Name | weatherhat JSON |
Version |
1.0.0
JSON |
| download |
home_page | None |
Summary | Library for the Pimoroni Weather HAT |
upload_time | 2024-06-21 10:50:59 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.7 |
license | MIT License Copyright (c) 2018 Pimoroni Ltd. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
pi
raspberry
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# Weather HAT Python Library & Examples
[](https://github.com/pimoroni/weatherhat-python/actions/workflows/test.yml)
[](https://coveralls.io/github/pimoroni/weatherhat-python?branch=master)
[](https://pypi.python.org/pypi/weatherhat)
[](https://pypi.python.org/pypi/weatherhat)
Weather HAT is a tidy all-in-one solution for hooking up climate and environmental sensors to a Raspberry Pi. It has a bright 1.54" LCD screen and four buttons for inputs. The onboard sensors can measure temperature, humidity, pressure and light. The RJ11 connectors will let you easily attach wind and rain sensors. It will work with any Raspberry Pi with a 40 pin header.
## Where to buy
* [Weather HAT](https://shop.pimoroni.com/products/weather-hat-only)
* [Weather HAT + Weather Sensors Kit](https://shop.pimoroni.com/products/weather-hat)
# Installing
We'd recommend using this library with Raspberry Pi OS Bookworm or later. It requires Python ≥3.7.
## Full install (recommended):
We've created an easy installation script that will install all pre-requisites and get your Weather HAT
up and running with minimal efforts. To run it, fire up Terminal which you'll find in Menu -> Accessories -> Terminal
on your Raspberry Pi desktop, as illustrated below:
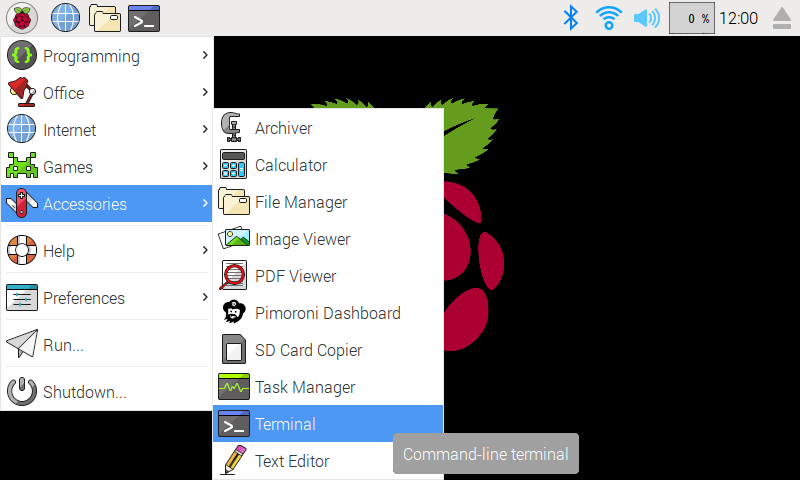
In the new terminal window type the commands exactly as it appears below (check for typos) and follow the on-screen instructions:
```bash
git clone https://github.com/pimoroni/weatherhat-python
cd weatherhat-python
./install.sh
```
**Note** Libraries will be installed in the "pimoroni" virtual environment, you will need to activate it to run examples:
```
source ~/.virtualenvs/pimoroni/bin/activate
```
## Development:
If you want to contribute, or like living on the edge of your seat by having the latest code, you can install the development version like so:
```bash
git clone https://github.com/pimoroni/weatherhat-python
cd weatherhat-python
./install.sh --unstable
```
## Install stable library from PyPi and configure manually
* Set up a virtual environment: `python3 -m venv --system-site-packages $HOME/.virtualenvs/pimoroni`
* Switch to the virtual environment: `source ~/.virtualenvs/pimoroni/bin/activate`
* Install the library: `pip install weatherhat`
In some cases you may need to us `sudo` or install pip with: `sudo apt install python3-pip`.
This will not make any configuration changes, so you may also need to enable:
* i2c: `sudo raspi-config nonint do_i2c 0`
* spi: `sudo raspi-config nonint do_spi 0`
You can optionally run `sudo raspi-config` or the graphical Raspberry Pi Configuration UI to enable interfaces.
