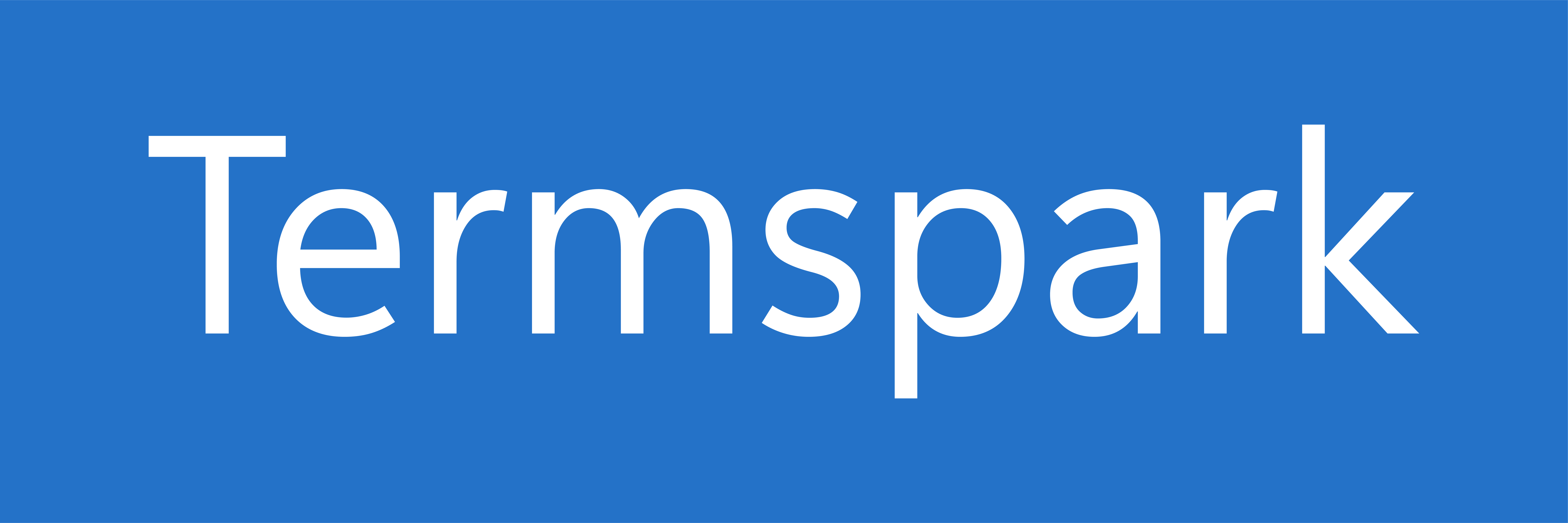
[](https://github.com/faissaloux/termspark/actions/workflows/tests.yml) [](https://codecov.io/gh/faissaloux/termspark) [](https://results.pre-commit.ci/latest/github/faissaloux/termspark/main)   
# Installation
```bash
pip install termspark
```
# Usage
### `print()`, but more!
Import Termspark's print and take advantage of all its features, [colors](#you-can-also-paint-your-content), [highlights](#you-can-also-paint-your-content), [styles](#style), [hyperlinks](#hyperlinks) and more ...
```python
from termspark import print
print(" Termspark ", "white", "blue", "italic")
print(" [@termspark](https://github.com/faissaloux/termspark) ", "black", "white", "italic, bold")
```

You can choose from `["left", "center", "right"]` to specify where to print by passing it as position parameter:
`print(" Termspark ", position="center")`.
You can enable the Full Width using full_width parameter:
`print(" Termspark ", highlight="blue", full_width=True)`.
### `input()`
input with colors, highlights, styles, and hyperlinks.
With `input(position=)` you can specify position where to put your input text `["left", "center", "right"]`.
With `input(full_width=)` you can enable full width `True | False`.
```python
from termspark import input
name = input(" What's your name? ", "white", "blue", "italic", "center", True)
```

#### Input Type
You can specify the input type by passing it to the `type=` parameter.
