# Flask-AdminLTE3
Flask-AdminLTE3 packages [AdminLTE3](https://adminlte.io/themes/dev/AdminLTE/index.html) into an extension that mostly consists
of a blueprint named 'adminlte'. It is Admin Theme for Flask-Admin.
## Installation
Installation using pip:
pip install Flask-AdminLTE3
## Compatibility
This package is compatible Python versions 2.7, 3.4, 3.5 and 3.6.
## Usage
Here is an example:
from flask_adminlte3 import AdminLTE3
[...]
AdminLTE3(app)
## Admin Theme for Flask-Admin
from flask_admin.contrib.sqla import ModelView
from flask_admin.contrib.fileadmin import FileAdmin
class AdminLTEModelView(ModelView):
list_template = 'flask-admin/model/list.html'
create_template = 'flask-admin/model/create.html'
edit_template = 'flask-admin/model/edit.html'
details_template = 'flask-admin/model/details.html'
create_modal_template = 'flask-admin/model/modals/create.html'
edit_modal_template = 'flask-admin/model/modals/edit.html'
details_modal_template = 'flask-admin/model/modals/details.html'
class AdminLTEFileAdmin(FileAdmin):
list_template = 'flask-admin/file/list.html'
upload_template = 'flask-admin/file/form.html'
mkdir_template = 'flask-admin/file/form.html'
rename_template = 'flask-admin/file/form.html'
edit_template = 'flask-admin/file/form.html'
upload_modal_template = 'flask-admin/file/modals/form.html'
mkdir_modal_template = 'flask-admin/file/modals/form.html'
rename_modal_template = 'flask-admin/file/modals/form.html'
edit_modal_template = 'flask-admin/file/modals/form.html'
class MyAdminIndexView(AdminIndexView):
@expose('/', methods=['GET', 'POST'])
def index(self):
return self.render('myadmin3/my_index.html')
from flask_admin import Admin
admin = Admin(name='Admin Dashboard',
