# Nectar2P
**Nectar2P** — A secure and fast open-source Python library for P2P file transfers, featuring optional encryption and NAT traversal support. With Nectar2P, you can easily transfer files between devices on the same network or across different networks.
## Features
- **Secure File Transfer**: Provides RSA and AES encryption for secure data transmission.
- **Optional Encryption**: Enable or disable encryption for file transfer as per requirement.
- **NAT Traversal**: Supports connections between devices behind NATs.
- **Modular Design**: Easily integrable and customizable for various use cases.
- **Format Support**: Nectar2P supports all file formats.
## Installation
`Nectar2P` requires Python 3.6+ and depends on the `cryptography` library. Follow these steps to install the project:
```bash
# Download or clone the project
# Install Nectar2P
pip install Nectar2P
```
## Usage
### Overview
`Nectar2P` provides two main classes for P2P file transfer:
- **NectarSender**: Used for sending files.
- **NectarReceiver**: Used for receiving files.
These classes support secure file transfer with optional encryption and NAT traversal.
### Basic Usage
#### File Sending (Sender)
```python
from nectar2p.nectar_sender import NectarSender
def main():
receiver_host = "public.receiver.ip"
receiver_port = 5000
sender = NectarSender(receiver_host, receiver_port, enable_encryption=True)
try:
sender.initiate_secure_connection()
sender.send_file("path/to/your/file.txt")
finally:
sender.close_connection()
if __name__ == "__main__":
main()
```
#### File Receiving (Receiver)
```python
from nectar2p.nectar_receiver import NectarReceiver
def main():
host = "0.0.0.0" # Allows connection from any IP
port = 5000
receiver = NectarReceiver(host, port, enable_encryption=True)
try:
receiver.wait_for_sender()
receiver.receive_file("path/to/save/file.txt")
finally:
receiver.close_connection()
if __name__ == "__main__":
main()
```
### Using NAT Traversal for Cross-Network Transfers
The `NectarSender` and `NectarReceiver` classes use a STUN server for NAT traversal, allowing direct connections between devices on different networks. Public IP addresses are automatically retrieved through the STUN server.
### Enabling/Disabling Encryption
Encryption can be optionally enabled or disabled during file transfer. When `enable_encryption` is set to `True`, RSA and AES encryption are used. When set to `False`, files are transferred without encryption.
```python
# Encryption enabled
sender = NectarSender("receiver_ip", 5000, enable_encryption=True)
# Encryption disabled
receiver = NectarReceiver("0.0.0.0", 5000, enable_encryption=False)
```
## Project Structure
Explanation of main files and folders used in the project:
```
nectar2p/
├── nectar2p/
│ ├── __init__.py # Main package file
│ ├── nectar_sender.py # Class managing file sending operations
│ ├── nectar_receiver.py # Class managing file receiving operations
│ ├── encryption/
│ │ ├── __init__.py # Encryption module
│ │ ├── rsa_handler.py # RSA operations
│ │ └── aes_handler.py # AES operations
│ ├── networking/
│ │ ├── __init__.py # Networking module
│ │ ├── connection.py # Connection operations
│ │ └── nat_traversal.py # NAT traversal operations
├── setup.py # Setup file
└── README.md # Project overview and instructions
```
## License
This project is licensed under the MIT License. See the `LICENSE` file for more details.
## Contributing
Contributions are welcome! Feel free to submit `pull requests` or open `issues` on GitHub for any bugs, suggestions, or improvements.