For a calculation example, to pass the input value into a calculation you don't need to convert it to `int` anymore, you just need to set it from `type` argument 🥳 .
```python
from termspark import input
birthyear = input(" Your year birth? ", "white", "blue", type=int)
print(f"Your age is: {2023 - birthyear}")
```
#### Input Callback
the `input()` supports callback too.
If you need to pass the input value into some function before returning the result, you need to pass it into the `callback=` argument.
```python
from termspark import input
def age_calc(birthyear, currentyear=2023):
return currentyear - birthyear
age = input(" Your year birth? ", "white", "blue", type=int, callback=age_calc)
print(f"Your age is: {age}")
```
### `line()`
To print empty line use `line()`, you can leave it empty or fill it with a repeated character, you can specify its color too.
```python
from termspark import line
line(".", "blue")
line(highlight="green")
line()
line("-")
```

## More control
```python
from termspark import TermSpark
TermSpark().print_right('RIGHT').spark()
TermSpark().spark_right('RIGHT').spark()
TermSpark().print_left('LEFT').spark()
TermSpark().spark_left('LEFT').spark()
TermSpark().print_center('CENTER').spark()
TermSpark().spark_center('CENTER').spark()
TermSpark().line('.').spark()
TermSpark().print_left('LEFT').print_right('RIGHT').set_separator('.').spark()
TermSpark().print_left('LEFT').print_center('CENTER').print_right('RIGHT').set_separator('.').spark()
TermSpark().spark_left('LEFT').spark_center('CENTER').spark_right('RIGHT').set_separator('.').spark()
```
> **Note**
> Separator can contain only one character.
### You can also paint your content
**Supported colors:**
- black
- red
- green
- yellow
- blue
- magenta
- cyan
- white
- gray / grey
- light red
- light green
- light yellow
- light blue
- light magenta
- light cyan
```python
from termspark import TermSpark
TermSpark().print_right('RIGHT', 'blue').spark()
TermSpark().print_left('LEFT', 'light red').spark()
TermSpark().print_center('CENTER', 'light_green').spark()
```
**Supported highlights:**
- black
- red
- green
- yellow
- blue
- magenta
- cyan
- white
- gray / grey
- light red
- light green
- light yellow
- light blue
- light magenta
- light cyan
```python
from termspark import TermSpark
TermSpark().print_right('RIGHT', None, 'light_magenta').spark()
TermSpark().print_left('LEFT', 'red', 'white').spark()
TermSpark().print_center('CENTER', 'white', 'light blue').spark()
```
### You can use different styles on same position
```python
from termspark import TermSpark
TermSpark().spark_left([' * ', 'gray', 'white'], [' Info ', 'white', 'blue']).spark()
TermSpark().spark_center([' * ', 'gray', 'white'], [' Warning ', 'white', 'yellow']).spark()
TermSpark().spark_right([' * ', 'gray', 'white'], [' Error ', 'white', 'red']).spark()
```
`You know you can use them all together 😉`
### Lines are too long to write a termspark line! 😑
```python
from termspark import TermSpark
TermSpark().spark_left([' * ', 'gray', 'white'], [' Info ', 'white', 'blue']).spark_center([' * ', 'gray', 'white'], [' Warning ', 'white', 'yellow']).spark_right([' * ', 'gray', 'white'], [' Error ', 'white', 'red']).spark()
```
#### You can separate them by calling each function in a line 🤤
```python
from termspark import TermSpark
termspark = TermSpark()
termspark.spark_left([' * ', 'gray', 'white'], [' Info ', 'white', 'blue'])
termspark.spark_center([' * ', 'gray', 'white'], [' Warning ', 'white', 'yellow'])
termspark.spark_right([' * ', 'gray', 'white'], [' Error ', 'white', 'red'])
termspark.spark()
```
#### Still too long 🙄 Got you 🤩
```python
from termspark import TermSpark
termspark = TermSpark()
termspark.spark_left([' * ', 'gray', 'white'])
termspark.spark_left(' Info ', 'white', 'blue')
termspark.spark_center([' * ', 'gray', 'white'])
termspark.spark_center([' Warning ', 'white', 'yellow'])
termspark.spark_right(' * ', 'gray', 'white')
termspark.spark_right([' Error ', 'white', 'red'])
termspark.spark()
```
### Raw
You can print raw version which is colors-code-free so you can print clean text into files for example.
