# CTkColorPicker
**A modern color picker made for customtkinter!**
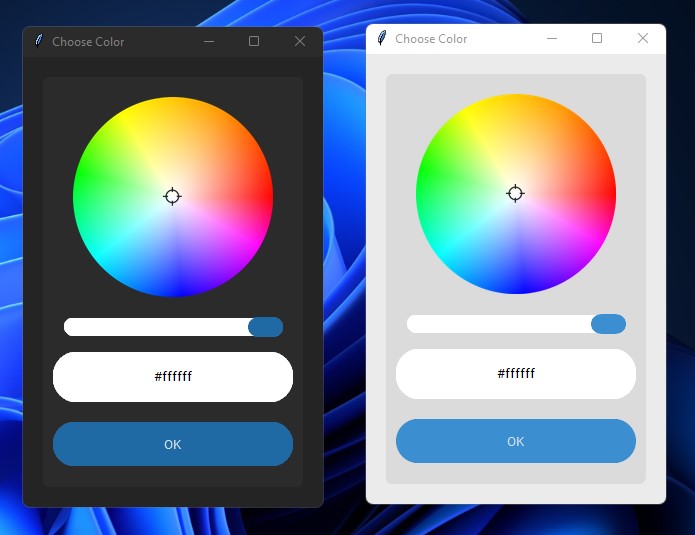
## Download
```
pip install CTkColorPicker
```
### [<img alt="GitHub repo size" src="https://img.shields.io/github/repo-size/Akascape/CTkColorPicker?&color=white&label=Source%20Code&logo=Python&logoColor=yellow&style=for-the-badge" width="300">](https://github.com/Akascape/CTkColorPicker/archive/refs/heads/main.zip)
## Requirements
- [customtkinter](https://github.com/TomSchimansky/CustomTkinter)
- [pillow](https://pypi.org/project/Pillow/)
### How to use?
```python
import customtkinter as ctk
from CTkColorPicker import *
def ask_color():
pick_color = AskColor() # open the color picker
color = pick_color.get() # get the color string
button.configure(fg_color=color)
root = ctk.CTk()
button = ctk.CTkButton(master=root, text="CHOOSE COLOR", text_color="black", command=ask_color)
button.pack(padx=30, pady=20)
root.mainloop()
```
## Options
| Arguments | Description |
|---------|-------------|
| width | set the overall size of the color picker window |
| title | change the title of color picker window |
| fg_color | change forground color of the color picker frame |
| bg_color | change background color of the color picker frame |
| button_color | change the color of the button and slider |
| button_hover_color | change the hover color of the buttons |
| text | change the default text of the 'OK' button |
| initial_color | set the default color of color picker (currently in beta stage) |
| slider_border | change the border width of slider |
| corner_radius | change the corner radius of all the widgets inside color picker |
| _**other button parameters_ | pass other button arguments if required |
# ColorPickerWidget
**This is a new color picker widget that can be placed inside a customtkinter frame.**

### Usage
```python
from CTkColorPicker import *
import customtkinter
root = customtkinter.CTk()
colorpicker = CTkColorPicker(root, width=500, command=lambda e: print(e))
colorpicker.pack(padx=10, pady=10)
root.mainloop()
```
## Options
| Arguments | Description |
|---------|-------------|
| master | parent widget |
| width | set the overall size of the color picker frame |
| fg_color | change forground color of the color picker frame |
| initial_color | set the default color of color picker (currently in beta stage) |
| slider_border | change the border width of slider |
| corner_radius | change the corner radius of all the widgets inside color picker |
| command | add a command when the color is changed |
| orientation | change orientation of slider and label |
| _**other slider parameters_ | pass other slider arguments if required |
**That's all, hope it will help!**
Raw data
{
"_id": null,
"home_page": "https://github.com/Akascape/CTkColorPicker",
"name": "CTkColorPicker",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "customtkinter,customtkinter-dialog,customtkinter-color-picker,customtkinter-colour-picker,customtkinter-color,customtkinter-color-chooser,color-picker,python-color-picker",
"author": "Akash Bora",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/08/d4/e10f2f5e827325eb51983e4b19793c7de8e1865d5db5c1da4857b6690732/CTkColorPicker-0.9.0.tar.gz",
"platform": null,
"description": "# CTkColorPicker\r\n**A modern color picker made for customtkinter!**\r\n\r\n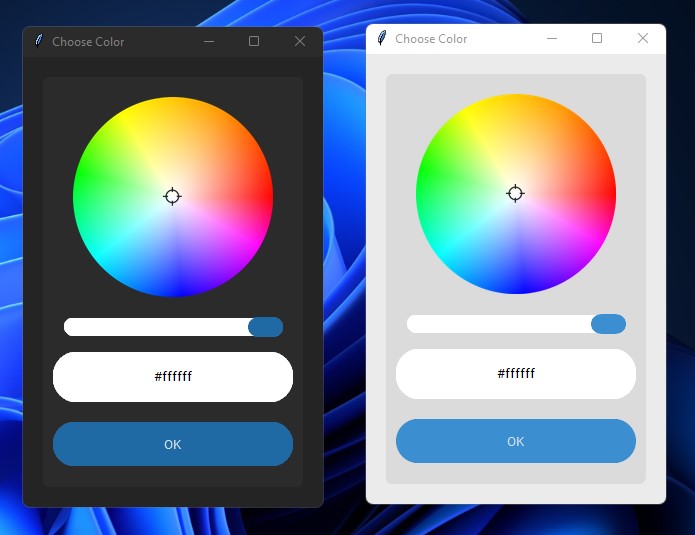\r\n\r\n## Download\r\n\r\n```\r\npip install CTkColorPicker\r\n```\r\n\r\n### [<img alt=\"GitHub repo size\" src=\"https://img.shields.io/github/repo-size/Akascape/CTkColorPicker?&color=white&label=Source%20Code&logo=Python&logoColor=yellow&style=for-the-badge\" width=\"300\">](https://github.com/Akascape/CTkColorPicker/archive/refs/heads/main.zip)\r\n\r\n## Requirements\r\n- [customtkinter](https://github.com/TomSchimansky/CustomTkinter)\r\n- [pillow](https://pypi.org/project/Pillow/)\r\n\r\n### How to use?