Name | ChatGPTWeb JSON |
Version |
0.2.46
JSON |
| download |
home_page | None |
Summary | ChatGPT PlayWright API |
upload_time | 2025-02-09 12:10:55 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.10 |
license | GPL3 |
keywords |
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# ChatGPT [](https://pypi.python.org/pypi/ChatGPTWeb)
ChatGPT playwright api,not openai api
一个不怎么使用网页的ChatGPT playwright api
# 待填坑 feature
- [x] 使用网页版 chatgpt | use chatgpt
- [x] 多人格预设与切换 | Multiple personality presets and switching
- [x] 聊天记录存储与导出 | Chat history storage and export
- [x] 自定义人设 | Customized persona
- [x] 重置聊天或回到某一时刻 | Reset a chat or go back to a certain moment
- [x] 多账号并发聊天 | Concurrent chatting with multiple accounts
- [x] 使用账号登录(暂不支持苹果)| Log in with your account (Apple is not supported yet)
- [x] GPT4 and PLUS
- [x] GPT4 upload file
- [ ] GPT4 download file
- [ ] 代码过于混乱等优化 | The code is too confusing and other optimizations
- [ ] 抽空完善readme | Take the time to improve the readme
# 安装/Install
linux & Windows
```bash
pip install ChatGPTWeb
playwright install firefox
```
### MsgData() 数据类型
```bash
from ChatGPTWeb.config import MsgData
class MsgData():
status: bool = False,
msg_type: typing.Optional[typing.Literal["old_session","back_loop","new_session"]] = "new_session",
msg_send: str = "hi",
# your msg
gpt_model: typing.Literal["text-davinci-002-render-sha", "gpt-4", "gpt-4o"] = "text-davinci-002-render-sha",
# if you use gpt4o by gptplus
msg_recv: str = "",
# gpt's msg
conversation_id: str = "",
# old session's conversation_id
p_msg_id: str = "",
# p_msg_id : the message's parent_message_id in this conversation id / 这个会话里某条消息的 parent_message_id
next_msg_id: str = "",
post_data: str = ""
arkose_data: str = "",
arkose_header: dict[str,str] = {},
arkose: str|None = ""
# if chatgpt use arkose
```
## 简单使用 / Simple practice
### copy __main__.py or this code to start / 复制 __main__.py 或者以下code来开始
```bash
import asyncio
from ChatGPTWeb import chatgpt
from ChatGPTWeb.config import Personality, MsgData
import aioconsole
sessions = [
{
"session_token": ""
},
{
"email": "xxx@hotmail.com",
"password": "",
# "mode":"openai" ,
"session_token": "",
},
{
"email": "xxx@outlook.com",
"password": "",
"mode": "microsoft",
"help": "xxxx@xx.com"
},
{
"email": "xxx@gmail.com",
"password": "",
"mode": "google"
},
,
{
"email": "xxx@hotmail.com",
"password": "",
"gptplus": True
}
]
# please remove account if u don't have | 请删除你不需要的登录方式
# if you only use session_token, automatic login after expiration is not supported | 仅使用session_token登录的话,不支持过期后的自动登录
# if you use an openai account to log in,
# pleases manually obtain the session_token in advance and add it together to reduce the possibility of openai verification
# 使用openai账号登录的话,请提前手动获取 session_token并一同添加,降低 openai 验证的可能性
personality_definition = Personality(
[
{
"name": "Programmer",
'value': 'You are python Programmer'
},
])
chat = chatgpt(sessions=sessions, begin_sleep_time=False, headless=True, stdout_flush=True)
# "begin_sleep_time=False" for testing only
# When using for the first time, if cloudflare exists, use headless=False and manually click Verify. After the session file is generated, switch it to True
# 初次使用时,如果存在cloudflare,请使用headless=False,并手动点击验证。在session文件生成后,再将其切换为True
async def main():
c_id = await aioconsole.ainput("your conversation_id if you have:")
# if u don't have,pleases enter empty
p_id = await aioconsole.ainput("your parent_message_id if you have:")
# if u don't have,pleases enter empty
data:MsgData = MsgData(conversation_id=c_id,p_msg_id=p_id)
while 1:
print("------------------------------")
data.msg_send = await aioconsole.ainput("input:")
print("------------------------------")
if data.msg_send == "quit":
break
elif data.msg_send == "gpt4o":
if data.gpt_model != "gpt-4o":
data.gpt_model = "gpt-4o"
data.conversation_id = ""
data.p_msg_id = ""
data.msg_send = await aioconsole.ainput("reinput:")
if data.msg_send == "what's the png":
with open("1.png","rb") as f:
file = IOFile(
content=f.read(),
name="1.png"
)
data.upload_file.append(file)
elif data.msg_send == "gpt3.5":
if data.gpt_model != "text-davinci-002-render-sha":
data.gpt_model = "text-davinci-002-render-sha"
data.conversation_id = ""
data.p_msg_id = ""
data.msg_send = await aioconsole.ainput("reinput:")
elif data.msg_send == "re":
data.msg_type = "back_loop"
data.p_msg_id = await aioconsole.ainput("your parent_message_id if you go back:")
elif data.msg_send == "reset":
data = await chat.back_init_personality(data)
print(f"ChatGPT:{data.msg_recv}")
continue
elif data.msg_send == "init_personality":
data.msg_send = "your ..."