base_template='myadmin3/my_master.html',
template_mode='bootstrap4',
index_view=MyAdminIndexView())
base template myadmin3/my_master.html code:
{% extends 'flask-admin/base.html' %}
[...]
index template myadmin3/my_index.html code:
{% extends 'myadmin3/my_master.html' %}
[...]
## Screenshots
Admin Area:
* Demo Model :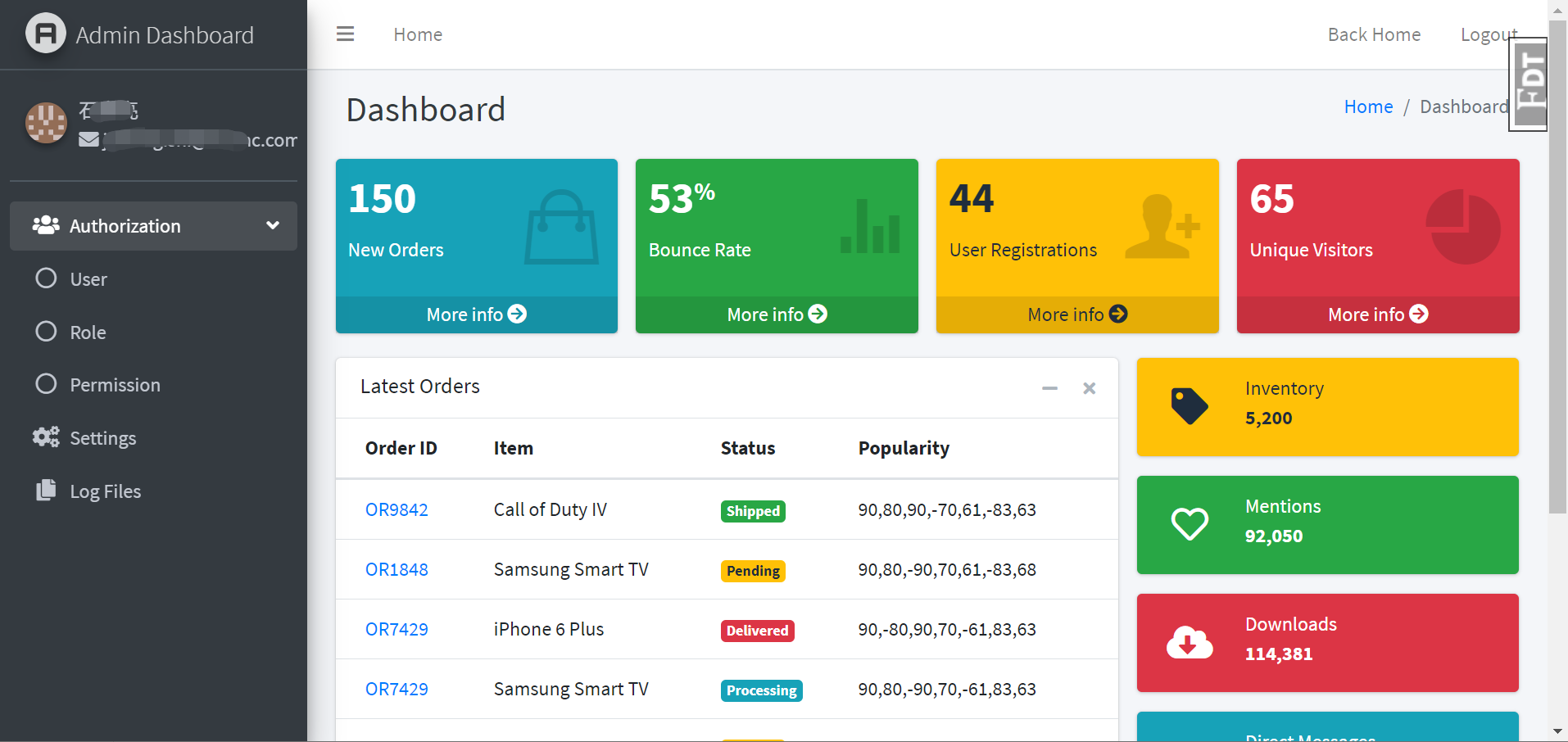
* Demo List Model: 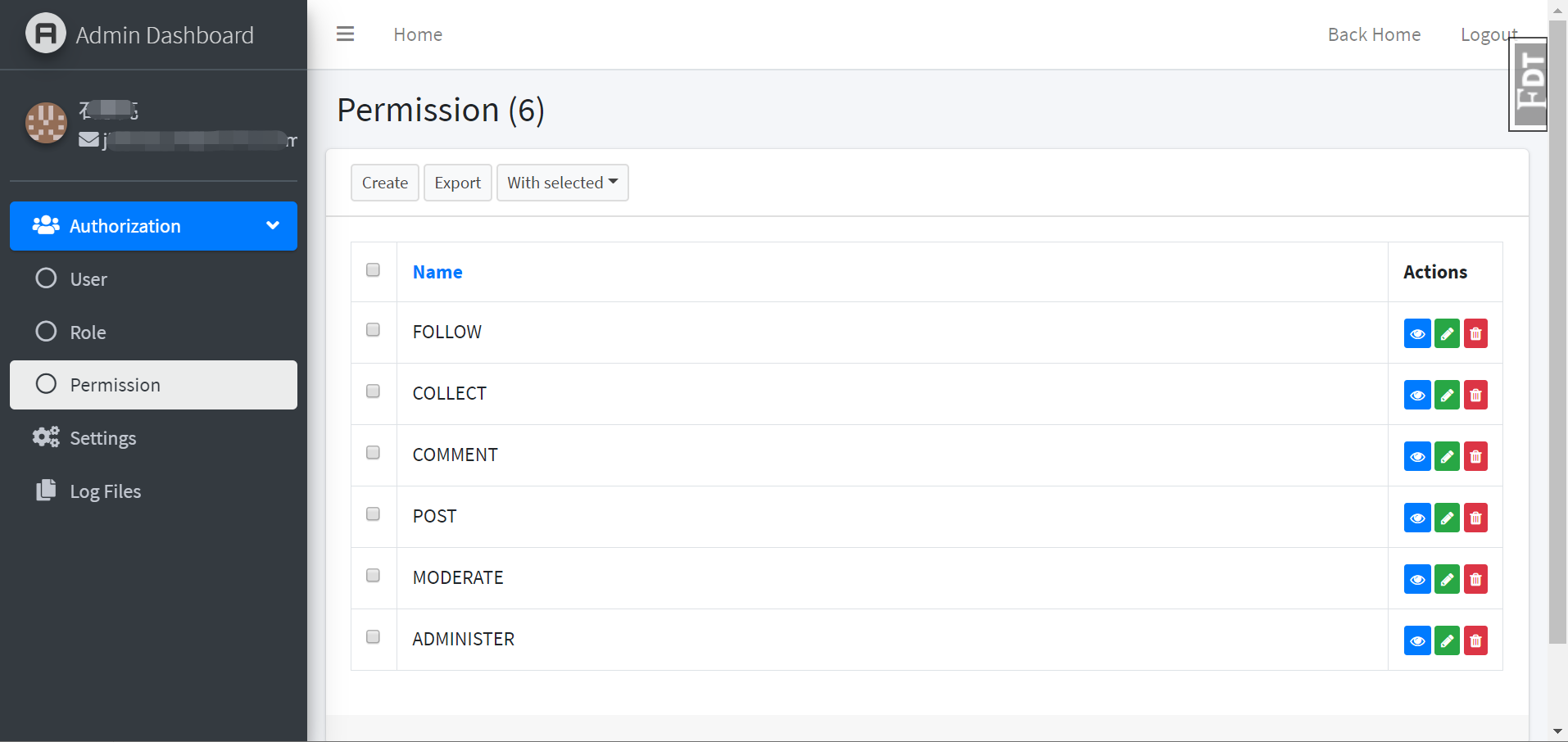
* Demo Creating Model: 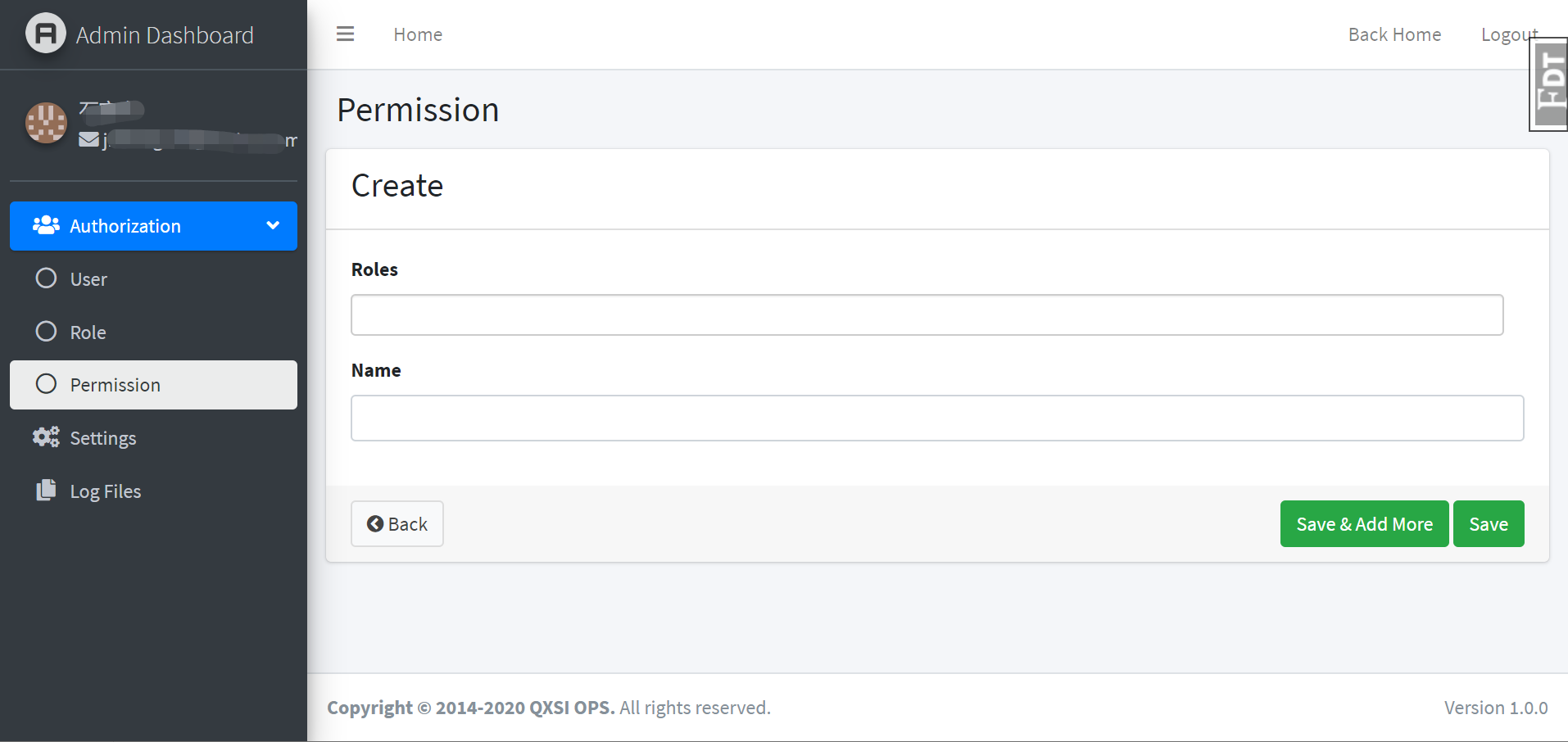
* Demo Editing Model: 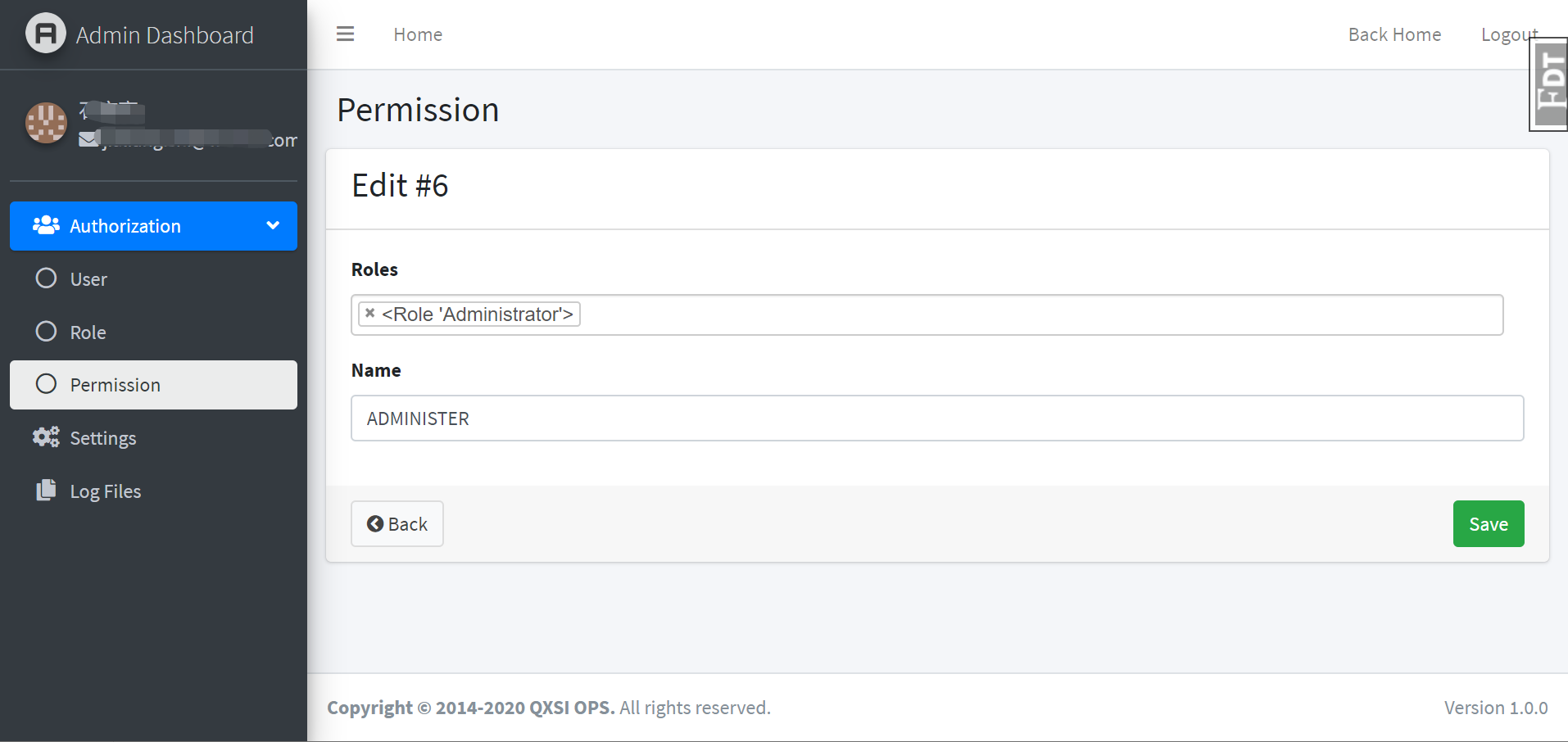
Raw data
{
"_id": null,
"home_page": "https://github.com/shijl0925/Flask-AdminLTE3",
"name": "Flask-AdminLTE3",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "",
"author": "Jialiang Shi",
"author_email": "kevin09254930sjl@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/47/3b/9979f5f29c5c5af99ac58479dc6abf0f2bb3e03419dd63628cefbdcc4f0f/Flask-AdminLTE3-1.0.9.tar.gz",
