# Dans_Diffaction
Reads crystallographic cif files, calculates crystal properties and simulates diffraction.
**Version 3.0**
[](https://doi.org/10.5281/zenodo.7725644)
[](https://mybinder.org/v2/gh/DanPorter/Dans_Diffraction/6be78ef800167276d61d3e73da3b74a8367dbbe7?urlpath=lab%2Ftree%2FDans_Diffraction.ipynb)
[](https://github.com/DanPorter/Dans_Diffraction)
By Dan Porter, Diamond Light Source
2023
#### TL;DR:
```text
$ ipython -i -m Dans_Diffraction
OR
$ ipython -m Dans_Diffraction gui
```
```python
"""Python Sctipt"""
import Dans_Diffraction as dif
xtl = dif.Crystal('some_file.cif')
print(xtl) # print Crystal structure parameters
# Print reflection list:
print(xtl.Scatter.print_all_reflections(energy_kev=5))
# Plot Powder pattern:
xtl.Plot.simulate_powder(energy_kev=8)
plt.show()
# Start graphical user interface:
xtl.start_gui()
```
Full code documentation available [here](https://danporter.github.io/Dans_Diffraction/).
Try it out on [mybinder!](https://mybinder.org/v2/gh/DanPorter/Dans_Diffraction/6be78ef800167276d61d3e73da3b74a8367dbbe7?urlpath=lab%2Ftree%2FDans_Diffraction.ipynb)
For comments, queries or bugs - email [dan.porter@diamond.ac.uk](mailto:dan.porter@diamond.ac.uk)
**Citation:** If you use this code (great!), please cite the published DOI: [10.5281/zenodo.3859501](https://doi.org/10.5281/zenodo.3859501)
# Installation
**Requirements:**
Python 3+ with packages: *Numpy*, *Matplotlib*, *Tkinter*.
BuiltIn packages used: *sys*, *os*, *re*, *glob*, *warnings*, *json*, *itertools*
Install stable version from PyPI:
```text
$ python -m pip install Dans-Diffraction
```
Or, install the latest version direct from GitHub:
```text
$ python -m pip install git+https://github.com/DanPorter/Dans_Diffraction.git
```
Or, Download the latest version from GitHub (with examples!):
```text
$ git clone https://github.com/DanPorter/Dans_Diffraction.git
```
# Operation
Dans_Diffraction is best run within an interactive python environment:
```text
$ ipython -i -m Dans_Diffraction
```
Dans_Diffraction can also be run in scripts as an import, example scripts are provided in the [Examples](https://github.com/DanPorter/Dans_Diffraction/blob/master/Examples) folder.
### Read CIF file
```python
import Dans_Diffraction as dif
xtl = dif.Crystal('some_file.cif')
xtl.info() # print Crystal structure parameters
help(xtl) # all functions (nearly!) are documented
```
### Alter atomic positions
```python
xtl.Cell.latt([2.85,2.85,10.8,90,90,120]) # set lattice parameters
xtl.Atoms.info() # Print Symmetric positions
xtl.Structure.info() # Print All positions in P1 symmetry (same structure and functions as xtl.Atoms)
# Symmetric positions
xtl.Atoms.changeatom(idx=0, u=0, v=0, w=0, type='Co', label='Co1')
xtl.Atoms.addatom(idx=0, u=0, v=0, w=0, type='Co', label='Co1')
# After adding or changing an atom in the Atoms class, re-generate the full structure using symmetry arguments:
xtl.generate_lattice()
# Full atomic structure in P1 symmetry
xtl.Structure.changeatom(idx=0, u=0, v=0, w=0, type='Co', label='Co1')
xtl.Structure.addatom(idx=0, u=0, v=0, w=0, type='Co', label='Co1')
# Plot crystal Structure
xtl.Plot.plot_crystal() # 3D plot
xtl.Plot.plot_layers() # 2D plot for layered materials
```

### Alter crystal symmetry
```python
xtl.Symmetry.info() # print symmetry arguments
xtl.Symmetry.addsym('x,y,z+1/2') # adds single symmetry operation
xtl.Symmetry.changesym(0, 'x,y,z+1/4')
xtl.Symmetry.load_spacegroup(194) # replaces current symmetry operations
# After adding or changing symmetry operations, regengerate the symmetry matrices
xtl.Symmetry.generate_matrices()
```
### Save structure as CIF
Lattice parameters, crystal structure and symmetry operations will be saved to the CIF.
If magnetic moments are defined, magnetic symmetry operations and moments will also be saved
and format changed to "*.mcif".
```python
xtl.write_cif('edited file.cif')
```
### Calculate Structure Factors
X-ray or neutron structure factors/ intensities are calculated based on the full unit cell structure, including atomic
form-factors (x-rays) or coherent scattering lengths (neutrons).
