[//]: # (generated using SlashBack 0.2.0)
# PyGLM
## OpenGL Mathematics \(GLM\) library for Python
**GLSL \+ Optional features \+ Python = PyGLM**
**A mathematics library for graphics programming\.**
**PyGLM** is a Python extension written in **C\+\+**\.
By using [GLM by G\-Truc](https://glm.g-truc.net) under the hood, it manages to bring **glm's features** to Python\.
Some features are unsupported \(such as most unstable extensions\)\.
If you encounter any issues or want to request a feature, please create an issue on the [issue tracker](https://github.com/Zuzu-Typ/PyGLM/issues)\.
**For a complete reference of the types and functions, please take a look at the [wiki](https://github.com/Zuzu-Typ/PyGLM/wiki)**\.
## Tiny Documentation
### Why PyGLM?
Besides the obvious \- being mostly compatible with **GLM** \- PyGLM offers a variety of features for **vector** and **matrix manipulation**\.
It has a lot of possible use cases, including **3D\-Graphics** \(OpenGL, DirectX, \.\.\.\), **Physics** and more\.
At the same time, it has **great performance**, usually being **a lot faster than numpy\!** \(see [end of page](#speed-comparison-to-numpy)\)
\(*depending on the individual function*\)
### Installation
**PyGLM** supports **Windows**, **Linux**, **MacOS** and other operating systems with either x86 \(**32\-bit**\) or x64 \(**64\-bit**\) architecture,
running **Python 3**\.5 or higher\. \(Prior versions of Python \- such as Python 2 \- were supported up to PyGLM version 0\.4\.8b1\)
It can be installed from the [PyPI](https://pypi.python.org/pypi/PyGLM) using [pip](https://pip.pypa.io/en/stable/):
``` batch
pip install PyGLM
# please make sure to install "PyGLM" and not "glm", which is a different module
```
And finally imported and used:
``` python
import glm
```
### Using PyGLM
PyGLM's syntax is very similar to the original GLM's syntax\.
There is no need to import anything but **glm**, as it already contains the entire package\.
For more information, take a look at the [wiki](https://github.com/Zuzu-Typ/PyGLM/wiki)\.
#### License requirements
Please make sure to **include the license for GLM** in your project when you use PyGLM\!
\(this is especially relevant for **binary distributions**, e\.g\. \*\.exe\)
You can do so by copying the ``` COPYING ``` file \(or it's contents\) to your project\.
#### Differences to glm
Instead of using double colons \(**::**\) for namespaces, periods \(**\.**\) are used, so
``` glm::vec2 ``` becomes ``` glm.vec2 ```\.
PyGLM supports the [buffer protocol](https://docs.python.org/3/c-api/buffer.html), meaning its compitible to other objects that support the buffer protocol,
such as ``` bytes ``` or ``` numpy.array ```
\(for example you can convert a glm matrix to a numpy array and vice versa\)\.
PyGLM is also capable of interpreting iterables \(such as tuples\) as vectors, so e\.g\. the following equasion is possible:
``` python
result = glm.vec2(1) * (2, 3)
```
*Note: This feature might not or only partially be available in PyGLM versions prior to 2\.0\.0*
PyGLM doesn't support precision qualifiers\. All types use the default precision \(``` packed_highp ```\)\.
If a glm function normally accepts ``` float ``` and ``` double ``` arguments, the higher precision \(``` double ```\) is used\.
There is no way to set preprocessor definitions \(macros\)\.
If \- for example \- you need to use the left handed coordinate system, you have to use **\*LH**, so
``` glm.perspective ``` becomes ``` glm.perspectiveLH ```\.
All types are initialized by default to avoid memory access violations\.
\(i\.e\. the macro ``` GLM_FORCE_CTOR_INIT ``` is defined\)
In case you need the size of a PyGLM datatype, you can use
``` python
glm.sizeof(<type>)
```
The function ``` glm.identity ``` requires a matrix type as it's argument\.
The function ``` glm.frexp(x, exp) ``` returns a tuple ``` (m, e) ```, if the input arguments are numerical\.
This function may issue a ``` UserWarning ```\. You can silence this warning using ``` glm.silence(1) ```\.
The function ``` glm.value_ptr(x) ``` returns a ctypes pointer of the respective type\.
I\.e\. if the datatype of ``` x ``` is ``` float ```, then a ``` c_float ``` pointer will be returned\.
Likewise the reverse\-functions \(such as ``` make_vec2(ptr) ```\) will take a ctypes pointer as their argument
and return \(in this case\) a 2 component vector of the pointers underlying type\.
``` glm.silence(ID) ``` can be used to silence specific warnings\.
Supplying an id of 0 will silence all warnings\.
### FAQ
#### How to pass the matrices generated by PyGLM to OpenGL functions?
You will find an overview on the \[[Passing data to external libs](https://github.com/Zuzu-Typ/PyGLM/wiki/Passing-data-to-external-libs/)\] page\.
#### Types and functions are not available after installing from the PyPI using ``` pip install glm ```
Most likely you've installed [glm](https://pypi.org/project/glm/), a JSON parser and not [PyGLM](https://pypi.org/project/PyGLM/) \(or a very early version of PyGLM\)\.
The correct install command is:
```
pip install PyGLM
```
#### Why is *<experimental extension name here>* not supported?
I prefer not to add too many experimental extensions to PyGLM, especially as they might change or be removed in the future and it is simply too much effort for me to keep up with all that\.
If you **need a specific experimental extension**, feel free to **submit a feature request** on the [issue tracker](https://github.com/Zuzu-Typ/PyGLM/issues)\.
I try adding them on a one\-by\-one basis\.
#### Why are Python versions prior to 3\.5 no longer supported?
Starting with version [0\.5\.0b1](https://github.com/Zuzu-Typ/PyGLM/releases/tag/0.5.0b1) I decided to use C\+\+ to build PyGLM, using [glm](https://glm.g-truc.net/) under the hood \- which requires C\+\+ 11 or upwards\.
Only Python versions 3\.5\+ support C\+\+ 11, thus I was forced to stop supporting older versions\.
The last version to support Python 2 and <3\.5 is [0\.4\.8b1](https://github.com/Zuzu-Typ/PyGLM/releases/tag/0.4.8b1)\.
### Short example
``` Python
>>> import glm
>>> v = glm.vec3()
>>> v.x = 7
>>> print(v.xxy)
vec3( 7, 7, 0 )
>>> m = glm.mat4()
>>> print(m)
[ 1 | 0 | 0 | 0 ]
[ 0 | 1 | 0 | 0 ]
[ 0 | 0 | 1 | 0 ]
[ 0 | 0 | 0 | 1 ]
>>> v = glm.vec4(1, 2, 3, 4)
>>> print(v + (8, 7, 6, 5))
vec4( 9, 9, 9, 9 )
```
### PyGLM in action
Wanna see what PyGLM can do?
Take a look at the [examples](https://github.com/Zuzu-Typ/LearnOpenGL-Python) from the popular LearnOpenGL tutorials by Joey De Vries running in Python using PyGLM\.
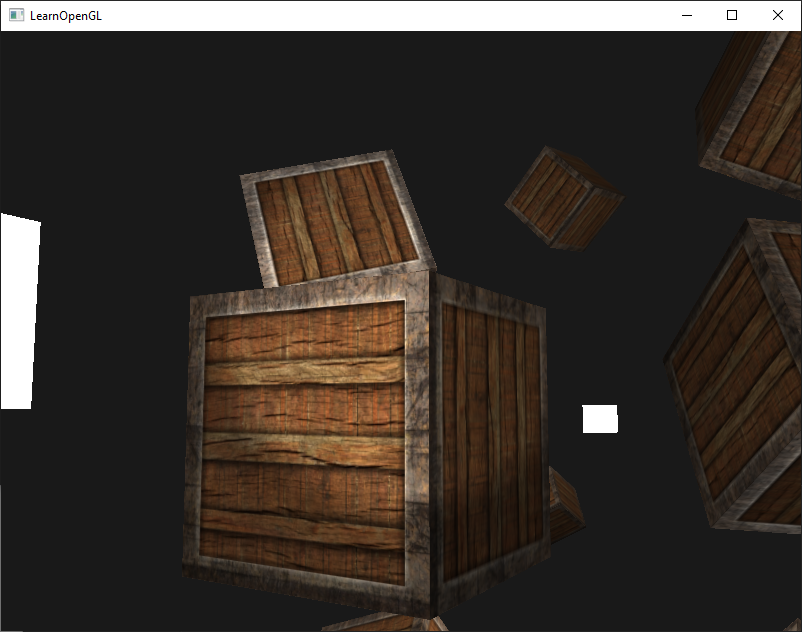
### Speed comparison to numpy
The following is the output generated by [test/PyGLM vs Numpy\.py](https://github.com/Zuzu-Typ/PyGLM/blob/master/test/PyGLM%20vs%20NumPy.py)
```
Evaluating performance of PyGLM compared to NumPy.
Running on platform 'win32'.
Python version:
3.10.0 (tags/v3.10.0:b494f59, Oct 4 2021, 19:00:18) [MSC v.1929 64 bit (AMD64)]
Comparing the following module versions:
PyGLM (DEFAULT) version 2.5.2
vs
NumPy version 1.21.3
________________________________________________________________________________
The following table shows information about a task to be achieved and the time
it took when using the given module. Lower time is better.
Each task is repeated ten times per module, only showing the best (i.e. lowest)
value.
