PyLMI-SDP
=========
*Symbolic linear matrix inequalities (LMI) and semi-definite programming (SDP) tools for Python*
This package includes a set of classes to represent and manipulate LMIs symbolically using [SymPy](http://sympy.org).
It also includes tools to export LMIs to [CVXOPT](http://abel.ee.ucla.edu/cvxopt/userguide/coneprog.html#semidefinite-programming) SDP input and to the [SDPA](http://sdpa.sourceforge.net/) format.
Depends on [SymPy](http://sympy.org) and [NumPy](http://www.numpy.org/); and optionally on [CVXOPT](http://cvxopt.org) and on [SciPy](http://www.scipy.org) (for sparse matrices).
Single codebase supporting both Python 2.7 and Python 3.x.
PyLMI-SDP is tested for various combinations of Python and sympy. See [here](https://github.com/cdsousa/PyLMI-SDP/actions/workflows/ci.yaml).
PyLMI-SDP is at [GitHub](https://github.com/cdsousa/PyLMI-SDP).
[](https://github.com/cdsousa/PyLMI-SDP/actions/workflows/ci.yaml)
[](https://coveralls.io/r/cdsousa/PyLMI-SDP?branch=master)
LMI Definition
--------------
### Examples
```Python
>>> from sympy import symbols, Matrix
>>> from lmi_sdp import LMI_PD, LMI_NSD
>>> variables = symbols('x y z')
>>> x, y, z = variables
>>> lmi = LMI_PD(Matrix([[x+1, y+2], [y+2, z+x]]))
>>> lmi
Matrix([
[x + 1, y + 2],
[y + 2, x + z]]) > 0
```
```Python
>>> from lmi_sdp import init_lmi_latex_printing
>>> from sympy import latex
>>> init_lmi_latex_printing()
>>> print(latex(lmi))
\left[\begin{matrix}x + 1 & y + 2\\y + 2 & x + z\end{matrix}\right] \succ 0
```
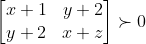
```Python
>>> print(latex(lmi.expanded(variables)))
\left[\begin{matrix}1.0 & 0.0\\0.0 & 1.0\end{matrix}\right] x + \left[\begin{matrix}0.0 & 1.0\\1.0 & 0.0\end{matrix}\right] y + \left[\begin{matrix}0.0 & 0.0\\0.0 & 1.0\end{matrix}\right] z + \left[\begin{matrix}1.0 & 2.0\\2.0 & 0.0\end{matrix}\right] \succ 0
```

```Python
>>> lmi_2 = LMI_NSD( Matrix([[-x, -y], [-y, -z-x]]), Matrix([[1, 2], [2, 0]]))
>>> lmi_2
Matrix([
[-x, -y],
[-y, -x - z]]) <= Matrix([
[1, 2],
[2, 0]])
>>> lmi_2.canonical()
Matrix([
[x + 1, y + 2],
[y + 2, x + z]]) >= 0
```
```Python
>>> print(latex(lmi_2))
\left[\begin{matrix}- x & - y\\- y & - x - z\end{matrix}\right] \preceq \left[\begin{matrix}1 & 2\\2 & 0\end{matrix}\right]
```
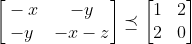
Convertion to CVXOPT SDP
------------------------
### Example
(from CVXOPT [SDP example](http://abel.ee.ucla.edu/cvxopt/userguide/coneprog.html#semidefinite-programming))
```Python
>>> from sympy import symbols, Matrix
>>> from lmi_sdp import LMI_NSD, init_lmi_latex_printing