```python
# Choose scattering options (see help(xtl.Scatter.setup_scatter))
xtl.Scatter.setup_scatter(type='x-ray', energy_keV=8.0)
# Allowed radiation types:
# 'xray','neutron','xray magnetic','neutron magnetic','xray resonant'
xtl.Scatter.print_all_refelctions() # Returns formated string of all allowed reflections
inten = xtl.Scatter.intensity([h,k,l]) # Returns intensity
twotheta, iten, reflections = xtl.Scatter.powder(units='twotheta')
# Plot Experimental Intensities
xtl.Plot.simulate_powder() # Powder pattern
xtl.Plot.simulate_hk0() # Reciprocal space plane
```

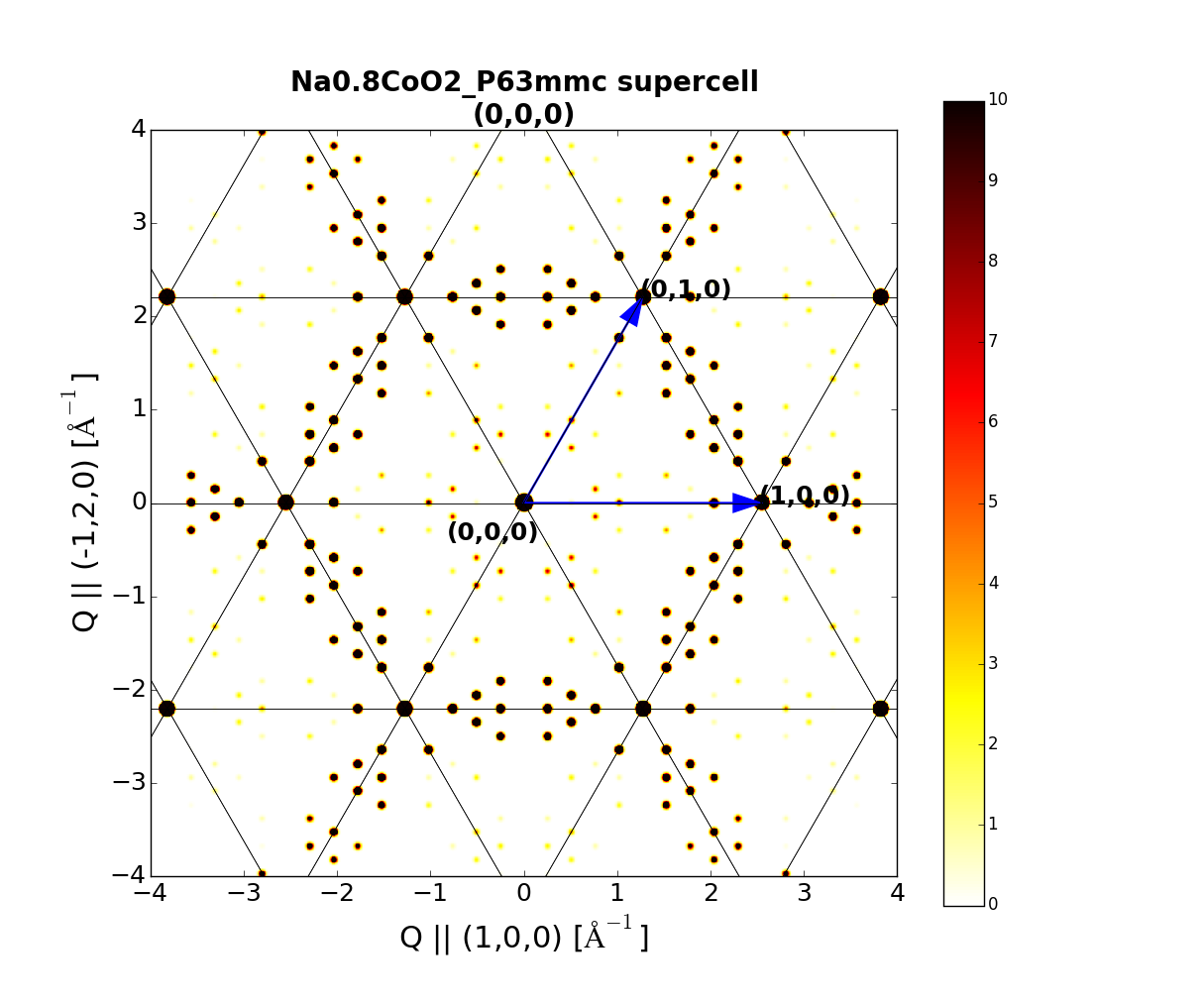
### Magnetic Structrues
*Magnetic structures and scattering are currently in development and shouldn't be treated as accurate!*
Simple magnetic structures can be loaded from magnetic cif (*.mcif) files. Magnetic moments are stored for each atomic
position as a vector. The crystal object has a seperate set of magnetic symmetry operations. Symmetry operations from the
tables of magnetic spacegroups can also be loaded. Only simple magnetic structures are allowed. There must be the same
number of magnetic symmetry operations as crystal symmetry operations and atomic positions can only have single moments
assigned.
```python
xtl = dif.Crystal('some_file.mcif')
xtl.Atoms.mxmymz() # return magnetic moment vectors on each ion
xtl.Symmetry.symmetry_operations_magnetic # magnetic symmetry operations (list of strings)
xtl.Symmetry.print_magnetic_spacegroups() # return str of available magnetic spacegroups, given crystal's spacegroup
xtl.Symmetry.load_magnetic_spacegroup(mag_spg_number) # loads mag. operations given mag. spacegroup number
```
Magnetic scattering is also available for neutrons and x-rays (both resonant and non-resonant), using the appropriate magnetic form-factors.
```python
Imag = xtl.Scatter.magnetic_neutron(HKL=[0,0,3])
Ires = xtl.Scatter.xray_resonant_magnetic(HKL=[0,0,3], energy_kev=2.838, azim_zero=[1, 0, 0], psi=0, polarisation='s-p', F0=0, F1=1, F2=0)
```
### Superstructures
Superstructures can be built using the Superstructure class, requring only a matrix to define the new phase:
```python
su = xtl.generate_superstructure([[2,0,0],[0,2,0],[0,0,1]])
```
Superstucture classes behave like Crystal classes, but have an additional 'Parent' property that references the original
crystal structure and additional behaviours partiular to superstructures. Superstructures loose their parent crystal and
magnetic symmetry, always being defined in P1 symmetry. So su.Atoms == su.Structure.
