# PyHaste
[](https://github.com/cocreators-ee/pyhaste/actions/workflows/publish.yaml)
[](https://github.com/astral-sh/ruff)
[](https://github.com/cocreators-ee/pyhaste/blob/master/.pre-commit-config.yaml)
[](https://pypi.org/project/pyhaste/)
[](https://pypi.org/project/pyhaste/)
[](https://opensource.org/licenses/BSD-3-Clause)
Python code speed analyzer.

Monitor the performance of your scripts etc. tools and understand where time is spent.
## Installation
It's a Python library, what do you expect?
```bash
pip install pyhaste
# OR
poetry add pyhaste
```
## Usage
To measure your code, `pyhaste` exports a `measure` context manager, give it a name as an argument. Once you want a report call `report` from `pyhaste`.
```python
import time
from pyhaste import measure, report, measure_wrap
@measure_wrap("prepare_task")
def prepare_task():
time.sleep(0.1)
@measure_wrap("find_items")
def find_items():
return [1, 2, 3]
@measure_wrap("process_item")
def process_item(item):
time.sleep(item * 0.1)
with measure("task"):
prepare_task()
for item in find_items():
process_item(item)
time.sleep(0.01)
report()
```
```
────────────────────────── PyHaste report ───────────────────────────
┏━━━━━━━━━━━━━━━━━━━━┳━━━━━━━━━┳━━━━━━━━┳━━━━━━━━┳━━━━━━━┳━━━━━━━━━━┓
┃ Name ┃ Time ┃ Tot % ┃ Rel % ┃ Calls ┃ Per call ┃
┡━━━━━━━━━━━━━━━━━━━━╇━━━━━━━━━╇━━━━━━━━╇━━━━━━━━╇━━━━━━━╇━━━━━━━━━━┩
│ task │ 0.700 s │ 98.58% │ │ 1 │ 0.700 s │
│ task ›process_item │ 0.600 s │ 84.49% │ 85.70% │ 3 │ 0.200 s │
│ task ›prepare_task │ 0.100 s │ 14.09% │ 14.29% │ 1 │ 0.100 s │
│ Unmeasured │ 0.010 s │ 1.42% │ │ │ │
│ task ›find_items │ 0.000 s │ 0.00% │ 0.00% │ 1 │ 0.000 s │
├────────────────────┼─────────┼────────┼────────┼───────┼──────────┤
│ Total │ 0.710 s │ 100% │ │ │ │
└────────────────────┴─────────┴────────┴────────┴───────┴──────────┘
```
In case you need more complex analysis, you might benefit from `pyhaste.Analyzer` and creating your own instances, e.g. for measuring time spent on separate tasks in a longer running job:
```python
import time
from random import uniform
from pyhaste import Analyzer
for item in [1, 2, 3]:
analyzer = Analyzer()
with analyzer.measure(f"process_item({item})"):
with analyzer.measure("db.find"):
time.sleep(uniform(0.04, 0.06) * item)
with analyzer.measure("calculate"):
with analyzer.measure("guestimate"):
with analyzer.measure("do_math"):
time.sleep(uniform(0.1, 0.15) * item)
with analyzer.measure("save"):
time.sleep(uniform(0.05, 0.075) * item)
time.sleep(uniform(0.01, 0.025) * item)
analyzer.report()
```
```
────────────────────────────────────────── PyHaste report ──────────────────────────────────────────
┏━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━┳━━━━━━━━━┳━━━━━━━━┳━━━━━━━━━┳━━━━━━━┳━━━━━━━━━━┓
┃ Name ┃ Time ┃ Tot % ┃ Rel % ┃ Calls ┃ Per call ┃
┡━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━╇━━━━━━━━━╇━━━━━━━━╇━━━━━━━━━╇━━━━━━━╇━━━━━━━━━━┩
│ process_item(1) │ 0.232 s │ 92.26% │ │ 1 │ 0.232 s │
│ process_item(1) ›calculate │ 0.122 s │ 48.38% │ 52.44% │ 1 │ 0.122 s │