+----------------------------------------+------------+------------+-----------+
| Description | PyGLM time | NumPy time | ratio |
+----------------------------------------+------------+------------+-----------+
| 3 component vector creation | | | |
| (100,000 times) | 8ms | 21ms | 2.69x |
+----------------------------------------+------------+------------+-----------+
| 3 component vector creation with | | | |
| custom components | | | |
| (50,000 times) | 8ms | 34ms | 4.32x |
+----------------------------------------+------------+------------+-----------+
| dot product | | | |
| (50,000 times) | 4ms | 49ms | 10.93x |
+----------------------------------------+------------+------------+-----------+
| cross product | | | |
| (25,000 times) | 2ms | 548ms | 234.34x |
+----------------------------------------+------------+------------+-----------+
| L2-Norm of 3 component vector | | | |
| (100,000 times) | 7ms | 310ms | 44.49x |
+----------------------------------------+------------+------------+-----------+
| 4x4 matrix creation | | | |
| (50,000 times) | 5ms | 11ms | 2.32x |
+----------------------------------------+------------+------------+-----------+
| 4x4 identity matrix creation | | | |
| (100,000 times) | 7ms | 176ms | 24.05x |
+----------------------------------------+------------+------------+-----------+
| 4x4 matrix transposition | | | |
| (50,000 times) | 5ms | 32ms | 6.19x |
+----------------------------------------+------------+------------+-----------+
| 4x4 multiplicative inverse | | | |
| (50,000 times) | 4ms | 1925ms | 470.77x |
+----------------------------------------+------------+------------+-----------+
| 3 component vector addition | | | |
| (100,000 times) | 5ms | 38ms | 7.17x |
+----------------------------------------+------------+------------+-----------+
| 4x4 matrix multiplication | | | |
| (100,000 times) | 7ms | 39ms | 5.36x |
+----------------------------------------+------------+------------+-----------+
| 4x4 matrix x vector multiplication | | | |
| (100,000 times) | 6ms | 116ms | 20.01x |
+----------------------------------------+------------+------------+-----------+
| TOTAL | 0.07s | 3.30s | 47.78x |
+----------------------------------------+------------+------------+-----------+
```
Raw data
{
"_id": null,
"home_page": "https://github.com/Zuzu-Typ/PyGLM",
"name": "PyGLM",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "GLM OpenGL matrix vector vec mat Mathematics 3D python python3 3 library python-c-api c-api math-library numpy pyrr pip pypi matrix-manipulation matrix-multiplication matrix-functions quaternion c glsl",
"author": "Zuzu_Typ",
"author_email": "zuzu.typ@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/59/25/c2ff0c5610b8146bbc4e1d85a2c07a2727769aa76b0b56a112f038778f89/pyglm-2.7.2.tar.gz",
"platform": "Windows",
"description": "[//]: # (generated using SlashBack 0.2.0)\n\n# PyGLM \n## OpenGL Mathematics \\(GLM\\) library for Python \n**GLSL \\+ Optional features \\+ Python = PyGLM** \n**A mathematics library for graphics programming\\.** \n \n**PyGLM** is a Python extension written in **C\\+\\+**\\. \nBy using [GLM by G\\-Truc](https://glm.g-truc.net) under the hood, it manages to bring **glm's features** to Python\\. \nSome features are unsupported \\(such as most unstable extensions\\)\\. \nIf you encounter any issues or want to request a feature, please create an issue on the [issue tracker](https://github.com/Zuzu-Typ/PyGLM/issues)\\. \n \n**For a complete reference of the types and functions, please take a look at the [wiki](https://github.com/Zuzu-Typ/PyGLM/wiki)**\\. \n \n## Tiny Documentation \n### Why PyGLM? \nBesides the obvious \\- being mostly compatible with **GLM** \\- PyGLM offers a variety of features for **vector** and **matrix manipulation**\\. \nIt has a lot of possible use cases, including **3D\\-Graphics** \\(OpenGL, DirectX, \\.\\.\\.\\), **Physics** and more\\. \n \nAt the same time, it has **great performance**, usually being **a lot faster than numpy\\!** \\(see [end of page](#speed-comparison-to-numpy)\\) \n\\(*depending on the individual function*\\) \n### Installation \n**PyGLM** supports **Windows**, **Linux**, **MacOS** and other operating systems with either x86 \\(**32\\-bit**\\) or x64 \\(**64\\-bit**\\) architecture, \nrunning **Python 3**\\.5 or higher\\. \\(Prior versions of Python \\- such as Python 2 \\- were supported up to PyGLM version 0\\.4\\.8b1\\) \n \nIt can be installed from the [PyPI](https://pypi.python.org/pypi/PyGLM) using [pip](https://pip.pypa.io/en/stable/): \n``` batch\npip install PyGLM\n# please make sure to install \"PyGLM\" and not \"glm\", which is a different module\n ``` \nAnd finally imported and used: \n``` python\nimport glm\n ``` \n### Using PyGLM \nPyGLM's syntax is very similar to the original GLM's syntax\\. \nThere is no need to import anything but **glm**, as it already contains the entire package\\. \n \nFor more information, take a look at the [wiki](https://github.com/Zuzu-Typ/PyGLM/wiki)\\. \n#### License requirements \nPlease make sure to **include the license for GLM** in your project when you use PyGLM\\! \n\\(this is especially relevant for **binary distributions**, e\\.g\\. \\*\\.exe\\) \n \nYou can do so by copying the ``` COPYING ``` file \\(or it's contents\\) to your project\\. \n#### Differences to glm \nInstead of using double colons \\(**::**\\) for namespaces, periods \\(**\\.**\\) are used, so \n``` glm::vec2 ``` becomes ``` glm.vec2 ```\\. \n \nPyGLM supports the [buffer protocol](https://docs.python.org/3/c-api/buffer.html), meaning its compitible to other objects that support the buffer protocol, \nsuch as ``` bytes ``` or ``` numpy.array ``` \n\\(for example you can convert a glm matrix to a numpy array and vice versa\\)\\. \nPyGLM is also capable of interpreting iterables \\(such as tuples\\) as vectors, so e\\.g\\. the following equasion is possible: \n``` python\nresult = glm.vec2(1) * (2, 3)\n ``` \n*Note: This feature might not or only partially be available in PyGLM versions prior to 2\\.0\\.0* \n \nPyGLM doesn't support precision qualifiers\\. All types use the default precision \\(``` packed_highp ```\\)\\. \n \nIf a glm function normally accepts ``` float ``` and ``` double ``` arguments, the higher precision \\(``` double ```\\) is used\\. \n \nThere is no way to set preprocessor definitions \\(macros\\)\\. \nIf \\- for example \\- you need to use the left handed coordinate system, you have to use **\\*LH**, so \n``` glm.perspective ``` becomes ``` glm.perspectiveLH ```\\. \n \nAll types are initialized by default to avoid memory access violations\\. \n\\(i\\.e\\. the macro ``` GLM_FORCE_CTOR_INIT ``` is defined\\) \n \nIn case you need the size of a PyGLM datatype, you can use \n``` python\nglm.sizeof(<type>)\n ``` \n \nThe function ``` glm.identity ``` requires a matrix type as it's argument\\. \n \nThe function ``` glm.frexp(x, exp) ``` returns a tuple ``` (m, e) ```, if the input arguments are numerical\\. \nThis function may issue a ``` UserWarning ```\\. You can silence this warning using ``` glm.silence(1) ```\\. \n \nThe function ``` glm.value_ptr(x) ``` returns a ctypes pointer of the respective type\\. \nI\\.e\\. if the datatype of ``` x ``` is ``` float ```, then a ``` c_float ``` pointer will be returned\\. \nLikewise the reverse\\-functions \\(such as ``` make_vec2(ptr) ```\\) will take a ctypes pointer as their argument \nand return \\(in this case\\) a 2 component vector of the pointers underlying type\\. \n \n``` glm.silence(ID) ``` can be used to silence specific warnings\\. \nSupplying an id of 0 will silence all warnings\\. \n \n \n### FAQ \n#### How to pass the matrices generated by PyGLM to OpenGL functions? \nYou will find an overview on the \\[[Passing data to external libs](https://github.com/Zuzu-Typ/PyGLM/wiki/Passing-data-to-external-libs/)\\] page\\. \n \n#### Types and functions are not available after installing from the PyPI using ``` pip install glm ``` \nMost likely you've installed [glm](https://pypi.org/project/glm/), a JSON parser and not [PyGLM](https://pypi.org/project/PyGLM/) \\(or a very early version of PyGLM\\)\\. \nThe correct install command is: \n``` \npip install PyGLM\n ``` \n \n#### Why is *<experimental extension name here>* not supported? \nI prefer not to add too many experimental extensions to PyGLM, especially as they might change or be removed in the future and it is simply too much effort for me to keep up with all that\\. \nIf you **need a specific experimental extension**, feel free to **submit a feature request** on the [issue tracker](https://github.com/Zuzu-Typ/PyGLM/issues)\\. \nI try adding them on a one\\-by\\-one basis\\. \n \n#### Why are Python versions prior to 3\\.5 no longer supported? \nStarting with version [0\\.5\\.0b1](https://github.com/Zuzu-Typ/PyGLM/releases/tag/0.5.0b1) I decided to use C\\+\\+ to build PyGLM, using [glm](https://glm.g-truc.net/) under the hood \\- which requires C\\+\\+ 11 or upwards\\. \nOnly Python versions 3\\.5\\+ support C\\+\\+ 11, thus I was forced to stop supporting older versions\\. \nThe last version to support Python 2 and <3\\.5 is [0\\.4\\.8b1](https://github.com/Zuzu-Typ/PyGLM/releases/tag/0.4.8b1)\\. \n \n### Short example \n``` Python\n>>> import glm\n>>> v = glm.vec3()\n>>> v.x = 7\n>>> print(v.xxy)\nvec3( 7, 7, 0 )\n\n>>> m = glm.mat4()\n>>> print(m)\n[ 1 | 0 | 0 | 0 ]\n[ 0 | 1 | 0 | 0 ]\n[ 0 | 0 | 1 | 0 ]\n[ 0 | 0 | 0 | 1 ]\n\n>>> v = glm.vec4(1, 2, 3, 4)\n>>> print(v + (8, 7, 6, 5))\nvec4( 9, 9, 9, 9 )\n ``` \n \n### PyGLM in action \nWanna see what PyGLM can do? \nTake a look at the [examples](https://github.com/Zuzu-Typ/LearnOpenGL-Python) from the popular LearnOpenGL tutorials by Joey De Vries running in Python using PyGLM\\. \n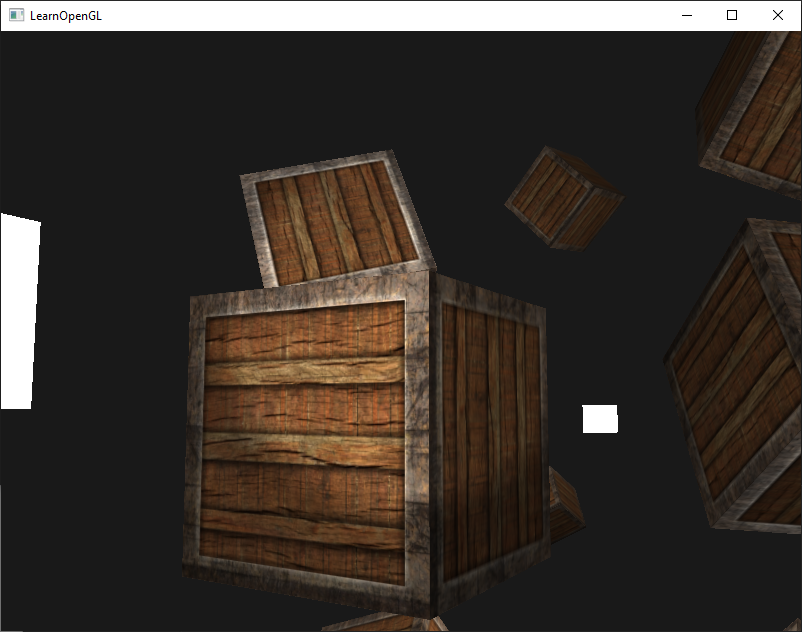 \n \n### Speed comparison to numpy \nThe following is the output generated by [test/PyGLM vs Numpy\\.py](https://github.com/Zuzu-Typ/PyGLM/blob/master/test/PyGLM%20vs%20NumPy.py) \n``` \nEvaluating performance of PyGLM compared to NumPy.