```python
print(su.parent.info()) # Parent structure
su.P # superstructure matrix
su.superhkl2parent([h, k, l]) # index superstructure hkl with parent cell
su.parenthkl2super([h, k, l]) # index parent hkl with supercell
```
### Multi-phase
Scattering from different crystal structures can be compared using the MultiCrystal class:
```python
xtls = xtl1 + xtl2
xtls.simulate_powder()
```
### Properties
The Crystal class contains a lot of atomic properties that can be exposed in the Properties class:
```python
xtl.Properties.info()
```
Calculated properties include:
- Molecular weight
- Density
- Diamagnetic suscpetibility
- x-ray absorption coefficient, attenuation length, transmission and refractive index
- Molecular charge balance
- Molecular mass fraction
- Atomic orbitals
- Magnetic exchange paths (in progress...)
Properties are calulated using the atomic structure along with atomic data stored in the folder [Dans_Diffraction/data](data).
### Multiple Scattering
Simulations of multiple scattering at different azimuths for a particular energy can be simulated. Based on [code by Dr Gareth Nisbet](https://journals.iucr.org/a/issues/2015/01/00/td5022/).
[](https://doi.org/10.5281/zenodo.12866).
```python
azimuth, intensity = xtl.Scatter.ms_azimuth([h,k,l], energy_kev=8)
```

### Graphical Front End

Start a new GUI, then select a cif file:
```text
$ ipython -i -m Dans_Diffraction gui
```
Or start the GUI from within the interactive console:
```python
dif.start_gui()
```
Using an already generated crystal:
```python
xtl.start_gui()
```
### Diffractometer Simulator

New in version 3.0.0. Simulate a generic detector situated around the crystal sample, with the ability to
control detector location shape and size and lattice orientation.
### FDMNES functionality
FDMNES is a powerful tool for simulating resonant x-ray diffraction, created by [Y. Joly and O. Bunau.](http://neel.cnrs.fr/spip.php?rubrique1007&lang=en)
The Dans_Diffraction FDMNES class allows for the automatic creation of input files and simple analysis of results.
The following command should be used to activate these features (only needs to be issued once).
```python
dif.activate_fdmnes()
```
Once activated, the FDMNES classes become available.
```python
fdm = dif.Fdmnes(xtl) # Create input files and run FDMNES
fdma = dif.FdmnesAnalysis(output_path, output_name) # Load output files and plot results
```
See class documentation for more information.
Once activated, FDMNES GUI elements become available from the main window, emulating functionality of the classes.

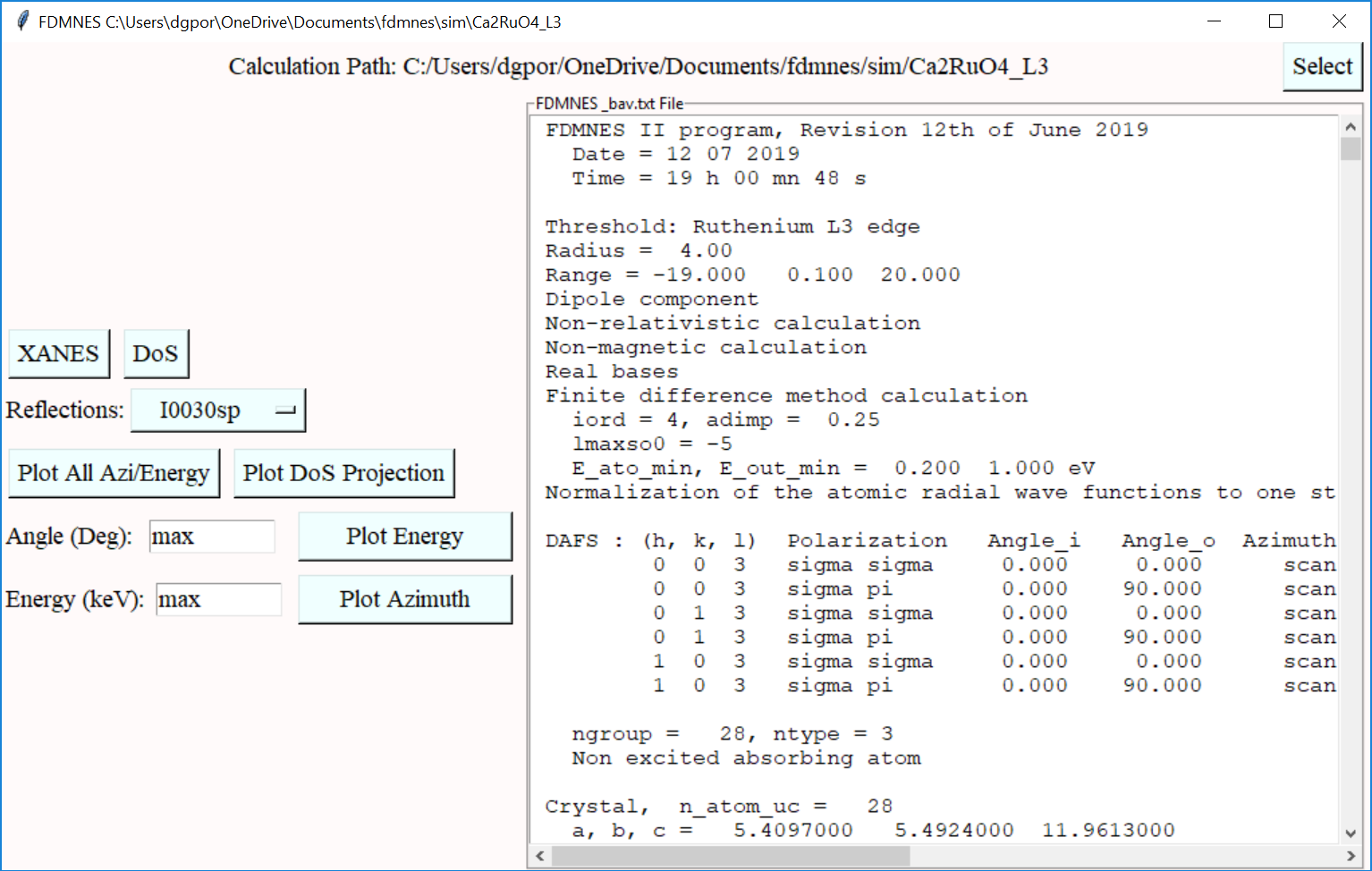
# Acknoledgements
| Date | Thanks to... |
|------------|-----------------------------------------------------------------------------------------|
| 2018 | Thanks to Hepesu for help with Python3 support and ideas about breaking up calculations |
| Dec 2019 | Thanks to Gareth Nisbet for allowing me to inlude his multiple scattering siumulation |
| April 2020 | Thanks to ChunHai Wang for helpful suggestions in readcif! |
| May 2020 | Thanks to AndreEbel for helpful suggestions on citations |
| Dec 2020 | Thanks to Chris Drozdowski for suggestions about reflection families |
| Jan 2021 | Thanks to aslarsen for suggestions about outputting the structure factor |