data = await chat.init_personality(data)
print(f"ChatGPT:{data.msg_recv}")
continue
elif data.msg_send == "history":
print(await chat.show_chat_history(data))
continue
elif data.msg_send == "status":
print(await chat.token_status())
continue
data = await chat.continue_chat(data)
if data.msg_recv == '':
print(f"error:{data.error_info}")
else:
print(f"ChatGPT:{data.msg_recv}")
data.error_info = ""
data.msg_recv = ""
data.p_msg_id = ""
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
```
### chatgpt 类参数 / class chatgpt parameters
```bash
sessions: list[dict] = [],
# 参考 简单使用 ,暂不支持苹果账号,不写mode默认为openai账号
# Refer to Simple practice. Apple accounts are not supported for the time being.
# If you do not write "mode", the default is openai account.
proxy: typing.Optional[str] = None,
# proxy = "http://127.0.0.1:1090"
# proxy = "http://user:pass@127.0.0.1:1090"
# chatgpt(proxy=proxy)
# 要用代理的话就像这样 | proxy like it if u need use
chat_file: Path = Path("data", "chat_history", "conversation"),
# 聊天记录保存位置,一般不需修改
# The location where the chat history is saved, generally does not need to be modified.
personality: Optional[Personality] = Personality([{"name": "cat", "value": "you are a cat now."}]),
# 默认人格,用于初始化,推荐你使用类方法去添加你个人使用的
# The default personality is used for initialization. It is recommended that you use class methods to add your own personal
log_status: bool = True,
# 是否启用日志,默认开启,推荐在使用__main__.py进行测试时关闭
# Whether to enable logging. It is enabled by default. It is recommended to turn it off when using __main__.py for testing.
plugin: bool = False,
# 是否作为一个nonebot2插件使用(其实是插入进一个已经创建了的协程程序里)
# Whether to use it as a nonebot2 plug-in (actually inserted into an already created coroutine program)
headless: bool = True,
# 无头浏览器模式 | Headless browser mode
begin_sleep_time: bool = True,
# 启动时的随即等待时间,默认开启,推荐仅在少量账号测试时关闭
# The immediate waiting time at startup is enabled by default. It is recommended to turn it off only when testing with a small number of accounts.
logger_level: Literal["DEBUG", "INFO", "WARNING", "ERROR"] = "INFO"
# 日志等级,默认INFO
# logger_level
stdout_flush: bool = False
# shell流式传输
# command shell refresh output
save_screen: bool = False
# 发送消息与刷新cookie失败时保存异常截图到文件(登录失败的截图会一直保持开启)
# Save exception screenshots to files when sending messages and refreshing cookies fail (screenshots of login failures will always remain open)
```
## chatgpt 类方法 / class chatgpt method
```bash
chat = chatgpt(sessions=sessions)
```
### async def continue_chat(self, msg_data: MsgData) -> MsgData
```bash
# 聊天处理入口,一般用这个
# Message processing entry, please use this
msg_data = MsgData()
msg_data.msg_send = "your msg"
msg_data = await chat.continue_chat(msg_data)
print(msg_data.msg_recv)
```
### async def show_chat_history(self, msg_data: MsgData) -> list
```bash
# 获取保存的聊天记录
# Get saved chat history
msg_data = MsgData()
msg_data.conversation_id = You want to read the conversation_id of the record | 你想要读取记录的conversation_id
chat_history_list:list = await chat.show_chat_history(msg_data)
```
### async def back_chat_from_input(self, msg_data: MsgData) -> MsgData
```bash
# You can enter the text that appeared last time, or the number of dialogue rounds starts from 1 , or p_msg_id
# 通过输入来回溯,你可以输入最后一次出现过的文字,或者对话回合序号(从1开始),或者最后一次出现在聊天中的关键词,或者 p_msg_id
# Note: backtracking will not reset the recorded chat files,
# please pay attention to whether the content displayed in the chat records exists when backtracking again
# 注意:回溯不会重置记录的聊天文件,请注意再次回溯时聊天记录展示的内容是否存在
msg_data = MsgData()
...
msg_data.msg_send = "pleases call me Tom"
# 如果这是第5条消息 | If this is the 5th message
...
# 通过序号 | by index
msg_data.msg_send = "5"
# 通过关键词 | by keyword
msg_data.msg_send = "Tom"
msg_data.conversation_id = "xxx"
msg_data = await chat.back_chat_from_input(msg_data)
```
### async def init_personality(self, msg_data: MsgData) -> MsgData
```bash
# 使用指定的人设创建一个新会话
# Create a new conversation with the specified persona
msg_data = MsgData()
person_name = "你保存的人设名|Your saved persona name"
msg_data.msg_send = person_name
msg_data = await chat.init_personality(msg_data)
print(msg_data.msg_recv)
```
### async def back_init_personality(self, msg_data: MsgData) -> MsgData
```bash
# 回到刚初始化人设之后
# Go back to just after initializing the character settings
msg_data = MsgData()
msg_data.conversation_id = "xxx"
msg_data = await chat.back_init_personality()
print(msg_data.msg_recv)
```
### async def add_personality(self, personality: dict)
```bash
# add personality,please input json just like this.
# 添加人格 ,请传像这样的json数据
personality: dict = {"name":"cat1","value":"you are a cat now1."}
await chat.add_personality(personality)
```
### async def show_personality_list(self) -> str
```bash
# show_personality_list | 展示人格列表
name_list: str = await chat.show_personality_list()
```
### async def del_personality(self, name: str) -> str
```bash
# del_personality by name | 删除人格根据名字
pserson_name = "xxx"
name_list: str = await chat.del_personality(person_name)
```
### async token_status(self) -> dict
```bash
# get work status|查看session token状态和工作状态
status: dict = await chat.token_status()
# cid_num may not match the number of sessions, because it only records sessions with successful sessions, which will be automatically resolved after a period of time.