Some of the examples have additional dependencies. You can install them with:
```bash
pip install fonts font-manrope pyyaml adafruit-io numpy pillow
```
You may also need to install `libatlas-base-dev`:
```
sudo apt install libatlas-base-dev
```
# Using The Library
Import the `weatherhat` module and create an instance of the `WeatherHAT` class.
```python
import weatherhat
sensor = weatherhat.WeatherHAT()
```
Weather HAT updates the sensors when you call `update(interval=5)`.
Temperature, pressure, humidity, light and wind direction are updated continuously.
Rain and Wind measurements are measured over an `interval` period. Weather HAT will count ticks of the rain gauge and (half) rotations of the anemometer, calculate rain/wind every `interval` seconds and reset the counts for the next pass.
For example the following code will update rain/wind speed every 5 seconds, and all other readings will be updated on demand:
```python
import time
import weatherhat
sensor = weatherhat.WeatherHAT()
while True:
sensor.update(interval=5.0)
time.sleep(1.0)
```
# Averaging Readings
The Weather HAT library supplies set of "history" classes intended to save readings over a period of time and provide access to things like minimum, maximum and average values with unit conversions.
For example `WindSpeedHistory` allows you to store wind readings and retrieve them in mp/h or km/h, in addition to determining the "gust" (maximum wind speed) in a given period of time:
```python
import time
import weatherhat
from weatherhat.history import WindSpeedHistory
sensor = weatherhat.WeatherHAT()
wind_speed_history = WindSpeedHistory()
while True:
sensor.update(interval=5.0)
if sensor.updated_wind_rain:
wind_speed_history.append(sensor.wind_speed)
print(f"Average wind speed: {wind_speed_history.average_mph()}mph")
print(f"Wind gust: {wind_speed_history.gust_mph()}mph")
time.sleep(1.0)
```
# Quick Reference
## Temperature
Temperature readings are given as degrees celsius and are measured from the Weather HAT's onboard BME280.
### Device Temperature
```python
sensor.device_temperature
```
Device temperature in degrees celsius.
This is the temperature read directly from the BME280 onboard Weather HAT. It's not compensated and tends to read slightly higher than ambient due to heat from the Pi.
### Compensated (Air) Temperature
```python
sensor.temperature
```
Temperature in degrees celsius.
This is the temperature once an offset has been applied. This offset is fixed, and taken from `sensor.temperature_offset`.
## Pressure
```python
sensor.pressure
```
Pressure in hectopascals.
## Humidity
```python
sensor.humidity
```
Humidity in %.
### Relative Humidity
```python
sensor.relative_humidity
```
Relative humidity in %.
Relative humidity is the water content of the air compensated for temperature, since warmer air can hold more water.
It's expressed as a percentage from 0 (no moisture) to 100 (fully saturated).
### Dew Point
```python
sensor.dewpoint
```
Dew point in degrees celsius.
Dew point is the temperature at which water - at the current humidity - will condense out of the air.
## Light / Lux
```python
sensor.lux
```
Light is given in lux.
Lux ranges from 0 (complete darkness) to 64,000 (full brightness).
## Wind
Both wind and rain are updated on an interval, rather than on-demand.
To see if an `update()` call has resulted in new wind/rain measurements, check:
```python
sensor.updated_wind_rain
```
### Wind Direction
```python
sensor.wind_direction
```
Wind direction in degrees.
Wind direction is measured using a potentiometer and uses an analog reading internally. This is converted to degrees for convenience, and will snap to the nearest 45-degree increment with 0 degrees indicating North.
### Wind Speed
```python
sensor.wind_speed
```
Wind speed in meters per second.
Weather HAT counts every half rotation and converts this to cm/s using the anemometer circumference and factor.
It's updated depending on the update interval requested.
## Rain
```python
sensor.rain
```
Rain amount in millimeters per second.
Weather HAT counts every "tick" of the rain gauge (roughly .28mm) over the given update internal and converts this into mm/sec.
