# 1inch.py
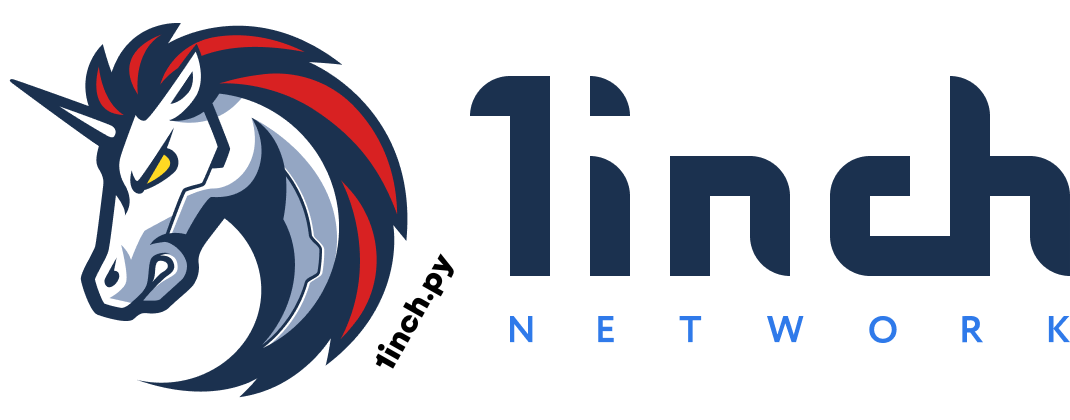
1inch.py is a wrapper around the 1inch API and price Oracle. It has full coverage of the swap API endpoint and All chains support by 1inch are included in the OneInchSwap and OneInchOracle methods.
Package also includes a helper method to ease the submission of transactions to the network. Limited chains currently supported.
## API Documentation
The full 1inch swap API docs can be found at https://docs.1inch.io/
## Installation
Use the package manager [pip](https://pip.pypa.io/en/stable/) to install 1inch.py.
```bash
pip install 1inch.py
```
## Usage
A quick note on decimals. The wrapper is designed for ease of use, and as such accepts amounts in "Ether" or whole units.
If you prefer, you can use decimal=0 and specify amounts in wei. This will also help with any potential floating point errors.
```python
from oneinch_py import OneInchSwap, TransactionHelper, OneInchOracle
rpc_url = "yourRPCURL.com"
binance_rpc = "adifferentRPCurl.com"
public_key = "yourWalletAddress"
private_key = "yourPrivateKey" #remember to protect your private key. Using environmental variables is recommended.
api_key = "" # 1 Inch API key
exchange = OneInchSwap(api_key, public_key)
bsc_exchange = OneInchSwap(api_key, public_key, chain='binance')
helper = TransactionHelper(api_key, rpc_url, public_key, private_key)
bsc_helper = TransactionHelper(api_key, binance_rpc, public_key, private_key, chain='binance')
oracle = OneInchOracle(rpc_url, chain='ethereum')
# See chains currently supported by the helper method:
helper.chains
# {"ethereum": "1", "binance": "56", "polygon": "137", "avalanche": "43114"}
# Straight to business:
# Get a swap and do the swap
result = exchange.get_swap("USDT", "ETH", 10, 0.5) # get the swap transaction
result = helper.build_tx(result) # prepare the transaction for signing, gas price defaults to fast.
result = helper.sign_tx(result) # sign the transaction using your private key
result = helper.broadcast_tx(result) #broadcast the transaction to the network and wait for the receipt.
