌโโโโฌโโโโโโข
โ Bonus โ F โ G โ
โ โโโโโโฆโโโโโโคโโโโงโโโโชโโโโโโฃ
โ SUM โ 100 โ 200 โ 300 โ
โโโโโโโฉโโโโโโงโโโโโโโโงโโโโโโ
"""
```
## โ๏ธ Options
All parameters are optional. At least one of `header`, `body`, and `footer` must be provided.
Refer to the [documentation](https://table2ascii.readthedocs.io/en/stable/api.html#table2ascii) for more information.
| Option | Supported Types | Description |
| :-----------------: | :-----------------------------------------------------------------------------: | :--------------------------------------------------------------------------------------------------: |
| `header` | `Sequence[SupportsStr]`, `None`<br/>(Default: `None`) | First table row seperated by header row separator. Values should support `str()` |
| `body` | `Sequence[Sequence[SupportsStr]]`, `None`<br/>(Default: `None`) | 2D List of rows for the main section of the table. Values should support `str()` |
| `footer` | `Sequence[SupportsStr]`, `None`<br/>(Default: `None`) | Last table row seperated by header row separator. Values should support `str()` |
| `column_widths` | `Sequence[Optional[int]]`, `None`<br/>(Default: `None` / automatic) | List of column widths in characters for each column |
| `alignments` | `Sequence[Alignment]`, `Alignment`, `None`<br/>(Default: `None` / all centered) | Column alignments<br/>(ex. `[Alignment.LEFT, Alignment.CENTER, Alignment.RIGHT, Alignment.DECIMAL]`) |
| `number_alignments` | `Sequence[Alignment]`, `Alignment`, `None`<br/>(Default: `None`) | Column alignments for numeric values. `alignments` will be used if not specified. |
| `style` | `TableStyle`<br/>(Default: `double_thin_compact`) | Table style to use for the table\* |
| `first_col_heading` | `bool`<br/>(Default: `False`) | Whether to add a heading column separator after the first column |
| `last_col_heading` | `bool`<br/>(Default: `False`) | Whether to add a heading column separator before the last column |
| `cell_padding` | `int`<br/>(Default: `1`) | The minimum number of spaces to add between the cell content and the cell border |
| `use_wcwidth` | `bool`<br/>(Default: `True`) | Whether to use [wcwidth][wcwidth] instead of `len()` to calculate cell width |
[wcwidth]: https://pypi.org/project/wcwidth/
\*See a list of all preset styles [here](https://table2ascii.readthedocs.io/en/latest/styles.html).
See the [API Reference](https://table2ascii.readthedocs.io/en/latest/api.html) for more info.
## ๐จโ๐จ Use cases
### ๐จ๏ธ Discord messages and embeds
- Display tables nicely inside markdown code blocks on Discord
- Useful for making Discord bots with [Discord.py](https://github.com/Rapptz/discord.py)
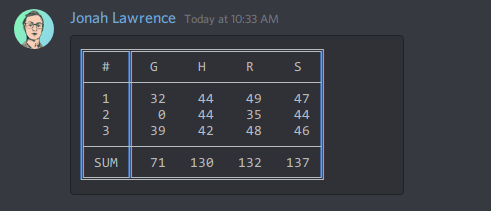
### ๐ป Terminal outputs
- Tables display nicely whenever monospace fonts are fully supported
- Tables make terminal outputs look more professional
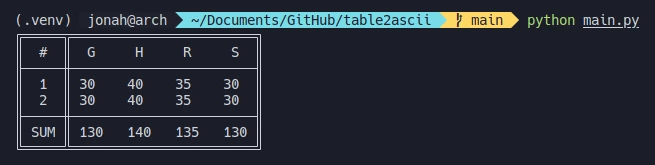
## ๐ค Contributing
Contributions are welcome!
See [CONTRIBUTING.md](https://github.com/DenverCoder1/table2ascii/blob/main/CONTRIBUTING.md) for more details on how to get involved.