### Total Rain
```python
sensor.rain_total
```
Total rain amount in millimeters for the current update period.
1.0.0
-----
* Repackage to hatch/pyproject
* Port to gpiod (Pi 5 support)
0.0.2
-----
* Values will now always be float
* Fixed backlight pin
* Fixed latest/average mph
0.0.1
-----
* Initial Release
Raw data
{
"_id": null,
"home_page": null,
"name": "weatherhat",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "Philip Howard <phil@pimoroni.com>",
"keywords": "Pi, Raspberry",
"author": null,
"author_email": "Philip Howard <phil@pimoroni.com>",
"download_url": "https://files.pythonhosted.org/packages/d0/ff/1a826cb0168495448412da603a7d2a88e94a2f938910befbed38e2ebd4bb/weatherhat-1.0.0.tar.gz",
"platform": null,
"description": "# Weather HAT Python Library & Examples\n\n[](https://github.com/pimoroni/weatherhat-python/actions/workflows/test.yml)\n[](https://coveralls.io/github/pimoroni/weatherhat-python?branch=master)\n[](https://pypi.python.org/pypi/weatherhat)\n[](https://pypi.python.org/pypi/weatherhat)\n\nWeather HAT is a tidy all-in-one solution for hooking up climate and environmental sensors to a Raspberry Pi. It has a bright 1.54\" LCD screen and four buttons for inputs. The onboard sensors can measure temperature, humidity, pressure and light. The RJ11 connectors will let you easily attach wind and rain sensors. It will work with any Raspberry Pi with a 40 pin header.\n\n## Where to buy\n\n* [Weather HAT](https://shop.pimoroni.com/products/weather-hat-only)\n* [Weather HAT + Weather Sensors Kit](https://shop.pimoroni.com/products/weather-hat)\n\n# Installing\n\nWe'd recommend using this library with Raspberry Pi OS Bookworm or later. It requires Python \u22653.7.\n\n## Full install (recommended):\n\nWe've created an easy installation script that will install all pre-requisites and get your Weather HAT\nup and running with minimal efforts. To run it, fire up Terminal which you'll find in Menu -> Accessories -> Terminal\non your Raspberry Pi desktop, as illustrated below:\n\n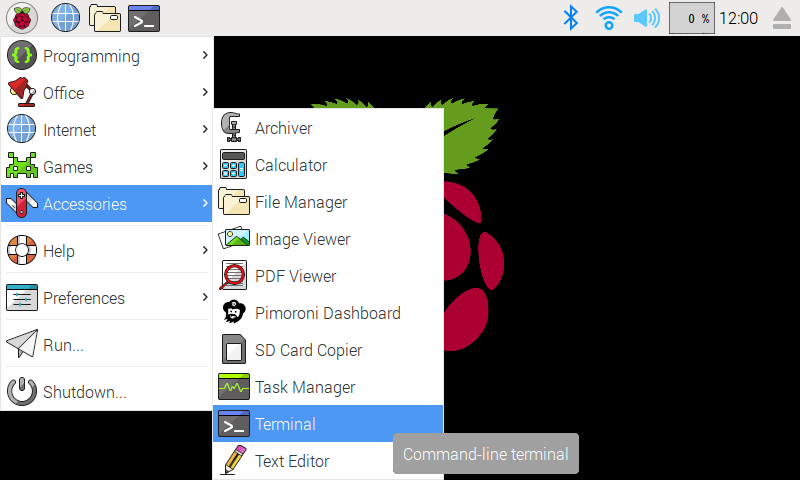\n\nIn the new terminal window type the commands exactly as it appears below (check for typos) and follow the on-screen instructions:\n\n```bash\ngit clone https://github.com/pimoroni/weatherhat-python\ncd weatherhat-python\n./install.sh\n```\n\n**Note** Libraries will be installed in the \"pimoroni\" virtual environment, you will need to activate it to run examples:\n\n```\nsource ~/.virtualenvs/pimoroni/bin/activate\n```\n\n## Development:\n\nIf you want to contribute, or like living on the edge of your seat by having the latest code, you can install the development version like so:\n\n```bash\ngit clone https://github.com/pimoroni/weatherhat-python\ncd weatherhat-python\n./install.sh --unstable\n```\n\n## Install stable library from PyPi and configure manually\n\n* Set up a virtual environment: `python3 -m venv --system-site-packages $HOME/.virtualenvs/pimoroni`\n* Switch to the virtual environment: `source ~/.virtualenvs/pimoroni/bin/activate`\n* Install the library: `pip install weatherhat`\n\nIn some cases you may need to us `sudo` or install pip with: `sudo apt install python3-pip`.\n\nThis will not make any configuration changes, so you may also need to enable:\n\n* i2c: `sudo raspi-config nonint do_i2c 0`\n* spi: `sudo raspi-config nonint do_spi 0`\n\nYou can optionally run `sudo raspi-config` or the graphical Raspberry Pi Configuration UI to enable interfaces.