## Contact
For any questions or suggestions, please feel free to reach out: [glimor@proton.me](mailto:glimor@proton.me)
## Support the Project
[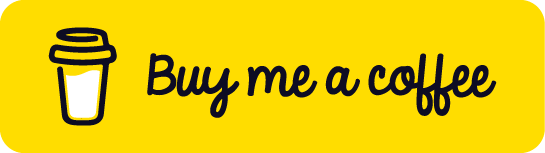](https://www.buymeacoffee.com/glimor)
Raw data
{
"_id": null,
"home_page": "https://github.com/Glimor/Nectar2P",
"name": "Nectar2P",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": null,
"keywords": "p2p file transfer, secure file transfer, encryption, NAT traversal",
"author": "Glimor",
"author_email": "glimor@proton.me",
"download_url": "https://files.pythonhosted.org/packages/e8/a7/144f26cede741c6f5121bf4fcc0f5a5103842a53700c5d527fe292daa6e0/nectar2p-1.0.1.tar.gz",
"platform": null,
"description": "\r\n# Nectar2P\r\n\r\n**Nectar2P** \u00e2\u20ac\u201d A secure and fast open-source Python library for P2P file transfers, featuring optional encryption and NAT traversal support. With Nectar2P, you can easily transfer files between devices on the same network or across different networks.\r\n\r\n## Features\r\n\r\n- **Secure File Transfer**: Provides RSA and AES encryption for secure data transmission.\r\n- **Optional Encryption**: Enable or disable encryption for file transfer as per requirement.\r\n- **NAT Traversal**: Supports connections between devices behind NATs.\r\n- **Modular Design**: Easily integrable and customizable for various use cases.\r\n- **Format Support**: Nectar2P supports all file formats.\r\n\r\n## Installation\r\n\r\n`Nectar2P` requires Python 3.6+ and depends on the `cryptography` library. Follow these steps to install the project:\r\n\r\n```bash\r\n# Download or clone the project\r\n# Install Nectar2P\r\npip install Nectar2P\r\n```\r\n\r\n## Usage\r\n\r\n### Overview\r\n\r\n`Nectar2P` provides two main classes for P2P file transfer:\r\n- **NectarSender**: Used for sending files.\r\n- **NectarReceiver**: Used for receiving files.\r\n\r\nThese classes support secure file transfer with optional encryption and NAT traversal.\r\n\r\n### Basic Usage\r\n\r\n#### File Sending (Sender)\r\n\r\n```python\r\nfrom nectar2p.nectar_sender import NectarSender\r\n\r\ndef main():\r\n receiver_host = \"public.receiver.ip\"\r\n receiver_port = 5000\r\n sender = NectarSender(receiver_host, receiver_port, enable_encryption=True)\r\n\r\n try:\r\n sender.initiate_secure_connection()\r\n sender.send_file(\"path/to/your/file.txt\")\r\n finally:\r\n sender.close_connection()\r\n\r\nif __name__ == \"__main__\":\r\n main()\r\n```\r\n\r\n#### File Receiving (Receiver)\r\n\r\n```python\r\nfrom nectar2p.nectar_receiver import NectarReceiver\r\n\r\ndef main():\r\n host = \"0.0.0.0\" # Allows connection from any IP\r\n port = 5000\r\n receiver = NectarReceiver(host, port, enable_encryption=True)\r\n\r\n try:\r\n receiver.wait_for_sender()\r\n receiver.receive_file(\"path/to/save/file.txt\")\r\n finally:\r\n receiver.close_connection()\r\n\r\nif __name__ == \"__main__\":\r\n main()\r\n```\r\n\r\n### Using NAT Traversal for Cross-Network Transfers\r\n\r\nThe `NectarSender` and `NectarReceiver` classes use a STUN server for NAT traversal, allowing direct connections between devices on different networks. Public IP addresses are automatically retrieved through the STUN server.\r\n\r\n### Enabling/Disabling Encryption\r\n\r\nEncryption can be optionally enabled or disabled during file transfer. When `enable_encryption` is set to `True`, RSA and AES encryption are used. When set to `False`, files are transferred without encryption.