| April 2021 | Thanks to Trygve Ræder for suggestions about x-ray scattering factors |
| Feb 2022 | Thanks to Mirko for pointing out the error in two-theta values in Scatter.powder |
| March 2022 | Thanks to yevgenyr for suggesting new peak profiles in Scatter.powder |
| Jan 2023 | Thanks to Anuradha Vibhakar for pointing out the error in f0 + if'-if'' |
| Jan 2023 | Thanks to Andreas Rosnes for testing the installation in jupyterlab |
| May 2023 | Thanks to Carmelo Prestipino for adding electron scattering factors |
| June 2023 | Thanks to Sergio I. Rincon for pointing out the rounding error in Scatter.powder |
Copyright
-----------------------------------------------------------------------------
Copyright 2023 Diamond Light Source Ltd.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
Files in this package covered by this licence:
* classes_crystal.py
* classes_scattering.py
* classes_plotting.py
* classes_properties.py
* classes_multicrystal.py
* classes_orientation.py
* classes_orbitals.py
* functions_general.py
* functions_plotting.py
* functions_scattering.py
* functions_crystallography.py
* tkgui/*.py
Other files are either covered by their own licence or not licenced for other use.
| Dr Daniel G Porter | [dan.porter@diamond.ac.uk](mailto:dan.porter@diamond.ac.uk) |
| ---- | ---- |
| [www.diamond.ac.uk](www.diamond.ac.uk) | Diamond Light Source, Chilton, Didcot, Oxon, OX11 0DE, U.K. |
Raw data
{
"_id": null,
"home_page": "https://github.com/DanPorter/Dans_Diffraction",
"name": "Dans-Diffraction",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "crystal,cif,diffraction,crystallography,science,x-ray,neutron,resonant,magnetic,magnetism,multiple scattering,fdmnes,super structure,spacegroup,space group,diffractometer",
"author": "Dan Porter",
"author_email": "d.g.porter@outlook.com",
"download_url": "https://files.pythonhosted.org/packages/53/cc/2862f720cb241197f538cd26ebb2a75e57eda6bd1af4cb316f9692bcd6b3/Dans_Diffraction-3.0.0.tar.gz",
"platform": null,
"description": "# Dans_Diffaction\nReads crystallographic cif files, calculates crystal properties and simulates diffraction.\n\n**Version 3.0**\n\n[](https://doi.org/10.5281/zenodo.7725644)\n[](https://mybinder.org/v2/gh/DanPorter/Dans_Diffraction/6be78ef800167276d61d3e73da3b74a8367dbbe7?urlpath=lab%2Ftree%2FDans_Diffraction.ipynb) \n[](https://github.com/DanPorter/Dans_Diffraction)\n\n\nBy Dan Porter, Diamond Light Source\n2023\n\n#### TL;DR:\n```text\n$ ipython -i -m Dans_Diffraction\nOR\n$ ipython -m Dans_Diffraction gui\n```\n\n```python\n\"\"\"Python Sctipt\"\"\"\nimport Dans_Diffraction as dif\nxtl = dif.Crystal('some_file.cif')\nprint(xtl) # print Crystal structure parameters\n\n# Print reflection list:\nprint(xtl.Scatter.print_all_reflections(energy_kev=5)) \n\n# Plot Powder pattern:\nxtl.Plot.simulate_powder(energy_kev=8)\nplt.show()\n\n# Start graphical user interface:\nxtl.start_gui()\n```\n\nFull code documentation available [here](https://danporter.github.io/Dans_Diffraction/).\n\nTry it out on [mybinder!](https://mybinder.org/v2/gh/DanPorter/Dans_Diffraction/6be78ef800167276d61d3e73da3b74a8367dbbe7?urlpath=lab%2Ftree%2FDans_Diffraction.ipynb)\n\nFor comments, queries or bugs - email [dan.porter@diamond.ac.uk](mailto:dan.porter@diamond.ac.uk)\n\n**Citation:** If you use this code (great!), please cite the published DOI: [10.5281/zenodo.3859501](https://doi.org/10.5281/zenodo.3859501)\n\n# Installation\n**Requirements:** \nPython 3+ with packages: *Numpy*, *Matplotlib*, *Tkinter*.