\n\nRunning on platform 'win32'.\n\nPython version:\n3.10.0 (tags/v3.10.0:b494f59, Oct 4 2021, 19:00:18) [MSC v.1929 64 bit (AMD64)]\n\nComparing the following module versions:\nPyGLM (DEFAULT) version 2.5.2\n vs\nNumPy version 1.21.3\n________________________________________________________________________________\n\nThe following table shows information about a task to be achieved and the time\nit took when using the given module. Lower time is better.\nEach task is repeated ten times per module, only showing the best (i.e. lowest)\nvalue.\n\n\n+----------------------------------------+------------+------------+-----------+\n| Description | PyGLM time | NumPy time | ratio |\n+----------------------------------------+------------+------------+-----------+\n| 3 component vector creation | | | |\n| (100,000 times) | 8ms | 21ms | 2.69x |\n+----------------------------------------+------------+------------+-----------+\n| 3 component vector creation with | | | |\n| custom components | | | |\n| (50,000 times) | 8ms | 34ms | 4.32x |\n+----------------------------------------+------------+------------+-----------+\n| dot product | | | |\n| (50,000 times) | 4ms | 49ms | 10.93x |\n+----------------------------------------+------------+------------+-----------+\n| cross product | | | |\n| (25,000 times) | 2ms | 548ms | 234.34x |\n+----------------------------------------+------------+------------+-----------+\n| L2-Norm of 3 component vector | | | |\n| (100,000 times) | 7ms | 310ms | 44.49x |\n+----------------------------------------+------------+------------+-----------+\n| 4x4 matrix creation | | | |\n| (50,000 times) | 5ms | 11ms | 2.32x |\n+----------------------------------------+------------+------------+-----------+\n| 4x4 identity matrix creation | | | |\n| (100,000 times) | 7ms | 176ms | 24.05x |\n+----------------------------------------+------------+------------+-----------+\n| 4x4 matrix transposition | | | |\n| (50,000 times) | 5ms | 32ms | 6.19x |\n+----------------------------------------+------------+------------+-----------+\n| 4x4 multiplicative inverse | | | |\n| (50,000 times) | 4ms | 1925ms | 470.77x |\n+----------------------------------------+------------+------------+-----------+\n| 3 component vector addition | | | |\n| (100,000 times) | 5ms | 38ms | 7.17x |\n+----------------------------------------+------------+------------+-----------+\n| 4x4 matrix multiplication | | | |\n| (100,000 times) | 7ms | 39ms | 5.36x |\n+----------------------------------------+------------+------------+-----------+\n| 4x4 matrix x vector multiplication | | | |\n| (100,000 times) | 6ms | 116ms | 20.01x |\n+----------------------------------------+------------+------------+-----------+\n| TOTAL | 0.07s | 3.30s | 47.78x |\n+----------------------------------------+------------+------------+-----------+\n ```\n",
"bugtrack_url": null,
"license": "zlib/libpng license",
"summary": "OpenGL Mathematics library for Python",
"version": "2.7.2",
"project_urls": {
"Homepage": "https://github.com/Zuzu-Typ/PyGLM"
},
"split_keywords": [
"glm",
"opengl",
"matrix",
"vector",
"vec",
"mat",
"mathematics",
"3d",
"python",
"python3",
"3",
"library",
"python-c-api",
"c-api",
"math-library",
"numpy",
"pyrr",
"pip",
"pypi",
"matrix-manipulation",
"matrix-multiplication",
"matrix-functions",
"quaternion",
"c",
"glsl"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "4d171d818ee38c7b0dfa9b115bdf74f6c6c79869062f3a05b227c3c675ba56a7",
"md5": "c423634f994188adf78efdb956d7d7d3",
"sha256": "8bb24e75770a37783a147a4d5f0fba4af1ff4694fbceef3dbaa4283827701d23"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "c423634f994188adf78efdb956d7d7d3",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 1594765,
"upload_time": "2024-09-11T22:16:59",
"upload_time_iso_8601": "2024-09-11T22:16:59.068539Z",
"url": "https://files.pythonhosted.org/packages/4d/17/1d818ee38c7b0dfa9b115bdf74f6c6c79869062f3a05b227c3c675ba56a7/PyGLM-2.7.2-cp310-cp310-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a36563aa541e6738cf177798446d7cb2209adc999d69807a8be68f5e7fd36a52",
"md5": "77055a0e2f4e09806db87363cb6e2bc9",
"sha256": "4764409849cb88c78f58bad0427d089c32083dda1740095cba9088e62cc30880"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "77055a0e2f4e09806db87363cb6e2bc9",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 1313036,
"upload_time": "2024-09-11T22:17:01",
"upload_time_iso_8601": "2024-09-11T22:17:01.341567Z",
"url": "https://files.pythonhosted.org/packages/a3/65/63aa541e6738cf177798446d7cb2209adc999d69807a8be68f5e7fd36a52/PyGLM-2.7.2-cp310-cp310-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "f82242ffbb61c58a79dad783801d317c077d16968d4941b92e4893a5acdb7a39",
"md5": "2de58c24293734ad45d069cd84e59239",
"sha256": "ad8fb3ae32bc777aa0cddaae0956fdaa77398eef030da5d89e4646e7c46056de"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "2de58c24293734ad45d069cd84e59239",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 10805347,
"upload_time": "2024-09-11T22:17:03",
"upload_time_iso_8601": "2024-09-11T22:17:03.212501Z",
"url": "https://files.pythonhosted.org/packages/f8/22/42ffbb61c58a79dad783801d317c077d16968d4941b92e4893a5acdb7a39/PyGLM-2.7.2-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "019f35157465dc69e17192be5c5e892157310dc68e37210f76e0d189918ebdd8",
"md5": "43a89772fed9420da1a540a177482cec",
"sha256": "be454b5b1782a718beec4475a548f8524ed162b896d9348a2c3698f964d57fbc"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-manylinux_2_17_i686.manylinux2014_i686.whl",
"has_sig": false,
"md5_digest": "43a89772fed9420da1a540a177482cec",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 10218061,
"upload_time": "2024-09-11T22:17:05",
"upload_time_iso_8601": "2024-09-11T22:17:05.418251Z",
"url": "https://files.pythonhosted.org/packages/01/9f/35157465dc69e17192be5c5e892157310dc68e37210f76e0d189918ebdd8/PyGLM-2.7.2-cp310-cp310-manylinux_2_17_i686.manylinux2014_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "57cbf3e7442841465940404eb67cf29bbfe78ca8e2cbb794d263bc97c7983709",
"md5": "b97436f2e4bb25e798d0bab9befecdfe",
"sha256": "4bb78cdbc8736e6e036dfe901f55ba1bfef47842a47134cc6ec28c65b4cc2cfb"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "b97436f2e4bb25e798d0bab9befecdfe",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 11275526,
"upload_time": "2024-09-11T22:17:08",
"upload_time_iso_8601": "2024-09-11T22:17:08.141602Z",
"url": "https://files.pythonhosted.org/packages/57/cb/f3e7442841465940404eb67cf29bbfe78ca8e2cbb794d263bc97c7983709/PyGLM-2.7.2-cp310-cp310-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6d5d917c743a2d218a9d9b26fe7229e6293c1023d78ca1e2f82490c289abe787",
"md5": "7a97b10981cd9a27d346632c0237d908",
"sha256": "975eec0ef89e8a7a42c6e6337102f9335040d483046cea5efca22025e4d632d7"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "7a97b10981cd9a27d346632c0237d908",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 11357075,
"upload_time": "2024-09-11T22:17:10",
"upload_time_iso_8601": "2024-09-11T22:17:10.741146Z",
"url": "https://files.pythonhosted.org/packages/6d/5d/917c743a2d218a9d9b26fe7229e6293c1023d78ca1e2f82490c289abe787/PyGLM-2.7.2-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "55939d00f4d7ceb1cc8a72fda71b318fa0a6c85dc53954f3fc6624394cad1e8d",
"md5": "9b488501c028bdc940f891d4c6929a28",
"sha256": "fbd9e91dbfdc40119c6d1be4992b7a8d03beb4c7b875c847f322c602dcc8877f"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-manylinux_2_27_aarch64.manylinux_2_28_aarch64.whl",
"has_sig": false,
"md5_digest": "9b488501c028bdc940f891d4c6929a28",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 10506932,
"upload_time": "2024-09-11T22:17:13",
"upload_time_iso_8601": "2024-09-11T22:17:13.355225Z",
"url": "https://files.pythonhosted.org/packages/55/93/9d00f4d7ceb1cc8a72fda71b318fa0a6c85dc53954f3fc6624394cad1e8d/PyGLM-2.7.2-cp310-cp310-manylinux_2_27_aarch64.manylinux_2_28_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6d42e4f382ce325072354c0b2de20a475541fb92c6db49fb970af9fe9c6519a0",
"md5": "4fb149029865c0f6f8917712c94e6c9e",
"sha256": "e801561c8c12bf7c27a710c80fba1de1bbad69ccde5695a2a6cfe3fae18ebb22"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-manylinux_2_27_s390x.manylinux_2_28_s390x.whl",
"has_sig": false,
"md5_digest": "4fb149029865c0f6f8917712c94e6c9e",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 10988552,
"upload_time": "2024-09-11T22:17:15",
"upload_time_iso_8601": "2024-09-11T22:17:15.954496Z",
"url": "https://files.pythonhosted.org/packages/6d/42/e4f382ce325072354c0b2de20a475541fb92c6db49fb970af9fe9c6519a0/PyGLM-2.7.2-cp310-cp310-manylinux_2_27_s390x.manylinux_2_28_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "102d11eebcfc660899b08450ff1c444522975f8031be0303e03b8225850100d6",
"md5": "0c16a11230e0d9abc4bcfc69a03593a7",
"sha256": "f9b365f3c427460625538c70f7f9b568149e2bc881ccf27cc89b9381b6c6837e"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-manylinux_2_27_x86_64.manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "0c16a11230e0d9abc4bcfc69a03593a7",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 10987105,
"upload_time": "2024-09-11T22:17:18",
"upload_time_iso_8601": "2024-09-11T22:17:18.753736Z",