"platform": "any",
"description": "# Flask-AdminLTE3\n\n\nFlask-AdminLTE3 packages [AdminLTE3](https://adminlte.io/themes/dev/AdminLTE/index.html) into an extension that mostly consists\nof a blueprint named 'adminlte'. It is Admin Theme for Flask-Admin.\n\n## Installation\n\nInstallation using pip:\n\n pip install Flask-AdminLTE3\n \n\n## Compatibility\n\nThis package is compatible Python versions 2.7, 3.4, 3.5 and 3.6.\n\n## Usage\n\nHere is an example:\n\n from flask_adminlte3 import AdminLTE3\n \n [...]\n \n AdminLTE3(app)\n\n\n## Admin Theme for Flask-Admin\n\n from flask_admin.contrib.sqla import ModelView\n from flask_admin.contrib.fileadmin import FileAdmin\n\n\n class AdminLTEModelView(ModelView):\n list_template = 'flask-admin/model/list.html'\n create_template = 'flask-admin/model/create.html'\n edit_template = 'flask-admin/model/edit.html'\n details_template = 'flask-admin/model/details.html'\n\n create_modal_template = 'flask-admin/model/modals/create.html'\n edit_modal_template = 'flask-admin/model/modals/edit.html'\n details_modal_template = 'flask-admin/model/modals/details.html'\n\n\n class AdminLTEFileAdmin(FileAdmin):\n list_template = 'flask-admin/file/list.html'\n\n upload_template = 'flask-admin/file/form.html'\n mkdir_template = 'flask-admin/file/form.html'\n rename_template = 'flask-admin/file/form.html'\n edit_template = 'flask-admin/file/form.html'\n\n upload_modal_template = 'flask-admin/file/modals/form.html'\n mkdir_modal_template = 'flask-admin/file/modals/form.html'\n rename_modal_template = 'flask-admin/file/modals/form.html'\n edit_modal_template = 