\nBuiltIn packages used: *sys*, *os*, *re*, *glob*, *warnings*, *json*, *itertools*\n\nInstall stable version from PyPI:\n```text\n$ python -m pip install Dans-Diffraction\n```\n\nOr, install the latest version direct from GitHub:\n```text\n$ python -m pip install git+https://github.com/DanPorter/Dans_Diffraction.git\n```\n\nOr, Download the latest version from GitHub (with examples!):\n```text\n$ git clone https://github.com/DanPorter/Dans_Diffraction.git\n```\n\n\n\n# Operation\nDans_Diffraction is best run within an interactive python environment:\n```text\n$ ipython -i -m Dans_Diffraction\n```\n\nDans_Diffraction can also be run in scripts as an import, example scripts are provided in the [Examples](https://github.com/DanPorter/Dans_Diffraction/blob/master/Examples) folder.\n### Read CIF file\n```python\nimport Dans_Diffraction as dif\nxtl = dif.Crystal('some_file.cif')\nxtl.info() # print Crystal structure parameters\nhelp(xtl) # all functions (nearly!) are documented\n```\n\n### Alter atomic positions\n```python\nxtl.Cell.latt([2.85,2.85,10.8,90,90,120]) # set lattice parameters\nxtl.Atoms.info() # Print Symmetric positions\nxtl.Structure.info() # Print All positions in P1 symmetry (same structure and functions as xtl.Atoms)\n# Symmetric positions\nxtl.Atoms.changeatom(idx=0, u=0, v=0, w=0, type='Co', label='Co1')\nxtl.Atoms.addatom(idx=0, u=0, v=0, w=0, type='Co', label='Co1')\n# After adding or changing an atom in the Atoms class, re-generate the full structure using symmetry arguments:\nxtl.generate_lattice()\n# Full atomic structure in P1 symmetry\nxtl.Structure.changeatom(idx=0, u=0, v=0, w=0, type='Co', label='Co1')\nxtl.Structure.addatom(idx=0, u=0, v=0, w=0, type='Co', label='Co1')\n# Plot crystal Structure\nxtl.Plot.plot_crystal() # 3D plot\nxtl.Plot.plot_layers() # 2D plot for layered materials\n```\n\n\n\n### Alter crystal symmetry\n```python\nxtl.Symmetry.info() # print symmetry arguments\nxtl.Symmetry.addsym('x,y,z+1/2') # adds single symmetry operation\nxtl.Symmetry.changesym(0, 'x,y,z+1/4')\nxtl.Symmetry.load_spacegroup(194) # replaces current symmetry operations\n# After adding or changing symmetry operations, regengerate the symmetry matrices\nxtl.Symmetry.generate_matrices()\n```\n\n### Save structure as CIF\nLattice parameters, crystal structure and symmetry operations will be saved to the CIF.\nIf magnetic moments are defined, magnetic symmetry operations and moments will also be saved\nand format changed to \"*.mcif\".\n```python\nxtl.write_cif('edited file.cif')\n```\n\n### Calculate Structure Factors\nX-ray or neutron structure factors/ intensities are calculated based on the full unit cell structure, including atomic \nform-factors (x-rays) or coherent scattering lengths (neutrons).