│ process_item(1) ›calculate ›guestimate │ 0.122 s │ 48.38% │ 100.00% │ 1 │ 0.122 s │
│ process_item(1) ›calculate ›guestimate ›do_math │ 0.122 s │ 48.37% │ 99.99% │ 1 │ 0.122 s │
│ process_item(1) ›save │ 0.058 s │ 23.23% │ 25.18% │ 1 │ 0.058 s │
│ process_item(1) ›db.find │ 0.052 s │ 20.64% │ 22.37% │ 1 │ 0.052 s │
│ Unmeasured │ 0.019 s │ 7.74% │ │ │ │
├─────────────────────────────────────────────────┼─────────┼────────┼─────────┼───────┼──────────┤
│ Total │ 0.251 s │ 100% │ │ │ │
└─────────────────────────────────────────────────┴─────────┴────────┴─────────┴───────┴──────────┘
────────────────────────────────────────── PyHaste report ──────────────────────────────────────────
┏━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━┳━━━━━━━━━┳━━━━━━━━┳━━━━━━━━━┳━━━━━━━┳━━━━━━━━━━┓
┃ Name ┃ Time ┃ Tot % ┃ Rel % ┃ Calls ┃ Per call ┃
┡━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━╇━━━━━━━━━╇━━━━━━━━╇━━━━━━━━━╇━━━━━━━╇━━━━━━━━━━┩
│ process_item(2) │ 0.511 s │ 94.66% │ │ 1 │ 0.511 s │
│ process_item(2) ›calculate │ 0.288 s │ 53.38% │ 56.40% │ 1 │ 0.288 s │
│ process_item(2) ›calculate ›guestimate │ 0.288 s │ 53.38% │ 100.00% │ 1 │ 0.288 s │
│ process_item(2) ›calculate ›guestimate ›do_math │ 0.288 s │ 53.38% │ 99.99% │ 1 │ 0.288 s │
│ process_item(2) ›save │ 0.125 s │ 23.10% │ 24.41% │ 1 │ 0.125 s │
│ process_item(2) ›db.find │ 0.098 s │ 18.16% │ 19.19% │ 1 │ 0.098 s │
│ Unmeasured │ 0.029 s │ 5.34% │ │ │ │
├─────────────────────────────────────────────────┼─────────┼────────┼─────────┼───────┼──────────┤
│ Total │ 0.540 s │ 100% │ │ │ │
└─────────────────────────────────────────────────┴─────────┴────────┴─────────┴───────┴──────────┘
────────────────────────────────────────── PyHaste report ──────────────────────────────────────────
┏━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━┳━━━━━━━━━┳━━━━━━━━┳━━━━━━━━━┳━━━━━━━┳━━━━━━━━━━┓
┃ Name ┃ Time ┃ Tot % ┃ Rel % ┃ Calls ┃ Per call ┃
┡━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━╇━━━━━━━━━╇━━━━━━━━╇━━━━━━━━━╇━━━━━━━╇━━━━━━━━━━┩
│ process_item(3) │ 0.749 s │ 93.21% │ │ 1 │ 0.749 s │
│ process_item(3) ›calculate │ 0.368 s │ 45.84% │ 49.18% │ 1 │ 0.368 s │
│ process_item(3) ›calculate ›guestimate │ 0.368 s │ 45.84% │ 100.00% │ 1 │ 0.368 s │
│ process_item(3) ›calculate ›guestimate ›do_math │ 0.368 s │ 45.84% │ 100.00% │ 1 │ 0.368 s │
│ process_item(3) ›save │ 0.217 s │ 27.07% │ 29.04% │ 1 │ 0.217 s │
│ process_item(3) ›db.find │ 0.163 s │ 20.29% │ 21.77% │ 1 │ 0.163 s │
│ Unmeasured │ 0.055 s │ 6.79% │ │ │ │
├─────────────────────────────────────────────────┼─────────┼────────┼─────────┼───────┼──────────┤
│ Total │ 0.803 s │ 100% │ │ │ │
└─────────────────────────────────────────────────┴─────────┴────────┴─────────┴───────┴──────────┘
```
## Development
Issues and PRs are welcome!
Please open an issue first to discuss the idea before sending a PR so that you know if it would be wanted or needs
re-thinking or if you should just make a fork for yourself.