>>>
>>> init_lmi_latex_printing()
>>>
>>> variables = symbols('x1 x2 x3')
>>> x1, x2, x3 = variables
>>>
>>> min_obj = x1 - x2 + x3
>>>
>>> LMI_1 = LMI_NSD(
... x1*Matrix([[-7, -11], [-11, 3]]) +
... x2*Matrix([[7, -18], [-18, 8]]) +
... x3*Matrix([[-2, -8], [-8, 1]]),
... Matrix([[33, -9], [-9, 26]]))
>>>
>>> LMI_2 = LMI_NSD(
... x1*Matrix([[-21, -11, 0], [-11, 10, 8], [0, 8, 5]]) +
... x2*Matrix([[0, 10, 16], [10, -10, -10], [16, -10, 3]]) +
... x3*Matrix([[-5, 2, -17], [2, -6, 8], [-17, 8, 6]]),
... Matrix([[14, 9, 40], [9, 91, 10], [40, 10, 15]]))
>>>
>>> min_obj
x1 - x2 + x3
```

```Python
>>> LMI_1.expanded(variables)
Matrix([
[ -7.0, -11.0],
[-11.0, 3.0]])*x1 + Matrix([
[ 7.0, -18.0],
[-18.0, 8.0]])*x2 + Matrix([
[-2.0, -8.0],
[-8.0, 1.0]])*x3 <= Matrix([
[33, -9],
[-9, 26]])
```

```Python
>>> LMI_2.expanded(variables)
Matrix([
[-21.0, -11.0, 0.0],
[-11.0, 10.0, 8.0],
[ 0.0, 8.0, 5.0]])*x1 + Matrix([
[ 0.0, 10.0, 16.0],
[10.0, -10.0, -10.0],
[16.0, -10.0, 3.0]])*x2 + Matrix([
[ -5.0, 2.0, -17.0],
[ 2.0, -6.0, 8.0],
[-17.0, 8.0, 6.0]])*x3 <= Matrix([
[14, 9, 40],
[ 9, 91, 10],
[40, 10, 15]])
```

```Python
>>> from cvxopt import solvers
>>> from lmi_sdp import to_cvxopt
>>>
>>> solvers.options['show_progress'] = False
>>>
>>> c, Gs, hs = to_cvxopt(min_obj, [LMI_1, LMI_2], variables)
>>>
>>> sol = solvers.sdp(c, Gs=Gs, hs=hs)
>>> print(sol['x'])
[-3.68e-01]
[ 1.90e+00]
[-8.88e-01]
<BLANKLINE>
```
Export to SDPA Format
---------------------
### Example
```Python
>>> from sympy import symbols, Matrix
>>> from lmi_sdp import LMI_PSD, to_sdpa_sparse
>>>
>>> variables = x1, x2 = symbols('x1 x2')
>>>
>>> min_obj = 10*x1 + 20*x2
>>> lmi_1 = LMI_PSD(
... -Matrix([[1, 0, 0, 0], [0, 2, 0, 0], [0, 0, 3, 0], [0, 0, 0, 4]]) +
... Matrix([[1, 0, 0, 0], [0, 1, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]])*x1 +
... Matrix([[0, 0, 0, 0], [0, 1, 0, 0], [0, 0, 5, 2], [0, 0, 2, 6]])*x2)
>>> lmi_1
Matrix([
[x1 - 1, 0, 0, 0],
[ 0, x1 + x2 - 2, 0, 0],
[ 0, 0, 5*x2 - 3, 2*x2],
[ 0, 0, 2*x2, 6*x2 - 4]]) >= 0
>>>
>>> dat = to_sdpa_sparse(min_obj, lmi_1, variables, comment='test sparse')
>>> print(dat)
"test sparse"
2 = ndim
3 = nblocks
1 1 2 = blockstruct
10.0, 20.0 = objcoeffs
0 1 1 1 1.0
0 2 1 1 2.0
0 3 1 1 3.0
0 3 2 2 4.0
1 1 1 1 1.0
1 2 1 1 1.0
2 2 1 1 1.0
2 3 1 1 5.0
2 3 1 2 2.0
2 3 2 2 6.0
<BLANKLINE>
```
Author
------
[Cristóvão Duarte Sousa](https://github.com/cdsousa)
Install
-------
From PyPi:
pip install PyLMI-SDP
From git source:
git clone https://github.com/cdsousa/PyLMI-SDP.git
cd PyLMI-SDP
python setup.py install
License
-------
