# SmileValidation v1.2.10
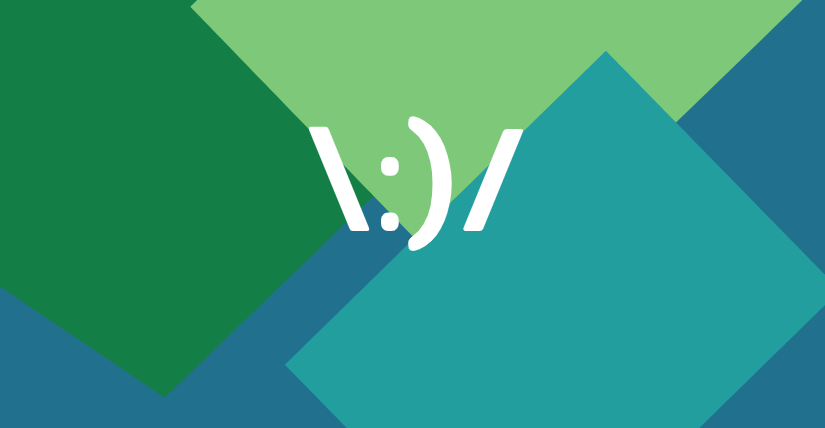
Python3 validation with a different approach.
Validating those form elements is enough to make me feel hurt. Time to bring a new technique type.
What's new v1.2.x:
- Adding RuleSchema into Validation class
- Improving rule of validation method
- Fix bugs
## Validation class
After adding elements to the collection, the core class includes a validation element that validates each element by calling IsValid().
```
from smilevalidation.Validation import Validation
# validition instance
v = Validation()
## integer validation
# add element with
v.addElement(
elementName= 'computer-quantity'
, elementValue= 2
, rule= v.rule.getInteger(
require= True
, maxValue= 5
, minValue= 1
, negative= False
)
)
## start validating
# true if every element is correct
if v.isValid():
Console.output(f'Everything is fine')
else:
Console.output(f'Error: {v.getErrorNumber()}')
```
## Comparison
For reality form validation, it does not just need validation with the value, they need also the comparison such as confirm password form. So does it? It should build for this purpose via addMatchedElement method and addNotMatchedElement. Both will validate the matched value and not matched value.
```
v.addMatchedElement(
elementName1= 'm1'
, elementValue1='hello'
, elementName2='m2'
, elementValue2='hello2'
)
v.addNotMatchedElement(
elementName1= 'm2'
, elementValue1='hello'
, elementName2='m1'
, elementValue2='hello2'
)
```
Then the IsValid() method will execute these too.
Full example:
```
# sample with two elements
from smilevalidation.Validator import Validator
from smilevalidation.Rule import Rule
# validition instance
v = Validation()
# match element value
v.addMatchedElement(
elementName1= 'm1'
, elementValue1='hello'
, elementName2='m2'
, elementValue2='hello2'
)
v.addNotMatchedElement(
elementName1= 'm2'
, elementValue1='hello'
, elementName2='m1'
, elementValue2='hello2'
)
## start validating
# true if every element is correct
if v.isValid():
Console.output(f'Everything is fine')
else:
Console.output(f'Error: {v.getErrorNumber()}')
```
## Rule Reusable
Extending or overriding the existing RuleSchema port
Example:
```
from Validation import Validation, RuleSchema
class Rule2(RuleSchema):
def getQuantityOne(self) -> dict:
"""
:return:
"""
return self.getInteger(
require= True
, maxValue= 5
, minValue= 1
, negative= False
)
# init
val = Validation()
# override the new rule
val.setRule(rule= Rule2())
```
getQualityOne method will present the new rule function
```
# Two elements with Rule2 class
from smilevalidation.Validation import Validation
# validition instance
v = Validation()
v.setRule(rule= Rule2())
## integer validation
# add element with
v.addElement(
elementName= 'computer-quantity'
, elementValue= 2
, rule= self.rule.getQuantityOne()
)
v.addElement(
elementName= 'tv-quantity'
, elementValue= 4
, rule= self.rule.getQuantityOne()
)
## start validating
# true if every element is correct
if v.isValid():
Console.output(f'Everything is fine')
else:
Console.output(f'Error: {v.getErrorNumber()}')
```
Full example
```
# validition instance
v = Validation()
## integer validation
# add element with
v.addElement(
elementName= 'computer-quantity'
, elementValue= 2
, rule= self.rule.getInteger(
require= True
, maxValue= 5
, minValue= 1
, negative= False
)
)
v.addElement(
elementName= 'tv-quantity'
, elementValue= 4
, rule= self.rule.getInteger(
require= True
, maxValue= 5
, minValue= 1
, negative= False
)
)
# match element value
v.addMatchedElement(
elementName1= 'm1'
, elementValue1='hello'
, elementName2='m2'
, elementValue2='hello2'
)
v.addNotMatchedElement(
elementName1= 'm2'
, elementValue1='hello'
, elementName2='m1'
, elementValue2='hello2'
)
## start validating
# true if every element is correct
if v.isValid():
Console.output(f'Everything is fine')
