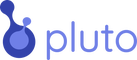
# Pluto Python SDK: `py-pluto`
A Python Software Development Kit (SDK) for interacting with the [Pluto Bio](https://pluto.bio), a cloud computational biology platform.
Other resources:
- [Pluto.bio](https://pluto.bio)
- [Pluto R Package](https://github.com/pluto-biosciences/pluto-sdk-R).
- [Pluto API](https://docs.pluto.bio)
## Table of Contents
- [Installation](#installation)
- [Using pip](#using-pip)
- [Local Installation](#local-installation)
- [Configuration](#configuration)
- [Usage](#usage)
- [Initialize the Client](#initialize-the-client)
- [Experiment Operations](#experiment-operations)
- [Sample and Assay Data](#sample-and-assay-data)
- [Plot Operations](#plot-operations)
- [Attachment Operations](#attachment-operations)
- [Examples](#examples)
- [Uploading a plot to Pluto Experiment using plotly](#example--uploading-a-plot-to-pluto-experiment-using-plotly)
- [Update an already existing plot in an experiment](#example--update-an-already-existing-plot-in-an-experiment)
- [List your experiments](#example--list-your-experiments)
- [List attachments in an experiment](#example--list-attachments-in-an-experiment)
- [Development](#development)
- [Build Distribution Package](#build-distribution-package)
- [Install from Source](#install-from-source)
- [Help & Support](#help-and-support)
## Installation
### Using pip
To install the SDK using pip, run the following command:
```bash
pip install PyPluto
```
### Local Installation
For a local setup, you can use a Python virtual environment.
```bash
python -m venv .venv
source .venv/bin/activate
pip install -r requirements.txt
```
## Configuration
Create an API key and set the environment variables to interact with the Pluto Bio platform. You can find your api token in the Pluto platform or visit [this help article](https://help.pluto.bio/en/articles/creating-your-api-token).
```bash
export PLUTO_API_URL=<API_URL>
export API_TOKEN=<API_TOKEN>
```
## Usage
### Initialize the Client
To start interacting with the Pluto Bio API, initialize the client with your API token.
```python
from plutobio import PlutoClient
pluto_bio = PlutoClient(token="YOUR_PLUTO_API_TOKEN")
```
### Experiment Operations
#### List Experiments
```python
experiments = pluto_bio.list_experiments()
```
#### Get a Specific Experiment
```python
experiment = pluto_bio.get_experiment("EXPERIMENT_UUID")
```
### Sample and Assay Data
#### Get Sample Data
```python
sample_data = pluto_bio.get_sample_data("EXPERIMENT_UUID", folder_path="data")
```
#### Get Assay Data
```python
assay_data = pluto_bio.get_assay_data("EXPERIMENT_UUID", folder_path="data")
```
### Plot Operations
#### List Plots
```python
plots = pluto_bio.list_plots("EXPERIMENT_UUID")
```
#### Get Data from a Plot
```python
plot_data = pluto_bio.get_plot_data("EXPERIMENT_UUID", "PLOT_UUID")
```
### Attachment Operations
#### List Attachments
```python
attachments = pluto_bio.list_attachments("EXPERIMENT_UUID")
```
#### Download Attachments
```python
attachment = pluto_bio.download_attachments("EXPERIMENT_UUID", "ATTACHMENT_UUID", folder_path="data")
```
#### Download QC report
```python
qc_report = pluto_bio.download_qc_report("PLX128193", folder_path="data")
```
#### Download BAM files
```python
bam_files = pluto_bio.download_bam_files("PLX128193", _bam_uuid, folder_path="data")
```
_Note: File will show up locally in your machine in a folder called data in the same directory where you ran the script_
## Examples
### Example : Uploading a plot to Pluto Experiment using plotly
```python
import plotly.graph_objects as go
from plotly.offline import plot
# Let's say we have the following plot
fruits = ["Apples", "Bananas", "Cherries", "Dates"]
quantities = [
10,
20,
15,
7,
]
# Create a bar plot
fig = go.Figure(
data=[
go.Bar(
x=fruits,
y=quantities,
marker_color="red"
)
]
)
# Customize the layout
fig.update_layout(
title="Fruit Quantities",
xaxis=dict(title="Fruit"),
yaxis=dict(title="Quantity"),
paper_bgcolor="rgba(0,0,0,0)",
plot_bgcolor="rgba(0,0,0,0)",
)
plot(fig, filename="bar_plot.html", auto_open=False)
pc.create_or_update_plot(
_experiment_uuid,
file_path="bar_plot.html",
)
```
### Example : Update an already existing plot in an experiment
To update an existing plot in your experiment, you will need the figure uuid. To find out the uuid click on the 3 dots in the figure, and select the option methods. The uuid that you want is under plot analysis ID.
