Name | HueBLE JSON |
Version |
1.0.6
JSON |
| download |
home_page | None |
Summary | Python module for controlling and monitoring Bluetooth Philips Hue bulbs |
upload_time | 2024-06-30 13:56:17 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.11 |
license | MIT License Copyright (c) 2024 Harvey Lelliott Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
philips hue
ble
home assistant
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# HueBLE
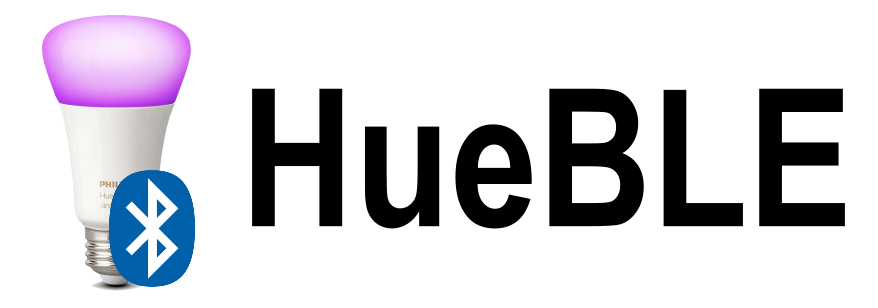
[](https://pypi.python.org/pypi/HueBLE)
[](https://hueble.readthedocs.io/en/latest/?badge=latest)
[](https://github.com/psf/black)
Python module for controlling Bluetooth Philips Hue lights.
- 👌 Free software: MIT license
- 🍝 Sauce: https://github.com/flip-dots/HueBLE
- 🖨️ Documentation: https://hueble.readthedocs.io/en/latest/
- 📦 PIP: https://pypi.org/project/HueBLE/
This Python module enables you to control Philips Hue Bluetooth lights directly
from your computer, without the need for a Hue bridge or ZigBee dongle.
It leverages the Bleak library to interact with Bluetooth Philips Hue lights.
## Features
- 💡 On/Off control
- 🌗 Brightness control
- 🌡️ Colour temp control
- 🌈 XY colour control
- ❔ Light state (power/brightness/temp/colour)
- ⚙️ Light configuration (name)
- 📊 Light metadata (manufacturer/model/zigbee address)
- 🤜 Supports push & polling models
- 🔂 Simple structure
- 📜 Mediocre documentation
- ✔️ More emojis than strictly necessary
## Requirements
- 🐍 Python 3.11+
- 📶 Bleak 0.19.0+
- 📶 bleak-retry-connector
## Supported Operating Systems
- 🐧 Linux (BlueZ)
- Ubuntu Desktop
- Arch (HomeAssistant OS)
- 🏢 Windows
- Windows 10
- 💾 Mac OSX
- Maybe?
## Documentation
https://hueble.readthedocs.io/en/latest/
## Installation
### PIP
```
pip install HueBLE
```
### Manual
HueBLE consists of a single file (HueBLE.py) which you can simply put in the
same directory as your program. If you are using manual installation make sure
the dependencies are installed as well.
```
pip install bleak bleak-retry-connector
```
## Examples
### Quick start example
Example code from example.py
```python
import asyncio
from bleak import BleakScanner
import HueBLE
async def main():
# Address of light to connect to
address = "F6:9B:48:A4:D2:D8"
# Obtain the BLEDevice from bleak
device = await BleakScanner.find_device_by_address(address)
# Initialize the light object
light = HueBLE.HueBleLight(device)
# Optionally we could call connect but it will be called automatically
# on the first request to the light. You might want to call this if
# you want to subscribe to state changes without changing the lights state.
# await light.connect()
# Will automatically connect to the light and turn it off
await light.set_power(False)
# Wait
await asyncio.sleep(5)
# Turn the light back on again
await light.set_power(True)
if __name__ == "__main__":
asyncio.run(main())
```
### Demo program
A more fully featured demo program can be found in ``` examples/demo.py ``` which demonstrates all of the implemented features.