\r\n```python\r\nimport customtkinter as ctk\r\nfrom CTkColorPicker import *\r\n\r\ndef ask_color():\r\n pick_color = AskColor() # open the color picker\r\n color = pick_color.get() # get the color string\r\n button.configure(fg_color=color)\r\n \r\nroot = ctk.CTk()\r\n\r\nbutton = ctk.CTkButton(master=root, text=\"CHOOSE COLOR\", text_color=\"black\", command=ask_color)\r\nbutton.pack(padx=30, pady=20)\r\nroot.mainloop()\r\n```\r\n\r\n## Options\r\n| Arguments | Description |\r\n|---------|-------------|\r\n| width | set the overall size of the color picker window |\r\n| title | change the title of color picker window |\r\n| fg_color | change forground color of the color picker frame |\r\n| bg_color | change background color of the color picker frame |\r\n| button_color | change the color of the button and slider |\r\n| button_hover_color | change the hover color of the buttons |\r\n| text | change the default text of the 'OK' button |\r\n| initial_color | set the default color of color picker (currently in beta stage) |\r\n| slider_border | change the border width of slider |\r\n| corner_radius | change the corner radius of all the widgets inside color picker |\r\n| _**other button parameters_ | pass other button arguments if required |\r\n\r\n# ColorPickerWidget\r\n**This is a new color picker widget that can be placed inside a customtkinter frame.**\r\n\r\n\r\n\r\n### Usage\r\n```python\r\nfrom CTkColorPicker import *\r\nimport customtkinter\r\n\r\nroot = customtkinter.CTk()\r\ncolorpicker = CTkColorPicker(root, width=500, command=lambda e: print(e))\r\ncolorpicker.pack(padx=10, pady=10)\r\nroot.mainloop()\r\n```\r\n\r\n## Options\r\n| Arguments | Description |\r\n|---------|-------------|\r\n| master | parent widget |\r\n| width | set the overall size of the color picker frame |\r\n| fg_color | change forground color of the color picker frame |\r\n| initial_color | set the default color of color picker (currently in beta stage) |\r\n| slider_border | change the border width of slider |\r\n| corner_radius | change the corner radius of all the widgets inside color picker |\r\n| command | add a command when the color is changed |\r\n| orientation | change orientation of slider and label |\r\n| _**other slider parameters_ | pass other slider arguments if required |\r\n\r\n**That's all, hope it will help!**\r\n",
"bugtrack_url": null,
"license": "Creative Commons Zero v1.0 Universal",
"summary": "A modern color picker for customtkinter",
"version": "0.9.0",
"project_urls": {
"Homepage": "https://github.com/Akascape/CTkColorPicker"
},
"split_keywords": [
"customtkinter",
"customtkinter-dialog",
"customtkinter-color-picker",
"customtkinter-colour-picker",
"customtkinter-color",
"customtkinter-color-chooser",
"color-picker",
"python-color-picker"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "5b8dc3f374fd7627deb35c37795e8799fc40f72a510fde0f465e6c792c58624e",
"md5": "41ad981f37a25ac653cd42374d3568b5",
"sha256": "8406f0c321c1fc3b513e1aa74200bc4a30bc03e2448247519907689031af190b"
},
"downloads": -1,
"filename": "CTkColorPicker-0.9.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "41ad981f37a25ac653cd42374d3568b5",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 123021,
"upload_time": "2023-12-11T10:29:19",
"upload_time_iso_8601": "2023-12-11T10:29:19.861831Z",
"url": "https://files.pythonhosted.org/packages/5b/8d/c3f374fd7627deb35c37795e8799fc40f72a510fde0f465e6c792c58624e/CTkColorPicker-0.9.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "08d4e10f2f5e827325eb51983e4b19793c7de8e1865d5db5c1da4857b6690732",
"md5": "b5d25c03c209aac85f08c4893068b4aa",
"sha256": "5f65e35d0cf9d0c9fee7e24f3f460e256641f0e335ab00c17425bb0d16060b0c"
},
"downloads": -1,
"filename": "CTkColorPicker-0.9.0.tar.gz",
"has_sig": false,
"md5_digest": "b5d25c03c209aac85f08c4893068b4aa",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 121563,
"upload_time": "2023-12-11T10:29:24",
"upload_time_iso_8601": "2023-12-11T10:29:24.356225Z",
"url": "https://files.pythonhosted.org/packages/08/d4/e10f2f5e827325eb51983e4b19793c7de8e1865d5db5c1da4857b6690732/CTkColorPicker-0.9.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-12-11 10:29:24",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Akascape",
"github_project": "CTkColorPicker",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "ctkcolorpicker"
}