"url": "https://files.pythonhosted.org/packages/10/2d/11eebcfc660899b08450ff1c444522975f8031be0303e03b8225850100d6/PyGLM-2.7.2-cp310-cp310-manylinux_2_27_x86_64.manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d9f09573701a40fabf180a4996f6ab822b71641ba3a25ad213004848e0aaf73c",
"md5": "8d3dc630af06dbe0bbb5ef8bc8be2562",
"sha256": "3c9f1771c8f0174361375c7b6d032095fd91e4e6d7b190117412984dd29f363c"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-musllinux_1_1_aarch64.whl",
"has_sig": false,
"md5_digest": "8d3dc630af06dbe0bbb5ef8bc8be2562",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 11927247,
"upload_time": "2024-09-11T22:17:22",
"upload_time_iso_8601": "2024-09-11T22:17:22.486597Z",
"url": "https://files.pythonhosted.org/packages/d9/f0/9573701a40fabf180a4996f6ab822b71641ba3a25ad213004848e0aaf73c/PyGLM-2.7.2-cp310-cp310-musllinux_1_1_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "c9928142a9f83e14d439098ed8295c5945ff401e8d3125615b9f868109c692c8",
"md5": "78b83919a0b92c0d1b9ada6a17904dff",
"sha256": "90eec5c8960b93830bbf2fbb93186c1a94aca03fbc71a0d5abd6c980042d1711"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-musllinux_1_1_i686.whl",
"has_sig": false,
"md5_digest": "78b83919a0b92c0d1b9ada6a17904dff",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 11137471,
"upload_time": "2024-09-11T22:17:25",
"upload_time_iso_8601": "2024-09-11T22:17:25.125543Z",
"url": "https://files.pythonhosted.org/packages/c9/92/8142a9f83e14d439098ed8295c5945ff401e8d3125615b9f868109c692c8/PyGLM-2.7.2-cp310-cp310-musllinux_1_1_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2264b33f43703273c10ade6d5e52d58213f61ddff4e34ca114d70b09c038297b",
"md5": "a43f99deb5bf44fb89f4d6ba7c147830",
"sha256": "3b01c09999976d1415fc96277750f0cced9be8c18fbbd578fd567508ce615272"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-musllinux_1_1_s390x.whl",
"has_sig": false,
"md5_digest": "a43f99deb5bf44fb89f4d6ba7c147830",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 12518376,
"upload_time": "2024-09-11T22:17:28",
"upload_time_iso_8601": "2024-09-11T22:17:28.458234Z",
"url": "https://files.pythonhosted.org/packages/22/64/b33f43703273c10ade6d5e52d58213f61ddff4e34ca114d70b09c038297b/PyGLM-2.7.2-cp310-cp310-musllinux_1_1_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "510aed91a095c762b51959d4bd222b57e4881f46c9422f3ab144afbe4cc0b65e",
"md5": "c42b316830519f6a807d1da44f3e3760",
"sha256": "7ab6fef17eab9c566ac81ace1242dc2a68ebc06a6235e755e49c1d8bc486a16a"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-musllinux_1_1_x86_64.whl",
"has_sig": false,
"md5_digest": "c42b316830519f6a807d1da44f3e3760",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 12492726,
"upload_time": "2024-09-11T22:17:31",
"upload_time_iso_8601": "2024-09-11T22:17:31.084177Z",
"url": "https://files.pythonhosted.org/packages/51/0a/ed91a095c762b51959d4bd222b57e4881f46c9422f3ab144afbe4cc0b65e/PyGLM-2.7.2-cp310-cp310-musllinux_1_1_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0564c3c18880896be2e0481adc215ea8fd13a8d053065e4bb06937e310e7a5a1",
"md5": "905b628cee90fb8a80ae081d1613b80d",
"sha256": "2b2818f81d3614154a622465bb9b46fae925287b28c607189f3c8716c8be3de0"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-win32.whl",
"has_sig": false,
"md5_digest": "905b628cee90fb8a80ae081d1613b80d",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 1238601,
"upload_time": "2024-09-11T22:17:33",
"upload_time_iso_8601": "2024-09-11T22:17:33.847767Z",
"url": "https://files.pythonhosted.org/packages/05/64/c3c18880896be2e0481adc215ea8fd13a8d053065e4bb06937e310e7a5a1/PyGLM-2.7.2-cp310-cp310-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d03a2fb911609ab64cbd1046c4d6388a04302e6cdf69e7e314c9b91c4ae7e81d",
"md5": "af78e69e8450829bd142f9b57b60dd3d",
"sha256": "82b49ff303f17300f5032842ad917e4e300f30ab8fecf5307bd4a937cea9267e"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-win_amd64.whl",
"has_sig": false,
"md5_digest": "af78e69e8450829bd142f9b57b60dd3d",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 1574523,
"upload_time": "2024-09-11T22:17:35",
"upload_time_iso_8601": "2024-09-11T22:17:35.274317Z",
"url": "https://files.pythonhosted.org/packages/d0/3a/2fb911609ab64cbd1046c4d6388a04302e6cdf69e7e314c9b91c4ae7e81d/PyGLM-2.7.2-cp310-cp310-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "f6eb15b45d4c1dd9f0bc92ab9196056e193ac942d5210654549165ad63624180",
"md5": "f6c18bbcd291b9d348734368ed470092",
"sha256": "1595b07a0a8809c9bbf57ee5c43b0ca7ba6dea6346fdf7e39b2b6bce06644301"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp310-cp310-win_arm64.whl",
"has_sig": false,
"md5_digest": "f6c18bbcd291b9d348734368ed470092",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 1128184,
"upload_time": "2024-09-11T22:17:36",
"upload_time_iso_8601": "2024-09-11T22:17:36.677435Z",
"url": "https://files.pythonhosted.org/packages/f6/eb/15b45d4c1dd9f0bc92ab9196056e193ac942d5210654549165ad63624180/PyGLM-2.7.2-cp310-cp310-win_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "302bacdd6489e49aa63da7da24b3841d8c13aab45c94de53dada841f4033b483",
"md5": "b1a0ac34b0db790cff637f7dcb6dbc1b",
"sha256": "2f739c81191e7ee4e8a84425d4ef14ae37fe895de6ce68691a4ef431567e3ef8"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "b1a0ac34b0db790cff637f7dcb6dbc1b",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 1594847,
"upload_time": "2024-09-11T22:17:38",
"upload_time_iso_8601": "2024-09-11T22:17:38.638452Z",
"url": "https://files.pythonhosted.org/packages/30/2b/acdd6489e49aa63da7da24b3841d8c13aab45c94de53dada841f4033b483/PyGLM-2.7.2-cp311-cp311-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "c6b0bf0a282b01159cd73d2f612cd8fa146c5e30eaa679d913093daaa37a1443",
"md5": "46375da964d1fe26b4547b012571a172",
"sha256": "5e4e754fd8f39c97bd0b1ee8a70726cc0b50bcd65468e7d6dd4b99978dabef75"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "46375da964d1fe26b4547b012571a172",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 1312922,
"upload_time": "2024-09-11T22:17:40",
"upload_time_iso_8601": "2024-09-11T22:17:40.493442Z",
"url": "https://files.pythonhosted.org/packages/c6/b0/bf0a282b01159cd73d2f612cd8fa146c5e30eaa679d913093daaa37a1443/PyGLM-2.7.2-cp311-cp311-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "fad9c485a95d52aa08ab1855d766d1cf0a6b1603c60076acc4aa779cc26bcd63",
"md5": "b1e7927b91a2da9611dea2dc6ce332ab",
"sha256": "c2fd2fc077ca9aecc8884635d786bb401ead80de928155fe644026ceb35eacf5"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "b1e7927b91a2da9611dea2dc6ce332ab",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 11358737,
"upload_time": "2024-09-11T22:17:42",
"upload_time_iso_8601": "2024-09-11T22:17:42.736305Z",
"url": "https://files.pythonhosted.org/packages/fa/d9/c485a95d52aa08ab1855d766d1cf0a6b1603c60076acc4aa779cc26bcd63/PyGLM-2.7.2-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0bd4b42055832a918ad45c5e213580aa0b13e844bb4d0aff6b854bc19705145a",
"md5": "7a870b92d8e7cd3632b98a68ee51de76",
"sha256": "06e6a9d1e73af37a2b57673b2cec1fa3993933e320ac6e574eea024c78398c00"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-manylinux_2_17_i686.manylinux2014_i686.whl",
"has_sig": false,
"md5_digest": "7a870b92d8e7cd3632b98a68ee51de76",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 10756790,
"upload_time": "2024-09-11T22:17:45",
"upload_time_iso_8601": "2024-09-11T22:17:45.323033Z",
"url": "https://files.pythonhosted.org/packages/0b/d4/b42055832a918ad45c5e213580aa0b13e844bb4d0aff6b854bc19705145a/PyGLM-2.7.2-cp311-cp311-manylinux_2_17_i686.manylinux2014_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4b980a16972223b0b36b8be9fdac24748d38db530fda9d8496db507565aa1b42",
"md5": "be5d1dddffcf081139c70bf71cc70deb",
"sha256": "7f241838c2cbfc5cf92bafe72499caa8397f2cafca5971ab1e6e8e2786dcc673"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "be5d1dddffcf081139c70bf71cc70deb",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 11866277,
"upload_time": "2024-09-11T22:17:47",
"upload_time_iso_8601": "2024-09-11T22:17:47.953498Z",
"url": "https://files.pythonhosted.org/packages/4b/98/0a16972223b0b36b8be9fdac24748d38db530fda9d8496db507565aa1b42/PyGLM-2.7.2-cp311-cp311-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "85bc7d4be6ed167e643ee7def4b7424b90a5218a8ff17f3627baf63a627b5213",
"md5": "5b844e2a591f7e07a140e8ded7785c4a",
"sha256": "9342297296bdf1c6c75aecca55c8ec4722b58c3d4d2fd1230166f141c6adde74"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "5b844e2a591f7e07a140e8ded7785c4a",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 11888818,
"upload_time": "2024-09-11T22:17:50",
"upload_time_iso_8601": "2024-09-11T22:17:50.932803Z",
"url": "https://files.pythonhosted.org/packages/85/bc/7d4be6ed167e643ee7def4b7424b90a5218a8ff17f3627baf63a627b5213/PyGLM-2.7.2-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6d7cbfa3da4526fa712f3278968e99f88e694b5ce140516bbf79f3f012fc06af",
"md5": "16e93e480933df9401b5259275fde379",
"sha256": "7f6c25474bc7cfa0fc539834b95ba56ca23072a54be8ef78d3df2cb0f028fe26"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-manylinux_2_27_aarch64.manylinux_2_28_aarch64.whl",
"has_sig": false,
"md5_digest": "16e93e480933df9401b5259275fde379",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 10923509,
"upload_time": "2024-09-11T22:17:53",
"upload_time_iso_8601": "2024-09-11T22:17:53.607592Z",
"url": "https://files.pythonhosted.org/packages/6d/7c/bfa3da4526fa712f3278968e99f88e694b5ce140516bbf79f3f012fc06af/PyGLM-2.7.2-cp311-cp311-manylinux_2_27_aarch64.manylinux_2_28_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7bbae6bb456c165b1ec19ecbfc7f6b34e4e4ae9bf2b3d87cfcd2d55f5dd85369",
"md5": "c49f3d4fd4db3c7ed6b818dfad5259c0",
