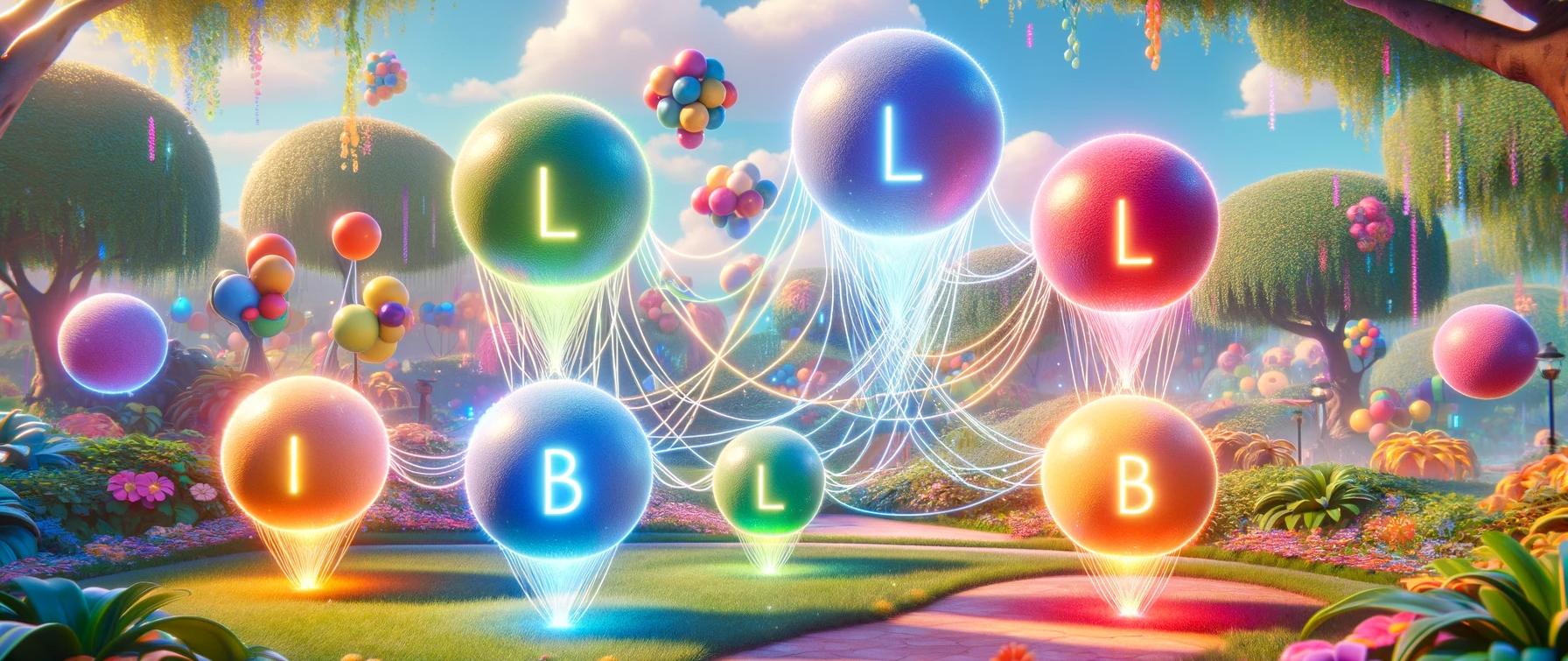
# LLlib - A Linked List Library for Python
[LLlib](https://pypi.org/project/LLlib/) is a simple Python library for creating [List Nodes]() / [Linked Lists]()
and performing dense operations (reverse in k-groups, swap in even length groups), design subsidiary structures (LRU/LFU caches, text editors, etc) and build derived classes based projects.
© Copyright 2023, All rights reserved to Hans Haller, MSc Graduate Student in Computer Science at Cranfield University, United Kingdom.
Author GitHub: https://github.com/Hnshlr
Python Package Index (PyPI): https://pypi.org/project/LLlib/
## Context
Linked lists are a fundamental data structure in computer science. They are used to store data in a linear fashion, and are often used to implement other data structures such as stacks, queues, and trees. Linked lists are also used to implement file systems, hash tables, and adjacency lists.
The objective of this package/library (tbd later) is to provide a simple and easy to use interface for creating linked lists and performing simple or complex operations on them. The library is written in Python, and is designed to be used with Python 3.9 or higher.
The core finality of these classes is for anyone to create their own class derived from the `LinkedList` class, and implement their architectures based on the methods provided by the library.
