# CTkToolTip
**Small tooltip pop-up label for displaying details on customtkinter widgets.**
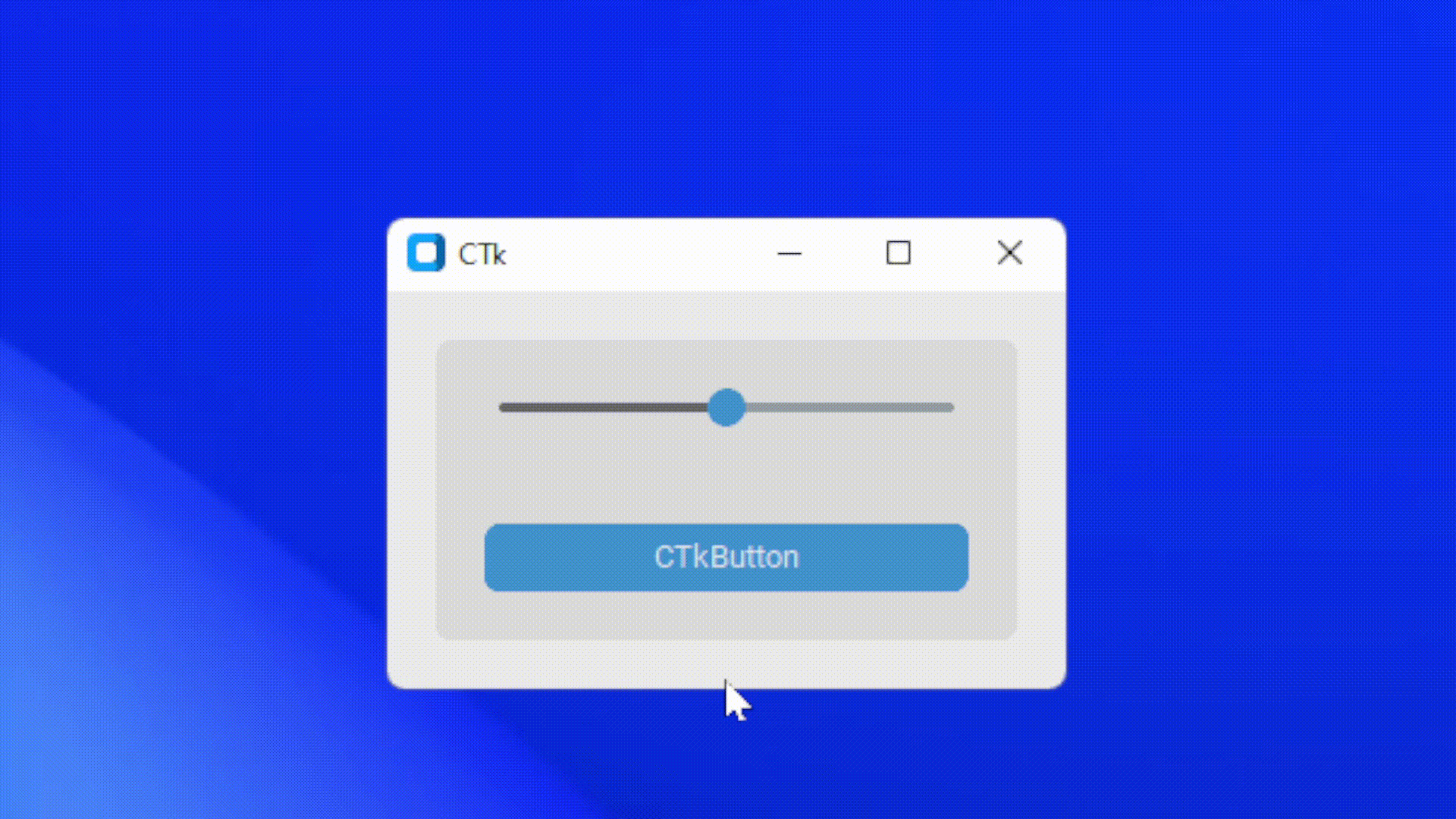
## Features
- Display over any CTk widget
- Configurable options
- Transparency effect
- Round corners
- Can be used with CTkSlider to show the value
- Dynamic offset
- Add delays
## Installation
```
pip install CTkToolTip
```
### [<img alt="GitHub repo size" src="https://img.shields.io/github/repo-size/Akascape/CTkToolTip?&color=white&label=Download%20Source%20Code&logo=Python&logoColor=yellow&style=for-the-badge" width="400">](https://github.com/Akascape/CTkToolTip/archive/refs/heads/main.zip)
## Example
**Simple Usage:**
```python
CTkToolTip(widget, message="Your Message")
```
**App Example:**
```python
import customtkinter
from CTkToolTip import *
def show_value(value):
tooltip_1.configure(message=int(value))
def show_text():
print(tooltip_2.get())
root = customtkinter.CTk()
slider = customtkinter.CTkSlider(root, from_=0, to=100, command=show_value)
slider.pack(fill="both", padx=20, pady=20)
tooltip_1 = CTkToolTip(slider, message="50")
button = customtkinter.CTkButton(root, command=show_text)
button.pack(fill="both", padx=20, pady=20)
tooltip_2 = CTkToolTip(button, delay=0.5, message="This is a CTkButton!")
