<img alt="DecayLanguage logo" src="https://raw.githubusercontent.com/scikit-hep/decaylanguage/master/images/DecayLanguage.png"/>
# DecayLanguage: describe, manipulate and convert particle decays
[](https://scikit-hep.org/)
[](https://pypi.python.org/pypi/decaylanguage)
[](https://github.com/conda-forge/decaylanguage-feedstock)
[](https://pypi.python.org/pypi/decaylanguage)
[](https://doi.org/10.5281/zenodo.3257423)
[](https://github.com/scikit-hep/decaylanguage/actions)
[](https://codecov.io/gh/scikit-hep/decaylanguage?branch=master)
[](https://decaylanguage.readthedocs.io)
[](https://mybinder.org/v2/gh/scikit-hep/decaylanguage/master?urlpath=lab/tree/notebooks/DecayLanguageDemo.ipynb)
DecayLanguage implements a language to describe and convert particle decays
between digital representations, effectively making it possible to interoperate
several fitting programs. Particular interest is given to programs dedicated
to amplitude analyses.
DecayLanguage provides tools to parse so-called .dec decay files,
and describe, manipulate and visualize decay chains.
## Installation
Just run the following:
```bash
pip install decaylanguage
```
You can use a virtual environment through pipenv or with `--user` if you know
what those are. [Python
3.8+](http://docs.python-guide.org/en/latest/starting/installation) supported
(see version 0.12 for Python 2 & 3.5 support, 0.14 for Python 3.6 support, 0.18 for Python 3.7 support).
<details><summary>Dependencies: (click to expand)</summary><p>
Required and compatibility dependencies will be automatically installed by pip.
### Required dependencies:
- [particle](https://github.com/scikit-hep/particle): PDG particle data and identification codes
- [NumPy](https://scipy.org/install.html): The numerical library for Python
- [pandas](https://pandas.pydata.org/): Tabular data in Python
- [attrs](https://github.com/python-attrs/attrs): DataClasses for Python
- [plumbum](https://github.com/tomerfiliba/plumbum): Command line tools
- [lark](https://github.com/lark-parser/lark): A modern parsing library for Python
- [graphviz](https://gitlab.com/graphviz/graphviz/) to render (DOT language) graph
descriptions and visualizations of decay chains.
### Python compatibility:
- [importlib_resources](http://importlib-resources.readthedocs.io/en/latest/) backport if using Python /< 3.9
</p></details>
## Getting started
The [Binder demo](https://mybinder.org/v2/gh/scikit-hep/decaylanguage/master?urlpath=lab/tree/notebooks/DecayLanguageDemo.ipynb)
is an excellent way to get started with `DecayLanguage`.
This is a quick user guide. For a full API docs, go [here](https://decaylanguage.readthedocs.io)
(note that it is presently work-in-progress).
### What is DecayLanguage?
`DecayLanguage` is a set of tools for building and transforming particle
decays:
1. It provides tools to parse so-called `.dec` decay files,
and describe, manipulate and visualize the resulting decay chains.
2. It implements a language to describe and convert particle decays
between digital representations, effectively making it possible to interoperate
several fitting programs. Particular interest is given to programs dedicated
to amplitude analyses.
### Particles
Particles are a key component when dealing with decays.
Refer to the [particle package](https://github.com/scikit-hep/particle)
for how to deal with particles and Monte Carlo particle identification codes.
### Parse decay files
Decay `.dec` files can be parsed simply with
```python
from decaylanguage import DecFileParser
parser = DecFileParser('my-decay-file.dec')
parser.parse()
# Inspect what decays are defined
parser.list_decay_mother_names()
# Print decay modes, etc. ...
```
A copy of the master DECAY.DEC file used by the LHCb experiment is provided
[here](https://github.com/scikit-hep/decaylanguage/tree/master/src/decaylanguage/data)
for convenience.
The `DecFileParser` class implements a series of methods giving access to all
information stored in decay files: the decays themselves, particle name aliases,
definitions of charge-conjugate particles, variable and Pythia-specific
definitions, etc.