## Installation
The [LLlib](https://pypi.org/project/LLlib/) library is available on [PyPI](https://pypi.org/) (Python Package Index, the official third-party software repository for Python), and can be installed using `pip`:
```shell
$ pip install LLlib
```
## Documentation
The documentation for the [LLlib](https://pypi.org/project/LLlib/) library is currently under development. The documentation will be available on [Read the Docs](https://readthedocs.org/), and will be accessible through the [LLlib](https://pypi.org/project/LLlib/) PyPI page.
## Usage
### Import the Package
````shell
$ python
````
````python
>>> from LLlib import *
````
### List Nodes
The [LLlib](https://pypi.org/project/LLlib/) library provides a `ListNode` class for creating list nodes. The `ListNode` class is a simple class that stores a value and a pointer to the next node in the list. The `ListNode` class can be used to create a linked list by chaining together multiple `ListNode` objects.
````python
>>> from LLlib import ListNode
>>> head = ListNode(1, ListNode(2, ListNode(3, ListNode(4, ListNode(5)))))
>>> str(head)
'1 -> 2 -> 3 -> 4 -> 5 -> None'
>>> ListNode.reverse_list_in_k_group(head, k=2)
>>> str(head)
'2 -> 1 -> 4 -> 3 -> 5 -> None'
````
Ultimately, one may create a class derived from the `ListNode` class, and implement their own methods and attributes.
As an example, to create a Spotify-like music player, one may create a `Song` class derived from the `ListNode` class, and implement their own methods and attributes related to the project at hand.
This class will allow the user to utilize `ListNode` methods and attributes to manipulate the data more efficiently (e.g. reversing the playlist, shuffling the songs, etc).
### Linked Lists
[LLlib](https://pypi.org/project/LLlib/) also provides a `LinkedList` class for creating linked lists. The `LinkedList` class is a simple class that stores a pointer to the head of the list. The `LinkedList` class can be used to create a complete linked lists (of `ListNode` objects) by chaining together multiple `ListNode` objects, using data structures such as lists, tuples, sets, dictionaries, etc.
````python
>>> from LLlib import LinkedList
>>> list = [1, 2, 3, 4, 5]
>>> head = LinkedList(list)
>>> str(head)
'1 -> 2 -> 3 -> 4 -> 5 -> None'
>>> jagged = [[1, 2, 3], [4, 5, [6, 7]], [8, 9, 10, 11]]
>>> head = LinkedList(jagged)
>>> str(head)
'1 -> 2 -> 3 -> 4 -> 5 -> 6 -> 7 -> 8 -> 9 -> 10 -> 11 -> None'
````
For more examples, see the [EXAMPLES.md](doc/EXAMPLES.md) markdown file.
## Acknowledgements
The [LLlib](https://pypi.org/project/LLlib/) library was developed by Hans Haller, MSc Graduate Student in Computer Science at Cranfield University, United Kingdom. Most of the code used is truly unique, and was developed by the author.
Most of the functions' concepts and ideas were inspired by Leetcode and HackerRank problems. All solutions were developed by the author, and in no way were copied from other sources.
## License
This project is licensed under the [MIT License](LICENSE).
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Library.
Copyright (c) 2023-2024, All rights reserved to Hans Haller.
Raw data
{
"_id": null,
"home_page": "https://github.com/Hnshlr/LinkedListLibrary",
"name": "LLlib",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "linkedlist,listnode,linked list,list node,singly linked list",
"author": "Hnshlr",