root.mainloop()
```
## Arguments
| Parameter | Description |
|-----------| ------------|
| **widget** | bind the tooltip to the ctk widget |
| **message** | show the message over the toolip |
| **delay** | add a small delay before showing the tooltip (default is 0.2) |
| follow | follow the mouse cursor while hovering (default is True) |
| x_offset | change the horizontal offset of the tooltip widget from mouse cursor |
| y_offset | change the vertical offset of the tooltip widget from mouse cursor |
| **alpha** | change the transparency effect of the tooltip (range: 0-1) |
| **bg_color** | change the background color of the tooltip |
| corner_radius | roundness of the corners |
| border_width | add a border around the tooltips (default is 0) |
| border_color | change the color of the border width |
| padding | add padx and pady inside the tooltip frame, tuple: (padx, pady) |
| **text_color** | change the text color of tooltip |
| wraplength | constrains the width of the tooltip, causing CTkToolTip, where required, to wrap the message at word boundaries. |
| font | label text font, tuple: (font_name, size) |
| justify | change the text display structure (left, right or center) |
| _*Other Label Parameters_ | _All other parameters for ctk label can be passed in ctktooltip_ |
## Methods
- **.configure(message, arguments...)**
configure the text and other parameters for the tooltip
- **.get()**
get the current text of tooltip
- **.hide()**
disables the tooltip from appearing
- **.show()**
enable the tooltip again
- **.is_disabled()**
check the tooltip state
### Thats all about CTkToolTips, hope it will help in your project!
Raw data
{
"_id": null,
"home_page": "https://github.com/Akascape/CTkToolTip",
"name": "CTkToolTip",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "customtkinter,tooltips,popup,widgets,tkinter-widgets,tooltip popup,floating window",
"author": "Akash Bora",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/a7/4d/0a9e703b0be6b36a12920c2f993c704a36a58df62552686d76a8c9e3886a/CTkToolTip-0.8.tar.gz",
"platform": null,