Raw data
{
"_id": null,
"home_page": "",
"name": "table2ascii",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "",
"keywords": "table,ascii,unicode,formatter",
"author": "",
"author_email": "Jonah Lawrence <jonah@freshidea.com>",
"download_url": "https://files.pythonhosted.org/packages/6c/3e/939ea977ef0dd9e25801407ff82d1873b7a3753825c22df5f2727dfca94c/table2ascii-1.1.3.tar.gz",
"platform": null,
"description": "# table2ascii\n\n[](https://github.com/DenverCoder1/table2ascii/actions/workflows/python-app.yml)\n[](https://pypi.org/project/table2ascii/)\n[](https://pepy.tech/project/table2ascii)\n[](https://github.com/DenverCoder1/table2ascii/blob/main/LICENSE)\n[](https://discord.gg/fPrdqh3Zfu)\n\nAn intuitive and type-safe library for converting 2D Python lists to fancy ASCII/Unicode tables\n\nDocumentation and examples are available at [table2ascii.rtfd.io](https://table2ascii.readthedocs.io/)\n\n## \ud83d\udce5 Installation\n\n`pip install -U table2ascii`\n\n**Requirements:** `Python 3.7+`\n\n## \ud83e\uddd1\u200d\ud83d\udcbb Usage\n\n### \ud83d\ude80 Convert lists to ASCII tables\n\n```py\nfrom table2ascii import table2ascii\n\noutput = table2ascii(\n header=[\"#\", \"G\", \"H\", \"R\", \"S\"],\n body=[[\"1\", \"30\", \"40\", \"35\", \"30\"], [\"2\", \"30\", \"40\", \"35\", \"30\"]],\n footer=[\"SUM\", \"130\", \"140\", \"135\", \"130\"],\n)\n\nprint(output)\n\n\"\"\"\n\u2554\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2557\n\u2551 # G H R S \u2551\n\u255f\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2562\n\u2551 1 30 40 35 30 \u2551\n\u2551 2 30 40 35 30 \u2551\n\u255f\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2562\n\u2551 SUM 130 140 135 130 \u2551\n\u255a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u255d\n\"\"\"\n```\n\n### \ud83c\udfc6 Set first or last column headings\n\n```py\nfrom table2ascii import table2ascii\n\noutput = table2ascii(\n body=[[\"Assignment\", \"30\", \"40\", \"35\", \"30\"], [\"Bonus\", \"10\", \"20\", \"5\", \"10\"]],\n first_col_heading=True,\n)\n\nprint(output)\n\n\"\"\"\n\u2554\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2566\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2557\n\u2551 Assignment \u2551 30 40 35 30 \u2551\n\u2551 Bonus \u2551 10 20 5 10 \u2551\n\u255a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2569\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u255d\n\"\"\"\n```\n\n### \ud83d\udcf0 Set column widths and alignments\n\n```py\nfrom table2ascii import table2ascii, Alignment\n\noutput = table2ascii(\n header=[\"Product\", \"Category\", \"Price\", \"Rating\"],\n body=[\n [\"Milk\", \"Dairy\", \"$2.99\", \"6.283\"],\n [\"Cheese\", \"Dairy\", \"$10.99\", \"8.2\"],\n [\"Apples\", \"Produce\", \"$0.99\", \"10.00\"],\n ],\n column_widths=[12, 12, 12, 12],\n alignments=[Alignment.LEFT, Alignment.CENTER, Alignment.RIGHT, Alignment.DECIMAL],\n)\n\nprint(output)\n\n\"\"\"\n\u2554\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2557\n\u2551 Product Category Price Rating \u2551\n\u255f\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2562\n\u2551 Milk Dairy $2.99 6.283 \u2551\n\u2551 Cheese Dairy $10.99 8.2 \u2551\n\u2551 Apples Produce $0.99 10.00 \u2551\n\u255a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u255d\n\"\"\"\n```\n\n### \ud83c\udfa8 Use a preset style\n\nSee a list of 30+ preset styles [here](https://table2ascii.readthedocs.io/en/latest/styles.html).