For local development, make sure you install [pre-commit](https://pre-commit.com/#install), then run:
```bash
pre-commit install
poetry install
poetry run ptw .
poetry run python example.py
cd fastapi_example
poetry run python example.py
```
## License
The code is released under the BSD 3-Clause license. Details in the [LICENSE.md](./LICENSE.md) file.
# Financial support
This project has been made possible thanks to [Cocreators](https://cocreators.ee) and [Lietu](https://lietu.net). You
can help us continue our open source work by supporting us
on [Buy me a coffee](https://www.buymeacoffee.com/cocreators).
[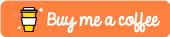](https://www.buymeacoffee.com/cocreators)
Raw data
{
"_id": null,
"home_page": "https://github.com/cocreators-ee/pyhaste/",
"name": "PyHaste",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.11",
"maintainer_email": null,
"keywords": "performance, measuring, benchmark, benchmarking, analyzer",
"author": "Janne Enberg",
"author_email": "janne.enberg@lietu.net",
"download_url": "https://files.pythonhosted.org/packages/e1/1c/3e569f6f60e2869e12e68dd15668701185381e8710a71a46cbded3605d99/pyhaste-1.1.2.tar.gz",
"platform": null,
"description": "# PyHaste\n\n[](https://github.com/cocreators-ee/pyhaste/actions/workflows/publish.yaml)\n[](https://github.com/astral-sh/ruff)\n[](https://github.com/cocreators-ee/pyhaste/blob/master/.pre-commit-config.yaml)\n[](https://pypi.org/project/pyhaste/)\n[](https://pypi.org/project/pyhaste/)\n[](https://opensource.org/licenses/BSD-3-Clause)\n\nPython code speed analyzer.\n\n\n\nMonitor the performance of your scripts etc. tools and understand where time is spent.\n\n## Installation\n\nIt's a Python library, what do you expect?\n\n```bash\npip install pyhaste\n# OR\npoetry add pyhaste\n```\n\n## Usage\n\nTo measure your code, `pyhaste` exports a `measure` context manager, give it a name as an argument. Once you want a report call `report` from `pyhaste`.\n\n```python\nimport time\n\nfrom pyhaste import measure, report, measure_wrap\n\n\n@measure_wrap(\"prepare_task\")\ndef prepare_task():\n time.sleep(0.1)\n\n\n@measure_wrap(\"find_items\")\ndef find_items():\n return [1, 2, 3]\n\n\n@measure_wrap(\"process_item\")\ndef process_item(item):\n time.sleep(item * 0.1)\n\n\nwith measure(\"task\"):\n prepare_task()\n\n for item in find_items():\n process_item(item)\n\ntime.sleep(0.01)\nreport()\n\n```\n\n```\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500 PyHaste report \u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\n\n\u250f\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2513\n\u2503 Name \u2503 Time \u2503 Tot % \u2503 Rel % \u2503 Calls \u2503 Per call \u2503\n\u2521\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2529\n\u2502 task \u2502 0.700 s \u2502 98.58% \u2502 \u2502 1 \u2502 0.700 s \u2502\n\u2502 task \u203aprocess_item \u2502 0.600 s \u2502 84.49% \u2502 85.70% \u2502 3 \u2502 0.200 s \u2502\n\u2502 task \u203aprepare_task \u2502 0.100 s \u2502 14.09% \u2502 14.29% \u2502 1 \u2502 0.100 s \u2502\n\u2502 Unmeasured \u2502 0.010 s \u2502 1.42% \u2502 \u2502 \u2502 \u2502\n\u2502 task \u203afind_items \u2502 0.000 s \u2502 0.00% \u2502 0.00% \u2502 1 \u2502 0.000 s \u2502\n\u251c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2524\n\u2502 Total \u2502 0.710 s \u2502 100% \u2502 \u2502 \u2502 \u2502\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\n```\n\nIn case you need more complex analysis, you might benefit from `pyhaste.Analyzer` and creating your own instances, e.g. for measuring time spent on separate tasks in a longer running job:\n\n```python\nimport time\nfrom random import uniform\nfrom pyhaste import Analyzer\n\nfor item in [1, 2, 3]:\n analyzer = Analyzer()\n with analyzer.measure(f\"process_item({item})\"):\n with analyzer.measure(\"db.find\"):\n time.sleep(uniform(0.04, 0.06) * item)\n with analyzer.measure(\"calculate\"):\n with analyzer.measure(\"guestimate\"):\n with analyzer.measure(\"do_math\"):\n time.sleep(uniform(0.1, 0.15) * item)\n with analyzer.measure(\"save\"):\n time.sleep(uniform(0.05, 0.075) * item)\n time.sleep(uniform(0.01, 0.025) * item)\n analyzer.report()\n```\n\n```\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500 PyHaste report \u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\n\n\u250f\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2513\n\u2503 Name \u2503 Time \u2503 Tot % \u2503 Rel % \u2503 Calls \u2503 Per call \u2503\n\u2521\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2529\n\u2502 process_item(1) \u2502 0.232 s \u2502 92.26% \u2502 \u2502 1 \u2502 0.232 s \u2502\n\u2502 process_item(1) \u203acalculate \u2502 0.122 s \u2502 48.38% \u2502 52.44% \u2502 1 \u2502 0.122 s \u2502\n\u2502 process_item(1) \u203acalculate \u203aguestimate \u2502 0.122 s \u2502 48.38% \u2502 100.00% \u2502 1 \u2502 0.122 s \u2502\n\u2502 process_item(1) \u203acalculate \u203aguestimate \u203ado_math \u2502 0.122 s \u2502 48.37% \u2502 99.99% \u2502 1 \u2502 0.122 s \u2502\n\u2502 process_item(1) \u203asave \u2502 0.058 s \u2502 23.23% \u2502 25.18% \u2502 1 \u2502 0.058 s \u2502\n\u2502 process_item(1) \u203adb.find \u2502 0.052 s \u2502 20.64% \u2502 22.37% \u2502 1 \u2502 0.052 s \u2502\n\u2502 Unmeasured \u2502 0.019 s \u2502 7.74% \u2502 \u2502 \u2502 \u2502\n\u251c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2524\n\u2502 Total \u2502 0.251 s \u2502 100% \u2502 \u2502 \u2502 \u2502\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\n\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500 PyHaste report \u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\n\n\u250f\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2513\n\u2503 Name \u2503 Time \u2503 Tot % \u2503 Rel % \u2503 Calls \u2503 Per call \u2503\n\u2521\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2529\n\u2502 process_item(2) \u2502 0.511 s \u2502 94.66% \u2502 \u2502 1 \u2502 0.511 s \u2502\n\u2502 process_item(2) \u203acalculate \u2502 0.288 s \u2502 53.38% \u2502 56.40% \u2502 1 \u2502 0.288 s \u2502\n\u2502 process_item(2) \u203acalculate \u203aguestimate \u2502 0.288 s \u2502 53.38% \u2502 100.00% \u2502 1 \u2502 0.288 s \u2502\n\u2502 process_item(2) \u203acalculate \u203aguestimate \u203ado_math \u2502 0.288 s \u2502 53.38% \u2502 99.99% \u2502 1 \u2502 0.288 s \u2502\n\u2502 process_item(2) \u203asave \u2502 0.125 s \u2502 23.10% \u2502 24.41% \u2502 1 \u2502 0.125 s \u2502\n\u2502 process_item(2) \u203adb.find \u2502 0.098 s \u2502 18.16% \u2502 19.19% \u2502 1 \u2502 0.098 s \u2502\n\u2502 Unmeasured \u2502 0.029 s \u2502 5.34% \u2502 \u2502 \u2502 \u2502\n\u251c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2524\n\u2502 Total \u2502 0.540 s \u2502 100% \u2502 \u2502 \u2502 \u2502\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\n\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500 PyHaste report \u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\n\n\u250f\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2533\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2513\n\u2503 Name \u2503 Time \u2503 Tot % \u2503 Rel % \u2503 Calls \u2503 Per call \u2503\n\u2521\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2547\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2529\n\u2502 process_item(3) \u2502 0.749 s \u2502 93.21% \u2502 \u2502 1 \u2502 0.749 s \u2502\n\u2502 process_item(3) \u203acalculate \u2502 0.368 s \u2502 45.84% \u2502 49.18% \u2502 1 \u2502 0.368 s \u2502\n\u2502 process_item(3) \u203acalculate \u203aguestimate \u2502 0.368 s \u2502 45.84% \u2502 100.00% \u2502 1 \u2502 0.368 s \u2502\n\u2502 process_item(3) \u203acalculate \u203aguestimate \u203ado_math \u2502 0.368 s \u2502 45.84% \u2502 100.00% \u2502 1 \u2502 0.368 s \u2502\n\u2502 process_item(3) \u203asave \u2502 0.217 s \u2502 27.07% \u2502 29.04% \u2502 1 \u2502 0.217 s \u2502\n\u2502 process_item(3) \u203adb.find \u2502 0.163 s \u2502 20.29% \u2502 21.77% \u2502 1 \u2502 0.163 s \u2502\n\u2502 Unmeasured \u2502 0.055 s \u2502 6.79% \u2502 \u2502 \u2502 \u2502\n\u251c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u253c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2524\n\u2502 Total \u2502 0.803 s \u2502 100% \u2502 \u2502 \u2502 \u2502\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\n```\n\n## Development\n\nIssues and PRs are welcome!\n\nPlease open an issue first to discuss the idea before sending a PR so that you know if it would be wanted or needs\nre-thinking or if you should just make a fork for yourself.\n\nFor local development, make sure you install [pre-commit](https://pre-commit.com/#install), then run:\n\n```bash\npre-commit install\npoetry install\npoetry run ptw .\npoetry run python example.py\n\ncd fastapi_example\npoetry run python example.py\n```\n\n## License\n\nThe code is released under the BSD 3-Clause license. Details in the [LICENSE.md](./LICENSE.md) file.\n\n# Financial support\n\nThis project has been made possible thanks to [Cocreators](https://cocreators.ee) and [Lietu](https://lietu.net). You\ncan help us continue our open source work by supporting us\non [Buy me a coffee](https://www.buymeacoffee.com/cocreators).\n\n[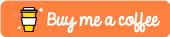](https://www.buymeacoffee.com/cocreators)\n\n",
"bugtrack_url": null,
"license": "BSD-3-Clause",
"summary": "Python code speed analyzer",
"version": "1.1.2",
"project_urls": {
"Documentation": "https://github.com/cocreators-ee/pyhaste/",
"Homepage": "https://github.com/cocreators-ee/pyhaste/",
"Repository": "https://github.com/cocreators-ee/pyhaste/"
},
"split_keywords": [
"performance",
" measuring",
" benchmark",
" benchmarking",
" analyzer"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "d7708e05ac6b9b4b482c055161325facec579c83072540c3e6eefa8c3edd1c29",
"md5": "d7810421e90bd01cdd860dbc0f94c017",
"sha256": "dfe3132d78e99e5c708d7c34b0fe8c56cca0a5e4f20ddb2d4513db665662a141"
},
"downloads": -1,
"filename": "pyhaste-1.1.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "d7810421e90bd01cdd860dbc0f94c017",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.11",
"size": 6118,
"upload_time": "2024-04-11T15:46:12",
"upload_time_iso_8601": "2024-04-11T15:46:12.182464Z",
"url": "https://files.pythonhosted.org/packages/d7/70/8e05ac6b9b4b482c055161325facec579c83072540c3e6eefa8c3edd1c29/pyhaste-1.1.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "e11c3e569f6f60e2869e12e68dd15668701185381e8710a71a46cbded3605d99",
"md5": "1c70a18d685ee146d742e6881aa1d2e4",
"sha256": "bd55f4cac260ad241618e03d3ddf214e7bc6c158d23771b30a913d7fc8437b18"
},
"downloads": -1,
"filename": "pyhaste-1.1.2.tar.gz",
"has_sig": false,
"md5_digest": "1c70a18d685ee146d742e6881aa1d2e4",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.11",
"size": 5524,
"upload_time": "2024-04-11T15:46:13",
"upload_time_iso_8601": "2024-04-11T15:46:13.863202Z",
"url": "https://files.pythonhosted.org/packages/e1/1c/3e569f6f60e2869e12e68dd15668701185381e8710a71a46cbded3605d99/pyhaste-1.1.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-04-11 15:46:13",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "cocreators-ee",
"github_project": "pyhaste",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "pyhaste"
}