"sha256": "31ab8e83cc2a48d8ba1c5b6e40ab4bbfc3ed648fceb4295ef630ebdeb1c36039"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-manylinux_2_27_s390x.manylinux_2_28_s390x.whl",
"has_sig": false,
"md5_digest": "c49f3d4fd4db3c7ed6b818dfad5259c0",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 11434160,
"upload_time": "2024-09-11T22:17:56",
"upload_time_iso_8601": "2024-09-11T22:17:56.208840Z",
"url": "https://files.pythonhosted.org/packages/7b/ba/e6bb456c165b1ec19ecbfc7f6b34e4e4ae9bf2b3d87cfcd2d55f5dd85369/PyGLM-2.7.2-cp311-cp311-manylinux_2_27_s390x.manylinux_2_28_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a71206c725d5edb9910dca7eee70514ed7bcd55bafbc7ea7b6bc7529a2f67ea8",
"md5": "e8e0bea26f0d8491a247134d31e8beb9",
"sha256": "a4feb3cc7c8c4e7d1246457ec99b5801a827bc263cc6b96db04ff58c0ce7fcab"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-manylinux_2_27_x86_64.manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "e8e0bea26f0d8491a247134d31e8beb9",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 11386870,
"upload_time": "2024-09-11T22:17:58",
"upload_time_iso_8601": "2024-09-11T22:17:58.904275Z",
"url": "https://files.pythonhosted.org/packages/a7/12/06c725d5edb9910dca7eee70514ed7bcd55bafbc7ea7b6bc7529a2f67ea8/PyGLM-2.7.2-cp311-cp311-manylinux_2_27_x86_64.manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a7d666987368da1fcd8fe6fed67c05631d2b2c443c62a5e0c440d8e2990acf4d",
"md5": "7d1d6300abd7b3d8aa6c32c8854a9dca",
"sha256": "5a12d2d0bf62f12869e1397274fa610772e1985120a6280f55b86404eaee80b8"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-musllinux_1_1_aarch64.whl",
"has_sig": false,
"md5_digest": "7d1d6300abd7b3d8aa6c32c8854a9dca",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 12324125,
"upload_time": "2024-09-11T22:18:01",
"upload_time_iso_8601": "2024-09-11T22:18:01.492618Z",
"url": "https://files.pythonhosted.org/packages/a7/d6/66987368da1fcd8fe6fed67c05631d2b2c443c62a5e0c440d8e2990acf4d/PyGLM-2.7.2-cp311-cp311-musllinux_1_1_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d990e5d488359d5a6b1d86621e724fba4f22b72099b72aaf38fb25a242e384b1",
"md5": "1ae9a9921c7b8bbfbee775589c7bc305",
"sha256": "fb7413edb23fd946b144013be44907335d8be1b3d7fcdb8206c7a5d292151f21"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-musllinux_1_1_i686.whl",
"has_sig": false,
"md5_digest": "1ae9a9921c7b8bbfbee775589c7bc305",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 11479399,
"upload_time": "2024-09-11T22:18:04",
"upload_time_iso_8601": "2024-09-11T22:18:04.226280Z",
"url": "https://files.pythonhosted.org/packages/d9/90/e5d488359d5a6b1d86621e724fba4f22b72099b72aaf38fb25a242e384b1/PyGLM-2.7.2-cp311-cp311-musllinux_1_1_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "29983f2af60d711c40792e5413724cf7c9f6d2ef5812143a00d9a3ed0033eaea",
"md5": "12df3b237fb1f0b5c561edac7c1629b8",
"sha256": "f75c6048e5b55611f4b3a9af1741448dcd0e8bc4d15f17993f22d58cf881c462"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-musllinux_1_1_s390x.whl",
"has_sig": false,
"md5_digest": "12df3b237fb1f0b5c561edac7c1629b8",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 12910345,
"upload_time": "2024-09-11T22:18:06",
"upload_time_iso_8601": "2024-09-11T22:18:06.584996Z",
"url": "https://files.pythonhosted.org/packages/29/98/3f2af60d711c40792e5413724cf7c9f6d2ef5812143a00d9a3ed0033eaea/PyGLM-2.7.2-cp311-cp311-musllinux_1_1_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5d0f9ac41a6142705839dda9529f4cbc59ef47192124c27bac177f27d06b094c",
"md5": "42df773f66875da5538584c7dd63ffb1",
"sha256": "0b059d5edf0f320d40f122c569bf44e79291f31f875a7ebe69de93f3f177f0e8"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-musllinux_1_1_x86_64.whl",
"has_sig": false,
"md5_digest": "42df773f66875da5538584c7dd63ffb1",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 12840034,
"upload_time": "2024-09-11T22:18:08",
"upload_time_iso_8601": "2024-09-11T22:18:08.818878Z",
"url": "https://files.pythonhosted.org/packages/5d/0f/9ac41a6142705839dda9529f4cbc59ef47192124c27bac177f27d06b094c/PyGLM-2.7.2-cp311-cp311-musllinux_1_1_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2aec6e5b0b306557f8aa568efeff201f4e241449dd243953a156d03a2cb70b7b",
"md5": "80ee63d2b52314a039a46d3b8c1253a1",
"sha256": "1f3c96faa2bbb6cc16b25c7b2fb941542e64768c2393f3244ed94e2dddf391da"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-win32.whl",
"has_sig": false,
"md5_digest": "80ee63d2b52314a039a46d3b8c1253a1",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 1255060,
"upload_time": "2024-09-11T22:18:11",
"upload_time_iso_8601": "2024-09-11T22:18:11.330046Z",
"url": "https://files.pythonhosted.org/packages/2a/ec/6e5b0b306557f8aa568efeff201f4e241449dd243953a156d03a2cb70b7b/PyGLM-2.7.2-cp311-cp311-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "53a9549861c8c2dcccbe31cbab37e37afbd64811376f941c198cc765d05c907f",
"md5": "4ca8c0c13c9b6004f8def15598eec542",
"sha256": "7eee8c7d56f7f75365848067f24c8578167f812877d0e37973e2075565ef1bcd"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-win_amd64.whl",
"has_sig": false,
"md5_digest": "4ca8c0c13c9b6004f8def15598eec542",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 1590653,
"upload_time": "2024-09-11T22:18:12",
"upload_time_iso_8601": "2024-09-11T22:18:12.646463Z",
"url": "https://files.pythonhosted.org/packages/53/a9/549861c8c2dcccbe31cbab37e37afbd64811376f941c198cc765d05c907f/PyGLM-2.7.2-cp311-cp311-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2718c17b202d8397cbe6f60f7bb5bc7437e5270025ff69b0df7fc7e3178f4aac",
"md5": "fc740e0ae40fe0c6dc62971bce9f5564",
"sha256": "124f94fc1e2b81a78c86813bfa667408a6cf6f973e12601125cc4bc7bd0abba8"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp311-cp311-win_arm64.whl",
"has_sig": false,
"md5_digest": "fc740e0ae40fe0c6dc62971bce9f5564",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 1142606,
"upload_time": "2024-09-11T22:18:14",
"upload_time_iso_8601": "2024-09-11T22:18:14.050806Z",
"url": "https://files.pythonhosted.org/packages/27/18/c17b202d8397cbe6f60f7bb5bc7437e5270025ff69b0df7fc7e3178f4aac/PyGLM-2.7.2-cp311-cp311-win_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a8cb14aaade9f5685e3b773bac9478478773be8584df566530a2b4dfc7424565",
"md5": "2dffc4f5ebfff3bddde55a4681850332",
"sha256": "f6566f32eb2e055e4384dcb938e80c2d4e933b9149cc98a0beac20b7527c423c"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "2dffc4f5ebfff3bddde55a4681850332",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 1600297,
"upload_time": "2024-09-11T22:18:15",
"upload_time_iso_8601": "2024-09-11T22:18:15.399575Z",
"url": "https://files.pythonhosted.org/packages/a8/cb/14aaade9f5685e3b773bac9478478773be8584df566530a2b4dfc7424565/PyGLM-2.7.2-cp312-cp312-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d6db5a41728e8899bbe120c2db7c348cadcc452a7f4fb5e36d2139b7e9bcd2db",
"md5": "965981b730a2c202fff6f2bd41e017aa",
"sha256": "afae3f389b7052955f1d64d14098c0cf259537d2a366bbd9b3a3604fb78cae1a"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "965981b730a2c202fff6f2bd41e017aa",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 1319076,
"upload_time": "2024-09-11T22:18:16",
"upload_time_iso_8601": "2024-09-11T22:18:16.679594Z",
"url": "https://files.pythonhosted.org/packages/d6/db/5a41728e8899bbe120c2db7c348cadcc452a7f4fb5e36d2139b7e9bcd2db/PyGLM-2.7.2-cp312-cp312-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d2590a504a5e3b1f6b5abf545aecd978568797e685092808191179d3aacaea86",
"md5": "724dae618f8b8a1dd3f9e761367b9589",
"sha256": "4882a69b78bca8152b0d7a03e369cea72d70e117b9b687f640e285d69ccc0007"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "724dae618f8b8a1dd3f9e761367b9589",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 11448695,
"upload_time": "2024-09-11T22:18:19",
"upload_time_iso_8601": "2024-09-11T22:18:19.082706Z",
"url": "https://files.pythonhosted.org/packages/d2/59/0a504a5e3b1f6b5abf545aecd978568797e685092808191179d3aacaea86/PyGLM-2.7.2-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "80c78d26e8641ed4b6c294ce76d0e97ee9159e4cab803e6b1dabea5c1e664645",
"md5": "94a95320d00dc592e79abbfeb824f0d4",
"sha256": "2b00f2726f49f1377030ef4f08cc23033ce2f5433a1e997467478cf562f01d04"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-manylinux_2_17_i686.manylinux2014_i686.whl",
"has_sig": false,
"md5_digest": "94a95320d00dc592e79abbfeb824f0d4",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 10826388,
"upload_time": "2024-09-11T22:18:21",
"upload_time_iso_8601": "2024-09-11T22:18:21.244105Z",
"url": "https://files.pythonhosted.org/packages/80/c7/8d26e8641ed4b6c294ce76d0e97ee9159e4cab803e6b1dabea5c1e664645/PyGLM-2.7.2-cp312-cp312-manylinux_2_17_i686.manylinux2014_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "215e29ec72a3590e963ac9444101bcd9ec0da3fa37b5031607964eb6efb43275",
"md5": "e780dda86fb29d7ca3c70f948ba2790a",
"sha256": "90eee4aff334923408865d6e2c818c86cbca2beee1f13579d7894c6a70b8870c"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "e780dda86fb29d7ca3c70f948ba2790a",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 11977088,
"upload_time": "2024-09-11T22:18:24",
"upload_time_iso_8601": "2024-09-11T22:18:24.122233Z",
"url": "https://files.pythonhosted.org/packages/21/5e/29ec72a3590e963ac9444101bcd9ec0da3fa37b5031607964eb6efb43275/PyGLM-2.7.2-cp312-cp312-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "bb8b234707dd2fb67afc92a0321f3ccb3da549196881ad71b03850101df846df",
"md5": "a856f96b6019af22524ecf9da7a37dce",
"sha256": "2cf2dbf7b10e781704fa71c79efed9a51240e81eea125dd25cfc375d55a6af10"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "a856f96b6019af22524ecf9da7a37dce",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 11975112,
"upload_time": "2024-09-11T22:18:26",
