Name | textual-filedrop JSON |
Version |
0.3.3
JSON |
| download |
home_page | |
Summary | FileDrop widget for Textual, easily drag and drop files into your terminal apps. |
upload_time | 2023-03-20 14:27:48 |
maintainer | |
docs_url | None |
author | agmmnn |
requires_python | >=3.7.8,<4.0.0 |
license | |
keywords |
|
VCS |
|
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
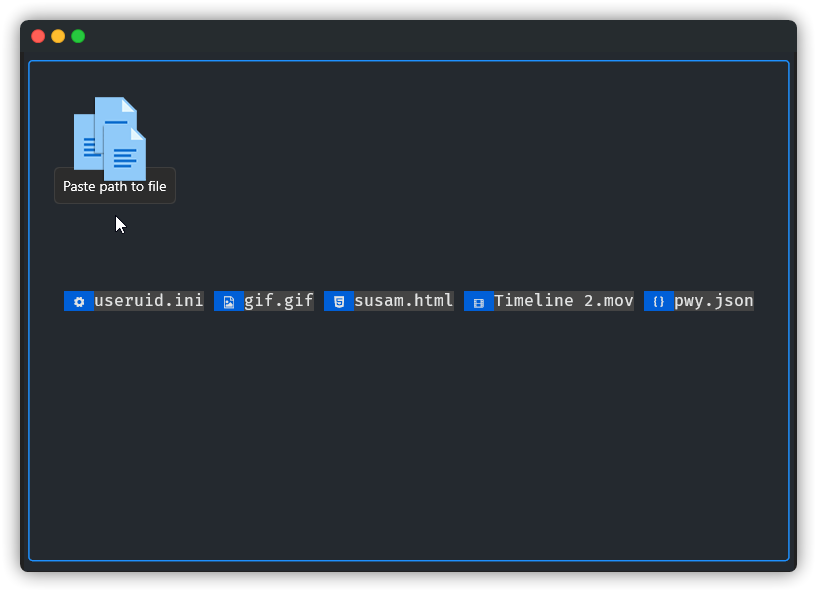
# textual-filedrop
Add filedrop support to your [Textual](https://github.com/textualize/textual/) apps, easily drag and drop files into your terminal apps.
> _Tested on `Windows` and [`macOS`](https://github.com/Textualize/textual/discussions/1414#discussioncomment-4467029)._
> _[Nerd Font](https://www.nerdfonts.com/font-downloads) is required to display file icons._
## Install
```
pip install textual-filedrop
```
or
```
git clone https://github.com/agmmnn/textual-filedrop.git
cd textual-filedrop
poetry install
```
## Note
Since version [0.10.0](https://github.com/Textualize/textual/releases/tag/v0.10.0) Textual supports [bubble](https://textual.textualize.io/guide/events/#bubbling) for the [paste event](https://textual.textualize.io/events/paste/) ([Textualize/textual#1434](https://github.com/Textualize/textual/issues/1434)). So if the terminal where your app is running treats the file drag and drop as a paste event, you can catch it yourself with the `on_paste` function without widget.
## Usage
### `getfiles`
`getfiles` returns an object containing the _path, filename, extension_ and _icon_ of the files.
```py
from textual_filedrop import getfiles
class MyApp(App):
...
def on_paste(self, event) -> None:
files = getfiles(event)
print(files)
```

### `FileDrop` Widget
As long as the `FileDrop` widget is in focus, it will give the information of the dragged files and render the file names with their icons on the screen.
```py
from textual_filedrop import FileDrop
```
```py
# add FileDrop widget to your app
yield FileDrop(id="filedrop")
```
```py
# focus the widget
self.query_one("#filedrop").focus()
```
```py
# when the files are dropped
def on_file_drop_dropped(self, message: FileDrop.Dropped) -> None:
path = message.path
filepaths = message.filepaths
filenames = message.filenames
filesobj = message.filesobj
print(path, filepaths, filenames, filesobj)
# output: path, [filepaths], [filenames], [filesobj]
```
You can find more examples [here](./examples).
## Examples
### [subdomain_lister.py](./examples/subdomain_lister.py)
Drag and drop the subdomain list files and see the results as a tree list.