else:
Console.output(f'Error: {v.getErrorNumber()}')
```
It is available on **PyPi** store via https://pypi.org/project/SmileValidation/ \
In order to support my work, please donate to me through <a class="bmc-button" target="_blank" href="https://www.buymeacoffee.com/sitthykun"><img src="https://cdn.buymeacoffee.com/buttons/bmc-new-btn-logo.svg" alt="Buy me a Pizza"><span style="margin-left:5px;font-size:28px !important;">Buy me a Coffee</span></a>
##### My unique slogan is:
a little developer in the big world \o/
Raw data
{
"_id": null,
"home_page": "https://github.com/sitthykun/smilevalidation",
"name": "SmileValidation",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.5",
"maintainer_email": "",
"keywords": "smilevalidation,smilevalidator,validation,validator",
"author": "Sitthykun LY",
"author_email": "ly.sitthykun@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/1e/3c/e620582e1061bc1a2c30bb1228ca0209703d0a4aca2029e39fa06765a04b/SmileValidation-1.2.10.tar.gz",
"platform": "All",
"description": "# SmileValidation v1.2.10\n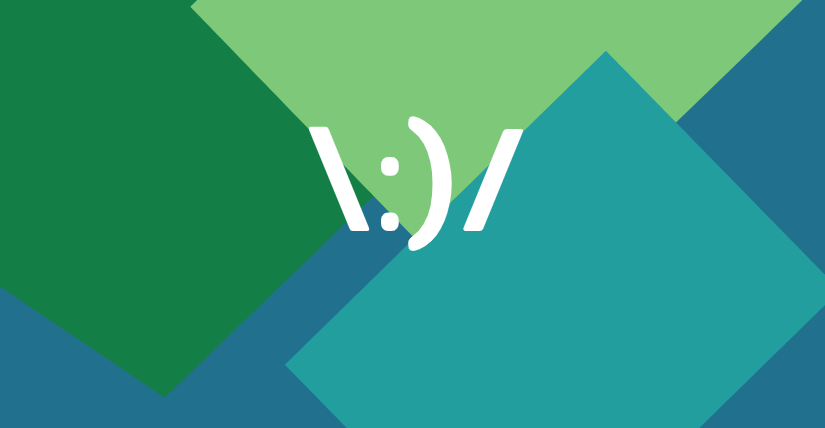\n\nPython3 validation with a different approach.\n\nValidating those form elements is enough to make me feel hurt. Time to bring a new technique type.\n\nWhat's new v1.2.x:\n- Adding RuleSchema into Validation class\n- Improving rule of validation method\n- Fix bugs\n## Validation class\nAfter adding elements to the collection, the core class includes a validation element that validates each element by calling IsValid().\n\n```\nfrom smilevalidation.Validation import Validation\n\n\n# validition instance\nv\t= Validation()\n\n## integer validation\n# add element with \nv.addElement(\n elementName= 'computer-quantity'\n , elementValue= 2\n , rule= v.rule.getInteger(\n\t\t\trequire= True\n\t\t\t, maxValue= 5\n\t\t\t, minValue= 1\n\t\t\t, negative= False\n\t\t)\n)\n\n## start validating\n# true if every element is correct\nif v.isValid():\n Console.output(f'Everything is fine')\n\nelse:\n Console.output(f'Error: {v.getErrorNumber()}')\n\n```\n\n## Comparison\nFor reality form validation, it does not just need validation with the value, they need also the comparison such as confirm password form. So does it? It should build for this purpose via addMatchedElement method and addNotMatchedElement. Both will validate the matched value and not matched value.\n```\nv.addMatchedElement(\n elementName1= 'm1'\n , elementValue1='hello'\n , elementName2='m2'\n , elementValue2='hello2'\n)\n\nv.addNotMatchedElement(\n elementName1= 'm2'\n , elementValue1='hello'\n , elementName2='m1'\n , elementValue2='hello2'\n)\n```\nThen the IsValid() method will execute these too.\n\nFull example:\n\n```\n# sample with two elements\nfrom smilevalidation.Validator import Validator\nfrom smilevalidation.Rule import Rule\n\n\n# validition instance\nv\t= Validation()\n\n# match element value\nv.addMatchedElement(\n elementName1= 'm1'\n , elementValue1='hello'\n , elementName2='m2'\n , elementValue2='hello2'\n)\n\nv.addNotMatchedElement(\n elementName1= 'm2'\n , elementValue1='hello'\n , elementName2='m1'\n , elementValue2='hello2'\n)\n\n## start validating\n# true if every element is correct\nif v.isValid():\n Console.output(f'Everything is fine')\n\nelse:\n Console.output(f'Error: {v.getErrorNumber()}')\n```\n\n## Rule Reusable\nExtending or overriding the existing RuleSchema port\n\nExample:\n```\nfrom Validation import Validation, RuleSchema\n\n\nclass Rule2(RuleSchema):\n\n def getQuantityOne(self) -> dict:\n \"\"\"\n \n :return:\n \"\"\"\n return self.getInteger(\n require= True\n , maxValue= 5\n , minValue= 1\n , negative= False\n )\n # init\n val = Validation()\n # override the new rule\n val.setRule(rule= Rule2())\n``` \ngetQualityOne method will present the new rule function\n\n```\n# Two elements with Rule2 class\nfrom smilevalidation.Validation