It can be handy to parse from a multi-line string rather than a file:
```python
s = """Decay pi0
0.988228297 gamma gamma PHSP;
0.011738247 e+ e- gamma PI0_DALITZ;
0.000033392 e+ e+ e- e- PHSP;
0.000000065 e+ e- PHSP;
Enddecay
"""
dfp = DecFileParser.from_string(s)
dfp.parse()
```
#### Advanced usage
The list of `.dec` file decay models known to the package can be inspected via
```python
from decaylanguage.dec import known_decay_models
```
Say you have to deal with a decay file containing a new model not yet on the list above.
Running the parser as usual will result in an error in the model parsing.
It is nevertheless easy to deal with this issue; no need to wait for a new release:
Just call `load_additional_decay_models` with the models you'd like to add as arguments
before parsing the file:
```python
dfp = DecFileParser('my_decfile.dec')
dfp.load_additional_decay_models("MyModel1", "MyModel2")
dfp.parse()
...
```
This being said, please do submit a pull request to add new models,
if you spot missing ones ...
### Visualize decay files
The class `DecayChainViewer` allows the visualization of parsed decay chains:
```python
from decaylanguage import DecayChainViewer
# Build the (dictionary-like) D*+ decay chain representation setting the
# D+ and D0 mesons to stable, to avoid too cluttered an image
d = dfp.build_decay_chains('D*+', stable_particles=('D+', 'D0'))
DecayChainViewer(d) # works in a notebook
```
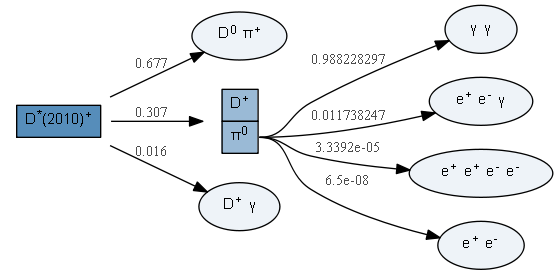
The actual graph is available as
```python
# ...
dcv = DecayChainViewer(d)
dcv.graph
```
making all `graphviz.Digraph` class properties and methods available, such as
```python
dcv.graph.render(filename='mygraph', format='pdf', view=True, cleanup=True)
```
In the same way, all `graphviz.Digraph` class attributes are settable
upon instantiation of `DecayChainViewer`:
```python
dcv = DecayChainViewer(chain, name='TEST', format='pdf')
```
### Universal representation of decay chains
A series of classes and methods have been designed to provide universal representations
of particle decay chains of any complexity, and to provide the ability
to convert between these representations.
Specifically, class- and dictionary-based representations have been implemented.
An example of a class-based representation of a decay chain is the following:
```python
>>> from decaylanguage import DaughtersDict, DecayMode, DecayChain
>>>
>>> dm1 = DecayMode(0.0124, 'K_S0 pi0', model='PHSP')
>>> dm2 = DecayMode(0.692, 'pi+ pi-')
>>> dm3 = DecayMode(0.98823, 'gamma gamma')
>>> dc = DecayChain('D0', {'D0':dm1, 'K_S0':dm2, 'pi0':dm3})
>>> dc
<DecayChain: D0 -> K_S0 pi0 (2 sub-decays), BF=0.0124>
```
Decay chains can be visualised with the `DecayChainViewer` class making use
of the dictionary representation `dc.to_dict()`, which is the simple
representation understood by `DecayChainViewer`, as see above:
```python
DecayChainViewer(dc.to_dict())
```
The fact that 2 representations of particle decay chains are provided ensures
the following:
1. Human-readable (class) and computer-efficient (dictionary) alternatives.
2. Flexibility for parsing, manipulation and storage of decay chain information.
### Decay modeling
The most common way to create a decay chain is to read in an [AmpGen]
style syntax from a file or a string. You can use:
```python
from decaylanguage.modeling import AmplitudeChain
lines, parameters, constants, states = AmplitudeChain.read_ampgen(text='''
EventType D0 K- pi+ pi+ pi-
D0[D]{K*(892)bar0{K-,pi+},rho(770)0{pi+,pi-}} 0 1 0.1 0 1 0.1
K(1460)bar-_mass 0 1460 1
K(1460)bar-_width 0 250 1
a(1)(1260)+::Spline::Min 0.18412
a(1)(1260)+::Spline::Max 1.86869
a(1)(1260)+::Spline::N 34
''')
```
Here, `lines` will be a list of AmplitudeChain lines (pretty print supported in Jupyter notebooks),
`parameters` will be a table of parameters (ranged parameters not yet supported),
`constants` will be a table of constants,
and `states` will be the list of known states (EventType).