# cid_num 可能和session数量对不上,因为它只记录会话成功的session,这在允许一段时间后会自动解决
```
## 在协程中使用 | use in Coroutine
```bash
chat = chatgpt(sessions=sessions, begin_sleep_time=False, headless=True, log_status=False, plugin=True)
async def any_async_method():
loop = asyncio.get_event_loop()
asyncio.run_coroutine_threadsafe(chat.__start__(loop),loop)
```
## 手动获取 session_token 的方法 | How to manually obtain session_token
After opening chat.openai.com and logging in, press F12 on the browser to open the developer tools and find the following cookies
打开chat.openai.com登录后,按下浏览器的F12以打开开发者工具,找到以下Cookie
[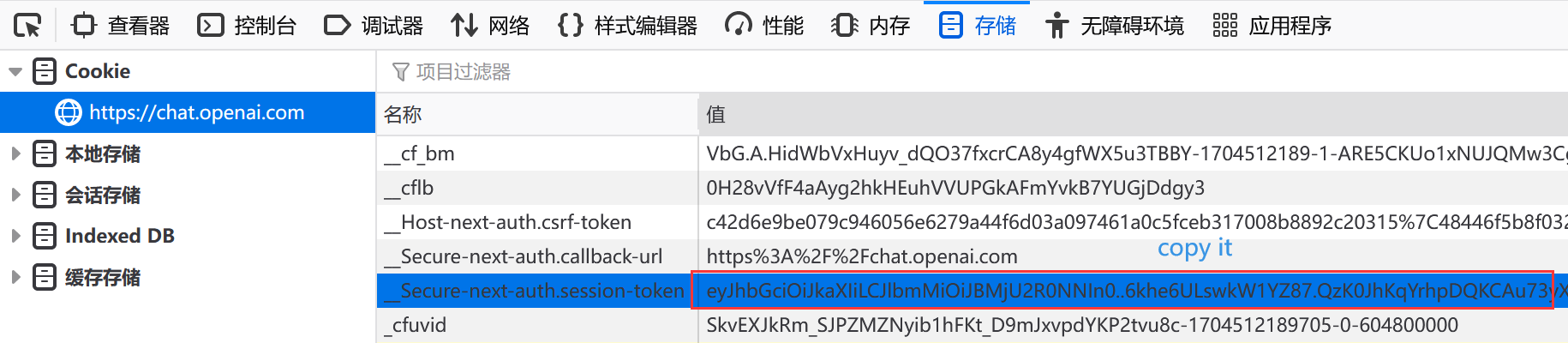](https://imgse.com/i/pizimDg)
## 可能遇到的问题 | possible problems
### 微软登录辅助邮箱验证 | microsoft email verify code
A file will be generated in the startup directory. Please put the verification code into it and save it. Pay attention to the log prompts.
启动目录下会生成文件,请将验证码填入其中并保存,注意日志提示
### openai登录辅助邮箱验证 | openai email verify code
Same microsoft.A file will be generated in the startup directory. Please put the verification code into it and save it. Pay attention to the log prompts.
和上面微软邮箱一样。启动目录下会生成文件,请将验证码填入其中并保存,注意日志提示
### 谷歌登录 | google login
Please log in to chatgpt manually using Google from your browser once, then visit `https://myaccount.google.com/` and use the browser plug-in Cookie-Editor to export the cookies of this page in json format.
When the "\{email_address\}_google_cookie.txt" file appears, paste the copied json into it and save it.