'flask-admin/file/modals/form.html'\n\n\n class MyAdminIndexView(AdminIndexView):\n @expose('/', methods=['GET', 'POST'])\n def index(self):\n return self.render('myadmin3/my_index.html')\n\n\n from flask_admin import Admin\n admin = Admin(name='Admin Dashboard',\n base_template='myadmin3/my_master.html',\n template_mode='bootstrap4',\n index_view=MyAdminIndexView())\n\n\nbase template myadmin3/my_master.html code:\n\n {% extends 'flask-admin/base.html' %}\n [...]\n\nindex template myadmin3/my_index.html code:\n\n {% extends 'myadmin3/my_master.html' %}\n [...]\n \n## Screenshots\n\nAdmin Area:\n\n* Demo Model :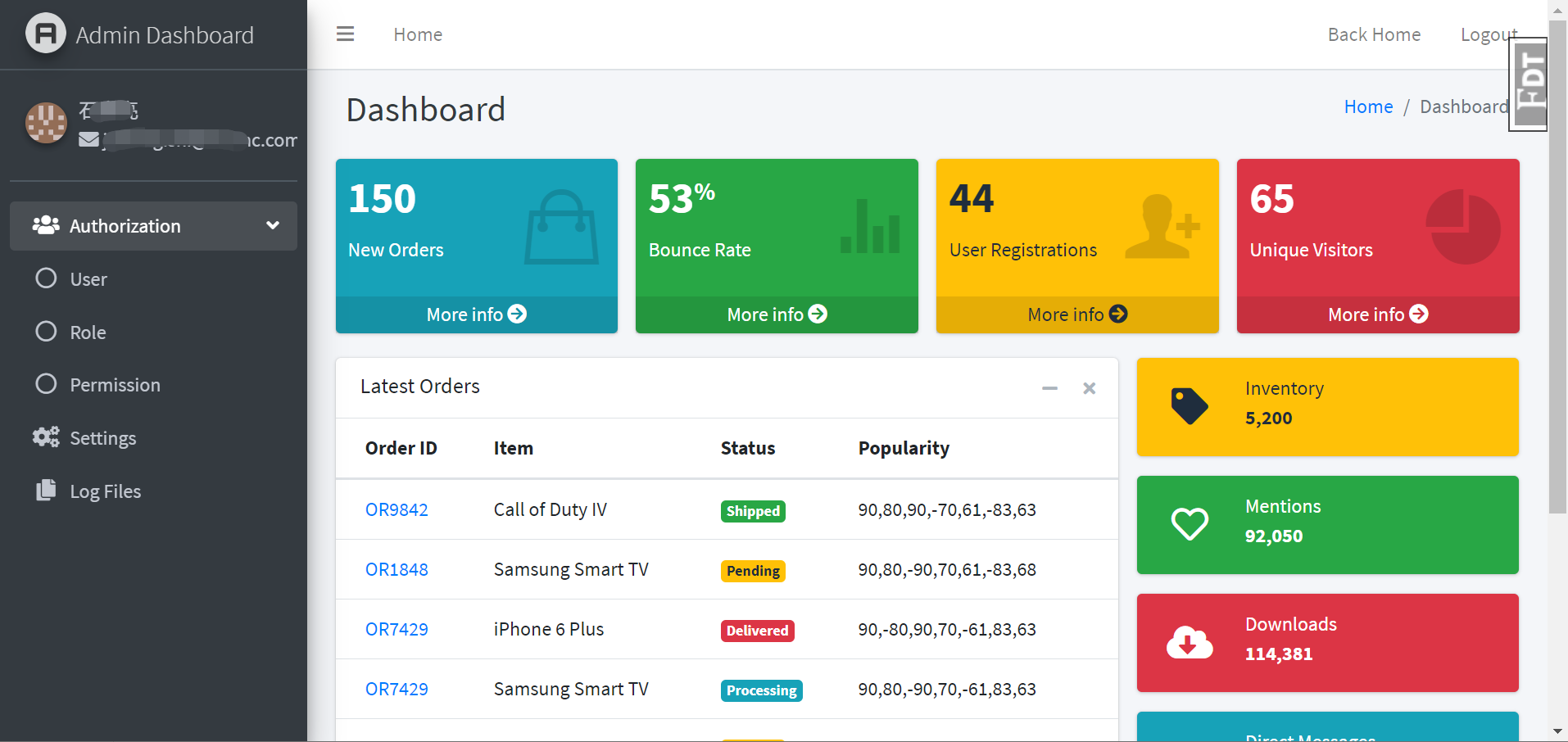\n\n* Demo List Model: 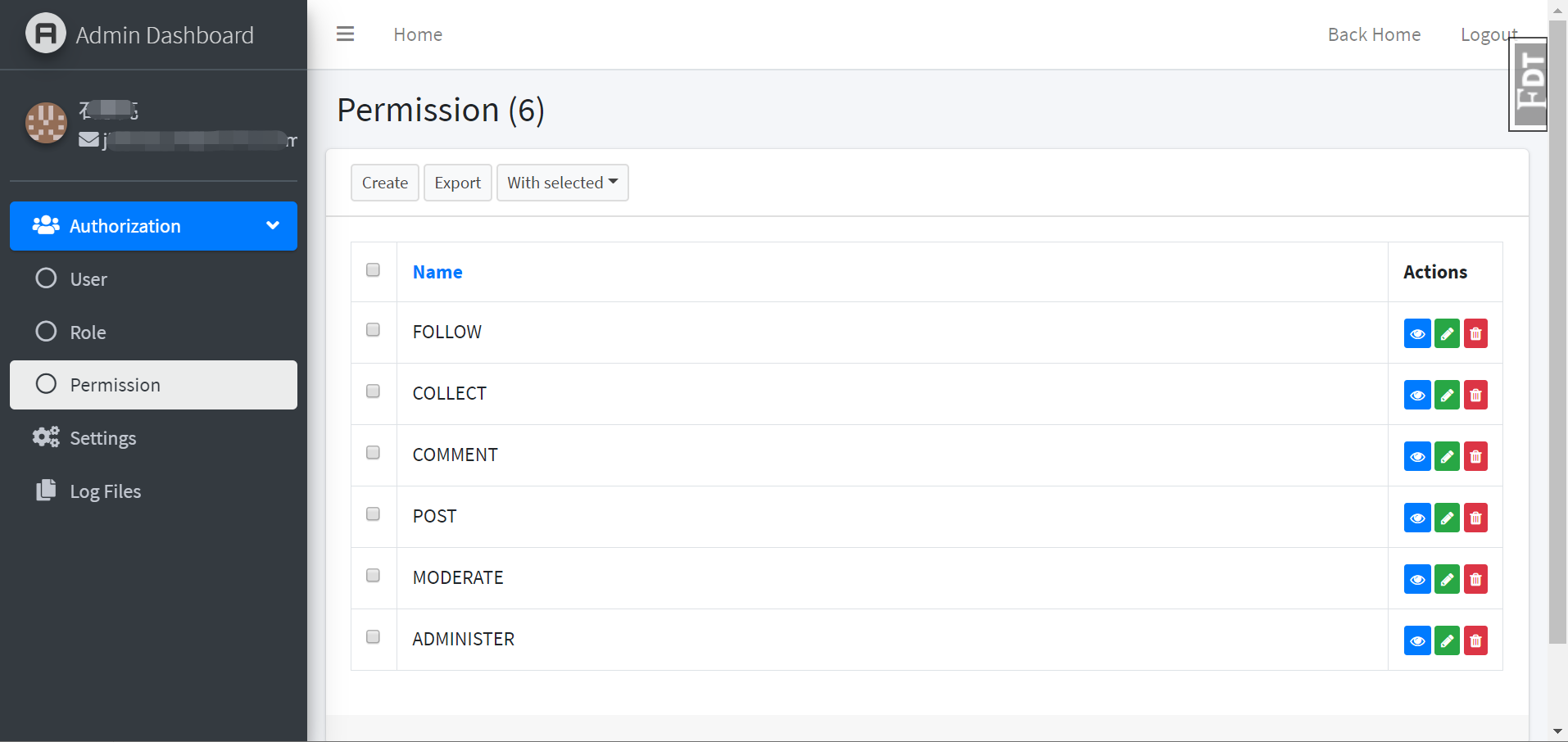\n\n* Demo Creating Model: 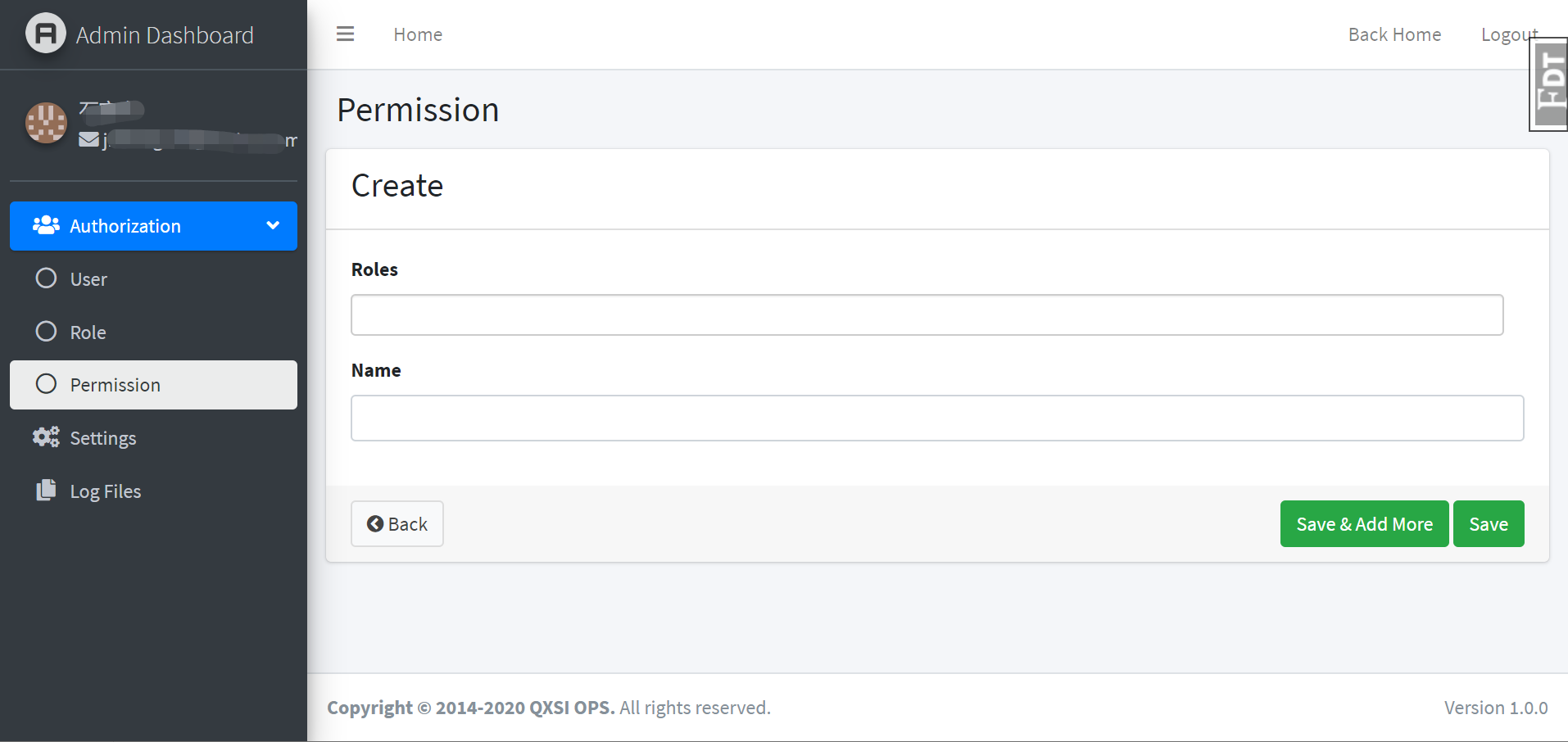\n\n* Demo Editing Model: 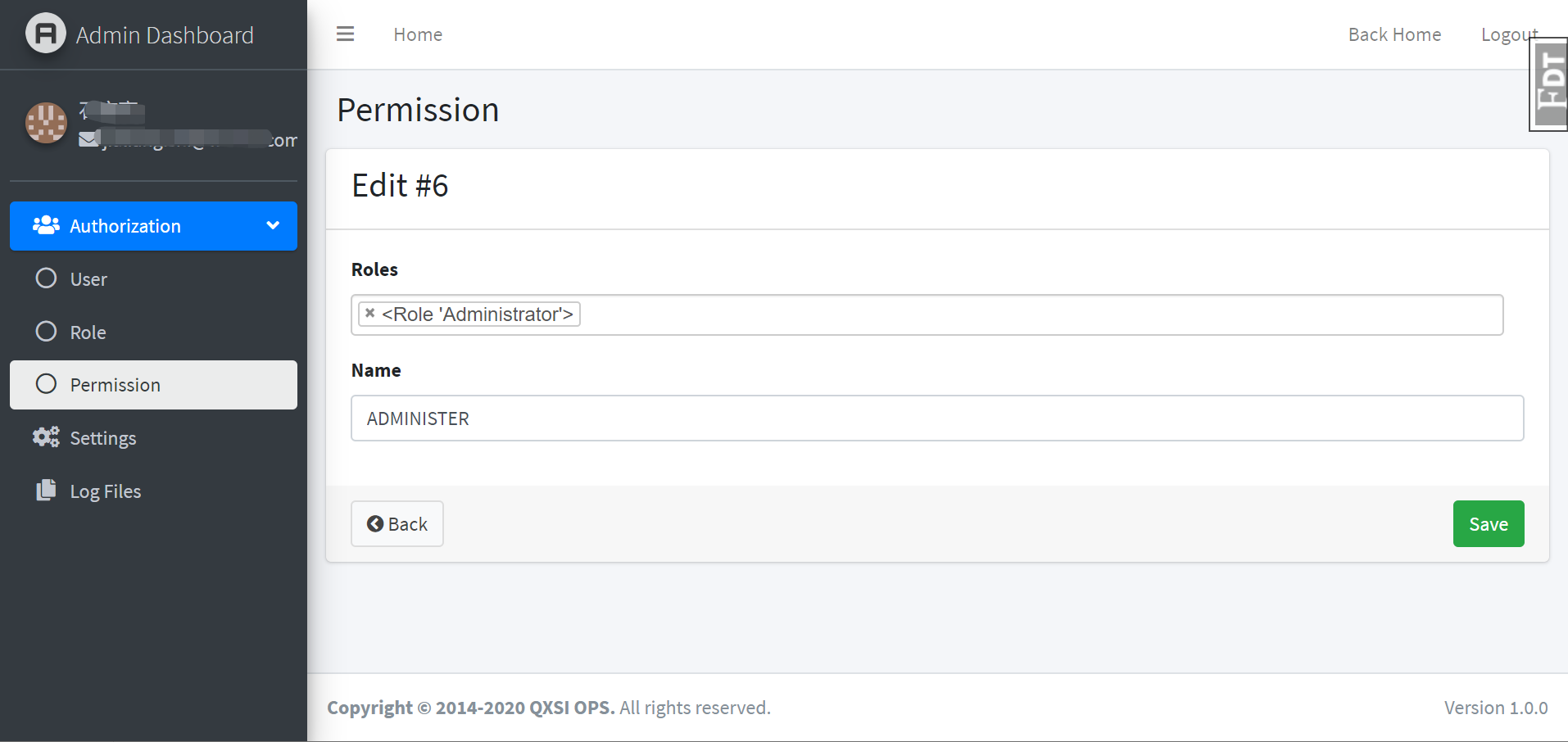\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "AdminLTE3 Theme for Flask-Admin.",