#### Converters
You can output to a format (currently only [GooFit] supported, feel free
to make a PR to add more). Use a subclass of DecayChain, in this case,
GooFitChain. To use the [GooFit] output, type from the shell:
```bash
python -m decaylanguage -G goofit myinput.opts
```
## Contributors
We hereby acknowledge the contributors that made this project possible ([emoji key](https://allcontributors.org/docs/en/emoji-key)):
<!-- ALL-CONTRIBUTORS-LIST:START - Do not remove or modify this section -->
<!-- prettier-ignore-start -->
<!-- markdownlint-disable -->
<table>
<tbody>
<tr>
<td align="center" valign="top" width="14.28%"><a href="http://cern.ch/eduardo.rodrigues"><img src="https://avatars.githubusercontent.com/u/5013581?v=4?s=100" width="100px;" alt="Eduardo Rodrigues"/><br /><sub><b>Eduardo Rodrigues</b></sub></a><br /><a href="#maintenance-eduardo-rodrigues" title="Maintenance">🚧</a> <a href="https://github.com/scikit-hep/decaylanguage/commits?author=eduardo-rodrigues" title="Code">💻</a> <a href="https://github.com/scikit-hep/decaylanguage/commits?author=eduardo-rodrigues" title="Documentation">📖</a></td>
<td align="center" valign="top" width="14.28%"><a href="http://iscinumpy.dev"><img src="https://avatars.githubusercontent.com/u/4616906?v=4?s=100" width="100px;" alt="Henry Schreiner"/><br /><sub><b>Henry Schreiner</b></sub></a><br /><a href="#maintenance-henryiii" title="Maintenance">🚧</a> <a href="https://github.com/scikit-hep/decaylanguage/commits?author=henryiii" title="Code">💻</a> <a href="https://github.com/scikit-hep/decaylanguage/commits?author=henryiii" title="Documentation">📖</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/yipengsun"><img src="https://avatars.githubusercontent.com/u/33738176?v=4?s=100" width="100px;" alt="Yipeng Sun"/><br /><sub><b>Yipeng Sun</b></sub></a><br /><a href="https://github.com/scikit-hep/decaylanguage/commits?author=yipengsun" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/chrisburr"><img src="https://avatars.githubusercontent.com/u/5220533?v=4?s=100" width="100px;" alt="Chris Burr"/><br /><sub><b>Chris Burr</b></sub></a><br /><a href="https://github.com/scikit-hep/decaylanguage/commits?author=chrisburr" title="Documentation">📖</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://www.lieret.net"><img src="https://avatars.githubusercontent.com/u/13602468?v=4?s=100" width="100px;" alt="Kilian Lieret"/><br /><sub><b>Kilian Lieret</b></sub></a><br /><a href="https://github.com/scikit-hep/decaylanguage/commits?author=klieret" title="Documentation">📖</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/sognetic"><img src="https://avatars.githubusercontent.com/u/10749132?v=4?s=100" width="100px;" alt="Moritz Bauer"/><br /><sub><b>Moritz Bauer</b></sub></a><br /><a href="https://github.com/scikit-hep/decaylanguage/commits?author=sognetic" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/FlorianReiss"><img src="https://avatars.githubusercontent.com/u/44642966?v=4?s=100" width="100px;" alt="FlorianReiss"/><br /><sub><b>FlorianReiss</b></sub></a><br /><a href="https://github.com/scikit-hep/decaylanguage/commits?author=FlorianReiss" title="Code">💻</a> <a href="https://github.com/scikit-hep/decaylanguage/commits?author=FlorianReiss" title="Documentation">📖</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://gitlab.cern.ch/users/admorris"><img src="https://avatars.githubusercontent.com/u/15155249?v=4?s=100" width="100px;" alt="Adam Morris"/><br /><sub><b>Adam Morris</b></sub></a><br /><a href="https://github.com/scikit-hep/decaylanguage/commits?author=admorris" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/ch2ohch2oh"><img src="https://avatars.githubusercontent.com/u/7986711?v=4?s=100" width="100px;" alt="Dazhi Wang"/><br /><sub><b>Dazhi Wang</b></sub></a><br /><a href="https://github.com/scikit-hep/decaylanguage/commits?author=ch2ohch2oh" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/manuelfs"><img src="https://avatars.githubusercontent.com/u/4977423?v=4?s=100" width="100px;" alt="Manuel Franco Sevilla"/><br /><sub><b>Manuel Franco Sevilla</b></sub></a><br /><a href="https://github.com/scikit-hep/decaylanguage/commits?author=manuelfs" title="Code">💻</a></td>
</tr>
</tbody>
</table>
<!-- markdownlint-restore -->
<!-- prettier-ignore-end -->
<!-- ALL-CONTRIBUTORS-LIST:END -->
This project follows the [all-contributors](https://github.com/all-contributors/all-contributors) specification.