请先从你的浏览器手动使用google登录chatgpt一次,然后访问`https://myaccount.google.com/`,使用浏览器插件Cookie-Editor导出该页面的Cookie为json格式。 当"\{email_address\}_google_cookie.txt"文件出现时,将复制的json粘贴进去并保存。
### cloudflare checkbox 验证挑战
When using for the first time, if cloudflare exists, use headless=False and manually click Verify. After the session file is generated, switch it to True
初次使用时,如果存在cloudflare,请使用headless=False,并手动点击验证。在session文件生成后,再将其切换为True
Raw data
{
"_id": null,
"home_page": null,
"name": "ChatGPTWeb",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": null,
"keywords": null,
"author": null,
"author_email": "nek0us <nekouss@mail.com>",
"download_url": "https://files.pythonhosted.org/packages/e7/8b/c4d9e2c5a6979cbfb45291d2a9513c24975c220818461457b2725568a71b/ChatGPTWeb-0.2.46.tar.gz",
"platform": null,
"description": "# ChatGPT [](https://pypi.python.org/pypi/ChatGPTWeb)\nChatGPT playwright api,not openai api\n\n\u4e00\u4e2a\u4e0d\u600e\u4e48\u4f7f\u7528\u7f51\u9875\u7684ChatGPT playwright api\n\n\n# \u5f85\u586b\u5751 feature\n- [x] \u4f7f\u7528\u7f51\u9875\u7248 chatgpt | use chatgpt\n- [x] \u591a\u4eba\u683c\u9884\u8bbe\u4e0e\u5207\u6362 | Multiple personality presets and switching\n- [x] \u804a\u5929\u8bb0\u5f55\u5b58\u50a8\u4e0e\u5bfc\u51fa | Chat history storage and export\n- [x] \u81ea\u5b9a\u4e49\u4eba\u8bbe | Customized persona\n- [x] \u91cd\u7f6e\u804a\u5929\u6216\u56de\u5230\u67d0\u4e00\u65f6\u523b | Reset a chat or go back to a certain moment\n- [x] \u591a\u8d26\u53f7\u5e76\u53d1\u804a\u5929 | Concurrent chatting with multiple accounts\n- [x] \u4f7f\u7528\u8d26\u53f7\u767b\u5f55\uff08\u6682\u4e0d\u652f\u6301\u82f9\u679c\uff09| Log in with your account (Apple is not supported yet)\n- [x] GPT4 and PLUS\n- [x] GPT4 upload file\n- [ ] GPT4 download file\n- [ ] \u4ee3\u7801\u8fc7\u4e8e\u6df7\u4e71\u7b49\u4f18\u5316 | The code is too confusing and other optimizations\n- [ ] \u62bd\u7a7a\u5b8c\u5584readme | Take the time to improve the readme\n\n\n# \u5b89\u88c5/Install\nlinux & Windows\n\n```bash\npip install ChatGPTWeb\nplaywright install firefox\n```\n\n\n### MsgData() \u6570\u636e\u7c7b\u578b\n```bash \nfrom ChatGPTWeb.config import MsgData\n\nclass MsgData(): \n status: bool = False,\n msg_type: typing.Optional[typing.Literal[\"old_session\",\"back_loop\",\"new_session\"]] = \"new_session\",\n msg_send: str = \"hi\",\n # your msg \n gpt_model: typing.Literal[\"text-davinci-002-render-sha\", \"gpt-4\", \"gpt-4o\"] = \"text-davinci-002-render-sha\",\n # if you use gpt4o by gptplus\n msg_recv: str = \"\",\n # gpt's msg\n conversation_id: str = \"\",\n # old session's conversation_id\n p_msg_id: str = \"\",\n # p_msg_id : the message's parent_message_id in this conversation id / \u8fd9\u4e2a\u4f1a\u8bdd\u91cc\u67d0\u6761\u6d88\u606f\u7684 parent_message_id\n next_msg_id: str = \"\",\n post_data: str = \"\"\n arkose_data: str = \"\",\n arkose_header: dict[str,str] = {},\n arkose: str|None = \"\"\n # if chatgpt use arkose\n```\n## \u7b80\u5355\u4f7f\u7528 / Simple practice\n\n### copy __main__.py or this code to start / \u590d\u5236 __main__.py \u6216\u8005\u4ee5\u4e0bcode\u6765\u5f00\u59cb\n```bash\nimport asyncio\nfrom ChatGPTWeb import chatgpt\nfrom ChatGPTWeb.config import Personality, MsgData\nimport aioconsole\n\nsessions = [\n {\n \"session_token\": \"\"\n\n },\n {\n \"email\": \"xxx@hotmail.com\",\n \"password\": \"\",\n # \"mode\":\"openai\" ,\n \"session_token\": \"\",\n },\n {\n \"email\": \"xxx@outlook.com\",\n \"password\": \"\",\n \"mode\": \"microsoft\",\n \"help\": \"xxxx@xx.com\"\n },\n {\n \"email\": \"xxx@gmail.com\",\n \"password\": \"\",\n \"mode\": \"google\"\n },\n ,\n {\n \"email\": \"xxx@hotmail.com\",\n \"password\": \"\",\n \"gptplus\": True\n }\n]\n# please remove account if u don't have | \u8bf7\u5220\u9664\u4f60\u4e0d\u9700\u8981\u7684\u767b\u5f55\u65b9\u5f0f \n# if you only use session_token, automatic