## If you already have token addresses you can pass those in instead of token names to all OneInchSwap functions that require a token argument
result = exchange.get_swap("0x7fc66500c84a76ad7e9c93437bfc5ac33e2ddae9", "0x43dfc4159d86f3a37a5a4b3d4580b888ad7d4ddd", 10, 0.5)
#USDT to ETH price on the Oracle. Note that you need to indicate the token decimal if it is anything other than 18.
oracle.get_rate_to_ETH("0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48", src_token_decimal=6)
# Get the rate between any two tokens.
oracle.get_rate(src_token="0x6B175474E89094C44Da98b954EedeAC495271d0F", dst_token="0x111111111117dC0aa78b770fA6A738034120C302")
exchange.health_check()
# 'OK'
# Address of the 1inch router that must be trusted to spend funds for the swap
exchange.get_spender()
# Generate data for calling the contract in order to allow the 1inch router to spend funds. Token symbol or address is required. If optional "amount" variable is not supplied (in ether), unlimited allowance is granted.
exchange.get_approve("USDT")
exchange.get_approve("0xdAC17F958D2ee523a2206206994597C13D831ec7", amount=100)
# Get the number of tokens (in Wei) that the router is allowed to spend. Option "send address" variable. If not supplied uses address supplied when Initialization the exchange object.
exchange.get_allowance("USDT")
exchange.get_allowance("0xdAC17F958D2ee523a2206206994597C13D831ec7", send_address="0x12345")
# Token List is stored in memory
exchange.tokens
# {
# '1INCH': {'address': '0x111111111117dc0aa78b770fa6a738034120c302',
# 'decimals': 18,
# 'logoURI': 'https://tokens.1inch.exchange/0x111111111117dc0aa78b770fa6a738034120c302.png',
# 'name': '1INCH Token',
# 'symbol': '1INCH'},
# 'ETH': {'address': '0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee',
# 'decimals': 18,
# 'logoURI': 'https://tokens.1inch.exchange/0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee.png',
# 'name': 'Ethereum',
# 'symbol': 'ETH'},
# ......
# }
# Returns the exchange rate of two tokens.
# Tokens can be provided as symbols or addresses
# "amount" is supplied in ether
# NOTE: When using custom tokens, the token decimal is assumed to be 18. If your custom token has a different decimal - please manually pass it to the function (decimal=x)
# Also returns the "price" of more expensive token in the cheaper tokens. Optional variables can be supplied as **kwargs
exchange.get_quote(from_token_symbol='ETH', to_token_symbol='USDT', amount=1)
# (
# {
# "fromToken": {
# "symbol": "ETH",
# "name": "Ethereum",
# "decimals": 18,
# "address": "0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee",
# "logoURI": "https://tokens.1inch.io/0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee.png",
# "tags": ["native"],
# },
# "toToken": {
# "symbol": "USDT",
# "name": "Tether USD",
# "address": "0xdac17f958d2ee523a2206206994597c13d831ec7",
# "decimals": 6,
# "logoURI": "https://tokens.1inch.io/0xdac17f958d2ee523a2206206994597c13d831ec7.png",
# "tags": ["tokens"],
# ...
# Decimal("1076.503093"),
# )
# Creates the swap data for two tokens.
# Tokens can be provided as symbols or addresses
# Optional variables can be supplied as **kwargs
# NOTE: When using custom tokens, the token decimal is assumed to be 18. If your custom token has a different decimal - please manually pass it to the function (decimal=x)
exchange.get_swap(from_token_symbol='ETH', to_token_symbol='USDT', amount=1, slippage=0.5)
# {
# "fromToken": {
# "symbol": "ETH",
# "name": "Ethereum",
# "decimals": 18,
# "address": "0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee",
# "logoURI": "https://tokens.1inch.io/0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee.png",
# "tags": ["native"],
# },
# "toToken": {
# "symbol": "USDT",
# "name": "Tether USD",
# "address": "0xdac17f958d2ee523a2206206994597c13d831ec7",
# "decimals": 6,
# "logoURI": "https://tokens.1inch.io/0xdac17f958d2ee523a2206206994597c13d831ec7.png",
# "tags": ["tokens"],
#
# ...
#
# ],
# "tx": {
# "from": "0x1d05aD0366ad6dc0a284C5fbda46cd555Fb4da27",
# "to": "0x1111111254fb6c44bac0bed2854e76f90643097d",
# "data": "0xe449022e00000000000000000000000000000000000000000000000006f05b59d3b20000000000000000000000000000000000000000000000000000000000001fed825a0000000000000000000000000000000000000000000000000000000000000060000000000000000000000000000000000000000000000000000000000000000140000000000000000000000011b815efb8f581194ae79006d24e0d814b7697f6cfee7c08",
# "value": "500000000000000000",
# "gas": 178993,
# "gasPrice": "14183370651",
# },
# }
```
## Contributing
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
Thanks to @Makbeta for all of their work in migrating the wrapper to the new 1inch api system.