```python
import plotly.graph_objects as go
from plotly.offline import plot
# Let's say we have the following plot
fruits = ["Apples", "Bananas", "Cherries", "Dates"]
quantities = [
10,
20,
15,
7,
]
# Create a bar plot
fig = go.Figure(
data=[
go.Bar(
x=fruits,
y=quantities,
marker_color="red"
)
]
)
# Customize the layout
fig.update_layout(
title="Fruit Quantities",
xaxis=dict(title="Fruit"),
yaxis=dict(title="Quantity"),
paper_bgcolor="rgba(0,0,0,0)",
plot_bgcolor="rgba(0,0,0,0)",
)
plot(fig, filename="bar_plot.html", auto_open=False)
pc.create_or_update_plot(
_experiment_uuid,
file_path="bar_plot.html",
plot_uuid=<PLOT_UUID> # example "6511b617-9a0e-4a61-bf30-a1f1eea7a2a4",
)
```
### Example : List your experiments
```python
from plutobio import PlutoClient
pluto_bio = PlutoClient(token="YOUR_PLUTO_API_TOKEN")
experiments = pluto_bio.list_experiments()
experiments = pluto_bio.list_experiments()
for experiment in experiments:
print(f"Pluto ID: {experiment} | Name: {experiments[experiment].name}")
>>>> Pluto ID: PLX128193 | Name: Novel therapeutics inhibiting viral replication in SARS-CoV-2
>>>> Pluto ID: PLX122909 | Name: testing
>>>> Pluto ID: PLX004016 | Name: test-analysis-btn
>>>> Pluto ID: PLX176024 | Name: New test title
>>>> Pluto ID: PLX175808 | Name: Testing banners
>>>> Pluto ID: PLX169820 | Name: Iowa Test - Copy of Copy of PLX160374: Cut and Run TM ET H3K16Ac
>>>> Pluto ID: PLX174997 | Name: Copy of Iowa Test - Copy of Copy of PLX160374: Cut and Run TM ET H3K16Acdaf
```
### Example : List attachments in an experiment
```
from plutobio import PlutoClient
pluto_bio = PlutoClient(token="YOUR_PLUTO_API_TOKEN")
attachments = pluto_bio.list_attachments("PLX128193")
for attachment in attachments:
print(f"Filename: {attachment.filename} | UUID: {attachment.uuid}")
>>>> Filename: SRR4238351_subsamp.fastq.gz | UUID: 634cd346-4674-4f3c-97ea-d17cf146453b
```
---
## Development
### Build Distribution Package
To build a distribution package, navigate to the project's root directory and run:
```bash
python setup.py sdist bdist_wheel
```
### Install from Source
It's recommended to use a virtual environment to install the Pluto SDK package from source.
```bash
python -m venv .venv
source .venv/bin/activate
pip install git+ssh://github.com/pluto-biosciences/py-pluto.git@VERSION_TAG
```
## Help and Support
For additional assistance please visit our [knowledge base](https://help.pluto.bio) or submit a support request via the [help portal](https://help.pluto.bio).