login after expiration is not supported | \u4ec5\u4f7f\u7528session_token\u767b\u5f55\u7684\u8bdd\uff0c\u4e0d\u652f\u6301\u8fc7\u671f\u540e\u7684\u81ea\u52a8\u767b\u5f55\n# if you use an openai account to log in, \n# pleases manually obtain the session_token in advance and add it together to reduce the possibility of openai verification\n# \u4f7f\u7528openai\u8d26\u53f7\u767b\u5f55\u7684\u8bdd\uff0c\u8bf7\u63d0\u524d\u624b\u52a8\u83b7\u53d6 session_token\u5e76\u4e00\u540c\u6dfb\u52a0\uff0c\u964d\u4f4e openai \u9a8c\u8bc1\u7684\u53ef\u80fd\u6027\n\npersonality_definition = Personality(\n [\n {\n \"name\": \"Programmer\",\n 'value': 'You are python Programmer'\n },\n ])\n\nchat = chatgpt(sessions=sessions, begin_sleep_time=False, headless=True, stdout_flush=True)\n# \"begin_sleep_time=False\" for testing only\n# When using for the first time, if cloudflare exists, use headless=False and manually click Verify. After the session file is generated, switch it to True\n# \u521d\u6b21\u4f7f\u7528\u65f6\uff0c\u5982\u679c\u5b58\u5728cloudflare\uff0c\u8bf7\u4f7f\u7528headless=False\uff0c\u5e76\u624b\u52a8\u70b9\u51fb\u9a8c\u8bc1\u3002\u5728session\u6587\u4ef6\u751f\u6210\u540e\uff0c\u518d\u5c06\u5176\u5207\u6362\u4e3aTrue\n\nasync def main():\n c_id = await aioconsole.ainput(\"your conversation_id if you have:\")\n # if u don't have,pleases enter empty\n p_id = await aioconsole.ainput(\"your parent_message_id if you have:\")\n # if u don't have,pleases enter empty\n data:MsgData = MsgData(conversation_id=c_id,p_msg_id=p_id)\n while 1:\n print(\"------------------------------\")\n data.msg_send = await aioconsole.ainput(\"input\uff1a\")\n print(\"------------------------------\")\n if data.msg_send == \"quit\":\n break\n elif data.msg_send == \"gpt4o\":\n if data.gpt_model != \"gpt-4o\":\n data.gpt_model = \"gpt-4o\"\n data.conversation_id = \"\"\n data.p_msg_id = \"\"\n data.msg_send = await aioconsole.ainput(\"reinput\uff1a\")\n if data.msg_send == \"what's the png\":\n with open(\"1.png\",\"rb\") as f:\n file = IOFile(\n content=f.read(),\n name=\"1.png\"\n )\n data.upload_file.append(file)\n elif data.msg_send == \"gpt3.5\":\n if data.gpt_model != \"text-davinci-002-render-sha\":\n data.gpt_model = \"text-davinci-002-render-sha\"\n data.conversation_id = \"\"\n data.p_msg_id = \"\"\n data.msg_send = await aioconsole.ainput(\"reinput\uff1a\")\n elif data.msg_send == \"re\":\n data.msg_type = \"back_loop\"\n data.p_msg_id = await aioconsole.ainput(\"your parent_message_id if you go back:\")\n elif data.msg_send == \"reset\":\n data = await chat.back_init_personality(data)\n print(f\"ChatGPT:{data.msg_recv}\")\n continue\n elif data.msg_send == \"init_personality\":\n data.msg_send = \"your ...\"\n data = await chat.init_personality(data)\n print(f\"ChatGPT:{data.msg_recv}\")\n continue\n elif data.msg_send == \"history\":\n print(await chat.show_chat_history(data))\n continue\n elif data.msg_send == \"status\":\n print(await chat.token_status())\n continue\n data = await chat.continue_chat(data)\n if data.msg_recv == '':\n print(f\"error:{data.error_info}\")\n else:\n print(f\"ChatGPT:{data.msg_recv}\")\n data.error_info = \"\"\n data.msg_recv = \"\"\n data.p_msg_id = \"\"\n \n \nloop = asyncio.get_event_loop()\nloop.run_until_complete(main()) \n```\n\n### chatgpt \u7c7b\u53c2\u6570 / class chatgpt parameters\n```bash\nsessions: list[dict] = [],\n# \u53c2\u8003 \u7b80\u5355\u4f7f\u7528 \uff0c\u6682\u4e0d\u652f\u6301\u82f9\u679c\u8d26\u53f7\uff0c\u4e0d\u5199mode\u9ed8\u8ba4\u4e3aopenai\u8d26\u53f7\n# Refer to Simple practice. Apple accounts are not supported for the time being. \n# If you do not write \"mode\", the default is openai account.