"upload_time_iso_8601": "2024-09-11T22:18:26.324834Z",
"url": "https://files.pythonhosted.org/packages/bb/8b/234707dd2fb67afc92a0321f3ccb3da549196881ad71b03850101df846df/PyGLM-2.7.2-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5bf0db27c5a19fe1a5167887f2ee0a1ccd6698d78beaa2c6f546644ce0c33ffc",
"md5": "afff5c11d0f57c4ea3ead8c94e46fd75",
"sha256": "231fab25223952af95d4559a7995ddb8a2e25729ede518e18967f6134168f6e7"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-manylinux_2_27_aarch64.manylinux_2_28_aarch64.whl",
"has_sig": false,
"md5_digest": "afff5c11d0f57c4ea3ead8c94e46fd75",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 11016297,
"upload_time": "2024-09-11T22:18:28",
"upload_time_iso_8601": "2024-09-11T22:18:28.447507Z",
"url": "https://files.pythonhosted.org/packages/5b/f0/db27c5a19fe1a5167887f2ee0a1ccd6698d78beaa2c6f546644ce0c33ffc/PyGLM-2.7.2-cp312-cp312-manylinux_2_27_aarch64.manylinux_2_28_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "cd89c0b31c857e27e6993fdf9c2e38599397b4462acef939f5bd33aa0509199d",
"md5": "2e372d8cfb140ff3bd486df2bcecac0d",
"sha256": "2bc164b1d00a254b6ace8ec3f8e32b690f4762ebd5fb069eff710eb303c16912"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-manylinux_2_27_s390x.manylinux_2_28_s390x.whl",
"has_sig": false,
"md5_digest": "2e372d8cfb140ff3bd486df2bcecac0d",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 11543906,
"upload_time": "2024-09-11T22:18:30",
"upload_time_iso_8601": "2024-09-11T22:18:30.783567Z",
"url": "https://files.pythonhosted.org/packages/cd/89/c0b31c857e27e6993fdf9c2e38599397b4462acef939f5bd33aa0509199d/PyGLM-2.7.2-cp312-cp312-manylinux_2_27_s390x.manylinux_2_28_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1b4c2818e1a11dec48d6f58437418a7b3928e2d4f953dad00c919d5485abf875",
"md5": "7525993d3eef928c68f8a1a42e930cb1",
"sha256": "74f6ad3f4723a11cacce43caa09781e1dc3f40e20e03d5433a93fa095ce2be46"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-manylinux_2_27_x86_64.manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "7525993d3eef928c68f8a1a42e930cb1",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 11470566,
"upload_time": "2024-09-11T22:18:33",
"upload_time_iso_8601": "2024-09-11T22:18:33.064440Z",
"url": "https://files.pythonhosted.org/packages/1b/4c/2818e1a11dec48d6f58437418a7b3928e2d4f953dad00c919d5485abf875/PyGLM-2.7.2-cp312-cp312-manylinux_2_27_x86_64.manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d58abdcb88bd4864d034b2dedf42a0c771f3e462bd0fd0e8279287b2fbeaa177",
"md5": "fcd298d0cc1d4b464c423770503c0986",
"sha256": "67a6e3fe523cdbbe3cbbf1320b19ea4e53292369c552382f86c4c5de09f2bbb7"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-musllinux_1_1_aarch64.whl",
"has_sig": false,
"md5_digest": "fcd298d0cc1d4b464c423770503c0986",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 12413702,
"upload_time": "2024-09-11T22:18:35",
"upload_time_iso_8601": "2024-09-11T22:18:35.288546Z",
"url": "https://files.pythonhosted.org/packages/d5/8a/bdcb88bd4864d034b2dedf42a0c771f3e462bd0fd0e8279287b2fbeaa177/PyGLM-2.7.2-cp312-cp312-musllinux_1_1_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "113a2d6a92d0c01bf193ebcb099909e48c20eb1238bab2cc34b836306ce48f88",
"md5": "9a7f25b00c9e6e8ada6b0de1101ed631",
"sha256": "88de3d9f942bb0e2286e8435545d2bc95c42c2df943aef788075d040707c4fac"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-musllinux_1_1_i686.whl",
"has_sig": false,
"md5_digest": "9a7f25b00c9e6e8ada6b0de1101ed631",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 11662058,
"upload_time": "2024-09-11T22:18:41",
"upload_time_iso_8601": "2024-09-11T22:18:41.054078Z",
"url": "https://files.pythonhosted.org/packages/11/3a/2d6a92d0c01bf193ebcb099909e48c20eb1238bab2cc34b836306ce48f88/PyGLM-2.7.2-cp312-cp312-musllinux_1_1_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5bbb157fc38afbe331f5dbd9f0408c61d28cc8854de1748b6dd9aed1beee9c30",
"md5": "9ccb86fe9faf1b2f599e7f248609fde4",
"sha256": "6dffb2e46437975fce30f966442a235411dad64fe22580895f8d7f4b67cc70a4"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-musllinux_1_1_s390x.whl",
"has_sig": false,
"md5_digest": "9ccb86fe9faf1b2f599e7f248609fde4",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 13187879,
"upload_time": "2024-09-11T22:18:43",
"upload_time_iso_8601": "2024-09-11T22:18:43.921521Z",
"url": "https://files.pythonhosted.org/packages/5b/bb/157fc38afbe331f5dbd9f0408c61d28cc8854de1748b6dd9aed1beee9c30/PyGLM-2.7.2-cp312-cp312-musllinux_1_1_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "c4e0e84c548b41e73b62159d5c7b6c759dcd3b9d10c4e0fc5a9663ab7a08c20b",
"md5": "7ed712cb0a4a949f701e5354d398c832",
"sha256": "04803e7481eb10a12842f3ac336ee8f4b07156d74588a8ba5c03089677856ba7"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-musllinux_1_1_x86_64.whl",
"has_sig": false,
"md5_digest": "7ed712cb0a4a949f701e5354d398c832",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 12967139,
"upload_time": "2024-09-11T22:18:46",
"upload_time_iso_8601": "2024-09-11T22:18:46.200285Z",
"url": "https://files.pythonhosted.org/packages/c4/e0/e84c548b41e73b62159d5c7b6c759dcd3b9d10c4e0fc5a9663ab7a08c20b/PyGLM-2.7.2-cp312-cp312-musllinux_1_1_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2b657350d27066daa363db4fc49a08626a47c7e1c561a2e76560087c84b456dc",
"md5": "9c91ed5d1dfc02c49e10817ad020928f",
"sha256": "9d2d6c7c73b33745b94031f9199d222944c4d69be9271c676f0cbbccbf217609"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-win32.whl",
"has_sig": false,
"md5_digest": "9c91ed5d1dfc02c49e10817ad020928f",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 1255391,
"upload_time": "2024-09-11T22:18:48",
"upload_time_iso_8601": "2024-09-11T22:18:48.475972Z",
"url": "https://files.pythonhosted.org/packages/2b/65/7350d27066daa363db4fc49a08626a47c7e1c561a2e76560087c84b456dc/PyGLM-2.7.2-cp312-cp312-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1d739192ab183ced445a1ac6b161a18b09d8097c12c1570766ac12db56ce45af",
"md5": "d7c73830f65faf4779640e9a6b5345d2",
"sha256": "29f8b321ce2021534738d5778b124b7d1efb6c8b7692a4ce745a85ec4e6fd85f"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-win_amd64.whl",
"has_sig": false,
"md5_digest": "d7c73830f65faf4779640e9a6b5345d2",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 1593111,
"upload_time": "2024-09-11T22:18:49",
"upload_time_iso_8601": "2024-09-11T22:18:49.897270Z",
"url": "https://files.pythonhosted.org/packages/1d/73/9192ab183ced445a1ac6b161a18b09d8097c12c1570766ac12db56ce45af/PyGLM-2.7.2-cp312-cp312-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "f3eedcd5e888ba9f9868ea4520f79dd87c7dc5ed6f500a51d9dc462ae1beb676",
"md5": "3bdad842e0e7f7feb3844f74dfd20b44",
"sha256": "7f1ab14bb46935055a765213a4536ce017870a102ffbb49b925dba9bb7de421b"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp312-cp312-win_arm64.whl",
"has_sig": false,
"md5_digest": "3bdad842e0e7f7feb3844f74dfd20b44",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 1143147,
"upload_time": "2024-09-11T22:18:51",
"upload_time_iso_8601": "2024-09-11T22:18:51.478922Z",
"url": "https://files.pythonhosted.org/packages/f3/ee/dcd5e888ba9f9868ea4520f79dd87c7dc5ed6f500a51d9dc462ae1beb676/PyGLM-2.7.2-cp312-cp312-win_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "994879bf8b664bd3d7991e20e94f07879d4268112c8fde99a10ac7afd0f79664",
"md5": "e2fa2c1d9c47aa026c8466284842494f",
"sha256": "6953d1c4205834dd3d64d3d022cbfb89ce99f8ea7e68f63f63458338836f1486"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "e2fa2c1d9c47aa026c8466284842494f",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 1593100,
"upload_time": "2024-09-11T22:18:53",
"upload_time_iso_8601": "2024-09-11T22:18:53.406049Z",
"url": "https://files.pythonhosted.org/packages/99/48/79bf8b664bd3d7991e20e94f07879d4268112c8fde99a10ac7afd0f79664/PyGLM-2.7.2-cp38-cp38-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "b18875c1ace4cf87d5997d31d49759b0ffc8b3c6bb5dd462c3c02a2efa79976a",
"md5": "0add1fb3e115007ebde8cb48cbc65162",
"sha256": "b511aefdf09ef2cbe53ffc0b19075460210e13627f7a9496fb14e181bc6096d8"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "0add1fb3e115007ebde8cb48cbc65162",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 1315605,
"upload_time": "2024-09-11T22:18:54",
"upload_time_iso_8601": "2024-09-11T22:18:54.715683Z",
"url": "https://files.pythonhosted.org/packages/b1/88/75c1ace4cf87d5997d31d49759b0ffc8b3c6bb5dd462c3c02a2efa79976a/PyGLM-2.7.2-cp38-cp38-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a545f16dfb0f329c85e0156bb229bec79a51fb21e7f804d326f308aa6d3081c9",
"md5": "248cb97485b8f30095894114e9ce9a35",
"sha256": "054d87d1509c17bcdc67d7e65b1fe3a593c1f92ffebd6e6e221e4623f1bcbbb3"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "248cb97485b8f30095894114e9ce9a35",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 10087345,
"upload_time": "2024-09-11T22:18:56",
"upload_time_iso_8601": "2024-09-11T22:18:56.203228Z",
"url": "https://files.pythonhosted.org/packages/a5/45/f16dfb0f329c85e0156bb229bec79a51fb21e7f804d326f308aa6d3081c9/PyGLM-2.7.2-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "998ab5891fe4dba0960ad501bda74b097ac5540056b3423d728397bb24845b19",
"md5": "195196c58b7a7acdc502ba8017d6b4fd",
"sha256": "d7f88cae54783a2adf40352507a401ba3654264d207794ea9190a6b0d503bec1"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-manylinux_2_17_i686.manylinux2014_i686.whl",
"has_sig": false,
"md5_digest": "195196c58b7a7acdc502ba8017d6b4fd",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 9593327,
"upload_time": "2024-09-11T22:18:58",
"upload_time_iso_8601": "2024-09-11T22:18:58.376473Z",