\n```python\n# Choose scattering options (see help(xtl.Scatter.setup_scatter))\nxtl.Scatter.setup_scatter(type='x-ray', energy_keV=8.0)\n# Allowed radiation types:\n# 'xray','neutron','xray magnetic','neutron magnetic','xray resonant'\nxtl.Scatter.print_all_refelctions() # Returns formated string of all allowed reflections\ninten = xtl.Scatter.intensity([h,k,l]) # Returns intensity\ntwotheta, iten, reflections = xtl.Scatter.powder(units='twotheta')\n# Plot Experimental Intensities\nxtl.Plot.simulate_powder() # Powder pattern\nxtl.Plot.simulate_hk0() # Reciprocal space plane\n```\n\n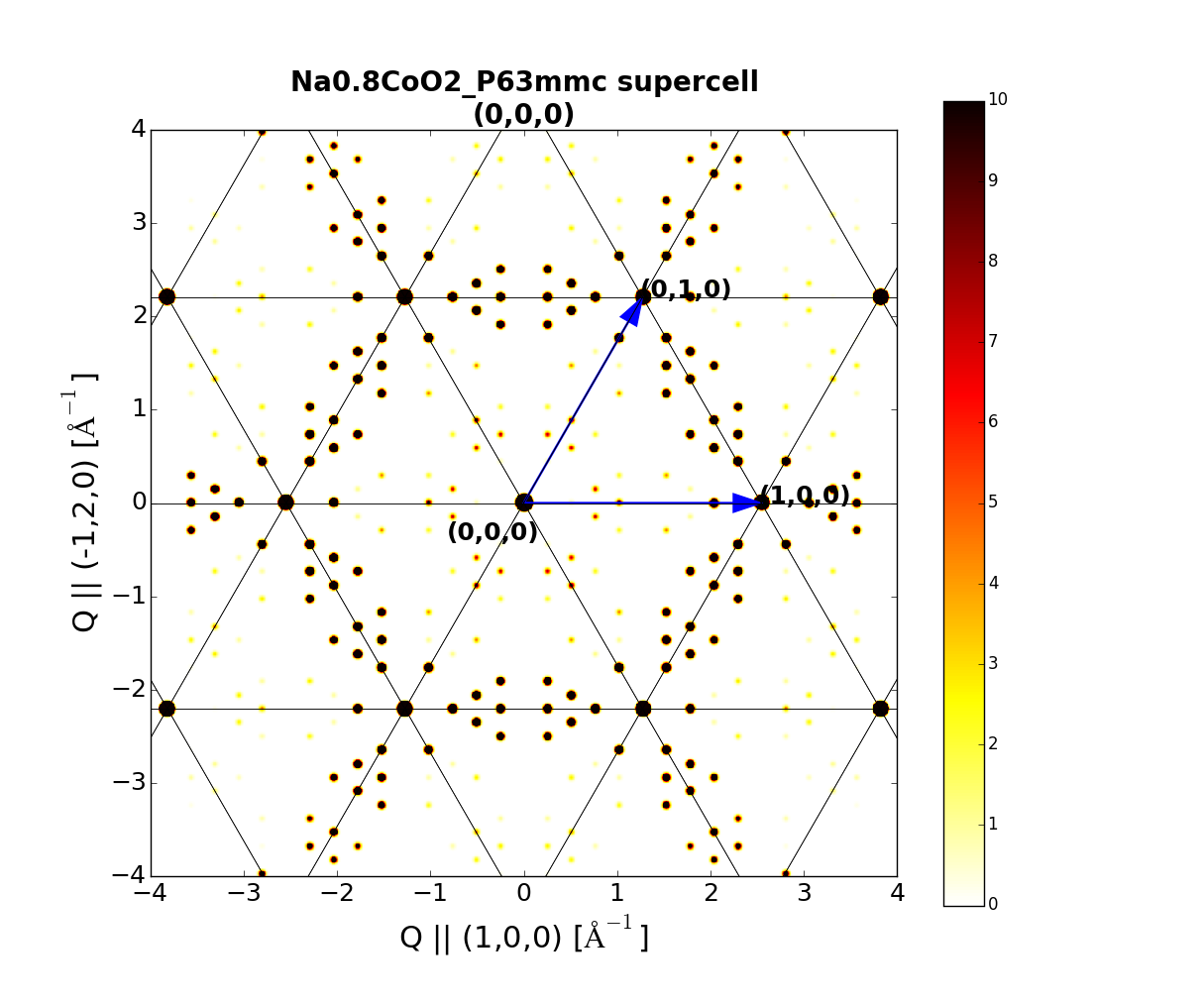\n\n\n### Magnetic Structrues\n*Magnetic structures and scattering are currently in development and shouldn't be treated as accurate!*\n\nSimple magnetic structures can be loaded from magnetic cif (*.mcif) files. Magnetic moments are stored for each atomic \nposition as a vector. The crystal object has a seperate set of magnetic symmetry operations. Symmetry operations from the \ntables of magnetic spacegroups can also be loaded. Only simple magnetic structures are allowed. There must be the same\nnumber of magnetic symmetry operations as crystal symmetry operations and atomic positions can only have single moments\nassigned.\n```python\nxtl = dif.Crystal('some_file.mcif')\nxtl.Atoms.mxmymz() # return magnetic moment vectors on each ion\nxtl.Symmetry.symmetry_operations_magnetic # magnetic symmetry operations (list of strings)\nxtl.Symmetry.print_magnetic_spacegroups() # return str of available magnetic spacegroups, given crystal's spacegroup\nxtl.Symmetry.load_magnetic_spacegroup(mag_spg_number) # loads mag. operations given mag. spacegroup number\n```\nMagnetic scattering is also available for neutrons and x-rays (both resonant and non-resonant), using the appropriate magnetic form-factors.\n```python\nImag = xtl.Scatter.magnetic_neutron(HKL=[0,0,3])\nIres = xtl.Scatter.xray_resonant_magnetic(HKL=[0,0,3], energy_kev=2.838, azim_zero=[1, 0, 0], psi=0, polarisation='s-p', F0=0, F1=1, F2=0)\n```\n\n### Superstructures\nSuperstructures can be built using the Superstructure class, requring only a matrix to define the new phase:\n```python\nsu = xtl.generate_superstructure([[2,0,0],[0,2,0],[0,0,1]])\n```\n\nSuperstucture classes behave like Crystal classes, but have an additional 'Parent' property that references the original \ncrystal structure and additional behaviours partiular to superstructures. Superstructures loose their parent crystal and\nmagnetic symmetry, always being defined in P1 symmetry. So su.Atoms == su.Structure.\n\n```python\nprint(su.parent.info()) # Parent structure\nsu.P # superstructure matrix \nsu.superhkl2parent([h, k, l]) # index superstructure hkl with parent cell\nsu.parenthkl2super([h, k, l]) # index parent hkl with supercell\n```\n\n### Multi-phase\nScattering from different crystal structures can be compared using the MultiCrystal class:\n```python\nxtls = xtl1 + xtl2\nxtls.simulate_powder()\n```\n\n\n### Properties\nThe Crystal class contains a lot of atomic properties that can be exposed in the Properties class:\n```python\nxtl.Properties.info()\n```\n\nCalculated properties include:\n - Molecular weight\n - Density\n - Diamagnetic suscpetibility \n - x-ray absorption coefficient, attenuation length, transmission and refractive index\n - Molecular charge balance\n - Molecular mass fraction\n - Atomic orbitals\n - Magnetic exchange paths (in progress...)\n\nProperties are calulated using the atomic structure along with atomic data stored in the folder [Dans_Diffraction/data](data).