Raw data
{
"_id": null,
"home_page": null,
"name": "PlutoBio",
"maintainer": null,
"docs_url": null,
"requires_python": "<4,>=3.10",
"maintainer_email": null,
"keywords": "PlutoBio, SDK, API, Biosciences, Pluto",
"author": "Pluto Team",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/48/73/1e2a5da7457dd7f2a24f95888b997f08d0edfb35c4b9f8aae96c315c69b7/plutobio-0.1.11.tar.gz",
"platform": null,
"description": "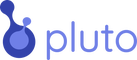\n\n# Pluto Python SDK: `py-pluto`\n\nA Python Software Development Kit (SDK) for interacting with the [Pluto Bio](https://pluto.bio), a cloud computational biology platform.\n\nOther resources:\n\n- [Pluto.bio](https://pluto.bio)\n- [Pluto R Package](https://github.com/pluto-biosciences/pluto-sdk-R).\n\n- [Pluto API](https://docs.pluto.bio)\n\n## Table of Contents\n\n- [Installation](#installation)\n - [Using pip](#using-pip)\n - [Local Installation](#local-installation)\n- [Configuration](#configuration)\n- [Usage](#usage)\n - [Initialize the Client](#initialize-the-client)\n - [Experiment Operations](#experiment-operations)\n - [Sample and Assay Data](#sample-and-assay-data)\n - [Plot Operations](#plot-operations)\n - [Attachment Operations](#attachment-operations)\n- [Examples](#examples)\n - [Uploading a plot to Pluto Experiment using plotly](#example--uploading-a-plot-to-pluto-experiment-using-plotly)\n - [Update an already existing plot in an experiment](#example--update-an-already-existing-plot-in-an-experiment)\n - [List your experiments](#example--list-your-experiments)\n - [List attachments in an experiment](#example--list-attachments-in-an-experiment)\n- [Development](#development)\n - [Build Distribution Package](#build-distribution-package)\n - [Install from Source](#install-from-source)\n- [Help & Support](#help-and-support)\n\n## Installation\n\n### Using pip\n\nTo install the SDK using pip, run the following command:\n\n```bash\npip install PyPluto\n```\n\n### Local Installation\n\nFor a local setup, you can use a Python virtual environment.\n\n```bash\npython -m venv .venv\nsource .venv/bin/activate\npip install -r requirements.txt\n```\n\n## Configuration\n\nCreate an API key and set the environment variables to interact with the Pluto Bio platform. You can find your api token in the Pluto platform or visit [this help article](https://help.pluto.bio/en/articles/creating-your-api-token).\n\n```bash\nexport PLUTO_API_URL=<API_URL>\nexport API_TOKEN=<API_TOKEN>\n```\n\n## Usage\n\n### Initialize the Client\n\nTo start interacting with the Pluto Bio API, initialize the client with your API token.\n\n```python\nfrom plutobio import PlutoClient\n\npluto_bio = PlutoClient(token=\"YOUR_PLUTO_API_TOKEN\")\n```\n\n### Experiment Operations\n\n#### List Experiments\n\n```python\nexperiments = pluto_bio.list_experiments()\n```\n\n#### Get a Specific Experiment\n\n```python\nexperiment = pluto_bio.get_experiment(\"EXPERIMENT_UUID\")\n```\n\n### Sample and Assay Data\n\n#### Get Sample Data\n\n```python\nsample_data = pluto_bio.get_sample_data(\"EXPERIMENT_UUID\", folder_path=\"data\")\n```\n\n#### Get Assay Data\n\n```python\nassay_data = pluto_bio.get_assay_data(\"EXPERIMENT_UUID\", folder_path=\"data\")\n```\n\n### Plot