\n\nproxy: typing.Optional[str] = None,\n# proxy = \"http://127.0.0.1:1090\"\n# proxy = \"http://user:pass@127.0.0.1:1090\"\n# chatgpt(proxy=proxy)\n# \u8981\u7528\u4ee3\u7406\u7684\u8bdd\u5c31\u50cf\u8fd9\u6837 | proxy like it if u need use\n\nchat_file: Path = Path(\"data\", \"chat_history\", \"conversation\"),\n# \u804a\u5929\u8bb0\u5f55\u4fdd\u5b58\u4f4d\u7f6e\uff0c\u4e00\u822c\u4e0d\u9700\u4fee\u6539\n# The location where the chat history is saved, generally does not need to be modified.\n\npersonality: Optional[Personality] = Personality([{\"name\": \"cat\", \"value\": \"you are a cat now.\"}]),\n# \u9ed8\u8ba4\u4eba\u683c\uff0c\u7528\u4e8e\u521d\u59cb\u5316\uff0c\u63a8\u8350\u4f60\u4f7f\u7528\u7c7b\u65b9\u6cd5\u53bb\u6dfb\u52a0\u4f60\u4e2a\u4eba\u4f7f\u7528\u7684\n# The default personality is used for initialization. It is recommended that you use class methods to add your own personal\n\nlog_status: bool = True,\n# \u662f\u5426\u542f\u7528\u65e5\u5fd7\uff0c\u9ed8\u8ba4\u5f00\u542f\uff0c\u63a8\u8350\u5728\u4f7f\u7528__main__.py\u8fdb\u884c\u6d4b\u8bd5\u65f6\u5173\u95ed\n# Whether to enable logging. It is enabled by default. It is recommended to turn it off when using __main__.py for testing.\n\nplugin: bool = False,\n# \u662f\u5426\u4f5c\u4e3a\u4e00\u4e2anonebot2\u63d2\u4ef6\u4f7f\u7528\uff08\u5176\u5b9e\u662f\u63d2\u5165\u8fdb\u4e00\u4e2a\u5df2\u7ecf\u521b\u5efa\u4e86\u7684\u534f\u7a0b\u7a0b\u5e8f\u91cc\uff09\n# Whether to use it as a nonebot2 plug-in (actually inserted into an already created coroutine program)\n\nheadless: bool = True,\n# \u65e0\u5934\u6d4f\u89c8\u5668\u6a21\u5f0f | Headless browser mode\n\nbegin_sleep_time: bool = True,\n# \u542f\u52a8\u65f6\u7684\u968f\u5373\u7b49\u5f85\u65f6\u95f4\uff0c\u9ed8\u8ba4\u5f00\u542f\uff0c\u63a8\u8350\u4ec5\u5728\u5c11\u91cf\u8d26\u53f7\u6d4b\u8bd5\u65f6\u5173\u95ed\n# The immediate waiting time at startup is enabled by default. It is recommended to turn it off only when testing with a small number of accounts.\n\nlogger_level: Literal[\"DEBUG\", \"INFO\", \"WARNING\", \"ERROR\"] = \"INFO\"\n# \u65e5\u5fd7\u7b49\u7ea7\uff0c\u9ed8\u8ba4INFO\n# logger_level\n\nstdout_flush: bool = False\n# shell\u6d41\u5f0f\u4f20\u8f93\n# command shell refresh output\n\nsave_screen: bool = False\n# \u53d1\u9001\u6d88\u606f\u4e0e\u5237\u65b0cookie\u5931\u8d25\u65f6\u4fdd\u5b58\u5f02\u5e38\u622a\u56fe\u5230\u6587\u4ef6\uff08\u767b\u5f55\u5931\u8d25\u7684\u622a\u56fe\u4f1a\u4e00\u76f4\u4fdd\u6301\u5f00\u542f\uff09\n# Save exception screenshots to files when sending messages and refreshing cookies fail (screenshots of login failures will always remain open)\n\n```\n\n## chatgpt \u7c7b\u65b9\u6cd5 / class chatgpt method\n```bash\nchat = chatgpt(sessions=sessions)\n```\n### async def continue_chat(self, msg_data: MsgData) -> MsgData\n```bash\n# \u804a\u5929\u5904\u7406\u5165\u53e3\uff0c\u4e00\u822c\u7528\u8fd9\u4e2a\n# Message processing entry, please use this\nmsg_data = MsgData()\nmsg_data.msg_send = \"your msg\" \nmsg_data = await chat.continue_chat(msg_data) \nprint(msg_data.msg_recv) \n```\n### async def show_chat_history(self, msg_data: MsgData) -> list\n```bash\n# \u83b7\u53d6\u4fdd\u5b58\u7684\u804a\u5929\u8bb0\u5f55\n# Get saved chat history\nmsg_data = MsgData()\nmsg_data.conversation_id = You want to read the conversation_id of the record | \u4f60\u60f3\u8981\u8bfb\u53d6\u8bb0\u5f55\u7684conversation_id\nchat_history_list:list = await chat.show_chat_history(msg_data) \n```\n### async def back_chat_from_input(self, msg_data: MsgData) -> MsgData\n```bash\n# You can enter the text that appeared last time, or the number of dialogue rounds starts from 1 , or p_msg_id\n# \u901a\u8fc7\u8f93\u5165\u6765\u56de\u6eaf,\u4f60\u53ef\u4ee5\u8f93\u5165\u6700\u540e\u4e00\u6b21\u51fa\u73b0\u8fc7\u7684\u6587\u5b57\uff0c\u6216\u8005\u5bf9\u8bdd\u56de\u5408\u5e8f\u53f7(\u4ece1\u5f00\u59cb)\uff0c\u6216\u8005\u6700\u540e\u4e00\u6b21\u51fa\u73b0\u5728\u804a\u5929\u4e2d\u7684\u5173\u952e\u8bcd\uff0c\u6216\u8005 p_msg_id\n\n# Note: backtracking will not reset the recorded chat files,\n# please pay attention to whether the content displayed in the chat records exists when backtracking again\n\n# \u6ce8\u610f\uff1a\u56de\u6eaf\u4e0d\u4f1a\u91cd\u7f6e\u8bb0\u5f55\u7684\u804a\u5929\u6587\u4ef6\uff0c\u8bf7\u6ce8\u610f\u518d\u6b21\u56de\u6eaf\u65f6\u804a\u5929\u8bb0\u5f55\u5c55\u793a\u7684\u5185\u5bb9\u662f\u5426\u5b58\u5728\n\nmsg_data = MsgData()\n...