## License
[MIT](https://choosealicense.com/licenses/mit/)
Raw data
{
"_id": null,
"home_page": "https://github.com/RichardAtCT/1inch_wrapper",
"name": "1inch.py",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7, <4",
"maintainer_email": "",
"keywords": "1inch,wrapper,aggregator,DEX",
"author": "RichardAt",
"author_email": "richardatk01@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/0f/50/b43aa93d327ab55b5794d37eaddbb6681021284c94a222533f46b8948149/1inch.py-2.0.3.tar.gz",
"platform": null,
"description": "\n# 1inch.py\n\n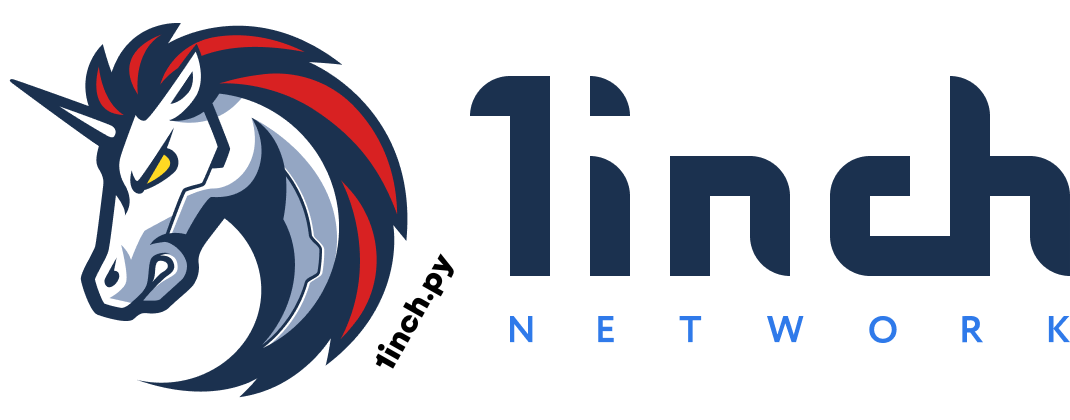\n\n1inch.py is a wrapper around the 1inch API and price Oracle. It has full coverage of the swap API endpoint and All chains support by 1inch are included in the OneInchSwap and OneInchOracle methods. \nPackage also includes a helper method to ease the submission of transactions to the network. Limited chains currently supported. \n\n## API Documentation\nThe full 1inch swap API docs can be found at https://docs.1inch.io/\n## Installation\n\nUse the package manager [pip](https://pip.pypa.io/en/stable/) to install 1inch.py.\n\n```bash\npip install 1inch.py\n```\n\n## Usage\n\nA quick note on decimals. The wrapper is designed for ease of use, and as such accepts amounts in \"Ether\" or whole units. \nIf you prefer, you can use decimal=0 and specify amounts in wei. This will also help with any potential floating point errors. \n\n\n```python\nfrom oneinch_py import OneInchSwap, TransactionHelper, OneInchOracle\n\nrpc_url = \"yourRPCURL.com\"\nbinance_rpc = \"adifferentRPCurl.com\"\npublic_key = \"yourWalletAddress\"\nprivate_key = \"yourPrivateKey\" #remember to protect your private key. Using environmental variables is recommended. \napi_key = \"\" # 1 Inch API key\n\nexchange = OneInchSwap(api_key, public_key)\nbsc_exchange = OneInchSwap(api_key, public_key, chain='binance')\nhelper = TransactionHelper(api_key, rpc_url, public_key, private_key)\nbsc_helper = TransactionHelper(api_key, binance_rpc, public_key, private_key, chain='binance')\noracle = OneInchOracle(rpc_url, chain='ethereum')\n\n\n# See chains currently supported by the helper method:\nhelper.chains\n# {\"ethereum\": \"1\", \"binance\": \"56\", \"polygon\": \"137\", \"avalanche\": \"43114\"}\n\n# Straight to business:\n# Get a swap and do the swap\nresult = exchange.get_swap(\"USDT\", \"ETH\", 10, 0.5) # get the swap transaction\nresult = helper.build_tx(result) # prepare the transaction for signing, gas price defaults to fast.\nresult = helper.sign_tx(result) # sign the transaction using your private key\nresult = helper.broadcast_tx(result) #broadcast the transaction to the network and wait for the receipt. \n\n## If you already have token addresses you can pass those in instead of token names to all OneInchSwap functions that require a token argument\nresult = exchange.get_swap(\"0x7fc66500c84a76ad7e9c93437bfc5ac33e2ddae9\", \"0x43dfc4159d86f3a37a5a4b3d4580b888ad7d4ddd\", 10, 0.5) \n\n\n#USDT to ETH price on the Oracle. Note that you need to indicate the token decimal if it is anything other than 18.