# TrafficLightAI
A python traffic simulation serving as a playground to create traffic light A.I. systems. The traffic simulation uses a cellular automata approach to simulate large traffic grids. The simulation is optimized with Numba.
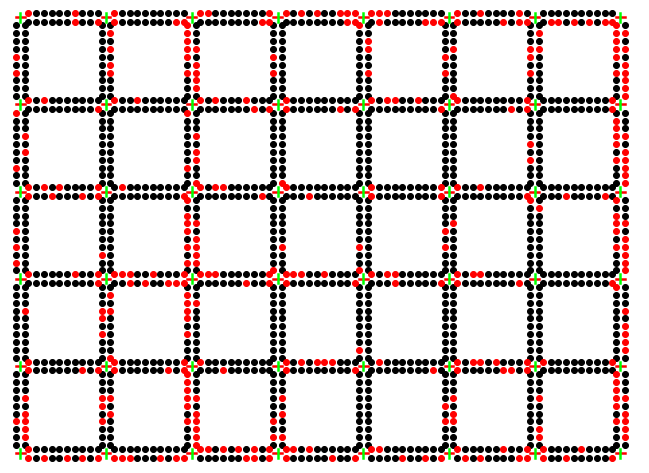
## Installation
```sh
pip install ai-traffic-light-simulator
```
## Example
```py
from traffic_simulation_numba import TrafficSimulation
# OR from traffic_simulation import TrafficSimulation
import random
NORTH_SOUTH_GREEN = 0
EAST_WEST_GREEN = 1
# A basic A.I. which randomly determines light timings
# Inputs: [North waiting, East waiting, South waiting, West Waiting, Previous Light Direction]
def my_ai(inputs):
if inputs[-1] == NORTH_SOUTH_GREEN:
return EAST_WEST_GREEN, random.randint(1,30)
if inputs[-1] == EAST_WEST_GREEN:
return NORTH_SOUTH_GREEN, random.randint(1,30)
# Make traffic simulation object with our naive A.I.
sim = TrafficSimulation(
my_ai,
grid_size_x=8,
grid_size_y=8,
lane_length=10,
max_speed=5,
in_rate=0.2,
initial_density=0.1,
seed=42
)
results = sim.run_simulation(1000) # Runs the simulation for 1000 ticks
print(results)
# Returns { 'cars_stopped': 131680, 'carbon_emissions': 672824 }
# Render a frame of the simulation after 1000 ticks
sim.render_frame("Small.png")
```
Raw data
{
"_id": null,
"home_page": "",
"name": "ai-traffic-light-simulator",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "",
"keywords": "artifical intelligence,traffic,traffic simulator,simulation,cellular automata",
"author": "",
"author_email": "",
"download_url": "",
"platform": null,
"description": "# TrafficLightAI\r\nA python traffic simulation serving as a playground to create traffic light A.I. systems. The traffic simulation uses a cellular automata approach to simulate large traffic grids. The simulation is optimized with Numba.\r\n\r\n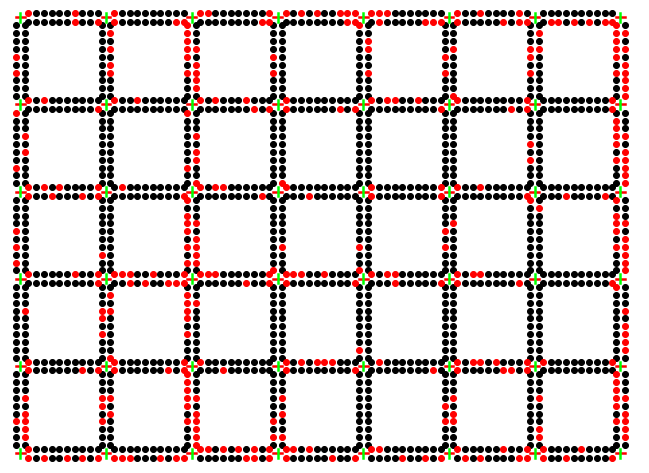\r\n\r\n\r\n## Installation\r\n\r\n```sh\r\npip install ai-traffic-light-simulator\r\n```\r\n\r\n## Example\r\n\r\n```py\r\nfrom traffic_simulation_numba import TrafficSimulation\r\n# OR from traffic_simulation import TrafficSimulation\r\nimport random\r\n\r\nNORTH_SOUTH_GREEN = 0\r\nEAST_WEST_GREEN = 1\r\n\r\n# A basic A.I. which randomly determines light timings\r\n# Inputs: [North waiting, East waiting, South waiting, West Waiting, Previous Light Direction]\r\ndef my_ai(inputs):\r\n if inputs[-1] == NORTH_SOUTH_GREEN:\r\n return EAST_WEST_GREEN, random.randint(1,30)\r\n if inputs[-1] == EAST_WEST_GREEN:\r\n return NORTH_SOUTH_GREEN, random.randint(1,30)\r\n\r\n# Make traffic simulation object with our naive A.I.\r\nsim = TrafficSimulation(\r\n my_ai, \r\n grid_size_x=8,\r\n grid_size_y=8, \r\n lane_length=10,\r\n max_speed=5, \r\n in_rate=0.2, \r\n initial_density=0.1, \r\n seed=42\r\n)\r\n\r\nresults = sim.run_simulation(1000) # Runs the simulation for 1000 ticks\r\nprint(results)\r\n# Returns { 'cars_stopped': 131680, 'carbon_emissions': 672824 }\r\n\r\n# Render a frame of the simulation after 1000 ticks\r\nsim.render_frame(\"Small.png\")\r\n```\r\n",
"bugtrack_url": null,
"license": "",
"summary": "Traffic simulation for traffic light A.I. training",
"version": "1.0.1",
"project_urls": {
"Homepage": "https://github.com/bart1259/TrafficLightAI"
},
"split_keywords": [
"artifical intelligence",
"traffic",
"traffic simulator",
"simulation",
"cellular automata"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "df36aafe120a50172d6d7b17d511bc990843bfe82b655a67fb7156d01ead8052",
"md5": "03371ad5ff10ed852a23476bce211090",
"sha256": "26bff7b19ee61f6194a22fc9894f4c5d2ddc7f6eb5bef27947e5d3bc41c2f6e7"
},
"downloads": -1,
"filename": "ai_traffic_light_simulator-1.0.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "03371ad5ff10ed852a23476bce211090",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 14228,
"upload_time": "2023-05-09T20:35:28",
"upload_time_iso_8601": "2023-05-09T20:35:28.647463Z",
"url": "https://files.pythonhosted.org/packages/df/36/aafe120a50172d6d7b17d511bc990843bfe82b655a67fb7156d01ead8052/ai_traffic_light_simulator-1.0.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-05-09 20:35:28",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "bart1259",
"github_project": "TrafficLightAI",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "ai-traffic-light-simulator"
}