## Acknowledgements
The UK Science and Technology Facilities Council (STFC) and the University of Liverpool
provide funding for Eduardo Rodrigues (2020-) to work on this project part-time.
Support for this work was provided by the National Science Foundation
cooperative agreement OAC-1450377 (DIANA/HEP) in 2016-2019
and has been provided by OAC-1836650 (IRIS-HEP) since 2019.
Any opinions, findings, conclusions or recommendations expressed in this material
are those of the authors and do not necessarily reflect the views of the National Science Foundation.
[AmpGen]: https://gitlab.cern.ch/lhcb/Gauss/tree/LHCBGAUSS-1058.AmpGenDev/Gen/AmpGen
[GooFit]: https://GooFit.github.io
Raw data
{
"_id": null,
"home_page": null,
"name": "decaylanguage",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "Scikit-HEP <scikit-hep-admins@googlegroups.com>",
"keywords": "HEP, chain, decay, particle, representation",
"author": null,
"author_email": "Eduardo Rodrigues <eduardo.rodrigues@cern.ch>, Henry Schreiner <henryfs@princeton.edu>",
"download_url": "https://files.pythonhosted.org/packages/7c/93/42bff92bca1fc3a48ebb516a22c90aab3c711d0e91c19ab90ab34a582a6b/decaylanguage-0.18.5.tar.gz",
"platform": null,
"description": "<img alt=\"DecayLanguage logo\" src=\"https://raw.githubusercontent.com/scikit-hep/decaylanguage/master/images/DecayLanguage.png\"/>\n\n# DecayLanguage: describe, manipulate and convert particle decays\n\n[](https://scikit-hep.org/)\n[](https://pypi.python.org/pypi/decaylanguage)\n[](https://github.com/conda-forge/decaylanguage-feedstock)\n[](https://pypi.python.org/pypi/decaylanguage)\n[](https://doi.org/10.5281/zenodo.3257423)\n\n[](https://github.com/scikit-hep/decaylanguage/actions)\n[](https://codecov.io/gh/scikit-hep/decaylanguage?branch=master)\n[](https://decaylanguage.readthedocs.io)\n\n[](https://mybinder.org/v2/gh/scikit-hep/decaylanguage/master?urlpath=lab/tree/notebooks/DecayLanguageDemo.ipynb)\n\n\nDecayLanguage implements a language to describe and convert particle decays\nbetween digital representations, effectively making it possible to interoperate\nseveral fitting programs. Particular interest is given to programs dedicated\nto amplitude analyses.\n\nDecayLanguage provides tools to parse so-called .dec decay files,\nand describe, manipulate and visualize decay chains.\n\n\n## Installation\n\nJust run the following:\n\n```bash\npip install decaylanguage\n```\n\nYou can use a virtual environment through pipenv or with `--user` if you know\nwhat those are. [Python\n3.8+](http://docs.python-guide.org/en/latest/starting/installation) supported\n(see version 0.12 for Python 2 & 3.5 support, 0.14 for Python 3.6 support, 0.18 for Python 3.7 support).\n\n<details><summary>Dependencies: (click to expand)</summary><p>\n\nRequired and compatibility dependencies will be automatically installed by pip.\n\n### Required dependencies:\n\n- [particle](https://github.com/scikit-hep/particle): PDG particle data and identification codes\n- [NumPy](https://scipy.org/install.html): The numerical library for Python\n- [pandas](https://pandas.pydata.org/): Tabular data in Python\n- [attrs](https://github.com/python-attrs/attrs): DataClasses for Python\n- [plumbum](https://github.com/tomerfiliba/plumbum): Command line tools\n- [lark](https://github.com/lark-parser/lark): A modern parsing library for Python\n- [graphviz](https://gitlab.com/graphviz/graphviz/) to render (DOT language) graph\n descriptions and visualizations of decay chains.