\noracle.get_rate_to_ETH(\"0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48\", src_token_decimal=6)\n\n# Get the rate between any two tokens.\noracle.get_rate(src_token=\"0x6B175474E89094C44Da98b954EedeAC495271d0F\", dst_token=\"0x111111111117dC0aa78b770fA6A738034120C302\")\n\nexchange.health_check()\n# 'OK'\n\n# Address of the 1inch router that must be trusted to spend funds for the swap\nexchange.get_spender()\n\n# Generate data for calling the contract in order to allow the 1inch router to spend funds. Token symbol or address is required. If optional \"amount\" variable is not supplied (in ether), unlimited allowance is granted.\nexchange.get_approve(\"USDT\")\nexchange.get_approve(\"0xdAC17F958D2ee523a2206206994597C13D831ec7\", amount=100)\n\n# Get the number of tokens (in Wei) that the router is allowed to spend. Option \"send address\" variable. If not supplied uses address supplied when Initialization the exchange object. \nexchange.get_allowance(\"USDT\")\nexchange.get_allowance(\"0xdAC17F958D2ee523a2206206994597C13D831ec7\", send_address=\"0x12345\")\n\n# Token List is stored in memory\nexchange.tokens\n# {\n# '1INCH': {'address': '0x111111111117dc0aa78b770fa6a738034120c302',\n# 'decimals': 18,\n# 'logoURI': 'https://tokens.1inch.exchange/0x111111111117dc0aa78b770fa6a738034120c302.png',\n# 'name': '1INCH Token',\n# 'symbol': '1INCH'},\n# 'ETH': {'address': '0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee',\n# 'decimals': 18,\n# 'logoURI': 'https://tokens.1inch.exchange/0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee.png',\n# 'name': 'Ethereum',\n# 'symbol': 'ETH'},\n# ......\n# }\n\n# Returns the exchange rate of two tokens. \n# Tokens can be provided as symbols or addresses\n# \"amount\" is supplied in ether\n# NOTE: When using custom tokens, the token decimal is assumed to be 18. If your custom token has a different decimal - please manually pass it to the function (decimal=x)\n# Also returns the \"price\" of more expensive token in the cheaper tokens. Optional variables can be supplied as **kwargs\nexchange.get_quote(from_token_symbol='ETH', to_token_symbol='USDT', amount=1)\n# (\n# {\n# \"fromToken\": {\n# \"symbol\": \"ETH\",\n# \"name\": \"Ethereum\",\n# \"decimals\": 18,\n# \"address\": \"0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee\",\n# \"logoURI\": \"https://tokens.1inch.io/0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee.png\",\n# \"tags\": [\"native\"],\n# },\n# \"toToken\": {\n# \"symbol\": \"USDT\",\n# \"name\": \"Tether USD\",\n# \"address\": \"0xdac17f958d2ee523a2206206994597c13d831ec7\",\n# \"decimals\": 6,\n# \"logoURI\": \"https://tokens.1inch.io/0xdac17f958d2ee523a2206206994597c13d831ec7.png\",\n# \"tags\": [\"tokens\"],\n# ...\n# Decimal(\"1076.503093\"),\n# )\n\n# Creates the swap data for two tokens.