\nmsg_data.msg_send = \"pleases call me Tom\" \n# \u5982\u679c\u8fd9\u662f\u7b2c5\u6761\u6d88\u606f | If this is the 5th message\n...\n\n# \u901a\u8fc7\u5e8f\u53f7 | by index\nmsg_data.msg_send = \"5\"\n# \u901a\u8fc7\u5173\u952e\u8bcd | by keyword\nmsg_data.msg_send = \"Tom\"\n\nmsg_data.conversation_id = \"xxx\"\nmsg_data = await chat.back_chat_from_input(msg_data)\n```\n### async def init_personality(self, msg_data: MsgData) -> MsgData\n```bash\n# \u4f7f\u7528\u6307\u5b9a\u7684\u4eba\u8bbe\u521b\u5efa\u4e00\u4e2a\u65b0\u4f1a\u8bdd\n# Create a new conversation with the specified persona\nmsg_data = MsgData()\nperson_name = \"\u4f60\u4fdd\u5b58\u7684\u4eba\u8bbe\u540d|Your saved persona name\"\nmsg_data.msg_send = person_name\nmsg_data = await chat.init_personality(msg_data)\nprint(msg_data.msg_recv)\n```\n### async def back_init_personality(self, msg_data: MsgData) -> MsgData\n```bash\n# \u56de\u5230\u521a\u521d\u59cb\u5316\u4eba\u8bbe\u4e4b\u540e\n# Go back to just after initializing the character settings\nmsg_data = MsgData()\nmsg_data.conversation_id = \"xxx\"\nmsg_data = await chat.back_init_personality()\nprint(msg_data.msg_recv)\n```\n### async def add_personality(self, personality: dict)\n```bash\n# add personality,please input json just like this.\n# \u6dfb\u52a0\u4eba\u683c ,\u8bf7\u4f20\u50cf\u8fd9\u6837\u7684json\u6570\u636e\npersonality: dict = {\"name\":\"cat1\",\"value\":\"you are a cat now1.\"}\nawait chat.add_personality(personality)\n```\n### async def show_personality_list(self) -> str\n```bash\n# show_personality_list | \u5c55\u793a\u4eba\u683c\u5217\u8868\nname_list: str = await chat.show_personality_list()\n```\n### async def del_personality(self, name: str) -> str\n```bash\n# del_personality by name | \u5220\u9664\u4eba\u683c\u6839\u636e\u540d\u5b57\npserson_name = \"xxx\"\nname_list: str = await chat.del_personality(person_name)\n```\n### async token_status(self) -> dict\n```bash\n# get work status|\u67e5\u770bsession token\u72b6\u6001\u548c\u5de5\u4f5c\u72b6\u6001\nstatus: dict = await chat.token_status()\n# cid_num may not match the number of sessions, because it only records sessions with successful sessions, which will be automatically resolved after a period of time. \n# cid_num \u53ef\u80fd\u548csession\u6570\u91cf\u5bf9\u4e0d\u4e0a\uff0c\u56e0\u4e3a\u5b83\u53ea\u8bb0\u5f55\u4f1a\u8bdd\u6210\u529f\u7684session\uff0c\u8fd9\u5728\u5141\u8bb8\u4e00\u6bb5\u65f6\u95f4\u540e\u4f1a\u81ea\u52a8\u89e3\u51b3\n```\n\n## \u5728\u534f\u7a0b\u4e2d\u4f7f\u7528 | use in Coroutine\n```bash\nchat = chatgpt(sessions=sessions, begin_sleep_time=False, headless=True, log_status=False, plugin=True)\n\nasync def any_async_method():\n loop = asyncio.get_event_loop()\n asyncio.run_coroutine_threadsafe(chat.__start__(loop),loop)\n```\n\n## \u624b\u52a8\u83b7\u53d6 session_token \u7684\u65b9\u6cd5 | How to manually obtain session_token\nAfter opening chat.openai.com and logging in, press F12 on the browser to open the developer tools and find the following cookies\n\n\u6253\u5f00chat.openai.com\u767b\u5f55\u540e\uff0c\u6309\u4e0b\u6d4f\u89c8\u5668\u7684F12\u4ee5\u6253\u5f00\u5f00\u53d1\u8005\u5de5\u5177\uff0c\u627e\u5230\u4ee5\u4e0bCookie\n\n[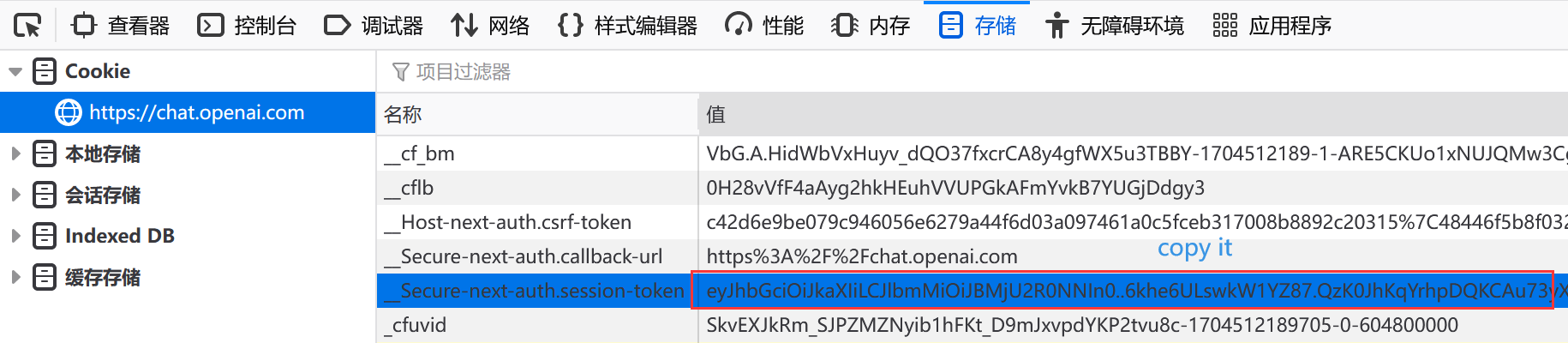](https://imgse.com/i/pizimDg)\n\n## \u53ef\u80fd\u9047\u5230\u7684\u95ee\u9898 | possible problems\n### \u5fae\u8f6f\u767b\u5f55\u8f85\u52a9\u90ae\u7bb1\u9a8c\u8bc1 | microsoft email verify code\nA file will be generated in the startup directory. Please put the verification code into it and save it. Pay attention to the log prompts.