Raw data
{
"_id": null,
"home_page": null,
"name": "HueBLE",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.11",
"maintainer_email": null,
"keywords": "Philips Hue, BLE, Home Assistant",
"author": null,
"author_email": "Harvey Lelliott <harveylelliott@duck.com>",
"download_url": "https://files.pythonhosted.org/packages/80/7b/c6a34de5aaa0e4036e9d80f2b0edaa5700bdf2a6df530ec4715ae967290b/hueble-1.0.6.tar.gz",
"platform": null,
"description": "# HueBLE\n\n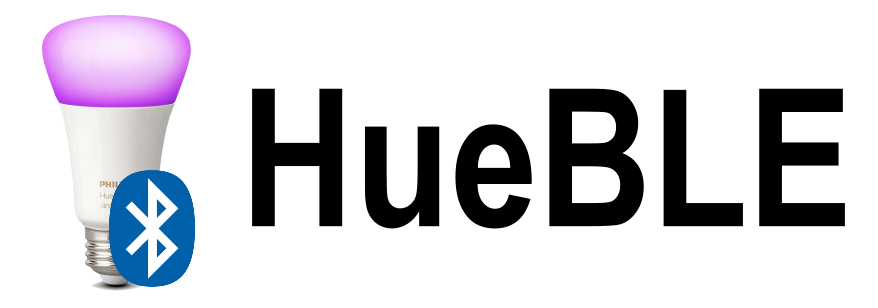\n\n[](https://pypi.python.org/pypi/HueBLE)\n[](https://hueble.readthedocs.io/en/latest/?badge=latest)\n[](https://github.com/psf/black)\n\nPython module for controlling Bluetooth Philips Hue lights.\n - \ud83d\udc4c Free software: MIT license\n - \ud83c\udf5d Sauce: https://github.com/flip-dots/HueBLE\n - \ud83d\udda8\ufe0f Documentation: https://hueble.readthedocs.io/en/latest/\n - \ud83d\udce6 PIP: https://pypi.org/project/HueBLE/\n\n\nThis Python module enables you to control Philips Hue Bluetooth lights directly\nfrom your computer, without the need for a Hue bridge or ZigBee dongle.\nIt leverages the Bleak library to interact with Bluetooth Philips Hue lights.\n\n\n## Features\n\n- \ud83d\udca1 On/Off control\n- \ud83c\udf17 Brightness control\n- \ud83c\udf21\ufe0f Colour temp control \n- \ud83c\udf08 XY colour control\n- \u2754 Light state (power/brightness/temp/colour)\n- \u2699\ufe0f Light configuration (name)\n- \ud83d\udcca Light metadata (manufacturer/model/zigbee address)\n- \ud83e\udd1c Supports push & polling models\n- \ud83d\udd02 Simple structure\n- \ud83d\udcdc Mediocre documentation\n- \u2714\ufe0f More emojis than strictly necessary\n\n\n## Requirements\n\n- \ud83d\udc0d Python 3.11+\n- \ud83d\udcf6 Bleak 0.19.0+\n- \ud83d\udcf6 bleak-retry-connector\n\n\n## Supported Operating Systems\n\n- \ud83d\udc27 Linux (BlueZ)\n - Ubuntu Desktop\n - Arch (HomeAssistant OS)\n- \ud83c\udfe2 Windows\n - Windows 10 \n- \ud83d\udcbe Mac OSX\n - Maybe?\n\n\n## Documentation\n\nhttps://hueble.readthedocs.io/en/latest/\n\n\n## Installation\n\n\n### PIP\n\n```\npip install HueBLE\n```\n\n\n### Manual\n\nHueBLE consists of a single file (HueBLE.py) which you can simply put in the\nsame directory as your program. If you are using manual installation make sure\nthe dependencies are installed as well.\n\n```\npip install bleak bleak-retry-connector\n```\n\n\n## Examples\n\n\n### Quick start example\n\nExample code from example.py\n\n```python\nimport asyncio\nfrom bleak import BleakScanner\nimport HueBLE\n\n\nasync def main():\n\n # Address of light to connect to\n address = \"F6:9B:48:A4:D2:D8\"\n\n # Obtain the BLEDevice from bleak\n device = await BleakScanner.find_device_by_address(address)\n\n # Initialize the light object\n light = HueBLE.HueBleLight(device)\n\n # Optionally we could call connect but it will be called automatically\n # on the first request to the light. You might want to call this if\n # you want to subscribe to state changes without changing the lights state.\n # await light.connect()\n\n # Will automatically connect to the light and turn it off\n await light.set_power(False)\n\n # Wait\n await asyncio.sleep(5)\n\n # Turn the light back on again\n await light.set_power(True)\n\nif __name__ == \"__main__\":\n asyncio.run(main())\n\n```\n\n\n### Demo program\n\nA more fully featured demo program can be found in ``` examples/demo.py ``` which demonstrates all of the implemented features.\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2024 Harvey Lelliott Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.",
"summary": "Python module for controlling and monitoring Bluetooth Philips Hue bulbs",
"version": "1.0.6",
"project_urls": {
"Changelog": "https://hueble.readthedocs.io/en/latest/history.html",
"Documentation": "https://hueble.readthedocs.io/en/latest/",
"Homepage": "https://github.com/flip-dots/HueBLE",
"Issues": "https://github.com/flip-dots/HueBLE/issues",
"Repository": "https://github.com/flip-dots/HueBLE"
},
"split_keywords": [
"philips hue",
" ble",
" home assistant"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "bba2536da85cbf173594a67185f33a26fc7a1b5835b4380eb50c9b6fb0b1f06f",
"md5": "2f1852014a4bc2781410b02c2e2c09df",
"sha256": "5ed02536efb64d0722de6513da82d68e557bdc88b2118110cdc96451d469b58f"
},
"downloads": -1,
"filename": "HueBLE-1.0.6-py3-none-any.whl",
"has_sig": false,
"md5_digest": "2f1852014a4bc2781410b02c2e2c09df",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.11",
"size": 13471,
"upload_time": "2024-06-30T13:56:15",
"upload_time_iso_8601": "2024-06-30T13:56:15.704583Z",
"url": "https://files.pythonhosted.org/packages/bb/a2/536da85cbf173594a67185f33a26fc7a1b5835b4380eb50c9b6fb0b1f06f/HueBLE-1.0.6-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "807bc6a34de5aaa0e4036e9d80f2b0edaa5700bdf2a6df530ec4715ae967290b",
"md5": "26d85d37b7a1c2f7cbde675d08b5697a",
"sha256": "08c6bde20651ec5c7daf7894a83930ae4519cb231d31718bc1c55df617d1169e"
},
"downloads": -1,
"filename": "hueble-1.0.6.tar.gz",
"has_sig": false,
"md5_digest": "26d85d37b7a1c2f7cbde675d08b5697a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.11",
"size": 15145,
"upload_time": "2024-06-30T13:56:17",
"upload_time_iso_8601": "2024-06-30T13:56:17.971705Z",
"url": "https://files.pythonhosted.org/packages/80/7b/c6a34de5aaa0e4036e9d80f2b0edaa5700bdf2a6df530ec4715ae967290b/hueble-1.0.6.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-30 13:56:17",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "flip-dots",
"github_project": "HueBLE",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "hueble"
}