Name | Sanzan JSON |
Version |
2.0.5
JSON |
| download |
home_page | |
Summary | Video encryption while maintaining playability. |
upload_time | 2023-01-13 07:48:56 |
maintainer | |
docs_url | None |
author | |
requires_python | |
license | |
keywords |
|
VCS |
|
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# Sanzan
[](https://github.com/kokseen1/Sanzan/actions/workflows/release.yml)
[](https://pypi.python.org/pypi/sanzan/)
Sanzan is a simple encryption library to encrypt and decrypt videos while maintaining playability.
It uses NumPy and OpenCV to perform video frame manipulation, while using FFmpeg and Pydub for audio manipulation. Sanzan can be used either from the command line or in a Python program.
### Encrypted Video
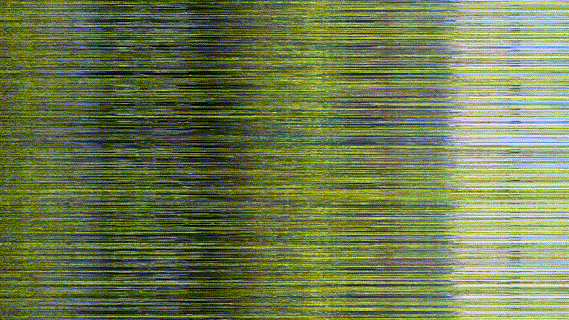
### Decrypted Video:
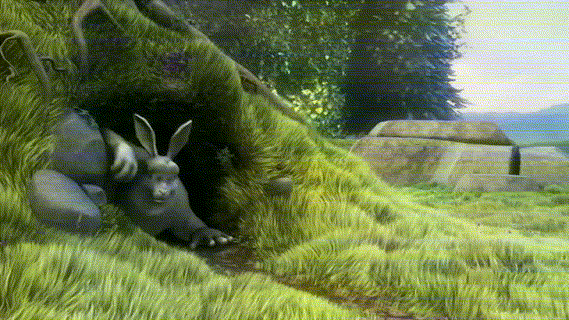
## Installation
### Prerequisite
[FFmpeg](https://www.ffmpeg.org/download.html) must be installed and in `PATH`.
```shell
pip install sanzan
```
## Usage
### Encryption
```shell
sz original.mp4 -k <key> -e -o encrypted.mp4
```
#### On a stream
```shell
sz https://youtu.be/dQw4w9WgXcQ -k <key> -e -o encrypted.mp4
```
### Decryption
```shell
sz encrypted.mp4 -k <key> -d -o decrypted.mp4
```
#### With preview
```shell
sz encrypted.mp4 -k <key> -d -o decrypted.mp4 -p
```
- Frames will be displayed as quickly as they are generated.
- Audio will only start playing after the whole stream has been processed.
### More Usage
Omit the `-k` argument to encrypt using randomly generated keyfiles.
Use the `-m` argument to specify mode: `audio`, `video` or `full` (default).
Use the `-q` flag for quiet mode.
#### Audio options
Use the `-a` argument to specify output audio format: `mp3`, `wav` (default), `flac`, etc.
Use the `-l` flag to use light encryption/decryption.
Use the `-dn` flag to apply denoising to the output audio.
Use the `-pp` flag to disable audio padding.
#### Video options
Use the `-s` argument to specify scramble method: `rows` (default), `cols`, `full`.
Use the `-f` flag to generate a different scramble order every frame.
### Python Usage
Alternatively, Sanzan provides a Python interface to programmatically access its functionality.
#### Encryption
```python
from sanzan import Sanzan
if __name__ == "__main__":
s = Sanzan("original.mp4")
s.set_password("1234")
s.encrypt("encrypted.mp4")
```
#### Decryption
```python
from sanzan import Sanzan
if __name__ == "__main__":
s = Sanzan("encrypted.mp4")
s.set_password("1234")
s.decrypt("decrypted.mp4", preview=True, denoise=True)
```
Raw data
{
"_id": null,
"home_page": "",
"name": "Sanzan",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "",
"author": "",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/a5/b5/3ba4e629ddb2b03ade0443a1ba33f4235a02e60fdc543a977d2ed8331d37/Sanzan-2.0.5.tar.gz",
"platform": null,
"description": "# Sanzan\n\n[](https://github.com/kokseen1/Sanzan/actions/workflows/release.yml)\n[](https://pypi.python.org/pypi/sanzan/)\n\nSanzan is a simple encryption library to encrypt and decrypt videos while maintaining playability.\n\nIt uses NumPy and OpenCV to perform video frame manipulation, while using FFmpeg and Pydub for audio manipulation. Sanzan can be used either from the command line or in a Python program.\n\n### Encrypted Video\n\n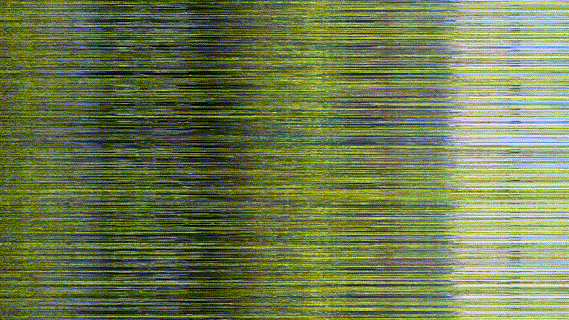\n\n### Decrypted Video:\n\n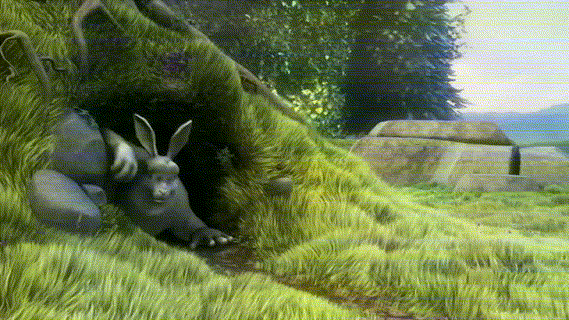\n\n## Installation\n\n### Prerequisite\n\n[FFmpeg](https://www.ffmpeg.org/download.html) must be installed and in `PATH`.