"url": "https://files.pythonhosted.org/packages/99/8a/b5891fe4dba0960ad501bda74b097ac5540056b3423d728397bb24845b19/PyGLM-2.7.2-cp38-cp38-manylinux_2_17_i686.manylinux2014_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "804cba90c34a153c99806fc3932c2a1f904cf8af8426362c9b97307fc3fe9bf5",
"md5": "de14f5b0d1fb08e289570bee81c75dfb",
"sha256": "23a3082d2219caf4ef5e3d1a4fd32d9753070fd40ffbebbc7c21d2b3b2cfc203"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "de14f5b0d1fb08e289570bee81c75dfb",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 10585206,
"upload_time": "2024-09-11T22:19:00",
"upload_time_iso_8601": "2024-09-11T22:19:00.650229Z",
"url": "https://files.pythonhosted.org/packages/80/4c/ba90c34a153c99806fc3932c2a1f904cf8af8426362c9b97307fc3fe9bf5/PyGLM-2.7.2-cp38-cp38-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9439df33d7c22300af7b0686064375487c6178b6b7d1fde80d43e47a23466d6e",
"md5": "2399a1b66e06f0208ac94a4f54ff2142",
"sha256": "51ccf3d92e1bba3374604c229a41bd64ab74e8924cec851576d866743af22349"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "2399a1b66e06f0208ac94a4f54ff2142",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 10688195,
"upload_time": "2024-09-11T22:19:03",
"upload_time_iso_8601": "2024-09-11T22:19:03.851182Z",
"url": "https://files.pythonhosted.org/packages/94/39/df33d7c22300af7b0686064375487c6178b6b7d1fde80d43e47a23466d6e/PyGLM-2.7.2-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ad05c70b774be0215aeb08630f2627ccf709c676d99471d54d2d2ab3ca70f473",
"md5": "001b869defb508d918fd2deeea776b78",
"sha256": "2e79b77507abdb146bef260efaf0141ea946bc3b96926152faf3b1b6a38d4f8d"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-manylinux_2_27_aarch64.manylinux_2_28_aarch64.whl",
"has_sig": false,
"md5_digest": "001b869defb508d918fd2deeea776b78",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 9833295,
"upload_time": "2024-09-11T22:19:06",
"upload_time_iso_8601": "2024-09-11T22:19:06.239453Z",
"url": "https://files.pythonhosted.org/packages/ad/05/c70b774be0215aeb08630f2627ccf709c676d99471d54d2d2ab3ca70f473/PyGLM-2.7.2-cp38-cp38-manylinux_2_27_aarch64.manylinux_2_28_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "03ccce7201f29ed2deb0a6a9785e49e42a4285179145ee99c84e8c559ba8346f",
"md5": "ee32002bc5548431449cac508cfedb1e",
"sha256": "4c0119f532b2d5cd9bebfb686bc34b245292256afa35d6a7fc558e21bdde7609"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-manylinux_2_27_s390x.manylinux_2_28_s390x.whl",
"has_sig": false,
"md5_digest": "ee32002bc5548431449cac508cfedb1e",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 10322982,
"upload_time": "2024-09-11T22:19:08",
"upload_time_iso_8601": "2024-09-11T22:19:08.695451Z",
"url": "https://files.pythonhosted.org/packages/03/cc/ce7201f29ed2deb0a6a9785e49e42a4285179145ee99c84e8c559ba8346f/PyGLM-2.7.2-cp38-cp38-manylinux_2_27_s390x.manylinux_2_28_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "eac328ed3d148d9faa0fb62c48f5e4bfcbedd98674c9126eb915f90b7940f362",
"md5": "cd339b08df87924e8a8e670a07a35308",
"sha256": "c7e8c36e6929f3234c15d40f66e6c435ac7044990a81ef4ea7827ad26130fe27"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-manylinux_2_27_x86_64.manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "cd339b08df87924e8a8e670a07a35308",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 10312659,
"upload_time": "2024-09-11T22:19:11",
"upload_time_iso_8601": "2024-09-11T22:19:11.696508Z",
"url": "https://files.pythonhosted.org/packages/ea/c3/28ed3d148d9faa0fb62c48f5e4bfcbedd98674c9126eb915f90b7940f362/PyGLM-2.7.2-cp38-cp38-manylinux_2_27_x86_64.manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4b8c30183b622d05e18b1e17cef6276a715ca878372af0614549f3b61397aa8a",
"md5": "08609a8514a83de39cd145b3cd5b60b6",
"sha256": "fb16e8493560bdaa6c5d9dc93020cad4226356471213e6d2d6eab4db73c062e4"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-musllinux_1_1_aarch64.whl",
"has_sig": false,
"md5_digest": "08609a8514a83de39cd145b3cd5b60b6",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 11488374,
"upload_time": "2024-09-11T22:19:14",
"upload_time_iso_8601": "2024-09-11T22:19:14.223864Z",
"url": "https://files.pythonhosted.org/packages/4b/8c/30183b622d05e18b1e17cef6276a715ca878372af0614549f3b61397aa8a/PyGLM-2.7.2-cp38-cp38-musllinux_1_1_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ad1e74b15e5f87aa47f1dbd6a566962582c8250bf6168b811b69773b3bc1c9aa",
"md5": "c66cd14b2cf248a44324b4a5c53fb5b2",
"sha256": "b27cafacf0bb40735a3073aee95f23928d519705ad72b4859df052cea1843b12"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-musllinux_1_1_i686.whl",
"has_sig": false,
"md5_digest": "c66cd14b2cf248a44324b4a5c53fb5b2",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 10807420,
"upload_time": "2024-09-11T22:19:16",
"upload_time_iso_8601": "2024-09-11T22:19:16.354872Z",
"url": "https://files.pythonhosted.org/packages/ad/1e/74b15e5f87aa47f1dbd6a566962582c8250bf6168b811b69773b3bc1c9aa/PyGLM-2.7.2-cp38-cp38-musllinux_1_1_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8f4669f21d1e28e66cc36c3d6fd00ae83c58db4dac9fee3f20b6be32c4110637",
"md5": "4fa798c845364dc0e642bd5f828d25f7",
"sha256": "bbcfb3d28de43b2fb6a3cc05cf8be34570d22c488d1c91cd289bafac771b524b"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-musllinux_1_1_s390x.whl",
"has_sig": false,
"md5_digest": "4fa798c845364dc0e642bd5f828d25f7",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 12091253,
"upload_time": "2024-09-11T22:19:18",
"upload_time_iso_8601": "2024-09-11T22:19:18.586986Z",
"url": "https://files.pythonhosted.org/packages/8f/46/69f21d1e28e66cc36c3d6fd00ae83c58db4dac9fee3f20b6be32c4110637/PyGLM-2.7.2-cp38-cp38-musllinux_1_1_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "cbb810cb4fd70df82b97bc1314869fb8fb04b99226a43d172c74f463ca95b13b",
"md5": "170954bddcb4f3ae93e4b725989eca36",
"sha256": "39cab97a88dd04b5890adbe90cb83a28e2e1298cf4403ee0d7d4ec8af8b04619"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-musllinux_1_1_x86_64.whl",
"has_sig": false,
"md5_digest": "170954bddcb4f3ae93e4b725989eca36",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 12070369,
"upload_time": "2024-09-11T22:19:21",
"upload_time_iso_8601": "2024-09-11T22:19:21.764921Z",
"url": "https://files.pythonhosted.org/packages/cb/b8/10cb4fd70df82b97bc1314869fb8fb04b99226a43d172c74f463ca95b13b/PyGLM-2.7.2-cp38-cp38-musllinux_1_1_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "59442962d36d7869aa80bfa4bb0f5a9ca28ba50b9866eb17df29fbda3e61a739",
"md5": "ea0c3915d0dfac6ccbadb39741fa79aa",
"sha256": "229c8050d5b8370bbfb1af4a70c78c3be0208c01db32d31d15cecccf9570e695"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-win32.whl",
"has_sig": false,
"md5_digest": "ea0c3915d0dfac6ccbadb39741fa79aa",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 1243835,
"upload_time": "2024-09-11T22:19:24",
"upload_time_iso_8601": "2024-09-11T22:19:24.863735Z",
"url": "https://files.pythonhosted.org/packages/59/44/2962d36d7869aa80bfa4bb0f5a9ca28ba50b9866eb17df29fbda3e61a739/PyGLM-2.7.2-cp38-cp38-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "fe0767ba9adb1eb2867605b1d2b9d559c872816d75a1b4390b06e5989bed6bf9",
"md5": "3d91b82eb5bc26eb54b2e5abba256da5",
"sha256": "6bd4f15f07772554e2a50fdb805050fbc38a150e5a6548bb1371f923394bdcf4"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp38-cp38-win_amd64.whl",
"has_sig": false,
"md5_digest": "3d91b82eb5bc26eb54b2e5abba256da5",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 1577783,
"upload_time": "2024-09-11T22:19:26",
"upload_time_iso_8601": "2024-09-11T22:19:26.340705Z",
"url": "https://files.pythonhosted.org/packages/fe/07/67ba9adb1eb2867605b1d2b9d559c872816d75a1b4390b06e5989bed6bf9/PyGLM-2.7.2-cp38-cp38-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8e1236cb264f6c4cf5ca9f5e76d3bcecc4129239625076df411924a290ce29fb",
"md5": "4d7e9d539e549c4b1c30545500b245b1",
"sha256": "0d7a0ce215f77e9c3cdb46a5c134e47c4a7c50531ee8b099d63ad742c6ff61de"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "4d7e9d539e549c4b1c30545500b245b1",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 1592884,
"upload_time": "2024-09-11T22:19:27",
"upload_time_iso_8601": "2024-09-11T22:19:27.885897Z",
"url": "https://files.pythonhosted.org/packages/8e/12/36cb264f6c4cf5ca9f5e76d3bcecc4129239625076df411924a290ce29fb/PyGLM-2.7.2-cp39-cp39-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7c40dc0ab011c9d3726b7989673806b2112b7796879532311cfadf07b4777019",
"md5": "a774f890348415aed97d74a506e49f78",
"sha256": "f3190b103f88b9bcc881c36a3f37271c5587918cdc3e35d4fb38e5f6ee865ef4"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "a774f890348415aed97d74a506e49f78",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 1311825,
"upload_time": "2024-09-11T22:19:29",
"upload_time_iso_8601": "2024-09-11T22:19:29.664461Z",
"url": "https://files.pythonhosted.org/packages/7c/40/dc0ab011c9d3726b7989673806b2112b7796879532311cfadf07b4777019/PyGLM-2.7.2-cp39-cp39-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "3c6355c937a740517a4981add648ab89988737ad8f1ad0b79f79e1d9be3f4cd2",
"md5": "a4d95cf0fd23665ea6a854cbcedaa404",
"sha256": "c72c12f6fdc509af4d717dc729d8e4dbd83243c57f7e284ad11534438f080549"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "a4d95cf0fd23665ea6a854cbcedaa404",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 10432276,
"upload_time": "2024-09-11T22:19:31",
"upload_time_iso_8601": "2024-09-11T22:19:31.193473Z",
"url": "https://files.pythonhosted.org/packages/3c/63/55c937a740517a4981add648ab89988737ad8f1ad0b79f79e1d9be3f4cd2/PyGLM-2.7.2-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ec2b75deb3e7bfb1dc803557e96868b367e665ac4aa7e34de589744de38d95ba",
"md5": "d88e2de00ceafdf448a4db187dd89b8f",