\n\n### Python compatibility:\n- [importlib_resources](http://importlib-resources.readthedocs.io/en/latest/) backport if using Python /< 3.9\n</p></details>\n\n\n## Getting started\n\nThe [Binder demo](https://mybinder.org/v2/gh/scikit-hep/decaylanguage/master?urlpath=lab/tree/notebooks/DecayLanguageDemo.ipynb)\nis an excellent way to get started with `DecayLanguage`.\n\nThis is a quick user guide. For a full API docs, go [here](https://decaylanguage.readthedocs.io)\n(note that it is presently work-in-progress).\n\n### What is DecayLanguage?\n\n`DecayLanguage` is a set of tools for building and transforming particle\ndecays:\n\n1. It provides tools to parse so-called `.dec` decay files,\nand describe, manipulate and visualize the resulting decay chains.\n\n2. It implements a language to describe and convert particle decays\nbetween digital representations, effectively making it possible to interoperate\nseveral fitting programs. Particular interest is given to programs dedicated\nto amplitude analyses.\n\n### Particles\n\nParticles are a key component when dealing with decays.\nRefer to the [particle package](https://github.com/scikit-hep/particle)\nfor how to deal with particles and Monte Carlo particle identification codes.\n\n### Parse decay files\n\nDecay `.dec` files can be parsed simply with\n\n```python\nfrom decaylanguage import DecFileParser\n\nparser = DecFileParser('my-decay-file.dec')\nparser.parse()\n\n# Inspect what decays are defined\nparser.list_decay_mother_names()\n\n# Print decay modes, etc. ...\n```\n\nA copy of the master DECAY.DEC file used by the LHCb experiment is provided\n[here](https://github.com/scikit-hep/decaylanguage/tree/master/src/decaylanguage/data)\nfor convenience.\n\nThe `DecFileParser` class implements a series of methods giving access to all\ninformation stored in decay files: the decays themselves, particle name aliases,\ndefinitions of charge-conjugate particles, variable and Pythia-specific\ndefinitions, etc.\n\nIt can be handy to parse from a multi-line string rather than a file:\n\n```python\ns = \"\"\"Decay pi0\n0.988228297 gamma gamma PHSP;\n0.011738247 e+ e- gamma PI0_DALITZ;\n0.000033392 e+ e+ e- e- PHSP;\n0.000000065 e+ e- PHSP;\nEnddecay\n\"\"\"\n\ndfp = DecFileParser.from_string(s)\ndfp.parse()\n```\n\n#### Advanced usage\n\nThe list of `.dec` file decay models known to the package can be inspected via\n\n```python\nfrom decaylanguage.dec import known_decay_models\n```\n\nSay you have to deal with a decay file containing a new model not yet on the list above.\nRunning the parser as usual will result in an error in the model parsing.\nIt is nevertheless easy to deal with this issue; no need to wait for a new release:\nJust call `load_additional_decay_models` with the models you'd like to add as arguments\nbefore parsing the file:\n\n```python\ndfp = DecFileParser('my_decfile.dec')\ndfp.load_additional_decay_models(\"MyModel1\", \"MyModel2\")\ndfp.parse()\n...\n```\n\nThis being said, please do submit a pull request to add new models,\nif you spot missing ones ...\n\n### Visualize decay files\n\nThe class `DecayChainViewer` allows the visualization of parsed decay chains:\n\n```python\nfrom decaylanguage import DecayChainViewer\n\n# Build the (dictionary-like) D*+ decay chain representation setting the\n# D+ and D0 mesons to stable, to avoid too cluttered an image\nd = dfp.build_decay_chains('D*+', stable_particles=('D+', 'D0'))\nDecayChainViewer(d) # works in a notebook\n```\n\n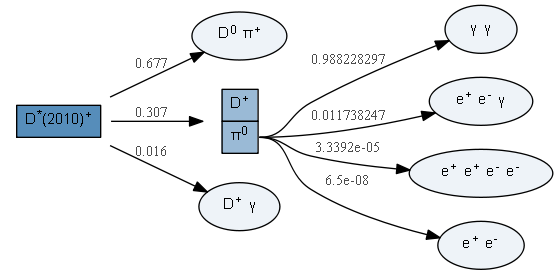\n\nThe actual graph is available as\n\n```python\n# ...