\n\n```py\nfrom table2ascii import table2ascii, Alignment, PresetStyle\n\noutput = table2ascii(\n header=[\"First\", \"Second\", \"Third\", \"Fourth\"],\n body=[[\"10\", \"30\", \"40\", \"35\"], [\"20\", \"10\", \"20\", \"5\"]],\n column_widths=[10, 10, 10, 10],\n style=PresetStyle.ascii_box\n)\n\nprint(output)\n\n\"\"\"\n+----------+----------+----------+----------+\n| First | Second | Third | Fourth |\n+----------+----------+----------+----------+\n| 10 | 30 | 40 | 35 |\n+----------+----------+----------+----------+\n| 20 | 10 | 20 | 5 |\n+----------+----------+----------+----------+\n\"\"\"\n\noutput = table2ascii(\n header=[\"First\", \"Second\", \"Third\", \"Fourth\"],\n body=[[\"10\", \"30\", \"40\", \"35\"], [\"20\", \"10\", \"20\", \"5\"]],\n style=PresetStyle.plain,\n cell_padding=0,\n alignments=Alignment.LEFT,\n)\n\nprint(output)\n\n\"\"\"\nFirst Second Third Fourth\n10 30 40 35\n20 10 20 5\n\"\"\"\n```\n\n### \ud83c\udfb2 Define a custom style\n\nCheck [`TableStyle`](https://github.com/DenverCoder1/table2ascii/blob/main/table2ascii/table_style.py) for more info and [`PresetStyle`](https://github.com/DenverCoder1/table2ascii/blob/main/table2ascii/preset_style.py) for examples.\n\n```py\nfrom table2ascii import table2ascii, TableStyle\n\nmy_style = TableStyle.from_string(\"*-..*||:+-+:+ *''*\")\n\noutput = table2ascii(\n header=[\"First\", \"Second\", \"Third\"],\n body=[[\"10\", \"30\", \"40\"], [\"20\", \"10\", \"20\"], [\"30\", \"20\", \"30\"]],\n style=my_style\n)\n\nprint(output)\n\n\"\"\"\n*-------.--------.-------*\n| First : Second : Third |\n+-------:--------:-------+\n| 10 : 30 : 40 |\n| 20 : 10 : 20 |\n| 30 : 20 : 30 |\n*-------'--------'-------*\n\"\"\"\n```\n\n### \ud83e\ude84 Merge adjacent cells\n\n```py\nfrom table2ascii import table2ascii, Merge, PresetStyle\n\noutput = table2ascii(\n header=[\"#\", \"G\", \"Merge\", Merge.LEFT, \"S\"],\n body=[\n [1, 5, 6, 200, Merge.LEFT],\n [2, \"E\", \"Long cell\", Merge.LEFT, Merge.LEFT],\n [\"Bonus\", Merge.LEFT, Merge.LEFT, \"F\", \"G\"],\n ],\n footer=[\"SUM\", \"100\", \"200\", Merge.LEFT, \"300\"],\n style=PresetStyle.double_thin_box,\n first_col_heading=True,\n)\n\nprint(output)\n\n\"\"\"\n\u2554\u2550\u2550\u2550\u2550\u2550\u2566\u2550\u2550\u2550\u2550\u2550\u2564\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2564\u2550\u2550\u2550\u2550\u2550\u2557\n\u2551 # \u2551 G \u2502 Merge \u2502 S \u2551\n\u2560\u2550\u2550\u2550\u2550\u2550\u256c\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2564\u2550\u2550\u2550\u2567\u2550\u2550\u2550\u2550\u2550\u2563\n\u2551 1 \u2551 5 \u2502 6 \u2502 200 \u2551\n\u255f\u2500\u2500\u2500\u2500\u2500\u256b\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2562\n\u2551 2 \u2551 E \u2502 Long cell \u2551\n\u255f\u2500\u2500\u2500\u2500\u2500\u2568\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2562\n\u2551 Bonus \u2502 F \u2502 G \u2551\n\u2560\u2550\u2550\u2550\u2550\u2550\u2566\u2550\u2550\u2550\u2550\u2550\u2564\u2550\u2550\u2550\u2567\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2563\n\u2551 SUM \u2551 100 \u2502 200 \u2502 300 \u2551\n\u255a\u2550\u2550\u2550\u2550\u2550\u2569\u2550\u2550\u2550\u2550\u2550\u2567\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2567\u2550\u2550\u2550\u2550\u2550\u255d\n\"\"\"\n```\n\n## \u2699\ufe0f Options\n\nAll parameters are optional. At least one of `header`, `body`, and `footer` must be provided.\n\nRefer to the [documentation](https://table2ascii.readthedocs.io/en/stable/api.html#table2ascii) for more information.