\n\n\n### Multiple Scattering\nSimulations of multiple scattering at different azimuths for a particular energy can be simulated. Based on [code by Dr Gareth Nisbet](https://journals.iucr.org/a/issues/2015/01/00/td5022/).\n [](https://doi.org/10.5281/zenodo.12866).\n\n```python\nazimuth, intensity = xtl.Scatter.ms_azimuth([h,k,l], energy_kev=8)\n```\n\n\n\n\n### Graphical Front End\n\n\nStart a new GUI, then select a cif file:\n```text\n$ ipython -i -m Dans_Diffraction gui\n```\nOr start the GUI from within the interactive console:\n```python\ndif.start_gui()\n```\nUsing an already generated crystal:\n```python\nxtl.start_gui()\n```\n\n### Diffractometer Simulator\n\n\nNew in version 3.0.0. Simulate a generic detector situated around the crystal sample, with the ability to \ncontrol detector location shape and size and lattice orientation.\n\n\n### FDMNES functionality\nFDMNES is a powerful tool for simulating resonant x-ray diffraction, created by [Y. Joly and O. Bunau.](http://neel.cnrs.fr/spip.php?rubrique1007&lang=en)\n\nThe Dans_Diffraction FDMNES class allows for the automatic creation of input files and simple analysis of results.\nThe following command should be used to activate these features (only needs to be issued once). \n```python\ndif.activate_fdmnes()\n```\nOnce activated, the FDMNES classes become available.\n```python\nfdm = dif.Fdmnes(xtl) # Create input files and run FDMNES\nfdma = dif.FdmnesAnalysis(output_path, output_name) # Load output files and plot results\n```\nSee class documentation for more information.\n\n\nOnce activated, FDMNES GUI elements become available from the main window, emulating functionality of the classes.\n\n\n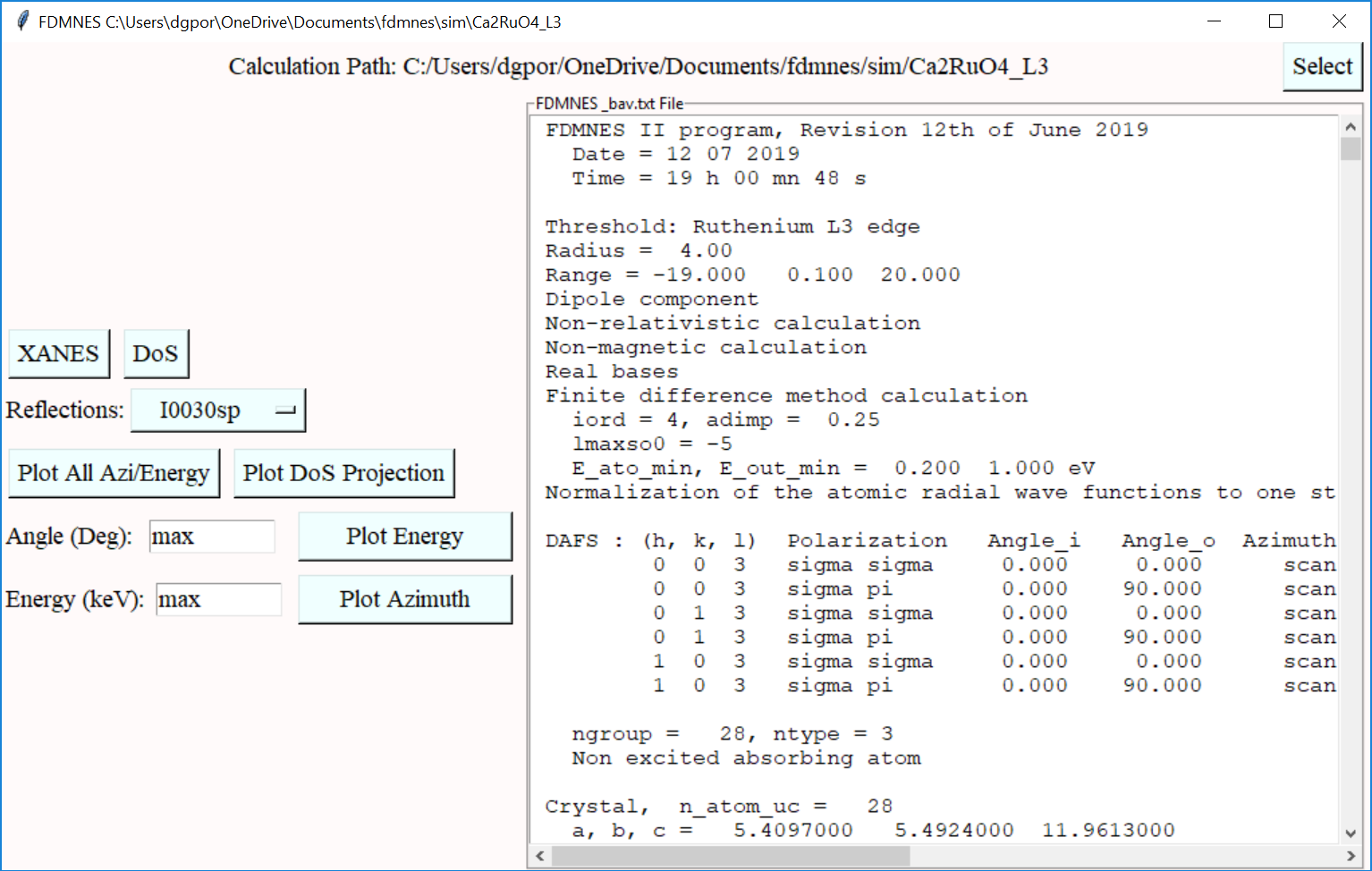\n\n\n# Acknoledgements\n| Date | Thanks to... |\n|------------|-----------------------------------------------------------------------------------------|\n| 2018 | Thanks to Hepesu for help with Python3 support and ideas about breaking up calculations |\n| Dec 2019 | Thanks to Gareth Nisbet for allowing me to inlude his multiple scattering siumulation |\n| April 2020 | Thanks to ChunHai Wang for helpful suggestions in readcif! |\n| May 2020 | Thanks to AndreEbel for helpful suggestions on citations |\n| Dec 2020 | Thanks to Chris Drozdowski for suggestions about reflection families |\n| Jan 2021 | Thanks to aslarsen for suggestions about outputting the structure factor |\n| April 2021 | Thanks to Trygve R\u00c3\u00a6der for suggestions about x-ray scattering factors |\n| Feb 2022 | Thanks to Mirko for pointing out the error in two-theta values in Scatter.powder |\n| March 2022 | Thanks to yevgenyr for suggesting new peak profiles in Scatter.powder |\n| Jan 2023 | Thanks to Anuradha Vibhakar for pointing out the error in f0 + if'-if'' |\n| Jan 2023 | Thanks to Andreas Rosnes for testing the installation in jupyterlab |\n| May 2023 | Thanks to Carmelo Prestipino for adding electron scattering factors |\n| June 2023 | Thanks to Sergio I. Rincon for pointing out the rounding error in Scatter.powder |\n\n\nCopyright\n-----------------------------------------------------------------------------\n Copyright 2023 Diamond Light Source Ltd.