\n\n```shell\npip install sanzan\n```\n\n## Usage\n\n### Encryption\n\n```shell\nsz original.mp4 -k <key> -e -o encrypted.mp4 \n```\n\n#### On a stream\n\n```shell\nsz https://youtu.be/dQw4w9WgXcQ -k <key> -e -o encrypted.mp4 \n```\n\n### Decryption\n\n```shell\nsz encrypted.mp4 -k <key> -d -o decrypted.mp4 \n```\n\n#### With preview\n\n```shell\nsz encrypted.mp4 -k <key> -d -o decrypted.mp4 -p\n```\n\n- Frames will be displayed as quickly as they are generated.\n- Audio will only start playing after the whole stream has been processed.\n\n### More Usage\n\nOmit the `-k` argument to encrypt using randomly generated keyfiles.\n\nUse the `-m` argument to specify mode: `audio`, `video` or `full` (default).\n\nUse the `-q` flag for quiet mode.\n\n#### Audio options\n\nUse the `-a` argument to specify output audio format: `mp3`, `wav` (default), `flac`, etc.\n\nUse the `-l` flag to use light encryption/decryption.\n\nUse the `-dn` flag to apply denoising to the output audio.\n\nUse the `-pp` flag to disable audio padding.\n\n#### Video options\n\nUse the `-s` argument to specify scramble method: `rows` (default), `cols`, `full`.\n\nUse the `-f` flag to generate a different scramble order every frame.\n\n### Python Usage\n\nAlternatively, Sanzan provides a Python interface to programmatically access its functionality.\n\n#### Encryption\n\n```python\nfrom sanzan import Sanzan\n\nif __name__ == \"__main__\":\n s = Sanzan(\"original.mp4\")\n s.set_password(\"1234\")\n s.encrypt(\"encrypted.mp4\")\n```\n\n#### Decryption\n\n```python\nfrom sanzan import Sanzan\n\nif __name__ == \"__main__\":\n s = Sanzan(\"encrypted.mp4\")\n s.set_password(\"1234\")\n s.decrypt(\"decrypted.mp4\", preview=True, denoise=True)\n```\n\n\n",
"bugtrack_url": null,
"license": "",
"summary": "Video encryption while maintaining playability.",
"version": "2.0.5",
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "b62747229818c05e4c4b1743eb3b2791ad7a6e4cf8771e57ba162e80549680e1",
"md5": "a988e0772bd82163c93d5a6d42aa56f8",
"sha256": "5431bac9fa46a6d3f151ed28e60f42f27511df3655d8842de2a93e65d7e36474"
},
"downloads": -1,
"filename": "Sanzan-2.0.5-py3-none-any.whl",
"has_sig": false,
"md5_digest": "a988e0772bd82163c93d5a6d42aa56f8",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 8831,
"upload_time": "2023-01-13T07:48:55",
"upload_time_iso_8601": "2023-01-13T07:48:55.233788Z",
"url": "https://files.pythonhosted.org/packages/b6/27/47229818c05e4c4b1743eb3b2791ad7a6e4cf8771e57ba162e80549680e1/Sanzan-2.0.5-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a5b53ba4e629ddb2b03ade0443a1ba33f4235a02e60fdc543a977d2ed8331d37",
"md5": "3a80e10529dfa75870f756cb6953d92a",
"sha256": "c4078c4c6351941a95db9cc409aff8b598b4c8326e60b31de42fdab0f6c8fcd1"
},
"downloads": -1,
"filename": "Sanzan-2.0.5.tar.gz",
"has_sig": false,
"md5_digest": "3a80e10529dfa75870f756cb6953d92a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 7309,
"upload_time": "2023-01-13T07:48:56",
"upload_time_iso_8601": "2023-01-13T07:48:56.413063Z",
"url": "https://files.pythonhosted.org/packages/a5/b5/3ba4e629ddb2b03ade0443a1ba33f4235a02e60fdc543a977d2ed8331d37/Sanzan-2.0.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-01-13 07:48:56",
"github": false,
"gitlab": false,
"bitbucket": false,
"lcname": "sanzan"
}