```python
from termspark import TermSpark
raw = TermSpark().print_left('LEFT').print_right('RIGHT').set_separator('.').raw()
```
### Force Width
You can customize width instead of the default full terminal width.
```python
from termspark import TermSpark
TermSpark().set_width(40).print_left("LEFT", "red").print_right("RIGHT", "blue").spark()
```
### Set content max width
You can specify max width of content depending on position using `max_[position](max_characters)`.
```python
from termspark import TermSpark
termspark = TermSpark()
termspark.spark_left(["LEFT", "red"])
termspark.spark_right(["RIGHT", "blue"])
termspark.max_left(2)
termspark.max_right(3)
termspark.spark()
```
This should show only "LE" on the left, and "RIG" on the right.
> **Warning**
> `max_[position]()` is only supported by sparkers.
### Full width
You can enable full width by using `full_width()`.
```python
from termspark import TermSpark
termspark = TermSpark()
termspark.spark_center(['Thanks for using Termspark!', 'white', 'green'])
termspark.full_width()
termspark.spark()
```
> **Warning**
> `full_width()` can only be used with one position.
### Separator
You can add color and highlight to separator too using `set_separator(content, color, highlight)`.
```python
termspark = TermSpark()
termspark.spark_left([' Author ', 'green'])
termspark.spark_right([' Faissal Wahabali ', 'green'])
termspark.set_separator('.', 'green')
termspark.spark()
```

### Line
You can add highlight a line by using `line(highlight=highlight)`.
```python
termspark = TermSpark()
termspark.line(highlight='green')
termspark.spark()
```

### Style
You can style your text by passing it to `print() style parameter` or to `spark([]) fourth list element`.
**Supported styles:**
- bold
- dim
- italic
- overline
- underline
- double underline
- strike through
- blink
- reverse
- hidden
> **Note**
> You can mix styles by separating them by commas.
```python
termspark = TermSpark()
termspark.print_center(' Termspark ', 'green', style='underline, overline, italic')
termspark.full_width()
termspark.spark()
```

### Hyperlinks
You can insert hyperlink using Markdown `[TEXT](LINK)`.
```python
termspark = TermSpark()
termspark.spark_left([" Author ", "green"])
termspark.spark_right([" [@faissaloux](https://github.com/faissaloux) ", "green"])
termspark.set_separator(".", "green")
termspark.spark()
```

Raw data
{
"_id": null,
"home_page": "https://github.com/faissaloux/termspark",
"name": "termspark",
"maintainer": "",
"docs_url": null,
"requires_python": "<4,>=3.8",
"maintainer_email": "",
"keywords": "terminal,cmd,design,colorful",
"author": "Faissal Wahabali",
"author_email": "contact@faissaloux.com",
"download_url": "",
"platform": null,