import Validation\n\n\n# validition instance\nv\t= Validation()\nv.setRule(rule= Rule2())\n\n## integer validation\n# add element with \nv.addElement(\n elementName= 'computer-quantity'\n , elementValue= 2\n , rule= self.rule.getQuantityOne()\n)\n\nv.addElement(\n elementName= 'tv-quantity'\n , elementValue= 4\n , rule= self.rule.getQuantityOne()\n)\n\n## start validating\n# true if every element is correct\nif v.isValid():\n Console.output(f'Everything is fine')\n\nelse:\n Console.output(f'Error: {v.getErrorNumber()}')\n```\n\nFull example\n```\n# validition instance\nv\t= Validation()\n\n## integer validation\n# add element with \nv.addElement(\n elementName= 'computer-quantity'\n , elementValue= 2\n , rule= self.rule.getInteger(\n\t\t\trequire= True\n\t\t\t, maxValue= 5\n\t\t\t, minValue= 1\n\t\t\t, negative= False\n\t\t)\n)\n\nv.addElement(\n elementName= 'tv-quantity'\n , elementValue= 4\n , rule= self.rule.getInteger(\n\t\t\trequire= True\n\t\t\t, maxValue= 5\n\t\t\t, minValue= 1\n\t\t\t, negative= False\n\t\t)\n)\n\n# match element value\nv.addMatchedElement(\n elementName1= 'm1'\n , elementValue1='hello'\n , elementName2='m2'\n , elementValue2='hello2'\n)\n\nv.addNotMatchedElement(\n elementName1= 'm2'\n , elementValue1='hello'\n , elementName2='m1'\n , elementValue2='hello2'\n)\n\n## start validating\n# true if every element is correct\nif v.isValid():\n Console.output(f'Everything is fine')\n\nelse:\n Console.output(f'Error: {v.getErrorNumber()}')\n\n```\n\nIt is available on **PyPi** store via https://pypi.org/project/SmileValidation/ \\\nIn order to support my work, please donate to me through <a class=\"bmc-button\" target=\"_blank\" href=\"https://www.buymeacoffee.com/sitthykun\"><img src=\"https://cdn.buymeacoffee.com/buttons/bmc-new-btn-logo.svg\" alt=\"Buy me a Pizza\"><span style=\"margin-left:5px;font-size:28px !important;\">Buy me a Coffee</span></a>\n\n##### My unique slogan is:\na little developer in the big world \\o/\n",
"bugtrack_url": null,
"license": "MIT License",
"summary": "Python3 Validation in another way",
"version": "1.2.10",
"project_urls": {
"Homepage": "https://github.com/sitthykun/smilevalidation"
},
"split_keywords": [
"smilevalidation",
"smilevalidator",
"validation",
"validator"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "8e55bda7e03b38416cd61831c39d05967afc7645e98fc07b2cdc305c95163198",
"md5": "62294bbd5f226bdee6dabf27a13a33c1",
"sha256": "a81cb0014fff7c0a371cf8c5a9f6df42ef58866114ed009c39d4111b2ff44a86"
},
"downloads": -1,
"filename": "SmileValidation-1.2.10-py3-none-any.whl",
"has_sig": false,
"md5_digest": "62294bbd5f226bdee6dabf27a13a33c1",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.5",
"size": 26700,
"upload_time": "2024-01-04T09:58:36",
"upload_time_iso_8601": "2024-01-04T09:58:36.364601Z",
"url": "https://files.pythonhosted.org/packages/8e/55/bda7e03b38416cd61831c39d05967afc7645e98fc07b2cdc305c95163198/SmileValidation-1.2.10-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1e3ce620582e1061bc1a2c30bb1228ca0209703d0a4aca2029e39fa06765a04b",
"md5": "391b3b3fa1dcc55a0d4ca30a103c069e",
"sha256": "85b903026a095239c82e4454d170c95b512e0800583e4d730d2a52f8bad7cf53"
},
"downloads": -1,
"filename": "SmileValidation-1.2.10.tar.gz",
"has_sig": false,
"md5_digest": "391b3b3fa1dcc55a0d4ca30a103c069e",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.5",
"size": 14880,
"upload_time": "2024-01-04T09:58:38",
"upload_time_iso_8601": "2024-01-04T09:58:38.527243Z",
"url": "https://files.pythonhosted.org/packages/1e/3c/e620582e1061bc1a2c30bb1228ca0209703d0a4aca2029e39fa06765a04b/SmileValidation-1.2.10.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-01-04 09:58:38",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "sitthykun",
"github_project": "smilevalidation",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "smilevalidation"
}