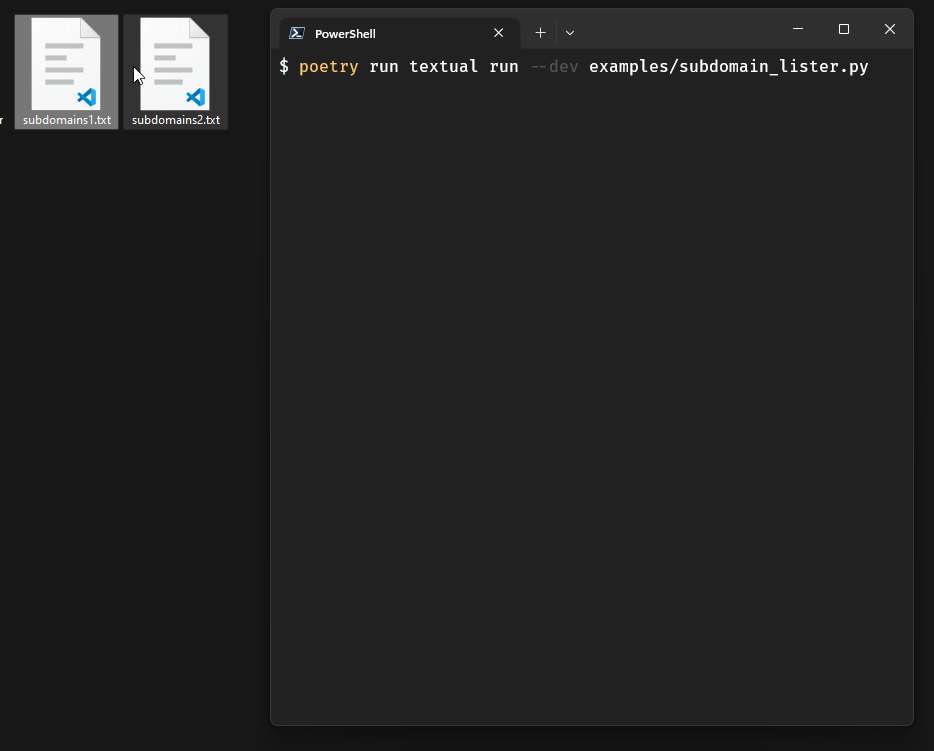
### [fullscreen.py](./examples/fullscreen.py)
Fullscreen example, will show the results in the textual console.
### [hidden.py](./examples/hidden.py)
As long as focus is on, the `FileDrop` widget will be active even if it is not visible on the screen.
### [without_widget.py](./examples/without_widget.py)
An example that renders the object with the information of the dragged files returned from the `getfiles` function to the screen with `rich.json`.
## Dev
```
poetry install
textual console
poetry run textual run --dev examples/subdomain_lister.py
```
Raw data
{
"_id": null,
"home_page": "",
"name": "textual-filedrop",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7.8,<4.0.0",
"maintainer_email": "",
"keywords": "",
"author": "agmmnn",
"author_email": "agmmnn@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/bc/3b/c4e4092e694f9d370ea0babf5b90b2733c77c2e9760fd8e8672a7669b3b9/textual_filedrop-0.3.3.tar.gz",
"platform": null,
"description": "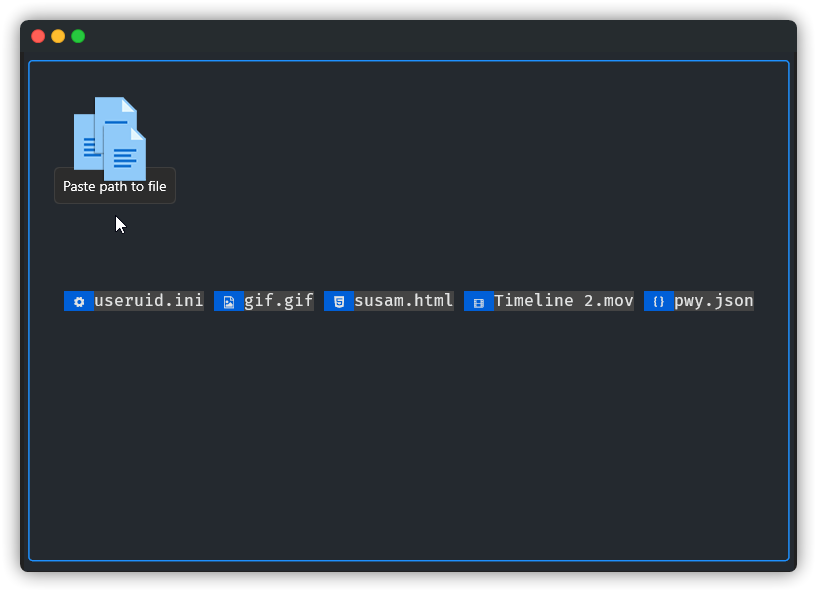\n\n# textual-filedrop\n\nAdd filedrop support to your [Textual](https://github.com/textualize/textual/) apps, easily drag and drop files into your terminal apps.\n\n> _Tested on `Windows` and [`macOS`](https://github.com/Textualize/textual/discussions/1414#discussioncomment-4467029)._\n\n> _[Nerd Font](https://www.nerdfonts.com/font-downloads) is required to display file icons._\n\n## Install\n\n```\npip install textual-filedrop\n```\n\nor\n\n```\ngit clone https://github.com/agmmnn/textual-filedrop.git\ncd textual-filedrop\npoetry install\n```\n\n## Note\n\nSince version [0.10.0](https://github.com/Textualize/textual/releases/tag/v0.10.0) Textual supports [bubble](https://textual.textualize.io/guide/events/#bubbling) for the [paste event](https://textual.textualize.io/events/paste/) ([Textualize/textual#1434](https://github.com/Textualize/textual/issues/1434)). So if the terminal where your app is running treats the file drag and drop as a paste event, you can catch it yourself with the `on_paste` function without widget.