Simplified BSD License. See [License File](LICENSE.txt)
Raw data
{
"_id": null,
"home_page": "http://github.com/cdsousa/PyLMI-SDP",
"name": "PyLMI-SDP",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "LMI SDP",
"author": "Cristovao D. Sousa",
"author_email": "crisjss@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/a1/5f/a040a63b55f9856bc5f68ecc947eef73913583f3eb3c5a07f9ae226a8b61/PyLMI-SDP-1.1.1.tar.gz",
"platform": null,
"description": "PyLMI-SDP\n=========\n\n*Symbolic linear matrix inequalities (LMI) and semi-definite programming (SDP) tools for Python*\n\nThis package includes a set of classes to represent and manipulate LMIs symbolically using [SymPy](http://sympy.org).\nIt also includes tools to export LMIs to [CVXOPT](http://abel.ee.ucla.edu/cvxopt/userguide/coneprog.html#semidefinite-programming) SDP input and to the [SDPA](http://sdpa.sourceforge.net/) format.\n\nDepends on [SymPy](http://sympy.org) and [NumPy](http://www.numpy.org/); and optionally on [CVXOPT](http://cvxopt.org) and on [SciPy](http://www.scipy.org) (for sparse matrices).\nSingle codebase supporting both Python 2.7 and Python 3.x.\nPyLMI-SDP is tested for various combinations of Python and sympy. See [here](https://github.com/cdsousa/PyLMI-SDP/actions/workflows/ci.yaml).\n\nPyLMI-SDP is at [GitHub](https://github.com/cdsousa/PyLMI-SDP).\n\n[](https://github.com/cdsousa/PyLMI-SDP/actions/workflows/ci.yaml)\n[](https://coveralls.io/r/cdsousa/PyLMI-SDP?branch=master)\n\nLMI Definition\n--------------\n\n### Examples\n\n```Python\n>>> from sympy import symbols, Matrix\n>>> from lmi_sdp import LMI_PD, LMI_NSD\n>>> variables = symbols('x y z')\n>>> x, y, z = variables\n>>> lmi = LMI_PD(Matrix([[x+1, y+2], [y+2, z+x]]))\n>>> lmi\nMatrix([\n[x + 1, y + 2],\n[y + 2, x + z]]) > 0\n\n```\n\n```Python\n>>> from lmi_sdp import init_lmi_latex_printing\n>>> from sympy import latex\n>>> init_lmi_latex_printing()\n>>> print(latex(lmi))\n\\left[\\begin{matrix}x + 1 & y + 2\\\\y + 2 & x + z\\end{matrix}\\right] \\succ 0\n\n```\n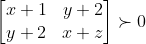\n\n```Python\n>>> print(latex(lmi.expanded(variables)))\n\\left[\\begin{matrix}1.0 & 0.0\\\\0.0 & 1.0\\end{matrix}\\right] x + \\left[\\begin{matrix}0.0 & 1.0\\\\1.0 & 0.0\\end{matrix}\\right] y + \\left[\\begin{matrix}0.0 & 0.0\\\\0.0 & 1.0\\end{matrix}\\right] z + \\left[\\begin{matrix}1.0 & 2.0\\\\2.0 & 0.0\\end{matrix}\\right] \\succ 0\n\n```\n\n\n```Python\n>>> lmi_2 = LMI_NSD( Matrix([[-x, -y], [-y, -z-x]]), Matrix([[1, 2], [2, 0]]))\n>>> lmi_2\nMatrix([\n[-x, -y],\n[-y, -x - z]]) <= Matrix([\n[1, 2],\n[2, 