"author_email": "hanshaller@outlook.fr",
"download_url": "https://files.pythonhosted.org/packages/91/26/df2fefe69116dd0e31ed7037e15d90d8b384040292ea8718cbeb6f6113b4/LLlib-0.0.2.tar.gz",
"platform": null,
"description": "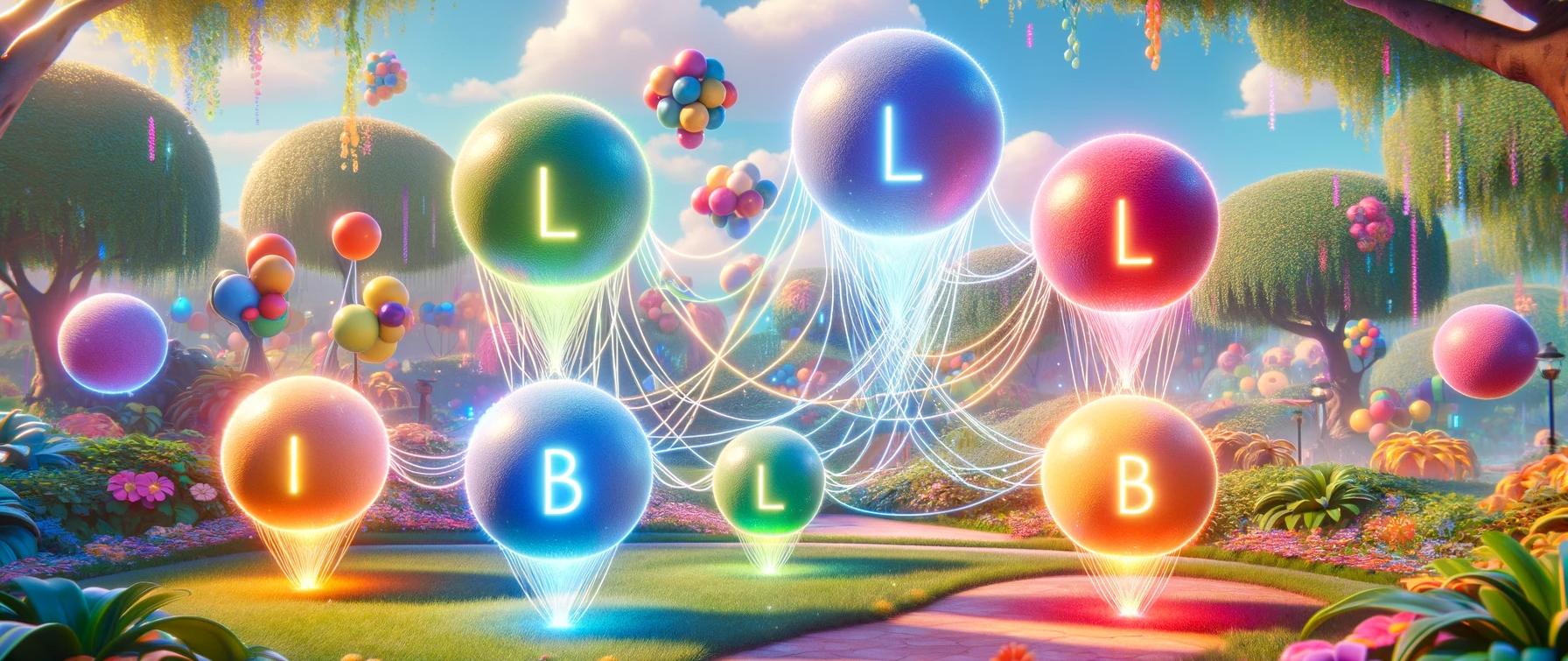\n\n# LLlib - A Linked List Library for Python\n\n[LLlib](https://pypi.org/project/LLlib/) is a simple Python library for creating [List Nodes]() / [Linked Lists]()\nand performing dense operations (reverse in k-groups, swap in even length groups), design subsidiary structures (LRU/LFU caches, text editors, etc) and build derived classes based projects.\n\n\u00a9 Copyright 2023, All rights reserved to Hans Haller, MSc Graduate Student in Computer Science at Cranfield University, United Kingdom.\n\nAuthor GitHub: https://github.com/Hnshlr\n\nPython Package Index (PyPI): https://pypi.org/project/LLlib/\n\n## Context\n\nLinked lists are a fundamental data structure in computer science. They are used to store data in a linear fashion, and are often used to implement other data structures such as stacks, queues, and trees. Linked lists are also used to implement file systems, hash tables, and adjacency lists.\n\nThe objective of this package/library (tbd later) is to provide a simple and easy to use interface for creating linked lists and performing simple or complex operations on them. The library is written in Python, and is designed to be used with Python 3.9 or higher.\n\nThe core finality of these classes is for anyone to create their own class derived from the `LinkedList` class, and implement their architectures based on the methods provided by the library.\n\n## Installation\n\nThe [LLlib](https://pypi.org/project/LLlib/) library is available on [PyPI](https://pypi.org/) (Python Package Index, the official third-party software repository for Python), and can be installed using `pip`:\n\n```shell\n$ pip install LLlib\n```\n\n## Documentation\n\nThe documentation for the [LLlib](https://pypi.org/project/LLlib/) library is currently under development. The documentation will be available on [Read the Docs](https://readthedocs.org/), and will be accessible through the [LLlib](https://pypi.org/project/LLlib/) PyPI page.\n\n\n## Usage\n\n### Import the Package\n\n````shell\n$ python\n````\n````python\n>>> from LLlib import *\n````\n\n### List Nodes\n\nThe [LLlib](https://pypi.org/project/LLlib/) library provides a `ListNode` class for creating list nodes. The `ListNode` class is a simple class that stores a value and a pointer to the next node in the list. The `ListNode` class can be used to create a linked list by chaining together multiple `ListNode` objects.\n\n````python\n>>> from LLlib import ListNode\n>>> head = ListNode(1, ListNode(2, ListNode(3, ListNode(4, ListNode(5)))))\n>>> str(head)\n'1 -> 2 -> 3 -> 4 -> 5 -> None'\n>>> ListNode.reverse_list_in_k_group(head, k=2)\n>>> str(head)\n'2 -> 1 -> 4 -> 3 -> 5 -> None'\n````\n\nUltimately, one may create a class derived from the `ListNode` class, and implement their own methods and attributes.\n\nAs an example, to create a Spotify-like music player, one may create a `Song` class derived from the `ListNode` class, and implement their own methods and attributes related to the project at hand.\n\nThis class will allow the user to utilize `ListNode` methods and attributes to manipulate the data more efficiently (e.g. reversing the playlist, shuffling the songs, etc).