\r\n\r\n```python\r\n# Encryption enabled\r\nsender = NectarSender(\"receiver_ip\", 5000, enable_encryption=True)\r\n\r\n# Encryption disabled\r\nreceiver = NectarReceiver(\"0.0.0.0\", 5000, enable_encryption=False)\r\n```\r\n\r\n## Project Structure\r\n\r\nExplanation of main files and folders used in the project:\r\n\r\n```\r\nnectar2p/\r\n\u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac nectar2p/\r\n\u00e2\u201d\u201a \u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac __init__.py # Main package file\r\n\u00e2\u201d\u201a \u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac nectar_sender.py # Class managing file sending operations\r\n\u00e2\u201d\u201a \u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac nectar_receiver.py # Class managing file receiving operations\r\n\u00e2\u201d\u201a \u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac encryption/\r\n\u00e2\u201d\u201a \u00e2\u201d\u201a \u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac __init__.py # Encryption module\r\n\u00e2\u201d\u201a \u00e2\u201d\u201a \u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac rsa_handler.py # RSA operations\r\n\u00e2\u201d\u201a \u00e2\u201d\u201a \u00e2\u201d\u201d\u00e2\u201d\u20ac\u00e2\u201d\u20ac aes_handler.py # AES operations\r\n\u00e2\u201d\u201a \u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac networking/\r\n\u00e2\u201d\u201a \u00e2\u201d\u201a \u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac __init__.py # Networking module\r\n\u00e2\u201d\u201a \u00e2\u201d\u201a \u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac connection.py # Connection operations\r\n\u00e2\u201d\u201a \u00e2\u201d\u201a \u00e2\u201d\u201d\u00e2\u201d\u20ac\u00e2\u201d\u20ac nat_traversal.py # NAT traversal operations\r\n\u00e2\u201d\u0153\u00e2\u201d\u20ac\u00e2\u201d\u20ac setup.py # Setup file\r\n\u00e2\u201d\u201d\u00e2\u201d\u20ac\u00e2\u201d\u20ac README.md # Project overview and instructions\r\n```\r\n\r\n## License\r\n\r\nThis project is licensed under the MIT License. See the `LICENSE` file for more details.\r\n\r\n## Contributing\r\n\r\nContributions are welcome! Feel free to submit `pull requests` or open `issues` on GitHub for any bugs, suggestions, or improvements.\r\n\r\n## Contact\r\n\r\nFor any questions or suggestions, please feel free to reach out: [glimor@proton.me](mailto:glimor@proton.me)\r\n\r\n## Support the Project\r\n\r\n[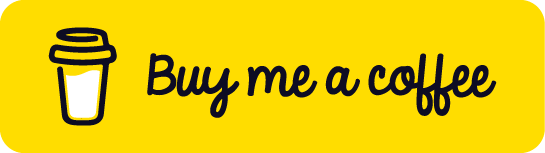](https://www.buymeacoffee.com/glimor)\r\n",
"bugtrack_url": null,
"license": null,
"summary": "A secure P2P file transfer library with optional encryption and NAT traversal support",
"version": "1.0.1",
"project_urls": {
"Homepage": "https://github.com/Glimor/Nectar2P"
},
"split_keywords": [
"p2p file transfer",
" secure file transfer",
" encryption",
" nat traversal"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "bed78a40f3ad2b69dc97a8f4327453ef6a2ea2a7dfad7c065cecbea25da2a592",
"md5": "88e4a335cc971204aa7021859bcf9247",
"sha256": "89612483b2e2143c2c54c4cd8b597d7f94d931c8a5efb792fcaaaff8f5af4e7d"
},
"downloads": -1,
"filename": "Nectar2P-1.0.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "88e4a335cc971204aa7021859bcf9247",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 8996,
"upload_time": "2024-10-31T20:04:21",
"upload_time_iso_8601": "2024-10-31T20:04:21.775476Z",
"url": "https://files.pythonhosted.org/packages/be/d7/8a40f3ad2b69dc97a8f4327453ef6a2ea2a7dfad7c065cecbea25da2a592/Nectar2P-1.0.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "e8a7144f26cede741c6f5121bf4fcc0f5a5103842a53700c5d527fe292daa6e0",
"md5": "936d07cb674edeac340130e3b0c6ad3e",
"sha256": "d0a3271f0a51828ba3ee2c9e68c6ffda01620869e54deac5f842335d9954164b"
},
"downloads": -1,
"filename": "nectar2p-1.0.1.tar.gz",
"has_sig": false,
"md5_digest": "936d07cb674edeac340130e3b0c6ad3e",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 7276,
"upload_time": "2024-10-31T20:04:24",
"upload_time_iso_8601": "2024-10-31T20:04:24.408582Z",
"url": "https://files.pythonhosted.org/packages/e8/a7/144f26cede741c6f5121bf4fcc0f5a5103842a53700c5d527fe292daa6e0/nectar2p-1.0.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-31 20:04:24",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Glimor",
"github_project": "Nectar2P",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "nectar2p"
}