0]])\n>>> lmi_2.canonical()\nMatrix([\n[x + 1, y + 2],\n[y + 2, x + z]]) >= 0\n\n```\n\n```Python\n>>> print(latex(lmi_2))\n\\left[\\begin{matrix}- x & - y\\\\- y & - x - z\\end{matrix}\\right] \\preceq \\left[\\begin{matrix}1 & 2\\\\2 & 0\\end{matrix}\\right]\n\n```\n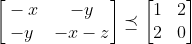\n\nConvertion to CVXOPT SDP\n------------------------\n\n### Example\n\n(from CVXOPT [SDP example](http://abel.ee.ucla.edu/cvxopt/userguide/coneprog.html#semidefinite-programming))\n\n```Python\n>>> from sympy import symbols, Matrix\n>>> from lmi_sdp import LMI_NSD, init_lmi_latex_printing\n>>>\n>>> init_lmi_latex_printing()\n>>>\n>>> variables = symbols('x1 x2 x3')\n>>> x1, x2, x3 = variables\n>>>\n>>> min_obj = x1 - x2 + x3\n>>>\n>>> LMI_1 = LMI_NSD(\n... x1*Matrix([[-7, -11], [-11, 3]]) +\n... x2*Matrix([[7, -18], [-18, 8]]) +\n... x3*Matrix([[-2, -8], [-8, 1]]),\n... Matrix([[33, -9], [-9, 26]]))\n>>>\n>>> LMI_2 = LMI_NSD(\n... x1*Matrix([[-21, -11, 0], [-11, 10, 8], [0, 8, 5]]) +\n... x2*Matrix([[0, 10, 16], [10, -10, -10], [16, -10, 3]]) +\n... x3*Matrix([[-5, 2, -17], [2, -6, 8], [-17, 8, 6]]),\n... Matrix([[14, 9, 40], [9, 91, 10], [40, 10, 15]]))\n>>>\n>>> min_obj\nx1 - x2 + x3\n\n```\n\n\n```Python\n>>> LMI_1.expanded(variables)\nMatrix([\n[ -7.0, -11.0],\n[-11.0, 3.0]])*x1 + Matrix([\n[ 7.0, -18.0],\n[-18.0, 8.0]])*x2 + Matrix([\n[-2.0, -8.0],\n[-8.0, 1.0]])*x3 <= Matrix([\n[33, -9],\n[-9, 26]])\n\n```\n\n\n```Python\n>>> LMI_2.expanded(variables)\nMatrix([\n[-21.0, -11.0, 0.0],\n[-11.0, 10.0, 8.0],\n[ 0.0, 8.0, 5.0]])*x1 + Matrix([\n[ 0.0, 10.0, 16.0],\n[10.0, -10.0, -10.0],\n[16.0, -10.0, 3.0]])*x2 + Matrix([\n[ -5.0, 2.0, -17.0],\n[ 2.0, -6.0, 8.0],\n[-17.0, 8.0, 6.0]])*x3 <= Matrix([\n[14, 9, 40],\n[ 9, 91, 10],\n[40, 10, 15]])\n\n```\n\n\n```Python\n>>> from cvxopt import solvers\n>>> from lmi_sdp import to_cvxopt\n>>>\n>>> solvers.options['show_progress'] = False\n>>>\n>>> c, Gs, hs = to_cvxopt(min_obj, [LMI_1, LMI_2], variables)\n>>>\n>>> sol = solvers.sdp(c, Gs=Gs, hs=hs)\n>>> print(sol['x'])\n[-3.68e-01]\n[ 1.90e+00]\n[-8.88e-01]\n<BLANKLINE>\n\n```\n\nExport to SDPA Format\n---------------------\n\n### Example\n\n```Python\n>>> from sympy import symbols, Matrix\n>>> from lmi_sdp import LMI_PSD, to_sdpa_sparse\n>>>\n>>> variables = x1, x2 = symbols('x1 x2')\n>>>\n>>> min_obj = 10*x1 + 20*x2\n>>> lmi_1 = LMI_PSD(\n... -Matrix([[1, 0, 0, 0], [0, 2, 0, 0], [0, 0, 3, 0], [0, 0, 0, 4]]) +\n... Matrix([[1, 0, 0, 0], [0, 1, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]])*x1 +\n... Matrix([[0, 0, 0, 0], [0, 1, 0, 