"description": "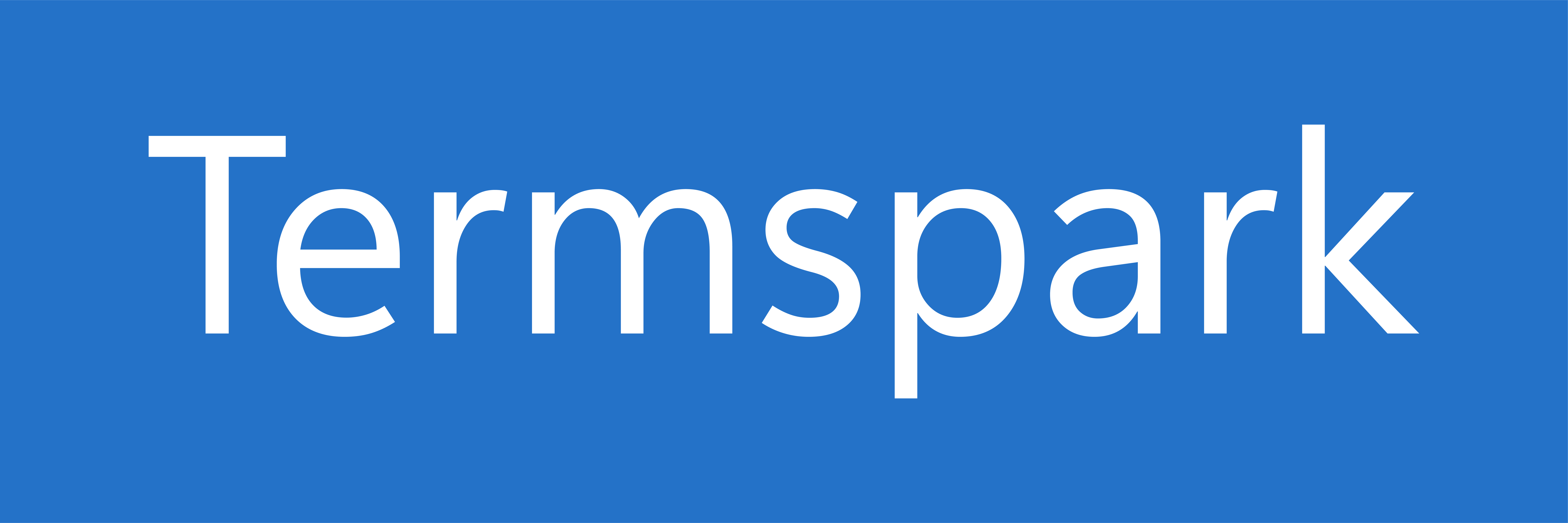\n\n[](https://github.com/faissaloux/termspark/actions/workflows/tests.yml) [](https://codecov.io/gh/faissaloux/termspark) [](https://results.pre-commit.ci/latest/github/faissaloux/termspark/main)   \n\n# Installation\n```bash\n pip install termspark\n```\n\n# Usage\n\n### `print()`, but more!\nImport Termspark's print and take advantage of all its features, [colors](#you-can-also-paint-your-content), [highlights](#you-can-also-paint-your-content), [styles](#style), [hyperlinks](#hyperlinks) and more ...\n\n```python\nfrom termspark import print\n\nprint(\" Termspark \", \"white\", \"blue\", \"italic\")\nprint(\" [@termspark](https://github.com/faissaloux/termspark) \", \"black\", \"white\", \"italic, bold\")\n```\n\n\nYou can choose from `[\"left\", \"center\", \"right\"]` to specify where to print by passing it as position parameter:\n`print(\" Termspark \", position=\"center\")`.\n\nYou can enable the Full Width using full_width parameter:\n`print(\" Termspark \", highlight=\"blue\", full_width=True)`.\n\n### `input()`\n\ninput with colors, highlights, styles, and hyperlinks.\n\nWith `input(position=)` you can specify position where to put your input text `[\"left\", \"center\", \"right\"]`.\nWith `input(full_width=)` you can enable full width `True | False`.\n\n```python\nfrom termspark import input\n\nname = input(\" What's your name? \", \"white\", \"blue\", \"italic\", \"center\", True)\n```\n\n\n\n#### Input Type\nYou can specify the input type by passing it to the `type=` parameter.\n\nFor a calculation example, to pass the input value into a calculation you don't need to convert it to `int` anymore, you just need to set it from `type` argument \ud83e\udd73 .\n```python\nfrom termspark import input\n\nbirthyear = input(\" Your year birth? \", \"white\", \"blue\", type=int)\nprint(f\"Your age is: {2023 - birthyear}\")\n```\n\n#### Input Callback\nthe `input()` supports callback too.\n\nIf you need to pass the input value into some function before returning the result, you need to pass it into the `callback=` argument.\n\n```python\nfrom termspark import input\n\ndef age_calc(birthyear, currentyear=2023):\n return currentyear - birthyear\n\nage = input(\" Your year birth? \", \"white\", \"blue\", type=int, callback=age_calc)\nprint(f\"Your age is: {age}\")\n```\n\n### `line()`\nTo print empty line use `line()`, you can leave it empty or fill it with a repeated character, you can specify its color too.\n\n```python\nfrom termspark import line\n\nline(\".\", \"blue\")\nline(highlight=\"green\")\nline()\nline(\"-\")\n```\n\n\n## More control\n```python\n from termspark import TermSpark\n\n TermSpark().print_right('RIGHT').spark()\n TermSpark().spark_right('RIGHT').spark()\n TermSpark().print_left('LEFT').spark()\n TermSpark().spark_left('LEFT').spark()\n TermSpark().print_center('CENTER').spark()\n TermSpark().spark_center('CENTER').spark()\n TermSpark().line('.').spark()\n\n TermSpark().print_left('LEFT').print_right('RIGHT').set_separator('.').spark()\n TermSpark().print_left('LEFT').print_center('CENTER').print_right('RIGHT').set_separator('.').spark()\n TermSpark().spark_left('LEFT').spark_center('CENTER').spark_right('RIGHT').set_separator('.').spark()\n```\n\n> **Note**\n> Separator can contain only one character.