\n\n## Usage\n\n### `getfiles`\n\n`getfiles` returns an object containing the _path, filename, extension_ and _icon_ of the files.\n\n```py\nfrom textual_filedrop import getfiles\n\nclass MyApp(App):\n...\n def on_paste(self, event) -> None:\n files = getfiles(event)\n print(files)\n```\n\n\n\n### `FileDrop` Widget\n\nAs long as the `FileDrop` widget is in focus, it will give the information of the dragged files and render the file names with their icons on the screen.\n\n```py\nfrom textual_filedrop import FileDrop\n```\n\n```py\n# add FileDrop widget to your app\nyield FileDrop(id=\"filedrop\")\n```\n\n```py\n# focus the widget\nself.query_one(\"#filedrop\").focus()\n```\n\n```py\n# when the files are dropped\ndef on_file_drop_dropped(self, message: FileDrop.Dropped) -> None:\n path = message.path\n filepaths = message.filepaths\n filenames = message.filenames\n filesobj = message.filesobj\n print(path, filepaths, filenames, filesobj)\n\n\n# output: path, [filepaths], [filenames], [filesobj]\n```\n\nYou can find more examples [here](./examples).\n\n## Examples\n\n### [subdomain_lister.py](./examples/subdomain_lister.py)\n\nDrag and drop the subdomain list files and see the results as a tree list.\n\n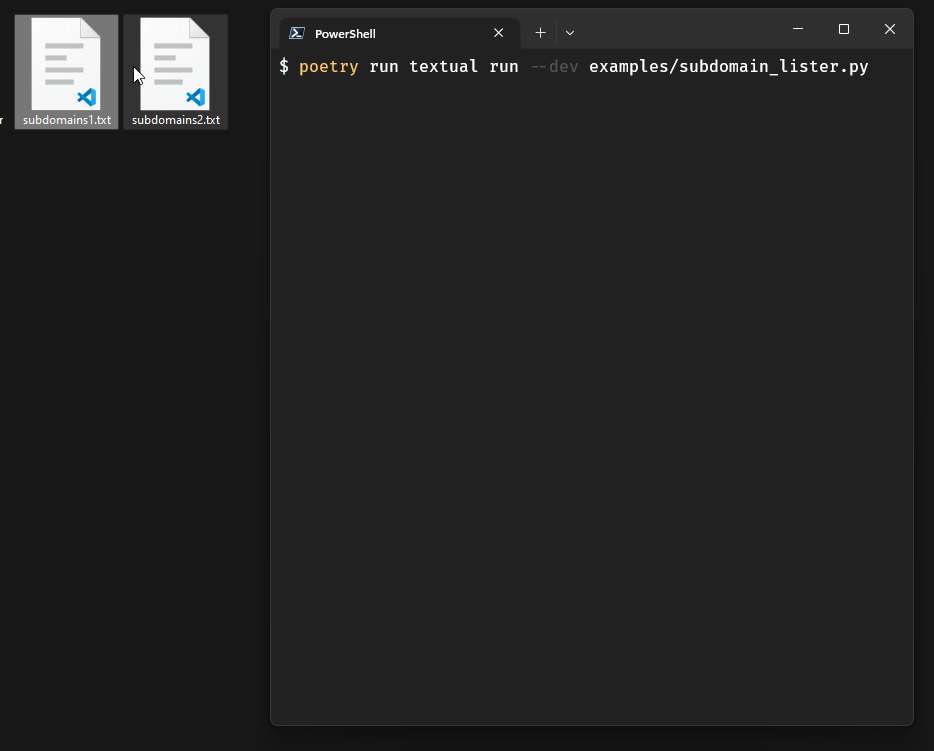\n\n### [fullscreen.py](./examples/fullscreen.py)\n\nFullscreen example, will show the results in the textual console.\n\n### [hidden.py](./examples/hidden.py)\n\nAs long as focus is on, the `FileDrop` widget will be active even if it is not visible on the screen.\n\n### [without_widget.py](./examples/without_widget.py)\n\nAn example that renders the object with the information of the dragged files returned from the `getfiles` function to the screen with `rich.json`.\n\n## Dev\n\n```\npoetry install\n\ntextual console\npoetry run textual run --dev examples/subdomain_lister.py\n```\n",