\ndcv = DecayChainViewer(d)\ndcv.graph\n```\n\nmaking all `graphviz.Digraph` class properties and methods available, such as\n\n```python\ndcv.graph.render(filename='mygraph', format='pdf', view=True, cleanup=True)\n```\n\nIn the same way, all `graphviz.Digraph` class attributes are settable\nupon instantiation of `DecayChainViewer`:\n\n```python\ndcv = DecayChainViewer(chain, name='TEST', format='pdf')\n```\n\n### Universal representation of decay chains\n\nA series of classes and methods have been designed to provide universal representations\nof particle decay chains of any complexity, and to provide the ability\nto convert between these representations.\nSpecifically, class- and dictionary-based representations have been implemented.\n\nAn example of a class-based representation of a decay chain is the following:\n\n```python\n>>> from decaylanguage import DaughtersDict, DecayMode, DecayChain\n>>>\n>>> dm1 = DecayMode(0.0124, 'K_S0 pi0', model='PHSP')\n>>> dm2 = DecayMode(0.692, 'pi+ pi-')\n>>> dm3 = DecayMode(0.98823, 'gamma gamma')\n>>> dc = DecayChain('D0', {'D0':dm1, 'K_S0':dm2, 'pi0':dm3})\n>>> dc\n<DecayChain: D0 -> K_S0 pi0 (2 sub-decays), BF=0.0124>\n```\n\nDecay chains can be visualised with the `DecayChainViewer` class making use\nof the dictionary representation `dc.to_dict()`, which is the simple\nrepresentation understood by `DecayChainViewer`, as see above:\n\n```python\nDecayChainViewer(dc.to_dict())\n```\n\nThe fact that 2 representations of particle decay chains are provided ensures\nthe following:\n\n1. Human-readable (class) and computer-efficient (dictionary) alternatives.\n2. Flexibility for parsing, manipulation and storage of decay chain information.\n\n### Decay modeling\n\nThe most common way to create a decay chain is to read in an [AmpGen]\nstyle syntax from a file or a string. You can use:\n\n```python\nfrom decaylanguage.modeling import AmplitudeChain\nlines, parameters, constants, states = AmplitudeChain.read_ampgen(text='''\nEventType D0 K- pi+ pi+ pi-\n\nD0[D]{K*(892)bar0{K-,pi+},rho(770)0{pi+,pi-}} 0 1 0.1 0 1 0.1\n\nK(1460)bar-_mass 0 1460 1\nK(1460)bar-_width 0 250 1\n\na(1)(1260)+::Spline::Min 0.18412\na(1)(1260)+::Spline::Max 1.86869\na(1)(1260)+::Spline::N 34\n''')\n```\n\nHere, `lines` will be a list of AmplitudeChain lines (pretty print supported in Jupyter notebooks),\n`parameters` will be a table of parameters (ranged parameters not yet supported),\n`constants` will be a table of constants,\nand `states` will be the list of known states (EventType).\n\n#### Converters\n\nYou can output to a format (currently only [GooFit] supported, feel free\nto make a PR to add more). Use a subclass of DecayChain, in this case,\nGooFitChain. To use the [GooFit] output, type from the shell:\n\n```bash\npython -m decaylanguage -G goofit myinput.opts\n```\n\n## Contributors\n\nWe hereby acknowledge the contributors that made this project possible ([emoji key](https://allcontributors.org/docs/en/emoji-key)):\n<!-- ALL-CONTRIBUTORS-LIST:START - Do not remove or modify this section -->\n<!-- prettier-ignore-start -->\n<!