\n\n### You can also paint your content\n\n**Supported colors:**\n- black\n- red\n- green\n- yellow\n- blue\n- magenta\n- cyan\n- white\n- gray / grey\n- light red\n- light green\n- light yellow\n- light blue\n- light magenta\n- light cyan\n\n```python\n from termspark import TermSpark\n\n TermSpark().print_right('RIGHT', 'blue').spark()\n TermSpark().print_left('LEFT', 'light red').spark()\n TermSpark().print_center('CENTER', 'light_green').spark()\n```\n\n**Supported highlights:**\n- black\n- red\n- green\n- yellow\n- blue\n- magenta\n- cyan\n- white\n- gray / grey\n- light red\n- light green\n- light yellow\n- light blue\n- light magenta\n- light cyan\n\n```python\n from termspark import TermSpark\n\n TermSpark().print_right('RIGHT', None, 'light_magenta').spark()\n TermSpark().print_left('LEFT', 'red', 'white').spark()\n TermSpark().print_center('CENTER', 'white', 'light blue').spark()\n```\n\n### You can use different styles on same position\n```python\n from termspark import TermSpark\n\n TermSpark().spark_left([' * ', 'gray', 'white'], [' Info ', 'white', 'blue']).spark()\n TermSpark().spark_center([' * ', 'gray', 'white'], [' Warning ', 'white', 'yellow']).spark()\n TermSpark().spark_right([' * ', 'gray', 'white'], [' Error ', 'white', 'red']).spark()\n```\n`You know you can use them all together \ud83d\ude09`\n\n### Lines are too long to write a termspark line! \ud83d\ude11\n```python\n from termspark import TermSpark\n\n TermSpark().spark_left([' * ', 'gray', 'white'], [' Info ', 'white', 'blue']).spark_center([' * ', 'gray', 'white'], [' Warning ', 'white', 'yellow']).spark_right([' * ', 'gray', 'white'], [' Error ', 'white', 'red']).spark()\n```\n#### You can separate them by calling each function in a line \ud83e\udd24\n```python\n from termspark import TermSpark\n\n termspark = TermSpark()\n termspark.spark_left([' * ', 'gray', 'white'], [' Info ', 'white', 'blue'])\n termspark.spark_center([' * ', 'gray', 'white'], [' Warning ', 'white', 'yellow'])\n termspark.spark_right([' * ', 'gray', 'white'], [' Error ', 'white', 'red'])\n termspark.spark()\n```\n#### Still too long \ud83d\ude44 Got you \ud83e\udd29\n```python\n from termspark import TermSpark\n\n termspark = TermSpark()\n termspark.spark_left([' * ', 'gray', 'white'])\n termspark.spark_left(' Info ', 'white', 'blue')\n termspark.spark_center([' * ', 'gray', 'white'])\n termspark.spark_center([' Warning ', 'white', 'yellow'])\n termspark.spark_right(' * ', 'gray', 'white')\n termspark.spark_right([' Error ', 'white', 'red'])\n termspark.spark()\n```\n\n### Raw\nYou can print raw version which is colors-code-free so you can print clean text into files for example.\n\n```python\n from termspark import TermSpark\n\n raw = TermSpark().print_left('LEFT').print_right('RIGHT').set_separator('.').raw()\n```\n\n### Force Width\nYou can customize width instead of the default full terminal width.\n\n```python\n from termspark import TermSpark\n\n TermSpark().set_width(40).print_left(\"LEFT\", \"red\").print_right(\"RIGHT\", \"blue\").spark()\n```\n\n### Set content max width\nYou can specify max width of content depending on position using `max_[position](max_characters)`.