"bugtrack_url": null,
"license": "",
"summary": "FileDrop widget for Textual, easily drag and drop files into your terminal apps.",
"version": "0.3.3",
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "5ad027261d4ca1a4a2e63aceead6efcabecb237b7b7df0dc805793dc3482b914",
"md5": "650407adee4e02f626396b285f78a0dc",
"sha256": "e330b6d4e55e230dd37608b5b29b33ccfa3993dedc4c95fcc06e92ca2452aa8c"
},
"downloads": -1,
"filename": "textual_filedrop-0.3.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "650407adee4e02f626396b285f78a0dc",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7.8,<4.0.0",
"size": 7826,
"upload_time": "2023-03-20T14:27:46",
"upload_time_iso_8601": "2023-03-20T14:27:46.912330Z",
"url": "https://files.pythonhosted.org/packages/5a/d0/27261d4ca1a4a2e63aceead6efcabecb237b7b7df0dc805793dc3482b914/textual_filedrop-0.3.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "bc3bc4e4092e694f9d370ea0babf5b90b2733c77c2e9760fd8e8672a7669b3b9",
"md5": "44314ff82f82e1bc2d36d5caa341393c",
"sha256": "8f0ddf38b615072820b89bd727a71353afa1223be54889cb0cf64d8ebae93888"
},
"downloads": -1,
"filename": "textual_filedrop-0.3.3.tar.gz",
"has_sig": false,
"md5_digest": "44314ff82f82e1bc2d36d5caa341393c",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7.8,<4.0.0",
"size": 7714,
"upload_time": "2023-03-20T14:27:48",
"upload_time_iso_8601": "2023-03-20T14:27:48.704699Z",
"url": "https://files.pythonhosted.org/packages/bc/3b/c4e4092e694f9d370ea0babf5b90b2733c77c2e9760fd8e8672a7669b3b9/textual_filedrop-0.3.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-03-20 14:27:48",
"github": false,
"gitlab": false,
"bitbucket": false,
"lcname": "textual-filedrop"
}