\n\n Licensed under the Apache License, Version 2.0 (the \"License\");\n you may not use this file except in compliance with the License.\n You may obtain a copy of the License at\n\n http://www.apache.org/licenses/LICENSE-2.0\n\n Unless required by applicable law or agreed to in writing, software\n distributed under the License is distributed on an \"AS IS\" BASIS,\n WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.\n See the License for the specific language governing permissions and\n limitations under the License.\n\nFiles in this package covered by this licence:\n* classes_crystal.py\n* classes_scattering.py\n* classes_plotting.py\n* classes_properties.py\n* classes_multicrystal.py\n* classes_orientation.py\n* classes_orbitals.py\n* functions_general.py\n* functions_plotting.py\n* functions_scattering.py\n* functions_crystallography.py\n* tkgui/*.py\n\nOther files are either covered by their own licence or not licenced for other use.\n\n| Dr Daniel G Porter | [dan.porter@diamond.ac.uk](mailto:dan.porter@diamond.ac.uk) |\n| ---- | ---- |\n| [www.diamond.ac.uk](www.diamond.ac.uk) | Diamond Light Source, Chilton, Didcot, Oxon, OX11 0DE, U.K. |\n\n\n",
"bugtrack_url": null,
"license": "",
"summary": "Generate diffracted intensities from crystals",
"version": "3.0.0",
"project_urls": {
"Homepage": "https://github.com/DanPorter/Dans_Diffraction"
},
"split_keywords": [
"crystal",
"cif",
"diffraction",
"crystallography",
"science",
"x-ray",
"neutron",
"resonant",
"magnetic",
"magnetism",
"multiple scattering",
"fdmnes",
"super structure",
"spacegroup",
"space group",
"diffractometer"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "9b4cd24f465ca24b62f9f48c83b8916bcb993eae69fd95b85decc39009b84c11",
"md5": "c346a036f2f1da84d37d41e01c462946",
"sha256": "109ea4dff328df538cf05adebcaf457fbb75f63e0b14d45ab60cf740c4579a4f"
},
"downloads": -1,
"filename": "Dans_Diffraction-3.0.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "c346a036f2f1da84d37d41e01c462946",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 3650039,
"upload_time": "2023-07-02T16:48:10",
"upload_time_iso_8601": "2023-07-02T16:48:10.732589Z",
"url": "https://files.pythonhosted.org/packages/9b/4c/d24f465ca24b62f9f48c83b8916bcb993eae69fd95b85decc39009b84c11/Dans_Diffraction-3.0.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "53cc2862f720cb241197f538cd26ebb2a75e57eda6bd1af4cb316f9692bcd6b3",
"md5": "c3bf64cc00e7e602321af83ae35ce2a7",
"sha256": "0916670f6c8ca2c8603f35e05f01a9840a5c0713416e1fa8480aae12be005fee"
},
"downloads": -1,
"filename": "Dans_Diffraction-3.0.0.tar.gz",
"has_sig": false,
"md5_digest": "c3bf64cc00e7e602321af83ae35ce2a7",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 3576126,
"upload_time": "2023-07-02T16:48:13",
"upload_time_iso_8601": "2023-07-02T16:48:13.601392Z",
"url": "https://files.pythonhosted.org/packages/53/cc/2862f720cb241197f538cd26ebb2a75e57eda6bd1af4cb316f9692bcd6b3/Dans_Diffraction-3.0.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-07-02 16:48:13",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "DanPorter",
"github_project": "Dans_Diffraction",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [
{
"name": "numpy",
"specs": []
},
{
"name": "matplotlib",
"specs": []
},
{
"name": "setuptools",
"specs": []
}
],
"lcname": "dans-diffraction"
}