\n```python\n from termspark import TermSpark\n\n termspark = TermSpark()\n termspark.spark_left([\"LEFT\", \"red\"])\n termspark.spark_right([\"RIGHT\", \"blue\"])\n termspark.max_left(2)\n termspark.max_right(3)\n termspark.spark()\n```\nThis should show only \"LE\" on the left, and \"RIG\" on the right.\n> **Warning**\n> `max_[position]()` is only supported by sparkers.\n\n### Full width\nYou can enable full width by using `full_width()`.\n\n```python\n from termspark import TermSpark\n\n termspark = TermSpark()\n termspark.spark_center(['Thanks for using Termspark!', 'white', 'green'])\n termspark.full_width()\n termspark.spark()\n```\n> **Warning**\n> `full_width()` can only be used with one position.\n\n### Separator\nYou can add color and highlight to separator too using `set_separator(content, color, highlight)`.\n```python\ntermspark = TermSpark()\ntermspark.spark_left([' Author ', 'green'])\ntermspark.spark_right([' Faissal Wahabali ', 'green'])\ntermspark.set_separator('.', 'green')\ntermspark.spark()\n```\n\n\n### Line\nYou can add highlight a line by using `line(highlight=highlight)`.\n```python\ntermspark = TermSpark()\ntermspark.line(highlight='green')\ntermspark.spark()\n```\n\n\n### Style\nYou can style your text by passing it to `print() style parameter` or to `spark([]) fourth list element`.\n\n**Supported styles:**\n- bold\n- dim\n- italic\n- overline\n- underline\n- double underline\n- strike through\n- blink\n- reverse\n- hidden\n\n> **Note**\n> You can mix styles by separating them by commas.\n\n```python\ntermspark = TermSpark()\ntermspark.print_center(' Termspark ', 'green', style='underline, overline, italic')\ntermspark.full_width()\ntermspark.spark()\n```\n\n\n### Hyperlinks\nYou can insert hyperlink using Markdown `[TEXT](LINK)`.\n```python\ntermspark = TermSpark()\ntermspark.spark_left([\" Author \", \"green\"])\ntermspark.spark_right([\" [@faissaloux](https://github.com/faissaloux) \", \"green\"])\ntermspark.set_separator(\".\", \"green\")\ntermspark.spark()\n```\n\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Takes control of terminal",
"version": "1.8.1",
"project_urls": {
"Homepage": "https://github.com/faissaloux/termspark"
},
"split_keywords": [
"terminal",
"cmd",
"design",
"colorful"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "96c7332d9a9649596c5255cbcedd956391b543aff6fa3f972d6fee74627c0594",
"md5": "1265df96cc4d7c37217163bc1860c868",
"sha256": "271bab6b344f8df588fb6a4bfa7e1798ee56e74ff9ead450131fa104f3d86c28"
},
"downloads": -1,
"filename": "termspark-1.8.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "1265df96cc4d7c37217163bc1860c868",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4,>=3.8",
"size": 21861,
"upload_time": "2023-12-11T22:43:57",
"upload_time_iso_8601": "2023-12-11T22:43:57.693690Z",
"url": "https://files.pythonhosted.org/packages/96/c7/332d9a9649596c5255cbcedd956391b543aff6fa3f972d6fee74627c0594/termspark-1.8.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-12-11 22:43:57",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "faissaloux",
"github_project": "termspark",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"lcname": "termspark"
}