-- markdownlint-disable -->\n<table>\n <tbody>\n <tr>\n <td align=\"center\" valign=\"top\" width=\"14.28%\"><a href=\"http://cern.ch/eduardo.rodrigues\"><img src=\"https://avatars.githubusercontent.com/u/5013581?v=4?s=100\" width=\"100px;\" alt=\"Eduardo Rodrigues\"/><br /><sub><b>Eduardo Rodrigues</b></sub></a><br /><a href=\"#maintenance-eduardo-rodrigues\" title=\"Maintenance\">\ud83d\udea7</a> <a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=eduardo-rodrigues\" title=\"Code\">\ud83d\udcbb</a> <a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=eduardo-rodrigues\" title=\"Documentation\">\ud83d\udcd6</a></td>\n <td align=\"center\" valign=\"top\" width=\"14.28%\"><a href=\"http://iscinumpy.dev\"><img src=\"https://avatars.githubusercontent.com/u/4616906?v=4?s=100\" width=\"100px;\" alt=\"Henry Schreiner\"/><br /><sub><b>Henry Schreiner</b></sub></a><br /><a href=\"#maintenance-henryiii\" title=\"Maintenance\">\ud83d\udea7</a> <a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=henryiii\" title=\"Code\">\ud83d\udcbb</a> <a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=henryiii\" title=\"Documentation\">\ud83d\udcd6</a></td>\n <td align=\"center\" valign=\"top\" width=\"14.28%\"><a href=\"https://github.com/yipengsun\"><img src=\"https://avatars.githubusercontent.com/u/33738176?v=4?s=100\" width=\"100px;\" alt=\"Yipeng Sun\"/><br /><sub><b>Yipeng Sun</b></sub></a><br /><a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=yipengsun\" title=\"Code\">\ud83d\udcbb</a></td>\n <td align=\"center\" valign=\"top\" width=\"14.28%\"><a href=\"https://github.com/chrisburr\"><img src=\"https://avatars.githubusercontent.com/u/5220533?v=4?s=100\" width=\"100px;\" alt=\"Chris Burr\"/><br /><sub><b>Chris Burr</b></sub></a><br /><a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=chrisburr\" title=\"Documentation\">\ud83d\udcd6</a></td>\n <td align=\"center\" valign=\"top\" width=\"14.28%\"><a href=\"https://www.lieret.net\"><img src=\"https://avatars.githubusercontent.com/u/13602468?v=4?s=100\" width=\"100px;\" alt=\"Kilian Lieret\"/><br /><sub><b>Kilian Lieret</b></sub></a><br /><a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=klieret\" title=\"Documentation\">\ud83d\udcd6</a></td>\n <td align=\"center\" valign=\"top\" width=\"14.28%\"><a href=\"https://github.com/sognetic\"><img src=\"https://avatars.githubusercontent.com/u/10749132?v=4?s=100\" width=\"100px;\" alt=\"Moritz Bauer\"/><br /><sub><b>Moritz Bauer</b></sub></a><br /><a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=sognetic\" title=\"Code\">\ud83d\udcbb</a></td>\n <td align=\"center\" valign=\"top\" width=\"14.28%\"><a href=\"https://github.com/FlorianReiss\"><img src=\"https://avatars.githubusercontent.com/u/44642966?v=4?s=100\" width=\"100px;\" alt=\"FlorianReiss\"/><br /><sub><b>FlorianReiss</b></sub></a><br /><a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=FlorianReiss\" title=\"Code\">\ud83d\udcbb</a> <a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=FlorianReiss\" title=\"Documentation\">\ud83d\udcd6</a></td>\n </tr>\n <tr>\n <td align=\"center\" valign=\"top\" width=\"14.28%\"><a href=\"https://gitlab.cern.ch/users/admorris\"><img src=\"https://avatars.githubusercontent.com/u/15155249?v=4?s=100\" width=\"100px;\" alt=\"Adam Morris\"/><br /><sub><b>Adam Morris</b></sub></a><br /><a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=admorris\" title=\"Code\">\ud83d\udcbb</a></td>\n <td align=\"center\" valign=\"top\" width=\"14.28%\"><a href=\"https://github.com/ch2ohch2oh\"><img