\n# Tokens can be provided as symbols or addresses\n# Optional variables can be supplied as **kwargs\n# NOTE: When using custom tokens, the token decimal is assumed to be 18. If your custom token has a different decimal - please manually pass it to the function (decimal=x)\n\nexchange.get_swap(from_token_symbol='ETH', to_token_symbol='USDT', amount=1, slippage=0.5)\n# {\n# \"fromToken\": {\n# \"symbol\": \"ETH\",\n# \"name\": \"Ethereum\",\n# \"decimals\": 18,\n# \"address\": \"0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee\",\n# \"logoURI\": \"https://tokens.1inch.io/0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee.png\",\n# \"tags\": [\"native\"],\n# },\n# \"toToken\": {\n# \"symbol\": \"USDT\",\n# \"name\": \"Tether USD\",\n# \"address\": \"0xdac17f958d2ee523a2206206994597c13d831ec7\",\n# \"decimals\": 6,\n# \"logoURI\": \"https://tokens.1inch.io/0xdac17f958d2ee523a2206206994597c13d831ec7.png\",\n# \"tags\": [\"tokens\"],\n#\n# ...\n#\n# ],\n# \"tx\": {\n# \"from\": \"0x1d05aD0366ad6dc0a284C5fbda46cd555Fb4da27\",\n# \"to\": \"0x1111111254fb6c44bac0bed2854e76f90643097d\",\n# \"data\": \"0xe449022e00000000000000000000000000000000000000000000000006f05b59d3b20000000000000000000000000000000000000000000000000000000000001fed825a0000000000000000000000000000000000000000000000000000000000000060000000000000000000000000000000000000000000000000000000000000000140000000000000000000000011b815efb8f581194ae79006d24e0d814b7697f6cfee7c08\",\n# \"value\": \"500000000000000000\",\n# \"gas\": 178993,\n# \"gasPrice\": \"14183370651\",\n# },\n# }\n\n\n```\n\n## Contributing\nPull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.\n\nThanks to @Makbeta for all of their work in migrating the wrapper to the new 1inch api system.\n\n\n## License\n[MIT](https://choosealicense.com/licenses/mit/)\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "a Python wrapper for the 1inch API",
"version": "2.0.3",
"project_urls": {
"Homepage": "https://github.com/RichardAtCT/1inch_wrapper"
},
"split_keywords": [
"1inch",
"wrapper",
"aggregator",
"dex"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "0f50b43aa93d327ab55b5794d37eaddbb6681021284c94a222533f46b8948149",
"md5": "477b789b8d185f1cc79443bddccd0935",
"sha256": "5e09169f25774b9600d291a7b1d2e9833d80b9fdeda5c7757ae0f6485af0a5bb"
},
"downloads": -1,
"filename": "1inch.py-2.0.3.tar.gz",
"has_sig": false,
"md5_digest": "477b789b8d185f1cc79443bddccd0935",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7, <4",
"size": 12363,
"upload_time": "2023-08-16T07:27:48",
"upload_time_iso_8601": "2023-08-16T07:27:48.968042Z",
"url": "https://files.pythonhosted.org/packages/0f/50/b43aa93d327ab55b5794d37eaddbb6681021284c94a222533f46b8948149/1inch.py-2.0.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-08-16 07:27:48",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "RichardAtCT",
"github_project": "1inch_wrapper",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "1inch.py"
}