"sha256": "e788c69bbe948cb9db142eeaff62025a9475dd5b57435fd696a71b5259287dc0"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-manylinux_2_17_i686.manylinux2014_i686.whl",
"has_sig": false,
"md5_digest": "d88e2de00ceafdf448a4db187dd89b8f",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 9881930,
"upload_time": "2024-09-11T22:19:34",
"upload_time_iso_8601": "2024-09-11T22:19:34.165988Z",
"url": "https://files.pythonhosted.org/packages/ec/2b/75deb3e7bfb1dc803557e96868b367e665ac4aa7e34de589744de38d95ba/PyGLM-2.7.2-cp39-cp39-manylinux_2_17_i686.manylinux2014_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4ec55222e6ac57b5afd213f483dc3fc458c0cd450a356ba26fd7646ddda64637",
"md5": "1736b28418c48395f66cf5aab4aedf5b",
"sha256": "79f70f52f5ef76a574a68b93c6983d3027739f01802d5694a3e774b7de5bafd9"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"has_sig": false,
"md5_digest": "1736b28418c48395f66cf5aab4aedf5b",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 10909186,
"upload_time": "2024-09-11T22:19:36",
"upload_time_iso_8601": "2024-09-11T22:19:36.339827Z",
"url": "https://files.pythonhosted.org/packages/4e/c5/5222e6ac57b5afd213f483dc3fc458c0cd450a356ba26fd7646ddda64637/PyGLM-2.7.2-cp39-cp39-manylinux_2_17_s390x.manylinux2014_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9aa0a21d70d21481c8c3e65ea2e66d01ab192665b1f247cba99fe4830d331f95",
"md5": "36b3045b495c0fbdcfa15f82e140480e",
"sha256": "a0ea75b21ab29b33f603fb5441adcafd81aa83a31b77b7ca33a65c9755b57706"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "36b3045b495c0fbdcfa15f82e140480e",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 10986348,
"upload_time": "2024-09-11T22:19:38",
"upload_time_iso_8601": "2024-09-11T22:19:38.555660Z",
"url": "https://files.pythonhosted.org/packages/9a/a0/a21d70d21481c8c3e65ea2e66d01ab192665b1f247cba99fe4830d331f95/PyGLM-2.7.2-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0c656a7e2a2348da9928131d7c65a6f93e028ccdc3f3a40d22108d16464ef8ca",
"md5": "05dfb9ad882905ee40d65d8779a70328",
"sha256": "f8ff87bd4c39d7787e1da95c7d3167f146336e87ef6107997daff40a558bf08c"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-manylinux_2_27_aarch64.manylinux_2_28_aarch64.whl",
"has_sig": false,
"md5_digest": "05dfb9ad882905ee40d65d8779a70328",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 10120230,
"upload_time": "2024-09-11T22:19:40",
"upload_time_iso_8601": "2024-09-11T22:19:40.673919Z",
"url": "https://files.pythonhosted.org/packages/0c/65/6a7e2a2348da9928131d7c65a6f93e028ccdc3f3a40d22108d16464ef8ca/PyGLM-2.7.2-cp39-cp39-manylinux_2_27_aarch64.manylinux_2_28_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "083b26a7f31fcf7fce1b5c2d3987ebd80fb270dbac5fb30d413317680e4ad14a",
"md5": "596ceac766da0a84382d10d63ed60488",
"sha256": "de7dbcd4f2a8bb851c845ce947cdd604f1d78f69733410c65a739422be6bae15"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-manylinux_2_27_s390x.manylinux_2_28_s390x.whl",
"has_sig": false,
"md5_digest": "596ceac766da0a84382d10d63ed60488",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 10613109,
"upload_time": "2024-09-11T22:19:43",
"upload_time_iso_8601": "2024-09-11T22:19:43.082608Z",
"url": "https://files.pythonhosted.org/packages/08/3b/26a7f31fcf7fce1b5c2d3987ebd80fb270dbac5fb30d413317680e4ad14a/PyGLM-2.7.2-cp39-cp39-manylinux_2_27_s390x.manylinux_2_28_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2bf884319af7dd6bf2bc4868a7e1d8997cdff033b14203baf5e38a546024d03a",
"md5": "9ee80ac7fe514d2e9a432a6a09539dcc",
"sha256": "4cf4d588a9bccf40977e19b847b81e239581674fecbbb525a23eac9d66ddee30"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-manylinux_2_27_x86_64.manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "9ee80ac7fe514d2e9a432a6a09539dcc",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 10588934,
"upload_time": "2024-09-11T22:19:45",
"upload_time_iso_8601": "2024-09-11T22:19:45.325754Z",
"url": "https://files.pythonhosted.org/packages/2b/f8/84319af7dd6bf2bc4868a7e1d8997cdff033b14203baf5e38a546024d03a/PyGLM-2.7.2-cp39-cp39-manylinux_2_27_x86_64.manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "afe38270cbfa7c1396d227e035711eaa90a3515941378eb9f4beebe224b02c45",
"md5": "5b32d401f82bad67591aee97379bbc98",
"sha256": "4600c596e362b5f4173d2b874a9c1599b9ae6024e69c36288fa9f15957710c35"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-musllinux_1_1_aarch64.whl",
"has_sig": false,
"md5_digest": "5b32d401f82bad67591aee97379bbc98",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 11515717,
"upload_time": "2024-09-11T22:19:47",
"upload_time_iso_8601": "2024-09-11T22:19:47.580776Z",
"url": "https://files.pythonhosted.org/packages/af/e3/8270cbfa7c1396d227e035711eaa90a3515941378eb9f4beebe224b02c45/PyGLM-2.7.2-cp39-cp39-musllinux_1_1_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "c5b166590404bddb5e80cc44a230420f5c1ca215ee52f85c0d3056a433847612",
"md5": "1e29aa20eb651d02bf2ed6b4d4f6dc52",
"sha256": "8394acb82b743fd43ae1283a9815094aa447e9016a1c4b1e3da83152acec39ae"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-musllinux_1_1_i686.whl",
"has_sig": false,
"md5_digest": "1e29aa20eb651d02bf2ed6b4d4f6dc52",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 10753896,
"upload_time": "2024-09-11T22:19:49",
"upload_time_iso_8601": "2024-09-11T22:19:49.877032Z",
"url": "https://files.pythonhosted.org/packages/c5/b1/66590404bddb5e80cc44a230420f5c1ca215ee52f85c0d3056a433847612/PyGLM-2.7.2-cp39-cp39-musllinux_1_1_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1e1261393768b0ab8cd01b5f8937be1621f60a3b831d8ff4b4d0b93c12b36ea0",
"md5": "dda8545777b5e93ef9199d8e890bfb0c",
"sha256": "69e80a9bc3c5aa9ca9550ceef179e9a29b1a8eb9443b229a7f55144b44c70684"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-musllinux_1_1_s390x.whl",
"has_sig": false,
"md5_digest": "dda8545777b5e93ef9199d8e890bfb0c",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 12098245,
"upload_time": "2024-09-11T22:19:52",
"upload_time_iso_8601": "2024-09-11T22:19:52.092149Z",
"url": "https://files.pythonhosted.org/packages/1e/12/61393768b0ab8cd01b5f8937be1621f60a3b831d8ff4b4d0b93c12b36ea0/PyGLM-2.7.2-cp39-cp39-musllinux_1_1_s390x.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7f89e28761531911d80e9e127e14e273cb7d114cdf925ab68401eb00710f2019",
"md5": "4f2ced54be0f383f8633effd6f778b62",
"sha256": "f0c77c0b5751206e870dba5c9a4d1105984b35d0f7de94b37a4d4d450d6079fc"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-musllinux_1_1_x86_64.whl",
"has_sig": false,
"md5_digest": "4f2ced54be0f383f8633effd6f778b62",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 12058188,
"upload_time": "2024-09-11T22:19:54",
"upload_time_iso_8601": "2024-09-11T22:19:54.547955Z",
"url": "https://files.pythonhosted.org/packages/7f/89/e28761531911d80e9e127e14e273cb7d114cdf925ab68401eb00710f2019/PyGLM-2.7.2-cp39-cp39-musllinux_1_1_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "75231e2d971943cb6b0a583dbb288137263fde5af93bb98c185d575418ce2281",
"md5": "cd70495160da2e23a07bb2c64aa49e78",
"sha256": "2b40075c6a020cb64dd3d93fd41886b75f5d0efa099f45383b486a3c1e81d608"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-win32.whl",
"has_sig": false,
"md5_digest": "cd70495160da2e23a07bb2c64aa49e78",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 1249346,
"upload_time": "2024-09-11T22:19:58",
"upload_time_iso_8601": "2024-09-11T22:19:58.120176Z",
"url": "https://files.pythonhosted.org/packages/75/23/1e2d971943cb6b0a583dbb288137263fde5af93bb98c185d575418ce2281/PyGLM-2.7.2-cp39-cp39-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8ca02dfae1d8d3da42181c71f36136db724b991893acb0663bedfa7d62add6e1",
"md5": "b5b3779addfe838529ead64181cc996b",
"sha256": "123d5b00f16e575575667b6cd4211ff8589c03bcba6ed14ac9ec4346a25486f9"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-win_amd64.whl",
"has_sig": false,
"md5_digest": "b5b3779addfe838529ead64181cc996b",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 1580994,
"upload_time": "2024-09-11T22:19:59",
"upload_time_iso_8601": "2024-09-11T22:19:59.802232Z",
"url": "https://files.pythonhosted.org/packages/8c/a0/2dfae1d8d3da42181c71f36136db724b991893acb0663bedfa7d62add6e1/PyGLM-2.7.2-cp39-cp39-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "c2ad5cf0632f34b4ab9204d65b462d75c5a1eaa7acf18e982b25d9fec8a613f8",
"md5": "056aa30cac72e039f99496804a7097f9",
"sha256": "233f67e6fb28ad5ec7e62c5d9340e32245671c5f5f75deb6dffb51d56ceaf717"
},
"downloads": -1,
"filename": "PyGLM-2.7.2-cp39-cp39-win_arm64.whl",
"has_sig": false,
"md5_digest": "056aa30cac72e039f99496804a7097f9",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 1126981,
"upload_time": "2024-09-11T22:20:02",
"upload_time_iso_8601": "2024-09-11T22:20:02.151364Z",
"url": "https://files.pythonhosted.org/packages/c2/ad/5cf0632f34b4ab9204d65b462d75c5a1eaa7acf18e982b25d9fec8a613f8/PyGLM-2.7.2-cp39-cp39-win_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5925c2ff0c5610b8146bbc4e1d85a2c07a2727769aa76b0b56a112f038778f89",
"md5": "08b67d6b5fee49af50ed72351819f7e2",
"sha256": "041e55860e34228f44d03bb448da30e0a6a1dc7e3b36da6a9229751c2baf0475"
},
"downloads": -1,
"filename": "pyglm-2.7.2.tar.gz",
"has_sig": false,
"md5_digest": "08b67d6b5fee49af50ed72351819f7e2",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 4643707,
"upload_time": "2024-09-11T22:20:04",
"upload_time_iso_8601": "2024-09-11T22:20:04.441788Z",
"url": "https://files.pythonhosted.org/packages/59/25/c2ff0c5610b8146bbc4e1d85a2c07a2727769aa76b0b56a112f038778f89/pyglm-2.7.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-09-11 22:20:04",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Zuzu-Typ",
"github_project": "PyGLM",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "pyglm"
}