src=\"https://avatars.githubusercontent.com/u/7986711?v=4?s=100\" width=\"100px;\" alt=\"Dazhi Wang\"/><br /><sub><b>Dazhi Wang</b></sub></a><br /><a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=ch2ohch2oh\" title=\"Code\">\ud83d\udcbb</a></td>\n <td align=\"center\" valign=\"top\" width=\"14.28%\"><a href=\"https://github.com/manuelfs\"><img src=\"https://avatars.githubusercontent.com/u/4977423?v=4?s=100\" width=\"100px;\" alt=\"Manuel Franco Sevilla\"/><br /><sub><b>Manuel Franco Sevilla</b></sub></a><br /><a href=\"https://github.com/scikit-hep/decaylanguage/commits?author=manuelfs\" title=\"Code\">\ud83d\udcbb</a></td>\n </tr>\n </tbody>\n</table>\n\n<!-- markdownlint-restore -->\n<!-- prettier-ignore-end -->\n\n<!-- ALL-CONTRIBUTORS-LIST:END -->\n\nThis project follows the [all-contributors](https://github.com/all-contributors/all-contributors) specification.\n\n## Acknowledgements\n\nThe UK Science and Technology Facilities Council (STFC) and the University of Liverpool\nprovide funding for Eduardo Rodrigues (2020-) to work on this project part-time.\n\nSupport for this work was provided by the National Science Foundation\ncooperative agreement OAC-1450377 (DIANA/HEP) in 2016-2019\nand has been provided by OAC-1836650 (IRIS-HEP) since 2019.\nAny opinions, findings, conclusions or recommendations expressed in this material\nare those of the authors and do not necessarily reflect the views of the National Science Foundation.\n\n\n[AmpGen]: https://gitlab.cern.ch/lhcb/Gauss/tree/LHCBGAUSS-1058.AmpGenDev/Gen/AmpGen\n[GooFit]: https://GooFit.github.io\n",
"bugtrack_url": null,
"license": null,
"summary": "A language to describe, manipulate and convert particle decays",
"version": "0.18.5",
"project_urls": {
"Homepage": "https://github.com/scikit-hep/decaylanguage"
},
"split_keywords": [
"hep",
" chain",
" decay",
" particle",
" representation"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "01a220f58afb3bbd45f1224ac2845c4e135057b1a6d11cba83b5e68ed17e8d2f",
"md5": "37b8282106f630ec7a4d0b770e0006eb",
"sha256": "7ddb2eb16fc89ef70fcfd09a255601490cdf81d849fcd69079fab83adfac7735"
},
"downloads": -1,
"filename": "decaylanguage-0.18.5-py3-none-any.whl",
"has_sig": false,
"md5_digest": "37b8282106f630ec7a4d0b770e0006eb",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 251655,
"upload_time": "2024-10-07T10:01:23",
"upload_time_iso_8601": "2024-10-07T10:01:23.997100Z",
"url": "https://files.pythonhosted.org/packages/01/a2/20f58afb3bbd45f1224ac2845c4e135057b1a6d11cba83b5e68ed17e8d2f/decaylanguage-0.18.5-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7c9342bff92bca1fc3a48ebb516a22c90aab3c711d0e91c19ab90ab34a582a6b",
"md5": "46be0cb873ea5d45e6b2f75f551bb636",
"sha256": "1e955efa0c5e76e222a92723856d94f5db7197ab28262036b895010007f739a2"
},
"downloads": -1,
"filename": "decaylanguage-0.18.5.tar.gz",
"has_sig": false,
"md5_digest": "46be0cb873ea5d45e6b2f75f551bb636",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 407293,
"upload_time": "2024-10-07T10:01:26",
"upload_time_iso_8601": "2024-10-07T10:01:26.216461Z",
"url": "https://files.pythonhosted.org/packages/7c/93/42bff92bca1fc3a48ebb516a22c90aab3c711d0e91c19ab90ab34a582a6b/decaylanguage-0.18.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-07 10:01:26",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "scikit-hep",
"github_project": "decaylanguage",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "decaylanguage"
}