\n\n| Option | Supported Types | Description |\n| :-----------------: | :-----------------------------------------------------------------------------: | :--------------------------------------------------------------------------------------------------: |\n| `header` | `Sequence[SupportsStr]`, `None`<br/>(Default: `None`) | First table row seperated by header row separator. Values should support `str()` |\n| `body` | `Sequence[Sequence[SupportsStr]]`, `None`<br/>(Default: `None`) | 2D List of rows for the main section of the table. Values should support `str()` |\n| `footer` | `Sequence[SupportsStr]`, `None`<br/>(Default: `None`) | Last table row seperated by header row separator. Values should support `str()` |\n| `column_widths` | `Sequence[Optional[int]]`, `None`<br/>(Default: `None` / automatic) | List of column widths in characters for each column |\n| `alignments` | `Sequence[Alignment]`, `Alignment`, `None`<br/>(Default: `None` / all centered) | Column alignments<br/>(ex. `[Alignment.LEFT, Alignment.CENTER, Alignment.RIGHT, Alignment.DECIMAL]`) |\n| `number_alignments` | `Sequence[Alignment]`, `Alignment`, `None`<br/>(Default: `None`) | Column alignments for numeric values. `alignments` will be used if not specified. |\n| `style` | `TableStyle`<br/>(Default: `double_thin_compact`) | Table style to use for the table\\* |\n| `first_col_heading` | `bool`<br/>(Default: `False`) | Whether to add a heading column separator after the first column |\n| `last_col_heading` | `bool`<br/>(Default: `False`) | Whether to add a heading column separator before the last column |\n| `cell_padding` | `int`<br/>(Default: `1`) | The minimum number of spaces to add between the cell content and the cell border |\n| `use_wcwidth` | `bool`<br/>(Default: `True`) | Whether to use [wcwidth][wcwidth] instead of `len()` to calculate cell width |\n\n[wcwidth]: https://pypi.org/project/wcwidth/\n\n\\*See a list of all preset styles [here](https://table2ascii.readthedocs.io/en/latest/styles.html).\n\nSee the [API Reference](https://table2ascii.readthedocs.io/en/latest/api.html) for more info.\n\n## \ud83d\udc68\u200d\ud83c\udfa8 Use cases\n\n### \ud83d\udde8\ufe0f Discord messages and embeds\n\n- Display tables nicely inside markdown code blocks on Discord\n- Useful for making Discord bots with [Discord.py](https://github.com/Rapptz/discord.py)\n\n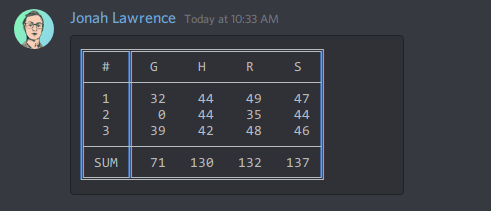\n\n### \ud83d\udcbb Terminal outputs\n\n- Tables display nicely whenever monospace fonts are fully supported\n- Tables make terminal outputs look more professional\n\n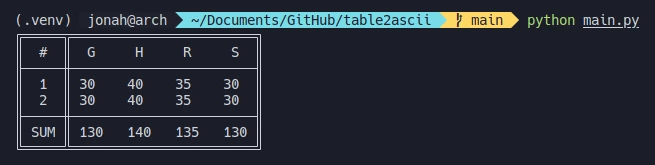\n\n## \ud83e\udd17 Contributing\n\nContributions are welcome!\n\nSee [CONTRIBUTING.md](https://github.com/DenverCoder1/table2ascii/blob/main/CONTRIBUTING.md) for more details on how to get involved.\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2021 Jonah Lawrence Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ",
"summary": "Convert 2D Python lists into Unicode/ASCII tables",
"version": "1.1.3",
"project_urls": {
"documentation": "https://table2ascii.rtfd.io",
"issue-tracker": "https://github.com/DenverCoder1/table2ascii/issues",
"repository": "https://github.com/DenverCoder1/table2ascii"
},
"split_keywords": [
"table",
"ascii",
"unicode",
"formatter"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "6c3e939ea977ef0dd9e25801407ff82d1873b7a3753825c22df5f2727dfca94c",
"md5": "37f50d30d3b23ed156bd043f21d6b63f",
"sha256": "9e28a6b34d6d83ecfa317dd2e8831b185f01cc3c4284c0c0943f074dcaa73a52"
},
"downloads": -1,
"filename": "table2ascii-1.1.3.tar.gz",
"has_sig": false,
"md5_digest": "37f50d30d3b23ed156bd043f21d6b63f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 28290,
"upload_time": "2023-10-20T16:46:43",
"upload_time_iso_8601": "2023-10-20T16:46:43.201984Z",
"url": "https://files.pythonhosted.org/packages/6c/3e/939ea977ef0dd9e25801407ff82d1873b7a3753825c22df5f2727dfca94c/table2ascii-1.1.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-10-20 16:46:43",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "DenverCoder1",
"github_project": "table2ascii",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"tox": true,
"lcname": "table2ascii"
}