Operations\n\n#### List Plots\n\n```python\nplots = pluto_bio.list_plots(\"EXPERIMENT_UUID\")\n```\n\n#### Get Data from a Plot\n\n```python\nplot_data = pluto_bio.get_plot_data(\"EXPERIMENT_UUID\", \"PLOT_UUID\")\n```\n\n### Attachment Operations\n\n#### List Attachments\n\n```python\nattachments = pluto_bio.list_attachments(\"EXPERIMENT_UUID\")\n```\n\n#### Download Attachments\n\n```python\nattachment = pluto_bio.download_attachments(\"EXPERIMENT_UUID\", \"ATTACHMENT_UUID\", folder_path=\"data\")\n```\n\n#### Download QC report\n\n```python\nqc_report = pluto_bio.download_qc_report(\"PLX128193\", folder_path=\"data\")\n```\n\n#### Download BAM files\n\n```python\nbam_files = pluto_bio.download_bam_files(\"PLX128193\", _bam_uuid, folder_path=\"data\")\n```\n\n_Note: File will show up locally in your machine in a folder called data in the same directory where you ran the script_\n\n## Examples\n\n### Example : Uploading a plot to Pluto Experiment using plotly\n\n```python\nimport plotly.graph_objects as go\nfrom plotly.offline import plot\n\n# Let's say we have the following plot\n\nfruits = [\"Apples\", \"Bananas\", \"Cherries\", \"Dates\"]\nquantities = [\n 10,\n 20,\n 15,\n 7,\n]\n\n\n# Create a bar plot\nfig = go.Figure(\n data=[\n go.Bar(\n x=fruits,\n y=quantities,\n marker_color=\"red\"\n )\n ]\n)\n\n# Customize the layout\nfig.update_layout(\n title=\"Fruit Quantities\",\n xaxis=dict(title=\"Fruit\"),\n yaxis=dict(title=\"Quantity\"),\n paper_bgcolor=\"rgba(0,0,0,0)\",\n plot_bgcolor=\"rgba(0,0,0,0)\",\n)\n\nplot(fig, filename=\"bar_plot.html\", auto_open=False)\n\npc.create_or_update_plot(\n _experiment_uuid,\n file_path=\"bar_plot.html\",\n)\n\n\n```\n\n### Example : Update an already existing plot in an experiment\n\nTo update an existing plot in your experiment, you will need the figure uuid. To find out the uuid click on the 3 dots in the figure, and select the option methods. The uuid that you want is under plot analysis ID.\n\n```python\nimport plotly.graph_objects as go\nfrom plotly.offline import plot\n\n# Let's say we have the following plot\n\nfruits = [\"Apples\", \"Bananas\", \"Cherries\", \"Dates\"]\nquantities = [\n 10,\n 20,\n 15,\n 7,\n]\n\n\n# Create a bar plot\nfig = go.Figure(\n data=[\n go.Bar(\n x=fruits,\n y=quantities,\n marker_color=\"red\"\n )\n ]\n)\n\n# Customize the layout\nfig.update_layout(\n title=\"Fruit Quantities\",\n xaxis=dict(title=\"Fruit\"),\n yaxis=dict(title=\"Quantity\"),\n paper_bgcolor=\"rgba(0,0,0,0)\",\n plot_bgcolor=\"rgba(0,0,0,0)\",\n)\n\nplot(fig, filename=\"bar_plot.html\", auto_open=False)\n\npc.create_or_update_plot(\n _experiment_uuid,\n file_path=\"bar_plot.html\",\n plot_uuid=<PLOT_UUID> # example \"6511b617-9a0e-4a61-bf30-a1f1eea7a2a4\",\n)\n\n\n```\n\n### Example : List your experiments\n\n```python\nfrom plutobio import PlutoClient\npluto_bio = PlutoClient(token=\"YOUR_PLUTO_API_TOKEN\")\n\nexperiments = pluto_bio.list_experiments()\n\nexperiments = pluto_bio.list_experiments()\nfor experiment in experiments:\n print(f\"Pluto ID: {experiment} | Name: {experiments[experiment].name}\")\n\n\n>>>> Pluto ID: PLX128193 | Name: Novel