"description": "# CTkToolTip\r\n**Small tooltip pop-up label for displaying details on customtkinter widgets.**\r\n\r\n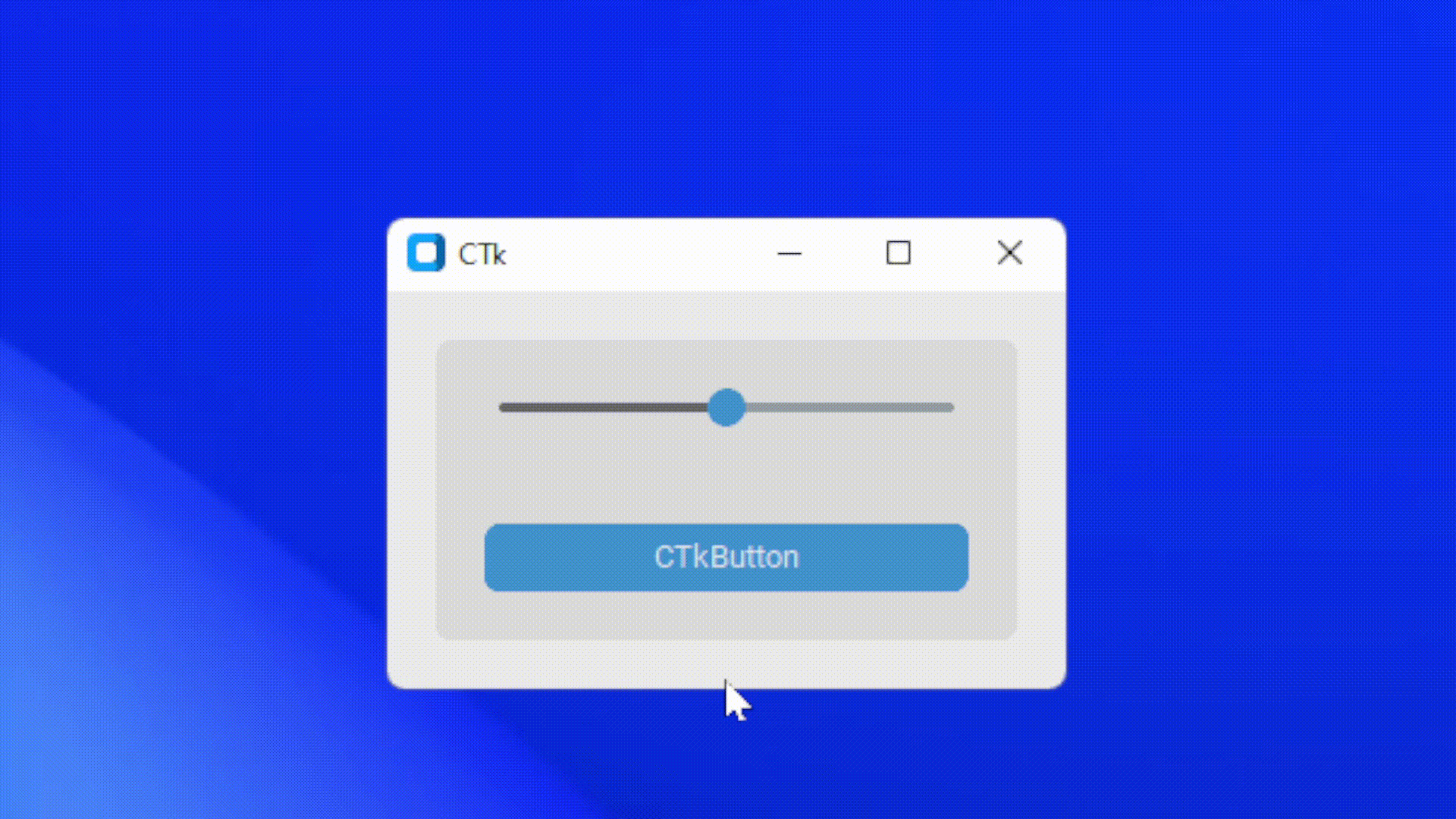\r\n\r\n## Features\r\n- Display over any CTk widget\r\n- Configurable options\r\n- Transparency effect\r\n- Round corners\r\n- Can be used with CTkSlider to show the value\r\n- Dynamic offset\r\n- Add delays\r\n\r\n## Installation\r\n\r\n```\r\npip install CTkToolTip\r\n```\r\n\r\n### [<img alt=\"GitHub repo size\" src=\"https://img.shields.io/github/repo-size/Akascape/CTkToolTip?&color=white&label=Download%20Source%20Code&logo=Python&logoColor=yellow&style=for-the-badge\" width=\"400\">](https://github.com/Akascape/CTkToolTip/archive/refs/heads/main.zip)\r\n\r\n## Example\r\n**Simple Usage:**\r\n```python\r\nCTkToolTip(widget, message=\"Your Message\")\r\n```\r\n**App Example:**\r\n```python\r\nimport customtkinter\r\nfrom CTkToolTip import *\r\n\r\ndef show_value(value):\r\n tooltip_1.configure(message=int(value))\r\n \r\ndef show_text():\r\n print(tooltip_2.get())\r\n\r\nroot = customtkinter.CTk()\r\n\r\nslider = customtkinter.CTkSlider(root, from_=0, to=100, command=show_value)\r\nslider.pack(fill=\"both\", padx=20, pady=20)\r\n\r\ntooltip_1 = CTkToolTip(slider, message=\"50\")\r\n\r\nbutton = customtkinter.CTkButton(root, command=show_text)\r\nbutton.pack(fill=\"both\", padx=20, pady=20)\r\n\r\ntooltip_2 = CTkToolTip(button, delay=0.5, message=\"This is a CTkButton!\")\r\n\r\nroot.mainloop()\r\n```\r\n\r\n## Arguments\r\n| Parameter | Description |\r\n|-----------| ------------|\r\n| **widget** | bind the tooltip to the ctk widget |\r\n| **message** | show the message over the toolip |\r\n| **delay** | add a small delay before showing the tooltip (default is 0.2) |\r\n| follow | follow the mouse cursor while hovering (default is True) |\r\n| x_offset | change the horizontal offset of the tooltip widget from mouse cursor |\r\n| y_offset | change the vertical offset of the tooltip widget from mouse cursor |\r\n| **alpha** | change the transparency effect of the tooltip (range: 0-1) |\r\n| **bg_color** | change the background color of the tooltip |\r\n| corner_radius | roundness of the corners |\r\n| border_width | add a border around the tooltips (default is 0) |\r\n| border_color | change the color of the border width |\r\n| padding | add padx and pady inside the tooltip frame, tuple: (padx, pady) |\r\n| **text_color** | change the text color of tooltip |\r\n| wraplength | constrains the width of the tooltip, causing CTkToolTip, where required, to wrap the message at word boundaries. |\r\n| font | label text font, tuple: (font_name, size) |\r\n| justify | change the text display structure (left, right or center) |\r\n| _*Other Label Parameters_ | _All other parameters for ctk label can be passed in ctktooltip_ |\r\n\r\n## Methods\r\n\r\n- **.configure(message, arguments...)