\n\n\u542f\u52a8\u76ee\u5f55\u4e0b\u4f1a\u751f\u6210\u6587\u4ef6\uff0c\u8bf7\u5c06\u9a8c\u8bc1\u7801\u586b\u5165\u5176\u4e2d\u5e76\u4fdd\u5b58\uff0c\u6ce8\u610f\u65e5\u5fd7\u63d0\u793a\n\n### openai\u767b\u5f55\u8f85\u52a9\u90ae\u7bb1\u9a8c\u8bc1 | openai email verify code\nSame microsoft.A file will be generated in the startup directory. Please put the verification code into it and save it. Pay attention to the log prompts.\n\n\u548c\u4e0a\u9762\u5fae\u8f6f\u90ae\u7bb1\u4e00\u6837\u3002\u542f\u52a8\u76ee\u5f55\u4e0b\u4f1a\u751f\u6210\u6587\u4ef6\uff0c\u8bf7\u5c06\u9a8c\u8bc1\u7801\u586b\u5165\u5176\u4e2d\u5e76\u4fdd\u5b58\uff0c\u6ce8\u610f\u65e5\u5fd7\u63d0\u793a\n\n### \u8c37\u6b4c\u767b\u5f55 | google login \nPlease log in to chatgpt manually using Google from your browser once, then visit `https://myaccount.google.com/` and use the browser plug-in Cookie-Editor to export the cookies of this page in json format.\nWhen the \"\\{email_address\\}_google_cookie.txt\" file appears, paste the copied json into it and save it.\n\n\u8bf7\u5148\u4ece\u4f60\u7684\u6d4f\u89c8\u5668\u624b\u52a8\u4f7f\u7528google\u767b\u5f55chatgpt\u4e00\u6b21\uff0c\u7136\u540e\u8bbf\u95ee`https://myaccount.google.com/`\uff0c\u4f7f\u7528\u6d4f\u89c8\u5668\u63d2\u4ef6Cookie-Editor\u5bfc\u51fa\u8be5\u9875\u9762\u7684Cookie\u4e3ajson\u683c\u5f0f\u3002 \u5f53\"\\{email_address\\}_google_cookie.txt\"\u6587\u4ef6\u51fa\u73b0\u65f6\uff0c\u5c06\u590d\u5236\u7684json\u7c98\u8d34\u8fdb\u53bb\u5e76\u4fdd\u5b58\u3002\n\n### cloudflare checkbox \u9a8c\u8bc1\u6311\u6218\nWhen using for the first time, if cloudflare exists, use headless=False and manually click Verify. After the session file is generated, switch it to True\n\n\u521d\u6b21\u4f7f\u7528\u65f6\uff0c\u5982\u679c\u5b58\u5728cloudflare\uff0c\u8bf7\u4f7f\u7528headless=False\uff0c\u5e76\u624b\u52a8\u70b9\u51fb\u9a8c\u8bc1\u3002\u5728session\u6587\u4ef6\u751f\u6210\u540e\uff0c\u518d\u5c06\u5176\u5207\u6362\u4e3aTrue\n\n",
"bugtrack_url": null,
"license": "GPL3",
"summary": "ChatGPT PlayWright API",
"version": "0.2.46",
"project_urls": {
"Homepage": "https://github.com/nek0us/ChatGPT",
"Repository": "https://github.com/nek0us/ChatGPT"
},
"split_keywords": [],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "fbfba92efbe7c856f508993310034a74289c14eb53e44e19c36b9d3672f856cc",
"md5": "63ac8a557a93413495152dc21b4bea62",
"sha256": "5b6b2804b5374a6aa5f8fbaa704a6f2ad20400d9f2ae3f6c747b3bdc4635e725"
},
"downloads": -1,
"filename": "ChatGPTWeb-0.2.46-py3-none-any.whl",
"has_sig": false,
"md5_digest": "63ac8a557a93413495152dc21b4bea62",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10",
"size": 56279,
"upload_time": "2025-02-09T12:10:54",
"upload_time_iso_8601": "2025-02-09T12:10:54.414549Z",
"url": "https://files.pythonhosted.org/packages/fb/fb/a92efbe7c856f508993310034a74289c14eb53e44e19c36b9d3672f856cc/ChatGPTWeb-0.2.46-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "e78bc4d9e2c5a6979cbfb45291d2a9513c24975c220818461457b2725568a71b",
"md5": "69d33076dce895d38250eec21ab5e16e",
"sha256": "a3f9bac15981203ff4093875bbac589d82c19bd6013a5b554d4e8a836210395c"
},
"downloads": -1,
"filename": "ChatGPTWeb-0.2.46.tar.gz",
"has_sig": false,
"md5_digest": "69d33076dce895d38250eec21ab5e16e",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10",
"size": 53742,
"upload_time": "2025-02-09T12:10:55",
"upload_time_iso_8601": "2025-02-09T12:10:55.557630Z",
"url": "https://files.pythonhosted.org/packages/e7/8b/c4d9e2c5a6979cbfb45291d2a9513c24975c220818461457b2725568a71b/ChatGPTWeb-0.2.46.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-02-09 12:10:55",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "nek0us",
"github_project": "ChatGPT",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "chatgptweb"
}