therapeutics inhibiting viral replication in SARS-CoV-2\n>>>> Pluto ID: PLX122909 | Name: testing\n>>>> Pluto ID: PLX004016 | Name: test-analysis-btn\n>>>> Pluto ID: PLX176024 | Name: New test title\n>>>> Pluto ID: PLX175808 | Name: Testing banners\n>>>> Pluto ID: PLX169820 | Name: Iowa Test - Copy of Copy of PLX160374: Cut and Run TM ET H3K16Ac\n>>>> Pluto ID: PLX174997 | Name: Copy of Iowa Test - Copy of Copy of PLX160374: Cut and Run TM ET H3K16Acdaf\n```\n\n### Example : List attachments in an experiment\n\n```\nfrom plutobio import PlutoClient\npluto_bio = PlutoClient(token=\"YOUR_PLUTO_API_TOKEN\")\n\nattachments = pluto_bio.list_attachments(\"PLX128193\")\nfor attachment in attachments:\n print(f\"Filename: {attachment.filename} | UUID: {attachment.uuid}\")\n\n>>>> Filename: SRR4238351_subsamp.fastq.gz | UUID: 634cd346-4674-4f3c-97ea-d17cf146453b\n```\n\n---\n\n## Development\n\n### Build Distribution Package\n\nTo build a distribution package, navigate to the project's root directory and run:\n\n```bash\npython setup.py sdist bdist_wheel\n```\n\n### Install from Source\n\nIt's recommended to use a virtual environment to install the Pluto SDK package from source.\n\n```bash\npython -m venv .venv\nsource .venv/bin/activate\npip install git+ssh://github.com/pluto-biosciences/py-pluto.git@VERSION_TAG\n```\n\n## Help and Support\n\nFor additional assistance please visit our [knowledge base](https://help.pluto.bio) or submit a support request via the [help portal](https://help.pluto.bio).\n",
"bugtrack_url": null,
"license": null,
"summary": "A Python SDK for Pluto.bio",
"version": "0.1.11",
"project_urls": null,
"split_keywords": [
"plutobio",
" sdk",
" api",
" biosciences",
" pluto"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "e21f2d006576badec529f874a9b91b15573c9d15c6753a7dcc995e4966c00631",
"md5": "9e65c3a751931bc0fe596beba8e07963",
"sha256": "ebbed37e9e9688f4b3e9a5312a258be3050a5cc6174c6c7cfdf2d830b44ef18c"
},
"downloads": -1,
"filename": "PlutoBio-0.1.11-py3-none-any.whl",
"has_sig": false,
"md5_digest": "9e65c3a751931bc0fe596beba8e07963",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4,>=3.10",
"size": 19155,
"upload_time": "2024-04-29T19:11:35",
"upload_time_iso_8601": "2024-04-29T19:11:35.629857Z",
"url": "https://files.pythonhosted.org/packages/e2/1f/2d006576badec529f874a9b91b15573c9d15c6753a7dcc995e4966c00631/PlutoBio-0.1.11-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "48731e2a5da7457dd7f2a24f95888b997f08d0edfb35c4b9f8aae96c315c69b7",
"md5": "a89ec41db41165de6e1928950646d28d",
"sha256": "849bd265b83349228f71b55137571e5832634ec31116c599f6e3398f6ce98e64"
},
"downloads": -1,
"filename": "plutobio-0.1.11.tar.gz",
"has_sig": false,
"md5_digest": "a89ec41db41165de6e1928950646d28d",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4,>=3.10",
"size": 13838,
"upload_time": "2024-04-29T19:11:37",
"upload_time_iso_8601": "2024-04-29T19:11:37.659193Z",
"url": "https://files.pythonhosted.org/packages/48/73/1e2a5da7457dd7f2a24f95888b997f08d0edfb35c4b9f8aae96c315c69b7/plutobio-0.1.11.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-04-29 19:11:37",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "plutobio"
}