\n\n### Linked Lists\n\n[LLlib](https://pypi.org/project/LLlib/) also provides a `LinkedList` class for creating linked lists. The `LinkedList` class is a simple class that stores a pointer to the head of the list. The `LinkedList` class can be used to create a complete linked lists (of `ListNode` objects) by chaining together multiple `ListNode` objects, using data structures such as lists, tuples, sets, dictionaries, etc.\n\n````python\n>>> from LLlib import LinkedList\n>>> list = [1, 2, 3, 4, 5]\n>>> head = LinkedList(list)\n>>> str(head)\n'1 -> 2 -> 3 -> 4 -> 5 -> None'\n>>> jagged = [[1, 2, 3], [4, 5, [6, 7]], [8, 9, 10, 11]]\n>>> head = LinkedList(jagged)\n>>> str(head)\n'1 -> 2 -> 3 -> 4 -> 5 -> 6 -> 7 -> 8 -> 9 -> 10 -> 11 -> None'\n````\n\nFor more examples, see the [EXAMPLES.md](doc/EXAMPLES.md) markdown file.\n\n## Acknowledgements\n\nThe [LLlib](https://pypi.org/project/LLlib/) library was developed by Hans Haller, MSc Graduate Student in Computer Science at Cranfield University, United Kingdom. Most of the code used is truly unique, and was developed by the author. \n\nMost of the functions' concepts and ideas were inspired by Leetcode and HackerRank problems. All solutions were developed by the author, and in no way were copied from other sources.\n\n## License\n\nThis project is licensed under the [MIT License](LICENSE).\n\nThe above copyright notice and this permission notice shall be\nincluded in all copies or substantial portions of the Library.\n\n\u200e\n\nCopyright (c) 2023-2024, All rights reserved to Hans Haller.\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A simple Python library for creating linked lists and performing operations.",
"version": "0.0.2",
"project_urls": {
"Homepage": "https://github.com/Hnshlr/LinkedListLibrary"
},
"split_keywords": [
"linkedlist",
"listnode",
"linked list",
"list node",
"singly linked list"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "fb85ec833c7613c56ed7845622be8de5418f250d73972eb706bd74c3f584f7bb",
"md5": "700180c481e75a0a1cc7c80251031f49",
"sha256": "cb1115c1e1b75ef99011eefeb959d48abecaa6334fc77dc32a4f0925c1fb848f"
},
"downloads": -1,
"filename": "LLlib-0.0.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "700180c481e75a0a1cc7c80251031f49",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 7486,
"upload_time": "2023-12-17T21:14:38",
"upload_time_iso_8601": "2023-12-17T21:14:38.910175Z",
"url": "https://files.pythonhosted.org/packages/fb/85/ec833c7613c56ed7845622be8de5418f250d73972eb706bd74c3f584f7bb/LLlib-0.0.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9126df2fefe69116dd0e31ed7037e15d90d8b384040292ea8718cbeb6f6113b4",
"md5": "515bca880112969a765a2a64064f569e",
"sha256": "9416b16d3d57a2798e6d46e3c9c121b5e8c31dfc3ab9b5154ddf2f41819016e7"
},
"downloads": -1,
"filename": "LLlib-0.0.2.tar.gz",
"has_sig": false,
"md5_digest": "515bca880112969a765a2a64064f569e",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 8160,
"upload_time": "2023-12-17T21:14:40",
"upload_time_iso_8601": "2023-12-17T21:14:40.486555Z",
"url": "https://files.pythonhosted.org/packages/91/26/df2fefe69116dd0e31ed7037e15d90d8b384040292ea8718cbeb6f6113b4/LLlib-0.0.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-12-17 21:14:40",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Hnshlr",
"github_project": "LinkedListLibrary",
"github_not_found": true,
"lcname": "lllib"
}