\n\nSome of the examples have additional dependencies. You can install them with:\n\n```bash\npip install fonts font-manrope pyyaml adafruit-io numpy pillow\n```\n\nYou may also need to install `libatlas-base-dev`:\n\n```\nsudo apt install libatlas-base-dev\n```\n\n# Using The Library\n\nImport the `weatherhat` module and create an instance of the `WeatherHAT` class.\n\n```python\nimport weatherhat\n\nsensor = weatherhat.WeatherHAT()\n```\n\nWeather HAT updates the sensors when you call `update(interval=5)`.\n\nTemperature, pressure, humidity, light and wind direction are updated continuously.\n\nRain and Wind measurements are measured over an `interval` period. Weather HAT will count ticks of the rain gauge and (half) rotations of the anemometer, calculate rain/wind every `interval` seconds and reset the counts for the next pass.\n\nFor example the following code will update rain/wind speed every 5 seconds, and all other readings will be updated on demand:\n\n```python\nimport time\nimport weatherhat\n\nsensor = weatherhat.WeatherHAT()\n\nwhile True:\n sensor.update(interval=5.0)\n time.sleep(1.0)\n```\n\n# Averaging Readings\n\nThe Weather HAT library supplies set of \"history\" classes intended to save readings over a period of time and provide access to things like minimum, maximum and average values with unit conversions.\n\nFor example `WindSpeedHistory` allows you to store wind readings and retrieve them in mp/h or km/h, in addition to determining the \"gust\" (maximum wind speed) in a given period of time:\n\n```python\nimport time\nimport weatherhat\nfrom weatherhat.history import WindSpeedHistory\n\nsensor = weatherhat.WeatherHAT()\nwind_speed_history = WindSpeedHistory()\n\nwhile True:\n sensor.update(interval=5.0)\n if sensor.updated_wind_rain:\n wind_speed_history.append(sensor.wind_speed)\n print(f\"Average wind speed: {wind_speed_history.average_mph()}mph\")\n print(f\"Wind gust: {wind_speed_history.gust_mph()}mph\")\n time.sleep(1.0)\n```\n\n# Quick Reference\n\n## Temperature\n\nTemperature readings are given as degrees celsius and are measured from the Weather HAT's onboard BME280.\n\n### Device Temperature\n\n```python\nsensor.device_temperature\n```\n\nDevice temperature in degrees celsius.\n\nThis is the temperature read directly from the BME280 onboard Weather HAT. It's not compensated and tends to read slightly higher than ambient due to heat from the Pi.\n\n### Compensated (Air) Temperature\n\n```python\nsensor.temperature\n```\n\nTemperature in degrees celsius.\n\nThis is the temperature once an offset has been applied. This offset is fixed, and taken from `sensor.temperature_offset`.\n\n## Pressure\n\n```python\nsensor.pressure\n```\n\nPressure in hectopascals.\n\n## Humidity\n\n```python\nsensor.humidity\n```\n\nHumidity in %.\n\n### Relative Humidity\n\n```python\nsensor.relative_humidity\n```\n\nRelative humidity in %.\n\nRelative humidity is the water content of the air compensated for temperature, since warmer air can hold more water.\n\nIt's expressed as a percentage from 0 (no moisture) to 100 (fully saturated).\n\n### Dew Point\n\n```python\nsensor.dewpoint\n```\n\nDew point in degrees celsius.\n\nDew point is the temperature at which water - at the current humidity - will condense out of the air.