0], [0, 0, 5, 2], [0, 0, 2, 6]])*x2)\n>>> lmi_1\nMatrix([\n[x1 - 1, 0, 0, 0],\n[ 0, x1 + x2 - 2, 0, 0],\n[ 0, 0, 5*x2 - 3, 2*x2],\n[ 0, 0, 2*x2, 6*x2 - 4]]) >= 0\n>>>\n>>> dat = to_sdpa_sparse(min_obj, lmi_1, variables, comment='test sparse')\n>>> print(dat)\n\"test sparse\"\n2 = ndim\n3 = nblocks\n1 1 2 = blockstruct\n10.0, 20.0 = objcoeffs\n0 1 1 1 1.0\n0 2 1 1 2.0\n0 3 1 1 3.0\n0 3 2 2 4.0\n1 1 1 1 1.0\n1 2 1 1 1.0\n2 2 1 1 1.0\n2 3 1 1 5.0\n2 3 1 2 2.0\n2 3 2 2 6.0\n<BLANKLINE>\n\n```\n\n\nAuthor\n------\n\n[Crist\u00f3v\u00e3o Duarte Sousa](https://github.com/cdsousa)\n\nInstall\n-------\n\nFrom PyPi:\n\n pip install PyLMI-SDP\n\nFrom git source:\n\n git clone https://github.com/cdsousa/PyLMI-SDP.git\n cd PyLMI-SDP\n python setup.py install\n\nLicense\n-------\n\nSimplified BSD License. See [License File](LICENSE.txt)\n",
"bugtrack_url": null,
"license": "BSD",
"summary": "Symbolic linear matrix inequalities (LMI) and semi-definite programming (SDP) tools for Python",
"version": "1.1.1",
"split_keywords": [
"lmi",
"sdp"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "a2ebfcea4c9a4bb71da0e90abef1700b08a2041bfbfd0c8001ee9e2e07bb6ce6",
"md5": "7be62b14e186884b2fb79efb426a082c",
"sha256": "4c2e7fec95bbfe8c94de3fdaa96159d129662312c8be527ba8e1a1ba72c34302"
},
"downloads": -1,
"filename": "PyLMI_SDP-1.1.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "7be62b14e186884b2fb79efb426a082c",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 10369,
"upload_time": "2023-03-20T11:37:19",
"upload_time_iso_8601": "2023-03-20T11:37:19.751693Z",
"url": "https://files.pythonhosted.org/packages/a2/eb/fcea4c9a4bb71da0e90abef1700b08a2041bfbfd0c8001ee9e2e07bb6ce6/PyLMI_SDP-1.1.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a15fa040a63b55f9856bc5f68ecc947eef73913583f3eb3c5a07f9ae226a8b61",
"md5": "694f353eb37793477f07c1ea352b2668",
"sha256": "202821bbf588434919fc4e0b16b4b24f10c7edd229432ae54c40be23077b28b6"
},
"downloads": -1,
"filename": "PyLMI-SDP-1.1.1.tar.gz",
"has_sig": false,
"md5_digest": "694f353eb37793477f07c1ea352b2668",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 9636,
"upload_time": "2023-03-20T11:37:21",
"upload_time_iso_8601": "2023-03-20T11:37:21.923642Z",
"url": "https://files.pythonhosted.org/packages/a1/5f/a040a63b55f9856bc5f68ecc947eef73913583f3eb3c5a07f9ae226a8b61/PyLMI-SDP-1.1.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-03-20 11:37:21",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "cdsousa",
"github_project": "PyLMI-SDP",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"lcname": "pylmi-sdp"
}