"version": "1.0.9",
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "e05ec39fb67fcf620812d36505e851e86f654e9221643d2c4244a409ee324763",
"md5": "6f0cec030fab346ae4cd4be5d6aa2b2f",
"sha256": "77a5c6c1b2d1b943181dc0662faad68e7c1c2d2c2c4eaec4647ddef4cea9ba45"
},
"downloads": -1,
"filename": "Flask_AdminLTE3-1.0.9-py3-none-any.whl",
"has_sig": false,
"md5_digest": "6f0cec030fab346ae4cd4be5d6aa2b2f",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 22737431,
"upload_time": "2022-11-15T01:49:46",
"upload_time_iso_8601": "2022-11-15T01:49:46.729396Z",
"url": "https://files.pythonhosted.org/packages/e0/5e/c39fb67fcf620812d36505e851e86f654e9221643d2c4244a409ee324763/Flask_AdminLTE3-1.0.9-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "473b9979f5f29c5c5af99ac58479dc6abf0f2bb3e03419dd63628cefbdcc4f0f",
"md5": "746cfd6a369629b8c3bb9477d07f92f2",
"sha256": "237b233cd26b98e415666dcde46dbcd24fc2ce336db6f9bf829a07a9347028bf"
},
"downloads": -1,
"filename": "Flask-AdminLTE3-1.0.9.tar.gz",
"has_sig": false,
"md5_digest": "746cfd6a369629b8c3bb9477d07f92f2",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 21426689,
"upload_time": "2022-11-15T01:50:19",
"upload_time_iso_8601": "2022-11-15T01:50:19.523208Z",
"url": "https://files.pythonhosted.org/packages/47/3b/9979f5f29c5c5af99ac58479dc6abf0f2bb3e03419dd63628cefbdcc4f0f/Flask-AdminLTE3-1.0.9.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2022-11-15 01:50:19",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "shijl0925",
"github_project": "Flask-AdminLTE3",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "flask-adminlte3"
}