\n\n## Light / Lux\n\n```python\nsensor.lux\n```\n\nLight is given in lux.\n\nLux ranges from 0 (complete darkness) to 64,000 (full brightness).\n\n## Wind\n\nBoth wind and rain are updated on an interval, rather than on-demand.\n\nTo see if an `update()` call has resulted in new wind/rain measurements, check:\n\n```python\nsensor.updated_wind_rain\n```\n\n### Wind Direction\n\n```python\nsensor.wind_direction\n```\n\nWind direction in degrees.\n\nWind direction is measured using a potentiometer and uses an analog reading internally. This is converted to degrees for convenience, and will snap to the nearest 45-degree increment with 0 degrees indicating North.\n\n### Wind Speed\n\n```python\nsensor.wind_speed\n```\n\nWind speed in meters per second.\n\nWeather HAT counts every half rotation and converts this to cm/s using the anemometer circumference and factor.\n\nIt's updated depending on the update interval requested.\n\n## Rain\n\n```python\nsensor.rain\n```\n\nRain amount in millimeters per second.\n\nWeather HAT counts every \"tick\" of the rain gauge (roughly .28mm) over the given update internal and converts this into mm/sec.\n\n### Total Rain\n\n```python\nsensor.rain_total\n```\n\nTotal rain amount in millimeters for the current update period.\n\n1.0.0\n-----\n\n* Repackage to hatch/pyproject\n* Port to gpiod (Pi 5 support)\n\n0.0.2\n-----\n\n* Values will now always be float\n* Fixed backlight pin\n* Fixed latest/average mph\n\n0.0.1\n-----\n\n* Initial Release\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2018 Pimoroni Ltd. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.",
"summary": "Library for the Pimoroni Weather HAT",
"version": "1.0.0",
"project_urls": {
"GitHub": "https://www.github.com/pimoroni/weatherhat-python",
"Homepage": "https://www.pimoroni.com"
},
"split_keywords": [
"pi",
" raspberry"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "553ac620bbeae58448c39f5db176215b57bcb9933f696f83229c9f9d6de1a7e3",
"md5": "2baf57ae13de06e3127ed08f986f64fa",
"sha256": "afb099b1a0ccb54005f9e14d41be31cf18e17cf1f67c04e72e27a4a00b615a91"
},
"downloads": -1,
"filename": "weatherhat-1.0.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "2baf57ae13de06e3127ed08f986f64fa",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 13117,
"upload_time": "2024-06-21T10:50:58",
"upload_time_iso_8601": "2024-06-21T10:50:58.058531Z",
"url": "https://files.pythonhosted.org/packages/55/3a/c620bbeae58448c39f5db176215b57bcb9933f696f83229c9f9d6de1a7e3/weatherhat-1.0.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d0ff1a826cb0168495448412da603a7d2a88e94a2f938910befbed38e2ebd4bb",
"md5": "cbdb9c8566a107eaf19534c98a826a8a",
"sha256": "e1bd27ff28a5e0c2da1bee9b16abb70073552f46cce1ba07d8637f3641c9de5a"
},
"downloads": -1,
"filename": "weatherhat-1.0.0.tar.gz",
"has_sig": false,
"md5_digest": "cbdb9c8566a107eaf19534c98a826a8a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 30868,
"upload_time": "2024-06-21T10:50:59",
"upload_time_iso_8601": "2024-06-21T10:50:59.420879Z",
"url": "https://files.pythonhosted.org/packages/d0/ff/1a826cb0168495448412da603a7d2a88e94a2f938910befbed38e2ebd4bb/weatherhat-1.0.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-21 10:50:59",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "pimoroni",
"github_project": "weatherhat-python",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"tox": true,
"lcname": "weatherhat"
}