**\r\n\r\n configure the text and other parameters for the tooltip\r\n- **.get()**\r\n\r\n get the current text of tooltip\r\n- **.hide()**\r\n\r\n disables the tooltip from appearing\r\n- **.show()**\r\n\r\n enable the tooltip again\r\n- **.is_disabled()**\r\n\r\n check the tooltip state\r\n \r\n### Thats all about CTkToolTips, hope it will help in your project!\r\n",
"bugtrack_url": null,
"license": "Creative Commons Zero v1.0 Universal",
"summary": "Customtkinter Tooltip widget",
"version": "0.8",
"project_urls": {
"Homepage": "https://github.com/Akascape/CTkToolTip"
},
"split_keywords": [
"customtkinter",
"tooltips",
"popup",
"widgets",
"tkinter-widgets",
"tooltip popup",
"floating window"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "aeb683d939da1d3e7d6b1202a8fe205b3fc08ef7a570dd6dfc18026bf144594c",
"md5": "6b637c01a9174af8f487effd5a8217a8",
"sha256": "dd91fc208596e14d0eea03eaa57b0d6095be995538ce2b87538e4266fa7a753d"
},
"downloads": -1,
"filename": "CTkToolTip-0.8-py3-none-any.whl",
"has_sig": false,
"md5_digest": "6b637c01a9174af8f487effd5a8217a8",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 8137,
"upload_time": "2023-08-08T09:41:16",
"upload_time_iso_8601": "2023-08-08T09:41:16.299677Z",
"url": "https://files.pythonhosted.org/packages/ae/b6/83d939da1d3e7d6b1202a8fe205b3fc08ef7a570dd6dfc18026bf144594c/CTkToolTip-0.8-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a74d0a9e703b0be6b36a12920c2f993c704a36a58df62552686d76a8c9e3886a",
"md5": "d16bda3cbcef0667bc57740d50796a10",
"sha256": "08596120b12c8fa9b9bff2931a3aef3a29719380def9a0a50380c3b6852b4564"
},
"downloads": -1,
"filename": "CTkToolTip-0.8.tar.gz",
"has_sig": false,
"md5_digest": "d16bda3cbcef0667bc57740d50796a10",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 8043,
"upload_time": "2023-08-08T09:41:18",
"upload_time_iso_8601": "2023-08-08T09:41:18.159364Z",
"url": "https://files.pythonhosted.org/packages/a7/4d/0a9e703b0be6b36a12920c2f993c704a36a58df62552686d76a8c9e3886a/CTkToolTip-0.8.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-08-08 09:41:18",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Akascape",
"github_project": "CTkToolTip",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "ctktooltip"
}